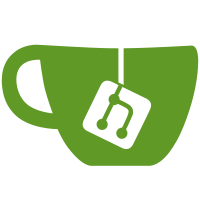
Load all at once code to support print. Open Command from the Start (PROMS2010) Menu. Enter key on treeview executes Open. The Treeview was retaining focus after opening procedures or accessory documents. This was fixed. Tab Bar initialized to the full width. Items were not always being displayed after the tree was clicked. The RO and Transition Info tabs are made invisible for accessory documents. The Tags, RO and Transition tabs are made invisible when no doucment is selected. Enter the DSO Framer window when it is created. Enter the Accessory page editor or step editor when a tab is selected. Remember the last selection in a step editor page when you return. Activate one of the remaining tabs when a tab is closed. Embed the pushpin images.
1201 lines
29 KiB
C#
1201 lines
29 KiB
C#
#define ItemWithContent
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using System.Xml;
|
|
using System.Drawing;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
#region Item
|
|
public partial class Item:IVEDrillDown
|
|
{
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0} {1}", MyContent.Number, MyContent.Text).Trim();
|
|
}
|
|
// TODO: Move to ItemInfo Extension
|
|
|
|
#region IVEDrillDown
|
|
public System.Collections.IList GetChildren()
|
|
{
|
|
return this.MyContent.ContentParts;
|
|
}
|
|
public bool HasChildren
|
|
{
|
|
get { return this.MyContent.ContentPartCount > 0; }
|
|
}
|
|
public Item MyProcedure
|
|
{
|
|
get
|
|
{
|
|
// Walk up active parents until the parent is not an item
|
|
Item tmp = this;
|
|
while (tmp.ActiveParent.GetType() != typeof(DocVersion)) tmp = (Item)tmp.ActiveParent;
|
|
return tmp;
|
|
}
|
|
} private IVEDrillDown _ActiveParent = null;
|
|
public IVEDrillDown ActiveParent
|
|
{
|
|
get
|
|
{
|
|
if (_ActiveParent == null)
|
|
{
|
|
if (MyPrevious != null)
|
|
_ActiveParent = _MyPrevious.ActiveParent;
|
|
else
|
|
{
|
|
if (ItemDocVersionCount > 0)
|
|
_ActiveParent = this.ItemDocVersions[0].MyDocVersion;
|
|
else
|
|
{
|
|
if (this.ItemParts == null || this.ItemPartCount == 0)
|
|
_ActiveParent = this;
|
|
else
|
|
_ActiveParent = this.ItemParts[0].MyContent.ContentItems[0].MyItem;
|
|
}
|
|
}
|
|
}
|
|
return _ActiveParent==this ? null : _ActiveParent;
|
|
}
|
|
}
|
|
private Format _ActiveFormat=null;// Added to cache ActiveFormat
|
|
public Format ActiveFormat
|
|
{
|
|
get
|
|
{
|
|
if(_ActiveFormat == null)
|
|
_ActiveFormat = (LocalFormat != null ? LocalFormat : ActiveParent.ActiveFormat);
|
|
return _ActiveFormat;
|
|
}
|
|
}
|
|
public Format LocalFormat
|
|
{
|
|
get { return MyContent.MyFormat; }
|
|
}
|
|
public DynamicTypeDescriptor MyConfig
|
|
{
|
|
get { return null; }
|
|
}
|
|
#endregion
|
|
}
|
|
#endregion
|
|
#region ItemInfo
|
|
public partial class ItemInfo:IVEDrillDownReadOnly
|
|
{
|
|
#region LoadAtOnce2
|
|
public static ItemInfo GetItemAndChildren2(int? itemID)
|
|
{
|
|
try
|
|
{
|
|
ItemInfo tmp = DataPortal.Fetch<ItemInfo>(new ItemAndChildrenCriteria2(itemID));
|
|
//_AllList.Add(tmp);
|
|
if (tmp.ErrorMessage == "No Record Found") tmp = null;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ItemInfoList.GetItemAndChildren2", ex);
|
|
}
|
|
}
|
|
// Criteria to get Item and children
|
|
[Serializable()]
|
|
private class ItemAndChildrenCriteria2
|
|
{
|
|
public ItemAndChildrenCriteria2(int? itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int? _ItemID;
|
|
public int? ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
// Data Portal to Get Item and Children
|
|
