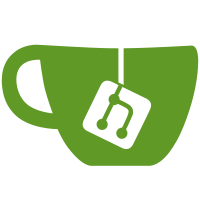
Changed lookup to use ROID rather than id when expanding the tree to show the selected RO. Previously used GetRoChildFromID which was not working
320 lines
9.9 KiB
C#
320 lines
9.9 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// DROUsageInfoList Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(DROUsageInfoListConverter))]
|
|
public partial class DROUsageInfoList : ReadOnlyListBase<DROUsageInfoList, DROUsageInfo>, ICustomTypeDescriptor, IDisposable
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Business Methods
|
|
internal new IList<DROUsageInfo> Items
|
|
{ get { return base.Items; } }
|
|
public void AddEvents()
|
|
{
|
|
foreach (DROUsageInfo tmp in this)
|
|
{
|
|
tmp.Changed += new DROUsageInfoEvent(tmp_Changed);
|
|
}
|
|
}
|
|
void tmp_Changed(object sender)
|
|
{
|
|
for (int i = 0; i < Count; i++)
|
|
{
|
|
if (base[i] == sender)
|
|
this.OnListChanged(new ListChangedEventArgs(ListChangedType.ItemChanged, i));
|
|
}
|
|
}
|
|
public void Dispose()
|
|
{
|
|
foreach (DROUsageInfo tmp in this)
|
|
{
|
|
tmp.Changed -= new DROUsageInfoEvent(tmp_Changed);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
public static DROUsageInfoList _DROUsageInfoList = null;
|
|
/// <summary>
|
|
/// Return a list of all DROUsageInfo.
|
|
/// </summary>
|
|
public static DROUsageInfoList Get()
|
|
{
|
|
try
|
|
{
|
|
if (_DROUsageInfoList != null)
|
|
return _DROUsageInfoList;
|
|
DROUsageInfoList tmp = DataPortal.Fetch<DROUsageInfoList>();
|
|
DROUsageInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
_DROUsageInfoList = tmp;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on DROUsageInfoList.Get", ex);
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Reset the list of all DROUsageInfo.
|
|
/// </summary>
|
|
public static void Reset()
|
|
{
|
|
_DROUsageInfoList = null;
|
|
}
|
|
// CSLATODO: Add alternative gets -
|
|
//public static DROUsageInfoList Get(<criteria>)
|
|
//{
|
|
// try
|
|
// {
|
|
// return DataPortal.Fetch<DROUsageInfoList>(new FilteredCriteria(<criteria>));
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// throw new DbCslaException("Error on DROUsageInfoList.Get", ex);
|
|
// }
|
|
//}
|
|
public static DROUsageInfoList GetByDocID(int docID)
|
|
{
|
|
try
|
|
{
|
|
DROUsageInfoList tmp = DataPortal.Fetch<DROUsageInfoList>(new DocIDCriteria(docID));
|
|
DROUsageInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on DROUsageInfoList.GetByDocID", ex);
|
|
}
|
|
}
|
|
public static DROUsageInfoList GetByRODbID(int rODbID)
|
|
{
|
|
try
|
|
{
|
|
DROUsageInfoList tmp = DataPortal.Fetch<DROUsageInfoList>(new RODbIDCriteria(rODbID));
|
|
DROUsageInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on DROUsageInfoList.GetByRODbID", ex);
|
|
}
|
|
}
|
|
private DROUsageInfoList()
|
|
{ /* require use of factory methods */ }
|
|
#endregion
|
|
#region Data Access Portal
|
|
private void DataPortal_Fetch()
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] DROUsageInfoList.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getDROUsages";
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new DROUsageInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("DROUsageInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("DROUsageInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
[Serializable()]
|
|
private class DocIDCriteria
|
|
{
|
|
public DocIDCriteria(int docID)
|
|
{
|
|
_DocID = docID;
|
|
}
|
|
private int _DocID;
|
|
public int DocID
|
|
{
|
|
get { return _DocID; }
|
|
set { _DocID = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(DocIDCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] DROUsageInfoList.DataPortal_FetchDocID", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getDROUsagesByDocID";
|
|
cm.Parameters.AddWithValue("@DocID", criteria.DocID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new DROUsageInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("DROUsageInfoList.DataPortal_FetchDocID", ex);
