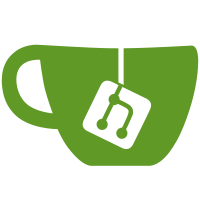
Removed the message box from the search save button which was incorreclt stateing that the output was saved to the clipboard.
2220 lines
76 KiB
C#
2220 lines
76 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using DevComponents.DotNetBar;
|
|
using DevComponents.AdvTree;
|
|
using Volian.Base.Library;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplaySearch : UserControl
|
|
{
|
|
#region Events
|
|
public event DisplaySearchEvent PrintRequest;
|
|
private void OnPrintRequest(DisplaySearchEventArgs args)
|
|
{
|
|
if (PrintRequest != null)
|
|
PrintRequest(this, args);
|
|
}
|
|
public event DisplaySearchEvent SearchComplete;
|
|
private void OnSearchComplete(DisplaySearchEventArgs args)
|
|
{
|
|
if (SearchComplete != null)
|
|
SearchComplete(this, args);
|
|
}
|
|
#endregion
|
|
#region Properties
|
|
private string _strSrchText = "";
|
|
private List<DocVersionInfo> lstCheckedDocVersions = new List<DocVersionInfo>();
|
|
private List<int> lstCheckedStepTypes = new List<int>();
|
|
private List<string> lstCheckedStepTypesStr = new List<string>();
|
|
private AnnotationDetails _AnnotationDetails = null;
|
|
ItemInfo _ItemInfo = null;
|
|
private DisplayTabControl _TabControl;
|
|
private DocVersionInfo _MyDocVersion;
|
|
private Color saveXpSetToSearchColor;
|
|
private Color saveXpStepTypeTitleColor;
|
|
private Color saveGpSrchAnnoTextColor;
|
|
private Color saveGpSrchTextColor;
|
|
private Color saveGpFindROsColor;
|
|
private Color saveGrpPanSearchResults;
|
|
private ItemInfoList _SearchResults;
|
|
public ItemInfoList SearchResults
|
|
{
|
|
get { return _SearchResults; }
|
|
set
|
|
{
|
|
_SearchResults = value;
|
|
if (value != null) // Don't select an item from the list when it is updated
|
|
_SearchResults.ListChanged += new ListChangedEventHandler(_SearchResults_ListChanged);
|
|
DisplayResults();
|
|
}
|
|
}
|
|
void _SearchResults_ListChanged(object sender, ListChangedEventArgs e)
|
|
{
|
|
lbSrchResults.SelectedIndex = -1; // Don't select an item from the new list
|
|
}
|
|
|
|
private string _DisplayMember = "SearchPath";
|
|
|
|
//public string Status
|
|
//{
|
|
// get { return tsslStatus.Text; }
|
|
// set { tsslStatus.Text = value; Application.DoEvents(); }
|
|
//}
|
|
|
|
private bool _OpenDocFromSearch;
|
|
|
|
public bool OpenDocFromSearch
|
|
{
|
|
get { return _OpenDocFromSearch; }
|
|
//set { _OpenDocFromSearch = value; }
|
|
}
|
|
public DocVersionInfo Mydocversion
|
|
{
|
|
get { return _MyDocVersion; }
|
|
set
|
|
{
|
|
_MyDocVersion = value;
|
|
if (_MyDocVersion != null)
|
|
{
|
|
if (_MyDocVersion.DocVersionAssociationCount > 0)
|
|
{
|
|
// if the count variable is not consistent with the actual list count,
|
|
// do a refresh. There was a bug, B2012-040, that was not reproducable..
|
|
// so this is an attempt to fix it.
|
|
if (_MyDocVersion.DocVersionAssociations.Count == 0)
|
|
{
|
|
_MyDocVersion.RefreshDocVersionAssociations();
|
|
if (_MyDocVersion.DocVersionAssociations.Count == 0) return;
|
|
}
|
|
MyROFSTLookup = _MyDocVersion.DocVersionAssociations[0].MyROFst.GetROFSTLookup(_MyDocVersion);
|
|
_MyRODbID = _MyDocVersion.DocVersionAssociations[0].MyROFst.RODbID;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
private ROFSTLookup _MyROFSTLookup;
|
|
public ROFSTLookup MyROFSTLookup
|
|
{
|
|
get { return _MyROFSTLookup; }
|
|
set
|
|
{
|
|
//if (!Visible) return; // don't reset anything if the form is invisible.
|
|
_MyROFSTLookup = value; // define the tree nodes based on this rofst
|
|
LoadROComboTree();
|
|
}
|
|
}
|
|
private bool _LoadingList = false;
|
|
public bool LoadingList
|
|
{
|
|
get { return _LoadingList; }
|
|
set { _LoadingList = value; }
|
|
}
|
|
|
|
private int LastResultsMouseOverIndex = -1;
|
|
|
|
private ProgressBarItem _ProgressBar;
|
|
|
|
public ProgressBarItem ProgressBar
|
|
{
|
|
get { return _ProgressBar; }
|
|
set { _ProgressBar = value; }
|
|
}
|
|
#endregion
|
|
|
|
#region setup
|
|
public DisplaySearch()
|
|
{
|
|
InitializeComponent();
|
|
LoadSearchTextListBox();
|
|
saveXpSetToSearchColor = xpSetToSearch.TitleStyle.BackColor1.Color;
|
|
saveXpStepTypeTitleColor = xpStepTypes.TitleStyle.BackColor1.Color;
|
|
saveGpSrchAnnoTextColor = gpSrchAnnoText.Style.BackColor;
|
|
saveGpSrchTextColor = gpSrchText.Style.BackColor;
|
|
saveGpFindROsColor = gpFindROs.Style.BackColor;
|
|
gpSrchText.Style.BackColor = Color.Yellow;
|
|
saveGrpPanSearchResults = grpPanSearchResults.Style.BackColor;
|
|
|
|
// start out with the procedure and type selection windows colasped
|
|
xpSetToSearch.Expanded = false;
|
|
xpStepTypes.Expanded = false;
|
|
rbtnSrchTxt.Checked = true;
|
|
gpSrchAnnoText.Enabled = true;
|
|
cmbResultsStyle.Enabled = false;
|
|
tabSearchTypes.SelectedTabChanged += new TabStrip.SelectedTabChangedEventHandler(tabSearchTypes_SelectedTabChanged);
|
|
// Don't do this here. If you do then the /DB parameter has not yet been set, which will cause the database menu to be displayed
|
|
//SetupContextMenu(); // so that the symbol list is available without selecting a procedure set or procedure
|
|
}
|
|
|
|
void tabSearchTypes_SelectedTabChanged(object sender, TabStripTabChangedEventArgs e)
|
|
{
|
|
if (e.NewTab == tabTranSearch)
|
|
{
|
|
//enable-disable doc version nodes based on version type if selected
|
|
if (cbxTranVersion.SelectedIndex > -1)
|
|
{
|
|
if (dicExpandedDocVersionNodes.Count > 0)
|
|
{
|
|
foreach (DevComponents.AdvTree.Node n in dicExpandedDocVersionNodes.Keys)
|
|
{
|
|
DocVersionInfo dvi = (DocVersionInfo)n.Tag;
|
|
if(cbxTranVersion.Tag.ToString().Contains(dvi.VersionID.ToString()))
|
|
n.Enabled = true;
|
|
else
|
|
n.Enabled = false;
|
|
}
|
|
}
|
|
}
|
|
//setup transition format versions
|
|
this.Cursor = Cursors.WaitCursor;
|
|
if (myFormatVersionList == null)
|
|
{
|
|
this.Refresh();
|
|
myFormatVersionList = FormatVersionList.GetFormatVersions();
|
|
cbxTranVersion.DisplayMember = "Title";
|
|
int lastLastFormatID = 0;
|
|
foreach (FormatVersion fv in myFormatVersionList)
|
|
{
|
|
if (fv.FormatID != lastLastFormatID)
|
|
{
|
|
cbxTranVersion.Items.Add(fv);
|
|
lastLastFormatID = fv.FormatID;
|
|
}
|
|
}
|
|
cbxTranVersion.SelectedIndexChanged += new EventHandler(cbxTranVersion_SelectedIndexChanged);
|
|
cbxTranFormat.SelectedIndexChanged += new EventHandler(cbxTranFormat_SelectedIndexChanged);
|
|
}
|
|
this.Cursor = Cursors.Default;
|
|
}
|
|
else
|
|
{
|
|
foreach (DevComponents.AdvTree.Node n in dicExpandedDocVersionNodes.Keys)
|
|
n.Enabled = true;
|
|
if (dicSelectedDocVersionNodes.Count > 0)
|
|
{
|
|
foreach (DevComponents.AdvTree.Node n in dicSelectedDocVersionNodes.Keys)
|
|
{
|
|
n.Enabled = true;
|
|
}
|
|
dicSelectedDocVersionNodes.Clear();
|
|
}
|
|
}
|
|
}
|
|
|
|
void cbxTranFormat_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
#region 1st cut
|
|
//if (cbxTranFormat.SelectedItem.ToString() == "All")
|
|
//{
|
|
// cbxTranCategory.Items.Clear();
|
|
// cbxTranCategory.Items.Add("All");
|
|
// cbxTranCategory.Items.Add("Internal");
|
|
// cbxTranCategory.Items.Add("External");
|
|
// cbxTranCategory.Items.Add("Outside");
|
|
// cbxTranCategory.SelectedIndex = 0;
|
|
//}
|
|
//else if (cbxTranFormat.SelectedItem.ToString().Contains("{Proc"))
|
|
//{
|
|
// cbxTranCategory.Items.Clear();
|
|
// cbxTranCategory.Items.Add("All");
|
|
// cbxTranCategory.Items.Add("External");
|
|
// cbxTranCategory.Items.Add("Outside");
|
|
// cbxTranCategory.SelectedIndex = 0;
|
|
//}
|
|
//else
|
|
//{
|
|
// cbxTranCategory.Items.Clear();
|
|
// cbxTranCategory.Items.Add("Internal");
|
|
// cbxTranCategory.SelectedIndex = 0;
|
|
//}
|
|
#endregion
|
|
#region 2nd cut
|
|
if (cbxTranFormat.SelectedItem.ToString().Contains("{Proc"))
|
|
{
|
|
cbxTranCategory.Items.Clear();
|
|
cbxTranCategory.Items.Add("All");
|
|
cbxTranCategory.Items.Add("External");
|
|
cbxTranCategory.Items.Add("Outside");
|
|
cbxTranCategory.SelectedIndex = 0;
|
|
}
|
|
else
|
|
{
|
|
cbxTranCategory.Items.Clear();
|
|
cbxTranCategory.Items.Add("All");
|
|
cbxTranCategory.Items.Add("Internal");
|
|
cbxTranCategory.Items.Add("External");
|
|
cbxTranCategory.Items.Add("Outside");
|
|
cbxTranCategory.SelectedIndex = 0;
|
|
}
|
|
#endregion
|
|
}
|
|
FormatVersionList myFormatVersionList;
|
|
private void LoadSearchTextListBox()
|
|
{
|
|
// Setup SearchText Combo
|
|
cbxTextSearchText.Items.Clear();
|
|
if (Properties.Settings.Default["SearchList"] != null && Properties.Settings.Default.SearchList.Count > 0)
|
|
{
|
|
foreach (string str in Properties.Settings.Default.SearchList)
|
|
cbxTextSearchText.Items.Add(str);
|
|
}
|
|
// Setup SearchAnnotation Combo
|
|
cbxTextSearchAnnotation.Items.Clear();
|
|
