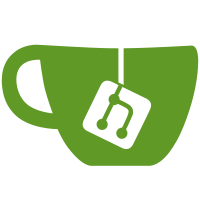
Cleanup memory after transition processing Adjusted width of Error Count Added memory usage to output Added Stored Procedure GetJustFormat Reduce Memory Use Removed extra using statement
561 lines
20 KiB
C#
561 lines
20 KiB
C#
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Text.RegularExpressions;
|
|
using Volian.Controls.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
private Item AddStep(OleDbConnection cn, string StepType, string Textm, string Recid, string stpseq, string structtype, Item FromItem, DateTime dts, string userid, bool conv_caret, string pth, DocVersion docver, FormatInfo fmt,Item parentItem, int frType, string seqRoTran)
|
|
{
|
|
//if (ProcNumber + "|" + stpseq == "STN FP-202|Q2S2")
|
|
// Console.WriteLine("here");
|
|
Content content = null;
|
|
Item item = null;
|
|
|
|
frmMain.UpdateLabels(0, 0, 1);
|
|
ConfigInfo ci = new ConfigInfo(null);
|
|
ci.AddItem("History", "RecID", Recid);
|
|
string stptext = null;
|
|
if (userid == null || userid == "") userid = "Migration";
|
|
int tok = -1;
|
|
//char[] chrarr = { '\x1', '\x2', '\x3', '\x5' };
|
|
char[] chrarr = { '\x1', '\x2', '\x3' };
|
|
try
|
|
{
|
|
// the textm field has step text, but also may have other text stored
|
|
// with it with a 'token' as a separator (see below).
|
|
tok = Textm.IndexOfAny(chrarr);
|
|
|
|
// This will find a continuous action flag without finding a hanging indent
|
|
if (tok == -1 && Textm[Textm.Length - 1] == '\x5')
|
|
tok = Textm.Length - 1;
|
|
|
|
if (tok < 0)
|
|
stptext = TextConvert.ConvertText(Textm, conv_caret);
|
|
else
|
|
stptext = TextConvert.ConvertText(Textm.Substring(0, tok), conv_caret);
|
|
//string stpTextOrg = stptext;
|
|
|
|
string seqcvt = stpseq;
|
|
|
|
string newstptyp = null;
|
|
bool ManualPagebreak = false;
|
|
char cbittst = StepType.PadRight(2)[0];
|
|
byte cbittstb = (byte) StepType.PadRight(2)[0];
|
|
|
|
//Console.WriteLine(string.Format("AddStep() StepType {0} cbitst = {1} OR'd 0x80 = {2}",StepType,Convert.ToInt16(cbittst),(cbittst & 0x80)));
|
|
if (cbittst != ' ' && (cbittst & 0xFF80) > 1)//(cbittst & 0x80) > 1)
|
|
{
|
|
ManualPagebreak = true;
|
|
ci.AddItem("Step", "ManualPagebreak", "True");
|
|
// conversion from 16bit characters to 32bit strings handles the higher bits differently.
|
|
// Thus we cannot do normal 'or'ing/'and'/ing of 0x7f & 0x80 to just get the step
|
|
// type without the pagebreak bit. The following two cases 'hardcode' the steptype
|
|
// based on that problem.
|
|
if ((Convert.ToInt16(cbittst)) == 9508) //page break on HIGH5 step type (40)
|
|
newstptyp = "40";
|
|
else if ((Convert.ToInt16(cbittst)) == 9618) //page break on LOSS OF AC type
|
|
newstptyp = "17";
|
|
else
|
|
newstptyp = StepType.Substring(1, 1);
|
|
}
|
|
// Need the content record for the RO & transitions.
|
|
//content = Content.MakeContent(null, stptext, 20000 + int.Parse(newstptyp!=null?newstptyp:StepType),null, ManualPagebreak?ci.ToString():null, dts, userid);
|
|
// 20000 flags step type item & 1 adjusts for 'base' in format files.
