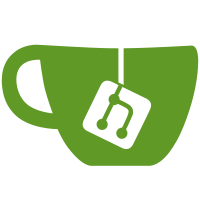
HasParent to Property rather than method Removed ActiveFormat from editable object Changed DefaultFormatSelection to use info objects Added Folder.MyFolderInfo Changed logic to use Info version of parent Changed Ancestor Lookup to use Info objects Fixed default value for disableduplex
1085 lines
33 KiB
C#
1085 lines
33 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using DescriptiveEnum;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
[Serializable]
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
//public class FolderConfig : INotifyPropertyChanged
|
|
public class FolderConfig : ConfigDynamicTypeDescriptor, INotifyPropertyChanged
|
|
{
|
|
#region DynamicTypeDescriptor
|
|
internal override bool IsReadOnly
|
|
{
|
|
get { return _Folder == null; }
|
|
}
|
|
#endregion
|
|
#region XML
|
|
private XMLProperties _Xp;
|
|
private XMLProperties Xp
|
|
{
|
|
get { return _Xp; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
//PROPGRID: Hide ParentLookup
|
|
[Browsable(false)]
|
|
public bool ParentLookup
|
|
{
|
|
get { return _Xp.ParentLookup; }
|
|
set { _Xp.ParentLookup = value; }
|
|
}
|
|
//PROPGRID: Hide AncestorLookup
|
|
//PROPGRID: Needed to comment out [NonSerialized] in order to hide this field from the property grid
|
|
//[NonSerialized]
|
|
//[Browsable(false)]
|
|
//private bool _AncestorLookup;
|
|
//[Browsable(false)]
|
|
//public bool AncestorLookup
|
|
//{
|
|
// get { return _AncestorLookup; }
|
|
// set { _AncestorLookup = value; }
|
|
//}
|
|
private Folder _Folder;
|
|
private FolderInfo _FolderInfo;
|
|
public FolderConfig(Folder folder)
|
|
{
|
|
_Folder = folder;
|
|
string xml = _Folder.Config;
|
|
if (xml == string.Empty) xml = "<config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
if (folder.MyFolderInfo.MyParent != null) _Xp.LookInAncestor += new XMLPropertiesEvent(Xp_LookInAncestorFolder);
|
|
}
|
|
private string Xp_LookInAncestorFolder(object sender, XMLPropertiesArgs args)
|
|
{
|
|
if (ParentLookup || args.AncestorLookup)
|
|
{
|
|
for (FolderInfo folder = _Folder.MyFolderInfo.MyParent; folder != null; folder = folder.MyParent)
|
|
{
|
|
string retval = folder.FolderConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
}
|
|
}
|
|
return string.Empty;
|
|
}
|
|
public bool HasParent
|
|
{
|
|
get
|
|
{
|
|
if (_Folder != null) return _Folder.ParentID != _Folder.FolderID;
|
|
if (_FolderInfo != null) return _FolderInfo.ParentID != _FolderInfo.FolderID;
|
|
return false;
|
|
}
|
|
}
|
|
//private string Xp_LookInAncestorFolderInfo(object sender, XMLPropertiesArgs args)
|
|
//{
|
|
// if (args.AncestorLookup || ParentLookup)
|
|
// {
|
|
// for (FolderInfo folder = _FolderInfo.MyParent; folder != null; folder = folder.MyParent)
|
|
// {
|
|
// string retval = folder.FolderConfig.GetValue(args.Group, args.Item);
|
|
// if (retval != string.Empty) return retval;
|
|
// }
|
|
// }
|
|
// return string.Empty;
|
|
//}
|
|
public FolderConfig(FolderInfo folderInfo)
|
|
{
|
|
_FolderInfo = folderInfo;
|
|
string xml = _FolderInfo.Config;
|
|
if (xml == string.Empty) xml = "<config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public FolderConfig(string xml)
|
|
{
|
|
if (xml == string.Empty) xml = "<config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public FolderConfig()
|
|
{
|
|
_Xp = new XMLProperties("<config/>");
|
|
}
|
|
internal string GetValue(string group, string item)
|
|
{
|
|
return _Xp[group, item];
|
|
}
|
|
#endregion
|
|
#region Local Properties
|
|
[Category("General")]
