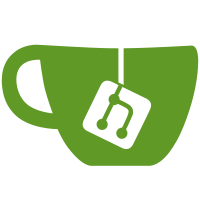
Load all at once code to support print. Open Command from the Start (PROMS2010) Menu. Enter key on treeview executes Open. The Treeview was retaining focus after opening procedures or accessory documents. This was fixed. Tab Bar initialized to the full width. Items were not always being displayed after the tree was clicked. The RO and Transition Info tabs are made invisible for accessory documents. The Tags, RO and Transition tabs are made invisible when no doucment is selected. Enter the DSO Framer window when it is created. Enter the Accessory page editor or step editor when a tab is selected. Remember the last selection in a step editor page when you return. Activate one of the remaining tabs when a tab is closed. Embed the pushpin images.
823 lines
25 KiB
C#
823 lines
25 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Drawing;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class StepPanel : Panel
|
|
{
|
|
#region Fields
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
/// <summary>
|
|
/// Procedure Item Info - Top ItemInfo
|
|
/// </summary>
|
|
private ItemInfo _MyProcedureItemInfo;
|
|
/// <summary>
|
|
/// This is not correct. There should be a dictionary of Section Layouts
|
|
/// </summary>
|
|
private StepSectionLayoutData _MyStepSectionLayoutData;
|
|
/// <summary>
|
|
/// Lookup Table to convert ItemInfo.ItemID to StepItem
|
|
/// </summary>
|
|
internal Dictionary<int, StepItem> _LookupStepItems;
|
|
/// <summary>
|
|
/// Currently selected RichTextBox
|
|
/// </summary>
|
|
private StepRTB _SelectedStepRTB = null;
|
|
/// <summary>
|
|
/// Currently selected ItemInfo
|
|
/// </summary>
|
|
internal ItemInfo _SelectedItemInfo;
|
|
private int _ItemMoving = 0;
|
|
private StepPanelSettings _MyStepPanelSettings;
|
|
private int _MaxRNO = -1; // TODO: Need to calculate MaxRNO on a section basis rather than for a panel
|
|
private Font _MyFont = null;
|
|
private Font _ProcFont = new Font("Arial", 12, FontStyle.Bold);
|
|
private Font _SectFont = new Font("Arial", 10, FontStyle.Bold);
|
|
private Font _StepFont = new Font("Arial", 10);
|
|
private Color _ActiveColor = Color.SkyBlue;
|
|
#if (DEBUG)
|
|
private Color _InactiveColor = Color.Linen;
|
|
private Color _TabColor = Color.Beige;
|
|
private Color _PanelColor = Color.LightGray;
|
|
#else
|
|
private Color _InactiveColor = Color.White;
|
|
private Color _TabColor = Color.White;
|
|
private Color _PanelColor = Color.White;
|
|
#endif
|
|
private bool _ShowLines = true;
|
|
private Graphics _MyGraphics = null;
|
|
private int _DPI = 0;
|
|
#endregion
|
|
#region Item Events
|
|
/// <summary>
|
|
/// Occurs when the user clicks tab of a StepItem
|
|
/// </summary>
|
|
public event StepPanelEvent ItemClick;
|
|
/// <summary>
|
|
/// Checks to see if the 'ItemClick' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnItemClick(object sender, StepPanelEventArgs args)
|
|
{
|
|
if (ItemClick != null) ItemClick(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the selected StepItem changes
|
|
/// </summary>
|
|
public event ItemSelectedChangedEvent ItemSelectedChanged;
|
|
/// <summary>
|
|
/// Checks to see if the 'ItemSelectedChanged' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnItemSelectedChanged(object sender, ItemSelectedChangedEventArgs args)
|
|
{
|
|
if (ItemSelectedChanged != null) ItemSelectedChanged(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user clicks on the Attachment Expander
|
|
/// </summary>
|
|
public event StepPanelAttachmentEvent AttachmentClicked;
|
|
/// <summary>
|
|
