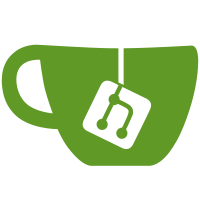
- Aligned Tables properly - Skip Section Title - Calculated Table Width - Fixed ChangeBar logic - Added Text and Debug layers - Adedd debug output to PDF - Changed Paginate logic - Adedd debug output to PDF - Move GetParagraphHeight - Added GetTableWidth - Added ChangeBar Properties - Added Debug and Text layers Removed fixed override color for SVG Removed simple PageBreak
215 lines
8.9 KiB
C#
215 lines
8.9 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using iTextSharp.text;
|
|
using iTextSharp.text.pdf;
|
|
using iTextSharp.text.factories;
|
|
using Itenso.Rtf;
|
|
using Itenso.Rtf.Parser;
|
|
using Itenso.Rtf.Interpreter;
|
|
using Itenso.Rtf.Support;
|
|
|
|
namespace Volian.Print.Library
|
|
{
|
|
public abstract partial class vlnPrintObject
|
|
{
|
|
public abstract float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin);
|
|
}
|
|
public partial class vlnPrintObjects : List<vlnPrintObject>
|
|
{
|
|
public float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
foreach (vlnPrintObject part in this)
|
|
{
|
|
yPageStart = part.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
}
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnParagraphs : List<vlnParagraph>
|
|
{
|
|
public float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
foreach (vlnParagraph child in this)
|
|
{
|
|
yPageStart = child.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
}
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnParagraph : vlnPrintObject
|
|
{
|
|
public float ParagraphToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
//Console.WriteLine("'{0}',{1},{2},{3},{4},{5},{6},{7},{8}", MyItemInfo.Path.Substring(_Prefix.Length).Trim(),
|
|
// cb.PdfWriter.CurrentPageNumber, yStart, YTop, yStart + YTop, yStart + YTop - _LastY, YTopMost, YSize, yPageStart);
|
|
//_LastY = yStart + YTop;
|
|
// 72 points per inchs. 504 is 7 inches wide. 3 1/2 inches wide: 252, 100);
|
|
if (_PartsAbove != null && _PartsAbove.Count > 0) yPageStart = PartsAbove.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
float yLocation = yPageStart - YOffset;
|
|
float retval = yLocation;
|
|
if (!MyItemInfo.IsStepSection) // Don't ouput the Step Section title
|
|
{
|
|
retval = Rtf2Pdf.TextAt(cb, IParagraph, XOffset, yLocation, Width, 100, PdfDebugText);
|
|
if (retval == 0)
|
|
{
|
|
cb.PdfDocument.NewPage();
|
|
yPageStart = yTopMargin + YTop;
|
|
yLocation = yPageStart - YOffset;
|
|
//MyItemInfo.ItemID, YSize, yPageSize, yLocation
|
|
Console.WriteLine("-1,'Yes','Forced Pagination',{0},{1},,{3},'{4}'", MyItemInfo.ItemID,YSize,0,yLocation,MyItemInfo.ShortPath);
|
|
retval = Rtf2Pdf.TextAt(cb, IParagraph, XOffset, yLocation, Width, 100, PdfDebugText);
|
|
}
|
|
}
|
|
//Height = yLocation - retval;
|
|
if (_PartsLeft != null && _PartsLeft.Count > 0) yPageStart = PartsLeft.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
if (_PartsRight != null && _PartsRight.Count > 0) yPageStart = PartsRight.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
if (_PartsBelow != null && _PartsBelow.Count > 0) yPageStart = PartsBelow.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
if (_PartsContainer != null && _PartsContainer.Count > 0) yPageStart = PartsContainer.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
return yPageStart;
|
|
}
|
|
private string PdfDebugText
|
|
{
|
|
get
|
|
{
|
|
return string.Format("ID={0} Type={1} TypeName='{2}' EveryNLines= {3}", MyItemInfo.ItemID, MyItemInfo.FormatStepType, MyItemInfo.FormatStepData.Type, MyItemInfo.FormatStepData.StepLayoutData.EveryNLines);
|
|
}
|
|
}
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YTopMost;
|
|
int paginate = Paginate(yLocation, yTopMargin, yBottomMargin);
|
|
switch (paginate)
|
|
{
|
|
case 1: // Break on High Level Step
|
|
cb.PdfDocument.NewPage();
|
|
yPageStart = yTopMargin + YTopMost;
|
|
break;
|
|
case 2: // Break within a Step
|
|
cb.PdfDocument.NewPage();
|
|
yPageStart = yTopMargin + YTopMost - 2 * _SixLinesPerInch;
|
|
break;
|
|
}
|
|
yPageStart = ChildrenAbove.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
yPageStart = ChildrenLeft.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
yPageStart = ParagraphToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
