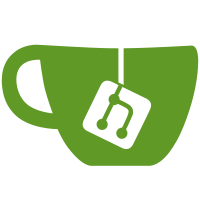
Base MyConfig on the type of item (Procedure, Section or Step) Keep the code from crashing iff the user selects a result in the search window that points to non-editable text
318 lines
11 KiB
C#
318 lines
11 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Controls.Library;
|
|
using System.Windows.Forms;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class StepTabPanel : DevComponents.DotNetBar.PanelDockContainer
|
|
{
|
|
#region Private Fields
|
|
private DisplayTabControl _MyDisplayTabControl;
|
|
private StepTabRibbon _MyStepTabRibbon;
|
|
public StepTabRibbon MyStepTabRibbon
|
|
{
|
|
get { return _MyStepTabRibbon; }
|
|
set { _MyStepTabRibbon = value; }
|
|
}
|
|
private StepPanel _MyStepPanel;
|
|
private DisplayTabItem _MyDisplayTabItem;
|
|
#endregion
|
|
#region Properties
|
|
/// <summary>
|
|
/// Container
|
|
/// </summary>
|
|
public DisplayTabControl MyDisplayTabControl
|
|
{
|
|
get { return _MyDisplayTabControl; }
|
|
//set { _MyDisplayTabControl = value; }
|
|
}
|
|
/// <summary>
|
|
/// StepPanel contained in this control.
|
|
/// </summary>
|
|
public Volian.Controls.Library.StepPanel MyStepPanel
|
|
{
|
|
get { return _MyStepPanel; }
|
|
//set { _MyStepPanel = value; }
|
|
}
|
|
/// <summary>
|
|
/// related DisplayTabItem
|
|
/// </summary>
|
|
public DisplayTabItem MyDisplayTabItem
|
|
{
|
|
get { return _MyDisplayTabItem; }
|
|
set { _MyDisplayTabItem = value; }
|
|
}
|
|
/// <summary>
|
|
/// Currently Selected ItemInfo
|
|
/// </summary>
|
|
public ItemInfo SelectedItemInfo
|
|
{
|
|
get { return _MyStepPanel.SelectedItemInfo; }
|
|
set
|
|
{
|
|
_MyStepPanel.SelectedEditItem = _MyStepPanel.GetEditItem(value);
|
|
if (_MyStepPanel.SelectedEditItem == null)
|
|
{
|
|
MessageBox.Show("The selected item is not available in the editor. One possible cause is that the data is 'not editable', check the section properties 'Editable Data' checkbox.", "Inaccessible Data", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
return;
|
|
}
|
|
if (_MyStepPanel.SelectedEditItem != null)
|
|
_MyStepPanel.SelectedEditItem.ItemSelect();
|
|
if (!_MyStepPanel.ItemSelectionChangeShown) _MyStepPanel.OnItemSelectedChanged(this, new ItemSelectedChangedEventArgs(_MyStepPanel.SelectedEditItem));
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Currently Selected EditItem
|
|
/// </summary>
|
|
public EditItem SelectedEditItem
|
|
{
|
|
get { return _MyStepPanel.SelectedEditItem; }
|
|
}
|
|
/// <summary>
|
|
/// Procedure ItemInfo
|
|
/// </summary>
|
|
public ItemInfo MyProcedureItemInfo
|
|
{
|
|
get { return _MyStepPanel.MyProcedureItemInfo; }
|
|
set { _MyStepPanel.MyProcedureItemInfo = value; }
|
|
}
|
|
private String _SearchString;
|
|
public String SearchString
|
|
{
|
|
get { return _SearchString; }
|
|
set { _SearchString = value; FindSearchString(); }
|
|
}
|
|
public void FindSearchString()
|
|
{
|
|
if (SelectedEditItem == null) return;// Uneditable Data
|
|
if (SelectedEditItem.MyItemInfo.IsTable)
|
|
{
|
|
// if not ro table, just make the mysteprtb active.
