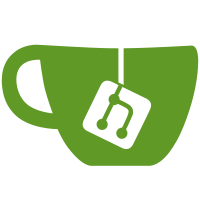
Correct dispose logic for transition destination ITEMs Correct cursor position after Transition Insert Correct logic to parse Transition Link Token Correct Save Config to dispose of ITEM Removed debug vlnStackTrace
780 lines
26 KiB
C#
780 lines
26 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.IO;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using XYPlots;
|
|
using DevComponents.DotNetBar;
|
|
using System.Text.RegularExpressions;
|
|
using Volian.Base.Library;
|
|
//using VG;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayRO : UserControl
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#region Properties
|
|
private DisplayTabControl _TabControl;
|
|
|
|
public DisplayTabControl TabControl
|
|
{
|
|
get { return _TabControl; }
|
|
set { _TabControl = value; }
|
|
}
|
|
private ROFstInfo _CurROFST = null;
|
|
private ROFstInfo _MyROFST;
|
|
public ROFstInfo MyROFST
|
|
{
|
|
get { return _MyROFST; }
|
|
set
|
|
{
|
|
if (!Visible) return; // don't reset anything if the form is invisible.
|
|
_MyROFST = value; // define the tree nodes based on this rofst
|
|
LoadTree();
|
|
}
|
|
}
|
|
private RoUsageInfo _CurROLink;
|
|
public RoUsageInfo CurROLink
|
|
{
|
|
get { return _CurROLink; }
|
|
set
|
|
{
|
|
if (!Visible) return; // don't reset anything if the form is invisible.
|
|
if (value != null) // modify - set the controls to the current ro
|
|
{
|
|
if (_CurROLink == value) return;
|
|
_CurROLink = value;
|
|
_SavCurROLink = _CurROLink;
|
|
UpdateROTree();
|
|
}
|
|
else // insert - clear out controls
|
|
{
|
|
_CurROLink = value;
|
|
tbROValue.Text = null;
|
|
lbROId.Text = "";
|
|
tvROFST.SelectedNode = null;
|
|
btnSaveRO.Enabled = btnCancelRO.Enabled = false;
|
|
}
|
|
}
|
|
}
|
|
private RoUsageInfo _SavCurROLink;
|
|
private StepRTB _MyRTB;
|
|
public StepRTB MyRTB
|
|
{
|
|
get { return _MyRTB; }
|
|
set
|
|
{
|
|
if (!Visible) return;
|
|
if (_MyRTB != null)
|
|
{
|
|
_MyRTB.LinkChanged -= new StepRTBLinkEvent(_MyRTB_LinkChanged);
|
|
_MyRTB.SelectionChanged -= new EventHandler(_MyRTB_SelectionChanged);
|
|
}
|
|
if (value == null) return;
|
|
_MyRTB = value;
|
|
_MyRTB.LinkChanged += new StepRTBLinkEvent(_MyRTB_LinkChanged);
|
|
_MyRTB.SelectionChanged+=new EventHandler(_MyRTB_SelectionChanged);
|
|
if (_MyRTB.MyLinkText == null)
|
|
{
|
|
CurROLink = null;
|
|
_SavCurROLink = null;
|
|
}
|
|
}
|
|
}
|
|
|
|
void _MyRTB_SelectionChanged(object sender, EventArgs e)
|
|
{
|
|
lbFound.SelectionMode = SelectionMode.None;
|
|
lbFound.DataSource = null;
|
|
//Spin through ROs looking for the selected text
|
|
string lookFor = _MyRTB.SelectedText;
|
|
List<ROFSTLookup.roChild> children = _MyROFST.ROFSTLookup.GetRosByValue(lookFor);
|
|
if (children != null)
|
|
{
|
|
lbFound.Visible = true;
|
|
lbFound.DataSource = children.ToArray();
|
|
lbFound.SelectionMode = SelectionMode.One;
|
|
lbFound.SelectedIndex = -1;
|
|
}
|
|
else
|
|
lbFound.Visible = false;
|
|
}
|
|
|
|
void _MyRTB_LinkChanged(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (_MyRTB.MyLinkText == null)
|
|
CurROLink = null;
|
|
else
|
|
{
|
|
CurROLink = args.MyLinkText.MyRoUsageInfo;
|
|
}
|
|
}
|
|
|
|
private DocVersionInfo _Mydvi;
|
|
|
|
public DocVersionInfo Mydvi
|
|
{
|
|
get { return _Mydvi; }
|
|
set { _Mydvi = value; }
|
|
}
|
|
|
|
|
|
