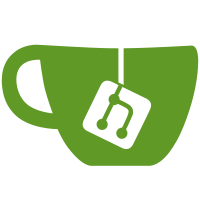
Revised user interface and added improvements to overall process Added code to change Batch Refresh to Administrative Tools
2879 lines
103 KiB
C#
2879 lines
103 KiB
C#
using System;
|
||
using System.Collections;
|
||
using System.Collections.Generic;
|
||
using System.Collections.Specialized;
|
||
using System.ComponentModel;
|
||
using System.Data;
|
||
using System.Drawing;
|
||
using System.Text;
|
||
using System.Windows.Forms;
|
||
using System.IO;
|
||
using System.Configuration;
|
||
using VEPROMS.CSLA.Library;
|
||
//using Csla;
|
||
using DevComponents;
|
||
using DevComponents.DotNetBar;
|
||
using DevComponents.DotNetBar.Rendering;
|
||
using VEPROMS.Properties;
|
||
using Volian.Controls.Library;
|
||
using DescriptiveEnum;
|
||
using Volian.Base.Library;
|
||
using Volian.Print.Library;
|
||
|
||
[assembly: log4net.Config.XmlConfigurator(Watch = true)]
|
||
|
||
namespace VEPROMS
|
||
{
|
||
enum PropPgStyle { Button = 1, Tab = 2, Grid = 3 };
|
||
|
||
public partial class frmVEPROMS : DevComponents.DotNetBar.Office2007RibbonForm
|
||
{
|
||
#region Log4Net
|
||
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
||
#endregion
|
||
#region PropertiesVariables
|
||
private int securityRole;
|
||
private bool _panelExpandedChanging = false;
|
||
Color _CommentTitleBckColor;
|
||
DocVersionInfo _SelectedDVI = null;
|
||
StepTabPanel _SelectedStepTabPanel = null;
|
||
public FindReplace dlgFindReplace = null;
|
||
public VlnSpellCheck SpellChecker = null;
|
||
|
||
public StepTabPanel SelectedStepTabPanel
|
||
{
|
||
get
|
||
{
|
||
return _SelectedStepTabPanel;
|
||
}
|
||
set
|
||
{
|
||
_SelectedStepTabPanel = value;
|
||
if (value == null) // DSO Tab Panel
|
||
{
|
||
dlgFindReplace.Visible = false; // Find/Replace dialog should not be visable for DSO tab panels
|
||
if (tc.SelectedDisplayTabItem != null && tc.SelectedDisplayTabItem.MyItemInfo != null) // 2nd part is for unassociated libdocs
|
||
SelectedDVI = tc.SelectedDisplayTabItem.MyItemInfo.MyDocVersion;
|
||
else
|
||
SelectedDVI = null;
|
||
}
|
||
else // Step Tab Panel
|
||
{
|
||
// The following line was broken into separate lines because a NullReferenceError was occuring.
|
||
// Now we should be able to tell what is wrong by which line causes the Null Reference Error
|
||
// RHM 20101217
|
||
//SelectedDVI = value.MyStepPanel.MyProcedureItemInfo.MyProcedure.ActiveParent as DocVersionInfo;
|
||
StepPanel stepPanel = value.MyStepPanel;
|
||
ItemInfo itemInfo = stepPanel.MyProcedureItemInfo;
|
||
ProcedureInfo procedureInfo = itemInfo.MyProcedure;
|
||
SelectedDVI = procedureInfo.MyDocVersion;
|
||
// Remove it first, if it wasn't set, this doesn't do anything, but if it was set, you don't want
|
||
// multiple events because the print dialog will be displayed for each time the event was added.
|
||
_SelectedStepTabPanel.MyStepTabRibbon.PrintRequest -= new StepTabRibbonEvent(MyStepTabRibbon_PrintRequest);
|
||
_SelectedStepTabPanel.MyStepTabRibbon.PrintRequest += new StepTabRibbonEvent(MyStepTabRibbon_PrintRequest);
|
||
_SelectedStepTabPanel.MyStepTabRibbon.ProgressBar = bottomProgBar;
|
||
}
|
||
}
|
||
}
|
||
|
||
public DocVersionInfo SelectedDVI
|
||
{
|
||
get { return _SelectedDVI; }
|
||
set
|
||
{
|
||
if (_SelectedDVI != value)
|
||
{
|
||
_SelectedDVI = value;
|
||
_SelectedROFst = null;
|
||
}
|
||
InitiateSearch(false);
|
||
InitiateDisplayReports(false);
|
||
}
|
||
}
|
||
// TODO: Should the following be an info
|
||
// For the initial release, we are assuming there will be only one rofst fro a docversion. Changes
|
||
// will be needed here if more than 1.
|
||
private ROFstInfo _SelectedROFst;
|
||
public ROFstInfo SelectedROFst
|
||
{
|
||
get
|
||
{
|
||
if (_SelectedROFst == null && SelectedDVI != null)
|
||
{
|
||
if (SelectedDVI.DocVersionAssociationCount <= 0)
|
||
{
|
||
MessageBox.Show("There is no Referenced Object (RO) data for this procedure set. Use the Properties for the set to define the RO data.");
|
||
return null;
|
||
}
|
||
_SelectedROFst = SelectedDVI.DocVersionAssociations[0].MyROFst;
|
||
}
|
||
return _SelectedROFst;
|
||
}
|
||
set { _SelectedROFst = value; }
|
||
}
|
||
#endregion
|
||
public frmVEPROMS()
|
||
{
|
||
// The following Try/Catch was added to protect against a problem seen by Kathy and Michelle
|
||
// on January 1, 2013. Michelle's user.config file was empty and PROMS would not run.
|
||
// This logic will delete a "Bad" config file to attempt to eliminate the problem, and
|
||
// instructs the user to try again.
|
||
try
|
||
{
|
||
if (Properties.Settings.Default.UpdateSettings)
|
||
{
|
||
Properties.Settings.Default.Upgrade();
|
||
Properties.Settings.Default.UpdateSettings = false;
|
||
Properties.Settings.Default.Save();
|
||
}
|
||
}
|
||
catch (Exception ex)
|
||
{
|
||
_MyLog.Error(ex.GetType().Name + " - " + ex.InnerException, ex);
|
||
if (ex.Message.StartsWith("Configuration system failed to initialize"))
|
||
{
|
||
string filename = ex.InnerException.Message;
|
||
filename = filename.Substring(filename.IndexOf("(") + 1);
|
||
filename = filename.Substring(0, filename.IndexOf("user.config") + 11);
|
||
FileInfo myfile = new FileInfo(filename);
|
||
myfile.Delete();
|
||
MessageBox.Show("Config file was corrupt, it has been deleted.\r\nTry Again", "Corrupt config file", MessageBoxButtons.OK, MessageBoxIcon.Error);
|
||
System.Diagnostics.Process.GetCurrentProcess().Kill();
|
||
}
|
||
}
|
||
// If first time close any remaining WinWords. These will sometimes get hung in memory and cause problems.
|
||
if (System.Diagnostics.Process.GetProcessesByName(System.Diagnostics.Process.GetCurrentProcess().ProcessName).Length == 1)
|
||
Volian.MSWord.WordDoc.KillWordApps();
|
||
// cleanup from previous run:
|
||
Volian.Base.Library.TmpFile.RemoveAllTmps();
|
||
|
||
if (VlnSettings.DebugMode)
|
||
{
|
||
//use local data (for development/debug mode)
|
||
if (Environment.MachineName == "RMARK-PC")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_RMARK_DEBUG";
|
||
else if (Environment.MachineName == "WINDOWS7-RHM")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_RMARK_DEBUG";
|
||
else if (Environment.UserName.ToUpper() == "BODINE")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_BODINE_DEBUG";
|
||
else
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_LOCAL";
|
||
}
|
||
else
|
||
{
|
||
// use server data (default)
|
||
// - except for the volian laptop and Rich's Demo version where we need to use local data
|
||
if (Environment.MachineName == "RMARK-PC")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_RMARK_DEMO";
|
||
else if (Environment.MachineName == "WINDOWS7-RHM")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_RMARK_DEMO";
|
||
else if (Environment.UserName.ToUpper() == "BODINE")
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_BODINE_DEMO";
|
||
else
|
||
VEPROMS.CSLA.Library.Database.ConnectionName = "VEPROMS_LOCAL";
|
||
}
|
||
InitializeComponent();
|
||
|
||
bottomProgBar.ValueChanged += new EventHandler(bottomProgBar_ValueChanged);
|
||
|
||
// When creating an XY Plot, a System.Drawing.Graphics is needed and it requires a form. Use the main
|
||
// form.
|
||
if (VlnSettings.DebugMode)
|
||
{
|
||
MSWordToPDF.DebugStatus = 1;
|
||
MSWordToPDF.OverrideColor = Color.Red;
|
||
}
|
||
else
|
||
{
|
||
MSWordToPDF.DebugStatus = 0;
|
||
MSWordToPDF.OverrideColor = Color.Transparent;
|
||
}
|
||
MSWordToPDF.FormForPlotGraphics = this;
|
||
|
||
// set the color of the ribbon
|
||
RibbonPredefinedColorSchemes.ChangeOffice2007ColorTable((eOffice2007ColorScheme)Settings.Default.SystemColor);
|
||
|
||
// LATER: get the user setting to control what is displayed for each tree node
|
||
// The logic is in the ToString() functions in FolderExt.cs and DocVersionExt.cs
|
||
//the GetFolder(1) function will read in the frist node (using ToString() to assign node text)
|
||
|
||
// Get the saved Tree Node Diplay settings
|
||
//if (Settings.Default["UseNameOnTreeNode"])
|
||
//{
|
||
//}
|
||
//if (Settings.Default["UseTitleOnTreeNode"])
|
||
//{
|
||
//}
|
||
cmbFont.DataSource = FontFamily.Families;
|
||
cmbFont.DisplayMember = "Name";
|
||
cmbFont.SelectedIndex = -1;
|
||
string[] parameters = System.Environment.CommandLine.Split(" ".ToCharArray());
|
||
foreach (string parameter in parameters)
|
||
{
|
||
if (parameter.StartsWith("/DB="))
|
||
Database.SelectedDatabase = parameter.Substring(4);
|
||
}
|
||
if (Properties.Settings.Default["DefaultDB"] != string.Empty)
|
||
Database.LastDatabase = Properties.Settings.Default.DefaultDB;
|
||
if (!FormatInfo.HasLatestChanges())
|
||
throw new Exception("Inconsistent Formats");
|
||
if (!ItemAuditInfo.IsChangeManagerVersion())
|
||
throw new Exception("Inconsistent Data");
|
||
if ((Database.LastDatabase ?? "") != (Database.SelectedDatabase ?? ""))
|
||
{
|
||
Properties.Settings.Default.MRIList = null;
|
||
Properties.Settings.Default.DefaultDB = Database.SelectedDatabase;
|
||
Properties.Settings.Default.Save();
|
||
}
|
||
displayBookMarks.SetupBookMarks();
|
||
DateTime dtSunday = DateTime.Now.AddDays(-((int)DateTime.Now.DayOfWeek));
|
||
ChangeLogFileName("LogFileAppender", Database.ActiveDatabase + " " + dtSunday.ToString("yyyyMMdd") + " ErrorLog.txt");
|
||
_MyLog.InfoFormat("\r\nSession Beginning\r\n<========={0}=========== User: {1}/{2} Started {3} =====================>"
|
||
, Application.ProductVersion, Environment.UserDomainName,Environment.UserName, DateTime.Now.ToString("dddd MMMM d, yyyy h:mm:ss tt"));
|
||
foreach (string parameter in parameters)
|
||
{
|
||
if (parameter.ToUpper().StartsWith("/UF="))
|
||
UpdateFormats(parameter.Substring(4));
|
||
else if (parameter.ToUpper().StartsWith("/UF"))
|
||
UpdateFormats(null);
|
||
}
|
||
VETreeNode tn = VETreeNode.GetFolder(1);
|
||
tv.Nodes.Add(tn);
|
||
tv.NodePSI += new vlnTreeViewPSIEvent(tv_NodePSI);
|
||
tv.NodeOpenProperty += new vlnTreeViewPropertyEvent(tv_NodeOpenProperty);
|
||
tv.NodeSelect += new Volian.Controls.Library.vlnTreeViewEvent(tv_NodeSelect);
|
||
tv.NodeNew += new vlnTreeViewEvent(tv_NodeNew);
|
||
tv.OpenItem += new vlnTreeViewItemInfoEvent(tv_OpenItem);
|
||
tv.DeleteItemInfo += new vlnTreeViewItemInfoDeleteEvent(tv_DeleteItemInfo);
|
||
tv.InsertItemInfo += new vlnTreeViewItemInfoInsertEvent(tv_InsertItemInfo);
|
||
tv.NodeInsert += new vlnTreeViewEvent(tv_NodeInsert);
|
||
tv.PasteItemInfo += new vlnTreeViewItemInfoPasteEvent(tv_PasteItemInfo);
|
||
tv.GetChangeId += new vlnTreeViewGetChangeIdEvent(tv_GetChangeId);
|
||
tv.NodeCopy += new vlnTreeViewEvent(tv_NodeCopy);
|
||
tv.ClipboardStatus += new vlnTreeViewClipboardStatusEvent(tv_ClipboardStatus);
|
||
tv.ProgressBar = bottomProgBar;
|
||
tc.ItemPaste += new StepPanelItemPastedEvent(tc_ItemPasted);
|
||
displayHistory.HistorySelectionChanged += new DisplayHistoryEvent(displayHistory_HistorySelectionChanged);
|
||
_CommentTitleBckColor = epAnnotations.TitleStyle.BackColor1.Color;
|
||
if (!btnAnnoDetailsPushPin.Checked)
|
||
epAnnotations.Expanded = false;
|
||
infoPanel.Expanded = false;
|
||
toolsPanel.Expanded = false;
|
||
displayTags.Visible = false;
|
||
ribbonControl1.ExpandedChanged += new EventHandler(ribbonControl1_ExpandedChanged);
|
||
dlgFindReplace = new FindReplace();
|
||
SpellChecker = new VlnSpellCheck();
|
||
displaySearch1.PrintRequest += new DisplaySearchEvent(displaySearch1_PrintRequest);
|
||
displayHistory.ChronologyPrintRequest += new DisplayHistoryReportEvent(displayHistory_ChronologyPrintRequest);
|
||
displayHistory.SummaryPrintRequest += new DisplayHistoryReportEvent(displayHistory_SummaryPrintRequest);
|
||
displayHistory.AnnotationRestored += new AnnotationRestoredHandler(displayHistory_AnnotationRestored);
|
||
displayReports.PrintRequest += new DisplayReportsEvent(displayReports_PrintRequest);
|
||
this.Activated += new EventHandler(frmVEPROMS_Activated);
|
||
VlnSettings.StepTypeToolType = Settings.Default.StepTypeToolTip;
|
||
displayLibDocs.PrintRequest += new DisplayLibDocEvent(displayLibDocs_PrintRequest);
|
||
ContentInfo.InfoChanged += new ContentInfoEvent(RefreshDisplayHistory);
|
||
AnnotationInfo.InfoChanged += new AnnotationInfoEvent(RefreshDisplayHistory);
|
||
ItemInfo.InfoRestored += new ItemInfoEvent(RefreshDisplayHistory);
|
||
ItemInfo.ItemDeleted += new ItemInfoEvent(RefreshDisplayHistory);
|
||
tv.PrintProcedure += new vlnTreeViewEvent(tv_PrintProcedure);
|
||
tv.PrintAllProcedures += new vlnTreeViewEvent(tv_PrintAllProcedures);
|
||
tv.ApproveProcedure += new vlnTreeViewEvent(tv_ApproveProcedure);
|
||
tv.ApproveAllProcedures += new vlnTreeViewEvent(tv_ApproveAllProcedures);
|
||
tv.ApproveSomeProcedures += new vlnTreeViewEvent(tv_ApproveSomeProcedures);
|
||
tv.ReportAllProceduresInconsistencies += new vlnTreeViewEvent(tv_ReportAllProceduresInconsistencies);
|
||
tv.RefreshCheckedOutProcedures += new vlnTreeViewEvent(tv_RefreshCheckedOutProcedures);
|
||
tv.ProcedureCheckedOutTo += new vlnTreeViewEvent(tv_ProcedureCheckedOutTo);
|
||
tv.ViewPDF += new vlnTreeViewPdfEvent(tv_ViewPDF);
|
||
displayApplicability.ApplicabilityViewModeChanged += new DisplayApplicability.DisplayApplicabilityEvent(displayApplicability_ApplicabilityViewModeChanged);
|
||
tv.ExportImportProcedureSets += new vlnTreeViewEvent(tv_ExportImportProcedureSets);
|
||
tv.PrintTransitionReport += new vlnTreeViewEvent(tv_PrintTransitionReport);
|
||
}
|
||
|
||
void bottomProgBar_ValueChanged(object sender, EventArgs e)
|
||
{
|
||
if (bottomProgBar.Value < 10 && bottomProgBar.ColorTable != eProgressBarItemColor.Normal)
|
||
bottomProgBar.ColorTable = eProgressBarItemColor.Normal;
|
||
}
|
||
|
||
string tv_GetChangeId(object sender, vlnTreeItemInfoEventArgs args)
|
||
{
|
||
tc.HandleChangeId(args.MyItemInfo, null);
|
||
return tc.ChgId;
|
||
}
|
||
ItemInfo tv_ClipboardStatus(object sender, vlnTreeEventArgs args)
|
||
{
|
||
return tc.MyCopyStep;
|
||
}
|
||
void tv_NodeCopy(object sender, vlnTreeEventArgs args)
|
||
{
|
||
VETreeNode tn = args.Node as VETreeNode;
|
||
ItemInfo ii = tn.VEObject as ItemInfo;
|
||
tc.MyCopyStep = ii;
|
||
}
|
||
void tv_PrintTransitionReport(object sender, vlnTreeEventArgs args)
|
||
{
|
||
FolderInfo fi = null;
|
||
ProcedureInfo pi = null;
|
||
if ((args.Node as VETreeNode).VEObject is FolderInfo)
|
||
fi = (args.Node as VETreeNode).VEObject as FolderInfo;
|
||
if ((args.Node as VETreeNode).VEObject is ProcedureInfo)
|
||
pi = (args.Node as VETreeNode).VEObject as ProcedureInfo;
|
||
if (fi != null) //working with folder
|
||
{
|
||
dlgTransitionReport dlg = new dlgTransitionReport(fi);
|
||
dlg.ShowDialog(this);
|
||
}
|
||
if (pi != null) //working with procedure
|
||
{
|
||
this.Cursor = Cursors.WaitCursor;
|
||
Application.DoEvents();
|
||
dlgTransitionReport dlg = new dlgTransitionReport(pi);
|
||
dlg.ShowDialog(this);
|
||
this.Cursor = Cursors.Default;
|
||
}
|
||
}
|
||
|
||
void tv_ExportImportProcedureSets(object sender, vlnTreeEventArgs args)
|
||
{
|
||
FolderInfo fi = null;
|
||
DocVersionInfo dvi = null;
|
||
ProcedureInfo pi = null;
|
||
if ((args.Node as VETreeNode).VEObject is FolderInfo)
|
||
fi = (args.Node as VETreeNode).VEObject as FolderInfo;
|
||
if ((args.Node as VETreeNode).VEObject is DocVersionInfo)
|
||
dvi = (args.Node as VETreeNode).VEObject as DocVersionInfo;
|
||
if ((args.Node as VETreeNode).VEObject is ProcedureInfo)
|
||
pi = (args.Node as VETreeNode).VEObject as ProcedureInfo;
|
||
if (fi != null)
|
||
{
|
||
string msg = string.Empty;
|
||
bool ok = MySessionInfo.CanCheckOutItem(fi.FolderID, CheckOutType.Folder, ref msg);
|
||
if (!ok)
|
||
{
|
||
if (args.Index == 0)
|
||
MessageBox.Show(this, msg, "Export Procedure Set Unavailable");
|
||
else
|
||
MessageBox.Show(this, msg, "Import Procedure Set Unavailable");
|
||
}
|
||
else
|
||
{
|
||
int ownerid = MySessionInfo.CheckOutItem(fi.FolderID, CheckOutType.Folder);
|
||
dlgExportImport dlg = new dlgExportImport(args.Index == 0 ? "Export" : "Import", fi);
|
||
dlg.ShowDialog(this);
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
if (args.Index == 1 && dlg.MyNewFolder != null)
|
||
tv.AddNewNode(dlg.MyNewFolder);
|
||
}
|
||
}
|
||
if (dvi != null)
|
||
{
|
||
string msg = string.Empty;
|
||
bool ok = MySessionInfo.CanCheckOutItem(dvi.VersionID, CheckOutType.DocVersion, ref msg);
|
||
if (!ok)
|
||
{
|
||
MessageBox.Show(this, msg, "Import Procedure Unavailable");
|
||
}
|
||
else
|
||
{
|
||
int ownerid = MySessionInfo.CheckOutItem(dvi.VersionID, CheckOutType.DocVersion);
|
||
dlgExportImport dlg = new dlgExportImport("Import", dvi);
|
||
dlg.ShowDialog(this);
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
if(dlg.MyNewProcedure != null)
|
||
tv.AddNewNode(dlg.MyNewProcedure);
|
||
}
|
||
}
|
||
if (pi != null)
|
||
{
|
||
string msg = string.Empty;
|
||
bool ok = MySessionInfo.CanCheckOutItem(pi.ItemID, CheckOutType.Procedure, ref msg);
|
||
if (!ok)
|
||
{
|
||
MessageBox.Show(this, msg, "Export Procedure Unavailable");
|
||
}
|
||
else
|
||
{
|
||
int ownerid = MySessionInfo.CheckOutItem(pi.ItemID, CheckOutType.Procedure);
|
||
dlgExportImport dlg = new dlgExportImport("Export", pi);
|
||
dlg.ShowDialog(this);
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
}
|
||
}
|
||
}
|
||
|
||
private void MakeDatabaseChanges()
|
||
{
|
||
// September 2012: Decided to store roimages as zipped. Any previous data may not have them zipped.