if (Properties.Settings.Default["SearchAList"] != null && Properties.Settings.Default.SearchAList.Count > 0)
|
|
{
|
|
foreach (string str in Properties.Settings.Default.SearchAList)
|
|
cbxTextSearchAnnotation.Items.Add(str);
|
|
}
|
|
}
|
|
|
|
void cbxTranVersion_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
this.Cursor = Cursors.WaitCursor;
|
|
string versionList = string.Empty;
|
|
string sep = string.Empty;
|
|
FormatVersion sfv = cbxTranVersion.SelectedItem as FormatVersion;
|
|
foreach (FormatVersion fv in myFormatVersionList)
|
|
{
|
|
if (fv.FormatID == sfv.FormatID)
|
|
{
|
|
versionList += sep + fv.VersionID;
|
|
sep = ",";
|
|
}
|
|
}
|
|
cbxTranVersion.Tag = versionList;
|
|
cbxTranFormatFillIn(versionList);
|
|
if (dicExpandedDocVersionNodes.Count > 0)
|
|
{
|
|
foreach (DevComponents.AdvTree.Node n in dicExpandedDocVersionNodes.Keys)
|
|
{
|
|
DocVersionInfo dvi = (DocVersionInfo)n.Tag;
|
|
if (cbxTranVersion.Tag.ToString().Contains(dvi.VersionID.ToString()))
|
|
n.Enabled = true;
|
|
else
|
|
n.Enabled = false;
|
|
}
|
|
}
|
|
|
|
List<DevComponents.AdvTree.Node> uncheckNodes = new List<Node>();
|
|
foreach (DevComponents.AdvTree.Node n in dicSelectedDocVersionNodes.Keys)
|
|
{
|
|
DocVersionInfo dvi = (DocVersionInfo)n.Tag;
|
|
if (!versionList.Contains(dvi.VersionID.ToString()))
|
|
uncheckNodes.Add(n);
|
|
else
|
|
n.Enabled = true;
|
|
}
|
|
while (uncheckNodes.Count > 0)
|
|
{
|
|
uncheckNodes[0].Checked = false;
|
|
uncheckNodes[0].Enabled = false;
|
|
uncheckNodes.Remove(uncheckNodes[0]);
|
|
}
|
|
if (lstCheckedDocVersions.Count > 0)
|
|
{
|
|
if (MessageBox.Show(this, "Do you want to expand to all relevant procedure sets for the selected format?", "Expand Procedure Sets", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
lstCheckedDocVersions.Clear();
|
|
foreach (DevComponents.AdvTree.Node n in dicSelectedDocVersionNodes.Keys)
|
|
uncheckNodes.Add(n);
|
|
while (uncheckNodes.Count > 0)
|
|
{
|
|
uncheckNodes[0].Checked = false;
|
|
uncheckNodes.Remove(uncheckNodes[0]);
|
|
}
|
|
dicSelectedDocVersionNodes.Clear();
|
|
}
|
|
}
|
|
this.Cursor = Cursors.Default;
|
|
}
|
|
|
|
private DevComponents.AdvTree.Node NewAdvTreeNode(string nodetext, bool selectable, bool chxbxvisable)
|
|
{
|
|
DevComponents.AdvTree.Node newnode;
|
|
newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = nodetext;
|
|
newnode.Selectable = selectable;
|
|
newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
newnode.CheckBoxThreeState = false;
|
|
newnode.CheckBoxVisible = chxbxvisable;
|
|
return newnode;
|
|
}
|
|
|
|
public void advTreeStepTypesFillIn()
|
|
{
|
|
DevComponents.AdvTree.Node topnode = new DevComponents.AdvTree.Node();
|
|
advTreeStepTypes.Nodes.Clear();
|
|
lstCheckedStepTypes.Clear();
|
|
lstCheckedStepTypesStr.Clear();
|
|
topnode.Text = "Types";
|
|
advTreeStepTypes.Nodes.Add(topnode);
|
|
|
|
FormatData fmtdata = _MyDocVersion.ActiveFormat.PlantFormat.FormatData;
|
|
List<StepDataRetval> lstSrchStpTypes = fmtdata.GetSearchableSteps(); // list of searchable step types
|
|
if (lstSrchStpTypes != null && lstSrchStpTypes.Count > 0)
|
|
{
|
|
DevComponents.AdvTree.Node newnode;
|
|
advTreeStepTypes.Nodes.Clear();
|
|
// Add a dummy node for searching Accessory Sections (MS Word sections)
|
|
//newnode = new DevComponents.AdvTree.Node();
|
|
//newnode.Text = "Accessory Sections";
|
|
//newnode.Selectable = true;
|
|
//newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
//newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
//newnode.CheckBoxThreeState = false;
|
|
//newnode.CheckBoxVisible = true;
|
|
newnode = NewAdvTreeNode("Accessory Sections", true, true);
|
|
advTreeStepTypes.Nodes.Add(newnode);
|
|
foreach (StepDataRetval sdr in lstSrchStpTypes)
|
|
{
|
|
StepDataList sdl = fmtdata.StepDataList; ;
|
|
StepData sd = sdl[sdr.Index]; // get the step type record
|
|
string parentName = sd.ParentType; // this gets the parent of the this step/substep type
|
|
|
|
//Console.WriteLine("{0} {1} - {2}", parentName,sd.Type,sdr.Name);
|
|
//newnode = new DevComponents.AdvTree.Node();
|
|
//newnode.Text = sdr.Name;
|
|
//newnode.Name = sd.Type; // this needed for the FindNodeByName() function
|
|
//newnode.Tag = sd;
|
|
//newnode.Selectable = true;
|
|
//newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
//newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
//newnode.CheckBoxThreeState = false;
|
|
//newnode.CheckBoxVisible = true;
|
|
if (parentName.Equals("Base"))
|
|
{
|
|
newnode = NewAdvTreeNode(sdr.Name, true, true);
|
|
newnode.Tag = sd;
|
|
newnode.Name = sd.Type;
|
|
DevComponents.AdvTree.Node pnode = NewAdvTreeNode(sd.Type, true, false); //create parent node (non selectable)
|
|
pnode.Name = sd.Type;
|
|
if (!sd.Type.Equals("Title")) // only use Title as a tree grouping to put Title with Text Right/Below substep types
|
|
pnode.Nodes.Add(newnode);
|
|
pnode.Nodes.Sort();
|
|
advTreeStepTypes.Nodes.Add(pnode);
|
|
}
|
|
else
|
|
{
|
|
newnode = NewAdvTreeNode(sdr.Name, true, true);
|
|
newnode.Name = sd.Type; // this needed for the FindNodeByName() function
|
|
newnode.Tag = sd;
|
|
|
|
// if the parent node exists in the tree, then add the new node to that parent
|
|
// else, it is a parent node, so add it as a new parent
|
|
|
|
DevComponents.AdvTree.Node parnode = advTreeStepTypes.FindNodeByName(parentName);
|
|
// Group by High Level Steps, Substeps, Cautions, Notes, Figures, Tables
|
|
while (!parentName.Equals("Base") && !parentName.Equals("Substep") && !parentName.Equals("High") &&
|
|
!parentName.Equals("Caution") && !parentName.Equals("Note") && !parentName.Equals("Table") &&
|
|
!parentName.Equals("Figure") && !parentName.Equals("Title") &&
|
|
!parentName.Equals("And") && !parentName.Equals("Or") && !parentName.Equals("Paragraph"))
|
|
{
|
|
StepData tmpsd = (StepData)parnode.Tag;
|
|
parentName = tmpsd.ParentType;
|
|
parnode = advTreeStepTypes.FindNodeByName(parentName);
|
|
}
|
|
if (parnode != null)
|
|
{
|
|
parnode.Nodes.Add(newnode);
|
|
parnode.Nodes.Sort(); // sort the second level of the tree
|
|
}
|
|
else
|
|
advTreeStepTypes.Nodes.Add(newnode);
|
|
}
|
|
}
|
|
advTreeStepTypes.Nodes.Sort(); // sort the first level of the tree
|
|
}
|
|
buildStepTypePannelTitle();
|
|
}
|
|
private int _TopFolderID = 1;
|
|
|
|
public int TopFolderID
|
|
{
|
|
get { return _TopFolderID; }
|
|
set { _TopFolderID = value; }
|
|
}
|
|
public void advTreeProcSetsFillIn(bool blSeachTabClicked)
|
|
{
|
|
DevComponents.AdvTree.Node topnode = null;
|
|
int cntnd = 0;
|
|
VETreeNode vtn = VETreeNode.GetFolder(TopFolderID);
|
|
FolderInfo fi = vtn.VEObject as FolderInfo;
|
|
int fiCount = fi.ChildFolderCount;
|
|
advTreeProcSets.Nodes.Clear();
|
|
lstCheckedDocVersions.Clear();
|
|
topnode = new DevComponents.AdvTree.Node();
|
|
topnode.Text = "Available Procedure Sets";
|
|
topnode.Tag = fi;
|
|
advTreeProcSets.Nodes.Add(topnode);
|
|
//advTreeProcSets.AfterNodeInsert += new TreeNodeCollectionEventHandler(advTreeProcSets_AfterNodeInsert);
|
|
|
|
if (fi.ChildFolders != null)
|
|
{
|
|
foreach (FolderInfo fic in fi.ChildFolders)
|
|
{
|
|
DevComponents.AdvTree.Node newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = fic.ToString();
|
|
newnode.Tag = fic;
|
|
|
|
//int tmp;
|
|
//if (topnode == null)
|
|
//{
|
|
// newnode.Text = "Available Procedure Sets";
|
|
// tmp = advTreeProcSets.Nodes.Add(newnode);
|
|
// topnode = newnode;
|
|
//}
|
|
//else
|
|
//{
|
|
// newnode.Selectable = true;
|
|
// newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
// newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
// newnode.CheckBoxThreeState = false;
|
|
// newnode.CheckBoxVisible = true;
|
|
// tmp = topnode.Nodes.Add(newnode);
|
|
//}
|
|
cntnd++;
|
|
if (fic.ChildFolderCount > 0 || fic.FolderDocVersionCount > 0) // allow for '+' for tree expansion
|
|
{
|
|
DevComponents.AdvTree.Node tnt = new DevComponents.AdvTree.Node();
|
|
tnt.Text = "VLN_DUMMY";
|
|
newnode.Nodes.Add(tnt);
|
|
topnode.Nodes.Add(newnode);
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
DevComponents.AdvTree.Node newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = fi.ToString();
|
|
newnode.Tag = fi;
|
|
//newnode.Checked = true;
|
|
//newnode.Selectable = true;
|
|
//newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
//newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
//newnode.CheckBoxThreeState = false;
|
|
//newnode.CheckBoxVisible = true;
|
|
AddDocVersionNodes(newnode, fi, true);
|
|
topnode.Nodes.Add(newnode);
|
|
lstCheckedDocVersions.Add(Mydocversion);
|
|
buildSetToSearchPanelTitle();
|
|
}
|
|
// if nothing was added to the tree, just put in the node above the docversions...