|
|
[DisplayName("Name")]
|
|
[Description("Name")]
|
|
public string Name
|
|
{
|
|
get { return (_Folder != null ? _Folder.Name : _FolderInfo.Name); }
|
|
set { if (_Folder != null) _Folder.Name = value; }
|
|
}
|
|
//PROPGRID: Hide Title
|
|
[Category("General")]
|
|
[Browsable(false)]
|
|
[DisplayName("Title")]
|
|
[Description("Title")]
|
|
public string Title
|
|
{
|
|
get { return (_Folder != null ? _Folder.Title : _FolderInfo.Title); }
|
|
set { _Folder.Title = value; }
|
|
}
|
|
//PROPGRID: Hide Short Name
|
|
[Category("General")]
|
|
[Browsable(false)]
|
|
[DisplayName("Short Name")]
|
|
[Description("Short Name")]
|
|
public string ShortName
|
|
{
|
|
get { return (_Folder != null ? _Folder.ShortName : _FolderInfo.ShortName); }
|
|
set { if (_Folder != null)_Folder.ShortName = value; }
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Format")]
|
|
[Description("Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string FormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_Folder != null && _Folder.MyFormat != null) return _Folder.MyFormat.FullName;
|
|
if (_FolderInfo != null && _FolderInfo.MyFormat != null) return _FolderInfo.MyFormat.FullName;
|
|
return null;
|
|
}
|
|
set
|
|
{
|
|
if (_Folder != null)
|
|
{
|
|
_Folder.MyFormat = FormatList.ToFormat(value);
|
|
//_Folder.ActiveFormat = null;
|
|
}
|
|
}
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Default Format")]
|
|
[Description("Default Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string DefaultFormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_Folder != null && _Folder.MyFolderInfo.MyParent != null && _Folder.MyFolderInfo.MyParent.ActiveFormat != null) return _Folder.MyFolderInfo.MyParent.ActiveFormat.FullName;
|
|
if (_FolderInfo != null && _FolderInfo.MyParent != null && _FolderInfo.MyParent.ActiveFormat != null) return _FolderInfo.MyParent.ActiveFormat.FullName;
|
|
return null;
|
|
}
|
|
}
|
|
public Folder MyFolder
|
|
{ get { return _Folder; } }
|
|
public FolderInfo MyFolderInfo
|
|
{ get { return _FolderInfo; } }
|
|
#endregion
|
|
#region ToString
|
|
public override string ToString()
|
|
{
|
|
string s = _Xp.ToString();
|
|
if (s == "<config/>" || s == "<config></config>") return string.Empty;
|
|
return s;
|
|
}
|
|
#endregion
|
|
#region GraphicsCategory // From veproms.ini
|
|
public bool CanWrite(string str)
|
|
{
|
|
return true;
|
|
}
|
|
[Category("Referenced Objects")]
|
|
[DisplayName("Graphic File Extension")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default File Extension")]
|
|
public string Graphics_defaultext
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Graphics", "defaultext"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("Graphics", "defaultext"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "TIF";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("Graphics", "defaultext"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "TIF";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["Graphics", "defaultext"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["Graphics", "defaultext"] = value; // save selected value
|
|
|
|
OnPropertyChanged("Graphics_defaultext");
|
|
}
|
|
}
|
|
#endregion
|
|
#region ColorCategory // From veproms.ini
|
|
// ** Note that not all possibilities from 16-bit will be added here, until
|
|
// ** it is determined how these will be used
|
|
// ** If this is used (unhidden), then we need to add logic to blank setting if the value
|
|
// ** we are saving is the same as the parent's value.