|
||
// Check the top folders config to see, and if needed, zip them:
|
||
ROImageInfo.CompressAllExistingImages += new ROImageInfoCompressionEvent(ROImageInfo_CompressAllExistingImages);
|
||
ROImageInfo.ZipImages();
|
||
ROImageInfo.CompressAllExistingImages -= new ROImageInfoCompressionEvent(ROImageInfo_CompressAllExistingImages);
|
||
|
||
}
|
||
|
||
void ROImageInfo_CompressAllExistingImages(object sender, ROImageInfoCompressionEventArgs args)
|
||
{
|
||
switch (args.Type)
|
||
{
|
||
case ROImageCompressionEventType.Complete:
|
||
ProgBarText = "Compressing Complete";
|
||
break;
|
||
case ROImageCompressionEventType.Initialize:
|
||
ProgBarMax = args.Total;
|
||
ProgBarText = "Compressing RO Images";
|
||
break;
|
||
case ROImageCompressionEventType.Update:
|
||
ProgBarValue = args.Current;
|
||
ProgBarText = args.FileName;
|
||
break;
|
||
default:
|
||
break;
|
||
}
|
||
}
|
||
|
||
static string _ErrorLogFileName;
|
||
|
||
public static string ErrorLogFileName
|
||
{
|
||
get { return frmVEPROMS._ErrorLogFileName; }
|
||
set { frmVEPROMS._ErrorLogFileName = value; }
|
||
}
|
||
static bool ChangeLogFileName(string AppenderName, string NewFilename)
|
||
{
|
||
log4net.Repository.ILoggerRepository RootRep;
|
||
RootRep = log4net.LogManager.GetRepository();
|
||
foreach (log4net.Appender.IAppender iApp in RootRep.GetAppenders())
|
||
{
|
||
if (iApp.Name.CompareTo(AppenderName) == 0
|
||
&& iApp is log4net.Appender.FileAppender)
|
||
{
|
||
log4net.Appender.FileAppender fApp = (log4net.Appender.FileAppender)iApp;
|
||
string folderPath = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
|
||
fApp.File = folderPath + @"\VEPROMS\" + NewFilename;
|
||
ErrorLogFileName = fApp.File;
|
||
fApp.ActivateOptions();
|
||
return true; // Appender found and name changed to NewFilename
|
||
}
|
||
}
|
||
return false;
|
||
}
|
||
|
||
void displayApplicability_ApplicabilityViewModeChanged(object sender, EventArgs args)
|
||
{
|
||
if (tc.SelectedDisplayTabItem == null) return;
|
||
StepPanel pnl = tc.SelectedDisplayTabItem.MyStepTabPanel.MyStepPanel;
|
||
if (pnl != null)
|
||
{
|
||
pnl.ApplDisplayMode = displayApplicability.ViewMode;
|
||
displayHistory.ApplDisplayMode = pnl.ApplDisplayMode;
|
||
//added by jcb 20130718 to support enabling/diabling create pdf button until user selects a unit
|
||
if (pnl.ApplDisplayMode > 0)
|
||
{
|
||
pnl.MyStepTabPanel.MyStepTabRibbon.btnPdfCreate.Enabled = true;
|
||
btnPrint.Visible = true;
|
||
}
|
||
else
|
||
{
|
||
pnl.MyStepTabPanel.MyStepTabRibbon.btnPdfCreate.Enabled = false;
|
||
btnPrint.Visible = false;
|
||
}
|
||
//end added by jcb 20130718
|
||
}
|
||
}
|
||
|
||
void tv_ViewPDF(object sender, vlnTreeViewPdfArgs args)
|
||
{
|
||
byte[] buffer;
|
||
if (args.MyWatermark != string.Empty)
|
||
buffer = PromsPrinter.WatermarkPDF(args.MyBuffer, args.MyWatermark);
|
||
else
|
||
buffer = args.MyBuffer;
|
||
string fileName = Volian.Base.Library.VlnSettings.TemporaryFolder + "\\" + args.MyFilename;
|
||
try
|
||
{
|
||
FileStream fs = new FileStream(fileName, FileMode.Create);
|
||
fs.Write(buffer, 0, buffer.Length);
|
||
fs.Close();
|
||
System.Diagnostics.Process.Start(fileName);
|
||
}
|
||
catch (Exception ex)
|
||
{
|
||
StringBuilder sb = new StringBuilder();
|
||
sb.AppendLine("Could not create");
|
||
sb.AppendLine();
|
||
sb.AppendLine(fileName + ".");
|
||
sb.AppendLine();
|
||
sb.AppendLine("If it is open, close and retry.");
|
||
MessageBox.Show(sb.ToString(), "Error on CreatePdf",MessageBoxButtons.OK,MessageBoxIcon.Warning);
|
||
// MessageBox.Show("Could not create " + fileName + ". If it is open, close and retry.", "Error on CreatePdf");
|
||
}
|
||
}
|
||
|
||
void displayHistory_AnnotationRestored(AnnotationInfo restoredAnnotationInfo, ItemInfo currentItem)
|
||
{
|
||
ctrlAnnotationDetails.UpdateAnnotationGrid(currentItem);
|
||
}
|
||
|
||
void tv_ReportAllProceduresInconsistencies(object sender, vlnTreeEventArgs args)
|
||
{
|
||
DocVersionInfo dvi = (args.Node as VETreeNode).VEObject as DocVersionInfo;
|
||
if (dvi == null) return;
|
||
this.Cursor = Cursors.WaitCursor;
|
||
ItemInfoList iil = ItemInfoList.GetAllInconsistencies(dvi.VersionID);
|
||
Volian.Print.Library.PDFConsistencyCheckReport rpt = new Volian.Print.Library.PDFConsistencyCheckReport(Volian.Base.Library.VlnSettings.TemporaryFolder + @"\AllInconsistencies.pdf", iil);
|
||
rpt.BuildAllReport(dvi);
|
||
this.Cursor = Cursors.Default;
|
||
}
|
||
|
||
void tv_ApproveSomeProcedures(object sender, vlnTreeEventArgs args)
|
||
{
|
||
DocVersionInfo dvi = (args.Node as VETreeNode).VEObject as DocVersionInfo;
|
||
if (dvi == null) return;
|
||
tc.SaveCurrentEditItem();
|
||
string message = string.Empty;
|
||
if (!MySessionInfo.CanCheckOutItem(dvi.VersionID, CheckOutType.DocVersion, ref message))
|
||
{
|
||
MessageBox.Show(this, message, "Working Draft Has Items Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
||
return;
|
||
}
|
||
int ownerid = MySessionInfo.CheckOutItem(dvi.VersionID, CheckOutType.DocVersion);
|
||
dvi.DocVersionConfig.SelectedSlave = args.UnitIndex;
|
||
dlgApproveProcedure dlg = new dlgApproveProcedure(dvi, true);
|
||
dlg.MySessionInfo = MySessionInfo;
|
||
dlg.ShowDialog(this);
|
||
displayHistory.RefreshList();
|
||
dvi.DocVersionConfig.SelectedSlave = 0;
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
}
|
||
|
||
void tv_ApproveAllProcedures(object sender, vlnTreeEventArgs args)
|
||
{
|
||
DocVersionInfo dvi = (args.Node as VETreeNode).VEObject as DocVersionInfo;
|
||
if (dvi == null) return;
|
||
tc.SaveCurrentEditItem();
|
||
string message = string.Empty;
|
||
if (!MySessionInfo.CanCheckOutItem(dvi.VersionID, CheckOutType.DocVersion, ref message))
|
||
{
|
||
MessageBox.Show(this, message, "Working Draft Has Items Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
||
return;
|
||
}
|
||
int ownerid = MySessionInfo.CheckOutItem(dvi.VersionID, CheckOutType.DocVersion);
|
||
dvi.DocVersionConfig.SelectedSlave = args.UnitIndex;
|
||
dlgApproveProcedure dlg = new dlgApproveProcedure(dvi);
|
||
dlg.MySessionInfo = MySessionInfo;
|
||
dlg.ShowDialog(this);
|
||
displayHistory.RefreshList();
|
||
dvi.DocVersionConfig.SelectedSlave = 0;
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
}
|
||
|
||
void tv_RefreshCheckedOutProcedures(object sender, vlnTreeEventArgs args)
|
||
{
|
||
OwnerInfoList.Reset();
|
||
OwnerInfoList oil = OwnerInfoList.Get();
|
||
Dictionary<int, int> dicProcCheckedOut = new Dictionary<int, int>();
|
||
Dictionary<int, int> dicDocCheckedOut = new Dictionary<int, int>();
|
||
foreach (OwnerInfo oi in oil)
|
||
{
|
||
if (oi.SessionID != MySessionInfo.SessionID && oi.OwnerType == (byte)CheckOutType.Procedure)
|
||
dicProcCheckedOut.Add(oi.OwnerItemID, oi.OwnerID);
|
||
else if (oi.SessionID != MySessionInfo.SessionID && oi.OwnerType == (byte)CheckOutType.Document)
|
||
dicDocCheckedOut.Add(oi.OwnerItemID, oi.OwnerID);
|
||
}
|
||
if (args.Node.IsExpanded)
|
||
{
|
||
foreach (TreeNode tn in args.Node.Nodes)
|
||
{
|
||
ProcedureInfo pi = (tn as VETreeNode).VEObject as ProcedureInfo;
|
||
if (pi != null && dicProcCheckedOut.ContainsKey(pi.ItemID))
|
||
tn.ForeColor = Color.Red;
|
||
else
|
||
tn.ForeColor = Color.Black;
|
||
bool expanded = tn.IsExpanded;
|
||
if (!expanded)
|
||
tn.Expand();
|
||
foreach (TreeNode tnn in tn.Nodes)
|
||
{
|
||
SectionInfo si = (tnn as VETreeNode).VEObject as SectionInfo;
|
||
if (si != null && si.MyContent.MyEntry != null)
|
||
{
|
||
if (dicDocCheckedOut.ContainsKey(si.MyContent.MyEntry.DocID))
|
||
tnn.ForeColor = Color.Red;
|
||
else
|
||
tnn.ForeColor = Color.Black;
|
||
}
|
||
}
|
||
if (!expanded)
|
||
tn.Collapse();
|
||
}
|
||
}
|
||
}
|
||
|
||
void tv_ProcedureCheckedOutTo(object sender, vlnTreeEventArgs args)
|
||
{
|
||
ProcedureInfo pi = null;
|
||
SectionInfo si = null;
|
||
pi = (args.Node as VETreeNode).VEObject as ProcedureInfo;
|
||
if(pi == null)
|
||
si = (args.Node as VETreeNode).VEObject as SectionInfo;
|
||
UserInfo ui = UserInfo.GetByUserID(MySessionInfo.UserID);
|
||
dlgCheckedOutProcedure cop = new dlgCheckedOutProcedure(pi, si, ui);
|
||
cop.ShowDialog(this);
|
||
tv_RefreshCheckedOutProcedures(sender, new vlnTreeEventArgs(args.Node.Parent, null, 0));
|
||
}
|
||
|
||
void tv_ApproveProcedure(object sender, vlnTreeEventArgs args)
|
||
{
|
||
ProcedureInfo pi = (args.Node as VETreeNode).VEObject as ProcedureInfo;
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = args.UnitIndex;
|
||
if (pi == null) return;
|
||
tc.SaveCurrentEditItem(pi);
|
||
string message = string.Empty;
|
||
if (!MySessionInfo.CanCheckOutItem(pi.ItemID, CheckOutType.Procedure, ref message))
|
||
{
|
||
MessageBox.Show(this, message, "Procedure Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
||
return;
|
||
}
|
||
int ownerid = MySessionInfo.CheckOutItem(pi.ItemID, 0);
|
||
dlgApproveProcedure dlg = new dlgApproveProcedure(pi);
|
||
dlg.MySessionInfo = MySessionInfo;
|
||
dlg.ShowDialog(this);
|
||
displayHistory.RefreshList();
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = 0;
|
||
MySessionInfo.CheckInItem(ownerid);
|
||
}
|
||
|
||
void tv_PrintAllProcedures(object sender, vlnTreeEventArgs args)
|
||
{
|
||
DocVersionInfo dvi = (args.Node as VETreeNode).VEObject as DocVersionInfo;
|
||
if (dvi == null) return;
|
||
tc.SaveCurrentEditItem();
|
||
DlgPrintProcedure prnDlg = new DlgPrintProcedure(dvi);
|
||
prnDlg.MySessionInfo = MySessionInfo;
|
||
prnDlg.SelectedSlave = args.UnitIndex;
|
||
prnDlg.ShowDialog(this); // RHM 20120925 - Center dialog over PROMS window
|
||
}
|
||
void tv_PrintProcedure(object sender, vlnTreeEventArgs args)
|
||
{
|
||
ProcedureInfo pi = (args.Node as VETreeNode).VEObject as ProcedureInfo;
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = args.UnitIndex;
|
||
if (pi == null) return;
|
||
tc.SaveCurrentEditItem(pi);
|
||
DlgPrintProcedure prnDlg = new DlgPrintProcedure(pi);
|
||
prnDlg.MySessionInfo = MySessionInfo;
|
||
prnDlg.SelectedSlave = args.UnitIndex;
|
||
// prnDlg.Show(this); // RHM 20120925 - Center dialog over PROMS window
|
||
prnDlg.ShowDialog(this); // RHM 20120925 - Center dialog over PROMS window
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = 0;
|
||
}
|
||
void RefreshDisplayHistory(object sender)
|
||
{
|
||
displayHistory.RefreshChangeList();
|
||
}
|
||
void displayHistory_HistorySelectionChanged(object sender, DisplayHistoryEventArgs args)
|
||
{
|
||
tc.OpenItem(ItemInfo.Get(args.ItemID));
|
||
}
|
||
void displayHistory_SummaryPrintRequest(object sender, DisplayHistoryReportEventArgs args)
|
||
{
|
||
Volian.Print.Library.PDFChronologyReport myChronoRpt = new Volian.Print.Library.PDFChronologyReport(args.ReportTitle, args.ProcedureInfo, args.AuditList, args.AnnotationList);
|
||
myChronoRpt.BuildSummary();
|
||
}
|
||
void displayHistory_ChronologyPrintRequest(object sender, DisplayHistoryReportEventArgs args)
|
||
{
|
||
Volian.Print.Library.PDFChronologyReport myChronoRpt = new Volian.Print.Library.PDFChronologyReport(args.ReportTitle, args.ProcedureInfo, args.AuditList, args.AnnotationList);
|
||
myChronoRpt.BuildChronology();
|
||
}
|
||
DialogResult tv_NodePSI(object sender, vlnTreeEventArgs args)
|
||
{
|
||
VETreeNode vNode = (VETreeNode)args.Node;
|
||
IVEDrillDownReadOnly veObj = vNode.VEObject;
|
||
ProcedureInfo myProc = veObj as ProcedureInfo;
|
||
string message = string.Empty;
|
||
if (!MySessionInfo.CanCheckOutItem(myProc.ItemID, CheckOutType.Procedure, ref message))
|
||
{
|
||
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
||
return DialogResult.None;
|
||
}
|
||
int ownerID = MySessionInfo.CheckOutItem(myProc.ItemID, 0);
|
||
frmPSI fpsi = new frmPSI(myProc);
|
||
DialogResult dr = fpsi.ShowDialog(this);
|
||
MySessionInfo.CheckInItem(ownerID);
|
||
return dr;
|
||
}
|
||
/// <summary>
|
||
/// Activate tmrTreeView so that the newly created Step recieves focus
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="args"></param>
|
||
void tv_NodeInsert(object sender, vlnTreeEventArgs args)
|
||
{
|
||
tmrTreeView.Enabled = true;
|
||
}
|
||
void frmVEPROMS_Activated(object sender, EventArgs e)
|
||
{
|
||
if (ActiveControl == tc) tc.HideCaret();
|
||
// refresh anything that pertains to external files or programs:
|
||
|
||
// if ro.fst was 'updated' from the roeditor, enable the update ro.fst values on
|
||
// the steptabribbon.