|
|
if (advTreeProcSets.Nodes.Count == 0)
|
|
{
|
|
cntnd++;
|
|
fi = Mydocversion.MyFolder;
|
|
topnode = new DevComponents.AdvTree.Node();
|
|
topnode.Text = fi.ToString();
|
|
advTreeProcSets.Nodes.Add(topnode);
|
|
topnode.Tag = fi;
|
|
}
|
|
|
|
advTreeProcSets.BeforeExpand += new DevComponents.AdvTree.AdvTreeNodeCancelEventHandler(advTreeProcSets_BeforeExpand);
|
|
|
|
// position to the procedure set in the tree if we have a procedure open
|
|
if (Mydocversion != null)
|
|
advTreeProcSetsPreSelect();
|
|
else
|
|
advTreeProcSets.Nodes[0].SelectedCell = advTreeProcSets.Nodes[0].Cells[0]; // select the first node - fixes cosmetic problem
|
|
|
|
if (blSeachTabClicked)
|
|
cbxTextSearchText.Focus(); // set initial focus to enter search text
|
|
}
|
|
|
|
//void advTreeProcSets_AfterNodeInsert(object sender, TreeNodeCollectionEventArgs e)
|
|
//{
|
|
// IVEDrillDownReadOnly tmp = e.Node.Tag as IVEDrillDownReadOnly;
|
|
// if (tmp != null)
|
|
// {
|
|
// Console.WriteLine("Has Children {0} {1}", tmp.HasChildren, tmp.ToString());
|
|
// FolderInfo fi = tmp as FolderInfo;
|
|
// //if (fi != null)
|
|
// // Console.WriteLine("dvi Count {0}", fi.FolderDocVersionCount);
|
|
// }
|
|
// vlnStackTrace.ShowStackLocal(e.Node.Text,1,10);
|
|
//}
|
|
private Dictionary<DevComponents.AdvTree.Node, bool> dicSelectedDocVersionNodes = new Dictionary<DevComponents.AdvTree.Node, bool>();
|
|
private Dictionary<DevComponents.AdvTree.Node, bool> dicExpandedDocVersionNodes = new Dictionary<DevComponents.AdvTree.Node, bool>();
|
|
void advTreeProcSets_BeforeExpand(object sender, DevComponents.AdvTree.AdvTreeNodeCancelEventArgs e)
|
|
{
|
|
DevComponents.AdvTree.Node par = e.Node;
|
|
// get first child's text, if it has one & if the text is VLN_DUMMY, load children
|
|
DevComponents.AdvTree.Node tn = null;
|
|
if (par.Nodes.Count > 0) tn = par.Nodes[0];
|
|
if (tn.Text == "VLN_DUMMY") // expand this
|
|
{
|
|
par.Nodes.Clear();
|
|
Object obj = par.Tag;
|
|
if (!(obj is FolderInfo)) return; // should always be folderinfo on expand
|
|
FolderInfo fi = (FolderInfo)obj;
|
|
if (fi.ChildFolderCount > 0)
|
|
{
|
|
foreach (FolderInfo fic in fi.ChildFolders)
|
|
{
|
|
DevComponents.AdvTree.Node newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = fic.ToString();
|
|
newnode.Tag = fic;
|
|
par.Nodes.Add(newnode);
|
|
if (fic.HasChildren) // allow for '+' for tree expansion
|
|
{
|
|
DevComponents.AdvTree.Node tnt = new DevComponents.AdvTree.Node();
|
|
tnt.Text = "VLN_DUMMY";
|
|
newnode.Nodes.Add(tnt);
|
|
}
|
|
}
|
|
}
|
|
else if (fi.FolderDocVersionCount > 0)
|
|
{
|
|
AddDocVersionNodes(par, fi, false);
|
|
}
|
|
}
|
|
}
|
|
|
|
private void AddDocVersionNodes(DevComponents.AdvTree.Node par, FolderInfo fi, bool isChecked)
|
|
{
|
|
foreach (DocVersionInfo dv in fi.FolderDocVersions)
|
|
{
|
|
//if ((VersionTypeEnum)dv.VersionType == VersionTypeEnum.WorkingDraft)
|
|
//{
|
|
DevComponents.AdvTree.Node newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = dv.ToString();
|
|
newnode.Tag = dv;
|
|
newnode.Selectable = true;
|
|
newnode.CheckBoxAlignment = DevComponents.AdvTree.eCellPartAlignment.NearCenter;
|
|
newnode.CheckBoxStyle = eCheckBoxStyle.CheckBox;
|
|
newnode.CheckBoxThreeState = false;
|
|
newnode.CheckBoxVisible = true;
|
|
newnode.Checked = isChecked;
|
|
if (tabSearchTypes.SelectedTab == tabTranSearch)
|
|
{
|
|
if (!dicSelectedDocVersionNodes.ContainsKey(newnode)) dicSelectedDocVersionNodes.Add(newnode, false);
|
|
newnode.Enabled = cbxTranVersion.Tag != null ? cbxTranVersion.Tag.ToString().Contains(dv.VersionID.ToString()) : false;
|
|
}
|
|
else
|
|
newnode.Enabled = true;
|
|
par.Nodes.Add(newnode);
|
|
dicExpandedDocVersionNodes.Add(newnode, newnode.Enabled);
|
|
//}
|
|
}
|
|
}
|
|
|
|
private Node LookInTree(NodeCollection monkeys, string bananna)
|
|
{
|
|
Node foundit = null;
|
|
foreach (Node chimp in monkeys)
|
|
{
|
|
if (chimp.Text.Equals(bananna))
|
|
{
|
|
foundit = chimp;
|
|
// need to select the node (cell) for it to be checked
|
|
chimp.SelectedCell = chimp.Cells[0];
|
|
if (chimp.Nodes.Count > 0)
|
|
chimp.Expand();
|
|
break;
|
|
}
|
|
chimp.Collapse();
|
|
}
|
|
return foundit;
|
|
}
|
|
|
|
private void advTreeProcSetsPreSelect()
|
|
{
|
|
bool keeplooking = true;
|
|
//build a stack (bread crumb trail) of where is procedure set came from within the tree.
|
|
Stack<string> crumbs = new Stack<string>();
|
|
//crumbs.Push(Mydocversion.Name); // ex: "working draft"
|
|
//crumbs.Push(Mydocversion.MyFolder.Name); // ex: "Emergency Procedures"
|
|
//crumbs.Push(Mydocversion.MyFolder.MyParent.Name); // ex: "STPNOC-South Texas"
|
|
//crumbs.Push(advTreeProcSets.Nodes[0].Text); //top node of my tree
|
|
|
|
crumbs.Push(Mydocversion.Name); // ex: "working draft"
|
|
crumbs.Push(Mydocversion.MyFolder.Name); // ex: "Emergency Procedures"
|
|
FolderInfo fi = Mydocversion.MyFolder.MyParent;
|
|
while (fi != null)
|
|
{
|
|
if (fi.MyParent != null)
|
|
crumbs.Push(fi.Name);
|
|
fi = fi.MyParent;
|
|
}
|
|
crumbs.Push(advTreeProcSets.Nodes[0].Text); //top node of my tree
|
|
|
|
// now walk the tree, looking for the node names we saved in the stack.
|
|
NodeCollection monkeys = advTreeProcSets.Nodes;
|
|
while (keeplooking)
|
|
{
|
|
Node climber = LookInTree(monkeys, crumbs.Pop());
|
|
keeplooking = (climber != null && crumbs.Count > 0);
|
|
if (keeplooking)
|
|
monkeys = climber.Nodes;
|
|
if (!keeplooking && climber != null)
|
|
{
|
|
climber.Checked = true;
|
|
}
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region ROFST Combo Tree
|
|
private void LoadROComboTree()
|
|
{
|
|
if (_MyROFSTLookup == null) return;
|
|
cmboTreeROs.Nodes.Clear();
|
|
this.cmboTreeROs.AdvTree.BeforeExpand += new DevComponents.AdvTree.AdvTreeNodeCancelEventHandler(this.cmboTreeROs_BeforeExpand);
|
|
cmboTreeROs.AdvTree.AfterExpand += new AdvTreeNodeEventHandler(AdvTree_AfterExpandorCollapse);
|
|
cmboTreeROs.AdvTree.AfterCollapse += new AdvTreeNodeEventHandler(AdvTree_AfterExpandorCollapse);
|
|
for (int i = 0; i < _MyROFSTLookup.myHdr.myDbs.Length; i++)
|
|
{
|
|
DevComponents.AdvTree.Node tn = new DevComponents.AdvTree.Node();
|
|
tn.Text = _MyROFSTLookup.myHdr.myDbs[i].dbiTitle;
|
|
tn.Tag = _MyROFSTLookup.myHdr.myDbs[i];
|
|
cmboTreeROs.Nodes.Add(tn);
|
|
AddDummyGroup(_MyROFSTLookup.myHdr.myDbs[i], tn);
|
|
}
|
|
}
|
|
void AdvTree_AfterExpandorCollapse(object sender, AdvTreeNodeEventArgs e)
|
|
{
|
|
Node bottomNode = BottomTreeNode(cmboTreeROs.AdvTree.Nodes);
|
|
Node lastNode = cmboTreeROs.AdvTree.Nodes[cmboTreeROs.AdvTree.Nodes.Count - 1];
|
|
int top = cmboTreeROs.AdvTree.Nodes[0].Bounds.Top;
|
|
int bottom = bottomNode.Bounds.Bottom + 5;
|
|
int hScrollBarHeight = cmboTreeROs.AdvTree.HScrollBar != null ? cmboTreeROs.AdvTree.HScrollBar.Height : 0;
|
|
bottom = bottomNode.Bounds.Bottom + 5;
|
|
cmboTreeROs.AdvTree.Size = new Size(cmboTreeROs.AdvTree.Size.Width, Math.Min(525, bottom - top + hScrollBarHeight));
|
|
if (cmboTreeROs.AdvTree.VScrollBar != null && bottom < cmboTreeROs.AdvTree.Size.Height)
|
|
{
|
|
int yLookFor = (bottom - cmboTreeROs.AdvTree.Size.Height) + 2 * hScrollBarHeight;
|
|
Node topNode = FindTreeNodeAt(cmboTreeROs.AdvTree.Nodes, yLookFor);
|
|
if (topNode != null)
|
|
topNode.EnsureVisible();
|
|
}
|
|
}
|
|
private Node FindTreeNodeAt(NodeCollection nodes, int y)
|
|
{
|
|
foreach (Node node in nodes)
|
|
{
|
|
if (node.Bounds.Top <= y && node.Bounds.Bottom >= y)
|
|
return node;
|
|
if (node.Bounds.Top > y)
|
|
{
|
|
if (node.PrevNode != null && node.PrevNode.Expanded)
|
|
return FindTreeNodeAt(node.PrevNode.Nodes, y);
|
|
return node;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
private Node BottomTreeNode(NodeCollection nodes)
|
|
{
|
|
Node bottomNode = nodes[nodes.Count - 1]; // Return bottom node in collection
|
|
if (bottomNode.Expanded) // If expanded return bottom child
|
|
return BottomTreeNode(bottomNode.Nodes);
|
|
return bottomNode;
|
|
}
|
|
private void AddDummyGroup(ROFSTLookup.rodbi rodbi, DevComponents.AdvTree.Node tn)
|
|
{
|
|
if (rodbi.children != null && rodbi.children.Length > 0)
|
|
{
|
|
DevComponents.AdvTree.Node tmp = new DevComponents.AdvTree.Node();
|
|
tmp.Text = "VLN_DUMMY_FOR_TREE";
|
|
tn.Nodes.Add(tmp);
|
|
}
|
|
}
|
|
private void cmboTreeROs_BeforeExpand(object sender, DevComponents.AdvTree.AdvTreeNodeCancelEventArgs e)
|
|
{
|
|
LoadChildren(e.Node);
|
|
}
|
|
|
|
private void LoadChildren(DevComponents.AdvTree.Node tn)
|
|
{
|
|
object tag = tn.Tag;
|
|
if (tn.HasChildNodes && tn.Nodes[0].Text != "VLN_DUMMY_FOR_TREE") return; // already loaded.
|
|
if (tn.HasChildNodes && tn.Nodes[0].Text == "VLN_DUMMY_FOR_TREE") tn.Nodes[0].Remove();
|
|
ROFSTLookup.rochild[] chld = null;
|
|
|
|
if (tn.Tag is ROFSTLookup.rodbi)
|
|
{
|
|
ROFSTLookup.rodbi db = (ROFSTLookup.rodbi)tn.Tag;
|
|
chld = db.children;
|
|
}
|
|
else if (tn.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild ch = (ROFSTLookup.rochild)tn.Tag;
|
|
chld = ch.children;
|
|
}
|
|
else
|
|
{
|
|
Console.WriteLine("error - no type");
|
|
return;
|
|
}
|
|
// if children, add dummy node
|
|
if (chld != null && chld.Length > 0)
|
|
{
|
|
ProgressBar_Initialize(chld.Length, tn.Text);
|
|
for (int i = 0; i < chld.Length; i++)
|
|
{
|
|
ProgressBar_SetValue(i);
|
|
DevComponents.AdvTree.Node tmp = null;
|
|
// if this is a group, i.e. type 0, add a dummy node
|
|
if (chld[i].type == 0 && chld[i].children == null)
|
|
//skip it.
|
|
// TODO: KBR how to handle this?
