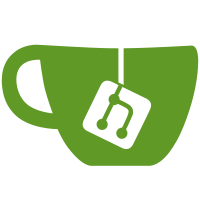
- New GenMac code - Anchors on form design - Exclude list to eliminate unsupported formats - Added namespace to svg element - Added black stroke attribute to line element Added Checkoffs Changed ChangeBar structures
595 lines
22 KiB
C#
595 lines
22 KiB
C#
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.IO;
|
|
|
|
namespace fmtxml
|
|
{
|
|
/// <summary>
|
|
/// Summary description for Form1.
|
|
/// </summary>
|
|
public class Form1 : System.Windows.Forms.Form
|
|
{
|
|
private System.Windows.Forms.Button btnCvtPagDoc;
|
|
private System.Windows.Forms.Label label1;
|
|
private System.Windows.Forms.Button btnExit;
|
|
private System.Windows.Forms.ListBox listBox1;
|
|
private System.Windows.Forms.Button btnCvtGenmac;
|
|
private System.Windows.Forms.Button btnCvtXmlToSVG;
|
|
//private C1.C1Pdf.C1PdfDocument c1PdfDocument1;
|
|
private Button btnCvtFormat;
|
|
private Button btnMergeFmtPagDoc;
|
|
private Label lblPathFmt;
|
|
private Button btnBrowseFmt;
|
|
private TextBox tbFmtPath;
|
|
private TextBox tbGenmacPath;
|
|
private Button btnBrowseGenmac;
|
|
private Label lblPathGenmac;
|
|
private Label lblResult;
|
|
private TextBox tbResultPath;
|
|
private Button btnBrowseResult;
|
|
private StatusStrip statusStrip1;
|
|
private ToolStripStatusLabel tsslStatus;
|
|
private Button btnRtfToSvg;
|
|
/// <summary>
|
|
/// Required designer variable.
|
|
/// </summary>
|
|
private System.ComponentModel.Container components = null;
|
|
|
|
public Form1()
|
|
{
|
|
//
|
|
// Required for Windows Form Designer support
|
|
//
|
|
InitializeComponent();
|
|
tbFmtPath.Text = @"c:\16bit\ve-proms\format";
|
|
tbGenmacPath.Text = @"C:\16bit\promsnt\genmac";
|
|
tbResultPath.Text = @"c:\development\";
|
|
//
|
|
// TODO: Add any constructor code after InitializeComponent call
|
|
//
|
|
}
|
|
public string MyStatus
|
|
{
|
|
get { return tsslStatus.Text; }
|
|
set { tsslStatus.Text = value; Application.DoEvents(); }
|
|
}
|
|
|
|
/// <summary>
|
|
/// Clean up any resources being used.
|
|
/// </summary>
|
|
protected override void Dispose( bool disposing )
|
|
{
|
|
if( disposing )
|
|
{
|
|
if (components != null)
|
|
{
|
|
components.Dispose();
|
|
}
|
|
}
|
|
base.Dispose( disposing );
|
|
}
|
|
|
|
#region Windows Form Designer generated code
|
|
/// <summary>
|
|
/// Required method for Designer support - do not modify
|
|
/// the contents of this method with the code editor.