|
||
if (SelectedStepTabPanel!=null && SelectedStepTabPanel.MyStepTabRibbon != null)
|
||
SelectedStepTabPanel.MyStepTabRibbon.SetUpdRoValBtn(SelectedStepTabPanel.MyStepTabRibbon.NewerRoFst());
|
||
}
|
||
void MyStepTabRibbon_PrintRequest(object sender, StepTabRibbonEventArgs args)
|
||
{
|
||
ProcedureInfo pi = args.Proc as ProcedureInfo;
|
||
//added by jcb 20130718 to support create pdf button when multiunit and user selects a unit
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = pi.ProcedureConfig.SelectedSlave;
|
||
//end added by jcb 20130718
|
||
if (pi == null) return;
|
||
DlgPrintProcedure prnDlg = new DlgPrintProcedure(pi);
|
||
//added by jcb 20130718 to support create pdf button when multiunit and user selects a unit
|
||
prnDlg.SelectedSlave = pi.ProcedureConfig.SelectedSlave == 0 ? -1 : pi.ProcedureConfig.SelectedSlave;
|
||
prnDlg.MySessionInfo = MySessionInfo;
|
||
//end added by jcb 20130718
|
||
prnDlg.ShowDialog(this); // RHM 20120925 - Center dialog over PROMS window
|
||
//added by jcb 20130718 to support create pdf button when multiunit and user selects a unit
|
||
pi.MyDocVersion.DocVersionConfig.SelectedSlave = 0;
|
||
//end added by jcb 20130718
|
||
}
|
||
void displaySearch1_PrintRequest(object sender, DisplaySearchEventArgs args)
|
||
{
|
||
Volian.Print.Library.PDFReport myReport = new Volian.Print.Library.PDFReport(args.ReportTitle, args.TypesSelected, args.MyItemInfoList,
|
||
Volian.Base.Library.VlnSettings.TemporaryFolder + @"\searchresults.pdf");
|
||
if (args.SearchString != null)
|
||
myReport.SearchString = args.SearchString;
|
||
myReport.Build();
|
||
}
|
||
|
||
void displayLibDocs_PrintRequest(object sender, DisplayLibDocEventArgs args)
|
||
{
|
||
Volian.Print.Library.PDFReport myReport = new Volian.Print.Library.PDFReport(args.ReportTitle, args.LibDocList, Volian.Base.Library.VlnSettings.TemporaryFolder + @"\LibDocUsage.pdf");
|
||
myReport.Build();
|
||
}
|
||
|
||
void displayReports_PrintRequest(object sender, DisplayReportsEventArgs args)
|
||
{
|
||
if (args.TypesSelected == "RO Usage")
|
||
{
|
||
Volian.Print.Library.PDFReport myReport = new Volian.Print.Library.PDFReport(args.ReportTitle, args.MyItemInfoList, Volian.Base.Library.VlnSettings.TemporaryFolder + @"\ROUsageReport.pdf", args.SortUsageByProcedure,args.IncludeMissingROs);
|
||
myReport.Build();
|
||
}
|
||
else if (args.TypesSelected == "Complete RO Report")
|
||
{
|
||
Volian.Print.Library.PDFReport myReport = new Volian.Print.Library.PDFReport(args.ReportTitle, args.RODataFile, Volian.Base.Library.VlnSettings.TemporaryFolder + @"\CompleteROReport.pdf", args.RofstLookup, args.CompleteROReport, args.ConvertCaretToDelta, args.IncludeEmptyROFields);
|
||
myReport.Build();
|
||
}
|
||
else if (args.TypesSelected == "RO Summary Report")
|
||
{
|
||
Volian.Print.Library.PDFReport myReport = new Volian.Print.Library.PDFReport(args.ReportTitle, Volian.Base.Library.VlnSettings.TemporaryFolder + @"\ROSummaryReport.pdf", args.RofstLookup, args.ROListForReport);
|
||
myReport.Build();
|
||
|
||
}
|
||
}
|
||
|
||
|
||
bool tv_InsertItemInfo(object sender, vlnTreeItemInfoInsertEventArgs args)
|
||
{
|
||
// Don't select the newly created Step. This will be handled by tmrTreeView
|
||
return tc.InsertRTBItem(args.MyItemInfo, args.StepText, args.InsertType, args.FromType, args.Type, false);
|
||
}
|
||
private bool tv_DeleteItemInfo(object sender, vlnTreeItemInfoEventArgs args)
|
||
{
|
||
if (displayHistory.MyEditItem != null && displayHistory.MyItemInfo.MyProcedure.ItemID == args.MyItemInfo.ItemID)
|
||
displayHistory.MyEditItem = null;
|
||
return tc.DeleteRTBItem(args.MyItemInfo);
|
||
//if (si == null) return false;
|
||
//si.RemoveItem();
|
||
//return true;
|
||
}
|
||
private bool tv_PasteItemInfo(object sender, vlnTreeItemInfoPasteEventArgs args)
|
||
{
|
||
return tc.PasteRTBItem(args.MyItemInfo, args.CopyStartID, args.PasteType, (int)args.Type);
|
||
}
|
||
private void frmVEPROMS_FormClosing(object sender, FormClosingEventArgs e)
|
||
{
|
||
if (MyActivityTimer != null)MyActivityTimer.Dispose();
|
||
if(MySessionInfo != null)MySessionInfo.EndSession();
|
||
// Save the location and size of the VE-PROMS appication for this user
|
||
if (this.WindowState == FormWindowState.Normal)
|
||
{
|
||
Settings.Default.Location = this.Location;
|
||
Settings.Default.Size = this.Size;
|
||
}
|
||
Settings.Default.WindowState = this.WindowState;
|
||
Settings.Default.QATItems = ribbonControl1.QatLayout;
|
||
SaveMRU();
|
||
//Settings.Default.Save();
|
||
Volian.Base.Library.DebugPagination.Close();
|
||
Volian.Base.Library.DebugText.Close();
|
||
}
|
||
void frmVEPROMS_FormClosed(object sender, System.Windows.Forms.FormClosedEventArgs e)
|
||
{
|
||
// Close any open documents
|
||
tc.Dispose();
|
||
// RHM 20121010
|
||
// Something was causing the process to remain in memory for an extended period of time
|
||
// after printing procedures. The following lines kills the process immediately.
|
||
try
|
||
{
|
||
System.Diagnostics.Process.GetCurrentProcess().Kill();
|
||
}
|
||
catch (Exception ex)
|
||
{
|
||
_MyLog.Warn("Attempting to Close", ex);
|
||
}
|
||
}
|
||
// Get the "Procedures" Panel Heading (title).
|
||
// by default the heading is "Procedures" (handled in the getting from the config)
|
||
// the heading is stored in the "title" field of the folder config.
|
||
// we currently only use the "title" field for the top (VEPROMS) tree node.
|
||
private string getProcedurePanelHeading()
|
||
{
|
||
VETreeNode jj_vetn = (VETreeNode)tv.Nodes[0]; // the VEPROMS tree node
|
||
return ((FolderConfig)jj_vetn.VEObject.MyConfig).Title; // get the panel heading
|
||
}
|
||
|
||
//private void btnStepRTF_Click(object sender, System.EventArgs e)
|
||
//{
|
||
// if (tc.MyStepRTB != null)
|
||
// {
|
||
// frmStepRTF frm = new frmStepRTF();
|
||
|
||
// frm.MyStepRTB = tc.MyStepRTB;
|
||
// frm.Show();
|
||
// }
|
||
//}
|
||
|
||
private SessionInfo MySessionInfo;
|
||
private System.Threading.Timer MyActivityTimer;
|
||
private DevComponents.DotNetBar.ButtonItem btnManageSecurity;
|
||
private DevComponents.DotNetBar.ButtonItem btnResetSecurity;
|
||
private DevComponents.DotNetBar.ButtonItem btnBatchRefresh;
|
||
private TabItemsToClose _MyCloseTabList = new TabItemsToClose();
|
||
public TabItemsToClose MyCloseTabList
|
||
{
|
||
get { return _MyCloseTabList; }
|
||
}
|
||
private void PingSession(Object obj)
|
||
{
|
||
List<int> myList = MySessionInfo.PingSession();
|
||
foreach (DisplayTabItem dti in tc.MyBar.Items)
|
||
{
|
||
if (!myList.Contains(dti.OwnerID))
|
||
MyCloseTabList.PushDTI(dti);
|
||
}
|
||
}
|
||
public Timer tmrCloseTabItems;
|
||
|
||
private void frmVEPROMS_Load(object sender, EventArgs e)
|
||
{
|
||
InitializeSecurity();
|
||
UpdateUser();
|
||
btnManageSecurity = new ButtonItem("btnManageSecurity", "Manage Security");
|
||
btnAdmin.SubItems.Add(btnManageSecurity);
|
||
btnManageSecurity.Click += new EventHandler(btnManageSecurity_Click);
|
||
//added by jcb
|
||
//menu item to reset security
|
||
//requires password to implement
|
||
btnResetSecurity = new ButtonItem("btnResetSecurity", "Reset Security");
|
||
btnAdmin.SubItems.Add(btnResetSecurity);
|
||
btnResetSecurity.Click += new EventHandler(btnResetSecurity_Click);
|
||
//end added by jcb
|
||
//batch refresh transitions
|
||
btnBatchRefresh = new ButtonItem("btnBatchRefresh", "Administrative Tools");
|
||
btnBatchRefresh.Click += new EventHandler(btnBatchRefresh_Click);
|
||
btnAdmin.SubItems.Add(btnBatchRefresh);
|
||
//end batch refresh transitions
|
||
UserInfo ui = null;
|
||
try
|
||
{
|
||
ui = UserInfo.GetByUserID(VlnSettings.UserID);
|
||
}
|
||
catch
|
||
{
|
||
MessageBox.Show("This database is not compatible with this version of PROMS. The PROMS program will terminate. Please contact Volian to assist in resolution.");
|
||
Application.Exit();
|
||
}
|
||
if (ui == null)
|
||
{
|
||
User u = User.MakeUser(VlnSettings.UserID, "", "", "", "", "", "", "", "", "", "", DateTime.Now, VlnSettings.UserID);
|
||
Group g = Group.Get(securityRole);
|
||
Membership.MakeMembership(u, g, null, "");
|
||
ui = UserInfo.Get(u.UID);
|
||
}
|
||
ctrlAnnotationDetails.MyUserInfo = ui;
|
||
bool isVisible = ui.IsAdministrator();
|
||
btnManageSecurity.Visible = isVisible;
|
||
btnUpdateFormats.Visible = isVisible;
|
||
btnResetSecurity.Visible = isVisible;
|
||
tmrCloseTabItems = new Timer();
|
||
tmrCloseTabItems.Interval = 100;
|
||
tmrCloseTabItems.Tick += new EventHandler(tmrCloseTabItems_Tick);
|
||
tmrCloseTabItems.Enabled = true;
|
||
MySessionInfo = SessionInfo.BeginSession(Environment.MachineName, System.Diagnostics.Process.GetCurrentProcess().Id);
|
||
if (MySessionInfo == null)
|
||
{
|
||
MessageBox.Show("This database is locked by the Administrator. Please try again later", "PROMS is Locked");
|
||
Application.Exit();
|
||
}
|
||
tc.MySessionInfo = MySessionInfo;
|
||
tv.MySessionInfo = MySessionInfo;
|
||
System.Threading.AutoResetEvent autoEvent = new System.Threading.AutoResetEvent(false);
|
||
//System.Threading.TimerCallback timerDelegate = new System.Threading.TimerCallback(MySessionInfo.PingSession);
|
||
System.Threading.TimerCallback timerDelegate = new System.Threading.TimerCallback(this.PingSession);
|
||
|
||
if (!System.Diagnostics.Process.GetCurrentProcess().ProcessName.ToLower().EndsWith("vshost"))
|
||
//MyActivityTimer = new System.Threading.Timer(timerDelegate, autoEvent, 30000, 30000);
|
||
MyActivityTimer = new System.Threading.Timer(timerDelegate, autoEvent, 10000, 10000);
|
||
|
||
//string debugMode = ConfigurationManager.AppSettings["Debug"];
|
||
//VlnSettings.DebugMode = bool.Parse(debugMode); // set debug for the Volian.Controls.Library
|
||
// get the saved location and size of the VE-PROMS appication for this user
|
||
this.txtSearch.KeyPress += new KeyPressEventHandler(txtSearch_KeyPress);
|
||
if (Settings.Default["Location"] != null) this.Location = Settings.Default.Location;
|
||
if (Settings.Default["Size"] != null) this.Size = Settings.Default.Size;
|
||
if (Settings.Default["WindowState"] != null) this.WindowState = Settings.Default.WindowState;
|
||
//if (Settings.Default.SaveTreeviewExpanded) epProcedures.Expanded = Settings.Default.TreeviewExpanded;
|
||
// if the Procedures panel was left open from the last session, then open it
|
||
epProcedures.Expanded = Settings.Default.TreeviewExpanded;
|
||
if (Settings.Default["QATItems"] != null) ribbonControl1.QatLayout = Settings.Default.QATItems;
|
||
|
||
// See if any database 'changes' need done and do them:
|
||
MakeDatabaseChanges();
|
||
|
||
_MyMRIList = MostRecentItemList.GetMRILst((System.Collections.Specialized.StringCollection)(Properties.Settings.Default["MRIList"]));
|
||
_MyMRIList.AfterRemove += new ItemInfoEvent(_MyMRIList_AfterRemove);
|
||
SetupMRU();
|
||
// if the user selected to "Remember Last" (was "Save Expanded") then use the MRU list to
|
||
// expand the tree to the last opened procedure
|
||
//if (epProcedures.Expanded && _MyMRIList.Count > 0)
|
||
if (Settings.Default.SaveTreeviewExpanded && _MyMRIList.Count > 0)
|
||
{
|
||
tv.AdjustTree(_MyMRIList[0].MyItemInfo.MyProcedure);
|
||
tv.SelectedNode.Expand();
|
||
SetCaption(tv.SelectedNode as VETreeNode);
|
||
}
|
||
// Assign the Procedure Panel's title (heading)
|
||
epProcedures.TitleText = getProcedurePanelHeading();// get the panel heading
|
||
// if the procedure panel is expanded, make sure we enable the splitter so the user can resize the panel
|
||
// Bug fix: B2013-89
|
||
expandableSplitter1.Enabled = epProcedures.Expanded;
|
||
|
||
displaySearch1.SetupAnnotationSearch(ctrlAnnotationDetails, tc);
|
||
AnnotationTypeInfoList.ListChanged += new AnnotationTypeInfoListEvent(AnnotationTypeInfoList_ListChanged);
|
||
ctrlAnnotationDetails.SetupAnnotations(displaySearch1);
|
||
SetupButtons();
|
||
displayBookMarks.MyDisplayTabControl = tc; // allows bookmark selection to bring up steps/docs
|
||
office2007StartButton1.MouseDown +=new MouseEventHandler(office2007StartButton1_MouseDown);
|
||
//displayRO.EnabledChanged += new EventHandler(displayRO_EnabledChanged);
|
||
tc.Enter += new EventHandler(tc_Enter);
|
||
tc.Leave += new EventHandler(tc_Leave);
|
||
tc.StatusChanged += new DisplayTabControlStatusEvent(tc_StatusChanged);
|
||
tc.ToggleRibbonExpanded += new DisplayTabControlEvent(tc_ToggleRibbonExpanded);
|
||
this.Deactivate += new EventHandler(frmVEPROMS_Deactivate);
|
||
if (VlnSettings.DemoMode) StepRTB.MyFontFamily = GetFamily("Bookman Old Style");