yPageStart = ChildrenRight.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
yPageStart = ChildrenBelow.ToPdf(cb, yPageStart, yTopMargin, yBottomMargin);
|
|
return yPageStart;
|
|
}
|
|
|
|
private int Paginate(float yLocation, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yPageSize = yTopMargin - yBottomMargin;
|
|
float yWithinMargins = yLocation - yBottomMargin;
|
|
if (MyItemInfo.ItemID == 879)
|
|
Console.Write(",'Here'");
|
|
if (MyItemInfo.IsStepSection) return 0; // Don't Paginate on a Step Section
|
|
if (YSize <= yWithinMargins)
|
|
{
|
|
if(MyItemInfo.IsHigh)
|
|
Console.WriteLine("1,'No','HLS will fit on page',{0},{1},{2}, {3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 0; // Don't Paginate if there is enough room
|
|
}
|
|
if (MyItemInfo.IsRNOPart && MyParent.XOffset < XOffset) return 0; // Don't paginate on an RNO to the right
|
|
if (MyItemInfo.IsHigh)
|
|
{
|
|
if (YSize < yPageSize) // if the entire step can fit on one page, do a page break
|
|
{
|
|
Console.WriteLine("2,'Yes','HLS will fit on 1 Page',{0},{1},{2}, {3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 1; // Paginate on High Level Steps
|
|
}
|
|
else // The entire step cannot fit go ahead and kick to the next page
|
|
{
|
|
if (yWithinMargins > yPageSize / 2)
|
|
{
|
|
// If High level Step will not fit, kick to the next page
|
|
Console.WriteLine("3,'No','HLS will have to split anyway',{0},{1},{2}, {3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 0;// Otherwise stay on this page
|
|
}
|
|
Console.WriteLine("3,'Yes','HLS will have to split',{0},{1},{2}, {3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 1; // Paginate on High Level Steps
|
|
}
|
|
}
|
|
if (yWithinMargins > yPageSize / 4)
|
|
{
|
|
Console.WriteLine("4,'No','Not Half way down the page',{0},{1},{2}, {3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 0; // More than half way down the page
|
|
}
|
|
//if (ChildrenBelow.Count > 0 && ChildrenBelow[0].YSize < yWithinMargins)
|
|
// return 0;
|
|
Console.WriteLine("5,'Yes','At least half the page is filled',{0},{1},{2},{3}, {4},'{5}'", MyItemInfo.ItemID, YSize, yPageSize, yWithinMargins, (int)(100 * yWithinMargins / yPageSize), MyItemInfo.ShortPath);
|
|
return 2;
|
|
}
|
|
}
|
|
public partial class vlnHeader : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YOffset;
|
|
Rtf2Pdf.TextAt(cb, IParagraph, XOffset, yLocation, HeaderWidth, 100,"");
|
|
return yPageStart;
|
|
}
|
|
}
|
|
|
|
public partial class vlnTab : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YOffset;
|
|
Rtf2Pdf.TextAt(cb, IParagraph, XOffset, yLocation, TabWidth, 100, "");
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnBox : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnChangeBar : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YOffset;
|
|
float yAdj = 3;
|
|
cb.SaveState();
|
|
cb.SetColorStroke(Color.GREEN);
|
|
cb.SetLineWidth(1);
|
|
cb.MoveTo(XOffset, yLocation-yAdj); // combination yStart and YOffset
|
|
cb.LineTo(XOffset, yLocation - MyParent.Height-yAdj);
|
|
cb.Stroke();
|
|
cb.RestoreState();
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnRNOSeparator : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YOffset;
|
|
Rtf2Pdf.TextAt(cb, IParagraph, XOffset, yLocation, Width, 100, "");
|
|
return yPageStart;
|
|
}
|
|
}
|
|
public partial class vlnMacro : vlnPrintObject
|
|
{
|
|
public override float ToPdf(PdfContentByte cb, float yPageStart, float yTopMargin, float yBottomMargin)
|
|
{
|
|
float yLocation = yPageStart - YOffset;
|
|
VlnSvgPageHelper _MyPageHelper = cb.PdfWriter.PageEvent as VlnSvgPageHelper;
|
|
PdfLayer textLayer = _MyPageHelper == null ? null : _MyPageHelper.TextLayer;
|
|
if (textLayer != null) cb.BeginLayer(textLayer);
|
|
MyContentByte = cb;
|
|
System.Drawing.Color push = PrintOverride.SvgColor;
|
|
PrintOverride.SvgColor = PrintOverride.TextColor == System.Drawing.Color.Empty ? System.Drawing.Color.Black : PrintOverride.TextColor ;
|
|
if (MyPageHelper != null && MyPageHelper.MySvg != null)
|
|
MyPageHelper.MySvg.DrawMacro(MacroDef, XOffset, yLocation, cb);
|
|
PrintOverride.SvgColor = push;
|
|
if (textLayer != null) cb.EndLayer();
|
|
return yPageStart;
|
|
}
|
|
}
|
|
}
|