|
|
//Console.WriteLine("ro junk");
|
|
continue;
|
|
else if (chld[i].value == null)
|
|
{
|
|
tmp = new DevComponents.AdvTree.Node();
|
|
tmp.Text = chld[i].title;
|
|
tmp.Tag = chld[i];
|
|
int index = FindIndex(tn.Nodes, tmp.Text);
|
|
tn.Nodes.Insert(index, tmp);
|
|
//tn.Nodes.Add(tmp);
|
|
DevComponents.AdvTree.Node sub = new DevComponents.AdvTree.Node();
|
|
sub.Text = "VLN_DUMMY_FOR_TREE";
|
|
tmp.Nodes.Add(sub);
|
|
}
|
|
else
|
|
{
|
|
tmp = new DevComponents.AdvTree.Node();
|
|
tmp.Text = chld[i].title;
|
|
tmp.Tag = chld[i];
|
|
int index = FindIndex(tn.Nodes, tmp.Text);
|
|
tn.Nodes.Insert(index, tmp);
|
|
//tn.Nodes.Add(tmp);
|
|
}
|
|
}
|
|
}
|
|
ProgressBar_Clear();
|
|
}
|
|
|
|
private int FindIndex(NodeCollection nodes, string value)
|
|
{
|
|
int index = 0;
|
|
foreach (Node node in nodes)
|
|
{
|
|
if (GreaterValue(node.Text, value)) return index;
|
|
index++;
|
|
}
|
|
return index;
|
|
}
|
|
// private static Regex _RegExGetNumber = new Regex(@"^ *[+-]?[.,0-9]+(E[+-]?[0-9]+)?");
|
|
private bool GreaterValue(string value1, string value2)
|
|
{
|
|
return DisplayRO.GreaterValue(value1, value2);
|
|
//Match match1 = _RegExGetNumber.Match(value1);
|
|
//Match match2 = _RegExGetNumber.Match(value2);
|
|
//if (match1.Success && match2.Success) // Compare the numeric value
|
|
//{
|
|
// double dbl1 = double.Parse(match1.ToString());
|
|
// double dbl2 = double.Parse(match2.ToString());
|
|
// return dbl1 > dbl2;
|
|
//}
|
|
//return String.Compare(value1, value2, true) > 0;
|
|
}
|
|
|
|
private void cmboTreeROs_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
if (cmboTreeROs.SelectedIndex == -1 || cmboTreeROs.SelectedNode.Tag is ROFSTLookup.rodbi)
|
|
cbxFndUnLnkROVals.Enabled = false;
|
|
else if (cmboTreeROs.SelectedNode.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ROFSTLookup.rochild ro = (ROFSTLookup.rochild)cmboTreeROs.SelectedNode.Tag;
|
|
cbxFndUnLnkROVals.Enabled = (ro.children == null);
|
|
}
|
|
if (!cbxFndUnLnkROVals.Enabled)
|
|
cbxFndUnLnkROVals.Checked = false;
|
|
}
|
|
|
|
private void gpFindROs_EnabledChanged(object sender, EventArgs e)
|
|
{
|
|
if (gpFindROs.Enabled)
|
|
gpFindROs.Style.BackColor = Color.Yellow;
|
|
else
|
|
gpFindROs.Style.BackColor = saveGpFindROsColor;
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Annotation Search
|
|
|
|
public void SetupAnnotationSearch(AnnotationDetails annotationDetails, DisplayTabControl tc)
|
|
{
|
|
_AnnotationDetails = annotationDetails;
|
|
List<AnnotationTypeSearch> annoTypes = new List<AnnotationTypeSearch>();
|
|
annoTypes.Add(new AnnotationTypeSearch("All Annotations", ""));
|
|
AnnotationTypeInfoList annoList = AnnotationTypeInfoList.Get();
|
|
foreach (AnnotationTypeInfo ati in annoList)
|
|
annoTypes.Add(new AnnotationTypeSearch(ati.Name, ati.TypeID.ToString()));
|
|
cbxAnnoTypes.DisplayMember = "Name";
|
|
cbxAnnoTypes.DataSource = annoTypes;
|
|
lbSrchResults.MouseMove += new MouseEventHandler(lbSrchResults_MouseMove);
|
|
_TabControl = tc;
|
|
}
|
|
|
|
|
|
private void cbxTextSearchAnnotation_Leave(object sender, EventArgs e)
|
|
{
|
|
InsertAnnotationSearchCriteria();
|
|
|
|
}
|
|
|
|
private void InsertAnnotationSearchCriteria()
|
|
{
|
|
if (!cbxTextSearchAnnotation.Text.Equals(string.Empty))
|
|
{
|
|
string tstr = cbxTextSearchAnnotation.Text;
|
|
if (!cbxTextSearchAnnotation.Items.Contains(tstr))
|
|
cbxTextSearchAnnotation.Items.Insert(0, tstr);
|
|
}
|
|
}
|
|
|
|
private void gpSrchAnnoText_EnabledChanged(object sender, EventArgs e)
|
|
{
|
|
if (gpSrchAnnoText.Enabled)
|
|
{
|
|
gpSrchAnnoText.Style.BackColor = Color.Yellow;
|
|
cbxTextSearchAnnotation.Focus();
|
|
}
|
|
else
|
|
gpSrchAnnoText.Style.BackColor = saveGpSrchAnnoTextColor;
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region ProgressBar
|
|
private void ProgressBar_Initialize(int max, string desc)
|
|
{
|
|
if (_ProgressBar != null)
|
|
{
|
|
_ProgressBar.Maximum = max;
|
|
_ProgressBar.Text = desc;
|
|
_ProgressBar.TextVisible = true;
|
|
}
|
|
}
|
|
|
|
private void ProgressBar_SetValue(int curval)
|
|
{
|
|
if (_ProgressBar != null)
|
|
_ProgressBar.Value = curval;
|
|
}
|
|
|
|
private void ProgressBar_Clear()
|
|
{
|
|
if (_ProgressBar != null)
|
|
{
|
|
_ProgressBar.Text = "";
|
|
_ProgressBar.Maximum = 0;
|
|
_ProgressBar.Value = 0;
|
|
_ProgressBar.TextVisible = false;
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
#region Search Results
|
|
private void DisplayResults()
|
|
{
|
|
_LoadingList = true;
|
|
lbSrchResults.DisplayMember = _DisplayMember;
|
|
if (_SearchResults != null)
|
|
{
|
|
if (cbSorted.Checked)
|
|
{
|
|
Csla.SortedBindingList<ItemInfo> sortedResults = new Csla.SortedBindingList<ItemInfo>(_SearchResults);
|
|
sortedResults.ApplySort(_DisplayMember, ListSortDirection.Ascending);
|
|
lbSrchResults.DataSource = sortedResults;
|
|
}
|
|
else
|
|
//PopulatelbSrcResults(_SearchResults);
|
|
lbSrchResults.DataSource = _SearchResults;
|
|
grpPanSearchResults.Text = string.Format("Search Results Found: {0}", _SearchResults.Count);
|
|
grpPanSearchResults.Style.BackColor = Color.LightGreen;// Color.YellowGreen; Color.DarkSeaGreen;
|
|
}
|
|
else
|
|
{
|
|
grpPanSearchResults.Text = "Search Results";
|
|
grpPanSearchResults.Style.BackColor = saveGrpPanSearchResults;
|
|
}
|
|
// Turn Print and Results display style on/off based on whether there are search results
|
|
if (lbSrchResults.Items.Count > 0)
|
|
{
|
|
btnPrnSrchRslts.Enabled = true;
|
|
btnClearSearchResults.Enabled = true;
|
|
btnCopySearchResults.Enabled = true;
|
|
btnSaveSearchResults.Enabled = true;
|
|
cmbResultsStyle.Enabled = true;
|
|
}
|
|
else
|
|
{
|
|
btnPrnSrchRslts.Enabled = false;
|
|
btnClearSearchResults.Enabled = false;
|
|
btnCopySearchResults.Enabled = false;
|
|
btnSaveSearchResults.Enabled = false;
|
|
cmbResultsStyle.Enabled = false;
|
|
}
|
|
|
|
lbSrchResults.SelectedIndex = -1;
|
|
LastResultsMouseOverIndex = -1;
|
|
_LoadingList = false;
|
|
|
|
}
|
|
|
|
//private void PopulatelbSrcResults(ICollection<ItemInfo> _SearchResults)
|
|
//{
|
|
// foreach (ItemInfo ii in _SearchResults)
|
|
// {
|
|
// lbSrchResults.Items.Add(ii);
|
|
// }
|
|
// Console.WriteLine("{0} Populate", DateTime.Now);
|
|
//}
|
|
//public void UpdateAnnotationSearchResults()
|
|
//{
|
|
// //AnnotationTypeInfo ati = cbxAnnoTypes.SelectedValue as AnnotationTypeInfo;
|
|
// //_LoadingList = true;
|
|
// //lbSrchResults.DataSource = ati.AnnotationTypeAnnotations;
|
|
// //lbSrchResults.SelectedIndex = -1;
|
|
// //LastResultsMouseOverIndex = -1;
|
|
// //if (lbSrchResults.Items.Count > 0)
|
|
// // btnPrnSrchRslts.Enabled = true;
|
|
// //else
|
|
// // btnPrnSrchRslts.Enabled = false;
|
|
// //_LoadingList = false;
|
|
//}
|
|
private void lbSrchResults_MouseMove(object sender, MouseEventArgs e)
|
|
{
|
|
int ResultsMouseOverIndex = lbSrchResults.IndexFromPoint(e.Location);
|
|
if (ResultsMouseOverIndex != -1 && ResultsMouseOverIndex != LastResultsMouseOverIndex)
|
|
{
|
|
ItemInfo ii = lbSrchResults.Items[ResultsMouseOverIndex] as ItemInfo;
|
|
if (cmbResultsStyle.Text == "Step Path")
|
|
toolTip1.SetToolTip(lbSrchResults, ii.DisplayText); // display the text in a tooltip
|
|
else
|
|
toolTip1.SetToolTip(lbSrchResults, ii.Path); // display location of corresponding procedure text in a tooltip
|
|
LastResultsMouseOverIndex = ResultsMouseOverIndex;
|
|
}
|
|
}
|
|
private bool _ProcessingSelectedValueChanged = false;
|
|
private void lbSrchResults_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (_LoadingList) return;
|
|
if (_ProcessingSelectedValueChanged) return;
|
|
_ProcessingSelectedValueChanged = true;
|
|
// If the list is being refreshed, then set the selection index to -1 (no selection)
|
|
if (_SearchResults.RefreshingList && lbSrchResults.SelectedIndex != -1)
|
|
lbSrchResults.SelectedIndex = -1;
|
|
else
|
|
{
|
|
_ItemInfo = lbSrchResults.SelectedValue as ItemInfo;
|
|
if ((tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[1]) && (_ItemInfo != null))
|
|
{
|
|
_AnnotationDetails.CurrentAnnotation = AnnotationInfo.Get(_ItemInfo.SearchAnnotationID);
|
|
if (_AnnotationDetails.CurrentAnnotation != null)
|
|
_TabControl.OpenItem(_AnnotationDetails.CurrentAnnotation.MyItem); // open the corresponding procedure text
|
|
_AnnotationDetails.FindCurrentAnnotation(); // position to corresponding row in annotation grid
|
|
}
|
|
else
|
|
{
|
|
if (_ItemInfo != null)
|
|
{
|
|
_OpenDocFromSearch = true;
|
|
DisplayTabItem dti = _TabControl.OpenItem(_ItemInfo); // open the corresponding procedure text
|
|
if (dti != null)
|
|
{
|
|
if (dti.MyDSOTabPanel != null)
|
|
dti.MyDSOTabPanel.SearchString = _strSrchText == null || _strSrchText == "" ? this.cbxTextSearchText.Text : _strSrchText;
|
|
if (dti.MyStepTabPanel != null)
|
|
dti.MyStepTabPanel.SearchString = _strSrchText == null || _strSrchText == "" ? this.cbxTextSearchText.Text : _strSrchText;
|
|
_OpenDocFromSearch = false;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
_ProcessingSelectedValueChanged = false;
|
|
}
|
|
|
|
private void cmbResultsStyle_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
switch (cmbResultsStyle.Text)
|
|
{
|
|
case "Document Path":
|
|
_DisplayMember = "SearchDVPath";
|
|
break;
|
|
case "Step Path":
|
|
_DisplayMember = "ShortSearchPath";
|
|
break;
|
|
case "Annotation Text":
|
|
_DisplayMember = "SearchAnnotationText";
|
|
break;
|
|
case "Document Text":
|
|
_DisplayMember = "DisplayText";
|
|
break;
|
|
default:
|
|
_DisplayMember = "SearchPath";
|
|
break;
|
|
}
|
|
DisplayResults();
|
|
}
|
|
|
|
private void cbSorted_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
Cursor savcursor = Cursor;
|
|
Application.DoEvents();
|
|
Cursor = Cursors.WaitCursor;
|
|
//Console.WriteLine(string.Format("Checked = {0}", cbSorted.Checked));
|
|
DisplayResults();
|
|
Cursor = savcursor;
|
|
}
|
|
#endregion
|
|
|
|
#region Search
|
|
private string DVISearchList
|
|
{
|
|
get
|
|
{
|
|
// append list of document versions to search
|
|
if (lstCheckedDocVersions.Count > 0)
|
|
{
|
|
string strRtnStr = "";
|
|
// get list of doc versions to search
|
|
foreach (DocVersionInfo dvi in lstCheckedDocVersions)
|
|
{
|
|
strRtnStr += string.Format(",{0}", dvi.VersionID.ToString());
|
|
}
|
|
return strRtnStr.Substring(1);
|
|
}
|
|
return "";
|
|
}
|
|
}
|
|
private string TypeSearchList
|
|
{
|
|
get
|
|
{
|
|
// append list of step types to search
|
|
if (lstCheckedStepTypes.Count > 0)
|
|
{
|
|
string strRtnStr = "";
|
|
// get list of selected types
|
|
foreach (int typ in lstCheckedStepTypes)
|
|
{
|
|
int tmp = typ;
|
|
if (tmp == 0)
|
|
tmp = 10000; // this is the accessory page type
|
|
else
|
|
tmp += 20000; // step/substep types
|
|
strRtnStr += string.Format(",{0}", tmp);
|
|
}
|
|
return strRtnStr.Substring(1);
|
|
}
|
|
return "";
|
|
}
|
|
}
|
|
private string TextSearchString
|
|
{
|
|
get
|
|
{
|
|