|
||
displaySearch1.Enter += new EventHandler(displaySearch1_Enter);
|
||
displayHistory.Enter += new EventHandler(displayHistory_Enter);
|
||
ctrlAnnotationDetails.Enter += new EventHandler(ctrlAnnotationDetails_Enter);
|
||
Application.DoEvents();
|
||
if (RunningNewRevision && ShowEULA() != DialogResult.OK)
|
||
{
|
||
Timer tmrShutDown = new Timer();
|
||
tmrShutDown.Interval = 250;
|
||
tmrShutDown.Tick += new EventHandler(tmrShutDown_Tick);
|
||
tmrShutDown.Enabled = true;
|
||
}
|
||
else
|
||
tmrAutomatic.Enabled = true;
|
||
//// Shutoff UpdateFormats for Production Mode
|
||
//if (Volian.Base.Library.VlnSettings.ProductionMode)
|
||
// btnAdmin.Visible = false;
|
||
StepTabRibbon.PasteNoReturnsSetting = Properties.Settings.Default.PasteNoReturns;
|
||
StepTabRibbon.PastePlainTextSetting = Properties.Settings.Default.PastePlainText;
|
||
Activate();
|
||
//FolderInfo fi = FolderInfo.Get(1);
|
||
//FormatInfo frmI = FormatInfo.Get(fi.FormatID ?? 1);
|
||
//if (frmI.PlantFormat.FormatData.ProcData.ChangeBarData.ChangeIds)
|
||
//{
|
||
// dlgChgId dlgCI = new dlgChgId(tc);
|
||
// dlgCI.ShowDialog(this);
|
||
//}
|
||
}
|
||
|
||
void txtSearch_KeyPress(object sender, KeyPressEventArgs e)
|
||
{
|
||
if (e.KeyChar == '\r')
|
||
{
|
||
e.Handled = true;
|
||
ItemInfo ii = null;
|
||
ContentInfo ci = null;
|
||
if (txtSearch.Text.Length > 0)
|
||
{
|
||
int id = 0;
|
||
if (txtSearch.Text.ToUpper().StartsWith("C="))
|
||
{
|
||
if (int.TryParse(txtSearch.Text.Substring(2), out id))
|
||
ci = ContentInfo.Get(id);
|
||
if (ci != null)
|
||
ii = ci.ContentItems[0];
|
||
}
|
||
else
|
||
{
|
||
if (int.TryParse(txtSearch.Text, out id))
|
||
ii = ItemInfo.Get(id);
|
||
}
|
||
if (ii != null)
|
||
{
|
||
tc.OpenItem(ii);
|
||
tv.AdjustTree(ii);
|
||
}
|
||
else
|
||
MessageBox.Show("No item found");
|
||
}
|
||
}
|
||
}
|
||
|
||
void btnBatchRefresh_Click(object sender, EventArgs e)
|
||
{
|
||
frmBatchRefresh frm = new frmBatchRefresh();
|
||
frm.ProgressBar = bottomProgBar;
|
||
frm.MySessionInfo = MySessionInfo;
|
||
frm.ShowDialog(this);
|
||
}
|
||
|
||
void tmrCloseTabItems_Tick(object sender, EventArgs e)
|
||
{
|
||
tmrCloseTabItems.Enabled = false;
|
||
while (MyCloseTabList.CountDTI > 0)
|
||
{
|
||
DisplayTabItem dti = MyCloseTabList.PopDTI();
|
||
if (dti.MyDSOTabPanel != null)
|
||
dti.MyDSOTabPanel.OverrideClose = true;
|
||
tc.CloseTabItem(dti);
|
||
}
|
||
}
|
||
|
||
private void InitializeSecurity()
|
||
{
|
||
Folder f = Folder.Get(1);
|
||
GroupInfo gi = GroupInfo.Get(1);
|
||
if (f.FolderConfig.Security_Group == 0)
|
||
{
|
||
f.FolderConfig.Security_Group = gi.GID;
|
||
f.Save();
|
||
}
|
||
securityRole = f.FolderConfig.Security_Group;
|
||
}
|
||
|
||
private string proxyUser;
|
||
private void UpdateUser()
|
||
{
|
||
string newUser = VlnSettings.GetCommand("U", null);
|
||
if (newUser != null)
|
||
VlnSettings.UserID = newUser;
|
||
newUser = VlnSettings.GetCommand("V3Pr0m5" + GetSecurityKey(), null);
|
||
if (newUser != null)
|
||
{
|
||
VlnSettings.UserID = newUser;
|
||
UserInfo ui = UserInfo.GetByUserID(VlnSettings.UserID);
|
||
User u;
|
||
if (ui != null)
|
||
{
|
||
u = User.Get(ui.UID);
|
||
foreach (UserMembership um in u.UserMemberships)
|
||
{
|
||
if (um.EndDate == null || um.EndDate == string.Empty)
|
||
{
|
||
Membership m = Membership.Get(um.UGID);
|
||
m.EndDate = DateTime.Now.ToShortDateString();
|
||
m.Save();
|
||
}
|
||
}
|
||
}
|
||
else
|
||
{
|
||
u = User.MakeUser(VlnSettings.UserID, "", "", "", "", "", "", "", "", "", "", DateTime.Now, VlnSettings.UserID);
|
||
Group g = Group.GetByGroupName("Administrators");
|
||
Membership.MakeMembership(u, g, null, "");
|
||
}
|
||
}
|
||
if(VlnSettings.GetCommandFlag("VeauLeeAnn" + GetSecurityKey()))
|
||
{
|
||
//pop up user list to select from
|
||
ContextMenuStrip cms = BuildUserMenu();
|
||
while (proxyUser == null)
|
||
{
|
||
cms.Show(new System.Drawing.Point((System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Width - cms.Width) / 2, (System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Height - cms.Height) / 2));
|
||
System.Windows.Forms.Application.DoEvents();
|
||
}
|
||
VlnSettings.UserID = proxyUser;
|
||
}
|
||
lblUser.Text = VlnSettings.UserID;
|
||
lblUser.MouseDown += new MouseEventHandler(lblUser_MouseDown);
|
||
}
|
||
private string GetSecurityKey()
|
||
{
|
||
Random rnd = new Random(DateTime.Now.Year + DateTime.Now.DayOfYear * 1000);
|
||
return rnd.Next(10000).ToString();
|
||
}
|
||
void lblUser_MouseDown(object sender, MouseEventArgs e)
|
||
{
|
||
if (e.Button == MouseButtons.Right)
|
||
{
|
||
/*
|
||
- Devin- Volian0
|
||
- Diane- Volian0
|
||
- Jess- Volian0
|
||
- John- Volian0
|
||
- Kathy- Volian0
|
||
- Jim- JCB2-HP
|
||
- Rich- WINDOWS7-RHM
|
||
- Paul- Paul-PC
|
||
- Michelle- Michelle-PC
|
||
- Harry<72>s Mac- WIN-04QLPEH7JKH
|
||
- Harry<72>s PC- Harry-7100
|
||
- Caitlin- Caitlin-PC
|
||
*/
|
||
if ("|VOLIAN0|JCB2-HP|WINDOWS7-RHM|PAUL-PC|MICHELLE-PC|WIN-O4QLPEH7JKH|HARRY-7100|CAITLIN-PC|".Contains("|" + Environment.UserDomainName.ToUpper() + "|"))
|
||
{
|
||
Random rnd = new Random(DateTime.Now.Year + DateTime.Now.DayOfYear * 1000);
|
||
MessageBox.Show(this, GetSecurityKey(), "Today's Security Key");
|
||
}
|
||
_MyLog.WarnFormat("Environment.UserName = '{0}'", Environment.UserName);
|
||
_MyLog.WarnFormat("Environment.UserDomainName = '{0}'", Environment.UserDomainName);
|
||
_MyLog.WarnFormat("Environment.MachineName = '{0}'", Environment.MachineName);
|
||
_MyLog.WarnFormat("Environment.OSVersion = '{0}'", Environment.OSVersion);
|
||
}
|
||
}
|
||
|
||
private ContextMenuStrip BuildUserMenu()
|
||
{
|
||
System.Windows.Forms.ContextMenuStrip cms = new System.Windows.Forms.ContextMenuStrip();
|
||
cms.Items.Add("Choose User");
|
||
System.Windows.Forms.ToolStripMenuItem tsmi = cms.Items[0] as System.Windows.Forms.ToolStripMenuItem;
|
||
tsmi.BackColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaption);// System.Drawing.Color.Pink;
|
||
tsmi.ForeColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaptionText);
|
||
tsmi.Font = new System.Drawing.Font(tsmi.Font, System.Drawing.FontStyle.Bold);
|
||
UserInfoList uil = UserInfoList.Get();
|
||
foreach (UserInfo ui in uil)
|
||
{
|
||
if (ui.UserMembershipCount > 0)
|
||
{
|
||
foreach (MembershipInfo mi in ui.UserMemberships)
|
||
{
|
||
if (mi.EndDate == string.Empty)
|
||
{
|
||
string txt = string.Format("{0} - {1}", ui.UserID, mi.MyGroup.GroupName);
|
||
ToolStripItem tsi = cms.Items.Add(txt, null, new EventHandler(User_Click));
|
||
tsi.Tag = ui;
|
||
break;
|
||
}
|
||
}
|
||
}
|
||
}
|
||
return cms;
|
||
}
|
||
|
||
private void User_Click(object sender, EventArgs e)
|
||
{
|
||
ToolStripMenuItem tsmi = sender as ToolStripMenuItem;
|
||
if (tsmi != null)
|
||
{
|
||
UserInfo ui = tsmi.Tag as UserInfo;
|
||
proxyUser = ui.UserID;
|
||
}
|
||
}
|
||
void btnManageSecurity_Click(object sender, EventArgs e)
|
||
{
|
||
dlgManageSecurity dlg = new dlgManageSecurity();
|
||
dlg.ShowDialog(this);
|
||
}
|
||
void btnResetSecurity_Click(object sender, EventArgs e)
|
||
{
|
||
string password = string.Empty;
|
||
if (ShowInputDialog(ref password) == DialogResult.OK)
|
||
{
|
||
if(password == "V3Pr0m5")
|
||
{
|
||
StringBuilder sb = new StringBuilder();
|
||
sb.AppendLine("***** WARNING *****");
|
||
sb.AppendLine();
|
||
sb.AppendLine("This action will delete all Groups, Users and Memberships from the database.");
|
||
sb.AppendLine("This action is NOT reversible.");
|
||
sb.AppendLine("Following this action the application will terminate.");
|
||
sb.AppendLine();
|
||
sb.AppendLine("Are you sure you want to continue?");
|
||
if (MessageBox.Show(sb.ToString(), "Confirm Security Reset", MessageBoxButtons.YesNoCancel, MessageBoxIcon.Stop) == DialogResult.Yes)
|
||
{
|
||
ResetSecurity.Execute();
|
||
Application.Exit();
|
||
}
|
||
}
|
||
else
|
||
MessageBox.Show("You have entered an incorrect password.");
|
||
}
|
||
}
|
||
private static DialogResult ShowInputDialog(ref string input)
|
||
{
|
||
System.Drawing.Size size = new System.Drawing.Size(200, 70);
|
||
Form inputBox = new Form();
|
||
|
||
inputBox.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
|
||
inputBox.ClientSize = size;
|
||
inputBox.Text = "Enter Password";
|
||
inputBox.StartPosition = FormStartPosition.CenterScreen;
|
||
|
||
System.Windows.Forms.TextBox textBox = new TextBox();
|
||
textBox.Size = new System.Drawing.Size(size.Width - 10, 23);
|
||
textBox.Location = new System.Drawing.Point(5, 5);
|
||
textBox.PasswordChar = '*';
|
||
textBox.Text = input;
|
||
inputBox.Controls.Add(textBox);
|
||
|
||
Button okButton = new Button();
|
||
okButton.DialogResult = System.Windows.Forms.DialogResult.OK;
|
||
okButton.Name = "okButton";
|
||
okButton.Size = new System.Drawing.Size(75, 23);
|
||
okButton.Text = "&OK";
|
||
okButton.Location = new System.Drawing.Point(size.Width - 80 - 80, 39);
|
||
inputBox.Controls.Add(okButton);
|
||
|
||
Button cancelButton = new Button();
|
||
cancelButton.DialogResult = System.Windows.Forms.DialogResult.Cancel;
|
||
cancelButton.Name = "cancelButton";
|
||
cancelButton.Size = new System.Drawing.Size(75, 23);
|
||
cancelButton.Text = "&Cancel";
|
||
cancelButton.Location = new System.Drawing.Point(size.Width - 80, 39);
|
||
inputBox.Controls.Add(cancelButton);
|
||
|
||
|
||
DialogResult result = inputBox.ShowDialog();
|
||
input = textBox.Text;
|
||
return result;
|
||
}
|
||
|
||
void tc_StatusChanged(object sender, DisplayTabControlStatusEventArgs args)
|
||
{
|
||
switch (args.Type)
|
||
{
|
||
case VolianStatusType.Initialize:
|
||
ProgBarMax = args.Count;
|
||
ProgBarText = args.Text;
|
||
ProgBarValue = 0;
|
||
break;
|
||
case VolianStatusType.Update:
|
||
ProgBarText = args.Text;
|
||
ProgBarValue = args.Count;
|
||
break;
|
||
case VolianStatusType.Complete:
|
||
ProgBarText = args.Text;
|
||
ProgBarValue = 0;
|
||
break;
|
||
default:
|
||
break;
|
||
}
|
||
}
|
||
void tmrShutDown_Tick(object sender, EventArgs e)
|
||
{
|
||
(sender as Timer).Enabled = false;
|
||
this.Close();
|
||
}
|
||
private DialogResult ShowEULA()
|
||
{
|
||
string eulaFile = string.Format(@"\{0}", VlnSettings.EULAfile);
|
||
string strEULA = System.Environment.CurrentDirectory + eulaFile;
|
||
frmViewTextFile ViewFile = new frmViewTextFile(strEULA, RichTextBoxStreamType.PlainText);
|
||
ViewFile.Text = "End-User License Agreement";
|
||
ViewFile.ButtonText = "Agree";
|
||
if (ViewFile.ShowDialog() == DialogResult.OK)
|
||
{
|
||
System.Version ver = System.Reflection.Assembly.GetExecutingAssembly().GetName().Version;
|
||
string thisVersion = ver.Major.ToString() + "." + ver.Minor.ToString();
|
||
Properties.Settings.Default.LastVersion = thisVersion;
|
||
return DialogResult.OK;
|
||
}
|
||
return DialogResult.Cancel;
|
||
}
|
||
private bool RunningNewRevision
|
||
{
|
||
get
|
||
{
|
||
string lastVersion = Properties.Settings.Default.LastVersion;
|
||
System.Version ver = System.Reflection.Assembly.GetExecutingAssembly().GetName().Version;
|
||
string thisVersion = ver.Major.ToString() + "." + ver.Minor.ToString();
|
||
return thisVersion != lastVersion;
|
||
}
|
||
}
|
||
void displayHistory_Enter(object sender, EventArgs e)
|
||
{
|
||
tc.HideCaret();
|
||
}
|
||
void AnnotationTypeInfoList_ListChanged()
|
||
{
|
||
displaySearch1.SetupAnnotationSearch(ctrlAnnotationDetails, tc);
|
||
ctrlAnnotationDetails.SetupAnnotations(displaySearch1);
|
||
}
|
||
private void RunAutomatic()
|
||
{
|
||
string[] parameters = System.Environment.CommandLine.Split(" ".ToCharArray());
|
||
bool ranAuto = false;
|
||
foreach (string parameter in parameters)
|
||
{
|
||
if(parameter.StartsWith("/P="))
|
||
{
|
||
string[] dvstrs = parameter.Substring(3).Split(",".ToCharArray());
|
||
foreach (string dvstr in dvstrs)
|
||
{
|
||
DocVersionInfo dvi = DocVersionInfo.Get(int.Parse(dvstr));
|
||
if (dvi != null)
|
||
{
|
||
DlgPrintProcedure prnDlg = new DlgPrintProcedure(dvi,true);
|
||
if (dvi.MultiUnitCount == 0)
|
||
prnDlg.SelectedSlave = -1;
|
||
prnDlg.ShowDialog(this); // RHM 20120925 - Center dialog over PROMS window
|
||
//prnDlg.FormClosed += new FormClosedEventHandler(prnDlg_FormClosed);
|
||
//while (!_RunNext) Application.DoEvents();
|
||
ranAuto = true;
|
||
}
|
||
}
|
||
}
|
||
}
|
||
if (ranAuto)
|
||
{
|
||
this.Close();
|
||
}
|
||
}
|
||
private bool _RunNext = false;
|
||
//void prnDlg_FormClosed(object sender, FormClosedEventArgs e)
|
||
//{
|
||
