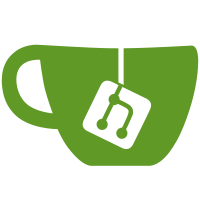
Added administrative tools of Identifying Disconnected Items and NonEditable Items Removed administrative tools CSLA methods since they were moved to AdminToolsExt. Added new module to handle administrative tools CSLA methods Added methods for administrative tools of Identifying Disconnected Items and NonEditable Items
160 lines
4.5 KiB
C#
160 lines
4.5 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using Csla.Validation;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public class ExecuteStoredProcedureRowsAffected : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#region Factory Methods
|
|
private string _StoredProcedure;
|
|
public string StoredProcedure
|
|
{
|
|
get { return _StoredProcedure; }
|
|
set { _StoredProcedure = value; }
|
|
}
|
|
private int _AffectedRows;
|
|
public int AffectedRows
|
|
{
|
|
get { return _AffectedRows; }
|
|
set { _AffectedRows = value; }
|
|
}
|
|
public static int Execute(string storedProcedure)
|
|
{
|
|
ExecuteStoredProcedureRowsAffected cmd = new ExecuteStoredProcedureRowsAffected();
|
|
cmd.StoredProcedure = storedProcedure;
|
|
DataPortal.Execute<ExecuteStoredProcedureRowsAffected>(cmd);
|
|
return cmd.AffectedRows;
|
|
}
|
|
#endregion
|
|
#region Server-Side code
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand(StoredProcedure, cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
AffectedRows = cmd.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ExecuteStoredProcedureRowsAffected Error", ex);
|
|
throw new ApplicationException("Failure on ExecuteStoredProcedureRowsAffected", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
public class ExecuteStoredProcedureRowCount : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#region Factory Methods
|
|
private string _StoredProcedure;
|
|
public string StoredProcedure
|
|
{
|
|
get { return _StoredProcedure; }
|
|
set { _StoredProcedure = value; }
|
|
}
|
|
private int _RowCount;
|
|
public int RowCount
|
|
{
|
|
get { return _RowCount; }
|
|
set { _RowCount = value; }
|
|
}
|
|
public static int Execute(string storedProcedure)
|
|
{
|
|
ExecuteStoredProcedureRowCount cmd = new ExecuteStoredProcedureRowCount();
|
|
cmd.StoredProcedure = storedProcedure;
|
|
DataPortal.Execute<ExecuteStoredProcedureRowCount>(cmd);
|
|
return cmd.RowCount;
|
|
}
|
|
#endregion
|
|
#region Server-Side code
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand(StoredProcedure, cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
SqlDataReader dr = cmd.ExecuteReader();
|
|
while (dr.Read())
|
|
{
|
|
RowCount = dr.GetInt32(0);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ExecuteStoredProcedureRowCount Error", ex);
|
|
throw new ApplicationException("Failure on ExecuteStoredProcedureRowCount", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
public class ExecuteStoredProcedureItemInfoList : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#region Factory Methods
|
|
private string _StoredProcedure;
|
|
public string StoredProcedure
|
|
{
|
|
get { return _StoredProcedure; }
|
|
set { _StoredProcedure = value; }
|
|
}
|
|
private List<ItemInfo> _ItemInfoList = new List<ItemInfo>();
|
|
public List<ItemInfo> ItemInfoList
|
|
{
|
|
get { return _ItemInfoList; }
|
|
set { _ItemInfoList = value; }
|
|
}
|
|
public static List<ItemInfo> Execute(string storedProcedure)
|
|
{
|
|
ExecuteStoredProcedureItemInfoList cmd = new ExecuteStoredProcedureItemInfoList();
|
|
cmd.StoredProcedure = storedProcedure;
|
|
DataPortal.Execute<ExecuteStoredProcedureItemInfoList>(cmd);
|
|
return cmd.ItemInfoList;
|
|
}
|
|
#endregion
|
|
#region Server-Side code
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand(StoredProcedure, cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
using (SafeDataReader dr = new SafeDataReader(cmd.ExecuteReader()))
|
|
{
|
|
while (dr.Read()) ItemInfoList.Add(new ItemInfo(dr));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ExecuteStoredProcedureItemInfoList Error", ex);
|
|
throw new ApplicationException("Failure on ExecuteStoredProcedureItemInfoList", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
} |