/// Checks to see if the 'AttachmentClicked' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnAttachmentClicked(object sender, StepPanelAttachmentEventArgs args)
|
|
{
|
|
if (AttachmentClicked != null) AttachmentClicked(sender, args);
|
|
else MessageBox.Show(args.MyStepItem.MyItemInfo.MyContent.MyEntry.MyDocument.DocumentTitle, "Unhandled Attachment Click", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
#endregion
|
|
#region Link Events
|
|
/// <summary>
|
|
/// Occurs when the user moves onto or off of a Link
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkActiveChanged;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkActiveChanged' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkActiveChanged(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkActiveChanged != null) LinkActiveChanged(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Active Changed", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to Insert a Transition
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkInsertTran;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkInsertTran' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkInsertTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkInsertTran != null) LinkInsertTran(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Insert Tran", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to Insert an RO
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkInsertRO;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkInsertRO' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkInsertRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkInsertRO != null) LinkInsertRO(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Insert RO", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user clicks a Link
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkClicked;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkClicked' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkClicked(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkClicked != null) LinkClicked(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Click", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to modify a Transition
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkModifyTran;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkModifyTran' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkModifyTran != null) LinkModifyTran(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Modify Tran", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user chooses to modify an RO
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkModifyRO;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkModifyRO' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkModifyRO != null) LinkModifyRO(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Modify RO", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public StepPanel()
|
|
{
|
|
InitializeComponent();
|
|
this.BackColorChanged += new EventHandler(StepPanel_BackColorChanged);
|
|
#if(DEBUG)
|
|
this.Paint += new PaintEventHandler(StepPanel_Paint);
|
|
this.DoubleClick += new EventHandler(StepPanel_DoubleClick); // Toggles Vertical lines on and off
|
|
#else
|
|
this.BackColor = Color.White;
|
|
#endif
|
|
this.AutoScroll = true;
|
|
}
|
|
public StepPanel(IContainer container)
|
|
{
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.BackColorChanged += new EventHandler(StepPanel_BackColorChanged);
|
|
#if(DEBUG)
|
|
this.Paint += new PaintEventHandler(StepPanel_Paint); // Toggles Vertical lines on and off
|
|
this.DoubleClick += new EventHandler(StepPanel_DoubleClick);
|
|
#else
|
|