// _RunNext = true;
|
||
//}
|
||
private FontFamily GetFamily(string name)
|
||
{
|
||
foreach (FontFamily ff in FontFamily.Families)
|
||
if (ff.Name == name) return ff;
|
||
return null;
|
||
}
|
||
void tc_ToggleRibbonExpanded(object sender, EventArgs args)
|
||
{
|
||
Volian.Base.Library.vlnStackTrace.ShowStackLocal("tc_ToggleRibbonExpanded {0}", ribbonControl1.Expanded);
|
||
ribbonControl1.Expanded = !ribbonControl1.Expanded;
|
||
}
|
||
void frmVEPROMS_Deactivate(object sender, EventArgs e)
|
||
{
|
||
tc.HideCaret(); // Hide the pseudo cursor (caret)
|
||
}
|
||
void tc_Leave(object sender, EventArgs e)
|
||
{
|
||
tc.ShowCaret();// Show the pseudo cursor (caret)
|
||
}
|
||
void tc_Enter(object sender, EventArgs e)
|
||
{
|
||
tc.HideCaret();// Hide the pseudo cursor (caret)
|
||
}
|
||
void displaySearch1_Enter(object sender, EventArgs e)
|
||
{
|
||
tc.HideCaret();// Hide the pseudo cursor (caret)
|
||
}
|
||
void ctrlAnnotationDetails_Enter(object sender, EventArgs e)
|
||
{
|
||
tc.HideCaret();// Hide the pseudo cursor (caret)
|
||
}
|
||
//void displayRO_EnabledChanged(object sender, EventArgs e)
|
||
//{
|
||
// Console.WriteLine("here");
|
||
//}
|
||
void _MyMRIList_AfterRemove(object sender)
|
||
{
|
||
SetupMRU();
|
||
}
|
||
private void SetupButtons()
|
||
{
|
||
if (!VlnSettings.DebugMode)
|
||
{
|
||
lblItemID.Visible = false;
|
||
btnItemInfo.Visible = false;
|
||
cmbFont.Visible = false;
|
||
btnEditItem.Visible = false;
|
||
lblResolution.Visible = false;
|
||
txtSearch.Visible = false;
|
||
}
|
||
}
|
||
#region MRU
|
||
private MostRecentItemList _MyMRIList;
|
||
private void SetupMRU()
|
||
{
|
||
icRecentDocs.SubItems.Clear();
|
||
if (_MyMRIList.Count > 0)
|
||
{
|
||
LabelItem lblItem = new LabelItem();
|
||
lblItem.Text = "Recent Documents:";
|
||
icRecentDocs.SubItems.Add(lblItem);
|
||
//icRecentDocs.SubItems.Add();
|
||
for (int i = 0; i < _MyMRIList.Count; i++)
|
||
{
|
||
MostRecentItem mri = _MyMRIList[i];
|
||
ButtonItem btnItem = new ButtonItem();
|
||
string menuTitle = mri.MenuTitle.Replace("\u2011", "-");
|
||
if (i < 9)
|
||
btnItem.Text = string.Format("<b>&{0}.</b> {1}", i + 1, menuTitle);
|
||
else
|
||
btnItem.Text = string.Format(" {1}", i + 1, menuTitle);
|
||
btnItem.Tag = mri.ItemID;
|
||
btnItem.Tooltip = mri.ToolTip;
|
||
btnItem.Click += new EventHandler(btnItem_Click);
|
||
icRecentDocs.SubItems.Add(btnItem);
|
||
}
|
||
}
|
||
}
|
||
|
||
void btnItem_Click(object sender, EventArgs e)
|
||
{
|
||
ButtonItem btnItem = (ButtonItem)sender;
|
||
MostRecentItem mri = _MyMRIList.Add((int)(btnItem.Tag));
|
||
//SaveMRU();
|
||
SetupMRU();
|
||
if (mri != null) tc.OpenItem(mri.MyItemInfo);
|
||
}
|
||
private void SaveMRU()
|
||
{
|
||
if(_MyMRIList != null) Properties.Settings.Default.MRIList = _MyMRIList.ToSettings();
|
||
Properties.Settings.Default.TreeviewExpanded = epProcedures.Expanded;
|
||
Properties.Settings.Default.Save();
|
||
}
|
||
#endregion
|
||
#region Tree View
|
||
/// <summary>
|
||
/// Get the selected tree node's properties
|
||
/// </summary>
|
||
/// <param name="node">VETreeNode</param>
|
||
bool SetupNodes(VETreeNode node)
|
||
{
|
||
if (_MyMRIList.Add(node.VEObject) != null)
|
||
SetupMRU();
|
||
#region Sample Display Table Code
|
||
// display an exiting table in that rtf grid thing
|
||
//if ((_LastStepInfo.MyContent.Type == 20007) || (_LastStepInfo.MyContent.Type == 20009))
|
||
//{
|
||
// //MessageBox.Show("Source Grid");
|
||
// //frmTable newtable1 = new frmTable(_LastStepInfo.MyContent.Text);
|
||
// //newtable1.ShowDialog();
|
||
// //MessageBox.Show("IGrid");
|
||
// //frmIGrid newtable2 = new frmIGrid(_LastStepInfo.MyContent.Text);
|
||
// //newtable2.ShowDialog();
|
||
// //MessageBox.Show("GridView"); //standard Visual Studio Control
|
||
// //frmGridView newtable3 = new frmGridView(_LastStepInfo.MyContent.Text);
|
||
// //newtable3.ShowDialog();
|
||
// MessageBox.Show("FlexCell");
|
||
// frmFlexCell newtable4 = new frmFlexCell(_LastStepInfo.MyContent.Text);
|
||
// newtable4.ShowDialog();
|
||
//}
|
||
#endregion
|
||
ItemInfo ii = node.VEObject as ItemInfo;
|
||
if (ii != null) tc.OpenItem(ii);
|
||
SetCaption(node);
|
||
return (ii != null); // return if successful on opening item in step editor or word
|
||
}
|
||
// The following code is used to setup the user interface depending on what
|
||
// is selected on the tree view (see vlnTreeView.cs)
|
||
void tv_NodeNew(object sender, vlnTreeEventArgs args)
|
||
{
|
||
VETreeNode vNode = (VETreeNode)args.Node;
|
||
IVEDrillDownReadOnly veObj = vNode.VEObject;
|
||
SectionInfo mySection = veObj as SectionInfo;
|
||
if (mySection != null && mySection.MyContent.MyEntry != null)
|
||
{
|
||
// if it is a word section, find the DisplayTabItem;
|
||
DisplayTabItem tabItem = tc.GetProcDisplayTabItem(mySection);
|
||
if(tabItem != null)tabItem.MyStepTabPanel.MyStepPanel.Reset();
|
||
}
|
||
// Don't select the newly created Step. This will be handled by tmrTreeView
|
||
//SetupNodes((VETreeNode)args.Node);
|
||
}
|
||
private void tv_NodeSelect(object sender, vlnTreeEventArgs args)
|
||
{
|
||
SetupNodes((VETreeNode)args.Node);
|
||
}
|
||
private void SetCaption(VETreeNode tn)
|
||
{
|
||
StringBuilder caption = new StringBuilder();
|
||
string sep = string.Empty;
|
||
while (tn != null)
|
||
{
|
||
if (tn.VEObject.GetType() == typeof(FolderInfo) || tn.VEObject.GetType() == typeof(DocVersionInfo))
|
||
{
|
||
//caption.Append(sep + tn.Text);
|
||
caption.Insert(0, tn.Text + sep);
|
||
sep = " - ";
|
||
}
|
||
tn = (VETreeNode)tn.Parent;
|
||
}
|
||
this.Text = caption.ToString();
|
||
}
|
||
void tv_OpenItem(object sender, vlnTreeItemInfoEventArgs args)
|
||
{
|
||
tc.OpenItem(args.MyItemInfo);
|
||
}
|
||
/// <summary>
|
||
/// When the treeview is clicked - a timer is set
|
||
/// This is done because the focus is returned to the treeview after the click event
|
||
/// This approach did not work and was replaced with the code below.
|
||
/// The problem was that each time the treeview was clicked, the last selected node
|
||
/// was opened again, or the edit window was repositioned.
|
||
/// If the item was deleted and another treenode expanded, the click to expand the
|
||
/// node would cause the deleted node to be selected.
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
//private void tv_Click(object sender, EventArgs e)
|
||
//{
|
||
//tv.Enabled = false;
|
||
//tmrTreeView.Enabled = true;
|
||
//}
|
||
/// <summary>
|
||
/// This opens nodes if the mouse is within the bounds of a node.
|
||
/// By using the timer, the focus can be passed to the edit window.
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
void tv_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
|
||
{
|
||
_ExpandingTree = false;
|
||
}
|
||
void tv_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
|
||
{
|
||
if (_ExpandingTree)
|
||
{
|
||
_ExpandingTree = false;
|
||
return;
|
||
}
|
||
if (e.Button != MouseButtons.Right)
|
||
{
|
||
Point newPoint = new Point(e.X, e.Y);
|
||
VETreeNode tn = tv.GetNodeAt(newPoint) as VETreeNode;
|
||
// Check to make sure that a node has been selected and
|
||
// that the mouse is within the bounds of the node.
|
||
if (tn != null && tn.Bounds.Left < newPoint.X)
|
||
{
|
||
tv.SelectedNode = tn;
|
||
tv.Enabled = false;
|
||
tmrTreeView.Enabled = true;
|
||
}
|
||
else
|
||
{
|
||
if (tn != null && tn.Bounds.Left < newPoint.X + 30)
|
||
return;
|
||
if (tc.SelectedDisplayTabItem != null && tc.SelectedDisplayTabItem.SelectedItemInfo != null)
|
||
{
|
||
tv.AdjustTree(tc.SelectedDisplayTabItem.SelectedItemInfo);
|
||
tc.SelectedDisplayTabItem.Focus();
|
||
SetCaption(tv.SelectedNode as VETreeNode);
|
||
}
|
||
}
|
||
}
|
||
}
|
||
/// <summary>
|
||
/// This event is fired from the timer after the treeview click event completes
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void tmrTreeView_Tick(object sender, EventArgs e)
|
||
{
|
||
bool giveTvFocus = true;
|
||
tmrTreeView.Enabled = false; // Timer has now fired
|
||
VETreeNode tn = tv.SelectedNode as VETreeNode;
|
||
if (tn != null) giveTvFocus = !SetupNodes(tn);
|
||
tv.Enabled = true;
|
||
if (giveTvFocus) tv.Focus();
|
||
}
|
||
private void tmrAutomatic_Tick(object sender, EventArgs e)
|
||
{
|
||
tmrAutomatic.Enabled = false; // Timer has now fired
|
||
RunAutomatic();
|
||
}
|
||
private bool _ExpandingTree=false;
|
||
private void tv_BeforeExpand(object sender, TreeViewCancelEventArgs e)
|
||
{
|
||
_ExpandingTree = true;
|
||
VETreeNode tn = ((VETreeNode)e.Node);
|
||
tn.LoadingChildrenDone += new VETreeNodeEvent(tn_LoadingChildrenDone);
|
||
tn.LoadingChildrenMax += new VETreeNodeEvent(tn_LoadingChildrenMax);
|
||
tn.LoadingChildrenValue += new VETreeNodeEvent(tn_LoadingChildrenValue);
|
||
tn.LoadingChildrenSQL += new VETreeNodeEvent(tn_LoadingChildrenSQL);
|
||
tn.LoadChildren(true);
|
||
}
|
||
#region Property Page and Grid
|
||
DialogResult tv_NodeOpenProperty(object sender, vlnTreePropertyEventArgs args)
|
||
{
|
||
this.Cursor = Cursors.WaitCursor;
|
||
DialogResult dr = DialogResult.Cancel;
|
||
if ((int)Settings.Default["PropPageStyle"] == (int)PropPgStyle.Grid)
|
||
{
|
||
frmPropGrid propGrid = new frmPropGrid(args.ConfigObject, args.Title);
|
||
dr = propGrid.ShowDialog();
|
||
}
|
||
else
|
||
{
|
||
if (args.FolderConfig != null)
|
||
{
|
||
frmFolderProperties frmfld = new frmFolderProperties(args.FolderConfig);
|
||
dr = frmfld.ShowDialog();
|
||
if (dr == DialogResult.OK)
|
||
epProcedures.TitleText = getProcedurePanelHeading();// get the panel heading
|
||
}
|
||
else if (args.DocVersionConfig != null)
|
||
{
|
||
frmVersionsProperties frmver = new frmVersionsProperties(args.DocVersionConfig);
|
||
frmver.ProgressBar = bottomProgBar;
|
||
dr = frmver.ShowDialog();
|
||
}
|
||
else if (args.ProcedureConfig != null)
|
||
{
|
||
string message = string.Empty;
|
||
if (!MySessionInfo.CanCheckOutItem(args.ProcedureConfig.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
||
{
|
||
MessageBox.Show(this, message, "Procedure Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
||
this.Cursor = Cursors.Default;
|
||
return DialogResult.None;
|
||
}
|
||
int ownerID = MySessionInfo.CheckOutItem(args.ProcedureConfig.MyProcedure.ItemID, 0);
|
||
frmProcedureProperties frmproc = new frmProcedureProperties(args.ProcedureConfig);
|
||
dr = frmproc.ShowDialog();
|
||
MySessionInfo.CheckInItem(ownerID);
|
||
}
|
||
else if (args.SectionConfig != null)
|
||
{
|
||
//// If this is a word document, close any edit sessions...
|
||
//ItemInfo ii = null;
|
||
//using (Section tmp = args.SectionConfig.MySection)
|
||
//{
|
||
// ii = ItemInfo.Get(tmp.ItemID);
|
||
// if (!ii.IsStepSection) tc.CloseWordItem(ii);
|
||
//}
|
||
|
||
frmSectionProperties frmsec = new frmSectionProperties(args.SectionConfig);
|
||
dr = frmsec.ShowDialog();
|
||
if (dr == DialogResult.OK && displayLibDocs.LibDocList != null)
|
||
{
|
||
displayLibDocs.LibDocListFillIn(tc);
|
||
displayLibDocs.SetSelectedLibDoc();
|
||
}
|
||
}
|
||
}
|
||
this.Cursor = Cursors.Default;
|
||
return dr;
|
||
}
|
||
#endregion
|
||
#endregion
|
||
#region ColorStuff
|
||
/// <summary>
|
||
/// Get a System.Drawing.Color from an Argb or color name
|
||
/// </summary>
|
||
/// <param name="strColor">Color Name or "[(alpha,)red,green,blue]"</param>
|
||
/// <returns></returns>
|
||
private static Color cGetColor(string strColor)
|
||
{
|
||
// This was copied from frmFolderProperties.CS
|
||
Color rtnColor;
|
||
if (strColor == null || strColor.Equals(""))
|
||
rtnColor = Color.White;
|
||
else
|
||
{
|
||
if (strColor[0] == '[')
|
||
{
|
||
string[] parts = strColor.Substring(1, strColor.Length - 2).Split(",".ToCharArray());
|
||
int parts_cnt = 0;
|
||
foreach (string s in parts)
|
||
{
|
||
parts[parts_cnt] = parts[parts_cnt].TrimStart(' '); // remove preceeding blanks
|
||
parts_cnt++;
|
||
}
|
||
if (parts_cnt == 3)
|
||
rtnColor = Color.FromArgb(Int32.Parse(parts[0]), Int32.Parse(parts[1]), Int32.Parse(parts[2]));
|
||
else
|
||
rtnColor = Color.FromArgb(Int32.Parse(parts[0].Substring(2)), Int32.Parse(parts[1].Substring(2)), Int32.Parse(parts[2].Substring(2)), Int32.Parse(parts[3].Substring(2)));
|
||
}
|
||
else rtnColor = Color.FromName(strColor);
|
||
}
|
||
return rtnColor;
|
||
}
|
||
//private void SetupEditorColors(DisplayPanel vlnCSLAPanel1, TabPage pg)
|
||
//{
|
||
// // setup color
|
||
// if (_LastFolderInfo == null)
|
||
// {
|
||
// // user didn't select a FolderInfo type of node.