private ProgressBarItem _ProgressBar;
|
|
|
|
public ProgressBarItem ProgressBar
|
|
{
|
|
get { return _ProgressBar; }
|
|
set { _ProgressBar = value; }
|
|
}
|
|
|
|
//public int ProgBarMax
|
|
//{
|
|
// get { return _ProgressBar.Maximum; }
|
|
// set { _ProgressBar.Maximum = value; }
|
|
//}
|
|
|
|
//public int ProgBarValue
|
|
//{
|
|
// get { return _ProgressBar.Value; }
|
|
// set { _ProgressBar.Value = value; }
|
|
//}
|
|
|
|
//public string ProgBarText
|
|
//{
|
|
// get { return _ProgressBar.Text; }
|
|
// set
|
|
// {
|
|
// _ProgressBar.TextVisible = true;
|
|
// _ProgressBar.Text = value;
|
|
// }
|
|
//}
|
|
|
|
#endregion
|
|
#region Constructors
|
|
public DisplayRO()
|
|
{
|
|
InitializeComponent();
|
|
_ProgressBar = null;
|
|
panelRoValue.BackColor = Color.Cornsilk;
|
|
panelValue.BackColor = Color.Cornsilk;
|
|
}
|
|
#endregion
|
|
#region Events
|
|
ROFSTLookup.rochild selectedChld;
|
|
private void tvROFST_AfterSelect(object sender, TreeViewEventArgs e)
|
|
{
|
|
tbROValue.Text = null;
|
|
lbROId.Text = "";
|
|
btnCancelRO.Enabled = false;
|
|
btnSaveRO.Enabled = false;
|
|
btnPreviewRO.Enabled = false;
|
|
|
|
if (e.Node.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild chld = (ROFSTLookup.rochild)e.Node.Tag;
|
|
selectedChld = chld;
|
|
if (chld.value != null)
|
|
{
|
|
RoUsageInfo SavROLink = null;
|
|
if (_SavCurROLink != null)
|
|
SavROLink = _SavCurROLink; ;
|
|
lbROId.Text = chld.appid;
|
|
btnSaveRO.Enabled = ((_SavCurROLink == null) || !(chld.roid.Substring(0, 12).ToLower().Equals(SavROLink.ROID.Substring(0, 12).ToLower())));
|
|
btnCancelRO.Enabled = ((_SavCurROLink != null) && chld.roid.Substring(0, 12).ToLower() != SavROLink.ROID.Substring(0, 12).ToLower());
|
|
switch (chld.type)
|
|
{
|
|
case 1: // standard (regular) text RO type
|
|
tbROValue.Text = chld.value;
|
|
btnPreviewRO.Enabled = false;
|
|
break;
|
|
case 2: // Table RO type
|
|
tbROValue.Text = "(Table)";
|
|
btnPreviewRO.Enabled = true;
|
|
break;
|
|
case 4: // X/Y Plot RO type
|
|
tbROValue.Text = "(Graph)";
|
|
btnPreviewRO.Enabled = true;
|
|
break;
|
|
case 8: // Intergrated Graphics RO type
|
|
tbROValue.Text = "(Image)";
|
|
btnPreviewRO.Enabled = true;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
//if (e.Node.Tag is ROFST.rochild)
|
|
//{
|
|
// ROFST.rochild chld = (ROFST.rochild)e.Node.Tag;
|
|
// if (chld.type == 1 && chld.value != null)
|
|
// {
|
|
// string SavROLink = "";
|
|
// if (_SavCurROLink != null)
|
|
// SavROLink = _SavCurROLink.Substring(_SavCurROLink.IndexOf(' ') + 1, 12);
|
|
// tbROValue.Text = chld.value;
|
|
// btnSaveRO.Enabled = ((_SavCurROLink == null) || !(chld.roid.Equals(SavROLink)));
|
|
// btnCancelRO.Enabled = ((_SavCurROLink != null) && !(chld.roid.Equals(SavROLink)));
|
|
// }
|
|
// //else
|
|
// //{
|
|
// // tbROValue.Text = null;
|
|
// // btnCancelRO.Enabled = btnSaveRO.Enabled = false;
|
|
// //}
|
|
//}
|
|
////else
|
|
////{
|
|
//// tbROValue.Text = null;
|
|
//// btnCancelRO.Enabled = btnSaveRO.Enabled = false;
|
|
////}
|
|
|
|
}
|
|
|
|
private void tvROFST_BeforeExpand(object sender, TreeViewCancelEventArgs e)
|
|
{
|
|
LoadChildren(e.Node);
|
|
}
|
|
private void LoadChildren(TreeNode tn)
|
|
{
|
|
object tag = tn.Tag;
|
|
if (tn.FirstNode != null && tn.FirstNode.Text != "VLN_DUMMY_FOR_TREE") return; // already loaded.