|
|
/// </summary>
|
|
private void InitializeComponent()
|
|
{
|
|
this.btnCvtPagDoc = new System.Windows.Forms.Button();
|
|
this.label1 = new System.Windows.Forms.Label();
|
|
this.btnExit = new System.Windows.Forms.Button();
|
|
this.listBox1 = new System.Windows.Forms.ListBox();
|
|
this.btnCvtGenmac = new System.Windows.Forms.Button();
|
|
this.btnCvtXmlToSVG = new System.Windows.Forms.Button();
|
|
this.btnCvtFormat = new System.Windows.Forms.Button();
|
|
this.btnMergeFmtPagDoc = new System.Windows.Forms.Button();
|
|
this.lblPathFmt = new System.Windows.Forms.Label();
|
|
this.btnBrowseFmt = new System.Windows.Forms.Button();
|
|
this.tbFmtPath = new System.Windows.Forms.TextBox();
|
|
this.tbGenmacPath = new System.Windows.Forms.TextBox();
|
|
this.btnBrowseGenmac = new System.Windows.Forms.Button();
|
|
this.lblPathGenmac = new System.Windows.Forms.Label();
|
|
this.lblResult = new System.Windows.Forms.Label();
|
|
this.tbResultPath = new System.Windows.Forms.TextBox();
|
|
this.btnBrowseResult = new System.Windows.Forms.Button();
|
|
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
|
|
this.tsslStatus = new System.Windows.Forms.ToolStripStatusLabel();
|
|
this.btnRtfToSvg = new System.Windows.Forms.Button();
|
|
this.statusStrip1.SuspendLayout();
|
|
this.SuspendLayout();
|
|
//
|
|
// btnCvtPagDoc
|
|
//
|
|
this.btnCvtPagDoc.Location = new System.Drawing.Point(181, 55);
|
|
this.btnCvtPagDoc.Name = "btnCvtPagDoc";
|
|
this.btnCvtPagDoc.Size = new System.Drawing.Size(167, 44);
|
|
this.btnCvtPagDoc.TabIndex = 0;
|
|
this.btnCvtPagDoc.Text = "Convert Page and Doc Styles in format directory (Step 2)";
|
|
this.btnCvtPagDoc.Click += new System.EventHandler(this.btnCvtPagDoc_Click);
|
|
//
|
|
// label1
|
|
//
|
|
this.label1.Location = new System.Drawing.Point(10, 205);
|
|
this.label1.Name = "label1";
|
|
this.label1.Size = new System.Drawing.Size(167, 16);
|
|
this.label1.TabIndex = 1;
|
|
this.label1.Text = "Converted:";
|
|
//
|
|
// btnExit
|
|
//
|
|
this.btnExit.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnExit.Location = new System.Drawing.Point(572, 194);
|
|
this.btnExit.Name = "btnExit";
|
|
this.btnExit.Size = new System.Drawing.Size(52, 24);
|
|
this.btnExit.TabIndex = 2;
|
|
this.btnExit.Text = "Exit";
|
|
this.btnExit.Click += new System.EventHandler(this.btnExit_Click);
|
|
//
|
|
// listBox1
|
|
//
|
|
this.listBox1.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
|
|
| System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.listBox1.Location = new System.Drawing.Point(8, 224);
|
|
this.listBox1.Name = "listBox1";
|
|
this.listBox1.Size = new System.Drawing.Size(616, 277);
|
|
this.listBox1.TabIndex = 3;
|
|
//
|
|
// btnCvtGenmac
|
|
//
|
|
this.btnCvtGenmac.Enabled = false;
|
|
this.btnCvtGenmac.Location = new System.Drawing.Point(8, 149);
|
|
this.btnCvtGenmac.Name = "btnCvtGenmac";
|
|
this.btnCvtGenmac.Size = new System.Drawing.Size(153, 40);
|
|
this.btnCvtGenmac.TabIndex = 4;
|
|
this.btnCvtGenmac.Text = "Convert genmac files to XML (Genmac Step 1)";
|
|
this.btnCvtGenmac.Click += new System.EventHandler(this.btnCvtGenmac_Click);
|
|
//
|
|
// btnCvtXmlToSVG
|
|
//
|
|
this.btnCvtXmlToSVG.Enabled = false;
|
|
this.btnCvtXmlToSVG.Location = new System.Drawing.Point(166, 147);
|
|
this.btnCvtXmlToSVG.Name = "btnCvtXmlToSVG";
|
|
this.btnCvtXmlToSVG.Size = new System.Drawing.Size(152, 44);
|
|
