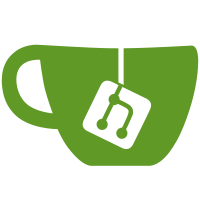
Changed lookup to use ROID rather than id when expanding the tree to show the selected RO. Previously used GetRoChildFromID which was not working
323 lines
9.8 KiB
C#
323 lines
9.8 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using Csla.Validation;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// DocumentDROUsages Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(DocumentDROUsagesConverter))]
|
|
public partial class DocumentDROUsages : BusinessListBase<DocumentDROUsages, DocumentDROUsage>, ICustomTypeDescriptor, IVEHasBrokenRules
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Business Methods
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
// One To Many
|
|
public DocumentDROUsage this[DROUsage myDROUsage]
|
|
{
|
|
get
|
|
{
|
|
foreach (DocumentDROUsage dROUsage in this)
|
|
if (dROUsage.DROUsageID == myDROUsage.DROUsageID)
|
|
return dROUsage;
|
|
return null;
|
|
}
|
|
}
|
|
public new System.Collections.Generic.IList<DocumentDROUsage> Items
|
|
{
|
|
get { return base.Items; }
|
|
}
|
|
public DocumentDROUsage GetItem(DROUsage myDROUsage)
|
|
{
|
|
foreach (DocumentDROUsage dROUsage in this)
|
|
if (dROUsage.DROUsageID == myDROUsage.DROUsageID)
|
|
return dROUsage;
|
|
return null;
|
|
}
|
|
public DocumentDROUsage Add(string roid, RODb myRODb) // One to Many
|
|
{
|
|
DocumentDROUsage dROUsage = DocumentDROUsage.New(roid, myRODb);
|
|
this.Add(dROUsage);
|
|
return dROUsage;
|
|
}
|
|
public void Remove(DROUsage myDROUsage)
|
|
{
|
|
foreach (DocumentDROUsage dROUsage in this)
|
|
{
|
|
if (dROUsage.DROUsageID == myDROUsage.DROUsageID)
|
|
{
|
|
Remove(dROUsage);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
public bool Contains(DROUsage myDROUsage)
|
|
{
|
|
foreach (DocumentDROUsage dROUsage in this)
|
|
if (dROUsage.DROUsageID == myDROUsage.DROUsageID)
|
|
return true;
|
|
return false;
|
|
}
|
|
public bool ContainsDeleted(DROUsage myDROUsage)
|
|
{
|
|
foreach (DocumentDROUsage dROUsage in DeletedList)
|
|
if (dROUsage.DROUsageID == myDROUsage.DROUsageID)
|
|
return true;
|
|
return false;
|
|
}
|
|
public bool IsDirtyList(List<object> list)
|
|
{
|
|
// any non-new deletions make us dirty
|
|
foreach (DocumentDROUsage item in DeletedList)
|
|
if (!item.IsNew)
|
|
return true;
|
|
// run through all the child objects
|
|
// and if any are dirty then then
|
|
// collection is dirty
|
|
foreach (DocumentDROUsage child in this)
|
|
if (child.IsDirtyList(list))
|
|
return true;
|
|
return false;
|
|
}
|
|
public override bool IsValid
|
|
{
|
|
get { return IsValidList(new List<object>()); }
|
|
}
|
|
public bool IsValidList(List<object> list)
|
|
{
|
|
// run through all the child objects
|
|
// and if any are invalid then the
|
|
// collection is invalid
|
|
foreach (DocumentDROUsage child in this)
|
|
if (!child.IsValidList(list))
|
|
{
|
|
//Console.WriteLine("Valid {0} Child {1} - {2}", child.IsValid, child.GetType().Name,child.ToString());
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
#endregion
|
|
#region ValidationRules
|
|
public IVEHasBrokenRules HasBrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = null;
|
|
foreach (DocumentDROUsage documentDROUsage in this)
|
|
if ((hasBrokenRules = documentDROUsage.HasBrokenRules) != null) return hasBrokenRules;
|
|
return hasBrokenRules;
|
|
}
|
|
}
|
|
public BrokenRulesCollection BrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = HasBrokenRules;
|
|
return (hasBrokenRules != null ? hasBrokenRules.BrokenRules : null);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
internal static DocumentDROUsages New()
|
|
{
|
|
return new DocumentDROUsages();
|
|
}
|
|
internal static DocumentDROUsages Get(SafeDataReader dr)
|
|
{
|
|
return new DocumentDROUsages(dr);
|
|
}
|
|
public static DocumentDROUsages GetByDocID(int docID)
|
|
{
|
|
try
|
|
{
|
|
return DataPortal.Fetch<DocumentDROUsages>(new DocIDCriteria(docID));
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on DocumentDROUsages.GetByDocID", ex);
|
|
}
|
|
}
|
|
private DocumentDROUsages()
|
|
{
|
|
MarkAsChild();
|
|
}
|
|
internal DocumentDROUsages(SafeDataReader dr)
|
|
{
|
|
MarkAsChild();
|
|
Fetch(dr);
|
|
}
|
|
#endregion
|
|
#region Data Access Portal
|
|
// called to load data from the database
|
|
private void Fetch(SafeDataReader dr)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
while (dr.Read())
|
|
this.Add(DocumentDROUsage.Get(dr));