|
|
int contenttype = (structtype == "R") ? 20040 : 20001 + int.Parse(newstptyp != null ? newstptyp : StepType);
|
|
content = Content.New(null, stptext, contenttype, null, ManualPagebreak ? ci.ToString() : null, dts, userid);
|
|
content.MyZContent.OldStepSequence = ProcNumber + "|" + stpseq;
|
|
|
|
// Before we save it, handle RO & Transitions tokens.
|
|
int tokrt = Textm.IndexOf('\x15');
|
|
bool txtdirty = false;
|
|
bool isROTable = false;
|
|
int rotype = 0;
|
|
if (tokrt > -1)
|
|
{
|
|
txtdirty = true;
|
|
stptext = MigrateRos(cn, stptext, seqRoTran, content, docver, conv_caret, ref rotype);
|
|
stptext = stptext.TrimEnd(" ".ToCharArray());
|
|
// if this is a table RO, then save a flag in the config
|
|
isROTable = (rotype == 2 || rotype == 3);
|
|
}
|
|
tokrt = Textm.IndexOf('\x3a6');
|
|
if (tokrt > -1)
|
|
{
|
|
txtdirty = true;
|
|
stptext = MigrateRos(cn, stptext, seqRoTran, content, docver, conv_caret, ref rotype);
|
|
stptext = stptext.TrimEnd(" ".ToCharArray());
|
|
}
|
|
|
|
// 16-bit code has the following two defines.
|
|
// #define TransitionMarker 0xC2
|
|
// #define ReferenceMarker 0xCB
|
|
// these two characters get converted (to unicode) from ado.net
|
|
// use the unicode chars.
|
|
char[] chrrotrn = { '\x252C', '\x2566' };
|
|
tokrt = Textm.IndexOfAny(chrrotrn);
|
|
_TransitionMigrationErrors = new List<string>();
|
|
if (tokrt > -1)
|
|
{
|
|
txtdirty = true;
|
|
stptext = MigrateTrans(cn, stptext, seqRoTran, content, pth);
|
|
stptext = stptext.TrimEnd(" ".ToCharArray());
|
|
}
|
|
//string fixStpText = FixStepText(stptext,content.Type);
|
|
string fixStpText = "";
|
|
if (IsATable(content.Type))
|
|
{
|
|
//if (ProcNumber == "EOP-ES-1.2")
|
|
// Console.WriteLine("here");
|
|
bool hasBorder = !WithoutBorder(content.Type);
|
|
fixStpText = ConvertTableToGrid(stptext, content, fmt, isROTable, hasBorder ? GridLinePattern.Single : GridLinePattern.None );
|
|
int? typ = ConvertTableType(content, fmt);
|
|
if (typ != null) content.Type = typ;
|
|
else _ContentMigrationErrors.Add(content.ContentID, null);
|
|
}
|
|
else
|
|
fixStpText = FixStepText(stptext);
|
|
if (fixStpText != stptext)
|
|
{
|
|
txtdirty = true;
|
|
stptext = fixStpText;
|
|
}
|
|
if (txtdirty)
|
|
{
|
|
content.Text = stptext;
|
|
//content.Save();
|
|
}
|
|
if (!content.IsSavable) ErrorRpt.ErrorReport(content);
|
|
List<string> migrationerrors = null;
|
|
if (_ContentMigrationErrors.ContainsKey(content.ContentID))
|
|
migrationerrors = _ContentMigrationErrors[content.ContentID];
|
|
content = content.Save();
|
|
// check if already created thru new during transition migration...
