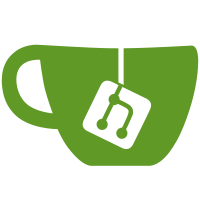
Load all at once code to support print. Open Command from the Start (PROMS2010) Menu. Enter key on treeview executes Open. The Treeview was retaining focus after opening procedures or accessory documents. This was fixed. Tab Bar initialized to the full width. Items were not always being displayed after the tree was clicked. The RO and Transition Info tabs are made invisible for accessory documents. The Tags, RO and Transition tabs are made invisible when no doucment is selected. Enter the DSO Framer window when it is created. Enter the Accessory page editor or step editor when a tab is selected. Remember the last selection in a step editor page when you return. Activate one of the remaining tabs when a tab is closed. Embed the pushpin images.
197 lines
6.2 KiB
C#
197 lines
6.2 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayTabItem : DevComponents.DotNetBar.DockContainerItem
|
|
{
|
|
#region Private Fields
|
|
private DisplayTabControl _MyDisplayTabControl;
|
|
private ItemInfo _MyItemInfo;
|
|
private StepTabPanel _MyStepTabPanel;
|
|
private string _MyKey;
|
|
private DSOTabPanel _MyDSOTabPanel;
|
|
#endregion
|
|
#region Properties
|
|
/// <summary>
|
|
/// ItemInfo associated with this DisplayTabItem
|
|
/// </summary>
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
//set { _MyItemInfo = value; }
|
|
}
|
|
/// <summary>
|
|
/// get Key Either:
|
|
/// "Item - " + Procedure ItemID for step pages
|
|
/// "Doc - " + DocumentID for Word Documents
|
|
/// </summary>
|
|
public string MyKey
|
|
{
|
|
get { return _MyKey; }
|
|
}
|
|
/// <summary>
|
|
/// Related StepTabPanel for a Step page
|
|
/// </summary>
|
|
public StepTabPanel MyStepTabPanel
|
|
{
|
|
get { return _MyStepTabPanel; }
|
|
set { _MyStepTabPanel = value; }
|
|
}
|
|
/// <summary>
|
|
/// Related DSOTabPanle for a Word page
|
|
/// </summary>
|
|
public DSOTabPanel MyDSOTabPanel
|
|
{
|
|
get { return _MyDSOTabPanel; }
|
|
set { _MyDSOTabPanel = value; }
|
|
}
|
|
/// <summary>
|
|
/// Current SelectedItemInfo for this page
|
|
/// </summary>
|
|
public ItemInfo SelectedItemInfo
|
|
{
|
|
get { return _MyStepTabPanel.SelectedItemInfo; }
|
|
set { _MyStepTabPanel.SelectedItemInfo = value; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public DisplayTabItem(IContainer container, DisplayTabControl myDisplayTabControl, ItemInfo myItemInfo, string myKey)
|
|
{
|
|
_MyKey = myKey;
|
|
_MyDisplayTabControl = myDisplayTabControl;
|
|
_MyItemInfo = myItemInfo;
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.Click += new EventHandler(DisplayTabItem_Click);
|
|
//this.GotFocus += new EventHandler(DisplayTabItem_GotFocus);
|
|
//this.LostFocus += new EventHandler(DisplayTabItem_LostFocus);
|
|
//this.MouseDown += new System.Windows.Forms.MouseEventHandler(DisplayTabItem_MouseDown);
|
|
//this.MouseUp += new System.Windows.Forms.MouseEventHandler(DisplayTabItem_MouseUp);
|
|
if (myItemInfo.MyContent.MyEntry == null)
|
|
SetupStepTabPanel();
|
|
else
|
|
SetupDSOTabPanel();
|
|
Name = string.Format("DisplayTabItem {0}", myItemInfo.ItemID);
|
|
}
|
|
protected override void OnDisplayedChanged()
|
|
{
|
|
//Console.WriteLine("=>=>=>=> OnDisplayedChanged");
|
|
if(_MyStepTabPanel != null)
|
|
_MyStepTabPanel.MyStepPanel.DisplayItemChanging = true;
|
|
base.OnDisplayedChanged();
|
|
if(_MyStepTabPanel != null)
|
|
_MyStepTabPanel.MyStepPanel.DisplayItemChanging = false;
|
|
//Console.WriteLine("<=<=<=<= OnDisplayedChanged");
|
|
}
|
|