if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[0])
|
|
return ConvertSpecialChars(cbxTextSearchText.Text);//string.Format("{0}", cbxTextSearchText.Text);
|
|
return "";
|
|
}
|
|
}
|
|
private string AnnotationSearchType
|
|
{
|
|
get
|
|
{
|
|
if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[1])
|
|
return ((AnnotationTypeSearch)cbxAnnoTypes.SelectedValue).ID;
|
|
return "";
|
|
}
|
|
}
|
|
private string ROSearchList
|
|
{
|
|
get
|
|
{
|
|
if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[2])
|
|
{ // RO Search
|
|
ROFSTLookup.rochild[] chld = null;
|
|
ROFSTLookup.rochild ch;
|
|
if (cmboTreeROs.SelectedNode != null)
|
|
{
|
|
if (cbxFndUnLnkROVals.Enabled && cbxFndUnLnkROVals.Checked)
|
|
{
|
|
ch = (ROFSTLookup.rochild)cmboTreeROs.SelectedNode.Tag;
|
|
_strSrchText = string.Format("{0}", ch.value);
|
|
return _strSrchText; // append RO Value text to search
|
|
}
|
|
else
|
|
{
|
|
if (cmboTreeROs.SelectedNode.Tag is ROFSTLookup.rodbi)
|
|
{
|
|
ROFSTLookup.rodbi db = (ROFSTLookup.rodbi)cmboTreeROs.SelectedNode.Tag;
|
|
return _MyRODbID.ToString() + ":" + string.Format("{0}", db.dbiID.ToString("X4"));
|
|
}
|
|
else if (cmboTreeROs.SelectedNode.Tag is ROFSTLookup.rochild)
|
|
{
|
|
ch = (ROFSTLookup.rochild)cmboTreeROs.SelectedNode.Tag;
|
|
chld = ch.children;
|
|
// build a list of ROs to search
|
|
return _MyRODbID.ToString() + ":" + GetROsToSearch(chld);
|
|
//return _MyRODbID.ToString() + ":" + ch.roid + "0000," + GetROsToSearch(chld);
|
|
//if (strRtnStr.EndsWith(","))
|
|
// strRtnStr = strRtnStr.Substring(0, strRtnStr.Length - 1);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return "";
|
|
}
|
|
}
|
|
|
|
private string ConvertSpecialChars(string str)
|
|
{
|
|
string rtnVal = str;
|
|
rtnVal = rtnVal.Replace("\u00A0", @"\u160?"); //convert \u00A0 to a hard space (\u00A0 shows as a blank in the search text field)
|
|
rtnVal = rtnVal.Replace("\n", "");
|
|
// Bug fix B2014-057
|
|
// if we are searching for a symbol character in all procedure sets MyDocVersion is null
|
|
// when MyDocVersion is null, get the symbol list directly from the PROMS base format (BaseAll.xml)
|
|
FormatData fmtdata = (_MyDocVersion != null) ? _MyDocVersion.ActiveFormat.PlantFormat.FormatData : FormatInfo.PROMSBaseFormat.FormatData;
|
|
if (fmtdata != null && fmtdata.SymbolList != null)
|
|
{
|
|
SymbolList sl = fmtdata.SymbolList;
|
|
if (sl != null)
|
|
{
|
|
foreach (Symbol sym in sl)
|
|
{
|
|
string rplace = string.Format(sym.Unicode < 256 ? @"\'{0:X2}" : @"\u{0}?", sym.Unicode); // bug fix B2014-057 we were not including the ? in the replace
|
|
rtnVal = rtnVal.Replace(((char)sym.Unicode).ToString(), rplace);
|
|
}
|
|
}
|
|
}
|
|
return rtnVal;
|
|
}
|
|
// Same funtion as GetROChildren in DisplayReport.cs
|
|
private string GetROsToSearch(ROFSTLookup.rochild[] chld)
|
|
{
|
|
string rtnstr = "";
|
|
if (chld == null) // get a single ROID
|
|
{
|
|
ROFSTLookup.rochild ro = (ROFSTLookup.rochild)cmboTreeROs.SelectedNode.Tag;
|
|
rtnstr += string.Format("{0}", ro.roid);
|
|
if (rtnstr.Length == 12) rtnstr += "0000"; // last four digits are used for multiple return values
|
|
}
|
|
else
|
|
{ // spin through the child list and get the ROIDs.
|
|
// if the child has children, then call this function recursivly
|
|
foreach (ROFSTLookup.rochild roc in chld)
|
|
{
|
|
if (roc.children != null)
|
|
rtnstr += GetROsToSearch(roc.children);
|
|
else
|
|
rtnstr += string.Format("{0},", roc.roid);
|
|
}
|
|
}
|
|
return rtnstr;
|
|
}
|
|
|
|
private void btnSearch_Click(object sender, EventArgs e)
|
|
{
|
|
DateTime start = DateTime.Now;
|
|
Cursor savcursor = Cursor;
|
|
// keeps track of index into combo box for results style. This combo box may have 3 or 4
|
|
// items depending on whether annotations exist.
|
|
int cmbResultsStyleIndex = -1;
|
|
try
|
|
{
|
|
lbSrchResults.DataSource = null;
|
|
lbSrchResults.Items.Clear();
|
|
toolTip1.SetToolTip(lbSrchResults, null);
|
|
Cursor = Cursors.WaitCursor;
|
|
SearchResults = null;
|
|
bool includeRTFformat = false;
|
|
bool includeSpecialChars = true;
|
|
// Build list of selected types that were searched
|
|
string typstr = null;
|
|
foreach (string s in lstCheckedStepTypesStr) typstr = typstr == null ? s : typstr + ", " + s;
|
|
TypesSelected = "Filtered By: " + ((typstr != null) ? typstr : "All Step Types");
|
|
//TypesSelected = "Step Types Searched: " + ((typstr != null) ? typstr : "all step types");
|
|
//TypesSelected = "Searched Step Types: " + ((typstr != null) ? typstr : "all step types");
|
|
//TypesSelected = (typstr != null) ? "Searched Step Types: " + typstr : "Searched All Step Types";
|
|
string unitPrefix = "";
|
|
if(Mydocversion != null)
|
|
unitPrefix = Mydocversion.DocVersionConfig.Unit_ProcedureNumber;
|
|
if (unitPrefix.EndsWith("#"))
|
|
unitPrefix = unitPrefix.Replace("#", "");
|
|
|
|
if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[0]) // Text Search
|
|
{
|
|
if (cbxSrchTypeUsage.Checked) // find just the usage of the selected types
|
|
{
|
|
if (lstCheckedStepTypes.Count == 0)
|
|
{
|
|
MessageBox.Show("Please select one or more types then press the Search button", "No Types Selected");
|
|
xpStepTypes.Expanded = true;
|
|
advTreeStepTypes.Focus();
|
|
xpStepTypes.TitleStyle.BackColor1.Color = Color.Crimson;
|
|
}
|
|
else
|
|
{
|
|
//string typstr = null;
|
|
//foreach (string s in lstCheckedStepTypesStr) typstr = typstr==null?s:typstr + ", " + s;
|
|
//ReportTitle = "Search for Selected Types"; //"Proms - Search by Type: " + typstr;
|
|
ReportTitle = "Step Element Report"; //"Proms - Search by Type: " + typstr;
|
|
TypesSelected = "Filtered By: " + typstr;
|
|
SearchString = null;
|
|
SearchResults = ItemInfoList.GetListFromTextSearch(DVISearchList, TypeSearchList, "", cbxBooleanTxtSrch.Checked ? 2 : cbxCaseSensitive.Checked ? 1 : 0, ItemSearchIncludeLinks.Value, includeRTFformat, includeSpecialChars, unitPrefix);
|
|
cmbResultsStyleIndex = 1; //display step locations in results
|
|
}
|
|
}
|
|
else
|
|
{
|
|
//if (textSearchString.Equals(""))
|
|
//{
|
|
// MessageBox.Show("Please enter some search text, then click the Search button", "No Search Text");
|
|
// cbxTextSearchText.Focus();
|
|
//}
|
|
//else
|
|
//{
|
|
//ReportTitle = string.Format("Proms - {0} Search for '{1}'", cbxBooleanTxtSrch.Checked ? "Boolean" : "Text", TextSearchString);
|
|
ReportTitle = string.Format("Search for '{0}'", TextSearchString);
|
|
SearchString = TextSearchString;
|
|
//TypesSelected = (typstr != null) ? "Searched Step Types: " + typstr : "Searched All Step Types";
|
|
//TypesSelected = "Searched Step Types: " + ((typstr != null) ? typstr : "All Step Types");
|
|
SearchResults = ItemInfoList.GetListFromTextSearch(DVISearchList, TypeSearchList, TextSearchString, cbxBooleanTxtSrch.Checked ? 2 : cbxCaseSensitive.Checked ? 1 : 0, cbxIncROTextSrch.Checked ? ItemSearchIncludeLinks.Value : ItemSearchIncludeLinks.Nothing, includeRTFformat, includeSpecialChars, unitPrefix);
|
|
cmbResultsStyleIndex = 3; // display step text in results
|
|
//}
|
|
}
|
|
}
|
|
else if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[1]) // Annotation Search
|
|
{
|
|
SearchString = null;
|
|
//ReportTitle = string.Format("Proms - Annotation Search for '{0}'", cbxTextSearchAnnotation.Text);
|
|
if (cbxTextSearchAnnotation.Text == null || cbxTextSearchAnnotation.Text == "")
|
|
{
|
|
ReportTitle = string.Format("Find {0}{1}", cbxAnnoTypes.Text, (cbxAnnoTypes.SelectedIndex > 0)?" Annotations" : "" );
|
|
}
|
|
else
|
|
{
|
|
ReportTitle = string.Format("Search {0}{1} For '{2}'", cbxAnnoTypes.Text, (cbxAnnoTypes.SelectedIndex > 0) ? " Annotations" : "", cbxTextSearchAnnotation.Text);
|
|
}
|
|
//ReportTitle = string.Format("Search Annotations for '{0}'", cbxTextSearchAnnotation.Text);
|
|
//string srchStr = ConvertSpecialChars(cbxTextSearchAnnotation.Text);//cbxTextSearchAnnotation.Text;
|
|
//TypesSelected = "Searched Step Types: " + ((typstr != null) ? typstr : "All Step Types");
|
|
|
|
//SearchResults = ItemInfoList.GetListFromAnnotationSearch(dviSearchList, typeSearchList, textSearchString, srchStr, cbxCaseSensitiveAnnoText.Checked);
|
|
|
|
SearchResults = ItemInfoList.GetListFromAnnotationSearch(DVISearchList, TypeSearchList, AnnotationSearchType, cbxTextSearchAnnotation.Text, cbxCaseSensitiveAnnoText.Checked,unitPrefix);
|
|
//UpdateAnnotationSearchResults();
|
|
cmbResultsStyleIndex = 2; // display annotation text in results
|
|
}
|
|
else if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[2]) // RO Search
|
|
{
|
|
SearchString = null;
|
|
//ReportTitle = "Proms - Referenced Object Search";
|
|
ReportTitle = string.Format("Search For Referenced Object:\n{0}", cmboTreeROs.Text);
|
|
//TypesSelected = "Searched Step Types: " + ((typstr != null) ? typstr : "All Step Types");
|
|
if (cbxFndUnLnkROVals.Enabled && cbxFndUnLnkROVals.Checked)
|
|
{
|
|
SearchResults = ItemInfoList.GetListFromTextSearch(DVISearchList, TypeSearchList, ROSearchList, cbxBooleanTxtSrch.Checked ? 2 : cbxCaseSensitive.Checked ? 1 : 0, ItemSearchIncludeLinks.Nothing, includeRTFformat, includeSpecialChars, unitPrefix);
|
|
cmbResultsStyleIndex = 3; // display step text in results
|
|
}
|
|
else
|
|
{
|
|
SearchResults = ItemInfoList.GetListFromROSearch(DVISearchList, TypeSearchList, ROSearchList, unitPrefix);
|
|
cmbResultsStyleIndex = 3; // display step text in results
|
|
}
|
|
}
|
|
else if (tabSearchTypes.SelectedTab == tabSearchTypes.Tabs[3]) //Transition Search
|
|
{
|
|
ReportTitle = string.Format("Search For Transitions: Transition Type: {0}, Transition Category: {1}", cbxTranFormat.SelectedItem, cbxTranCategory.SelectedItem);
|
|
string docVersionList = string.Empty;
|
|
if (lstCheckedDocVersions.Count == 0)
|
|
docVersionList = cbxTranVersion.Tag.ToString();
|
|
else
|
|
{
|
|
string sep = string.Empty;
|
|
foreach (DocVersionInfo dvi in lstCheckedDocVersions)
|
|
{
|
|
docVersionList += sep + dvi.VersionID.ToString();
|
|
sep = ",";
|
|
}
|
|
}
|
|
// added TypeSearchList for bug fix B2015-055
|
|
SearchResults = ItemInfoList.GetListFromTransitionSearch(docVersionList, cbxTranFormat.SelectedIndex - 1, cbxTranCategory.SelectedItem.ToString() == "All" ? "" : cbxTranCategory.SelectedItem.ToString(), TypeSearchList);
|
|
cmbResultsStyleIndex = 3; // display step text in results
|
|
}
|
|
if (SearchResults != null)
|
|
{
|
|
AddMessageForEmptyAnnotations();
|
|
if (cmbResultsStyleIndex == 3 && cmbResultsStyle.Items.Count == 3) cmbResultsStyleIndex--;
|
|
cmbResultsStyle.SelectedIndex = cmbResultsStyleIndex;
|
|
DisplayResults();
|
|
if (SearchResults != null && SearchResults.Count == 0)
|
|
{
|
|
MessageBox.Show("No Matches Found.", "Search");
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
while (ex.InnerException != null)
|
|
ex = ex.InnerException;
|
|
string tmpmsg = (cbxBooleanTxtSrch.Checked && ex.Message.Contains("Syntax error") && ex.Message.Contains("full-text search")) ?