this.btnCvtXmlToSVG.TabIndex = 5;
|
|
this.btnCvtXmlToSVG.Text = "Convert Genmac_XML to SVG (Genmac Step 2)";
|
|
this.btnCvtXmlToSVG.Click += new System.EventHandler(this.btnCvtXmlToSVG_Click);
|
|
//
|
|
// btnCvtFormat
|
|
//
|
|
this.btnCvtFormat.Location = new System.Drawing.Point(8, 55);
|
|
this.btnCvtFormat.Name = "btnCvtFormat";
|
|
this.btnCvtFormat.Size = new System.Drawing.Size(168, 44);
|
|
this.btnCvtFormat.TabIndex = 8;
|
|
this.btnCvtFormat.Text = "Convert Format in format directory (Step 1)";
|
|
this.btnCvtFormat.UseVisualStyleBackColor = true;
|
|
this.btnCvtFormat.Click += new System.EventHandler(this.btnCvtFormat_Click);
|
|
//
|
|
// btnMergeFmtPagDoc
|
|
//
|
|
this.btnMergeFmtPagDoc.Location = new System.Drawing.Point(353, 55);
|
|
this.btnMergeFmtPagDoc.Name = "btnMergeFmtPagDoc";
|
|
this.btnMergeFmtPagDoc.Size = new System.Drawing.Size(168, 44);
|
|
this.btnMergeFmtPagDoc.TabIndex = 9;
|
|
this.btnMergeFmtPagDoc.Text = "Integrate Fmt, Doc & Pag to single xml (Step 3)";
|
|
this.btnMergeFmtPagDoc.UseVisualStyleBackColor = true;
|
|
this.btnMergeFmtPagDoc.Click += new System.EventHandler(this.btnMergeFmtPagDoc_Click);
|
|
//
|
|
// lblPathFmt
|
|
//
|
|
this.lblPathFmt.AutoSize = true;
|
|
this.lblPathFmt.Location = new System.Drawing.Point(6, 36);
|
|
this.lblPathFmt.Name = "lblPathFmt";
|
|
this.lblPathFmt.Size = new System.Drawing.Size(104, 13);
|
|
this.lblPathFmt.TabIndex = 10;
|
|
this.lblPathFmt.Text = "Path To Format Files";
|
|
//
|
|
// btnBrowseFmt
|
|
//
|
|
this.btnBrowseFmt.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseFmt.Location = new System.Drawing.Point(502, 31);
|
|
this.btnBrowseFmt.Name = "btnBrowseFmt";
|
|
this.btnBrowseFmt.Size = new System.Drawing.Size(127, 20);
|
|
this.btnBrowseFmt.TabIndex = 11;
|
|
this.btnBrowseFmt.Text = "Browse (Format)";
|
|
this.btnBrowseFmt.UseVisualStyleBackColor = true;
|
|
this.btnBrowseFmt.Click += new System.EventHandler(this.btnBrowseFmt_Click);
|
|
//
|
|
// tbFmtPath
|
|
//
|
|
this.tbFmtPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbFmtPath.Location = new System.Drawing.Point(129, 32);
|
|
this.tbFmtPath.Name = "tbFmtPath";
|
|
this.tbFmtPath.Size = new System.Drawing.Size(358, 20);
|
|
this.tbFmtPath.TabIndex = 12;
|
|
//
|
|
// tbGenmacPath
|
|
//
|
|
this.tbGenmacPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbGenmacPath.Enabled = false;
|
|
this.tbGenmacPath.Location = new System.Drawing.Point(129, 125);
|
|
this.tbGenmacPath.Name = "tbGenmacPath";
|
|
this.tbGenmacPath.Size = new System.Drawing.Size(358, 20);
|
|
this.tbGenmacPath.TabIndex = 15;
|
|
//
|
|
// btnBrowseGenmac
|
|
//
|
|
this.btnBrowseGenmac.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseGenmac.Enabled = false;
|
|
this.btnBrowseGenmac.Location = new System.Drawing.Point(502, 124);
|
|
this.btnBrowseGenmac.Name = "btnBrowseGenmac";
|
|
this.btnBrowseGenmac.Size = new System.Drawing.Size(127, 20);
|
|
this.btnBrowseGenmac.TabIndex = 14;
|
|
this.btnBrowseGenmac.Text = "Browse (Genmac)";
|
|
this.btnBrowseGenmac.UseVisualStyleBackColor = true;
|
|
this.btnBrowseGenmac.Click += new System.EventHandler(this.btnBrowseGenmac_Click);
|
|
//
|
|
// lblPathGenmac
|
|
//
|
|
this.lblPathGenmac.AutoSize = true;
|
|
this.lblPathGenmac.Enabled = false;
|
|
this.lblPathGenmac.Location = new System.Drawing.Point(6, 129);
|
|
this.lblPathGenmac.Name = "lblPathGenmac";
|
|
this.lblPathGenmac.Size = new System.Drawing.Size(112, 13);