|
||
// // walk up the tree to find the first FolderInfo node type
|
||
// VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
||
// while (tn != null && tn.VEObject.GetType() != typeof(FolderInfo))
|
||
// tn = (VETreeNode)tn.Parent;
|
||
// _LastFolderInfo = (FolderInfo)(tn.VEObject);
|
||
// _LastFolder = _LastFolderInfo.Get();
|
||
// }
|
||
|
||
// if ((_LastFolderInfo.FolderConfig.Color_editbackground != null) && !(_LastFolderInfo.FolderConfig.Color_editbackground.Equals("")))
|
||
// {
|
||
// vlnCSLAPanel1.ActiveColor = cGetColor(_LastFolderInfo.FolderConfig.Color_editbackground);
|
||
// }
|
||
// if ((_LastFolderInfo.FolderConfig.Default_BkColor != null) && !(_LastFolderInfo.FolderConfig.Default_BkColor.Equals("")))
|
||
// {
|
||
// vlnCSLAPanel1.InactiveColor = _LastFolderInfo.FolderConfig.Default_BkColor;
|
||
// vlnCSLAPanel1.TabColor = vlnCSLAPanel1.InactiveColor;
|
||
// vlnCSLAPanel1.PanelColor = vlnCSLAPanel1.InactiveColor;
|
||
// pg.BackColor = vlnCSLAPanel1.InactiveColor;
|
||
// }
|
||
//}
|
||
#endregion
|
||
#region Table Insert Sample Code
|
||
// TODO: for tables
|
||
//private void btnInsTable_Click(object sender, EventArgs e)
|
||
//{
|
||
// Point loc = btnInsTable.DisplayRectangle.Location;
|
||
// loc.X += 300;
|
||
// int top = this.Top + (btnInsTable.Size.Height * 2);
|
||
// TablePickerDlg(sender, e, loc, top);
|
||
//}
|
||
|
||
//private void TablePickerDlg(object sender, EventArgs e, Point loc, int top)
|
||
//{
|
||
// Accentra.Controls.TablePicker tp = new Accentra.Controls.TablePicker();
|
||
// tp.Location = loc;
|
||
// tp.Top += top;
|
||
// tp.Show();
|
||
// while (tp.Visible)
|
||
// {
|
||
// Application.DoEvents();
|
||
// System.Threading.Thread.Sleep(0);
|
||
// }
|
||
// // This was used to display a dialog containing a table grid
|
||
// // using a product called Source Grid - was for demo purposes only
|
||
// //
|
||
// //if (!tp.Cancel)
|
||
// //{
|
||
// // frmTable newtable = new frmTable(tp.SelectedRows, tp.SelectedColumns);
|
||
// // newtable.Show();
|
||
// //}
|
||
//}
|
||
#endregion
|
||
#region Progress Bar
|
||
|
||
/// <summary>
|
||
/// Used for the status bar in the lower left corner of the main screen
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="args"></param>
|
||
void tn_LoadingChildrenSQL(object sender, VETreeNodeEventArgs args)
|
||
{
|
||
ProgBarText = "Loading SQL";
|
||
}
|
||
|
||
/// <summary>
|
||
/// Used for the status bar in the lower left corner of the main screen
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="args"></param>
|
||
void tn_LoadingChildrenValue(object sender, VETreeNodeEventArgs args)
|
||
{
|
||
ProgBarValue = args.Value;
|
||
}
|
||
|
||
/// <summary>
|
||
/// Used for the status bar in the lower left corner of the main screen
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="args"></param>
|
||
void tn_LoadingChildrenMax(object sender, VETreeNodeEventArgs args)
|
||
{
|
||
ProgBarMax = args.Value;
|
||
ProgBarText = "Loading...";
|
||
}
|
||
|
||
/// <summary>
|
||
/// Used for the status bar in the lower left corner of the main screen
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="args"></param>
|
||
void tn_LoadingChildrenDone(object sender, VETreeNodeEventArgs args)
|
||
{
|
||
if (VlnSettings.DebugMode)
|
||
ProgBarText = args.Info + " Seconds";
|
||
else
|
||
ProgBarText = "";
|
||
}
|
||
|
||
public int ProgBarMax
|
||
{
|
||
get { return bottomProgBar.Maximum; }
|
||
set { bottomProgBar.Maximum = value; }
|
||
}
|
||
|
||
public int ProgBarValue
|
||
{
|
||
get { return bottomProgBar.Value; }
|
||
set { bottomProgBar.Value = value; }
|
||
}
|
||
|
||
public string ProgBarText
|
||
{
|
||
get { return bottomProgBar.Text; }
|
||
set
|
||
{
|
||
bottomProgBar.TextVisible = true;
|
||
bottomProgBar.Text = value;
|
||
}
|
||
}
|
||
|
||
#endregion
|
||
#region Find/Replace and Search
|
||
|
||
/// <summary>
|
||
/// Find/Replace button on the ribbon
|
||
/// Display the Find/Replace dialog
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
//private void btnFindRplDlg_Click_1(object sender, EventArgs e)
|
||
//{
|
||
// FindReplace frmFindRepl = new FindReplace();
|
||
// frmFindRepl.Show();
|
||
//}
|
||
|
||
/// <summary>
|
||
/// Global Search button on the ribbon
|
||
/// Opens the Information Pannel and selects the Results tab
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
//private void btnGlbSrch_Click(object sender, EventArgs e)
|
||
//{
|
||
// toolsPanel.Expanded = true;
|
||
// toolsTabs.SelectedTab = toolstabResults;
|
||
//}
|
||
|
||
#endregion
|
||
#region Similar Steps
|
||
|
||
/// <summary>
|
||
/// Similar Steps button on the ribbon
|
||
/// Opens the Information Pannel and selects the Results tab
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnSimStps_Click(object sender, EventArgs e)
|
||
{
|
||
//infoPanel.Expanded = true;
|
||
//infoTabs.SelectedTab = toolstabResults;
|
||
//btnSimStpsRslt.Checked = true;
|
||
}
|
||
|
||
#endregion
|
||
#region Help/About
|
||
|
||
/// <summary>
|
||
/// About button on the ribbon
|
||
/// Display the About dialog
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnAbout_Click(object sender, EventArgs e)
|
||
{
|
||
AboutVEPROMS about = new AboutVEPROMS();
|
||
about.ShowDialog();
|
||
}
|
||
|
||
/// <summary>
|
||
/// Volian Web button on the ribbon
|
||
/// display the Volian web site on a pop up form
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnVlnWeb_Click(object sender, EventArgs e)
|
||
{
|
||
VlnWeb veWWW = new VlnWeb();
|
||
veWWW.Show();
|
||
}
|
||
|
||
#endregion
|
||
#region Ribbon
|
||
/// <summary>
|
||
/// This Opens the treeView or opens the selected item in the TreeView
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnOpen_Click(object sender, EventArgs e)
|
||
{
|
||
if (!epProcedures.Expanded) // If panel not expanded - expand it.
|
||
{
|
||
epProcedures.Expanded = true;
|
||
if (tv.Nodes.Count > 0 && tv.SelectedNode == null)
|
||
tv.SelectedNode = tv.Nodes[0];
|
||
tv.Focus();
|
||
}
|
||
else
|
||
{
|
||
// TODO: DeleteMe
|
||
//VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
||
tv.OpenNode();
|
||
}
|
||
}
|
||
private void btnNew_Click(object sender, EventArgs e)
|
||
{
|
||
if (!epProcedures.Expanded) return;
|
||
VETreeNode vtn = tv.SelectedNode as VETreeNode;
|
||
if (vtn == null) return; // nothing was selected.
|
||
if (btnNew.SubItems.Count > 0) return; // submenu will be displayed
|
||
vtn.Expand();
|
||
|
||
// Determine type of 'new' based on tree node's object type. The
|
||
// only options here are those that would not have created, based on
|
||
// containers, a submenu (see the office2007buttonstartbutton1_click code)
|
||
FolderInfo fi = vtn.VEObject as FolderInfo;
|
||
if (fi != null)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Folder);
|
||
return;
|
||
}
|
||
|
||
DocVersionInfo dvi = vtn.VEObject as DocVersionInfo;
|
||
if (dvi != null)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Procedure);
|
||
return;
|
||
}
|
||
|
||
// All other types are handled with sub-menus.
|
||
|
||
}
|
||
|
||
/// <summary>
|
||
/// Options button on the dialog that appears when the V icon is clicked (top left of application window)
|
||
/// note that the "V icon" is also called the Office 2007 Start Button
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnOptions_Click(object sender, EventArgs e)
|
||
{
|
||
frmSysOptions VeSysOpts = new frmSysOptions();
|
||
VeSysOpts.ShowDialog();
|
||
StepTabRibbon.PasteNoReturnsSetting = Properties.Settings.Default.PasteNoReturns;
|
||
StepTabRibbon.PastePlainTextSetting = Properties.Settings.Default.PastePlainText;
|
||
}
|
||
/// <summary>
|
||
/// Exit button on the dialog that appears when the V icon is clicked (top left of application window)
|
||
/// note that the "V icon" is also called the Office 2007 Start Button
|
||
/// </summary>
|
||
/// <param name="sender"></param>
|
||
/// <param name="e"></param>
|
||
private void btnExit_Click(object sender, EventArgs e)
|
||
{
|
||
this.Close();
|
||
}
|
||
#endregion
|
||
#region InfoTabRO
|
||
private void infotabRO_Click(object sender, EventArgs e)
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabRO;
|
||
//if (dtp == null) return;
|
||
|
||
//displayRO.Mydvi = SelectedDVI;
|
||
displayRO.MyROFSTLookup = SelectedROFst.GetROFSTLookup(SelectedDVI);
|
||
displayRO.MyRTB = (SelectedStepTabPanel == null) ? null :
|
||
SelectedStepTabPanel.MyStepPanel.SelectedEditItem == null ? null : SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyStepRTB;
|
||
displayRO.TabControl = tc;
|
||
|
||
displayRO.ProgressBar = bottomProgBar;
|
||
}
|
||
#endregion
|
||
#region InfoTabTransition
|
||
private void infotabTransition_Click(object sender, EventArgs e)
|
||
{
|
||
if (tc == null || tc.SelectedDisplayTabItem == null) return;
|
||
if (SelectedStepTabPanel == null) return;
|
||
displayTransition.MyRTB = SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyStepRTB;
|
||
//displayTransition.RangeColor = global::VEPROMS.Properties.Settings.Default.TransitionRangeColor;
|
||
displayTransition.CurTrans = null;
|
||
}
|
||
#endregion
|
||
#region InfoTabLibDocs
|
||
private void tabItemLibDocs_Click(object sender, EventArgs e)
|
||
{
|
||
displayLibDocs.RefreshLibDocPanel(tc);
|
||
//displayLibDocs.LibDocListFillIn(tc);
|
||
}
|
||
private void tv_SectionShouldClose(object sender, vlnTreeSectionInfoEventArgs args)
|
||
{
|
||
if (!args.MySectionInfo.IsStepSection) tc.CloseWordItem(args.MySectionInfo, args.IsDeleting);
|
||
else
|
||
{
|
||
if (tc == null) return;
|
||
StepTabPanel tp = tc.GetProcedureTabPanel(args.MySectionInfo as ItemInfo);
|
||
if (tp == null) return;
|
||
DisplayTabItem ti = tp.MyDisplayTabItem;
|
||
tc.CloseTabItem(ti);
|
||
}
|
||
}
|
||
private void tv_WordSectionDeleted(object sender, WordSectionEventArgs args)
|
||
{
|
||
// is it a library document - if so and libdoc panel is visible, update lists on panel.
|
||
// entry is null if it's a table of contents.
|
||
if ((!args.MySectionInfo.IsStepSection) && (args.MySectionInfo.MyContent.MyEntry != null) && ((args.MySectionInfo.MyContent.MyEntry.MyDocument.LibTitle ?? "") != "") && (toolsPanel.Expanded))
|
||
{
|
||
if (toolsTabs.SelectedTab == tabItemLibDocs)
|
||
{
|
||
displayLibDocs.RefreshLibDocPanel(tc);
|
||
}
|
||
}
|
||
}
|
||
#endregion
|
||
#region InfoTabTags
|
||
private void infotabTags_Click(object sender, EventArgs e)
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabTags;
|
||
displayTags.MyEditItem = (SelectedStepTabPanel == null) ? null : SelectedStepTabPanel.MyStepPanel.SelectedEditItem;
|
||
}
|
||
#endregion
|
||
#region InfoTabBookMarks
|
||
private void infotabBookMarks_Click(object sender, EventArgs e)
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabTags;
|
||
displayTags.MyEditItem = (SelectedStepTabPanel == null) ? null : SelectedStepTabPanel.MyStepPanel.SelectedEditItem;
|
||
}
|
||
#endregion
|
||
#region PanelSupport
|
||
private void tc_WordSectionClose(object sender, WordSectionEventArgs args)
|
||
{
|
||
if (!args.MySectionInfo.IsStepSection) tc.CloseWordItem(args.MySectionInfo);
|
||
}
|
||
private void tc_WordSectionDeleted(object sender, WordSectionEventArgs args)
|
||
{
|
||
// is it a library document - if so and libdoc panel is visible, update lists on panel.
|
||
if ((!args.MySectionInfo.IsStepSection) && (args.MySectionInfo.MyContent.MyEntry != null && (args.MySectionInfo.MyContent.MyEntry.MyDocument.LibTitle ?? "") != "") && (toolsPanel.Expanded))
|
||
{
|
||
if (toolsTabs.SelectedTab == tabItemLibDocs)
|
||
{
|
||
displayLibDocs.RefreshLibDocPanel(tc);
|
||
}
|
||
}
|
||
}
|
||
private void tc_ItemPasted(object sender, vlnTreeItemInfoPasteEventArgs args)
|
||
{
|
||
// all this needs to do is refresh the libdoc display - if it is visible
|
||
// and the current item is word section, i.e. it may be a lib doc & would
|
||
// require refresh of the lib doc panel.
|
||
if (toolsTabs.SelectedTab == tabItemLibDocs && args.MyItemInfo.IsSection)
|
||
{
|
||
SectionInfo si = args.MyItemInfo as SectionInfo;
|
||
if (si != null && (si.MyContent.MyEntry.MyDocument.LibTitle ?? "") != "") displayLibDocs.RefreshLibDocPanel(tc);
|
||
}
|
||
displayHistory.RefreshChangeList();
|
||
}
|
||
private void tc_PanelTabDisplay(object sender, StepPanelTabDisplayEventArgs args)
|
||
{
|
||
if (args.PanelTabName == "Bookmarks")
|
||
{
|
||
toolsPanel.Expanded = true;
|
||
toolsTabs.SelectedTab = toolsTabBookMarks;
|
||
if (_CurrentItem != null && !dlgFindReplace.Visible) displayBookMarks.AddBookMark(_CurrentItem);
|
||
}
|
||
else if (args.PanelTabName == "Global Search")
|
||
{
|
||
toolsPanel.Expanded = true;
|
||
toolsTabs.SelectedTab = toolstabResults;
|
||
}
|
||
else if (args.PanelTabName == "LibDocs")
|
||
{
|
||
toolsPanel.Expanded = true;
|
||
toolsTabs.SelectedTab = tabItemLibDocs;
|
||
toolsTabs.SelectedTab.PerformClick();
|
||
}
|
||
else if (args.PanelTabName == "Annots")
|
||
{
|
||
epAnnotations.Expanded = true;
|
||
}
|
||
else if (args.PanelTabName == "Change Step Type")
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabTags;
|
||
displayTags.HighlightChangeStep();
|
||
}
|
||
else if (args.PanelTabName == "FndRpl")
|
||
{
|
||
dlgFindReplace.InApproved = (_SelectedDVI != null && _SelectedDVI.VersionType == 127);
|
||
dlgFindReplace.Mydocversion = _SelectedDVI;
|
||
dlgFindReplace.Visible = true;
|
||
dlgFindReplace.MyDisplayBookMarks = displayBookMarks;
|
||
}
|
||
else if (args.PanelTabName == "SpellChecker")
|
||
{
|
||
SpellChecker.DoSpellCheck();
|
||
}
|
||
else if (args.PanelTabName == "DisplayROUpdateROFST")
|
||
{
|
||
_SelectedROFst = null;
|
||
displayRO.MyROFSTLookup = SelectedROFst.GetROFSTLookup(SelectedDVI);
|
||
displayRO.RefreshRoTree();
|
||
}
|
||
}
|
||
private void tc_SelectedDisplayTabItemChanged(object sender, EventArgs args)
|
||
{
|
||
// If the current procedure or section in the treeview doesn't match then change the treeview selection.
|
||
//jcb added for error B2012-117
|
||
ItemInfo tvii;
|
||
if (tv.SelectedNode == null)
|
||
tvii = null;
|
||
else
|
||
tvii = ((tv.SelectedNode as VETreeNode).VEObject) as ItemInfo;
|
||
if (tc.SelectedDisplayTabItem == null) return;
|
||
if (tc.SelectedDisplayTabItem.MyItemInfo == null) return; // library document.