|
|
if (dicTrans_ItemIds.ContainsKey(ProcNumber + "|" + seqRoTran))
|
|
{
|
|
item = dicTrans_ItemIds[ProcNumber + "|" + seqRoTran];
|
|
item.MyPrevious = FromItem;
|
|
item.MyContent = content;
|
|
item.DTS = dts;
|
|
item.UserID = userid;
|
|
if (!item.IsSavable) ErrorRpt.ErrorReport(item);
|
|
item = item.Save();
|
|
dicTrans_ItemIds.Remove(ProcNumber + "|" + seqRoTran);
|
|
dicTrans_MigrationErrors.Remove(ProcNumber + "|" + seqRoTran);
|
|
}
|
|
else
|
|
item = Item.MakeItem(FromItem, content, content.DTS, content.UserID);
|
|
|
|
// If we converted a Table to a Grid, then insert an Annotation to alert the user to verify it
|
|
if (IsATable(content.Type))//item.MyContent.MyGrid != null)
|
|
{
|
|
ItemAnnotation ia = item.ItemAnnotations.Add(VerificationRequiredType);
|
|
ia.SearchText = "Verify Table Conversion";
|
|
ia.UserID = "Migration";
|
|
item.Save();
|
|
}
|
|
if (InvalidROs.Count > 0)
|
|
{
|
|
foreach (string str in InvalidROs)
|
|
{
|
|
ItemAnnotation iaro = item.ItemAnnotations.Add(MigrationErrorType);
|
|
iaro.SearchText = str;
|
|
iaro.UserID = "Migration";
|
|
}
|
|
item.Save();
|
|
InvalidROs.Clear();
|
|
}
|
|
if (frType > 0)
|
|
{
|
|
using (Content c = Content.Get(parentItem.ContentID))
|
|
{
|
|
c.ContentParts.Add(frType, item);
|
|
if (!c.IsSavable) ErrorRpt.ErrorReport(c);
|
|
c.Save();
|
|
}
|
|
}
|
|
|
|
// Remove styles that user entered but are automatically done via the format
|
|
string tstr = null;
|
|
using (ItemInfo myInfo = item.MyItemInfo) // do this so that ItemInfo doesn't stay in cache
|
|
tstr = myInfo.RemoveRtfStyles(content.Text, fmt);
|
|
if (tstr != content.Text)
|
|
{
|
|
item.MyContent.Text = tstr;
|
|
item.Save();
|
|
}
|
|
|
|
if (migrationerrors != null)
|
|
{
|
|
foreach (string str in migrationerrors)
|
|
{
|
|
ItemAnnotation ia = item.ItemAnnotations.Add(MigrationErrorType);
|
|
ia.SearchText = str;
|
|
}
|
|
if (!item.IsSavable) ErrorRpt.ErrorReport(item);
|
|
item.Save();
|
|
}
|
|
if (_TransitionMigrationErrors.Count > 0)
|
|
{
|
|
foreach (string str in _TransitionMigrationErrors)
|
|
{
|
|
if (!dicTrans_MigrationErrors.ContainsKey(str))
|
|
dicTrans_MigrationErrors.Add(str, new List<Item>());
|
|
dicTrans_MigrationErrors[str].Add(item);
|
|
}
|
|
}
|
|
dicTrans_ItemDone[ProcNumber + "|" + seqRoTran] = item;
|
|
dicOldStepSequence[content] = seqcvt;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
frmMain.AddError(ex, "AddStep {0}",stpseq);
|
|
//Console.WriteLine("{0} {1}", ex.GetType().Name, ex.Message);
|
|
log.Error("Save Step");
|
|
log.ErrorFormat("oldstepsequence = {0}", stpseq);
|
|
log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
log.ErrorFormat(ex.StackTrace);
|
|
item = null;
|
|
}
|
|
|
|
// now add on any support pieces of text associated with the step.
|
|
// These include:
|
|
// '\1' comment
|
|
// '\2' multiple change ids and/or change message
|
|
// '\3' linked sequence
|
|
// '\3\3'override tab
|
|
// '\5' continuous action summary flag (only if last char in string)
|
|
// This assumes that these tokens are stored in reverse order that
|
|
// they are processed.