|
|
this.lblPathGenmac.TabIndex = 13;
|
|
this.lblPathGenmac.Text = "Path To Genmac Files";
|
|
//
|
|
// lblResult
|
|
//
|
|
this.lblResult.AutoSize = true;
|
|
this.lblResult.Location = new System.Drawing.Point(13, 9);
|
|
this.lblResult.Name = "lblResult";
|
|
this.lblResult.Size = new System.Drawing.Size(123, 13);
|
|
this.lblResult.TabIndex = 16;
|
|
this.lblResult.Text = "Path To Result Directory";
|
|
//
|
|
// tbResultPath
|
|
//
|
|
this.tbResultPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbResultPath.Location = new System.Drawing.Point(154, 7);
|
|
this.tbResultPath.Name = "tbResultPath";
|
|
this.tbResultPath.Size = new System.Drawing.Size(333, 20);
|
|
this.tbResultPath.TabIndex = 17;
|
|
//
|
|
// btnBrowseResult
|
|
//
|
|
this.btnBrowseResult.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseResult.Location = new System.Drawing.Point(502, 3);
|
|
this.btnBrowseResult.Name = "btnBrowseResult";
|
|
this.btnBrowseResult.Size = new System.Drawing.Size(127, 20);
|
|
this.btnBrowseResult.TabIndex = 18;
|
|
this.btnBrowseResult.Text = "Browse (Result)";
|
|
this.btnBrowseResult.UseVisualStyleBackColor = true;
|
|
this.btnBrowseResult.Click += new System.EventHandler(this.btnBrowseResult_Click);
|
|
//
|
|
// statusStrip1
|
|
//
|
|
this.statusStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
|
|
this.tsslStatus});
|
|
this.statusStrip1.Location = new System.Drawing.Point(0, 548);
|
|
this.statusStrip1.Name = "statusStrip1";
|
|
this.statusStrip1.Size = new System.Drawing.Size(631, 22);
|
|
this.statusStrip1.TabIndex = 19;
|
|
this.statusStrip1.Text = "statusStrip1";
|
|
//
|
|
// tsslStatus
|
|
//
|
|
this.tsslStatus.Name = "tsslStatus";
|
|
this.tsslStatus.Size = new System.Drawing.Size(39, 17);
|
|
this.tsslStatus.Text = "Ready";
|
|
//
|
|
// btnRtfToSvg
|
|
//
|
|
this.btnRtfToSvg.Location = new System.Drawing.Point(353, 145);
|
|
this.btnRtfToSvg.Name = "btnRtfToSvg";
|
|
this.btnRtfToSvg.Size = new System.Drawing.Size(152, 44);
|
|
this.btnRtfToSvg.TabIndex = 20;
|
|
this.btnRtfToSvg.Text = "Convert Genmac RTF to SVG (GenMac One Step)";
|
|
this.btnRtfToSvg.Click += new System.EventHandler(this.btnRtfToSvg_Click);
|
|
//
|
|
// Form1
|
|
//
|
|
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
|
|
this.ClientSize = new System.Drawing.Size(631, 570);
|
|
this.Controls.Add(this.btnRtfToSvg);
|
|
this.Controls.Add(this.statusStrip1);
|
|
this.Controls.Add(this.btnBrowseResult);
|
|
this.Controls.Add(this.tbResultPath);
|
|
this.Controls.Add(this.lblResult);
|
|
this.Controls.Add(this.tbGenmacPath);
|
|
this.Controls.Add(this.btnBrowseGenmac);
|
|
this.Controls.Add(this.lblPathGenmac);
|
|
this.Controls.Add(this.tbFmtPath);
|
|
this.Controls.Add(this.btnBrowseFmt);
|
|
this.Controls.Add(this.lblPathFmt);
|
|
this.Controls.Add(this.btnMergeFmtPagDoc);
|
|
this.Controls.Add(this.btnCvtFormat);
|
|
this.Controls.Add(this.btnCvtXmlToSVG);
|
|
this.Controls.Add(this.btnCvtGenmac);
|
|
this.Controls.Add(this.listBox1);
|
|
this.Controls.Add(this.btnExit);
|
|
this.Controls.Add(this.label1);
|
|
this.Controls.Add(this.btnCvtPagDoc);
|
|
this.Name = "Form1";
|
|
this.Text = "Form1";
|
|
this.statusStrip1.ResumeLayout(false);
|
|
this.statusStrip1.PerformLayout();
|
|
this.ResumeLayout(false);
|
|
this.PerformLayout();
|
|
|
|
}
|
|
#endregion
|
|
|
|
/// <summary>
|
|
/// The main entry point for the application.