|
|
|
|
//PROPGRID: Hide Editor Color for ROs
|
|
[Category("Editor Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Step Editor Colors - Referenced Objects")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Color used to highlight an RO in procedure text")]
|
|
public string Color_ro
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "ro"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "ro"] = value;
|
|
OnPropertyChanged("Color_ro");
|
|
}
|
|
}
|
|
//PROPGRID: Hide Editor Color for Transitions
|
|
[Category("Editor Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Step Editor Colors - Transitions")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Color used to highlight a Transition in procedure text")]
|
|
public string Color_transition
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "transition"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "transition"] = value;
|
|
OnPropertyChanged("Color_transition");
|
|
}
|
|
}
|
|
//PROPGRID: Hide Active Text Background Color
|
|
[Category("Editor Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Step Editor Colors - Active Background")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("editbackground")]
|
|
public string Color_editbackground
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "editbackground"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "editbackground"] = value;
|
|
OnPropertyChanged("Color_editbackground");
|
|
}
|
|
}
|
|
//PROPGRID: Hide color setting for Black
|
|
[Category("Color")]
|
|
[Browsable(false)]
|
|
[DisplayName("black")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("black")]
|
|
public string Color_black
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "black"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "black"] = value;
|
|
OnPropertyChanged("Color_black");
|
|
}
|
|
}
|
|
//PROPGRID: Hide Color Setting for Blue
|
|
[Category("Color")]
|
|
[Browsable(false)]
|
|
[DisplayName("blue")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("blue")]
|
|
public string Color_blue
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "blue"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "blue"] = value;
|
|
OnPropertyChanged("Color_blue");
|
|
}
|
|
}
|
|
#endregion // From veproms.ini
|
|
#region SystemPrintCategory // From veproms.ini
|
|
[Category("Print Settings")]
|
|
[DisplayName("Override Underline Thickness (dots)")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Underline Width")]
|
|
public int SysPrint_UWidth
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "UnderlineWidth"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("SystemPrint", "UnderlineWidth"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return 10;// default to volian default
|
|
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("SystemPrint", "UnderlineWidth"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "10";
|
|
|
|
if (parval.Equals(value.ToString()))
|
|
_Xp["SystemPrint", "UnderlineWidth"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["SystemPrint", "UnderlineWidth"] = value.ToString(); // save selected value
|
|
|
|
OnPropertyChanged("SysPrint_UWidth");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Adjust Starting Print Position (dots)")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Vertical Offset")]
|
|
public int SysPrint_VOffset
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "VerticalOffset"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("SystemPrint", "VerticalOffset"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return 0;// default to volian default
|
|
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("SystemPrint", "VerticalOffset"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "0";
|
|
|
|
if (parval.Equals(value.ToString()))
|
|
_Xp["SystemPrint", "VerticalOffset"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["SystemPrint", "VerticalOffset"] = value.ToString(); // save selected value
|
|
|
|
OnPropertyChanged("SysPrint_VOffset");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Override Normal Pen Width (dots)")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Stroke Width")]
|
|
public int SysPrint_StrokeWidth
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "StrokeWidth"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("SystemPrint", "StrokeWidth"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return 0;// default to volian default
|
|
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("SystemPrint", "StrokeWidth"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "0";
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["SystemPrint", "StrokeWidth"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["SystemPrint", "StrokeWidth"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("SysPrint_StrokeWidth");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Override Bold Pen Width (dots)")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Stroke Width Bold")]
|
|
public int SysPrint_StrokeWidthBold
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "StrokeWidthBold"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("SystemPrint", "StrokeWidthBold"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return 0;// default to volian default
|
|
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("SystemPrint", "StrokeWidthBold"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "0";
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["SystemPrint", "StrokeWidthBold"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["SystemPrint", "StrokeWidthBold"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("SysPrint_StrokeWidthBold");
|
|
}
|
|
}
|
|
#endregion
|
|
#region StartupCategory // From veproms.ini
|
|
//PROPGRID: Hide Startup Message Title
|
|
//PROPGRID: The Startup Grouping is not being used right now, thus not visable.