|
|
if (tn.FirstNode != null && tn.FirstNode.Text == "VLN_DUMMY_FOR_TREE") tn.FirstNode.Remove();
|
|
ROFSTLookup.rochild[] chld = null;
|
|
|
|
if (tn.Tag is ROFSTLookup.rodbi)
|
|
{
|
|
ROFSTLookup.rodbi db = (ROFSTLookup.rodbi)tn.Tag;
|
|
chld = db.children;
|
|
}
|
|
else if (tn.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild ch = (ROFSTLookup.rochild)tn.Tag;
|
|
chld = ch.children;
|
|
}
|
|
else
|
|
{
|
|
Console.WriteLine("error - no type");
|
|
return;
|
|
}
|
|
// if children, add dummy node
|
|
if (chld != null && chld.Length > 0)
|
|
{
|
|
ProgressBar_Initialize(chld.Length, tn.Text);
|
|
for (int i = 0; i < chld.Length; i++)
|
|
{
|
|
ProgressBar_SetValue(i);
|
|
TreeNode tmp = null;
|
|
// if this is a group, i.e. type 0, add a dummy node
|
|
if (chld[i].type == 0 && chld[i].children == null)
|
|
//skip it.
|
|
// TODO: KBR how to handle this?
|
|
//Console.WriteLine("ro junk");
|
|
continue;
|
|
else if (/*chld[i].type == 1 && */ chld[i].value == null)
|
|
{
|
|
tmp = new TreeNode(chld[i].title);
|
|
tmp.Tag = chld[i];
|
|
tn.Nodes.Add(tmp);
|
|
TreeNode sub = new TreeNode("VLN_DUMMY_FOR_TREE");
|
|
tmp.Nodes.Add(sub);
|
|
}
|
|
//else if (chld[i].type == 2 && chld[i].value == null) // table
|
|
//{
|
|
// if ((_MyRTB.MyItemInfo.MyContent.Type % 10000) == (int)E_FromType.Table)
|
|
// {
|
|
// tmp = new TreeNode(chld[i].title);
|
|
// tmp.Tag = chld[i];
|
|
// tn.Nodes.Add(tmp);
|
|
// TreeNode sub = new TreeNode("VLN_DUMMY_FOR_TREE");
|
|
// tmp.Nodes.Add(sub);
|
|
// }
|
|
//}
|
|
else
|
|
{
|
|
tmp = new TreeNode(chld[i].title);
|
|
tmp.Tag = chld[i];
|
|
int index = FindIndex(tn.Nodes, tmp.Text);
|
|
tn.Nodes.Insert(index,tmp);
|
|
}
|
|
}
|
|
}
|
|
ProgressBar_Clear();
|
|
}
|
|
private int FindIndex(TreeNodeCollection nodes, string value)
|
|
{
|
|
int index = 0;
|
|
foreach (TreeNode node in nodes)
|
|
{
|
|
if (GreaterValue(node.Text, value)) return index;
|
|
index++;
|
|
}
|
|
return index;
|
|
}
|
|
private static Regex _RegExGetNumber = new Regex(@"^ *[+-]?[.,0-9]+(E[+-]?[0-9]+)?");
|
|
private bool GreaterValue(string value1, string value2)
|
|
{
|
|
Match match1 = _RegExGetNumber.Match(value1);
|
|
Match match2 = _RegExGetNumber.Match(value2);
|
|
if (match1.Success && match2.Success) // Compare the numeric value
|
|
{
|
|
double dbl1 = double.Parse(match1.ToString());
|
|
double dbl2 = double.Parse(match2.ToString());
|
|
return dbl1 > dbl2;
|
|
}
|
|
return String.Compare(value1, value2, true) > 0;
|
|
}
|
|
public void RefreshRoTree()
|
|
{
|
|
string roid = null;
|
|
if (tvROFST.SelectedNode != null)
|
|
{
|
|
if (tvROFST.SelectedNode.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild chld = (ROFSTLookup.rochild)tvROFST.SelectedNode.Tag;
|
|
roid = chld.roid;
|
|
}
|
|
}
|
|
_CurROFST = null;
|
|
LoadTree();
|
|
if (_SelectedRoidBeforeRoEditor != null)
|
|
{
|
|
ExpandTree(_SelectedRoidBeforeRoEditor);
|
|
_SelectedRoidBeforeRoEditor = null;
|
|
}
|
|
}
|
|
private void LoadTree()
|
|
{
|
|
if (_MyROFST == null) return;
|
|
if (_MyROFST == _CurROFST) return;
|
|
tvROFST.Nodes.Clear();
|
|
_CurROFST = _MyROFST;
|
|
for (int i = 0; i < _MyROFST.ROFSTLookup.myHdr.myDbs.Length; i++)
|
|
{
|
|
TreeNode tn = new TreeNode(_MyROFST.ROFSTLookup.myHdr.myDbs[i].dbiTitle);
|
|
tn.Tag = _MyROFST.ROFSTLookup.myHdr.myDbs[i];
|
|
tvROFST.Nodes.Add(tn);
|
|
AddDummyGroup(_MyROFST.ROFSTLookup.myHdr.myDbs[i], tn);
|
|
}
|
|
}
|
|
private void AddDummyGroup(ROFSTLookup.rodbi rodbi, TreeNode tn)
|
|
{
|
|
if (rodbi.children != null && rodbi.children.Length > 0)
|
|
{
|
|
TreeNode tmp = new TreeNode("VLN_DUMMY_FOR_TREE");
|
|
tn.Nodes.Add(tmp);
|
|
}
|
|
}
|
|
private void UpdateROTree()
|
|
{
|
|
// walk down from root of tree, expanding values in the string
|
|
// that represents the ro link.