|
||
if (tc.SelectedDisplayTabItem.MyItemInfo.IsProcedure)
|
||
{
|
||
if (tvii == null || tvii.IsSection || tc.SelectedDisplayTabItem.MyItemInfo.ItemID != tvii.MyProcedure.ItemID)
|
||
tv.AdjustTree(tc.SelectedDisplayTabItem.MyItemInfo);
|
||
}
|
||
else
|
||
{
|
||
if (tvii == null || tc.SelectedDisplayTabItem.MyItemInfo.ItemID != tvii.ItemID)
|
||
tv.AdjustTree(tc.SelectedDisplayTabItem.MyItemInfo);
|
||
}
|
||
|
||
// the following line will determine if the format uses the change id feature, and if so
|
||
// either prompts the user for change id, or uses an already input one if the procedure has been
|
||
// accessed. This gets run when the user clicks on procedure tabs in the step editor.
|
||
tc.HandleChangeId(tc.SelectedDisplayTabItem.MyItemInfo, tc.SelectedDisplayTabItem);
|
||
|
||
SetCaption(tv.SelectedNode as VETreeNode);
|
||
displayApplicability.MyDisplayTabItem = tc.SelectedDisplayTabItem;
|
||
if (tc.SelectedDisplayTabItem.MyItemInfo.MyDocVersion.DocVersionAssociationCount > 0)
|
||
displayRO.MyROFSTLookup = tc.SelectedDisplayTabItem.MyItemInfo.MyDocVersion.DocVersionAssociations[0].MyROFst.GetROFSTLookup(tc.SelectedDisplayTabItem.MyItemInfo.MyDocVersion);
|
||
lblUser.Text = tc.SelectedDisplayTabItem.MyUserRole;
|
||
if (tc.SelectedDisplayTabItem.MyItemInfo.MyDocVersion.MultiUnitCount > 1)
|
||
btnPrint.Visible = false;
|
||
else
|
||
btnPrint.Visible = true;
|
||
}
|
||
private void tc_StepPanelModeChange(object sender, StepRTBModeChangeEventArgs args)
|
||
{
|
||
this.lblEditView.Text = args.ViewMode == E_ViewMode.Edit ? "Edit" : "View";
|
||
this.dlgFindReplace.ToggleReplaceTab(args.ViewMode);
|
||
}
|
||
private ItemInfo _CurrentItem = null;
|
||
private StepRTB _LastStepRTB = null;
|
||
private void tc_ItemSelectedChanged(object sender, ItemSelectedChangedEventArgs args)
|
||
{
|
||
btnFixMSWord.Visible = (args != null && ( args.MyItemInfo != null && args.MyEditItem == null));
|
||
if (_LastStepRTB != null && !_LastStepRTB.Disposing && !_LastStepRTB.Closed)
|
||
_LastStepRTB.EditModeChanged -= new StepRTBEvent(_LastStepRTB_EditModeChanged);
|
||
_LastStepRTB = args != null && args.MyEditItem != null ? args.MyEditItem.MyStepRTB : null;
|
||
if (_LastStepRTB != null) _LastStepRTB.EditModeChanged += new StepRTBEvent(_LastStepRTB_EditModeChanged);
|
||
lblEditView.Text = " ";
|
||
if (args == null)
|
||
{
|
||
_CurrentItem = null;
|
||
ctrlAnnotationDetails.CurrentAnnotation = null;
|
||
ctrlAnnotationDetails.Annotations = null;
|
||
infotabFoldoutMaint.Visible = infotabRO.Visible = infotabTransition.Visible = infotabTags.Visible = infotabHistory.Visible = infotabApplicability.Visible = false;
|
||
// When infotabTags is set to InVisible, the matching panel also needs to be set to invisible
|
||
infotabControlPanelTags.Visible = false;
|
||
displayTags.MyEditItem = null;
|
||
displayTags.Visible = false;
|
||
SelectedStepTabPanel = null;
|
||
lblItemID.Text = "No Item Selected";
|
||
lblItemID.ForeColor = Color.Yellow;
|
||
}
|
||
else
|
||
{
|
||
lblItemID.Text = string.Format("ItemID = {0}", args.MyItemInfo.ItemID);
|
||
lblItemID.ForeColor = Color.DarkBlue;
|
||
if (_CurrentItem != args.MyItemInfo)
|
||
{
|
||
if (_CurrentItem != null) _CurrentItem.Deleted -= new ItemInfoEvent(_CurrentItem_Deleted);
|
||
_CurrentItem = args.MyItemInfo;
|
||
}
|
||
//vlnStackTrace.ShowStack("enter tc_ItemSelectedChanged {0}", _CurrentItem);
|
||
if (args.MyEditItem == null)
|
||
{
|
||
infotabTransition.Visible = false;
|
||
infotabRO.Visible = false;
|
||
if (args.MyItemInfo.MyDocVersion.DocVersionAssociations != null)
|
||
{
|
||
displayRO.MyROFST = args.MyItemInfo.MyDocVersion.DocVersionAssociations[0].MyROFst;
|
||
displayRO.MyRTB = null;
|
||
infotabRO.Visible = true;
|
||
}
|
||
infotabTags.Visible = false;
|
||
infotabApplicability.Visible = false;
|
||
// Not sure why but the following line was causing a hang if
|
||
// you opened a procedure
|
||
// and then created a word section
|
||
// and then you selected the procedure "Tab"
|
||
//infotabHistory.Visible = false;
|
||
displayBookMarks.MyEditItem = null;
|
||
displayFoldoutMaint.Visible = false;
|
||
//vlnStackTrace.ShowStack("enter tc_ItemSelectedChanged {0}", _CurrentItem);
|
||
}
|
||
else
|
||
{
|
||
if (args.MyEditItem.MyItemInfo.IsSection || args.MyEditItem.MyItemInfo.IsProcedure)
|
||
{
|
||
infotabRO.Visible = infotabTransition.Visible = false;
|
||
infotabTags.Visible = false;
|
||
displayTags.Visible = false;
|
||
}
|
||
else
|
||
{
|
||
infotabTags.Visible = true;
|
||
if (_LastStepRTB != null)
|
||
{
|
||
infotabRO.Visible = _LastStepRTB.MyItemInfo.MyDocVersion.DocVersionAssociationCount > 0;
|
||
infotabTransition.Visible = true;
|
||
displayRO.Enabled = _LastStepRTB.EditMode || _LastStepRTB.IsRoTable;
|
||
displayTransition.Enabled = _LastStepRTB.EditMode;
|
||
}
|
||
else
|
||
infotabRO.Visible = infotabTransition.Visible = false;
|
||
|
||
// When infotabTags is set to Visible, the matching panel also needs to be set to visible
|
||
// the other panels will appear as they are selected by the user.
|
||
infotabControlPanelTags.Visible = true;
|
||
displayTags.IsVisible = true;
|
||
}
|
||
infotabHistory.Visible = true;
|
||
if (args.MyItemInfo.MyDocVersion.MultiUnitCount > 1 && !args.MyItemInfo.IsProcedure )
|
||
{
|
||
infotabApplicability.Visible = true;
|
||
displayApplicability.MyItemInfo = args.MyEditItem.MyItemInfo;
|
||
}
|
||
else
|
||
infotabApplicability.Visible = false;
|
||
if (args.MyEditItem.MyItemInfo.ActiveFormat != null)
|
||
{
|
||
// see if format has floating foldouts, and if so, display the panel.
|
||
if (args.MyEditItem.MyItemInfo.ActiveFormat.PlantFormat.FormatData.PrintData.AlternateFloatingFoldout)
|
||
{
|
||
|
||
displayFoldoutMaint.Visible = true;
|
||
infotabFoldoutMaint.Visible = true;
|
||
displayFoldoutMaint.Enabled = true;
|
||
displayFoldoutMaint.MyItemInfo = args.MyItemInfo;
|
||
}
|
||
}
|
||
// When infotabTags is set to Visible, it is given focus. The next line returns focus to the StepRTB
|
||
args.MyEditItem.SetFocus();
|
||
displayTransition.MyRTB = args.MyEditItem.MyStepRTB;
|
||
|
||
displayRO.MyRTB = args.MyEditItem.MyStepRTB;
|
||
displayTags.MyEditItem = args.MyEditItem;
|
||
displayBookMarks.MyEditItem = args.MyEditItem;
|
||
displayHistory.MyEditItem = args.MyEditItem;
|
||
|
||
displayRO.ProgressBar = bottomProgBar;
|
||
|
||
lblEditView.Text = args.MyEditItem.MyStepPanel.VwMode == E_ViewMode.Edit ? "Edit" : "View" ;
|
||
_CurrentItem.Deleted += new ItemInfoEvent(_CurrentItem_Deleted);
|
||
dlgFindReplace.MyEditItem = args.MyEditItem;
|
||
SpellChecker.MyEditItem = args.MyEditItem;
|
||
}
|
||
if (args.MyEditItem != null)
|
||
{
|
||
SelectedStepTabPanel = args.MyEditItem.MyStepPanel.MyStepTabPanel;
|
||
displayRO.ROTypeFilter = SelectedStepTabPanel.MyStepPanel.SelectedEditItem is Volian.Controls.Library.GridItem && (SelectedStepTabPanel.MyStepPanel.SelectedEditItem as Volian.Controls.Library.GridItem).MyFlexGrid.IsRoTable ?
|
||
E_ROValueType.Table : (SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyItemInfo.IsFigure) ? E_ROValueType.Image : E_ROValueType.Text;
|
||
if (_LastStepRTB.IsRoTable && _LastStepRTB.MyItemInfo.MyContent.ContentRoUsageCount > 0)
|
||
displayRO.CurROLink = _LastStepRTB.MyItemInfo.MyContent.ContentRoUsages[0];
|
||
}
|
||
else
|
||
{
|
||
SelectedDVI = args.MyItemInfo.MyDocVersion;
|
||
displayRO.ROTypeFilter = E_ROValueType.All; // allow all RO types for Word attachments (but fix)
|
||
}
|
||
}
|
||
ctrlAnnotationDetails.UpdateAnnotationGrid(_CurrentItem);
|
||
AnnotationPanelView();
|
||
btnPrint.Enabled = (_CurrentItem != null);
|
||
}
|
||
|
||
void _LastStepRTB_EditModeChanged(object sender, EventArgs args)
|
||
{
|
||
if (_LastStepRTB.EditMode) // going into edit mode in a cell of the grid.
|
||
displayRO.Enabled = displayTransition.Enabled = _LastStepRTB.EditMode;
|
||
else // going out of edit mode in a cell of the grid.
|
||
{
|
||
if (this.ActiveControl != tc || (!_LastStepRTB.Parent.Focused && (infotabRO.IsSelected || infotabTransition.IsSelected))) return;
|
||
displayRO.Enabled = displayTransition.Enabled = _LastStepRTB.EditMode;
|
||
}
|
||
}
|
||
|
||
void _CurrentItem_Deleted(object sender)
|
||
{
|
||
//displayBookMarks.DeleteItemBookMarkPanel(_CurrentItem);
|
||
}
|
||
#endregion
|
||
#region Annotations
|
||
/// <summary>
|
||
/// Display or hide the Annotation Details panel
|
||
/// </summary>
|
||
private void AnnotationPanelView()
|
||
{
|
||
if (ctrlAnnotationDetails.Annotations != null && ctrlAnnotationDetails.Annotations.Count != 0)
|
||
{
|
||
if (Settings.Default.AutoPopUpAnnotations) //cbAnnotationPopup.Checked
|
||
epAnnotations.Expanded = true;
|
||
else
|
||
epAnnotations.TitleStyle.BackColor1.Color = Color.Yellow;
|
||
}
|
||
else
|
||
{
|
||
if (!btnAnnoDetailsPushPin.Checked)
|
||
epAnnotations.Expanded = false;
|
||
epAnnotations.TitleStyle.BackColor1.Color = _CommentTitleBckColor;
|
||
ctrlAnnotationDetails.AnnotationText = null;
|
||
}
|
||
}
|
||
#endregion
|
||
#region LinkSupport
|
||
private void tc_LinkActiveChanged(object sender, StepPanelLinkEventArgs args)
|
||
{
|
||
// determine if any infotabs are visisble, and if it is the Transition,
|
||
// change the curitem for the transition to the current item.
|
||
if (infoPanel.Expanded == true && infoTabs.SelectedTab == infotabTransition)
|
||
{
|
||
displayTransition.CurTrans = null;
|
||
}
|
||
}
|
||
private void tc_LinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabTransition;
|
||
if (SelectedStepTabPanel == null) return;
|
||
//displayTransition.RangeColor = global::VEPROMS.Properties.Settings.Default.TransitionRangeColor;
|
||
displayTransition.MyRTB = SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyStepRTB;
|
||
displayTransition.CurTrans = args.MyLinkText.MyTransitionInfo;
|
||
}
|
||
private void tc_LinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
||
{
|
||
infoPanel.Expanded = true;
|
||
infoTabs.SelectedTab = infotabRO;
|
||
|
||
if (SelectedStepTabPanel == null) return;
|
||
displayRO.MyROFSTLookup = SelectedROFst.GetROFSTLookup(SelectedDVI);
|
||
displayRO.MyRTB = SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyStepRTB;
|
||
displayRO.CurROLink = args.MyLinkText.MyRoUsageInfo;
|
||
displayRO.ROTypeFilter = SelectedStepTabPanel.MyStepPanel.SelectedEditItem is Volian.Controls.Library.GridItem && (SelectedStepTabPanel.MyStepPanel.SelectedEditItem as Volian.Controls.Library.GridItem).MyFlexGrid.IsRoTable ?
|
||
E_ROValueType.Table : (SelectedStepTabPanel.MyStepPanel.SelectedEditItem.MyItemInfo.IsFigure) ? E_ROValueType.Image: E_ROValueType.Text;
|
||
//displayRO.Mydvi = SelectedDVI;
|
||
displayRO.ProgressBar = bottomProgBar;
|
||
displayRO.TabControl = tc;
|
||
}
|
||
#endregion
|
||
#region VButton
|
||
private void office2007StartButton1_Click(object sender, EventArgs e)
|
||
{
|
||
// If the V-Button is clicked, check to see what tree node is s selected
|
||
// to set up the New submenus. The New submenus are dependent on the type of
|
||
// object selected, i.e. folder, docversion, etc.
|
||
|
||
// reset in case previous selection changed items.
|
||
btnNew.SubItems.Clear();
|
||
btnNew.Enabled = false;
|
||
|
||
VETreeNode vtn = tv.SelectedNode as VETreeNode;
|
||
if (vtn == null) return;
|
||
btnNew.Enabled = true;
|
||
vtn.Expand();
|
||
|
||
// Folders can have either folders & docversions, but
|
||
// not a mix.
|
||
FolderInfo fi = vtn.VEObject as FolderInfo;
|
||
if (fi != null)
|
||
{
|
||
if (fi.FolderDocVersionCount > 0)
|
||
{
|
||
btnNew.Enabled = false;
|
||
return;
|
||
}
|
||
// if at top, 'VEPROMS', folder and childfolders below this only
|
||
// option is to create a new (sub)folder, i.e. no submenu items.
|
||
if (fi.ChildFolderCount > 0 && fi.MyParent == null) return;
|
||
//if (fi.ChildFolderCount == 0) // submenu folders/docversion
|
||
//{
|
||
if (fi.MyParent != null)
|
||
{
|
||
ButtonItem fldbbtn = new ButtonItem("fldbtn", "Folder Before");
|
||
ButtonItem fldabtn = new ButtonItem("fldabtn", "Folder After");
|
||
btnNew.SubItems.Add(fldbbtn);
|
||
btnNew.SubItems.Add(fldabtn);
|
||
fldbbtn.Click += new EventHandler(fldbbtn_Click);
|
||
fldabtn.Click += new EventHandler(fldabtn_Click);
|
||
}
|
||
ButtonItem fldbtn = new ButtonItem("fldbtn", "Folder");
|
||
btnNew.SubItems.Add(fldbtn);
|
||
fldbtn.Click += new EventHandler(fldbtn_Click);
|
||
if (fi.ChildFolderCount == 0)
|
||
{
|
||
ButtonItem dvbtn = new ButtonItem("dvbtn", "Document Version");
|
||
btnNew.SubItems.Add(dvbtn);
|
||
dvbtn.Click += new EventHandler(dvbtn_Click);
|
||
}
|
||
return;
|
||
//}
|
||
}
|
||
|
||
// DocVersions can only have procedures, so no sub-menu
|
||
DocVersionInfo dvi = vtn.VEObject as DocVersionInfo;
|
||
if (dvi != null) return;
|
||
|
||
// Procedures can have a section added or a new procedure before
|
||
// or after.