|
|
//void DisplayTabItem_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_MouseUp");
|
|
//}
|
|
|
|
//void DisplayTabItem_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_MouseDown");
|
|
//}
|
|
|
|
//void DisplayTabItem_LostFocus(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_LostFocus");
|
|
//}
|
|
|
|
//void DisplayTabItem_GotFocus(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_GotFocus");
|
|
//}
|
|
#endregion
|
|
#region Event Handlers
|
|
/// <summary>
|
|
/// Updates SelectedStepItem when the user selects a DisplayTabItem
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void DisplayTabItem_Click(object sender, EventArgs e)
|
|
{
|
|
// Tell the TabControl that the ItemSelected has changed
|
|
DisplayTabItem myTabItem = sender as DisplayTabItem;
|
|
if(myTabItem == null)return;
|
|
StepTabPanel myTabPanel = myTabItem.MyStepTabPanel as StepTabPanel;
|
|
if(myTabPanel == null) return;
|
|
if (MyStepTabPanel.SelectedStepItem == null) return;
|
|
_MyDisplayTabControl.OnItemSelectedChanged(this,new ItemSelectedChangedEventArgs(MyStepTabPanel.SelectedStepItem));
|
|
}
|
|
#endregion
|
|
#region private Methods
|
|
/// <summary>
|
|
/// Creates and sets-up a StepTabPanel
|
|
/// </summary>
|
|
private void SetupStepTabPanel()
|
|
{
|
|
((System.ComponentModel.ISupportInitialize)(_MyDisplayTabControl.MyBar)).BeginInit();
|
|
_MyDisplayTabControl.MyBar.SuspendLayout();
|
|
_MyStepTabPanel = new StepTabPanel(_MyDisplayTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyStepTabPanel;
|
|
Name = "tabItem Item " + _MyItemInfo.ItemID;
|
|
Text = _MyItemInfo.TabTitle;
|
|
Tooltip = _MyItemInfo.TabToolTip;
|
|
//
|
|
_MyDisplayTabControl.Controls.Add(_MyStepTabPanel);
|
|
_MyDisplayTabControl.MyBar.Items.Add(this);
|
|
_MyDisplayTabControl.MyBar.Width = 300; // This triggers the bar to resize itself
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyStepTabPanel.MyDisplayTabItem = this;
|
|
((System.ComponentModel.ISupportInitialize)(_MyDisplayTabControl.MyBar)).EndInit();
|
|
_MyDisplayTabControl.MyBar.ResumeLayout(false);
|
|
}
|
|
/// <summary>
|
|
/// Creates and sets-up a DSOTabPanel
|
|
/// </summary>
|
|
private void SetupDSOTabPanel()
|
|
{
|
|
EntryInfo myEntry = _MyItemInfo.MyContent.MyEntry;
|
|
_MyDSOTabPanel = new DSOTabPanel(myEntry.MyDocument, _MyDisplayTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyDSOTabPanel;
|
|
Name = "tabItem Item " + _MyItemInfo.ItemID;
|
|
Text = _MyItemInfo.TabTitle;
|
|
Tooltip = _MyItemInfo.TabToolTip;
|
|
//
|
|
//Console.WriteLine("this.Focus {0}", Name);
|
|
//this.Focus();
|
|
//Console.WriteLine("Controls.Add {0}", Name);
|
|
_MyDisplayTabControl.Controls.Add(_MyDSOTabPanel);
|
|
//Console.WriteLine("Enabled = false {0}", Name);
|
|
//_MyDisplayTabControl.MyBar.Enabled = false;
|
|
DSOTabPanel.IgnoreEnter = true;
|
|
Console.WriteLine("AddRange {0}", Name);
|
|
_MyDisplayTabControl.MyBar.Items.AddRange(new DevComponents.DotNetBar.BaseItem[] {
|
|
this});
|
|
//
|
|
// tabPanel
|
|
//
|
|
//Console.WriteLine("Enabled = true {0}", Name);
|
|
//_MyDisplayTabControl.MyBar.Enabled = true;
|
|
//Console.WriteLine("SelectedDisplayTabItem {0}", Name);
|
|
_MyDisplayTabControl.SelectedDisplayTabItem = this;
|
|
//Console.WriteLine("MyDisplayTabItem {0}", Name);
|
|
_MyDSOTabPanel.MyDisplayTabItem = this;
|
|
DSOTabPanel.IgnoreEnter = false;
|
|
//_MyDisplayTabControl.OnItemSelectedChanged(this, new ItemSelectedChangedEventArgs(MyItemInfo));
|
|
}
|
|
#endregion
|
|
}
|
|
}
|