|
|
//string tmpstr = _CurROLink;
|
|
//int sp = tmpstr.IndexOf(" "); // because parse of ro info is wrong!!
|
|
//int rousageid = System.Convert.ToInt32(tmpstr.Substring(0, sp));
|
|
ExpandTree(_CurROLink.ROID);// tmpstr.Substring(sp + 1, tmpstr.Length - sp - 1);
|
|
}
|
|
|
|
private void ExpandTree(string roid)
|
|
{
|
|
string db = roid.Substring(0, 4);
|
|
bool multValSel = false;
|
|
if (roid.Length == 16)
|
|
multValSel = true;
|
|
|
|
ROFSTLookup.rochild rochld = MyROFST.ROFSTLookup.GetRoChild(roid.Substring(0, 12).ToUpper());
|
|
// use this to walk up tree until database - this is used to expand tree.
|
|
List<int> path = new List<int>();
|
|
int myid = rochld.ID;
|
|
while (myid > 0)
|
|
{
|
|
path.Insert(0, myid);
|
|
myid = rochld.ParentID;
|
|
rochld = MyROFST.ROFSTLookup.GetRoChildFromID(myid);
|
|
if (rochld.ID == -1) myid = -1;
|
|
}
|
|
TreeNode tnExpand = null;
|
|
int titm = System.Convert.ToInt32(db);
|
|
// find database first
|
|
foreach (TreeNode tn in tvROFST.Nodes)
|
|
{
|
|
ROFSTLookup.rodbi thisdb = (ROFSTLookup.rodbi)tn.Tag;
|
|
if (thisdb.dbiID == titm)
|
|
{
|
|
LoadChildren(tn);
|
|
tnExpand = tn;
|
|
break;
|
|
}
|
|
}
|
|
if (tnExpand == null) return; // something went wrong?
|
|
// use the path id list to load/find the treeview's nodes.
|
|
foreach (int citm in path)
|
|
{
|
|
if (citm != System.Convert.ToInt32(db))
|
|
{
|
|
LoadChildren(tnExpand);
|
|
tnExpand.Expand();
|
|
foreach (TreeNode tn in tnExpand.Nodes)
|
|
{
|
|
ROFSTLookup.rochild chld = (ROFSTLookup.rochild)tn.Tag;
|
|
if (chld.ID == citm)
|
|
{
|
|
tnExpand = tn;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
//if (tnExpand != null) tvROFST.SelectedNode = tnExpand;
|
|
|
|
if (tnExpand != null)
|
|
{
|
|
// If a multiple return value, try to select the proper node
|
|
if (multValSel)
|
|
{
|
|
LoadChildren(tnExpand);
|
|
tnExpand.Expand();
|
|
foreach (TreeNode tn in tnExpand.Nodes)
|
|
{
|
|
ROFSTLookup.rochild chld = (ROFSTLookup.rochild)tn.Tag;
|
|
if (chld.roid == roid)
|
|
{
|
|
tnExpand = tn;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
tvROFST.SelectedNode = tnExpand;
|
|
}
|
|
}
|
|
private void btnSaveRO_Click(object sender, EventArgs e)
|
|
{
|
|
if (tbROValue.Text == null || tbROValue.Text == "")
|
|
{
|
|
MessageBox.Show("Must select an RO Value from the tree.");
|
|
return;
|
|
}
|
|
Object obj = tvROFST.SelectedNode.Tag;
|
|
if (obj is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild roch = (ROFSTLookup.rochild)obj;
|
|
DisplayTabItem dti = _TabControl.SelectedDisplayTabItem; //.OpenItem(_ItemInfo); // open the corresponding procedure text
|
|
if (dti.MyDSOTabPanel != null) // A Word Document tab is the active tab
|
|
{
|
|
ROFSTLookup.rodbi[] dbs = MyROFST.ROFSTLookup.GetRODatabaseList();
|
|
ROFSTLookup.rodbi db = dbs[int.Parse(roch.roid.Substring(0, 4))-1];
|
|
string accPrefix = db.dbiAP.Replace(_Mydvi.DocVersionConfig.RODefaults_graphicsprefix,"IG");
|
|
accPrefix = accPrefix.Replace(_Mydvi.DocVersionConfig.RODefaults_setpointprefix, "SP");
|
|
// string accPrefix = (roch.type == 8) ? _Mydvi.DocVersionConfig.RODefaults_graphicsprefix : _Mydvi.DocVersionConfig.RODefaults_setpointprefix;