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
[Serializable()]
|
|
private class DocIDCriteria
|
|
{
|
|
public DocIDCriteria(int docID)
|
|
{
|
|
_DocID = docID;
|
|
}
|
|
private int _DocID;
|
|
public int DocID
|
|
{
|
|
get { return _DocID; }
|
|
set { _DocID = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(DocIDCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] DocumentDROUsages.DataPortal_FetchDocID", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getDROUsagesByDocID";
|
|
cm.Parameters.AddWithValue("@DocID", criteria.DocID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
while (dr.Read()) this.Add(new DocumentDROUsage(dr));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("DocumentDROUsages.DataPortal_FetchDocID", ex);
|
|
throw new DbCslaException("DocumentDROUsages.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
internal void Update(Document document)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
try
|
|
{
|
|
// update (thus deleting) any deleted child objects
|
|
foreach (DocumentDROUsage obj in DeletedList)
|
|
obj.DeleteSelf(document);// Deletes related record
|
|
// now that they are deleted, remove them from memory too
|
|
DeletedList.Clear();
|
|
// add/update any current child objects
|
|
foreach (DocumentDROUsage obj in this)
|
|
{
|
|
if (obj.IsNew)
|
|
obj.Insert(document);
|
|
else
|
|
obj.Update(document);
|
|
}
|
|
}
|
|
finally
|
|
{
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
}
|
|
#endregion
|
|
#region ICustomTypeDescriptor impl
|
|
public String GetClassName()
|
|
{ return TypeDescriptor.GetClassName(this, true); }
|
|
public AttributeCollection GetAttributes()
|
|
{ return TypeDescriptor.GetAttributes(this, true); }
|
|
public String GetComponentName()
|
|
{ return TypeDescriptor.GetComponentName(this, true); }
|
|
public TypeConverter GetConverter()
|
|
{ return TypeDescriptor.GetConverter(this, true); }
|
|
public EventDescriptor GetDefaultEvent()
|
|
{ return TypeDescriptor.GetDefaultEvent(this, true); }
|
|
public PropertyDescriptor GetDefaultProperty()
|
|
{ return TypeDescriptor.GetDefaultProperty(this, true); }
|
|
public object GetEditor(Type editorBaseType)
|
|
{ return TypeDescriptor.GetEditor(this, editorBaseType, true); }
|
|
public EventDescriptorCollection GetEvents(Attribute[] attributes)
|
|
{ return TypeDescriptor.GetEvents(this, attributes, true); }
|
|
public EventDescriptorCollection GetEvents()
|
|
{ return TypeDescriptor.GetEvents(this, true); }
|
|
public object GetPropertyOwner(PropertyDescriptor pd)
|
|
{ return this; }
|
|
/// <summary>
|
|
/// Called to get the properties of this type. Returns properties with certain
|
|
/// attributes. this restriction is not implemented here.
|
|
/// </summary>
|
|
/// <param name="attributes"></param>
|
|
/// <returns></returns>
|
|
public PropertyDescriptorCollection GetProperties(Attribute[] attributes)
|
|
{ return GetProperties(); }
|
|
/// <summary>
|
|
/// Called to get the properties of this type.
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
public PropertyDescriptorCollection GetProperties()
|
|
{
|
|
// Create a collection object to hold property descriptors
|
|
PropertyDescriptorCollection pds = new PropertyDescriptorCollection(null);
|
|
// Iterate the list
|
|
for (int i = 0; i < this.Items.Count; i++)
|
|
{
|
|
// Create a property descriptor for the item and add to the property descriptor collection
|
|
DocumentDROUsagesPropertyDescriptor pd = new DocumentDROUsagesPropertyDescriptor(this, i);
|
|
pds.Add(pd);
|
|
}
|
|
// return the property descriptor collection
|
|
return pds;
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Property Descriptor
|
|
/// <summary>
|
|
/// Summary description for CollectionPropertyDescriptor.
|
|
/// </summary>
|
|
public partial class DocumentDROUsagesPropertyDescriptor : vlnListPropertyDescriptor
|
|
{
|
|
private DocumentDROUsage Item { get { return (DocumentDROUsage)_Item; } }
|
|
public DocumentDROUsagesPropertyDescriptor(DocumentDROUsages collection, int index) : base(collection, index) { ;}
|
|
}
|
|
#endregion
|
|
#region Converter
|
|
internal class DocumentDROUsagesConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is DocumentDROUsages)
|
|
{
|
|
// Return department and department role separated by comma.
|
|
return ((DocumentDROUsages)value).Items.Count.ToString() + " DROUsages";
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|