|
||
ProcedureInfo pi = vtn.VEObject as ProcedureInfo;
|
||
if (pi != null)
|
||
{
|
||
ButtonItem pbbtn = new ButtonItem("pfbtn", "Procedure Before");
|
||
ButtonItem pabtn = new ButtonItem("pabtn", "Procedure After");
|
||
ButtonItem sctbtn = new ButtonItem("sctbtn", "Section");
|
||
btnNew.SubItems.Add(pbbtn);
|
||
btnNew.SubItems.Add(pabtn);
|
||
btnNew.SubItems.Add(sctbtn);
|
||
pbbtn.Click += new EventHandler(pbbtn_Click);
|
||
pabtn.Click += new EventHandler(pabtn_Click);
|
||
sctbtn.Click += new EventHandler(sctbtn_Click);
|
||
return;
|
||
}
|
||
|
||
// Sections can have sections before, after, new subsections & if is
|
||
// a step section, can have steps
|
||
SectionInfo si = vtn.VEObject as SectionInfo;
|
||
if (si != null)
|
||
{
|
||
ButtonItem sbbtn = new ButtonItem("sbbtn", "Section Before");
|
||
ButtonItem sabtn = new ButtonItem("sabtn", "Section After");
|
||
ButtonItem subbtn = new ButtonItem("subbtn", "SubSection");
|
||
btnNew.SubItems.Add(sbbtn);
|
||
btnNew.SubItems.Add(sabtn);
|
||
btnNew.SubItems.Add(subbtn);
|
||
sbbtn.Click += new EventHandler(sbbtn_Click);
|
||
sabtn.Click += new EventHandler(sabtn_Click);
|
||
subbtn.Click += new EventHandler(subbtn_Click);
|
||
if (si.IsStepSection)
|
||
{
|
||
ButtonItem stpbtn = new ButtonItem("stpbtn", "New Step");
|
||
btnNew.SubItems.Add(stpbtn);
|
||
stpbtn.Click += new EventHandler(stpbtn_Click);
|
||
}
|
||
return;
|
||
}
|
||
|
||
// Steps can have steps before or after only.
|
||
StepInfo stpi = vtn.VEObject as StepInfo;
|
||
if (stpi != null)
|
||
{
|
||
ButtonItem stpbbtn = new ButtonItem("stpbbtn", "New Step Before");
|
||
ButtonItem stpabtn = new ButtonItem("stpabtn", "New Step After");
|
||
btnNew.SubItems.Add(stpbbtn);
|
||
btnNew.SubItems.Add(stpabtn);
|
||
stpbbtn.Click += new EventHandler(stpbbtn_Click);
|
||
stpabtn.Click += new EventHandler(stpabtn_Click);
|
||
return;
|
||
}
|
||
}
|
||
|
||
void fldabtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.FolderAfter);
|
||
}
|
||
|
||
void fldbbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.FolderBefore);
|
||
}
|
||
|
||
void stpabtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.StepAfter);
|
||
}
|
||
|
||
void stpbbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.StepBefore);
|
||
}
|
||
|
||
void subbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Section);
|
||
}
|
||
|
||
void sabtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.SectionAfter);
|
||
}
|
||
|
||
void sbbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.SectionBefore);
|
||
}
|
||
|
||
void stpbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Step);
|
||
}
|
||
|
||
void sctbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Section);
|
||
}
|
||
|
||
void pabtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.ProcedureAfter);
|
||
}
|
||
|
||
void pbbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.ProcedureBefore);
|
||
}
|
||
|
||
void dvbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.DocVersion);
|
||
}
|
||
|
||
void fldbtn_Click(object sender, EventArgs e)
|
||
{
|
||
tv.tv_NodeNew(vlnTreeView.MenuSelections.Folder);
|
||
}
|
||
private void btnSave_Click(object sender, EventArgs e)
|
||
{
|
||
}
|
||
#endregion
|
||
#region PanelEvents
|
||
private void infoPanel_Click(object sender, EventArgs e)
|
||
{
|
||
displayTags.MyEditItem = (SelectedStepTabPanel == null) ? null : SelectedStepTabPanel.MyStepPanel.SelectedEditItem;
|
||
}
|
||
//private void tv_SectionShouldClose(object sender, vlnTreeSectionInfoEventArgs args)
|
||
//{
|
||
// if (!args.MySectionInfo.IsStepSection) tc.CloseWordItem(args.MySectionInfo);
|
||
//}
|
||
|
||
private void infotabResults_Click(object sender, EventArgs e)
|
||
{
|
||
toolsPanel.Expanded = true;
|
||
InitiateSearch(true);
|
||
}
|
||
private void InitiateSearch(bool searchFocus)
|
||
{
|
||
if (toolsPanel.Expanded)
|
||
{
|
||
if (displaySearch1.Mydocversion == null)//!displaySearch1.OpenDocFromSearch)
|
||
{
|
||
if (SelectedDVI != null)
|
||
{
|
||
displaySearch1.Mydocversion = SelectedDVI;
|
||
displaySearch1.advTreeStepTypesFillIn();
|
||
}
|
||
displaySearch1.advTreeProcSetsFillIn(searchFocus);
|
||
}
|
||
}
|
||
}
|
||
|
||
private void toosTabReports_Click(object sender, EventArgs e)
|
||
{
|
||
toolsPanel.Expanded = true;
|
||
InitiateDisplayReports(true);
|
||
}
|
||
|
||
private void InitiateDisplayReports(bool reportFocus)
|
||
{
|
||
if (toolsPanel.Expanded)
|
||
{
|
||
if (SelectedDVI != null)
|
||
displayReports.Mydocversion = SelectedDVI;
|
||
displayReports.advTreeProcSetsFillIn(reportFocus);
|
||
displayReports.advTreeROFillIn(reportFocus);
|
||
displayReports.SelectReferencedObjectTab(); // to enable RO selection
|
||
}
|
||
}
|
||
|
||
private void ribbonControl1_ExpandedChanged(object sender, EventArgs e)
|
||
{
|
||
Console.WriteLine("Size {0}", ribbonControl1.Expanded);
|
||
// TODO: Need to send message to all StepTabPanels and tell them to minimize their ribbons.
|
||
tc.RibbonExpanded = ribbonControl1.Expanded;
|
||
}
|
||
|
||
private void btnItemInfo_Click(object sender, EventArgs e)
|
||
{
|
||
if (tc.SelectedDisplayTabItem != null && tc.SelectedDisplayTabItem.SelectedItemInfo != null)
|
||
{
|
||
frmPropGrid pg = new frmPropGrid(tc.SelectedDisplayTabItem.SelectedItemInfo, tc.SelectedDisplayTabItem.SelectedItemInfo.ShortPath);
|
||
pg.Show();
|
||
}
|
||
else
|
||
MessageBox.Show("Select Item First", "Item not selected", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
||
}
|
||
private void lblItemID_Click(object sender, System.EventArgs e)
|
||
{
|
||
if (tc.SelectedDisplayTabItem != null && tc.SelectedDisplayTabItem.SelectedItemInfo != null)
|
||
{
|
||
ItemInfo myitem = tc.SelectedDisplayTabItem.SelectedItemInfo;
|
||
FolderInfo myfolder = myitem.MyDocVersion.MyFolder;
|
||
string sep = "";
|
||
string fPath = "";
|
||
while (myfolder.FolderID != myfolder.ParentID)
|
||
{
|
||
fPath = myfolder.Name + sep + fPath;
|
||
sep = "/";
|
||
myfolder = myfolder.MyParent;
|
||
}
|
||
Clipboard.Clear();
|
||
DataObject mydo = new DataObject(DataFormats.Text, string.Format("{0} - {1}", fPath, myitem.ShortPath));
|
||
Clipboard.SetDataObject(mydo);
|
||
}
|
||
}
|
||
private void btnFixMSWord_Click(object sender, EventArgs e)
|
||
{
|
||
if (tc.SelectedDisplayTabItem != null && tc.SelectedDisplayTabItem.MyDSOTabPanel != null)
|
||
{
|
||
string btnText = btnFixMSWord.Text;
|
||
btnFixMSWord.FixedSize = btnFixMSWord.Size;
|
||
btnFixMSWord.Text = "Processing ...";
|
||
this.Cursor = Cursors.WaitCursor;
|
||
tc.SelectedDisplayTabItem.MyDSOTabPanel.FixSymbolCharacters();
|
||
btnFixMSWord.Text = btnText;
|
||
this.Cursor = Cursors.Default;
|
||
}
|
||
}
|
||
|
||
private void epAnnotations_Resize(object sender, EventArgs e)
|
||
{
|
||
if (epAnnotations.Expanded && !_panelExpandedChanging)
|
||
{
|
||
// if the height of the panel is smaller than the titleheight+20
|
||
//(due to the user dragging the splitter down), then un-expand the
|
||
// panel and set the height to the titleheight+75
|
||
if (epAnnotations.Size.Height < epAnnotations.TitleHeight + 20)
|
||
{
|
||
Size sz = new Size(epAnnotations.Size.Width, epAnnotations.TitleHeight + 75);
|
||
epAnnotations.Size = sz;
|
||
epAnnotations.Expanded = false;
|
||
}
|
||
}
|
||
}
|
||
|
||
private void resizeVerticalExpandedPanel(object sender, EventArgs e)
|
||
{
|
||
ExpandablePanel ep = (ExpandablePanel)sender;
|
||
if (ep.Expanded && !_panelExpandedChanging)
|
||
{
|
||
// if the width of the panel is smaller than the titleheight+20
|
||
//(due to the user dragging the splitter over), then un-expand the
|
||
// panel and set the width to the titleheight+50
|
||
if (ep.Size.Width < ep.TitleHeight + 10)
|
||
{
|
||
Size sz = new Size(ep.TitleHeight + 50, ep.Size.Height);
|
||
ep.Size = sz;
|
||
ep.Expanded = false;
|
||
}
|
||
}
|
||
}
|
||
|
||
private void expandPanelExpandedChanging(object sender, ExpandedChangeEventArgs e)
|
||
{
|
||
_panelExpandedChanging = true;
|
||
}
|
||
|
||
private void toolsPanel_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
||
{
|
||
_panelExpandedChanging = false;
|
||
expandableSplitter4.Enabled = toolsPanel.Expanded;
|
||
if (toolsPanel.Expanded)
|
||
{
|
||
InitiateSearch(true);
|
||
InitiateDisplayReports(true);
|
||
}
|
||
}
|
||
|
||
private void epAnnotations_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
||
{
|
||
_panelExpandedChanging = false;
|
||
expandableSplitter2.Enabled = epAnnotations.Expanded;
|
||
}
|
||
|
||
private void epProcedures_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
||
{
|
||
_panelExpandedChanging = false;
|
||
expandableSplitter1.Enabled = epProcedures.Expanded;
|
||
}
|
||
|
||
private void infoPanel_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
||
{
|
||
_panelExpandedChanging = false;
|
||
expandableSplitter3.Enabled = infoPanel.Expanded;
|
||
// if our active tab is a Word attachment, call the Click even on the RO tab
|
||
// to ensure that the RO FST is loaded. This fixes a bug where if the first thing you
|
||
// open is a Word attachment, the RO panel would not have the RO's listed.
|
||
if (infoPanel.Expanded && _CurrentItem != null && _CurrentItem.HasWordContent && infotabRO.Visible)
|
||
infotabRO_Click(sender, e);
|
||
}
|
||
#endregion
|
||
|
||
private void btnShortCuts_Click(object sender, EventArgs e)
|
||
{
|
||
ShortcutLists scListdlg = new ShortcutLists();
|
||
scListdlg.Show();
|
||
}
|
||
|
||
private void cmbFont_SelectedIndexChanged(object sender, EventArgs e)
|
||
{
|
||
StepRTB.MyFontFamily = cmbFont.SelectedValue as FontFamily;
|
||
}
|
||
|
||
private void btnPrint_Click(object sender, EventArgs e)
|
||
{
|
||
//VETreeNode vtn = tv.SelectedNode as VETreeNode;
|
||
//DocVersionInfo dvi = vtn.VEObject as DocVersionInfo;
|
||
//while (dvi == null)
|
||
//{
|
||
// vtn = vtn.Parent as VETreeNode;
|
||
// dvi = vtn.VEObject as DocVersionInfo;
|
||
//}
|
||
//string pnum = DisplayText.StaticStripRtfCommands(this._CurrentItem.MyProcedure.ProcedureConfig.Number).Replace("\\u8209?", "-");
|
||
DlgPrintProcedure prnDlg = new DlgPrintProcedure(this._CurrentItem.MyProcedure);//dvi.DocVersionConfig,pnum);
|
||
prnDlg.MySessionInfo = MySessionInfo;
|
||
prnDlg.ShowDialog(this); // RHM 20120925 - Center dialog over PROMS window
|
||
}
|
||
|
||
private void lblResolution_Click(object sender, EventArgs e)
|
||
{
|
||
if (this.WindowState != FormWindowState.Normal)
|
||
{
|
||
this.WindowState = FormWindowState.Normal;
|
||
}
|
||
else if (this.Size.Width != 1280)
|
||
{
|
||
this.Size = new Size(1280, 800);
|
||
}
|
||
else
|
||
{
|
||
this.Size = new Size(1024, 768);
|
||
}
|
||
}
|
||
|
||
private void frmVEPROMS_Resize(object sender, EventArgs e)
|
||
{
|
||
lblResolution.Text = string.Format("Resolution {0} x {1}", Size.Width, Size.Height);
|
||
}
|
||
private void office2007StartButton1_MouseDown(object sender, MouseEventArgs e)
|
||
{
|
||
// Refresh the MostRecentlyUsedList
|
||
_MyMRIList.Refresh();
|
||
SetupMRU();
|
||
}
|
||
|
||
private void btnEditItem_Click(object sender, EventArgs e)
|
||
{
|
||
frmPropGrid pg = new frmPropGrid(tc.SelectedDisplayTabItem.MyStepTabPanel.SelectedEditItem, "EditItem");
|
||
pg.Show();
|
||
}
|
||
private void btnUpdateFormat_Click(object sender, EventArgs e)
|
||
{
|
||
UpdateFormats(null);
|
||
}
|
||
|
||
private void UpdateFormats(string mypath)
|
||
{
|
||
if (mypath == null)
|
||
{
|
||
string fmtPath = Properties.Settings.Default.FormatPath ?? "";
|
||
DirectoryInfo di = null;
|
||
do
|
||
{
|
||
fbd.Description = "Select folder containing FmtAll and GenMacAll folders to update formats";
|
||
fbd.SelectedPath = fmtPath;
|
||
DialogResult dr = fbd.ShowDialog();
|
||
if (dr == DialogResult.Cancel)
|
||
{
|
||
bottomProgBar.Text = "Format Update Cancelled";
|
||
return;
|
||
}
|
||
di = new DirectoryInfo(fbd.SelectedPath);
|
||
fmtPath = di.FullName;
|
||
} while (((fmtPath ?? "") == "") || (!di.Exists || !Directory.Exists(di.FullName + @"\fmtall") || !Directory.Exists(di.FullName + @"\genmacall")));
|
||
Properties.Settings.Default.FormatPath = fbd.SelectedPath;
|
||
Properties.Settings.Default.Save();
|
||
mypath = di.FullName;
|
||
}
|
||
string fmtPathAll = mypath + @"\fmtall";
|
||
string genmacPathAll = mypath + @"\genmacall";
|
||
Format.FormatLoaded += new FormatEvent(Format_FormatLoaded);
|
||
Format.UpdateFormats(fmtPathAll, genmacPathAll);
|
||
Format.FormatLoaded -= new FormatEvent(Format_FormatLoaded);
|
||
}
|
||
|
||
void Format_FormatLoaded(object sender, FormatEventArgs args)
|
||
{
|
||
if (args.Status.EndsWith("Formats to Load"))
|
||
{
|
||
bottomProgBar.Value = 0;
|
||
bottomProgBar.Maximum = int.Parse(args.Status.Split(" ".ToCharArray())[0]);
|
||
}
|
||
else
|
||
{
|
||
if (args.Status.StartsWith("Error"))
|
||
MessageBox.Show(args.Status, "Error Loading Format", MessageBoxButtons.OK, MessageBoxIcon.Error);
|
||
else if (!args.Status.Contains("SubFormat")) bottomProgBar.Value++;
|
||
}
|
||
bottomProgBar.Text = args.Status;
|
||
bottomProgBar.TextVisible = true;
|
||
Application.DoEvents();
|
||
}
|
||
|
||
private void btnSendErrorLog_Click(object sender, EventArgs e)
|
||
{
|
||
|
||
frmSendErrorLog frm = new frmSendErrorLog(Properties.Settings.Default.OutlookEmail, Properties.Settings.Default["SMTPServer"].ToString(), Properties.Settings.Default["SMTPUser"].ToString(),ErrorLogFileName);
|
||
if (frm.ShowDialog(this) == DialogResult.OK)
|
||
{
|
||
Properties.Settings.Default.OutlookEmail = frm.OutlookEmail;
|
||
Properties.Settings.Default.SMTPServer = frm.SMTPServer;
|
||
Properties.Settings.Default.SMTPUser = frm.SMTPUser;
|
||
Properties.Settings.Default.Save();
|
||
MessageBox.Show("PROMS Error Log successfully sent to Volian support");
|
||
}
|
||
}
|
||
}
|
||
#region Lock stuff
|
||
public class TabItemsToClose : Stack<DisplayTabItem>
|
||
{
|
||
public void PushDTI(DisplayTabItem dti)
|
||
{
|
||
lock (this)
|
||
{
|
||
if(!this.Contains(dti))
|
||
this.Push(dti);
|
||
}
|
||
}
|
||
public DisplayTabItem PopDTI()
|
||
{
|
||
lock (this)
|
||
{
|
||
return this.Pop();
|
||
}
|
||
}
|
||
public int CountDTI
|
||
{
|
||
get
|
||
{
|
||
lock (this)
|
||
{
|
||
return this.Count;
|
||
}
|
||
}
|
||
}
|
||
}
|
||
#endregion
|
||
}
|