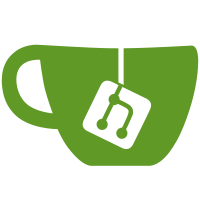
Added DebugStatus to MSWordToPDF Class Removed PDF info from Document Config Moved PDF info from Documents to PDFs Table Added MSWordPageCount Property Delete PDFs when RO Value Changes in a related document
115 lines
2.7 KiB
C#
115 lines
2.7 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
[Serializable]
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
public class DocumentConfig : ConfigDynamicTypeDescriptor, INotifyPropertyChanged
|
|
{
|
|
#region XML
|
|
private XMLProperties _Xp;
|
|
private XMLProperties Xp
|
|
{
|
|
get { return _Xp; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public Document _Document;
|
|
private DocumentInfo _DocumentInfo;
|
|
public DocumentConfig(Document document)
|
|
{
|
|
_Document = document;
|
|
string xml = _Document.Config;
|
|
if (xml == string.Empty) xml = "<config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public DocumentConfig(DocumentInfo documentInfo)
|
|
{
|
|
_DocumentInfo = documentInfo;
|
|
string xml = _DocumentInfo.Config;
|
|
if (xml == string.Empty) xml = "<config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
internal string GetValue(string group, string item)
|
|
{
|
|
return _Xp[group, item];
|
|
}
|
|
#endregion
|
|
#region Properties
|
|
[Category("General")]
|
|
[DisplayName("Name")]
|
|
[Description("Name")]
|
|
public string Name
|
|
{
|
|
get { return (_Document != null ? _Document.LibTitle : _DocumentInfo.LibTitle); }
|
|
set { if (_Document != null) _Document.LibTitle = value; }
|
|
}
|
|
[Category("General")]
|
|
[DisplayName("Comment")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Comment")]
|
|
public string LibDoc_Comment
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["LibDoc", "Comment"];// get the saved value
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
_Xp["LibDoc", "Comment"] = value;
|
|
OnPropertyChanged("LibDoc_Comment");
|
|
}
|
|
}
|
|
// RHM 20110415 - These are now stored in the PDF table.
|
|
//[Category("Printing")]
|
|
//[DisplayName("Length")]
|
|
//[Description("Length of Document in Full and Partial Pages")]
|
|
//public float Printing_Length
|
|
//{
|
|
// get
|
|
// {
|
|
// string s = _Xp["Printing", "Length"];// get the saved value
|
|
|
|
// return float.Parse(s);
|
|
// }
|
|
// set
|
|
// {
|
|
// _Xp["Printing", "Length"] = string.Format("{0:0.0000}", value);
|
|
// OnPropertyChanged("Printing_Length");
|
|
// }
|
|
//}
|
|
//[Category("Printing")]
|
|
//[DisplayName("Color")]
|
|
//[Description("Color of Document Text")]
|
|
//public Color Printing_Color
|
|
//{
|
|
// get
|
|
// {
|
|
// string sColor = _Xp["Printing", "Color"];
|
|
// return ColorConfig.ColorFromString(sColor);
|
|
// }
|
|
// set
|
|
// {
|
|
// _Xp["Printing", "Color"] = value.ToString();
|
|
// OnPropertyChanged("Printing_Color");
|
|
// }
|
|
//}
|
|
#endregion
|
|
#region ToString
|
|
public override string ToString()
|
|
{
|
|
string s = _Xp.ToString();
|
|
if (s == "<Config/>" || s == "<Config></Config>") return string.Empty;
|
|
return s;
|
|
}
|
|
#endregion
|
|
}
|
|
}
|