|
|
[Category("Startup Message")]
|
|
[DisplayName("Startup MessageBox Title")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Startup MessageBox Title")]
|
|
[Browsable(false)]
|
|
public string Startup_MsgTitle
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Startup", "MessageBoxTitle"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Startup", "MessageBoxTitle"] = value;
|
|
OnPropertyChanged("Startup_MsgTitle");
|
|
}
|
|
}
|
|
//PROPGRID: Hide Startup Message File
|
|
[Category("Startup Message")]
|
|
[DisplayName("Startup Message File")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Startup Message File")]
|
|
[Browsable(false)]
|
|
public string Startup_MsgFile
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Startup", "MessageFile"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Startup", "MessageFile"] = value;
|
|
OnPropertyChanged("Startup_MsgFile");
|
|
}
|
|
}
|
|
#endregion
|
|
#region ProcedureListTabStopsCategory // From veproms.ini
|
|
[Category("ProcedureListTabStops")]
|
|
//PROPGRID: Hide Procedure Number Tab
|
|
// ** Note that we will need to add logic it handle the reset to the parent value
|
|
[Browsable(false)]
|
|
[DisplayName("Procedure Number Tab")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Procedure Number Tab")]
|
|
public int ProcListTab_Number
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["ProcedureListTabStops", "ProcedureNumberTab"];
|
|
if (s == string.Empty) return 75;
|
|
return int.Parse(_Xp["ProcedureListTabStops", "ProcedureNumberTab"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["ProcedureListTabStops", "ProcedureNumberTab"] = value.ToString();
|
|
OnPropertyChanged("ProcListTab_Number");
|
|
}
|
|
}
|
|
[Category("ProcedureListTabStops")]
|
|
//PROPGRID: Hide Procedure Title Tab
|
|
// ** Note that we will need to add logic it handle the reset to the parent value
|
|
[Browsable(false)]
|
|
[DisplayName("Procedure Title Tab")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Procedure Title Tab")]
|
|
public int ProcListTab_Title
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["ProcedureListTabStops", "ProcedureTitleTab"];
|
|
if (s == string.Empty) return 175;
|
|
return int.Parse(_Xp["ProcedureListTabStops", "ProcedureTitleTab"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["ProcedureListTabStops", "ProcedureTitleTab"] = value.ToString();
|
|
OnPropertyChanged("ProcListTab_Title");
|
|
}
|
|
}
|
|
#endregion
|
|
#region FormatCategory
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum FormatColumns : int
|
|
{
|
|
[Description("Format Default")]
|
|
Default = 0,
|
|
[Description("Single Column")]
|
|
OneColumn,
|
|
[Description("Dual Column")]
|
|
TwoColumn,
|
|
[Description("Triple Column")]
|
|
ThreeColumn,
|
|
[Description("Quad Column")]
|
|
FourColumns
|
|
}
|
|
[Category("Editor Settings")]
|
|
[DisplayName("Step Editor Columns")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Column Layout")]
|
|
public FormatColumns Format_Columns
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["format", "columns"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("format", "columns"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return FormatColumns.Default; // default to volian default
|
|
|
|
return (FormatColumns)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("format", "columns"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(FormatColumns.Default)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["format", "columns"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["format", "columns"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Format_Columns");
|
|
}
|
|
}
|
|
// This is needed for the Data Loader
|
|
[Category("Format Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Format")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Plant Format")]
|
|
public string Format_Plant
|
|
{
|
|
get
|
|
{
|
|
return _Xp["format", "plant"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["format", "plant"] = value;
|
|
OnPropertyChanged("Format_Plant");
|
|
}
|
|
}
|
|
#endregion
|
|
#region PrintSettingsCategory // From curset.dat
|
|
//PROPGRID: Hide Print Number of Copies
|
|
[Category("Print Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Number of Copies")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Number of Copies")]
|
|
public int Print_NumCopies
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "numcopies"];
|
|
if (s == string.Empty) return 1;
|
|
return int.Parse(_Xp["PrintSettings", "numcopies"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "numcopies"] = value.ToString();
|
|
OnPropertyChanged("Print_NumCopies");
|
|
}
|
|
}
|
|
public enum PrintPagination : int
|
|
{
|
|