|
|
GridItem grd = SelectedEditItem as GridItem;
|
|
grd.SetSearchCell(SearchString);
|
|
}
|
|
// convert the '-' to the unicode non-breaking dash (\u8209? or \u2011 hex)
|
|
if (!SelectedEditItem.MyStepRTB.IsRoTable)
|
|
SelectedEditItem.MyStepRTB.Find(SearchString.Replace('-','\u2011'));
|
|
}
|
|
#endregion
|
|
#region Contructors
|
|
public StepTabPanel(DisplayTabControl myDisplayTabControl)
|
|
{
|
|
_MyDisplayTabControl = myDisplayTabControl;
|
|
InitializeComponent();
|
|
SetupStepTabPanel();
|
|
SetupStepPanel();
|
|
SetupStepTabRibbon();
|
|
}
|
|
#endregion
|
|
#region Private Methods - Setup
|
|
/// <summary>
|
|
/// Setup StepTabRibbon
|
|
/// </summary>
|
|
private void SetupStepTabRibbon()
|
|
{
|
|
_MyStepTabRibbon = new StepTabRibbon();
|
|
_MyStepTabRibbon.Dock = System.Windows.Forms.DockStyle.Top;
|
|
_MyStepTabRibbon.Location = new System.Drawing.Point(0, 0);
|
|
_MyStepTabRibbon.Name = "displayTabRibbon1";
|
|
//_MyTabRibbon.MyDisplayRTB = null;
|
|
_MyStepTabRibbon.MyEditItem = null;
|
|
this.Controls.Add(_MyStepTabRibbon);
|
|
_MyStepTabRibbon.Expanded = _MyDisplayTabControl.RibbonExpanded;
|
|
}
|
|
/// <summary>
|
|
/// Setup this within control
|
|
/// </summary>
|
|
private void SetupStepTabPanel()
|
|
{
|
|
Dock = System.Windows.Forms.DockStyle.Fill;
|
|
this.Enter += new EventHandler(StepTabPanel_Enter);
|
|
}
|
|
/// <summary>
|
|
/// Setup StepPanel
|
|
/// </summary>
|
|
private void SetupStepPanel()
|
|
{
|
|
//this.Font = new Font("Microsoft Sans Serif", 8.25F, FontStyle.Regular, GraphicsUnit.Point, ((byte)(0)));
|
|
_MyStepPanel = new Volian.Controls.Library.StepPanel(this.components);
|
|
this.Controls.Add(_MyStepPanel);
|
|
//
|
|
// _MyPanel
|
|
//
|
|
_MyStepPanel.AutoScroll = true;
|
|
_MyStepPanel.Dock = System.Windows.Forms.DockStyle.Fill;
|
|
_MyStepPanel.LinkClicked +=new Volian.Controls.Library.StepPanelLinkEvent(_MyStepPanel_LinkClicked);
|
|
_MyStepPanel.LinkActiveChanged += new Volian.Controls.Library.StepPanelLinkEvent(_MyStepPanel_LinkActiveChanged);
|
|
_MyStepPanel.LinkInsertTran += new StepPanelLinkEvent(_MyStepPanel_LinkInsertTran);
|
|
_MyStepPanel.LinkInsertRO += new StepPanelLinkEvent(_MyStepPanel_LinkInsertRO);
|
|
_MyStepPanel.LinkModifyTran += new StepPanelLinkEvent(_MyStepPanel_LinkModifyTran);
|
|
_MyStepPanel.LinkModifyRO += new StepPanelLinkEvent(_MyStepPanel_LinkModifyRO);
|
|
_MyStepPanel.ItemClick +=new Volian.Controls.Library.StepPanelEvent(_MyStepPanel_ItemClick);
|
|
_MyStepPanel.AttachmentClicked += new Volian.Controls.Library.StepPanelAttachmentEvent(_MyStepPanel_AttachmentClicked);
|
|
_MyStepPanel.ItemSelectedChanged += new ItemSelectedChangedEvent(_MyStepPanel_ItemSelectedChanged);
|
|
//_MyStepPanel.ModeChange += new Volian.Controls.Library.StepPanelModeChangeEvent(_MyStepPanel_ModeChange);
|
|
_MyStepPanel.TabDisplay += new Volian.Controls.Library.StepPanelTabDisplayEvent(_MyStepPanel_TabDisplay);
|
|
_MyStepPanel.WordSectionClose += new StepPanelWordSectionCloseEvent(_MyStepPanel_WordSectionClose);
|
|
_MyStepPanel.WordSectionDeleted += new StepPanelWordSectionDeletedEvent(_MyStepPanel_WordSectionDeleted);
|
|
_MyStepPanel.ItemPasted += new StepPanelItemPastedEvent(_MyStepPanel_ItemPasted);
|
|
}
|
|
|
|
|
|
#endregion
|
|
#region Event Handlers
|
|
private bool _ShowingItem = false;
|
|
public bool ShowingItem
|
|
{
|
|
get { return _ShowingItem; }
|
|
set { _ShowingItem = value; }
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user clicks on a StepTabPanel
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void StepTabPanel_Enter(object sender, EventArgs e)
|
|
{
|
|
if (_ShowingItem) return;
|
|
_ShowingItem = true;
|
|
//if (ItemSelected != null)
|
|
_MyStepPanel.ItemShow();
|
|
_MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetUpdRoValBtn(_MyStepPanel.MyStepTabPanel.MyStepTabRibbon.NewerRoFst());