|
|
throw new DbCslaException("DROUsageInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
[Serializable()]
|
|
private class RODbIDCriteria
|
|
{
|
|
public RODbIDCriteria(int rODbID)
|
|
{
|
|
_RODbID = rODbID;
|
|
}
|
|
private int _RODbID;
|
|
public int RODbID
|
|
{
|
|
get { return _RODbID; }
|
|
set { _RODbID = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(RODbIDCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] DROUsageInfoList.DataPortal_FetchRODbID", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getDROUsagesByRODbID";
|
|
cm.Parameters.AddWithValue("@RODbID", criteria.RODbID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new DROUsageInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("DROUsageInfoList.DataPortal_FetchRODbID", ex);
|
|
throw new DbCslaException("DROUsageInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
#endregion
|
|
#region ICustomTypeDescriptor impl
|
|
public String GetClassName()
|
|
{ return TypeDescriptor.GetClassName(this, true); }
|
|
public AttributeCollection GetAttributes()
|
|
{ return TypeDescriptor.GetAttributes(this, true); }
|
|
public String GetComponentName()
|
|
{ return TypeDescriptor.GetComponentName(this, true); }
|
|
public TypeConverter GetConverter()
|
|
{ return TypeDescriptor.GetConverter(this, true); }
|
|
public EventDescriptor GetDefaultEvent()
|
|
{ return TypeDescriptor.GetDefaultEvent(this, true); }
|
|
public PropertyDescriptor GetDefaultProperty()
|
|
{ return TypeDescriptor.GetDefaultProperty(this, true); }
|
|
public object GetEditor(Type editorBaseType)
|
|
{ return TypeDescriptor.GetEditor(this, editorBaseType, true); }
|
|
public EventDescriptorCollection GetEvents(Attribute[] attributes)
|
|
{ return TypeDescriptor.GetEvents(this, attributes, true); }
|
|
public EventDescriptorCollection GetEvents()
|
|
{ return TypeDescriptor.GetEvents(this, true); }
|
|
public object GetPropertyOwner(PropertyDescriptor pd)
|
|
{ return this; }
|
|
/// <summary>
|
|
/// Called to get the properties of this type. Returns properties with certain
|
|
/// attributes. this restriction is not implemented here.
|
|
/// </summary>
|
|
/// <param name="attributes"></param>
|
|
/// <returns></returns>
|
|
public PropertyDescriptorCollection GetProperties(Attribute[] attributes)
|
|
{ return GetProperties(); }
|
|
/// <summary>
|
|
/// Called to get the properties of this type.
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
public PropertyDescriptorCollection GetProperties()
|
|
{
|
|
// Create a collection object to hold property descriptors
|
|
PropertyDescriptorCollection pds = new PropertyDescriptorCollection(null);
|
|
// Iterate the list
|
|
for (int i = 0; i < this.Items.Count; i++)
|
|
{
|
|
// Create a property descriptor for the item and add to the property descriptor collection
|
|
DROUsageInfoListPropertyDescriptor pd = new DROUsageInfoListPropertyDescriptor(this, i);
|
|
pds.Add(pd);
|
|
}
|
|
// return the property descriptor collection
|
|
return pds;
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Property Descriptor
|
|
/// <summary>
|
|
/// Summary description for CollectionPropertyDescriptor.
|
|
/// </summary>
|
|
public partial class DROUsageInfoListPropertyDescriptor : vlnListPropertyDescriptor
|
|
{
|
|
private DROUsageInfo Item { get { return (DROUsageInfo)_Item; } }
|
|
public DROUsageInfoListPropertyDescriptor(DROUsageInfoList collection, int index) : base(collection, index) { ;}
|
|
}
|
|
#endregion
|
|
#region Converter
|
|
internal class DROUsageInfoListConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is DROUsageInfoList)
|
|
{
|
|
// Return department and department role separated by comma.
|
|
return ((DROUsageInfoList)value).Items.Count.ToString() + " DROUsages";
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|