this.BackColor = Color.White;
|
|
#endif
|
|
this.AutoScroll = true;
|
|
}
|
|
void StepPanel_BackColorChanged(object sender, EventArgs e)
|
|
{
|
|
// Walk through controls & set colors
|
|
InactiveColor = PanelColor = BackColor;
|
|
foreach (Control ctrl in Controls)
|
|
{
|
|
if (ctrl.GetType() == typeof(StepItem))
|
|
{
|
|
StepItem rtb = (StepItem)ctrl;
|
|
rtb.BackColor = BackColor;
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region Private Methods
|
|
/// <summary>
|
|
/// Expands an Item and It's Parents as need to display an item
|
|
/// </summary>
|
|
/// <param name="myItemInfo">Item to Expand</param>
|
|
private void ExpandAsNeeded(ItemInfo myItemInfo)
|
|
{
|
|
int id = myItemInfo.ItemID;
|
|
if (_LookupStepItems.ContainsKey(id)) // If the item is currently displayed
|
|
{
|
|
_LookupStepItems[id].AutoExpand(); // Expand it if it should expand
|
|
}
|
|
else
|
|
{
|
|
ExpandAsNeeded((ItemInfo)myItemInfo.ActiveParent); // Expand it's parent
|
|
if (!_LookupStepItems.ContainsKey(id)) // Expand Parent if not expanded
|
|
{
|
|
int parentId = ((ItemInfo)myItemInfo.ActiveParent).ItemID;
|
|
_LookupStepItems[parentId].AutoExpand();
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region Properties
|
|
/// <summary>
|
|
/// Procedure Item Info - Top ItemInfo
|
|
/// Get and Set - Set stes-up all of the
|
|
/// </summary>
|
|
public ItemInfo MyProcedureItemInfo
|
|
{
|
|
get { return _MyProcedureItemInfo; }
|
|
set
|
|
{
|
|
//// TIMING: DisplayItem.TimeIt("pMyItem Start");
|
|
_MyProcedureItemInfo = value;
|
|
if(value != null)
|
|
_MyStepSectionLayoutData = _MyProcedureItemInfo.ActiveFormat.PlantFormat.FormatData.SectData.StepSectionData.StepSectionLayoutData;
|
|
//// TIMING: DisplayItem.TimeIt("pMyItem Layout");
|
|
//this.Layout += new LayoutEventHandler(DisplayPanel_Layout);
|
|
//this.Scroll += new ScrollEventHandler(DisplayPanel_Scroll);
|
|
//// TIMING: DisplayItem.TimeIt("pMyItem Scroll");
|
|
Controls.Clear();
|
|
_LookupStepItems = new Dictionary<int, StepItem>();
|
|
//// TIMING: DisplayItem.TimeIt("pMyItem Clear");
|
|
//SuspendLayout();
|
|
StepItem tmpStepItem = new StepItem(_MyProcedureItemInfo, this, null, ChildRelation.None, false);
|
|
//ResumeLayout();
|
|
//// TIMING: DisplayItem.TimeIt("pMyItem End");
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Get or Set currently selected RichTextBox (StepRTB)
|
|
/// </summary>
|
|
public StepRTB SelectedStepRTB
|
|
{
|
|
get { return _SelectedStepRTB; }
|
|
set
|
|
{
|
|
value.BackColor = ActiveColor; // Set the active color
|
|
if (_SelectedStepRTB == value) return; // Same - No Change
|
|
if (_SelectedStepRTB != null)
|
|
{
|
|
_SelectedStepRTB.BackColor = InactiveColor;
|
|
_SelectedStepRTB.SaveText(); // Save any changes to the text
|
|
}
|
|
_SelectedStepRTB = value;
|
|
if (_SelectedItemInfo.ItemID != value.MyItemInfo.ItemID)
|
|
SelectedItemInfo = value.MyItemInfo;
|
|
//vlnStackTrace.ShowStack("_DisplayRTB = {0}", _DisplayRTB.MyItem.ItemID);// Show StackTrace
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Gets or Sets the SelectedItemInfo
|
|
/// Activates and Expands as necessary
|
|
/// </summary>
|
|
public ItemInfo SelectedItemInfo
|
|
{
|
|
get { return _SelectedItemInfo; }
|
|
set
|
|
{
|
|
//vlnStackTrace.ShowStack("SelectedItemInfo {0} => {1}",_SelectedItemInfo, value);
|
|
_SelectedItemInfo = value;
|
|
int id = value.ItemID;
|
|
ExpandAsNeeded(value);
|
|
StepItem itm = _LookupStepItems[id];
|
|
itm.ItemSelect();
|
|
OnItemSelectedChanged(this, new ItemSelectedChangedEventArgs(itm));
|
|
//vlnStackTrace.ShowStack("_ItemSelected = {0}", _ItemSelected.ItemID);// Show StackTrace