|
|
|
|
|
|
bool recdirty = false;
|
|
try
|
|
{
|
|
|
|
if (tok >= 0 && tok != Textm.Length)
|
|
{
|
|
string tkstring = Textm.Substring(tok);
|
|
int nxttok = 0;
|
|
|
|
if (tkstring[tkstring.Length - 1] == '\x5') // Continuous Action Summary
|
|
{
|
|
recdirty = true;
|
|
//if (ci == null) ci = new ConfigInfo(null);
|
|
ci.AddItem("Step", "ContActSum", "True");
|
|
tkstring = tkstring.Substring(0, tkstring.Length - 1); // strip off Continuous Action Token
|
|
}
|
|
|
|
if ((nxttok = tkstring.IndexOf("\x3\x3")) > -1) // Enhanced Override Tab
|
|
{
|
|
//Console.WriteLine("Override Tab: {0}", tkstring.Substring(nxttok + 1));
|
|
ci.AddItem("Step", "OverrideTab", tkstring.Substring(nxttok + 2));
|
|
recdirty = true;
|
|
//recdirty |= AddContentDetail(content, STP_OVR_TAB, tkstring.Substring(nxttok + 2));
|
|
tkstring = tkstring.Substring(0, nxttok);
|
|
}
|
|
|
|
if ((nxttok = tkstring.IndexOf("\x3")) > -1) //Linked Sequence
|
|
{
|
|
//Console.WriteLine("Linked Seq: {0}", tkstring.Substring(nxttok + 1));
|
|
ci.AddItem("Step", "LinkedSeq", TextConvert.ConvertSeq(tkstring.Substring(nxttok + 1)));
|
|
recdirty = true;
|
|
//recdirty |= AddContentDetail(content, STP_LNK_SEQ, TextConvert.ConvertSeq(tkstring.Substring(nxttok + 1)));
|
|
tkstring = tkstring.Substring(0, nxttok);
|
|
}
|
|
|
|
if ((nxttok = tkstring.IndexOf("\x2")) > -1) // Multiple Change Ids
|
|
{
|
|
//Console.WriteLine("Multiple Change ID: {0}", tkstring.Substring(nxttok + 1));
|
|
ci.AddItem("Step", "MultipleChangeID", tkstring.Substring(nxttok + 1));
|
|
recdirty = true;
|
|
//recdirty |= AddContentDetail(content, STP_MULT_CHGID, tkstring.Substring(nxttok + 1));
|
|
tkstring = tkstring.Substring(0, nxttok);
|
|
}
|
|
|
|
if ((nxttok = tkstring.IndexOf("\x1")) > -1) // Comment
|
|
{
|
|
// add the comment to annotation table. Set type to comment.
|
|
//log.InfoFormat("Comment text: {0}", tkstring.Substring(nxttok + 1));
|
|
Annotation annot = Annotation.MakeAnnotation(item, CommentType, null, tkstring.Substring(nxttok + 1), null, dts, userid);
|
|
}
|
|
}
|
|
|
|
// also see if a check-off needs added.
|
|
if (Recid[0] != '0')
|
|
{
|
|
recdirty = true;
|
|
string chkindx = Recid[0].ToString();
|
|
//if (ci == null) ci = new ConfigInfo(null);
|
|
ci.AddItem("Step", "CheckOffIndex", chkindx);
|
|
}
|
|
|
|
// here's where it knows if it's a linked step (or in processstep)
|
|
if (Recid[1] != '0')
|
|
{
|
|
// do linked step stuff.
|
|
}
|
|
|
|
// if checkoffs or the continuous action summary flag, save the xml.
|
|
if (recdirty)
|
|
{
|
|
if (ci.ItemCount != 0) content.Config = ci.ToString();
|
|
if (!content.IsSavable) ErrorRpt.ErrorReport(content);
|
|
content.Save();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//Console.WriteLine("Subtexts: {0} {1}", ex.GetType().Name, ex.Message);
|
|
frmMain.AddError(ex, "AddStep 2 {0}", stpseq);
|
|
log.Error("Save Step part 2");
|
|
log.ErrorFormat("oldstepsequence = {0}", stpseq);
|
|
log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
log.ErrorFormat(ex.StackTrace);
|
|
}
|
|
return item;
|
|
}
|
|
|
|
private int? ConvertTableType(Content content, FormatInfo fmt)
|
|
{
|
|
// set the type to the first format type from this step type walking up the stepdata format
|
|
// data until finding a !InActive.
