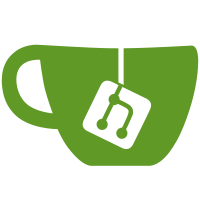
Load all at once code to support print. Open Command from the Start (PROMS2010) Menu. Enter key on treeview executes Open. The Treeview was retaining focus after opening procedures or accessory documents. This was fixed. Tab Bar initialized to the full width. Items were not always being displayed after the tree was clicked. The RO and Transition Info tabs are made invisible for accessory documents. The Tags, RO and Transition tabs are made invisible when no doucment is selected. Enter the DSO Framer window when it is created. Enter the Accessory page editor or step editor when a tab is selected. Remember the last selection in a step editor page when you return. Activate one of the remaining tabs when a tab is closed. Embed the pushpin images.
330 lines
11 KiB
C#
330 lines
11 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Controls.Library;
|
|
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DSOTabPanel : DevComponents.DotNetBar.PanelDockContainer
|
|
{
|
|
#region Private Fields
|
|
private DisplayTabControl _MyDisplayTabControl;
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private AxDSOFramer.AxFramerControl _MyDSOFramer;
|
|
private TransparentPanel _MyTransparentPanel;
|
|
private static int _Count = 0;
|
|
private DocumentInfo _MyDocumentInfo;
|
|
private int _MyCount;
|
|
private DisplayTabItem _MyDisplayTabItem;
|
|
private DSOFile _DSOFile;
|
|
#endregion
|
|
#region Public Properties
|
|
/// <summary>
|
|
/// Count of DSO Pages open. Limited to 18 in DisplayTabControl
|
|
/// </summary>
|
|
public static int Count
|
|
{
|
|
get { return _Count; }
|
|
set { _Count = value; }
|
|
}
|
|
/// <summary>
|
|
/// Pointer to the related DisplayTabItem
|
|
/// </summary>
|
|
public DisplayTabItem MyDisplayTabItem
|
|
{
|
|
get { return _MyDisplayTabItem; }
|
|
set { _MyDisplayTabItem = value; }
|
|
}
|
|
/// <summary>
|
|
/// DocumentInfo record for the Word document
|
|
/// </summary>
|
|
public DocumentInfo MyDocumentInfo
|
|
{
|
|
get { return _MyDocumentInfo; }
|
|
}
|
|
/// <summary>
|
|
/// Temporary Word file used for editing.
|
|
/// </summary>
|
|
internal DSOFile MyDSOFile
|
|
{
|
|
get
|
|
{
|
|
if (_DSOFile == null)
|
|
_DSOFile = new DSOFile(_MyDocumentInfo);
|
|
return _DSOFile;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Dirty status. Only saved if dirty.
|
|
/// </summary>
|
|
public bool IsDirty
|
|
{
|
|
get { return _MyDSOFramer.IsDirty; }
|
|
}
|
|
#endregion
|
|
//private frmPG _frm = null;
|
|
#region Constructors
|
|
public DSOTabPanel(DocumentInfo documentInfo, DisplayTabControl myDisplayTabControl)
|
|
{
|
|
_MyDisplayTabControl = myDisplayTabControl;
|
|
InitializeComponent();
|
|
SetupDSOTabPanel();
|
|
_MyDocumentInfo = documentInfo;
|
|
SetupDSO();
|
|
//_frm = new frmPG(_MyDSOFramer);
|
|
//_frm.Show();
|
|
}
|
|
#endregion
|
|
#region Private Methods
|
|
private void SetupDSOTabPanel()
|
|
{
|
|
Dock = System.Windows.Forms.DockStyle.Fill; // Automatically Fill the panel
|
|
}
|
|
private void SetupDSO()
|
|
{
|
|
_Count++; // Increment the count of open Word documents (Limit in DisplayTabControl)
|
|
_MyCount = _Count;
|
|
this._MyTransparentPanel = new TransparentPanel();
|
|
this._MyDSOFramer = new AxDSOFramer.AxFramerControl();
|
|
((System.ComponentModel.ISupportInitialize)(this._MyDSOFramer)).BeginInit();
|
|
this.Controls.Add(this._MyDSOFramer);
|
|
this.Controls.Add(this._MyTransparentPanel); // A transparent panel is added over top of the DSO Framer window so that
|
|
// the related tab can be activated when the user clicks on a Word Document. Since the Word document is actually running
|
|
// in a different thread, it does not behave properly with focus events.
|
|
this.components.Add(this._MyDSOFramer);
|
|
this.components.Add(this._MyTransparentPanel);
|
|
this._MyTransparentPanel.Dock = System.Windows.Forms.DockStyle.Fill;
|
|
this._MyTransparentPanel.Font = new System.Drawing.Font("Tahoma", 14.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
|
|
this._MyTransparentPanel.ForeColor = System.Drawing.Color.Brown; // This is the color used to show InActive on the right side on the Word
|
|
// document menu line.