|
|
/// </summary>
|
|
[STAThread]
|
|
static void Main()
|
|
{
|
|
Application.Run(new Form1());
|
|
}
|
|
private static string[] _ExcludeList = "ANO,ATA,ATLAS,LPL,MYA,NAE,NAI,NAS,PAC,RBN,SCE,SRS,SUM,VCS,VP,WPF,YAE".Split(",".ToCharArray());
|
|
public static bool Excluded(FileInfo fi)
|
|
{
|
|
foreach (string prefix in _ExcludeList)
|
|
if (fi.Name.ToUpper().StartsWith(prefix)) return true;
|
|
return false;
|
|
}
|
|
|
|
private void btnCvtPagDoc_Click(object sender, System.EventArgs e)
|
|
{
|
|
CleanupDestinationFolder("*p.xml");
|
|
CleanupDestinationFolder("*d.xml");
|
|
// get all of the files in the e:\ve-proms\format directory.
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text); // "C:\\16bit\\ve-proms\\format");
|
|
FileInfo[] fis = di.GetFiles("*.pag");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
string fn = fi.Name.Substring(0, fi.Name.Length - 4);
|
|
AddFileToListBox(fi);
|
|
FmtToXml fx = new FmtToXml(fn, tbFmtPath.Text);
|
|
}
|
|
}
|
|
fis = di.GetFiles("*.y*");
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
AddFileToListBox(fi);
|
|
FmtToXml fx = new FmtToXml(fi.Name, tbFmtPath.Text);
|
|
}
|
|
}
|
|
MessageBox.Show("DONE Converting Page and Document Styles to XML");
|
|
}
|
|
|
|
private void AddFileToListBox(FileInfo fi)
|
|
{
|
|
this.listBox1.Items.Add(fi.Name);
|
|
this.listBox1.SelectedIndex = this.listBox1.Items.Count - 1;
|
|
MyStatus = "Processing " + fi.Name;
|
|
Application.DoEvents();
|
|
}
|
|
|
|
private void ClearListBox()
|
|
{
|
|
listBox1.Items.Clear();
|
|
}
|
|
|
|
private void btnExit_Click(object sender, System.EventArgs e)
|
|
{
|
|
Application.Exit();
|
|
}
|
|
|
|
private void btnCvtGenmac_Click(object sender, System.EventArgs e)
|
|
{
|
|
// before doing the genmac change, use cpp -DRTF file after genmac.h
|
|
// has been emptied out on files in \promsnt\genmac. This creates
|
|
// precompiled files only, i.e. gets rid of some of the junk in the files.
|
|
// This conversion is done 1 time. After 32-bit print is done, the xml
|
|
// files will be used rather than the genmac.