|
|
bool inactive = true;
|
|
int indx = (int)content.Type - 20000;
|
|
while (inactive)
|
|
{
|
|
StepData stpData = fmt.PlantFormat.FormatData.StepDataList[indx];
|
|
if (!stpData.Inactive) return (Convert.ToInt32(stpData.Index) + 20000);
|
|
// find parent node, i.e. Type="parentType"
|
|
string parType = stpData.ParentType;
|
|
foreach (StepData sd in fmt.PlantFormat.FormatData.StepDataList)
|
|
if (parType == sd.Type) indx = (int)sd.Index;
|
|
inactive = stpData.Inactive;
|
|
}
|
|
return null;
|
|
}
|
|
|
|
private Regex _ReplaceSpaceNewLine = new Regex(@"(?<=\\[^' \\?\r\n\t]*) (?=\r\n)");
|
|
private Regex _ReplaceTokenSpaceToken = new Regex(@"(?<=\\[^' \\?\r\n\t]*) (?=\\)");
|
|
private string FixStepText(string stepText)
|
|
{
|
|
stepText = stepText.Replace("\xF8", @"\'f8");
|
|
stepText = stepText.Replace(@"\par ", "\r\n");
|
|
stepText = _ReplaceTokenSpaceToken.Replace(stepText, "");
|
|
//stepText = stepText.Replace(@"\v0 \", @"\v0\");
|
|
//Change Token Order to match RTB output
|
|
stepText = stepText.Replace(@"\v0\b0", @"\b0\v0");
|
|
stepText = stepText.Replace(@"\b0\ulnone", @"\ulnone\b0");
|
|
stepText = _ReplaceSpaceNewLine.Replace(stepText, "");
|
|
return stepText;
|
|
}
|
|
|
|
private Regex _RemoveComments = new Regex(@"\\v .*?\\v0( |$)");
|
|
|
|
private string ConvertTableToGrid(string stepText, Content content, FormatInfo fmt, bool isROTable, GridLinePattern border)
|
|
{
|
|
string savethis = stepText;
|
|
string strGrid = "";
|
|
VlnFlexGrid grd = new VlnFlexGrid(1, 1);
|
|
grd.IsRoTable = isROTable;
|
|
VE_Font vefont = fmt.PlantFormat.FormatData.StepDataList.Table.Font;
|
|
Font GridFont = new Font(vefont.Family, (float)vefont.Size);
|
|
grd.Font = GridFont; // this also changes the default Row Height "Rows.DefaultSize"
|
|
grd.ParseTableFromText(stepText,border);
|
|
grd.AutoSizeCols();
|
|
grd.AutoSizeRows();
|
|
grd.MakeRTFcells();
|
|
|
|
using (StringWriter sw = new StringWriter())
|
|
{
|
|
grd.WriteXml(sw);
|
|
//Console.WriteLine(sw.GetStringBuilder().ToString());
|
|
content.MyGrid.Data = sw.GetStringBuilder().ToString();
|
|
strGrid = grd.GetSearchableText();
|
|
sw.Close();
|
|
}
|
|
return strGrid;
|
|
}
|
|
|
|
private bool IsATable(int? contenttype)
|
|
{
|
|
bool rtnval = false;
|
|
switch (contenttype)
|
|
{
|
|
case 20008: // Centered table
|
|
case 20010: // AER table
|
|
case 20033: // AER table without boarder
|
|
case 20034: // Centered table without boarder
|
|
rtnval = true;
|
|
break;
|
|
}
|
|
return rtnval;
|
|
}
|
|
private bool WithoutBorder(int? contenttype)
|
|
{
|
|
bool rtnval = false;
|
|
switch (contenttype)
|
|
{
|
|
case 20033: // AER table without boarder
|
|
case 20034: // Centered table without boarder
|
|
rtnval = true;
|
|
break;
|
|
}
|
|
return rtnval;
|
|
}
|
|
|
|
//private static bool AddContentDetail(Content content, int type, string strn)
|
|
//{
|
|
// if (strn != null && strn.Trim() != "" )
|
|
