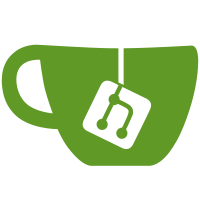
- If the range does not have any characters return an empty string Refresh Most Recent Items when the office button is pressed Added OrdinalChanged Event Used OrdinalChanged Event rather than MyContent.Changed Event when Ordinal is updated. Use OrdinalChanged Event to update Tree Node Refresh MRU list as items are added Use OrdinalChanged Event to update Step Tab - RTBFillIn changed to only update the RTB when the contents change - AdjustSizeForContents changed to raise the HeightChanged event when the Height changes Update the treeview tabs even if the step is not open.
226 lines
6.1 KiB
C#
226 lines
6.1 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Windows.Forms;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public delegate void MostRecentItemEvent();
|
|
|
|
public class MostRecentItemList : List<MostRecentItem>
|
|
{
|
|
public event ItemInfoEvent AfterRemove;
|
|
internal void OnAfterRemove(object sender)
|
|
{
|
|
if (AfterRemove != null) AfterRemove(sender);
|
|
}
|
|
private int _MaxItems = 10;
|
|
public int MaxItems
|
|
{
|
|
get { return _MaxItems; }
|
|
set { _MaxItems = value; }
|
|
}
|
|
public new MostRecentItem Add(MostRecentItem myMRI)
|
|
{
|
|
Refresh();
|
|
MostRecentItem tmp = null;
|
|
// Look for the ItemID
|
|
foreach (MostRecentItem mri in this)
|
|
if (mri.ItemID == myMRI.ItemID)
|
|
tmp = mri;
|
|
// If it exists - remove it
|
|
if (tmp != null)
|
|
{
|
|
tmp.MyItemInfo.BeforeDelete -= new ItemInfoEvent(MyItemInfo_BeforeDelete);
|
|
Remove(tmp);
|
|
}
|
|
// Insert it in the first place in the list
|
|
Insert(0, myMRI);
|
|
// If more than MaxItems exist remove the items beyond MaxItems
|
|
if (MaxItems > 0)
|
|
while (Count > MaxItems) RemoveAt(MaxItems);
|
|
myMRI.MyItemInfo.BeforeDelete += new ItemInfoEvent(MyItemInfo_BeforeDelete);
|
|
return myMRI;
|
|
}
|
|
public void Refresh()
|
|
{
|
|
foreach (MostRecentItem mri in this)
|
|
mri.Refresh();
|
|
}
|
|
|
|
// delete the item from the most recently used list. This event gets handled
|
|
// when a delete item occurs.
|
|
void MyItemInfo_BeforeDelete(object sender)
|
|
{
|
|
ItemInfo ii = sender as ItemInfo;
|
|
if (ii != null)
|
|
{
|
|
MostRecentItem tmp = null;
|
|
// Look for the ItemID
|
|
foreach (MostRecentItem mri in this)
|
|
if (mri.ItemID == ii.ItemID)
|
|
tmp = mri;
|
|
// If it exists - remove it
|
|
if (tmp != null)
|
|
Remove(tmp);
|
|
OnAfterRemove(null);
|
|
}
|
|
}
|
|
public MostRecentItem Add(int itemID)
|
|
{
|
|
ItemInfo tmp = ItemInfo.Get(itemID);
|
|
if (tmp == null)
|
|
{
|
|
MessageBox.Show("This step no longer exists", "Invalid Most Recent Item", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
return null;
|
|
}
|
|
return Add(new MostRecentItem(tmp));
|
|
}
|
|
public MostRecentItem Add(ItemInfo myItem)
|
|
{
|
|
return Add(new MostRecentItem(myItem));
|
|
}
|
|
public MostRecentItem Add(string s)
|
|
{
|
|
MostRecentItem mri = MostRecentItem.ParseString(s);
|
|
if(mri != null)
|
|
Add(mri);
|
|
return mri;
|
|
}
|
|
public MostRecentItem Add(IVEDrillDownReadOnly myDrillDown)
|
|
{
|
|
if (typeof(ItemInfo).IsAssignableFrom(myDrillDown.GetType()))
|
|
return Add(new MostRecentItem((ItemInfo)myDrillDown));
|
|
return null;
|
|
}
|
|
public static MostRecentItemList GetMRILst(System.Collections.Specialized.StringCollection list)
|
|
{
|
|
return GetMRILst(list, 10);
|
|
}
|
|
|
|
public static MostRecentItemList GetMRILst(System.Collections.Specialized.StringCollection list, int maxItems)
|
|
{