|
|
|
|
DirectoryInfo di = new DirectoryInfo(tbGenmacPath.Text + @"\preproc"); // "C:\\16bit\\promsnt\\genmac\\preproc");
|
|
FileInfo[] fis = di.GetFiles("*.i");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// results go to e:\\proms.net\\genmac.xml\\convert
|
|
if (!Excluded(fi))
|
|
{
|
|
AddFileToListBox(fi);
|
|
GenToXml gn = new GenToXml(fi.Name, tbGenmacPath.Text, tbResultPath.Text);
|
|
}
|
|
}
|
|
MessageBox.Show("DONE Converting genmacs to XML - Results are in " + tbResultPath.Text + @"\genmacall\convert");
|
|
}
|
|
|
|
private void btnCvtXmlToSVG_Click(object sender, System.EventArgs e)
|
|
{
|
|
// delete current svg's
|
|
DirectoryInfo disvg = new DirectoryInfo(tbResultPath.Text + @"\genmacall"); //(@"C:\development\proms.net\genmac.xml");
|
|
FileInfo[] fissvg = disvg.GetFiles("*.svg");
|
|
foreach (FileInfo fi in fissvg)fi.Delete();
|
|
DirectoryInfo di = new DirectoryInfo(tbResultPath.Text + @"\genmacall\convert");
|
|
FileInfo[] fis = di.GetFiles("*.xml");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// results go to e:\proms.net\genmac.xml
|
|
AddFileToListBox(fi);
|
|
GenXmlToSvg gn = new GenXmlToSvg(fi.Name, tbResultPath.Text);
|
|
}
|
|
MessageBox.Show("DONE Converting genmacs.xmls to drawing svg - Results are in " + tbResultPath.Text + @"\genmacall");
|
|
}
|
|
|
|
|
|
private void btnCvtFormat_Click(object sender, EventArgs e)
|
|
{
|
|
CleanupDestinationFolder("*f.xml");
|
|
DateTime tStart = DateTime.Now;
|
|
// first convert base format - for now use genfmt.
|
|
MyStatus = "Processing Base";
|
|
FmtFileToXml gx = new FmtFileToXml(null, "BASE", "GEN", tbFmtPath.Text); // Create BASE from GEN format
|
|
|
|
// get all of the format files in the e:\ve-proms\format directory.
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text); // "C:\\16bit\\ve-proms\\format");
|
|
//DirectoryInfo di = new DirectoryInfo("E:\\proms.net\\exe\\fmtxml");
|
|
// This excludes Background format files *.BFM which use a different structure
|
|
FileInfo[] fis = di.GetFiles("*.fmt");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
string fn = fi.Name.Substring(0, fi.Name.Length - 4);
|
|
if (fn.ToLower() != "base")
|
|
{
|
|
AddFileToListBox(fi);
|
|
FmtFileToXml fx = new FmtFileToXml(gx, fn, fn, tbFmtPath.Text);
|
|
}
|
|
}
|
|
}
|
|
MyStatus = string.Format("DONE Converting Formats to XML {0:F3} seconds", TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds);
|
|
MessageBox.Show("DONE Converting Formats to XML");
|
|
}
|
|
|
|
private void CleanupDestinationFolder(string pattern)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo("fmt_xml");
|
|
FileInfo[] fis = di.GetFiles(pattern);
|
|
foreach (FileInfo fi in fis) fi.Delete();
|
|
}
|
|
|
|
private void btnMergeFmtPagDoc_Click(object sender, EventArgs e)
|
|
{
|
|
ClearResults(tbResultPath.Text);
|
|
DateTime tStart = DateTime.Now;
|
|
MyStatus = "Merging...";
|
|
// get all of the generated xml format files in the fmt_xml directory and merge
|
|
// the fmt/doc/pag & subformats into one file.