// {
|
|
// content.ContentDetails.Add(type, strn);
|
|
// return true;
|
|
// }
|
|
// return false;
|
|
//}
|
|
|
|
private string GetParent(string s)
|
|
{
|
|
string retval = "S";
|
|
if (s.Length > 1)
|
|
{
|
|
int l = s.Length;
|
|
if ("!*".IndexOf(s[l - 2]) > -1)
|
|
{
|
|
if (l > 2) retval = s.Substring(0, l - 2);
|
|
}
|
|
else
|
|
{
|
|
retval = s.Substring(0, l - 1);
|
|
}
|
|
}
|
|
return retval;
|
|
}
|
|
private string GetStructType(string s)
|
|
{
|
|
string retval = "S";
|
|
if (s.Length > 1)
|
|
{
|
|
int l = s.Length;
|
|
if ("!*".IndexOf(s[l - 2]) > -1)
|
|
{
|
|
if (s[l - 2] == '!') retval = "C";
|
|
else retval = "N";
|
|
}
|
|
else
|
|
{
|
|
if (s[l - 1] == '$') retval = "R";
|
|
if (s[l - 1] == '#') retval = "T";
|
|
}
|
|
}
|
|
return retval;
|
|
}
|
|
private Item MigrateStep(OleDbConnection cn, DataTable dt, DataRowView drv, Item FromItem, bool conv_caret, string pth, DocVersion docver, FormatInfo fmt, Item parentItem, int frTypeParam)
|
|
{
|
|
try
|
|
{
|
|
string sType = GetStructType(drv["CSequence"].ToString());
|
|
Item item = AddStep(cn, drv["Type"].ToString()
|
|
, (drv["textm"] == DBNull.Value ? drv["Text"].ToString() : drv["Textm"].ToString())
|
|
, drv["Recid"].ToString(), drv["CStep"].ToString() + drv["CSequence"].ToString(), "S", FromItem // was str
|
|
, GetDTS(drv["Date"].ToString(), drv["Time"].ToString()), drv["Initials"].ToString(), conv_caret, pth, docver, fmt, parentItem, frTypeParam
|
|
, drv["Step"].ToString() + drv["CSequence"].ToString());
|
|
|
|
//Content cont = Content.MakeContent(null,(drv["textm"] == DBNull.Value ? drv["Text"].ToString() : drv["Textm"].ToString()),drv["Type"]+20000,null,null,
|
|
// GetDTS(drv["Date"].ToString(), drv["Time"].ToString()), drv["Initials"].ToString());
|
|
//Item item = Item.MakeItem(FromItem, cont, cont.DTS, cont.UserID);
|
|
//Structure str = AddStructure(FromType, FromID, 3, tmpid, drv["CStep"].ToString() + drv["CSequence"].ToString(),
|
|
// GetDTS(drv["Date"].ToString(), drv["Time"].ToString()), drv["Initials"].ToString());
|
|
//Step stp = AddStep(cn, drv["Type"].ToString()
|
|
// , (drv["textm"] == DBNull.Value ? drv["Text"].ToString() : drv["Textm"].ToString())
|
|
// , drv["Recid"].ToString(), drv["CStep"].ToString() + drv["CSequence"].ToString(), "S", str
|
|
// , GetDTS(drv["Date"].ToString(), drv["Time"].ToString()), drv["Initials"].ToString());
|
|
Dictionary<string, Item> dicStep = new Dictionary<string, Item>();
|
|
dicStep[drv["CSequence"].ToString()] = item;
|
|
Dictionary<string, Dictionary<string, Item>> dicStruct = new Dictionary<string, Dictionary<string, Item>>();
|
|
Dictionary<string, Item> dicBase = new Dictionary<string, Item>();
|
|
dicStruct[drv["CSequence"].ToString()] = dicBase;
|
|
dicBase[""] = item;
|
|
// Logic to add Sub-steps
|
|
string sQry = "CStep = '" + drv["CStep"].ToString() + "' and CSequence <> 'S'";
|
|
// sort order - for sections use currentrows.