|
|
}
|
|
}
|
|
private bool _DisplayItemChanging = false;
|
|
public bool DisplayItemChanging
|
|
{
|
|
get { return _DisplayItemChanging; }
|
|
set { _DisplayItemChanging = value; }
|
|
}
|
|
/// <summary>
|
|
/// Returns the SelectedStepItem
|
|
/// </summary>
|
|
public StepItem SelectedStepItem
|
|
{
|
|
get { return (_SelectedItemInfo != null) ? _LookupStepItems[_SelectedItemInfo.ItemID] : null; }
|
|
}
|
|
/// <summary>
|
|
/// Displays the selected StepItem
|
|
/// </summary>
|
|
public void ItemShow()
|
|
{
|
|
if (_SelectedItemInfo != null)
|
|
{
|
|
SelectedStepItem.ItemShow();
|
|
OnItemSelectedChanged(this, new ItemSelectedChangedEventArgs(SelectedStepItem));
|
|
}
|
|
}
|
|
public void MouseWheel(MouseEventArgs e)
|
|
{
|
|
base.OnMouseWheel(e);
|
|
}
|
|
/// <summary>
|
|
/// Used to track movement other than scrolling
|
|
/// 0 - Indicates no other movement
|
|
/// > 0 - Indicates that other movement is happening
|
|
/// </summary>
|
|
public int ItemMoving
|
|
{
|
|
get { return _ItemMoving; }
|
|
set { _ItemMoving = value; }
|
|
}
|
|
/// <summary>
|
|
/// Lazy loaded StepPanelSettings
|
|
/// </summary>
|
|
public StepPanelSettings MyStepPanelSettings
|
|
{
|
|
get
|
|
{
|
|
if (_MyStepPanelSettings == null) _MyStepPanelSettings = new StepPanelSettings(this);
|
|
return _MyStepPanelSettings;
|
|
}
|
|
set { _MyStepPanelSettings = value;}
|
|
}
|
|
// TODO: This needs to move to StepItem and use the format for the current section
|
|
/// <summary>
|
|
/// Gets the MaxRNO from the StepSectionLayoutData
|
|
/// </summary>
|
|
public int MaxRNO
|
|
{
|
|
get
|
|
{
|
|
if(_MaxRNO == -1)
|
|
{
|
|
int pmode = Convert.ToInt32(_MyStepSectionLayoutData.PMode) - 1;
|
|
_MaxRNO = Convert.ToInt32( _MyStepSectionLayoutData.MaxRNOTable.Split(",".ToCharArray())[pmode]);
|
|
}
|
|
return _MaxRNO;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Gets or Sets the font for the Panel
|
|
/// </summary>
|
|
public Font MyFont
|
|
{
|
|
get { return _MyFont; }
|
|
set { _ProcFont = _SectFont = _StepFont = _MyFont = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the font for the Procedure Text
|
|
/// </summary>
|
|
public Font ProcFont
|
|
{
|
|
get { return _ProcFont; }
|
|
set { _ProcFont = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the font for the Section Text
|
|
/// </summary>
|
|
public Font SectFont
|
|
{
|
|
get { return _SectFont; }
|
|
set { _SectFont = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the Step Font
|
|
/// </summary>
|
|
public Font StepFont
|
|
{
|
|
get { return _StepFont; }
|
|
set { _StepFont = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or Sets the Active Color for the Panel
|
|
/// </summary>
|
|
public Color ActiveColor
|
|
{
|
|
get { return _ActiveColor; }
|
|
set { _ActiveColor = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the InActive Color for the Panel
|
|
/// </summary>
|
|
public Color InactiveColor
|
|
{
|
|
get { return _InactiveColor; }
|
|
set { _InactiveColor = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the Tab Color
|
|
/// </summary>
|
|
public Color TabColor
|
|
{
|
|
get { return _TabColor; }
|
|
set { _TabColor = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets the Panel Color
|
|
/// </summary>
|
|
public Color PanelColor
|
|
{
|
|
get { return _PanelColor; }
|
|
set { _PanelColor = value;
|
|
BackColor = value;
|
|
}
|
|
}
|
|
#endregion
|
|
#region DisplayConversions
|
|
/// <summary>
|
|
/// gets a Graphic object for the panel
|
|
/// </summary>
|
|
public Graphics MyGraphics
|
|
{
|
|
get
|
|
{
|
|
if (_MyGraphics == null)
|
|