|
|
string AccPageID = string.Format("<{0}-{1}>", accPrefix, roch.appid);// makes <SP1-A.1> for example
|
|
Console.WriteLine(AccPageID);
|
|
//string AccPageID = string.Format("{0} <{1}-{2}>", ConvertSymbolsAndStuff(selectedChld.value), accPrefix, roch.appid); // value and accesory ID
|
|
//string AccPageID = string.Format("{0} {1}", ConvertSymbolsAndStuff(selectedChld.value), string.Format(@"#Link:ReferencedObject:<NewID> {0} {1}", roch.roid, _MyROFST.MyRODb.RODbID)); // value and link reference
|
|
//if (MessageBox.Show(AccPageID,"Place on Windows Clipboard?",MessageBoxButtons.YesNo) == DialogResult.Yes)
|
|
// Clipboard.SetText(AccPageID);
|
|
|
|
// Insert the RO text at the current cursor position in the word document
|
|
// NOTE: assuming any type of RO can be put in an Accessory (MSWord) Document
|
|
if (dti.MyDSOTabPanel != null)
|
|
dti.MyDSOTabPanel.InsertText(AccPageID);
|
|
}
|
|
else if (_MyRTB != null) // a Procedure Steps section tab is active
|
|
{
|
|
_MyRTB.inRoAdd = true;
|
|
if (CheckROSelection(roch)) // check for RO type is valid for this type of step/substep
|
|
{
|
|
//int ss = _MyRTB.SelectionStart;
|
|
// the roid may be 12 or 16 chars long, with the last 4 set if there is unit specific
|
|
// menuing. Pad to 12 to store in the rousage table.
|
|
string padroid = (roch.roid.Length <= 12) ? roch.roid + "0000" : roch.roid;
|
|
string linktxt = string.Format(@"#Link:ReferencedObject:<NewID> {0} {1}", padroid, _MyROFST.MyRODb.RODbID);
|
|
// Resolve symbols and scientific notation in the RO return value
|
|
string valtxt = ConvertSymbolsAndStuff(selectedChld.value);
|
|
int ss = _MyRTB.SelectionStart; // Remember where the link is being added
|
|
_MyRTB.InsertRO(valtxt, linktxt); // Insert the LINK
|
|
_MyRTB.SaveText(); // Save the text with the LINK - This also moves the cursor to the end of the text
|
|
// By selecting a starting position within a link, StepRTB (HandleSelectionChange) will select the link
|
|
_MyRTB.Select(ss + 7 + valtxt.Length , 0);// Select the link, Try 7 for "<Start]" plus the length of the value
|
|
//Console.WriteLine("'{0}'",_MyRTB.Text.Substring(_MyRTB.SelectionStart,_MyRTB.SelectionLength));
|
|
string linkText = _MyRTB.Text.Substring(_MyRTB.SelectionStart, _MyRTB.SelectionLength);
|
|
if (_MyLog.IsInfoEnabled && (linkText.Contains("NewID") || linkText.Contains("CROUSGID")))
|
|
_MyLog.InfoFormat("ItemID {0}, LinkText '{1}'", _MyRTB.MyItemInfo.ItemID, linkText);
|
|
_MyRTB.Select(_MyRTB.SelectionStart + _MyRTB.SelectionLength, 0);// Move cursor to end of LINK
|
|
_MyRTB.Focus();
|
|
}
|
|
_MyRTB.inRoAdd = false;
|
|
btnSaveRO.Enabled = btnCancelRO.Enabled = btnPreviewRO.Enabled = false;
|
|
_SavCurROLink = null;
|
|
CurROLink = null;
|
|
}
|
|
//else // we're in an Word attachment
|
|
//{
|
|
// string accPrefix = (roch.type == 8) ? _Mydvi.DocVersionConfig.RODefaults_graphicsprefix : _Mydvi.DocVersionConfig.RODefaults_setpointprefix;
|
|
// string AccPageID = string.Format("<{0}-{1}>", accPrefix, roch.appid);
|
|
// //TODO: CAN WE AUTOMATICALLY PLACE RO ONTO WORD ATTACHEMNT?