|
|
DirectoryInfo di = new DirectoryInfo("fmt_xml");
|
|
//testDirectoryInfo di = new DirectoryInfo("E:\\proms.net\\exe\\fmtxml");
|
|
FileInfo[] fis = di.GetFiles("*f.xml");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// see if all three, format, doc & pag exist - if not, print error,
|
|
// if so, process
|
|
string docname = "fmt_xml\\" + fi.Name.Substring(0, fi.Name.Length - 5) + "p.xml";
|
|
string pagname = "fmt_xml\\" + fi.Name.Substring(0, fi.Name.Length - 5) + "d.xml";
|
|
if (File.Exists(docname) && File.Exists(pagname))
|
|
{
|
|
AddFileToListBox(fi);
|
|
EntireFormat ef = new EntireFormat("fmt_xml\\" + fi.Name, tbResultPath.Text);
|
|
}
|
|
else
|
|
Console.WriteLine("For {0}, page or document file does not exist.", fi.Name);
|
|
}
|
|
MyStatus = string.Format("DONE Merging Formats {0:F3} seconds", TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds);
|
|
MessageBox.Show("DONE Merging Formats - Results are in " + tbResultPath.Text + @"fmtall'");
|
|
}
|
|
|
|
private void ClearResults(string path)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo(path);
|
|
if (di.Exists == false) return;
|
|
di = di.GetDirectories("fmtall")[0];
|
|
FileInfo[] fis = di.GetFiles("*.xml");
|
|
foreach (FileInfo fi in fis)
|
|
fi.Delete();
|
|
}
|
|
private void btnBrowseFmt_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbFmtPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
if (Directory.Exists(fbd.SelectedPath)) tbFmtPath.Text = fbd.SelectedPath;
|
|
}
|
|
|
|
private void btnBrowseGenmac_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbFmtPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
{
|
|
if (Directory.Exists(fbd.SelectedPath)) tbGenmacPath.Text = fbd.SelectedPath;
|
|
}
|
|
}
|
|
|
|
private void btnBrowseResult_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbResultPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
{
|
|
if (Directory.Exists(fbd.SelectedPath)) tbResultPath.Text = fbd.SelectedPath;
|
|
}
|
|
}
|
|
private void btnRtfToSvg_Click(object sender, EventArgs e)
|
|
{
|
|
// delete current svg's
|
|
DirectoryInfo disvg = new DirectoryInfo(tbResultPath.Text + @"\genmacall"); //(@"C:\development\proms.net\genmac.xml");
|
|
FileInfo[] fissvg = disvg.GetFiles("*.svg");
|
|
foreach (FileInfo fi in fissvg) fi.Delete();
|
|
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text);
|
|
FileInfo[] fis = di.GetFiles("*.rtf");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
|
|
// results go to e:\proms.net\genmac.xml
|
|
AddFileToListBox(fi);
|
|
RtfToSvg myConvert = new RtfToSvg(fi);
|
|
if (myConvert.IsGenMac)
|
|
myConvert.Save(tbResultPath.Text + @"genmacall\" + fi.Name.ToLower().Replace(".rtf", ".svg"));
|
|
}
|
|
}
|
|
MessageBox.Show("DONE converting GenMac RTF files to drawing svg - Results are in " + tbResultPath.Text + @"genmacall");
|
|
}
|
|
// old code:
|
|
//private void button5_Click(object sender, System.EventArgs e)
|
|
//{
|
|
|
|
// DirectoryInfo di = new DirectoryInfo("C:\\16bit\\ve-proms.net\\genmac.xml");
|
|
// FileInfo[] fis = di.GetFiles("*.xml");
|
|
// listBox1.Items.Clear();
|
|
// foreach (FileInfo fi in fis)
|
|
// {
|
|
// this.listBox1.Items.Add(fi.Name);
|
|
// //XmlToPdf pdf = new XmlToPdf("C:\\16bit\\ve-proms.net\\genmac.xml",fi.Name);
|
|
// }
|
|
// MessageBox.Show("DONE generating PDF files from XML");
|
|
//}
|
|
|
|
//private void button6_Click(object sender, System.EventArgs e)
|
|
//{
|
|
// DirectoryInfo di = new DirectoryInfo("C:\\16bit\\proms.net\\genmac.xml");
|
|
// FileInfo[] fis = di.GetFiles("*.svg");
|
|
// listBox1.Items.Clear();
|
|
// foreach (FileInfo fi in fis)
|
|
// {
|
|
// this.listBox1.Items.Add(fi.Name);
|
|
// //SvgToPdf pdf = new SvgToPdf("C:\\16bit\\proms.net\\genmac.xml", fi.Name);
|
|
// }
|
|
// MessageBox.Show("DONE generating PDF files from SVG");
|
|
//}
|
|
|
|
}
|
|
}
|