private void DataPortal_Fetch(ItemAndChildrenCriteria2 criteria)
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getItem";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
SpinThroughChildren();
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Database.LogException("ItemInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("ItemInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
_AllList.Remove(this);
|
|
}
|
|
private void SpinThroughChildren()
|
|
{
|
|
if(MyContent.ContentPartCount > 0)
|
|
foreach(PartInfo partInfo in MyContent.ContentParts)
|
|
foreach (ItemInfo itemInfo in partInfo.MyItems)
|
|
itemInfo.SpinThroughChildren();
|
|
}
|
|
#endregion
|
|
#region LoadAtOnce
|
|
// Method to Get Item and children
|
|
public static ItemInfo GetItemAndChildren(int? itemID,int? parentID)
|
|
{
|
|
try
|
|
{
|
|
ItemInfo tmp = DataPortal.Fetch<ItemInfo>(new ItemAndChildrenCriteria(itemID,parentID));
|
|
//_AllList.Add(tmp);
|
|
if (tmp.ErrorMessage == "No Record Found") tmp = null;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ItemInfoList.GetChildren", ex);
|
|
}
|
|
}
|
|
// Criteria to get Item and children
|
|
[Serializable()]
|
|
private class ItemAndChildrenCriteria
|
|
{
|
|
public ItemAndChildrenCriteria(int? itemID, int? parentID)
|
|
{
|
|
_ItemID = itemID;
|
|
_ParentID = parentID;
|
|
}
|
|
private int? _ItemID;
|
|
public int? ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
private int? _ParentID;
|
|
public int? ParentID
|
|
{
|
|
get { return _ParentID; }
|
|
set { _ParentID = value; }
|
|
}
|
|
}
|
|
// Data Portal to Get Item and Children
|
|
private void DataPortal_Fetch(ItemAndChildrenCriteria criteria)
|
|
{
|
|
//ItemInfo tmp = null;
|
|
Dictionary<int, ItemInfo> lookup = new Dictionary<int, ItemInfo>(); ;
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "vesp_ListItemAndChildren";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
cm.Parameters.AddWithValue("@ParentID", criteria.ParentID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
while (dr.Read())
|
|
{
|
|
if (dr.GetInt32("Level")==0)
|
|
{
|
|
//tmp = itemInfo;
|
|
ReadData(dr);
|
|
AddContent(dr);
|
|
lookup[this.ItemID] = this;
|
|
}
|
|
else
|
|
{
|
|
ItemInfo itemInfo = null;
|
|
int itemType = dr.GetInt32("Type") / 10000;
|
|
switch (itemType)
|
|
{
|
|
case 0:
|
|
itemInfo = new ProcedureInfo(dr);
|
|
break;
|
|
case 1:
|
|
itemInfo = new SectionInfo(dr);
|
|
break;
|
|
case 2:
|
|
itemInfo = new StepInfo(dr);
|
|
break;
|
|
}
|
|
// Load Children
|
|
itemInfo.AddContent(dr);
|
|
ItemInfo parent = lookup[dr.GetInt32("ParentID")];
|
|
itemInfo._ActiveParent = parent;
|
|
itemInfo._ActiveSection = (itemInfo.IsSection ? itemInfo : parent._ActiveSection);
|
|
parent.AddPart(dr, itemInfo);
|
|
lookup.Add(itemInfo.ItemID, itemInfo);
|
|
}
|
|
}
|
|
//Console.WriteLine("I'm here {0}",this.MyContent.ContentPartCount);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Database.LogException("ItemInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("ItemInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
private void AddPart(SafeDataReader dr, ItemInfo itemInfo)
|
|
{
|
|
// Either a new PartInfo or an existing PartInfo
|
|
if (dr.IsDBNull(dr.GetOrdinal("PreviousID")))
|
|
{
|
|
//PartInfo prt = new PartInfo(dr, itemInfo);
|
|
_MyContent.AddPart(dr, itemInfo);
|
|
}
|
|
else
|
|
{
|
|
foreach (PartInfo pi in MyContent.ContentParts)
|
|
{
|
|
if (dr.GetInt32("FromType") == (int)pi.PartType)
|
|
{
|
|
if (pi._MyItems == null)
|
|
pi._MyItems = new ItemInfoList(itemInfo);