|
|
// //if (MessageBox.Show(AccPageID,"Place on Windows Clipboard?",MessageBoxButtons.YesNo) == DialogResult.Yes)
|
|
// // Clipboard.SetText(AccPageID);
|
|
// if (dti.MyDSOTabPanel != null)
|
|
// dti.MyDSOTabPanel.InsertText(AccPageID);
|
|
//}
|
|
}
|
|
}
|
|
|
|
private bool CheckROSelection(ROFSTLookup.rochild selectedRO)
|
|
{
|
|
bool goodToGo = true;
|
|
bool replacingRO = (_SavCurROLink != null);
|
|
string insrpl = (replacingRO) ? "Cannot Replace" : "Cannot Insert";
|
|
string errormsg = "";
|
|
switch (selectedRO.type)
|
|
{
|
|
case 1: // regular text RO
|
|
if (_MyRTB.MyItemInfo.IsFigure)
|
|
{
|
|
errormsg = (replacingRO) ? "a Figure with a non-figure." : "a text RO in a Figure type.";
|
|
goodToGo = false;
|
|
}
|
|
break;
|
|
case 2: // table RO
|
|
if (_MyRTB.MyItemInfo.IsFigure)
|
|
{
|
|
errormsg = (replacingRO) ? "a Figure with a non-figure." : "a table into a Figure type.";
|
|
goodToGo = false;
|
|
}
|
|
else if (!_MyRTB.MyItemInfo.IsTable)
|
|
{
|
|
errormsg = (replacingRO) ? "a non-table RO with a Table RO." : "a table into a non-table type.";
|
|
//TODO: Prompt user to insert a new Table substep type and place this RO into it
|
|
goodToGo = false;
|
|
}
|
|
break;
|
|
case 4: // X/Y Plot RO type
|
|
if (!_MyRTB.MyItemInfo.IsAccPages)
|
|
{
|
|
errormsg = (replacingRO) ? "a non-X/Y Plot RO with an X/Y Plot RO." : "an X/Y Plot RO in an non-Accessory Page type.";
|
|
//TODO: Prompt user to insert a new substep type that handles x/y Plots and place this RO into it
|
|
goodToGo = false;
|
|
}
|
|
break;
|
|
case 8: // figure (intergrated graphics)
|
|
if (!_MyRTB.MyItemInfo.IsFigure && !_MyRTB.MyItemInfo.IsAccPages)
|
|
{
|
|
errormsg = (replacingRO) ? "a graphics RO with a non-graphcis RO." : "a Graphics RO in an non-Figure or a non-Accessory Page type.";
|
|
//TODO: Prompt user to insert a new substep type that handles x/y Plots and place this RO into it
|
|
goodToGo = false;
|
|
}
|
|
break;
|
|
}
|
|
if (!goodToGo)
|
|
MessageBox.Show(string.Format("{0} {1}", insrpl, errormsg), (replacingRO)?"Invalid RO Replacement":"Invalid RO Insert");
|
|
return goodToGo;
|
|
}
|
|
|
|
private void btnCancelRO_Click(object sender, EventArgs e)
|
|
{
|
|
_CurROLink = _SavCurROLink;
|
|
btnCancelRO.Enabled = false;
|
|
UpdateROTree();
|
|
}
|
|
|
|
private void btnPreviewRO_Click(object sender, EventArgs e)
|
|
{
|
|
if (selectedChld.type == 8) // integrated graphic
|
|
{
|
|
string fname = selectedChld.value.Substring(0, selectedChld.value.IndexOf('\n'));
|
|
int thedot = fname.LastIndexOf('.');
|
|
if (thedot == -1 || (thedot != (fname.Length - 4)))
|
|
fname += string.Format(".{0}", MyROFST.MyRODb.RODbConfig.GetDefaultGraphicExtension());
|
|
ROImageInfo tmp = ROImageInfo.GetByROFstID_FileName(MyROFST.ROFstID, fname);
|
|
|
|
if (tmp !=null)
|
|
{
|
|
PreviewROImage pvROImg = new PreviewROImage(tmp.Content, selectedChld.title);
|
|
pvROImg.ShowDialog();
|
|
}
|
|
else
|
|
MessageBox.Show("Cannot Find Image Data");
|
|
}
|
|
else if (selectedChld.type == 2) // table
|
|
{
|
|
PreviewMultiLineRO pmlROTable = new PreviewMultiLineRO(selectedChld.value, selectedChld.title);
|
|
pmlROTable.ShowDialog();
|
|
}
|
|
else if (selectedChld.type == 4) // x/y plot
|
|
{
|
|
/*
|
|
//PreviewMultiLineRO pmlROTable = new PreviewMultiLineRO(selectedChld.value, selectedChld.title);
|
|
//pmlROTable.ShowDialog(); // This will show the graph commands
|
|
this.Cursor = Cursors.WaitCursor;
|
|
// TemporaryFolder is magical. It will automatically (behind the scenes) build a path to a temporary
|
|
// folder on the user's machine. It does this only the first time it's reference (GET), after that
|
|
// it uses what it has.