|
|
"Place \"\" around words that you are searching for, so that parser can better understand what you are trying to find" :
|
|
ex.Message;
|
|
MessageBox.Show(tmpmsg, "Search Error: " + ex.GetType().Name);
|
|
}
|
|
finally
|
|
{
|
|
Cursor = savcursor;
|
|
}
|
|
//Console.WriteLine("{0} Milliseconds", TimeSpan.FromTicks(DateTime.Now.Ticks - start.Ticks).TotalMilliseconds);
|
|
OnSearchComplete(new DisplaySearchEventArgs(TimeSpan.FromTicks(DateTime.Now.Ticks - start.Ticks)));
|
|
//if (VlnSettings.DebugMode)
|
|
// MessageBox.Show(string.Format("{0} Milliseconds", TimeSpan.FromTicks(DateTime.Now.Ticks - start.Ticks).TotalMilliseconds));
|
|
}
|
|
|
|
private void AddMessageForEmptyAnnotations()
|
|
{
|
|
bool hasAnnot = false;
|
|
foreach (ItemInfo ii in SearchResults)
|
|
{
|
|
if (ii.ItemAnnotationCount > 0)
|
|
{
|
|
// RHM - can an iteminfo have an itemannotationcount>0 and not have searchannotationtext.
|
|
if (ii.SearchAnnotationText == null) ii.SearchAnnotationText = AnnotationInfo.Get(ii.ItemAnnotations[0].AnnotationID).SearchText;
|
|
}
|
|
|
|
if (ii.SearchAnnotationText == null) ii.SearchAnnotationText = "None - [" + ii.DisplayText + "]";
|
|
else
|
|
{
|
|
hasAnnot = true;
|
|
}
|
|
}
|
|
cmbResultsStyle.Items.Clear();
|
|
cmbResultsStyle.Items.Add(comboItem1);
|
|
cmbResultsStyle.Items.Add(comboItem2);
|
|
if (hasAnnot) cmbResultsStyle.Items.Add(comboItem3);
|
|
cmbResultsStyle.Items.Add(comboItem4);
|
|
}
|
|
|
|
private void cbxTextSearchText_Leave(object sender, EventArgs e)
|
|
{
|
|
InsertSearchCriteria();
|
|
}
|
|
|
|
private void InsertSearchCriteria()
|
|
{
|
|
if (!cbxTextSearchText.Text.Equals(string.Empty))
|
|
{
|
|
string tstr = cbxTextSearchText.Text;
|
|
// if its already exists in the list - remove it
|
|
if (cbxTextSearchText.Items.Contains(tstr))
|
|
cbxTextSearchText.Items.Remove(tstr);
|
|
// Add the new criteria to the top of the list
|
|
cbxTextSearchText.Items.Insert(0, tstr);
|
|
// set the text to the new criteria
|
|
cbxTextSearchText.Text = tstr;
|
|
// If there are more than 10 remove the last one
|
|
if (cbxTextSearchText.Items.Count > 10)
|
|
cbxTextSearchText.Items.RemoveAt(10);
|
|
}
|
|
}
|
|
|
|
private void cbxSrchTypeUsage_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
gpSrchText.Enabled = !cbxSrchTypeUsage.Checked;
|
|
if (!cbxSrchTypeUsage.Checked)
|
|
xpStepTypes.TitleStyle.BackColor1.Color = saveXpStepTypeTitleColor;
|
|
else
|
|
{
|
|
xpStepTypes.Expanded = true;
|
|
if (lstCheckedStepTypes.Count == 0)
|
|
xpStepTypes.TitleStyle.BackColor1.Color = Color.Crimson;
|
|
advTreeStepTypes.Focus();
|
|
|
|
}
|
|
}
|
|
|
|
private void rbtnSrchTxt_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
if (rbtnSrchTxt.Checked)
|
|
cbxTextSearchText.Focus();
|
|
|
|
}
|
|
|
|
|
|
private void gpSrchText_EnabledChanged(object sender, EventArgs e)
|
|
{
|
|
if (gpSrchText.Enabled)
|
|
gpSrchText.Style.BackColor = cbxBooleanTxtSrch.Checked ? Color.Orange : Color.Yellow;
|
|
else
|
|
gpSrchText.Style.BackColor = saveGpSrchTextColor;
|
|
}
|
|
|
|
private void tabStepTypeSearch_Click(object sender, EventArgs e)
|
|
{
|
|
if (rbtnSrchTxt.Checked)
|
|
{
|
|
cbxTextSearchText.Focus();
|
|
cbxBooleanTxtSrch_CheckedChanged(sender, e);
|
|
}
|
|
}
|
|
|
|
private void ProcessEnterKey(object sender, KeyPressEventArgs e)
|
|
{
|
|
if (e.KeyChar == '\r') // enter key pressed
|
|
{
|
|
e.Handled = true;
|
|
btnSearch_Click(sender, e);
|
|
if (sender == cbxTextSearchText)
|
|
InsertSearchCriteria();
|
|
else
|
|
InsertAnnotationSearchCriteria();
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
#region ProcSetSelection
|
|
private int _MyRODbID;
|
|
private void advTreeProcSets_AfterCheck(object sender, DevComponents.AdvTree.AdvTreeCellEventArgs e)
|
|
{
|
|
DevComponents.AdvTree.Node n = advTreeProcSets.SelectedNode;
|
|
dicSelectedDocVersionNodes[n] = n.Checked;
|
|
if (n.Checked)
|
|
{
|
|
n.Style = DevComponents.AdvTree.NodeStyles.Apple;
|
|
lstCheckedDocVersions.Add((DocVersionInfo)n.Tag);
|
|
if (lstCheckedDocVersions.Count == 1)//Mydocversion == null)
|
|
{
|
|
Mydocversion = (DocVersionInfo)n.Tag;
|
|
advTreeStepTypesFillIn();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
n.Style = null;
|
|
lstCheckedDocVersions.Remove((DocVersionInfo)n.Tag);
|
|
if (lstCheckedDocVersions.Count == 1)
|
|
{
|
|
if (Mydocversion != lstCheckedDocVersions[0])
|
|
{
|
|
Mydocversion = lstCheckedDocVersions[0];
|
|
advTreeStepTypesFillIn();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (lstCheckedDocVersions.Count == 0)
|
|
Mydocversion = null;
|
|
// do this if either none, or more than one procedure set selected
|
|
advTreeStepTypes.Nodes.Clear();
|
|
lstCheckedStepTypes.Clear();
|
|
lstCheckedStepTypesStr.Clear();
|
|
Node newnode = new DevComponents.AdvTree.Node();
|
|
newnode.Text = "....select a procedure set for types to appear...";
|
|
advTreeStepTypes.Nodes.Add(newnode);
|
|
buildStepTypePannelTitle();
|
|
}
|
|
}
|
|
//// Enable the RO combo list only if at least one procedure set node
|
|
//// is selected
|
|
//cmboTreeROs.Enabled = (lstCheckedDocVersions.Count > 0);
|
|
//gpFindROs.Enabled = cmboTreeROs.Enabled;
|
|
|
|
SetupContextMenu();
|
|
|
|
buildSetToSearchPanelTitle();
|
|
}
|
|
|
|
private void cbxTranFormatFillIn(string vid)
|
|
{
|
|
vid = vid.IndexOf(",") < 0 ? vid : vid.Substring(0, cbxTranVersion.Tag.ToString().IndexOf(","));
|
|
DocVersionInfo dvi = DocVersionInfo.Get(int.Parse(vid));
|
|
TransTypeList ttl = dvi.ActiveFormat.PlantFormat.FormatData.TransData.TransTypeList;
|
|
cbxTranFormat.Items.Clear();
|
|
cbxTranFormat.Items.Add("All");
|
|
for (int i = 0; i < ttl.MaxIndex; i++)
|
|
cbxTranFormat.Items.Add(new TransItem(ttl[i].TransMenu.Replace("?.", ""), ttl[i].TransFormat.Replace("?.", "")));
|
|
cbxTranFormat.SelectedIndex = 0;
|
|
cbxTranCategory.Items.Clear();
|
|
cbxTranCategory.Items.Add("All");
|
|
cbxTranCategory.Items.Add("Internal");
|
|
cbxTranCategory.Items.Add("External");
|
|
cbxTranCategory.Items.Add("Outside");
|
|
cbxTranCategory.SelectedIndex = 0;
|
|
/*
|
|
groupPanelTranFmt.Style.BackColor = (_CurTrans == null && _TranFmtIndx==0) ? Color.Yellow : Color.Orange;
|
|
E_TransUI etm = (E_TransUI)_CurItemFrom.ActiveFormat.PlantFormat.FormatData.TransData.TransTypeList[_TranFmtIndx].TransUI;
|
|
_DoingRange = (etm & E_TransUI.StepLast) == E_TransUI.StepLast;
|
|
*/
|
|
}
|
|
|
|
|
|
private void xpSetToSearch_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
|
{
|
|
buildSetToSearchPanelTitle();
|
|
}
|
|
|
|
private void buildSetToSearchPanelTitle()
|
|
{
|
|
if (lstCheckedDocVersions.Count == 0)
|
|
{
|
|
xpSetToSearch.TitleText = string.Format("All Procedure Sets Selected");
|
|
xpSetToSearch.TitleStyle.BackColor1.Color = Color.PapayaWhip;
|
|
btnSearch.Enabled = true;
|
|
tabSearchTypes.Enabled = true; // enable all the search tabs
|
|
// xpSetToSearch.Expanded = true;
|
|
// xpSetToSearch.TitleStyle.BackColor1.Color = Color.Crimson;
|
|
// btnSearch.Enabled = false;
|
|
// tabSearchTypes.Enabled = false; // disable all the search tabs
|
|
// //if (xpSetToSearch.Expanded)
|
|
// xpSetToSearch.TitleText = "Select Procedure Sets to Search";
|
|
// //else
|
|
// // xpSetToSearch.TitleText = "Click Here to Select Procedure Sets";
|
|
|
|
// //xpSetToSearch.TitleStyle.BackColor1.Color = saveXpSetToSearchColor;
|
|
}
|
|
else //if (lstCheckedDocVersions.Count > 0)
|
|
{
|
|
// display the number of selected procedure sets whether pannel is expanded or not
|
|
xpSetToSearch.TitleText = string.Format("{0} Procedure Sets Selected", lstCheckedDocVersions.Count);
|
|
xpSetToSearch.TitleStyle.BackColor1.Color = Color.PapayaWhip;
|
|
btnSearch.Enabled = true;
|
|
tabSearchTypes.Enabled = true; // enable all the search tabs
|
|
}
|
|
|
|
}
|
|
|
|
private void advTreeProcSets_AfterNodeSelect(object sender, AdvTreeNodeEventArgs e)
|
|
{
|
|
DevComponents.AdvTree.Node n = advTreeProcSets.SelectedNode;
|
|
if (advTreeStepTypes.Nodes.Count == 1 && n.Checked)
|
|
{
|
|
Mydocversion = (DocVersionInfo)n.Tag;
|
|
advTreeStepTypesFillIn();
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region StepTypeSelection