_MyGraphics = CreateGraphics();
|
|
return _MyGraphics;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Gets the DPI setting for the current graphics setting
|
|
/// </summary>
|
|
internal int DPI
|
|
{
|
|
get
|
|
{
|
|
if (_DPI == 0)
|
|
_DPI = Convert.ToInt32(MyGraphics.DpiX);
|
|
return _DPI;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Converts an integer value from Twips to Pixels
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public int ToDisplay(int value)
|
|
{
|
|
//return (DPI * value) / 864;
|
|
return (DPI * value) / 72;
|
|
}
|
|
/// <summary>
|
|
/// Converts an integer? value from Twips to Pixels
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public int ToDisplay(int? value)
|
|
{
|
|
return ToDisplay((int)value);
|
|
}
|
|
/// <summary>
|
|
/// Converts an string value from Twips to Pixels
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public int ToDisplay(string value)
|
|
{
|
|
return ToDisplay(Convert.ToInt32(value));
|
|
}
|
|
/// <summary>
|
|
/// Converts a value from a list in a string from Twips to Pixels
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public int ToDisplay(string value,int i)
|
|
{
|
|
string s = value.Split(",".ToCharArray())[i];
|
|
return ToDisplay(s);
|
|
}
|
|
#endregion
|
|
#region Debug Methods
|
|
/// <summary>
|
|
/// Gets or sets ShowLines so that vertical lines are shown for debugging purposes
|
|
/// </summary>
|
|
public bool ShowLines
|
|
{
|
|
get { return _ShowLines; }
|
|
set { _ShowLines = value; }
|
|
}
|
|
/// <summary>
|
|
/// Draw a vertical line
|
|
/// </summary>
|
|
/// <param name="g"></param>
|
|
/// <param name="x"></param>
|
|
private void VerticalLine(Graphics g, int x)
|
|
{
|
|
Pen bluePen = new Pen(Color.CornflowerBlue,1);
|
|
g.DrawLine(bluePen, x, 0, x, this.Height);
|
|
}
|
|
/// <summary>
|
|
/// Toggle the vertical lines on and off
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void StepPanel_DoubleClick(object sender, EventArgs e)
|
|
{
|
|
ShowLines = !ShowLines;
|
|
Refresh();
|
|
}
|
|
private void StepPanel_Paint(object sender, PaintEventArgs e)
|
|
{
|
|
if (ShowLines)
|
|
{
|
|
//int fifth = Height / 5;
|
|
//Rectangle r1 = new Rectangle(0, 0, Width, Height - fifth);
|
|
////Brush b = new System.Drawing.Drawing2D.LinearGradientBrush(r1, Color.FromArgb(128, 0, 32), Color.FromArgb(96, 0, 16), 90);
|
|
//Brush b = new System.Drawing.Drawing2D.LinearGradientBrush(r1, Color.FromArgb(255,128, 0, 32), Color.FromArgb(255,96, 0, 16),System.Drawing.Drawing2D.LinearGradientMode.Vertical);
|
|
//e.Graphics.FillRectangle(b, r1);
|
|
//r1 = new Rectangle(0, Height - fifth, Width, fifth);
|
|
//b = new System.Drawing.Drawing2D.LinearGradientBrush(r1, Color.FromArgb(255,96, 0, 16), Color.FromArgb(255,128, 0, 32), 90);
|
|
//e.Graphics.FillRectangle(b, r1);
|
|
//VerticalLine(e.Graphics, 60);
|
|
//VerticalLine(e.Graphics, 102);
|
|
//VerticalLine(e.Graphics, 415);
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Output all of the StepItem controls to the log
|
|
/// </summary>
|
|
private void ListControls()
|
|
{
|
|
// Walk through the controls and find the next control for each
|
|
if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("'Item','Next'");
|
|
foreach (Control control in Controls)
|
|
if (control.GetType() == typeof(StepItem))
|
|
{
|
|
StepItem rtb = (StepItem)control;
|
|
StepItem nxt = rtb.NextDownStepItem;
|
|
if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("{0},{1}", rtb.MyID, nxt == null ? 0 : nxt.MyID);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
public partial class StepPanelSettings
