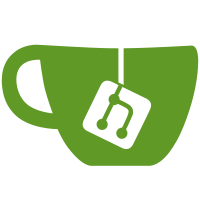
Add Memory Usage summary to the log file. GetJustFormat to reduce memory use Fix code so that children are not disposed while they are being used Added new method GetJustFormat to reduce memory use Fix logic so that content is not used after it is disposed Verify that Content object is not disposed before using Text Dispose of parts when Content object is disposed Verify that ContentInfo object is not disposed before using Text Dispose of parts when ContentInfo object is disposed Place brackets around DB names to support names containing periods. Dispose of parts when Item object is disposed Removed inapproriate Dispose in MakeItem Dispose of parts when ItemInfo object is disposed Remove event handler when ItemInfoList object is disposed Dispose of parts when Transtion object is disposed Dispose of parts when ZTransition object is disposed
236 lines
8.8 KiB
C#
236 lines
8.8 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Diagnostics;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// Database Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
public static partial class Database
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
public static void LogException(string s, Exception ex)
|
|
{
|
|
int i = 0;
|
|
Console.WriteLine("Error - {0}", s);
|
|
for (; ex != null; ex = ex.InnerException)
|
|
{
|
|
Console.WriteLine("{0}{1} - {2}", "".PadLeft(++i * 2), ex.GetType().ToString(), ex.Message);
|
|
}
|
|
}
|
|
private static bool _LoggingInfo = false; // By default don't log info
|
|
public static bool LoggingInfo
|
|
{
|
|
get { return _LoggingInfo; }
|
|
set { _LoggingInfo = value; }
|
|
}
|
|
static System.Diagnostics.Process _CurrentProcess = System.Diagnostics.Process.GetCurrentProcess();
|
|
public static void LogInfo(string s, int hashCode)
|
|
{
|
|
if (_LoggingInfo)
|
|
Console.WriteLine("{0} MB {1}", _CurrentProcess.WorkingSet64 / 1000000, string.Format(s, hashCode));
|
|
}
|
|
public static void LogDebug(string s, int hashCode)
|
|
{
|
|
if (_LoggingInfo)
|
|
Console.WriteLine("{0} MB {1}", _CurrentProcess.WorkingSet64 / 1000000, string.Format(s, hashCode));
|
|
}
|
|
private static string _ConnectionName = "VEPROMS";
|
|
public static string ConnectionName
|
|
{
|
|
get { return Database._ConnectionName; }
|
|
set { Database._ConnectionName = value; _VEPROMS_Connection = null; /* Reset Connection */ }
|
|
}
|
|
private static string _VEPROMS_Connection;
|
|
public static string VEPROMS_Connection
|
|
{
|
|
get
|
|
{
|
|
if (_VEPROMS_Connection != null) // Use Lazy Load
|
|
return _VEPROMS_Connection;
|
|
DateTime.Today.ToLongDateString();
|
|
// If DBConnection.XML exists, use the connection string from DBConnection.XML
|
|
string cnOverride = System.Windows.Forms.Application.StartupPath + @"\DBConnection.XML";
|
|
if (System.IO.File.Exists(cnOverride))
|
|
{
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.Load(cnOverride);
|
|
System.Xml.XmlNode xn = xd.SelectSingleNode("DBConnection/@string");
|
|
if (xn != null)
|
|
{
|
|
return _VEPROMS_Connection = xn.InnerText;
|
|
}
|
|
}
|
|
// Otherwise get the value from the ConfigurationManager
|
|
ConnectionStringSettings cs = ConfigurationManager.ConnectionStrings[ConnectionName];
|
|
if (cs == null)
|
|
{
|
|
throw new ApplicationException("Database.cs Could not find connection " + ConnectionName);
|
|
}
|
|
string constr = cs.ConnectionString;
|
|
if (constr.Contains("{MENU}"))
|
|
{
|
|
constr = ChooseDatabase(constr);
|
|
}
|
|
return _VEPROMS_Connection = constr;
|
|
}
|
|
set { _VEPROMS_Connection = value; }
|
|
}
|
|
private static string _SelectedDatabase;
|
|
public static string SelectedDatabase
|
|
{
|
|
get { return Database._SelectedDatabase; }
|
|
set { Database._SelectedDatabase = value; }
|
|
}
|
|
private static string _LastDatabase="NoDefault";
|
|
public static string LastDatabase
|
|
{
|
|
get { return Database._LastDatabase; }
|
|
set { Database._LastDatabase = value; }
|
|
}
|
|
private static string ChooseDatabase(string constr)
|
|
{
|
|
if (LastDatabase != "NoDefault" && LastDatabase != "")
|
|
{
|
|
if (System.Windows.Forms.MessageBox.Show("Open " + LastDatabase, "Reopen Last Database", System.Windows.Forms.MessageBoxButtons.YesNo, System.Windows.Forms.MessageBoxIcon.Question) == System.Windows.Forms.DialogResult.Yes)
|
|
{
|
|
SelectedDatabase = LastDatabase;
|
|
return constr.Replace("{MENU}", _SelectedDatabase);
|
|
}
|
|
}
|
|