|
|
private void advTreeStepTypes_AfterCheck(object sender, DevComponents.AdvTree.AdvTreeCellEventArgs e)
|
|
{
|
|
xpStepTypes.TitleStyle.BackColor1.Color = saveXpStepTypeTitleColor;
|
|
DevComponents.AdvTree.Node n = advTreeStepTypes.SelectedNode;
|
|
StepData sd = (StepData)n.Tag;
|
|
if (n.Checked)
|
|
{
|
|
n.Style = DevComponents.AdvTree.NodeStyles.Apple;
|
|
if (sd == null)
|
|
{
|
|
if (!lstCheckedStepTypesStr.Contains("Accessory Sections"))
|
|
{
|
|
lstCheckedStepTypes.Add(0); //use zero to identify attachment search
|
|
lstCheckedStepTypesStr.Add("Accessory Sections");
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (!lstCheckedStepTypesStr.Contains(advTreeStepTypes.SelectedNode.Text))
|
|
{
|
|
lstCheckedStepTypes.Add((int)sd.Index);
|
|
lstCheckedStepTypesStr.Add(advTreeStepTypes.SelectedNode.Text);
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
n.Style = null;
|
|
if (sd == null)
|
|
{
|
|
lstCheckedStepTypesStr.Remove("Accessory Sections");
|
|
lstCheckedStepTypes.Remove(0);
|
|
}
|
|
else
|
|
{
|
|
lstCheckedStepTypes.Remove((int)sd.Index);
|
|
//lstCheckedStepTypesStr.Remove(sd.Type);
|
|
lstCheckedStepTypesStr.Remove(advTreeStepTypes.SelectedNode.Text);
|
|
}
|
|
}
|
|
buildStepTypePannelTitle();
|
|
|
|
}
|
|
|
|
private void xpStepTypes_ExpandedChanged(object sender, ExpandedChangeEventArgs e)
|
|
{
|
|
buildStepTypePannelTitle();
|
|
}
|
|
|
|
private void buildStepTypePannelTitle()
|
|
{
|
|
if (lstCheckedStepTypes.Count == 0)
|
|
{
|
|
if (xpStepTypes.Expanded)
|
|
{
|
|
xpStepTypes.TitleText = "Select Step Elements To Search";//"Select Types To Search";
|
|
advTreeStepTypes.Focus();
|
|
advTreeStepTypes.Nodes[0].SelectedCell = advTreeStepTypes.Nodes[0].Cells[0];
|
|
}
|
|
else
|
|
xpStepTypes.TitleText = "Click Here To Search By Step Element";//"Click Here To Search By Types";
|
|
|
|
xpStepTypes.TitleStyle.BackColor1.Color = saveXpStepTypeTitleColor;
|
|
}
|
|
else //lstCheckedStepTypes.Count > 0
|
|
{
|
|
// show how many selected whether pannel is expanded or not
|
|
//xpStepTypes.TitleText = string.Format("{0} Step Styles Selected", lstCheckedStepTypes.Count);
|
|
xpStepTypes.TitleText = string.Format("{0} Step Element{0} Selected", lstCheckedStepTypes.Count, (lstCheckedStepTypes.Count > 1)?"s":"");
|
|
xpStepTypes.TitleStyle.BackColor1.Color = Color.PapayaWhip;
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region ContextMenu
|
|
|
|
private void btnSym_Click(object sender, EventArgs e)
|
|
{
|
|
DevComponents.DotNetBar.ButtonItem b = (DevComponents.DotNetBar.ButtonItem)sender;
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = (string)b.Text;
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = (string)b.Text;
|
|
}
|
|
|
|
private void cmFndTxtInsHardSp_Click(object sender, EventArgs e)
|
|
{
|
|
// We use \u00A0 to represent a hard space because it show in the search text field as a blank
|
|
// It will get translated to a real hard space character prior to searching
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = "\u00A0";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = "\u00A0";
|
|
}
|
|
// Changed to a public so that it could be called after the /DB parameter has been processed
|
|
public void SetupContextMenu()
|
|
{
|
|
galSymbols.SubItems.Clear();
|
|
// Bug fix B2014-057
|
|
// if we are searching for a symbol character in all procedure sets MyDocVersion is null
|
|
// when MyDocVersion is null, get the symbol list directly from the PROMS base format (BaseAll.xml)
|
|
// this will populate the context menu of the Search text entry field so that a symbol can be selected without first selecting a procedure set
|
|
FormatData fmtdata = (_MyDocVersion != null) ? _MyDocVersion.ActiveFormat.PlantFormat.FormatData : FormatInfo.PROMSBaseFormat.FormatData;
|
|
if (fmtdata != null && fmtdata.SymbolList != null)
|
|
{
|
|
SymbolList sl = fmtdata.SymbolList;
|
|
if (sl == null || sl.Count <= 0)
|
|
{
|
|
MessageBox.Show("No symbols are available, check with administrator");
|
|
return;
|
|
}
|
|
foreach (Symbol sym in sl)
|
|
{
|
|
DevComponents.DotNetBar.ButtonItem btnCM = new DevComponents.DotNetBar.ButtonItem();
|
|
btnCM.Text = string.Format("{0}", (char)sym.Unicode);
|
|
// to name button use unicode rather than desc, desc may have spaces or odd chars
|
|
btnCM.Name = "btnCM" + sym.Unicode.ToString();
|
|
btnCM.Tooltip = sym.Desc;
|
|
btnCM.Tag = string.Format(@"{0}", sym.Unicode);
|
|
btnCM.FontBold = true;
|
|
btnCM.Click += new System.EventHandler(btnSym_Click);
|
|
galSymbols.SubItems.Add(btnCM);
|
|
}
|
|
}
|
|
}
|
|
|
|
private void cmFndTxtCut_Click(object sender, EventArgs e)
|
|
{
|
|
Clipboard.Clear();
|
|
DataObject myDO = new DataObject(DataFormats.Text, cbxTextSearchAnnotation.Focused ? cbxTextSearchAnnotation.SelectedText : cbxTextSearchText.SelectedText);
|
|
Clipboard.SetDataObject(myDO);
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = "";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = "";
|
|
|
|
}
|
|
|
|
private void cmFndTxtCopy_Click(object sender, EventArgs e)
|
|
{
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
DataObject myDO = new DataObject(DataFormats.Text, cbxTextSearchAnnotation.Focused ? cbxTextSearchAnnotation.SelectedText : cbxTextSearchText.SelectedText);
|
|
if (cbxTextSearchText.Focused || cbxTextSearchAnnotation.Focused)
|
|
{
|
|
Clipboard.Clear();
|
|
Clipboard.SetDataObject(myDO);
|
|
}
|
|
}
|
|
|
|
private void cmFndTxtPaste_Click(object sender, EventArgs e)
|
|
{
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
IDataObject myDO = Clipboard.GetDataObject();
|
|
//if (myDO.GetDataPresent(DataFormats.Rtf))
|
|
//{
|
|
// RichTextBox rtb = new RichTextBox();
|
|
// rtb.SelectedRtf = ItemInfo.StripLinks(myDO.GetData(DataFormats.Rtf).ToString());
|
|
|
|
// if (cbxTextSearchAnnotation.Focused)
|
|
// cbxTextSearchAnnotation.SelectedText = rtb.Text;
|
|
// else if (cbxTextSearchText.Focused)
|
|
// cbxTextSearchText.SelectedText = rtb.Text;// .SelectedText;//myDO.GetData(DataFormats.Text,true).ToString();
|
|
//}
|
|
//else if (myDO.GetDataPresent(DataFormats.Text))
|
|
if (myDO.GetDataPresent(DataFormats.Text))
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = Clipboard.GetText();
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = Clipboard.GetText();//myDO.GetData(DataFormats.Text).ToString();
|
|
}
|
|
|
|
private void btnCMIFindText_PopupOpen(object sender, PopupOpenEventArgs e)
|
|
{
|
|
// the context menu is available with just a mouse hover, even if the combo box does not have focus
|
|
// ... set the focus to which ever combo box initiated the context menu
|
|
if (tabAnnotationSearch.IsSelected)
|
|
{
|
|
if (!cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.Focus();
|
|
}
|
|
else if (tabStepTypeSearch.IsSelected)
|
|
{
|
|
if (!cbxTextSearchText.Focused)
|
|
cbxTextSearchText.Focus();
|
|
}
|
|
}
|
|
#endregion
|
|
private void buttonItem2_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = "*";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = "*";
|
|
|
|
}
|
|
private void buttonItem3_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = "?";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = "?";
|
|
|
|
}
|
|
private void buttonItem4_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = "?*";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = "?*";
|
|
}
|
|
private void cbxBooleanTxtSrch_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
// If the Boolean search is not working - run the following two commands in SQL:
|
|
// use veproms
|
|
// ALTER FULLTEXT INDEX ON Contents START FULL POPULATION
|
|
// ALTER FULLTEXT INDEX ON Documents START FULL POPULATION
|
|
// use master
|
|
//Boolean search uses a list of noise, or STOP, words. This list can be found
|
|
// in file: C:\Program Files\Microsoft SQL Server\MSSQL.1\MSSQL\FTData\noiseenu.txt.
|
|
// Another file exists in that directory, tsenu.xml, this is a thesaurus. RHM tried
|
|
// to use around 2010, but couldn't get it to work for words like 'dg' versus 'diesel generator',
|
|
// and 'sg' versus 'steam generator'.