|
|
DataView dv = new DataView(dt, sQry, "StepNo,Level,SubStepNo", DataViewRowState.CurrentRows);
|
|
//dataGrid1.DataSource=dv;
|
|
//Loop through DataView and add Steps one at a time
|
|
//Console.WriteLine("={0}",drv["Step"]);
|
|
int FrType = 0; // type of relationship (not type of step)
|
|
Item FrItem = item;
|
|
foreach (DataRowView drvs in dv)
|
|
{
|
|
//Console.WriteLine(">{0}",drvs["CStep"]);
|
|
|
|
string sParent = GetParent(drvs["CSequence"].ToString());
|
|
if (dicStep.ContainsKey(sParent))
|
|
{
|
|
Item itemp = dicStep[sParent];
|
|
sType = GetStructType(drvs["CSequence"].ToString());
|
|
Dictionary<string,Item> dicStr = dicStruct[sParent];
|
|
// check if a step type of 'sType' exists to see if child/sibling
|
|
//Content contc = Content.MakeContent(null,(drvs["textm"] == DBNull.Value ? drvs["Text"].ToString() : drvs["Textm"].ToString()),drvs["Type"]+20000,null,null,
|
|
// GetDTS(drvs["Date"].ToString(), drvs["Time"].ToString()), drvs["Initials"].ToString()));
|
|
Item itemc = null;
|
|
if (dicStr.ContainsKey(sType)) // next sibling
|
|
{
|
|
FrItem = dicStr[sType];
|
|
FrType = 0;
|
|
}
|
|
else // child of a node
|
|
{
|
|
FrItem = null;
|
|
FrType = (3 + ("CNRST".IndexOf(sType)));
|
|
}
|
|
|
|
itemc = AddStep(cn, drvs["Type"].ToString()
|
|
, (drvs["textm"] == DBNull.Value ? drvs["Text"].ToString() : drvs["Textm"].ToString())
|
|
, drvs["Recid"].ToString(), drvs["CStep"].ToString() + drvs["CSequence"].ToString()
|
|
, GetStructType(drvs["sequence"].ToString()), FrItem
|
|
, GetDTS(drvs["Date"].ToString(), drvs["Time"].ToString()), drvs["Initials"].ToString(), conv_caret, pth, docver, fmt, itemp, FrType
|
|
, drvs["Step"].ToString() + drvs["CSequence"].ToString());
|
|
//if (FrType > 0 )
|
|
//{
|
|
// itemp.MyContent.ContentParts.Add(FrType, itemc);
|
|
// if (!itemp.MyContent.IsSavable) ErrorRpt.ErrorReport(itemp.MyContent);
|
|
// itemp.MyContent.Save();
|
|
//}
|
|
dicStr[sType] = itemc;
|
|
dicStruct[drvs["CSequence"].ToString()] = new Dictionary<string, Item>();
|
|
dicStep[drvs["CSequence"].ToString()] = itemc;
|
|
}
|
|
else
|
|
{
|
|
log.ErrorFormat("Parent {0} Could not be found for {1}", sParent, drvs["sequence"].ToString());
|
|
}
|
|
}
|
|
dv.Dispose();
|
|
return item;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//Console.WriteLine("{0} {1}", ex.GetType().Name, ex.Message);
|
|
frmMain.AddError(ex, "MigrateStep");
|
|
log.Error("PROCESS STEP");
|
|
log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
log.ErrorFormat(ex.StackTrace);
|
|
return null;
|
|
}
|
|
}
|
|
}
|
|
} |