|
|
//this._MyTransPanel.Location = new System.Drawing.Point(0, 0);
|
|
//this._MyTransPanel.Name = "transPanel1";
|
|
//this._MyTransPanel.Size = new System.Drawing.Size(370, 423);
|
|
//this._MyTransPanel.TabIndex = 1;
|
|
this._MyTransparentPanel.Click += new EventHandler(_MyTransparentPanel_Click);
|
|
this._MyDSOFramer.Dock = System.Windows.Forms.DockStyle.Fill;
|
|
//System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(WordDSOTab));
|
|
//this._DSOFramer.OcxState = ((System.Windows.Forms.AxHost.State)(resources.GetObject("_FC.OcxState")));
|
|
((System.ComponentModel.ISupportInitialize)(this._MyDSOFramer)).EndInit();
|
|
this._MyDSOFramer.Open(MyDSOFile.MyFile.FullName);
|
|
this._MyDSOFramer.Menubar = false;
|
|
this._MyDSOFramer.Titlebar = false;
|
|
//if (_MyCount < 20)
|
|
// this._MyDSOFramer.FrameHookPolicy = DSOFramer.dsoFrameHookPolicy.dsoResetNow;
|
|
this._MyDSOFramer.BeforeDocumentClosed += new AxDSOFramer._DFramerCtlEvents_BeforeDocumentClosedEventHandler(_MyDSOFramer_BeforeDocumentClosed);
|
|
this._MyDSOFramer.OnSaveCompleted += new AxDSOFramer._DFramerCtlEvents_OnSaveCompletedEventHandler(_MyDSOFramer_OnSaveCompleted);
|
|
//this._MyDSOFramer.LostFocus += new EventHandler(_MyDSOFramer_LostFocus);
|
|
//this._MyDSOFramer.GotFocus += new EventHandler(_MyDSOFramer_GotFocus);
|
|
//this._MyDSOFramer.Enter += new EventHandler(_MyDSOFramer_Enter);
|
|
//this._MyDSOFramer.Leave += new EventHandler(_MyDSOFramer_Leave);
|
|
//this._MyDSOFramer.OnActivationChange += new AxDSOFramer._DFramerCtlEvents_OnActivationChangeEventHandler(_MyDSOFramer_OnActivationChange);
|
|
this.Enter += new EventHandler(DSOTabPanel_Enter);
|
|
this.Leave += new EventHandler(DSOTabPanel_Leave);
|
|
//this.GotFocus += new EventHandler(DSOTabPanel_GotFocus);
|
|
//this.LostFocus += new EventHandler(DSOTabPanel_LostFocus);
|
|
}
|
|
|
|
//void _MyDSOFramer_Leave(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSO Leave {0}", this.MyDocumentInfo.DocID);
|
|
//}
|
|
|
|
//void _MyDSOFramer_Enter(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSO Enter {0}", this.MyDocumentInfo.DocID);
|
|
//}
|
|
|
|
//void _MyDSOFramer_GotFocus(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSO Got Focus {0}",this.MyDocumentInfo.DocID);
|
|
//}
|
|
|
|
//void _MyDSOFramer_LostFocus(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSO Lost Focus {0}", this.MyDocumentInfo.DocID);
|
|
//}
|
|
public void EnterPanel()
|
|
{
|
|
DSOTabPanel_Enter(this, new EventArgs());
|
|
}
|
|
//void DSOTabPanel_LostFocus(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSOTabPanel_LostFocus {0} DocID {1} Index {2} {3}", _In_DSOTabPanel_Enter, this._MyDocumentInfo.DocID, _MyDisplayTabControl.MyBar.SelectedDockTab, sender.GetType().FullName);
|
|
//}
|
|
//void DSOTabPanel_GotFocus(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStack("DSOTabPanel_GotFocus {0} DocID {1} Index {2} {3}", _In_DSOTabPanel_Enter, this._MyDocumentInfo.DocID, _MyDisplayTabControl.MyBar.SelectedDockTab, sender.GetType().FullName);
|
|
//}
|
|
#endregion
|
|
#region Event Handlers
|
|
/// <summary>
|
|
/// When the user leaves a Word document, place the transparent frame on top with the words "InActive" in the upper right
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void DSOTabPanel_Leave(object sender, EventArgs e)
|
|
{
|
|
//vlnStackTrace.ShowStack("DSOTabPanel_Leave {0} DocID {1} Index {2} {3}", _In_DSOTabPanel_Enter, this._MyDocumentInfo.DocID, _MyDisplayTabControl.MyBar.SelectedDockTab, sender.GetType().FullName);
|
|
_MyTransparentPanel.BringToFront();
|
|
}
|
|
/// <summary>
|
|
/// Force this item to be selected when the transparent window is clicked.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void _MyTransparentPanel_Click(object sender, EventArgs e)
|
|
{
|
|
this.Select();
|
|
}
|
|
/// <summary>
|
|
/// If the user presses the save button, tell the file to save it's contents to the database