|
|
if (cbxBooleanTxtSrch.Checked)
|
|
{
|
|
gpSrchText.Style.BackColor = Color.Orange;
|
|
btnCMIFindText.SubItems[1].SubItems[2].Visible = false;
|
|
btnCMIFindText.SubItems[1].SubItems[3].Visible = true;
|
|
//cbxCaseSensitive.Checked = false;
|
|
//cbxCaseSensitive.Enabled = false;
|
|
//cbxIncROTextSrch.Checked = true;
|
|
//cbxIncROTextSrch.Enabled = false;
|
|
cbxCaseSensitive.Enabled = false;
|
|
cbxIncROTextSrch.Enabled = false;
|
|
}
|
|
else
|
|
{
|
|
gpSrchText.Style.BackColor = Color.Yellow;
|
|
btnCMIFindText.SubItems[1].SubItems[2].Visible = true;
|
|
btnCMIFindText.SubItems[1].SubItems[3].Visible = false;
|
|
//cbxCaseSensitive.Enabled = true;
|
|
//cbxIncROTextSrch.Enabled = true;
|
|
cbxCaseSensitive.Enabled = true;
|
|
cbxIncROTextSrch.Enabled = true;
|
|
}
|
|
}
|
|
private void cbxBooleanAnoTxtSrch_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
if (cbxBooleanAnoTxtSrch.Checked)
|
|
{
|
|
gpSrchAnnoText.Style.BackColor = Color.Orange;
|
|
btnCMIFindText.SubItems[1].SubItems[2].Visible = false;
|
|
btnCMIFindText.SubItems[1].SubItems[3].Visible = true;
|
|
}
|
|
else
|
|
{
|
|
gpSrchAnnoText.Style.BackColor = Color.Yellow;
|
|
btnCMIFindText.SubItems[1].SubItems[2].Visible = true;
|
|
btnCMIFindText.SubItems[1].SubItems[3].Visible = false;
|
|
}
|
|
}
|
|
private void btnAND_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = " AND ";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = " AND ";
|
|
|
|
}
|
|
private void btnOR_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = " OR ";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = " OR ";
|
|
|
|
}
|
|
private void btnNOT_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbxTextSearchAnnotation.Focused)
|
|
cbxTextSearchAnnotation.SelectedText = " NOT ";
|
|
else if (cbxTextSearchText.Focused)
|
|
cbxTextSearchText.SelectedText = " NOT ";
|
|
}
|
|
private void tabAnnotationSearch_Click(object sender, EventArgs e)
|
|
{
|
|
cbxAnnoTypes.Focus();
|
|
cbxBooleanAnoTxtSrch_CheckedChanged(sender, e);
|
|
}
|
|
private void btnClearSearchResults_Click(object sender, EventArgs e)
|
|
{
|
|
lbSrchResults.DataSource = null;
|
|
_SearchResults = null;
|
|
DisplayResults();
|
|
}
|
|
private void btnLoadSearchResults_Click(object sender, System.EventArgs e)
|
|
{
|
|
if (ofdSearchResults.ShowDialog(this) == DialogResult.OK)
|
|
{
|
|
|
|
lbSrchResults.DataSource = null;
|
|
lbSrchResults.Items.Clear();
|
|
toolTip1.SetToolTip(lbSrchResults, null);
|
|
Cursor = Cursors.WaitCursor;
|
|
SearchResults = ItemInfoList.GetByContentID(0);
|
|
int k = ofdSearchResults.FilterIndex;
|
|
switch (k)
|
|
{
|
|
case 1: //xml
|
|
{
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.Load(ofdSearchResults.FileName);
|
|
int sti = int.Parse(xd.SelectSingleNode("search/criteria/@index").InnerText);
|
|
switch (sti)
|
|
{
|
|
case 0: //text
|
|
{
|
|
cbxTextSearchText.Text = xd.SelectSingleNode("search/criteria/@text").InnerText;
|
|
cbxCaseSensitive.Checked = bool.Parse(xd.SelectSingleNode("search/criteria/@case").InnerText);
|
|
cbxIncROTextSrch.Checked = bool.Parse(xd.SelectSingleNode("search/criteria/@ro").InnerText);
|
|
tabSearchTypes.SelectedTab = tabStepTypeSearch;
|
|
//cmbResultsStyle.SelectedIndex = int.Parse(xd.SelectSingleNode("search/results/@style").InnerText);
|
|
cmbResultsStyle.Text = xd.SelectSingleNode("search/results/@style").InnerText;
|
|
System.Xml.XmlNodeList nl = xd.SelectNodes("search/results/item");
|
|
foreach (System.Xml.XmlNode nd in nl)
|
|
{
|
|
int itemID = int.Parse(nd.Attributes.GetNamedItem("id").InnerText);
|
|
ItemInfo ii = ItemInfo.Get(itemID);
|
|
SearchResults.AddItemInfo(ii);
|
|
}
|
|
break;
|
|
}
|
|
case 1: //annotation
|
|
{
|
|
cbxAnnoTypes.Text = xd.SelectSingleNode("search/criteria/@type").InnerText;
|
|
cbxTextSearchAnnotation.Text = xd.SelectSingleNode("search/criteria/@text").InnerText;
|
|
cbxCaseSensitiveAnnoText.Checked = bool.Parse(xd.SelectSingleNode("search/criteria/@case").InnerText);
|
|
tabSearchTypes.SelectedTab = tabAnnotationSearch;
|
|
cmbResultsStyle.Text = xd.SelectSingleNode("search/results/@style").InnerText;
|
|
System.Xml.XmlNodeList nl = xd.SelectNodes("search/results/item");
|
|
foreach (System.Xml.XmlNode nd in nl)
|
|
{
|
|
int itemID = int.Parse(nd.Attributes.GetNamedItem("id").InnerText);
|
|
ItemInfo ii = ItemInfo.Get(itemID);
|
|
SearchResults.AddItemInfo(ii);
|
|
}
|
|
break;
|
|
}
|
|
case 2: //referenced object
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
break;
|
|
}
|
|
case 2: //csv
|
|
{
|
|
break;
|
|
}
|
|
case 3: //tsv
|
|
{
|
|
break;
|
|
}
|
|
case 4: //bsf
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
DisplayResults();
|
|
Cursor = Cursors.Default;
|
|
}
|
|
}
|
|
|
|
private void btnSaveSearchResults_Click(object sender, System.EventArgs e)
|
|
{
|
|
ICollection<ItemInfo> myList = lbSrchResults.DataSource as ICollection<ItemInfo>;
|
|
if (sfdSearchResults.ShowDialog(this) == DialogResult.OK)
|
|
{
|
|
int k = sfdSearchResults.FilterIndex;
|
|
switch (k)
|
|
{
|
|
case 1: //xml
|
|
{
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
System.Xml.XmlElement xe = xd.CreateElement("search");
|
|
xd.AppendChild(xe);
|
|
|
|
int sti = tabSearchTypes.SelectedTabIndex;
|
|
switch (sti)
|
|
{
|
|
case 0: //text
|
|
{
|
|
xe = xd.CreateElement("criteria");
|
|
System.Xml.XmlAttribute xa = xd.CreateAttribute("index");
|
|
xa.InnerText = sti.ToString();
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("text");
|
|
xa.InnerText = cbxTextSearchText.Text;
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("case");
|
|
xa.InnerText = cbxCaseSensitive.Checked.ToString();
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("ro");
|
|
xa.InnerText = cbxIncROTextSrch.Checked.ToString();
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xd.DocumentElement.AppendChild(xe);
|
|
xe = xd.CreateElement("results");
|
|
xa = xd.CreateAttribute("style");
|
|
//xa.InnerText = cmbResultsStyle.SelectedIndex.ToString();
|
|
xa.InnerText = cmbResultsStyle.Text;
|
|
xe.Attributes.SetNamedItem(xa);
|
|
foreach (ItemInfo ii in myList)
|
|
{
|
|
System.Xml.XmlElement xee = xd.CreateElement("item");
|
|
xa = xd.CreateAttribute("id");
|
|
xa.InnerText = ii.ItemID.ToString();
|
|
xee.Attributes.SetNamedItem(xa);
|
|
xe.AppendChild(xee);
|
|
}
|
|
xd.DocumentElement.AppendChild(xe);
|
|
xd.Save(sfdSearchResults.FileName);
|
|
break;
|
|
}
|
|
case 1: //annotation
|
|
{
|
|
xe = xd.CreateElement("criteria");
|
|
System.Xml.XmlAttribute xa = xd.CreateAttribute("index");
|
|
xa.InnerText = sti.ToString();
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("type");
|
|
xa.InnerText = cbxAnnoTypes.Text;
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("text");
|
|
xa.InnerText = cbxTextSearchAnnotation.Text;
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xa = xd.CreateAttribute("case");
|
|
xa.InnerText = cbxCaseSensitiveAnnoText.Checked.ToString();
|
|
xe.Attributes.SetNamedItem(xa);
|
|
xd.DocumentElement.AppendChild(xe);
|
|
xe = xd.CreateElement("results");
|
|
xa = xd.CreateAttribute("style");
|
|
//xa.InnerText = cmbResultsStyle.SelectedIndex.ToString();
|
|
xa.InnerText = cmbResultsStyle.Text;
|
|
xe.Attributes.SetNamedItem(xa);
|
|
foreach (ItemInfo ii in myList)
|
|
{
|
|
System.Xml.XmlElement xee = xd.CreateElement("item");
|
|
xa = xd.CreateAttribute("id");
|
|
xa.InnerText = ii.ItemID.ToString();
|
|
xee.Attributes.SetNamedItem(xa);
|
|
xe.AppendChild(xee);
|
|
}
|
|
xd.DocumentElement.AppendChild(xe);
|
|
xd.Save(sfdSearchResults.FileName);
|
|
break;
|
|
}
|
|
case 2: //referenced object
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
break;
|
|
}
|
|
case 2: //csv
|
|
{
|
|
break;
|
|
}
|
|
case 3: //tsv
|
|
{
|
|
break;
|
|
}
|
|
case 4: //bsf
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
//string fn = sfdSearchResults.FileName;
|
|
//StringBuilder sb = new StringBuilder();
|
|
//sb.Append("\"Location\"\t\"Type\"\t\"Text\"\t\"High-Level\"\t\"Annotations\"");
|
|
//foreach (ItemInfo myItem in myList)
|
|
//{
|
|
// sb.Append(string.Format("\r\n\"{0}\"\t\"{1}\"\t\"{2}\"\t\"{3}\"", myItem.ShortPath, myItem.ToolTip,
|
|
// myItem.DisplayText, !myItem.IsSection && !myItem.IsHigh ? (myItem.MyHLS == null ? "" : myItem.MyHLS.DisplayText) : ""));
|
|
// if (myItem.ItemAnnotationCount > 0)
|
|
// foreach (AnnotationInfo myAnnotation in myItem.ItemAnnotations)
|
|
// sb.Append(string.Format("\t\"{0}\"", myAnnotation.SearchText));
|
|
//}
|
|
//Clipboard.Clear();
|
|
//Clipboard.SetText(sb.ToString());
|
|
//MessageBox.Show("Results Copied to Clipboard", "Copy Results", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
}
|
|
|
|
private void btnCopySearchResults_Click(object sender, EventArgs e)
|
|
{
|
|
ICollection<ItemInfo> myList = lbSrchResults.DataSource as ICollection<ItemInfo>;
|
|
StringBuilder sb = new StringBuilder();
|
|
sb.Append("\"Location\"\t\"Type\"\t\"Text\"\t\"High-Level\"\t\"Annotations\"");
|
|
foreach (ItemInfo myItem in myList)
|
|
{
|
|
sb.Append(string.Format("\r\n\"{0}\"\t\"{1}\"\t\"{2}\"\t\"{3}\"", myItem.ShortPath, myItem.ToolTip,
|
|
myItem.DisplayText, !myItem.IsSection && !myItem.IsHigh ? (myItem.MyHLS == null ? "" : myItem.MyHLS.DisplayText) : ""));
|
|
if (myItem.ItemAnnotationCount > 0)
|
|
foreach (AnnotationInfo myAnnotation in myItem.ItemAnnotations)
|
|
sb.Append(string.Format("\t\"{0}\"", myAnnotation.SearchText));
|
|
}
|
|
Clipboard.Clear();
|
|
Clipboard.SetText(sb.ToString());
|
|
MessageBox.Show("Results Copied to Clipboard", "Copy Results", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private string _ReportTitle;
|
|
public string ReportTitle
|
|
{
|
|
get { return _ReportTitle; }
|
|
set { _ReportTitle = value; }
|
|
}
|
|
private string _SearchString = null;
|
|
public string SearchString
|
|
{
|
|
get { return _SearchString; }
|
|
set { _SearchString = value; }
|
|
}
|
|
private string _TypesSelected;
|
|
|
|
public string TypesSelected
|
|
{
|
|
get { return _TypesSelected; }
|
|
set { _TypesSelected = value; }
|
|
}
|
|
private void btnPrnSrchRslts_Click(object sender, EventArgs e)
|
|
{
|
|
OnPrintRequest(new DisplaySearchEventArgs(ReportTitle, TypesSelected, SearchString, lbSrchResults.DataSource as ICollection<ItemInfo>));
|
|
}
|
|
private void panSearchButtons_Resize(object sender, EventArgs e)
|
|
{
|
|
cmbResultsStyle.Left = labelX1.Right + 3;
|
|
cmbResultsStyle.Width = btnClearSearchResults.Left - cmbResultsStyle.Left - 3;
|
|
}
|
|
}
|
|
#region Annoation Search Type Class
|
|
// this class is used to generate the list of annotations to search.
|
|
// this also allow us to add a dummy type which is used to search for all annotations
|
|
public class AnnotationTypeSearch
|
|
{
|
|
private string _Name;
|
|
public string Name
|
|
{
|
|
get { return _Name; }
|
|
set { _Name = value; }
|
|
}
|
|
private string _ID;
|
|
public string ID
|
|
{
|
|
get { return _ID; }
|
|
set { _ID = value; }
|
|
}
|
|
public AnnotationTypeSearch(string name, string id)
|
|
{
|
|
_Name = name;
|
|
_ID = id;
|
|
}
|
|
|
|
}
|
|
#endregion
|
|
public class DisplaySearchEventArgs
|
|
{
|
|
private string _ReportTitle;
|
|
public string ReportTitle
|
|
{
|
|
get { return _ReportTitle; }
|
|
set { _ReportTitle = value; }
|
|
}
|
|
private string _SearchString = null;
|
|
public string SearchString
|
|
{
|
|
get { return _SearchString; }
|
|
set { _SearchString = value; }
|
|
}
|
|
private string _TypesSelected;
|
|
public string TypesSelected
|
|
{
|
|
get { return _TypesSelected; }
|
|
set { _TypesSelected = value; }
|
|
}
|
|
private ICollection<ItemInfo> _MyItemInfoList;
|
|
public ICollection<ItemInfo> MyItemInfoList
|
|
{
|
|
get { return _MyItemInfoList; }
|
|
set { _MyItemInfoList = value; }
|
|
}
|
|
private TimeSpan _HowLong = TimeSpan.FromTicks(0);
|
|
public TimeSpan HowLong
|
|
{
|
|
get { return _HowLong; }
|
|
set { _HowLong = value; }
|
|
}
|
|
public DisplaySearchEventArgs(TimeSpan howLong)
|
|
{
|
|
HowLong = howLong;
|
|
}
|
|
public DisplaySearchEventArgs(string reportTitle, string typesSelected, string searchString, ICollection<ItemInfo> myItemInfoList)
|
|
{
|
|
_ReportTitle = reportTitle;
|
|
_TypesSelected = typesSelected;
|
|
_MyItemInfoList = myItemInfoList;
|
|
_SearchString = searchString;
|
|
}
|
|
}
|
|
public delegate void DisplaySearchEvent(object sender, DisplaySearchEventArgs args);
|
|
}
|