Free = 0, Fixed, Auto
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Pagination")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Pagination")]
|
|
public PrintPagination Print_Pagination
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Pagination"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "Pagination"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintPagination.Auto;// default to volian default
|
|
|
|
return (PrintPagination)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "Pagination"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintPagination.Auto)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "Pagination"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "Pagination"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_Pagination");
|
|
}
|
|
}
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum PrintWatermark : int
|
|
{
|
|
None = 0, Reference, Draft, Master, Sample,
|
|
[Description("Information Only")]
|
|
InformationOnly
|
|
}
|
|
|
|
[Category("Print Settings")]
|
|
[DisplayName("Watermark")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Watermark")]
|
|
public PrintWatermark Print_Watermark
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Watermark"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "Watermark"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintWatermark.Draft;// default to volian default
|
|
|
|
return (PrintWatermark)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "Watermark"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintWatermark.Draft)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "Watermark"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "Watermark"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_Watermark");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Disable Automatic Duplexing")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Disable Duplex Printing")]
|
|
public bool Print_DisableDuplex
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "disableduplex"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "disableduplex"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "false";// default to volian default
|
|
|
|
return bool.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "disableduplex"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "False";
|
|
|
|
if (parval.Equals(value.ToString()))
|
|
_Xp["PrintSettings", "disableduplex"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "disableduplex"] = value.ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_DisableDuplex");
|
|
}
|
|
}
|
|
// Change Bar Use from 16-bit code:
|
|
// No Default
|
|
// Without Change Bars
|
|
// With Default Change Bars
|
|
// With User Specified Change Bars
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum PrintChangeBar : int
|
|
{
|
|
[Description("Select Before Printing")]
|
|
SelectBeforePrinting = 0,
|
|
[Description("Without Change Bars")]
|
|
Without,
|
|
[Description("With Default Change Bars")]
|
|
WithDefault,
|
|
[Description("Use Custom Change Bars")]
|
|
WithUserSpecified
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change Bar")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Change Bar Use")]
|
|
public PrintChangeBar Print_ChangeBar
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBar"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBar"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBar.SelectBeforePrinting;// default to volian default
|
|
|
|
return (PrintChangeBar)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBar"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBar.SelectBeforePrinting)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBar"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBar"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBar");
|
|
}
|
|
}
|
|
// User Specified Change Bar Location from16-bit code:
|
|
// With Text
|
|
// Outside Box
|
|
// AER on LEFT, RNO on Right
|
|
// To the Left of Text
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum PrintChangeBarLoc : int
|
|
{
|
|
[Description("With Text")]
|
|
WithText = 0,
|
|
[Description("Outside Box")]
|
|
OutsideBox,
|
|
[Description("AER on Left RNO on Right")]
|
|
AERleftRNOright,
|
|
[Description("To the Left of the Text")]
|
|
LeftOfText
|
|
}
|
|
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change Bar Position")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Specified Change Bar Location")]
|
|
public PrintChangeBarLoc Print_ChangeBarLoc
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarLoc"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBarLoc"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBarLoc.WithText;// default to volian default
|
|