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
public StepPanelSettings(StepPanel panel)
|
|
{
|
|
_MyStepPanel = panel;
|
|
}
|
|
public StepPanelSettings()
|
|
{
|
|
}
|
|
private StepPanel _MyStepPanel;
|
|
[Browsable(false)]
|
|
public StepPanel MyStepPanel
|
|
{
|
|
get { return _MyStepPanel; }
|
|
set { _MyStepPanel = value; }
|
|
}
|
|
private float _CircleXOffset = -4;
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Horizontal Offset")]
|
|
public float CircleXOffset
|
|
{
|
|
get { return _CircleXOffset; }
|
|
set { _CircleXOffset = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private float _CircleYOffset = -13;
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Vertical Offset")]
|
|
public float CircleYOffset
|
|
{
|
|
get { return _CircleYOffset; }
|
|
set { _CircleYOffset = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private Font _CircleFont = new Font("Arial Unicode MS", 23);
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Font")]
|
|
public Font CircleFont
|
|
{
|
|
get { return _CircleFont; }
|
|
set { _CircleFont = value; if(_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private Color _CircleColor = Color.Black;
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Color")]
|
|
public Color CircleColor
|
|
{
|
|
get { return _CircleColor; }
|
|
set { _CircleColor = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CircleDiameter = 25;
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Diameter")]
|
|
public int CircleDiameter
|
|
{
|
|
get { return _CircleDiameter; }
|
|
set { _CircleDiameter = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CircleWeight = 2;
|
|
[Category("Circle")]
|
|
[DisplayName("Circle Pen Weight")]
|
|
public int CircleWeight
|
|
{
|
|
get { return _CircleWeight; }
|
|
set { _CircleWeight = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private float _NumberLocationX = 20F;
|
|
[Category("Number")]
|
|
[DisplayName("Number Location X")]
|
|
public float NumberLocationX
|
|
{
|
|
get { return _NumberLocationX; }
|
|
set { _NumberLocationX = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private float _NumberLocationY = 4;
|
|
[Category("Number")]
|
|
[DisplayName("Number Location Y")]
|
|
public float NumberLocationY
|
|
{
|
|
get { return _NumberLocationY; }
|
|
set { _NumberLocationY = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private SizeF _NumberSize = new SizeF(200F, 23F);
|
|
[Category("Number")]
|
|
[DisplayName("Number Size")]
|
|
public SizeF NumberSize
|
|
{
|
|
get { return _NumberSize; }
|
|
set { _NumberSize = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _TableWidthAdjust = 4;
|
|
[Category("Table")]
|
|
[DisplayName("Table Width Adjust")]
|
|
public int TableWidthAdjust
|
|
{
|
|
get { return _TableWidthAdjust; }
|
|
set { _TableWidthAdjust = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CheckOffWeight = 1;
|
|
[Category("CheckOff")]
|
|
[DisplayName("CheckOff Pen Weight")]
|
|
public int CheckOffWeight
|
|
{
|
|
get { return _CheckOffWeight; }
|
|
set { _CheckOffWeight = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private Color _CheckOffColor = Color.Black;
|
|
[Category("CheckOff")]
|
|
[DisplayName("CheckOff Color")]
|
|
public Color CheckOffColor
|
|
{
|
|
get { return _CheckOffColor; }
|
|
set { _CheckOffColor = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CheckOffSize =12;
|
|
[Category("CheckOff")]
|
|
[DisplayName("CheckOff Size")]
|
|
public int CheckOffSize
|
|
{
|
|
get { return _CheckOffSize; }
|
|
set { _CheckOffSize = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CheckOffX =0;
|
|
[Category("CheckOff")]
|
|