|
|
_ShowingItem = false;
|
|
_MyDisplayTabControl.SelectedDisplayTabItem = MyDisplayTabItem;
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the cursor moves onto or off of a link
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkActiveChanged(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnLinkActiveChanged(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to add a transition
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkInsertTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnLinkInsertTran(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to add an RO
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkInsertRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnLinkInsertRO(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chosses to modify a transition
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnLinkModifyTran(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to Modify an RO
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnLinkModifyRO(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the Selected Item changes
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_ItemSelectedChanged(object sender, ItemSelectedChangedEventArgs args)
|
|
{
|
|
_MyStepTabRibbon.MyEditItem = args.MyEditItem;
|
|
_MyDisplayTabControl.OnItemSelectedChanged(sender, args);
|
|
}
|
|
// Occurs when the Mode Changes
|
|
//void _MyStepPanel_ModeChange(object sender, StepRTBModeChangeEventArgs args)
|
|
//{
|
|
// _MyDisplayTabControl.OnModeChange(sender, args);
|
|
//}
|
|
|
|
/// <summary>
|
|
/// Occurs when the user clicks on the Attachment Expander
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private ItemInfo curDSOItem = null;
|
|
void _MyStepPanel_AttachmentClicked(object sender, StepPanelAttachmentEventArgs args)
|
|
{
|
|
if (curDSOItem != null) return;
|
|
curDSOItem = args.MyEditItem.MyItemInfo;
|
|
_MyDisplayTabControl.OpenItem(args.MyEditItem.MyItemInfo);
|
|
curDSOItem = null;
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user clicks on the tab next to an item
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_ItemClick(object sender, StepPanelEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnItemClick(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user clicks on an item
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void _MyStepPanel_LinkClicked(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (args.MyLinkText.LinkInfoText.IndexOf("Transition") > -1)
|
|
{
|
|
ItemInfo item = args.MyLinkText.MyTranToItemInfo;
|
|
if (item == null || item.MyDocVersion == null || (item.PreviousID == null && item.ItemPartCount == 0 && item.ItemDocVersionCount == 0))
|
|
{
|
|
MessageBox.Show("This transition is invalid", "Invalid Transition", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
return; // Not a valid transition
|
|
}
|
|
_MyDisplayTabControl.OpenItem(item);
|
|
}
|
|
else
|
|
Console.WriteLine("Bring Up roeditor"); //TODO: Need to bring up roeditor or infopanel
|
|
}
|
|
void _MyStepPanel_TabDisplay(object sender, StepPanelTabDisplayEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnPanelTabDisplay(sender, args);
|
|
}
|
|
void _MyStepPanel_WordSectionClose(object sender, WordSectionEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnWordSectionClose(sender, args);
|
|
}
|
|
void _MyStepPanel_WordSectionDeleted(object sender, WordSectionEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnWordSectionDeleted(sender, args);
|
|
}
|
|
void _MyStepPanel_ItemPasted(object sender, vlnTreeItemInfoPasteEventArgs args)
|
|
{
|
|
_MyDisplayTabControl.OnItemPaste(sender, args);
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return string.Format("StepTabPanel Procedure Item {0} {1}",
|
|
MyDisplayTabItem.MyItemInfo.ItemID, MyDisplayTabItem.MyItemInfo.DisplayNumber);
|
|
}
|
|
#endregion
|
|
}
|
|
}
|