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void _MyDSOFramer_OnSaveCompleted(object sender, AxDSOFramer._DFramerCtlEvents_OnSaveCompletedEvent e)
|
|
{
|
|
MyDSOFile.SaveFile();
|
|
}
|
|
/// <summary>
|
|
/// Before a document closes check to see if it's contents should be saved.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void _MyDSOFramer_BeforeDocumentClosed(object sender, AxDSOFramer._DFramerCtlEvents_BeforeDocumentClosedEvent e)
|
|
{
|
|
try
|
|
{
|
|
this.Enter -= new EventHandler(DSOTabPanel_Enter);
|
|
this.Leave -= new EventHandler(DSOTabPanel_Leave);
|
|
SaveDirty();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
_MyLog.Warn("Before Closing Document ", ex);
|
|
}
|
|
}
|
|
public static bool IgnoreEnter = false;
|
|
private bool _In_DSOTabPanel_Enter=false;
|
|
/// <summary>
|
|
/// When a Word document is selected make sure it's tab is activated and
|
|
/// the SelectedItem for the DisplayTabControl is updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void DSOTabPanel_Enter(object sender, EventArgs e)
|
|
{
|
|
if (IgnoreEnter) return;
|
|
_MyTransparentPanel.SendToBack();
|
|
try
|
|
{
|
|
_MyDSOFramer.EventsEnabled = true;
|
|
_MyDSOFramer.FrameHookPolicy = DSOFramer.dsoFrameHookPolicy.dsoResetNow;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.ErrorFormat("DSOTabPage_Enter", ex);
|
|
}
|
|
if (_In_DSOTabPanel_Enter) return;
|
|
//vlnStackTrace.ShowStack("DSOTabPanel_Enter {0} DocID {1} Index {2} {3}",_In_DSOTabPanel_Enter, this._MyDocumentInfo.DocID, _MyDisplayTabControl.MyBar.SelectedDockTab, sender.GetType().FullName);
|
|
_In_DSOTabPanel_Enter = true;
|
|
_MyDisplayTabControl.OnItemSelectedChanged(this,new ItemSelectedChangedEventArgs(MyDisplayTabItem.MyItemInfo));
|
|
_MyDSOFramer.Focus();
|
|
_In_DSOTabPanel_Enter = false;
|
|
}
|
|
#endregion
|
|
#region Public Methods
|
|
/// <summary>
|
|
/// Save the contents of the Word Document to a file
|
|
/// and save the file to the database
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
public bool SaveDSO()
|
|
{
|
|
bool result = true;
|
|
try
|
|
{
|
|
_MyDSOFramer.Save();
|
|
MyDSOFile.SaveFile();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("SaveDSO", ex);
|
|
result = false;
|
|
}
|
|
return result;
|
|
}
|
|
/// <summary>
|
|
/// Check to see if a Word document should be saved. If it is dirty ask the user if the
|
|
/// changes should be changed. Save the changes if the user says "yes".
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
public bool SaveDirty()
|
|
{
|
|
if (_MyDSOFramer.IsDirty)
|
|
{
|
|
// TODO: Should be based upon Item rather than Document.
|
|
if (MessageBox.Show("Save changes to " + _MyDisplayTabItem.MyItemInfo.TabTitle + "\r\n" + _MyDisplayTabItem.MyItemInfo.TabToolTip, "Document has Changed", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
return SaveDSO();
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
/// <summary>
|
|
/// Cleans-up the DSO Framer window
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
public bool CloseDSO()
|
|
{
|
|
return CloseDSO(false);
|
|
}
|
|
/// <summary>
|
|
/// Cleans-up the DSO Framer window
|
|
/// </summary>
|
|
/// <param name="force"></param>
|
|
/// <returns></returns>
|
|
public bool CloseDSO(bool force)
|
|
{
|
|
_MyLog.Debug("CloseDSO");
|
|
bool result = true;
|
|
try
|
|
{
|
|
_MyDSOFramer.Close();
|
|
Controls.Remove(_MyDSOFramer);
|
|
components.Remove(_MyDSOFramer);
|
|
_MyDSOFramer.Dispose();
|
|
_MyDSOFramer = null;
|
|
_Count--;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("SaveDSO - " + this.Name, ex);
|
|
result = false;
|
|
}
|
|
return result;
|
|
}
|
|
/// <summary>
|
|
/// Activates the current DSO Framer window (Word)
|
|
/// </summary>
|
|
public void Activate()
|
|
{
|
|
try
|
|
{
|
|
this._MyDSOFramer.Activate();
|
|
if (_MyCount < 20)
|
|
this._MyDSOFramer.FrameHookPolicy = DSOFramer.dsoFrameHookPolicy.dsoResetNow;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Activate", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
}
|