|
|
string pdfname = TemporaryFolder; //@"G:\debugdat\testplot\";
|
|
pdfname += "\\" + selectedChld.appid + ".pdf";
|
|
//if (File.Exists(pdfname))
|
|
// File.Delete(pdfname);
|
|
XYPlot ROGraph = new XYPlot(selectedChld.value);
|
|
VGOut_C1PDF pdf = VGOut_C1PDF.Create();
|
|
ROGraph.Process(pdf);
|
|
pdf.Save(pdfname);
|
|
this.Cursor = Cursors.Default;
|
|
|
|
// Run an internet browser window
|
|
WebBrowser wb = new WebBrowser(pdfname);
|
|
wb.TitleText = selectedChld.title;
|
|
wb.ShowDialog();// .Show();
|
|
|
|
// Run the default PDF reader
|
|
//System.Diagnostics.Process ActRdr = System.Diagnostics.Process.Start(pdfname);
|
|
//ActRdr.WaitForExit();
|
|
|
|
// Wait for the PDF viewing process to close then delete the temporary file
|
|
// wait for a one minute max
|
|
if (!WaitToDeleteFile(pdfname,1))
|
|
MessageBox.Show(string.Format("The PDF viewer was holding this file open: \n\n{0}",pdfname),"Cannot Delete Temporary File");
|
|
*/
|
|
frmXYPlot plot = new frmXYPlot(selectedChld.appid + " - " + selectedChld.title, selectedChld.value);
|
|
plot.Show();
|
|
}
|
|
}
|
|
private void ProgressBar_Initialize(int max, string desc)
|
|
{
|
|
if (_ProgressBar != null)
|
|
{
|
|
_ProgressBar.Maximum = max;
|
|
_ProgressBar.Text = desc;
|
|
_ProgressBar.TextVisible = true;
|
|
}
|
|
}
|
|
|
|
private void ProgressBar_SetValue(int curval)
|
|
{
|
|
if (_ProgressBar != null)
|
|
{
|
|
_ProgressBar.Value = curval;
|
|
}
|
|
}
|
|
|
|
private void ProgressBar_Clear()
|
|
{
|
|
if (_ProgressBar != null)
|
|
{
|
|
_ProgressBar.Text = "";
|
|
_ProgressBar.Maximum = 0;
|
|
_ProgressBar.Value = 0;
|
|
_ProgressBar.TextVisible = false;
|
|
}
|
|
}
|
|
|
|
#region Utils
|
|
private string ConvertSymbolsAndStuff(string instr)
|
|
{
|
|
string outstr = instr;
|
|
|
|
outstr = outstr.Replace("`", @"\'b0"); // convert backquote to degree - left over from DOS days.
|
|
outstr = outstr.Replace("\xa0", @"\u160?"); // hardspace
|
|
outstr = outstr.Replace("\xb0", @"\'b0"); // degree
|
|
outstr = outstr.Replace("\x7f", @"\f1\u916?\f0"); // delta
|
|
outstr = outstr.Replace("\x2265", @"\f1\u8805?\f0"); // greater than or equal
|
|
outstr = outstr.Replace("\x2264", @"\f1\u8804?\f0"); // less than or equal
|
|
outstr = outstr.Replace("\xB1", @"\'b1"); // plus minus
|
|
outstr = outstr.Replace("\x3A3", @"\f1\u931?\f0"); // sigma
|
|
outstr = outstr.Replace("\x3C4", @"\f1\u947?\f0"); // gamma
|
|
outstr = outstr.Replace("\xBD", @"\'bd"); // half
|
|
outstr = outstr.Replace("\x25A0", @"\f1\u9604?\f0"); // accum 2584
|
|
outstr = outstr.Replace("\x7", @"\f1\u9679?\f0"); // bullet 25CF
|
|
outstr = outstr.Replace("\x2248", @"\f1\u8776?\f0"); // approx eq
|
|
outstr = outstr.Replace("\x2261", @"\f1\u8773?\f0"); // similar eq 2245
|
|
outstr = outstr.Replace("\xF7", @"\'f7"); // division
|
|
outstr = outstr.Replace("\x221A", @"\f1\u8730?\f0"); // square root
|
|
outstr = outstr.Replace("\x393", @"\f1\u961?\f0"); // rho 3C1
|
|
outstr = outstr.Replace("\x3C0", @"\f1\u960?\f0"); // pi
|
|
outstr = outstr.Replace("\xb5", @"\f1\u956?\f0"); // micro 3BC (try e6, if not work try 109)
|
|