System.Windows.Forms.ContextMenuStrip cms = BuildDatabaseMenu(constr);
|
|
while (_SelectedDatabase == null)
|
|
{
|
|
cms.Show(new System.Drawing.Point((System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Width - cms.Width) / 2, (System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Height - cms.Height) / 2));
|
|
System.Windows.Forms.Application.DoEvents();
|
|
}
|
|
return constr.Replace("{MENU}", _SelectedDatabase);
|
|
}
|
|
private static System.Windows.Forms.ContextMenuStrip BuildDatabaseMenu(string constr)
|
|
{
|
|
string tmp = constr.Replace("{MENU}", "master");
|
|
SqlConnection cn = new SqlConnection(tmp);
|
|
cn.Open();
|
|
// SqlDataAdapter da = new SqlDataAdapter("select name from sysdatabases where name like 'VEP%' order by name", cn);
|
|
SqlDataAdapter da = new SqlDataAdapter("select name, case when object_id('[' + name + ']..Items') is null then 'Not PROMS' when object_id('[' + name + ']..Revisions') is not null then 'Approval' when object_id('[' + name + ']..ContentAudits') is not null then 'Change Manager' else 'Original' end functionality from sysdatabases where name not in ('master','model','msdb','tempdb') order by name", cn);
|
|
DataSet ds = new DataSet();
|
|
da.Fill(ds);
|
|
cn.Close();
|
|
System.Windows.Forms.ContextMenuStrip cms = new System.Windows.Forms.ContextMenuStrip();
|
|
cms.Items.Add("Choose Database");
|
|
System.Windows.Forms.ToolStripMenuItem tsmi = cms.Items[0] as System.Windows.Forms.ToolStripMenuItem;
|
|
tsmi.BackColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaption);// System.Drawing.Color.Pink;
|
|
tsmi.ForeColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaptionText);
|
|
tsmi.Font = new System.Drawing.Font(tsmi.Font, System.Drawing.FontStyle.Bold);
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
{
|
|
if (dr["functionality"].ToString() == "Approval")
|
|
cms.Items.Add(dr["name"].ToString(), null, new EventHandler(Database_Click));
|
|
}
|
|
return cms;
|
|
}
|
|
|
|
static void Database_Click(object sender, EventArgs e)
|
|
{
|
|
System.Windows.Forms.ToolStripMenuItem tsmi = sender as System.Windows.Forms.ToolStripMenuItem;
|
|
if (tsmi != null)
|
|
{
|
|
_SelectedDatabase = tsmi.Text;
|
|
}
|
|
}
|
|
|
|
public static SqlConnection VEPROMS_SqlConnection
|
|
{
|
|
get
|
|
{
|
|
string strConn = VEPROMS_Connection; // If failure - Fail (Don't try to catch)
|
|
// Attempt to make a connection
|
|
try
|
|
{
|
|
SqlConnection cn = new SqlConnection(strConn);
|
|
cn.Open();
|
|
return cn;
|
|
}
|
|
catch (SqlException exsql)
|
|
{
|
|
const string strAttachError = "An attempt to attach an auto-named database for file ";
|
|
if (exsql.Message.StartsWith(strAttachError))
|
|
{// Check to see if the file is missing
|
|
string sFile = exsql.Message.Substring(strAttachError.Length);
|
|
sFile = sFile.Substring(0, sFile.IndexOf(" failed"));
|
|
// "An attempt to attach an auto-named database for file <mdf file> failed"
|
|
if (strConn.ToLower().IndexOf("user instance=true") < 0)
|
|
{
|
|
throw new ApplicationException("Connection String missing attribute: User Instance=True");
|
|
}
|
|
if (System.IO.File.Exists(sFile))
|
|
{
|
|
throw new ApplicationException("Database file " + sFile + " Cannot be opened\r\n", exsql);
|
|
}
|
|
else
|
|
{
|
|
throw new FileNotFoundException("Database file " + sFile + " Not Found", exsql);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
throw new ApplicationException("Failure on Connect", exsql);
|
|
}
|
|
}
|
|
catch (Exception ex)// Throw Application Exception on Failure
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Connection Error", ex);
|
|
throw new ApplicationException("Failure on Connect", ex);
|
|
}
|
|
}
|
|
}
|
|
public static void PurgeData()
|
|
{
|
|
try
|
|
{
|
|
//SqlConnection cn = VEPROMS_SqlConnection;
|
|
//SqlCommand cmd = new SqlCommand("purgedata", cn);
|
|
using (SqlConnection cn = VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand("purgedata", cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
cmd.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Purge Error", ex);
|
|
throw new ApplicationException("Failure on Purge", ex);
|
|
}
|
|
}
|
|
}
|
|
public class DbCslaException : Exception
|
|
{
|
|
internal DbCslaException(string message, Exception innerException) : base(message, innerException) { ;}
|
|
internal DbCslaException(string message) : base(message) { ;}
|
|
internal DbCslaException() : base() { ;}
|
|
} // Class
|
|
} // Namespace
|