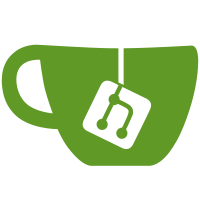
Added error handling for External Transitions to deleted Procedure. Added File extension parameter to Make Document DB Command Line Parameter now supports server name and ItemIDs. Added Separate Window Preference Added capability to Update Format and then close. Command Line Parameter /P= with no IDs shutsdown immediately. Changed code so that the Anotation panels stay expanded. Added Console Writeline output when Formats are updated. Attached Console Output to Parent. This allows the Console output to be output to a command window when a Batch FIle is used to Update Formats.
74 lines
3.7 KiB
C#
74 lines
3.7 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Windows.Forms;
|
|
using Volian.Base.Library;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
static class Program
|
|
{
|
|
private static readonly log4net.ILog _log = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
[System.Runtime.InteropServices.DllImport("KERNEL32.DLL")]
|
|
static extern bool AttachConsole(int dwProcessId);
|
|
private const int ATTACH_PARENT_PROCESS = -1; /// <summary>
|
|
/// The main entry point for the application.
|
|
/// </summary>
|
|
[STAThread]
|
|
static void Main()
|
|
{
|
|
Application.EnableVisualStyles();
|
|
Application.SetCompatibleTextRenderingDefault(false);
|
|
// Attach to the parent process via AttachConsole SDK call
|
|
AttachConsole(ATTACH_PARENT_PROCESS);
|
|
if (System.Diagnostics.Process.GetCurrentProcess().ProcessName.ToLower().EndsWith("vshost"))
|
|
{
|
|
Application.Run(new frmVEPROMS());
|
|
}
|
|
else
|
|
{
|
|
Application.ThreadException += new System.Threading.ThreadExceptionEventHandler(Application_ThreadException);
|
|
Application.SetUnhandledExceptionMode(UnhandledExceptionMode.CatchException);
|
|
AppDomain.CurrentDomain.UnhandledException += new UnhandledExceptionEventHandler(CurrentDomain_UnhandledException);
|
|
Application.Run(new frmVEPROMS());
|
|
}
|
|
}
|
|
|
|
static void CurrentDomain_UnhandledException(object sender, UnhandledExceptionEventArgs e)
|
|
{
|
|
_log.Error("PROMS2010 Main UnhandledException", e.ExceptionObject as Exception);
|
|
Application.Exit();
|
|
}
|
|
|
|
static void Application_ThreadException(object sender, System.Threading.ThreadExceptionEventArgs e)
|
|
{
|
|
// Roaming folders copied to the server when the user logs off the client computer in a Domain environment.
|
|
// Windows uses the Local folder for application data that does not roam with the user. Usually this data is
|
|
// either machine specific or too large to roam. The AppData\Local folder in Windows Vista is the same as
|
|
// the Documents and Settings\username\Local Settings\Application Data folder in Windows XP.
|
|
// Windows uses the Roaming folder for application specific data, such as custom dictionaries, which are
|
|
// machine independent and should roam with the user profile. The AppData\Roaming folder in Windows Vista
|
|
// is the same as the Documents and Settings\username\Application Data folder in Windows XP.
|
|
// SpecialFolder.LocalApplicationData returns \Local & SpecialFolder.ApplicationData returns \Roaming
|
|
// for example - the SpecialFolder:
|
|
// LocalApplicationData: C:\Users\Kathy.VOLIAN0\AppData\Local
|
|
// ApplicationData: C:\Users\Kathy.VOLIAN0\AppData\Roaming
|
|
// CommonApplicationData: C:\ProgramData
|
|
// MyDocuments: C:\Users\Kathy.VOLIAN0\Documents
|
|
|
|
// The error log is created using log4Net. The variables that were tested were:
|
|
// <param name="File" value="${USERPROFILE}/My Documents/VEPROMS/ErrorLog.txt" /> - puts in document directory
|
|
// <param name="File" value="${APPDATA}/Volian/Proms2010/ErrorLog.txt" /> - puts in roaming directory
|
|
// <param name="File" value="${LOCALAPPDATA}/Temp/VEPROMS/ErrorLog.txt" /> - Vista - puts in local directory. !Exist for XP
|
|
|
|
// Decided to use 'documents directory' for error log file so that it is easily accessible by user:
|
|
string dpath = frmVEPROMS.ErrorLogFileName;
|
|
if (dpath == null || dpath == "")
|
|
dpath = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments) + @"\VEPROMS\ErrorLog.txt";
|
|
_log.Error("Proms Main ThreadException", e.Exception);
|
|
string msg = string.Format("An error has occurred so the program will terminate. Please send the error log, {0}, to Volian.",dpath);
|
|
if(!System.Environment.CommandLine.Contains("/P="))
|
|
MessageBox.Show(msg);
|
|
Application.Exit();
|
|
}
|
|
}
|
|
} |