|
|
MostRecentItemList mril = new MostRecentItemList();
|
|
mril.MaxItems = maxItems;
|
|
if (list != null)
|
|
for (int i = list.Count - 1; i >= 0;i-- )// Add in reverse order so first is last and last is first
|
|
mril.Add(list[i]);
|
|
return mril;
|
|
}
|
|
public System.Collections.Specialized.StringCollection ToSettings()
|
|
{
|
|
if (Count == 0) return null;
|
|
Refresh();
|
|
System.Collections.Specialized.StringCollection retval = new System.Collections.Specialized.StringCollection();
|
|
foreach (MostRecentItem mri in this)
|
|
retval.Add(mri.ToString());
|
|
return retval;
|
|
}
|
|
} // Class
|
|
public class MostRecentItem
|
|
{
|
|
[NonSerialized]
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get
|
|
{
|
|
if (_MyItemInfo == null)
|
|
_MyItemInfo = ItemInfo.Get(_ItemID);
|
|
return _MyItemInfo;
|
|
}
|
|
set
|
|
{
|
|
_ItemID = value.ItemID;
|
|
_MyItemInfo = value;
|
|
_MenuTitle = GetMenuTitle();
|
|
_ToolTip = GetToolTip(_MyItemInfo);
|
|
}
|
|
}
|
|
internal void Refresh()
|
|
{
|
|
MyItemInfo = MyItemInfo;
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
private string _MenuTitle;
|
|
public string MenuTitle
|
|
{
|
|
get
|
|
{
|
|
if (_MenuTitle == null)
|
|
_MenuTitle = GetMenuTitle();
|
|
return _MenuTitle;
|
|
}
|
|
set { _MenuTitle = value; }
|
|
}
|
|
private string GetMenuTitle()
|
|
{
|
|
return MyItemInfo.Path;
|
|
}
|
|
private string _ToolTip;
|
|
public string ToolTip
|
|
{
|
|
get
|
|
{
|
|
if (_ToolTip == null)
|
|
_ToolTip = GetToolTip(MyItemInfo);
|
|
return _ToolTip;
|
|
}
|
|
set { _ToolTip = value; }
|
|
}
|
|
private static string GetToolTip(ItemInfo item)
|
|
{
|
|
// reset active parent if null
|
|
// if (MyItemInfo.MyProcedure.ActiveParent == null) MyItemInfo.MyProcedure.ActiveParent = null;
|
|
DocVersionInfo tmp = (DocVersionInfo)(item.MyProcedure.ActiveParent);
|
|
StringBuilder sb = new StringBuilder();
|
|
int indent = BuildPath(tmp.MyFolder, ref sb);
|
|
return sb.ToString();
|
|
}
|
|
private static int BuildPath(FolderInfo folderInfo, ref StringBuilder sb)
|
|
{
|
|
if (folderInfo.MyParent == null) return 0;
|
|
int indent = BuildPath(folderInfo.MyParent, ref sb);
|
|
sb.Append(string.Format("{0}{1}\n", "".PadLeft(indent * 2), folderInfo.Name));
|
|
return indent;
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0}~{1}~{2}", _ItemID, MenuTitle, ToolTip);
|
|
}
|
|
public static MostRecentItem ParseString(string str)
|
|
{
|
|
string[] parts = str.Split("~".ToCharArray());
|
|
int itemID = int.Parse(parts[0]);
|
|
ItemInfo item = ItemInfo.Get(itemID);
|
|
if (item == null) return null;
|
|
if (parts.Length > 1 && parts[1] != item.Path && !RetainBadMRIs())
|
|
return null; // Path doesn't match
|
|
if (parts.Length > 2 && parts[2] != GetToolTip(item) && !RetainBadMRIs())
|
|
return null;// Tooltip doesn't match
|
|
//Console.WriteLine("{0},'{1}'", parts[0], parts[1]);
|
|
return new MostRecentItem(item);
|
|
}
|
|
private static int _RetainBadMRIs = 0;
|
|
private static bool RetainBadMRIs()
|
|
{
|
|
if (_RetainBadMRIs == 0)
|
|
{
|
|
System.Windows.Forms.DialogResult result = System.Windows.Forms.MessageBox.Show("Do you want to retain old Recently Used Entries?", "Retain Recent Locations", System.Windows.Forms.MessageBoxButtons.YesNo, System.Windows.Forms.MessageBoxIcon.Question);
|
|
_RetainBadMRIs = result == System.Windows.Forms.DialogResult.Yes ? 2 : 1;
|
|
}
|
|
return _RetainBadMRIs == 2;
|
|
}
|
|
public MostRecentItem(ItemInfo myItem)
|
|
{
|
|
MyItemInfo = myItem;
|
|
}
|
|
}
|
|
}
|