|
|
return (PrintChangeBarLoc)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBarLoc"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBarLoc.WithText)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBarLoc"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBarLoc"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBarLoc");
|
|
}
|
|
}
|
|
|
|
// Change Bar Text from16-bit code:
|
|
// Date and Change ID
|
|
// Revision Number
|
|
// Change ID
|
|
// No Change Bar Message
|
|
// User Defined Message
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum PrintChangeBarText : int
|
|
{
|
|
[Description("Date and Change ID")]
|
|
DateChgID = 0,
|
|
[Description("Revision Number")]
|
|
RevNum,
|
|
[Description("Change ID")]
|
|
ChgID,
|
|
[Description("No Change Bar Text")]
|
|
None,
|
|
[Description("Custom Change Bar Text")]
|
|
UserDef
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change bar Text Type")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Change Bar Text")]
|
|
public PrintChangeBarText Print_ChangeBarText
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarText"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBarText"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBarText.DateChgID;// default to volian default
|
|
|
|
return (PrintChangeBarText)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBarText"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBarText.DateChgID)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBarText"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBarText"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBarText");
|
|
}
|
|
}
|
|
|
|
//PROPGRID: Hide User Format
|
|
// ** If we unhide this, logic to reset to parent setting will need to be added
|
|
[Category("Print Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("User Format")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Format")]
|
|
public string Print_UserFormat
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "userformat"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "userformat"] = value;
|
|
OnPropertyChanged("Print_UserFormat");
|
|
}
|
|
}
|
|
|
|
[Category("Format Settings")]
|
|
[DisplayName("Custom Change Bar Message Line One")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message1")]
|
|
public string Print_UserCBMess1
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "usercbmess1"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "usercbmess1"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "usercbmess1"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["PrintSettings", "usercbmess1"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "usercbmess1"] = value; // save selected value
|
|
|
|
OnPropertyChanged("Print_UserCBMess1");
|
|
}
|
|
}
|
|
|
|
[Category("Format Settings")]
|
|
[DisplayName("Custom Change Bar Message Line Two")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message2")]
|
|
public string Print_UserCBMess2
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "usercbmess2"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "usercbmess2"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "usercbmess2"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["PrintSettings", "usercbmess2"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "usercbmess2"] = value; // save selected value
|
|
|
|
OnPropertyChanged("Print_UserCBMess2");
|
|
}
|
|
}
|
|
#endregion
|
|
#region EditorSettingsCategory
|
|
//[Category("Defaults")]
|
|
//PROPGRID: Hide text background color
|
|
|
|
[Category("Editor Settings")]
|
|
[Browsable(false)]
|
|
[DisplayName("Step Editor Colors - Non Active Background")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Background Color")]
|
|
public Color Default_BkColor
|
|
{
|
|
get
|
|
{
|
|
string sColor = _Xp["default", "BkColor"];
|
|
if (sColor == string.Empty) sColor = "White";
|
|
if (sColor[0] == '[')
|
|
{
|
|
string[] parts = sColor.Substring(1, sColor.Length - 2).Split(",".ToCharArray());
|
|
return Color.FromArgb(Int32.Parse(parts[0]), Int32.Parse(parts[1]), Int32.Parse(parts[2]));
|
|
}
|
|
else return Color.FromName(sColor);
|
|
}
|
|
set
|
|
{
|
|
if (value.IsNamedColor) _Xp["default", "BkColor"] = value.Name;
|
|
else
|
|
{
|
|
_Xp["default", "BkColor"] = string.Format("[{0},{1},{2}]", value.R, value.G, value.B);
|
|
}
|
|
OnPropertyChanged("Default_BkColor");
|
|
}
|
|
}
|
|
#endregion
|
|
#region General Support
|
|
public bool CheckUniqueName(string p)
|
|
{
|
|
FolderInfo parent = FolderInfo.Get(_Folder.ParentID);
|
|
foreach (FolderInfo fi in parent.ChildFolders)
|
|
{
|
|
if (fi.FolderID != _Folder.FolderID && p == fi.Name) return false;
|
|
}
|
|
return true;
|
|
}
|
|
#endregion
|
|
}
|
|
}
|