outstr = outstr.Replace("\x3B4", @"\f1\u948?\f0"); // lower case delta
|
|
outstr = outstr.Replace("\x3C3", @"\f1\u963?\f0"); // lower case sigma
|
|
outstr = outstr.Replace("\xBC", @"\'bc"); // quarter
|
|
outstr = outstr.Replace("\x3C6", @"\'d8"); // dist zero, D8
|
|
outstr = outstr.Replace("\xC9", @"\f1\u274?\f0"); // energy, 112
|
|
outstr = outstr.Replace("\xEC", @"\'ec"); // grave
|
|
outstr = outstr.Replace("\x2502", @"\f1\u9474?\f0"); // bar
|
|
outstr = outstr.Replace("\x3B5", @"\f1\u949?\f0"); // epsilon
|
|
outstr = outstr.Replace("\x398", @"\f1\u952?\f0"); // theta, 3B8
|
|
outstr = outstr.Replace("\x221E", @"\f1\u8857?\f0"); // dot in oval, 2299
|
|
outstr = outstr.Replace("\xBF", @"\f1\u964?\f0"); // tau, 3C4
|
|
outstr = outstr.Replace("\x2310", @"\f1\u9830?\f0"); // diamond, 2666
|
|
outstr = outstr.Replace("\x2192", @"\f1\u8594?\f0");
|
|
outstr = outstr.Replace("\x2190", @"\f1\u8592?\f0");
|
|
outstr = outstr.Replace("\x2191", @"\f1\u8593?\f0");
|
|
outstr = outstr.Replace("\x2193", @"\f1\u8595?\f0");
|
|
outstr = outstr.Replace("\x2207", @"\f1\u8711?\f0");
|
|
if (MyRTB.MyItemInfo.ActiveFormat.PlantFormat.FormatData.SectData.ConvertCaretToDelta)
|
|
outstr = outstr.Replace("^", @"\f1\u916?\f0");
|
|
outstr = ROFSTLookup.ConvertFortranFormatToScienctificNotation(outstr);
|
|
outstr = outstr.Replace("\r\n", @"\par ");
|
|
return outstr;
|
|
}
|
|
|
|
|
|
/// <summary>
|
|
/// Keep trying to delete the given file until successful OR until MaxMinutes has elasped
|
|
/// </summary>
|
|
/// <param name="fname">File to delete (including path)</param>
|
|
/// <param name="MaxMinutes">Maxium Minutes to keep trying</param>
|
|
/// <returns></returns>
|
|
private static bool WaitToDeleteFile(string fname, int MaxMinutes)
|
|
{
|
|
bool deletedfile = false;
|
|
DateTime dt_start = DateTime.Now;
|
|
DateTime dt_loop = dt_start;
|
|
int mins_start = dt_start.Minute;
|
|
int mins_loop = dt_loop.Minute;
|
|
while (!deletedfile && ((mins_loop - mins_start) < MaxMinutes))
|
|
{
|
|
try
|
|
{
|
|
dt_loop = DateTime.Now;
|
|
File.Delete(fname);
|
|
deletedfile = true;
|
|
}
|
|
catch
|
|
{
|
|
continue; // The process is still holding file, try again.
|
|
}
|
|
}
|
|
return deletedfile;
|
|
}
|
|
|
|
#endregion // utils
|
|
|
|
private string _SelectedRoidBeforeRoEditor = null;
|
|
private void lbROId_DoubleClick(object sender, EventArgs e)
|
|
{
|
|
if (tvROFST.SelectedNode == null) return;
|
|
string roapp = Environment.GetEnvironmentVariable("roapp");
|
|
Object obj = tvROFST.SelectedNode.Tag;
|
|
if (obj is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild roch = (ROFSTLookup.rochild)obj;
|
|
_SelectedRoidBeforeRoEditor = roch.roid;
|
|
string args = "\"" + _MyROFST.MyRODb.FolderPath + "\" " + roch.roid.ToLower();
|
|
System.Diagnostics.Process.Start(roapp, args);
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
private void lbFound_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
ROFSTLookup.roChild child = lbFound.SelectedValue as ROFSTLookup.roChild;
|
|
if (child != null)
|
|
{
|
|
ExpandTree(child.MyChild.roid);
|
|
}
|
|
}
|
|
}
|
|
}
|