[DisplayName("CheckOff X")]
|
|
public int CheckOffX
|
|
{
|
|
get { return _CheckOffX; }
|
|
set { _CheckOffX = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private int _CheckOffY =5;
|
|
[Category("CheckOff")]
|
|
[DisplayName("CheckOff Y")]
|
|
public int CheckOffY
|
|
{
|
|
get { return _CheckOffY; }
|
|
set { _CheckOffY = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
|
|
private int _ChangeBarWeight = 1;
|
|
[Category("ChangeBar")]
|
|
[DisplayName("ChangeBar Pen Weight")]
|
|
public int ChangeBarWeight
|
|
{
|
|
get { return _ChangeBarWeight; }
|
|
set { _ChangeBarWeight = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
private Color _ChangeBarColor = Color.Black;
|
|
[Category("ChangeBar")]
|
|
[DisplayName("ChangeBar Color")]
|
|
public Color ChangeBarColor
|
|
{
|
|
get { return _ChangeBarColor; }
|
|
set { _ChangeBarColor = value; if (_MyStepPanel != null) _MyStepPanel.Refresh(); }
|
|
}
|
|
}
|
|
public partial class StepPanelEventArgs
|
|
{
|
|
private StepItem _MyStepItem;
|
|
public StepItem MyStepItem
|
|
{
|
|
get { return _MyStepItem; }
|
|
set { _MyStepItem = value; }
|
|
}
|
|
private MouseEventArgs _MyMouseEventArgs;
|
|
public MouseEventArgs MyMouseEventArgs
|
|
{
|
|
get { return _MyMouseEventArgs; }
|
|
set { _MyMouseEventArgs = value; }
|
|
}
|
|
|
|
public StepPanelEventArgs(StepItem myStepItem, MouseEventArgs myMouseEventArgs)
|
|
{
|
|
_MyStepItem = myStepItem;
|
|
_MyMouseEventArgs = myMouseEventArgs;
|
|
}
|
|
}
|
|
public partial class ItemSelectedChangedEventArgs
|
|
{
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set { _MyItemInfo = value; }
|
|
}
|
|
private StepItem _MyStepItem = null;
|
|
public StepItem MyStepItem
|
|
{
|
|
get { return _MyStepItem; }
|
|
set { _MyStepItem = value; }
|
|
}
|
|
public ItemSelectedChangedEventArgs(ItemInfo myItemInfo)
|
|
{
|
|
_MyItemInfo = myItemInfo;
|
|
}
|
|
public ItemSelectedChangedEventArgs(StepItem myStepItem)
|
|
{
|
|
_MyItemInfo = myStepItem.MyItemInfo;
|
|
_MyStepItem = myStepItem;
|
|
}
|
|
}
|
|
public partial class StepPanelAttachmentEventArgs
|
|
{
|
|
private StepItem _MyStepItem;
|
|
public StepItem MyStepItem
|
|
{
|
|
get { return _MyStepItem; }
|
|
set { _MyStepItem = value; }
|
|
}
|
|
public StepPanelAttachmentEventArgs(StepItem myStepItem)
|
|
{
|
|
_MyStepItem = myStepItem;
|
|
}
|
|
}
|
|
public partial class StepPanelLinkEventArgs : EventArgs
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private StepItem _LinkedStepItem;
|
|
public StepItem LinkedStepItem
|
|
{
|
|
get { return _LinkedStepItem; }
|
|
//set { _LinkedStepItem = value; }
|
|
}
|
|
private string _LinkInfoText;
|
|
public string LinkInfoText
|
|
{
|
|
get { return _LinkInfoText; }
|
|
}
|
|
private LinkText _MyLinkText;
|
|
public LinkText MyLinkText
|
|
{
|
|
get { return _MyLinkText; }
|
|
}
|
|
public StepPanelLinkEventArgs(StepItem linkedStepItem, string linkInfoText)
|
|
{
|
|
_LinkedStepItem = linkedStepItem;
|
|
_LinkInfoText = linkInfoText;
|
|
_MyLinkText = new LinkText(_LinkInfoText);
|
|
//if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("\r\n LinkInfo '{0}'\r\n", linkInfo.LinkText);
|
|
}
|
|
}
|
|
public delegate void StepPanelEvent(object sender, StepPanelEventArgs args);
|
|
public delegate void ItemSelectedChangedEvent(object sender, ItemSelectedChangedEventArgs args);
|
|
public delegate void StepPanelLinkEvent(object sender, StepPanelLinkEventArgs args);
|
|
public delegate void StepPanelAttachmentEvent(object sender, StepPanelAttachmentEventArgs args);
|
|
public delegate void StepRTBLinkEvent(object sender, StepPanelLinkEventArgs args);
|
|
}
|