|
|
else
|
|
pi.MyItems.AddItem(itemInfo);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
public bool IsType(string type)
|
|
{
|
|
int stepType = ((int)MyContent.Type) % 10000;
|
|
StepDataList sdlist = ActiveFormat.PlantFormat.FormatData.StepDataList;
|
|
StepData sd = sdlist[stepType];
|
|
// TODO: RHM20071115 while (sd.Index != 0)
|
|
// TODO: RHM20071115 {
|
|
// TODO: RHM20071115 if (sd.Type == type) return true;
|
|
// TODO: RHM20071115 sd = sdlist[sd.ParentType];
|
|
// TODO: RHM20071115 }
|
|
return false;
|
|
}
|
|
public bool IsCaution
|
|
{
|
|
get
|
|
{
|
|
return IsType("CAUTION");
|
|
}
|
|
}
|
|
public bool IsNote
|
|
{
|
|
get
|
|
{
|
|
return IsType("NOTE");
|
|
}
|
|
}
|
|
public bool IsTable
|
|
{
|
|
get
|
|
{
|
|
return IsType("TABLE");
|
|
}
|
|
}
|
|
public bool IsFigure
|
|
{
|
|
get
|
|
{
|
|
return IsType("FIGURE");
|
|
}
|
|
}
|
|
public bool IsOr
|
|
{
|
|
get
|
|
{
|
|
return IsType("OR");
|
|
}
|
|
}
|
|
public bool IsAnd
|
|
{
|
|
get
|
|
{
|
|
return IsType("AND");
|
|
}
|
|
}
|
|
public bool IsEquipmentList
|
|
{
|
|
get
|
|
{
|
|
return IsType("EQUIPMENTLIST");
|
|
}
|
|
}
|
|
public bool IsTitle
|
|
{
|
|
get
|
|
{
|
|
return IsType("TITLE");
|
|
}
|
|
}
|
|
public bool IsAccPages
|
|
{
|
|
get
|
|
{
|
|
return IsType("ACCPAGES");
|
|
}
|
|
}
|
|
public bool IsParagraph
|
|
{
|
|
get
|
|
{
|
|
return IsType("PARAGRAPH");
|
|
}
|
|
}
|
|
public bool IsDefault
|
|
{
|
|
get
|
|
{
|
|
return IsType("DEFAULT");
|
|
}
|
|
}
|
|
public bool IsContAcSequential
|
|
{
|
|
get
|
|
{
|
|
return IsType("CONTACSEQUENTIAL");
|
|
}
|
|
}
|
|
public bool IsHigh
|
|
{
|
|
get
|
|
{
|
|
// check to see if ActiveParent is a section, if so it is a high level step
|
|
if (MyContent.Type / 10000 != 2) return false;
|
|
ItemInfo parent = ActiveParent as ItemInfo;
|
|
if (parent == null) return false;
|
|
if ((parent.MyContent.Type / 10000) == 1)
|
|
return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsSequential
|
|
{
|
|
get
|
|
{
|
|
if (((MyContent.Type / 10000) == 2) && ((((int)MyContent.Type) % 10000) == 0)) return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsRNO
|
|
{
|
|
get
|
|
{
|
|
return ((ItemPartCount > 0) && (ItemParts[0].PartType == E_FromType.RNO));
|
|
}
|
|
}
|
|
public bool IsInRNO
|
|
{
|
|
get
|
|
{
|
|
if (IsHigh) return false;
|
|
if (IsRNO) return true;
|
|
ItemInfo parent = ActiveParent as ItemInfo;
|
|
if (parent == null) return false;
|
|
return parent.IsInRNO;
|
|
}
|
|
}
|
|
public ItemInfo FirstSibling
|
|
{
|
|
get
|
|
{
|
|
ItemInfo temp = this;
|
|
while (temp.MyPrevious != null) temp = temp.MyPrevious;
|
|
return temp;
|
|
}
|
|
}
|
|
public bool IsSubStep
|
|
{
|
|
get
|
|
{
|
|
ItemInfo temp = FirstSibling;
|
|
return ((temp.ItemPartCount > 0) && (temp.ItemParts[0].PartType == E_FromType.Step));
|
|
}
|
|
}
|
|
public bool IsInSubStep
|
|
{
|
|
get
|
|
{
|
|
if (IsHigh) return false;
|
|
if (IsSubStep) return true;
|
|
ItemInfo parent = ActiveParent as ItemInfo;
|
|
if (parent == null) return false;
|
|
return parent.IsInSubStep;
|
|
}
|
|
}
|
|
public bool IsInFirstLevelSubStep
|
|
{
|
|
get
|
|
{
|
|
ItemInfo temp = FirstSibling;
|
|
if(temp.ActiveParent.GetType() == typeof(VEPROMS.CSLA.Library.DocVersionInfo))return false;
|
|
while (((ItemInfo)temp.ActiveParent).IsHigh == false) temp = ((ItemInfo)temp.ActiveParent).FirstSibling;
|
|
return temp.IsSubStep;
|
|
}
|
|
}
|
|
public bool IsStepSection
|
|
{
|
|
get
|
|
{
|
|
if (MyContent.Type == 10000) return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsSection
|
|
{
|
|
get
|
|
{
|
|
if ((MyContent.Type / 10000) == 1) return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsDefaultSection
|
|
{
|
|
get
|
|
{
|
|
// check to see if ActiveParent is a section, if so it is a high level step
|
|
if (MyContent.Type / 10000 != 1) return false;
|
|
// get procedure section and then
|
|
ItemInfo parent = (ItemInfo)ActiveParent;
|
|
if (!parent.IsProcedure) return false;
|
|
ProcedureConfig pc = (ProcedureConfig)parent.MyConfig;
|
|
int sectstartid = -1;
|
|
string ss = pc == null ? null : pc.SectionStart;
|
|
if (ss != null) sectstartid = System.Convert.ToInt32(ss);
|
|
else return false;
|
|
if (ItemID == sectstartid) return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsProcedure
|
|
{
|
|
get
|
|
{
|
|
if ((MyContent.Type / 10000) == 0) return true;
|
|
return false;
|
|
}
|
|
}
|
|
public bool IsStep
|
|
{
|
|
get
|
|
{
|
|
if ((MyContent.Type / 10000) == 2) return true;
|
|
return false;
|
|
}
|
|
}
|
|
private E_FromType ItemType
|
|
{
|
|
get
|
|
{
|
|
if (MyContent.Type == 0) return E_FromType.Procedure;
|
|
if (MyContent.Type < 20000) return E_FromType.Section;
|
|
return E_FromType.Step;
|
|
}
|
|
}
|
|
public Item GetByType()
|
|
{
|
|
Item tmp = null;
|
|
switch (ItemType)
|
|
{
|
|
case E_FromType.Procedure:
|
|
tmp = Procedure.Get(_ItemID);
|
|
break;
|
|
//case E_FromType.Section:
|
|
// itemInfo = new Section(dr);
|
|
// break;
|
|
//default:
|
|
// itemInfo = new Step(dr);
|
|
// break;
|
|
}
|
|
return tmp;
|
|
}
|
|
private int? _Ordinal;
|
|
public int Ordinal
|
|
{
|
|
get
|
|
{
|
|
if (_Ordinal == null)
|
|
{
|
|
if (MyPrevious != null)
|
|
_Ordinal = MyPrevious.Ordinal + 1;
|
|
else
|
|
_Ordinal = 1;
|
|
}
|
|
return (int) _Ordinal;
|
|
}
|
|
}
|
|
public string CslaType
|
|
{ get { return this.GetType().FullName; } }
|
|
public override string ToString()
|
|
{
|
|
//Item editable = Item.GetExistingByPrimaryKey(_ItemID);
|
|
//return string.Format("{0}{1} {2}", (editable == null ? "" : (editable.IsDirty ? "* " : "")),
|
|
// (MyContent.Type >= 20000 ? Ordinal.ToString() + "." : MyContent.Number), MyContent.Text);
|
|
ContentInfo cont = MyContent;
|
|
string number = cont.Number;
|
|
if (cont.Type >= 20000) number = Ordinal.ToString() + ".";
|
|
return string.Format("{0} {1}", number, cont.Text).Trim();
|
|
//return string.Format("{0} {1}", cont.Number, cont.Text);
|
|
//return "Now is the time for all good men to come to the aid of their country!";
|
|
}
|
|
//public string ToString(string str,System.IFormatProvider ifp)
|
|
//{
|
|
// return ToString();
|
|
//}
|
|
public string nz(string str, string def)
|
|
{
|
|
return (str == null ? def : str);
|
|
}
|
|
public string Path
|
|
{
|
|
get
|
|
{
|
|
string number = (MyContent.Type >= 20000 ? Ordinal.ToString() + "." : (nz(MyContent.Number,"")==""? MyContent.Text: MyContent.Number));
|
|
ItemInfo parent = this;
|
|
while (parent.MyPrevious != null) parent = parent.MyPrevious;
|
|
if (parent.ItemPartCount == 0)
|
|
return number + ", " + MyContent.Text;
|
|
else
|
|
{
|
|
PartInfo partInfo = parent.ItemParts[0];
|
|
return partInfo.MyContent.ContentItems.Items[0].Path + " " + ((E_FromType)partInfo.FromType).ToString() + " " + number;
|
|
}
|
|
}
|
|
}
|
|
public ContentInfo ParentContent
|
|
{
|
|
get
|
|
{
|
|
string number = (MyContent.Type >= 20000 ? Ordinal.ToString() + "." : (nz(MyContent.Number, "") == "" ? MyContent.Text : MyContent.Number));
|
|
ItemInfo parent = this;
|
|
while (parent.MyPrevious != null) parent = parent.MyPrevious;
|
|
if (parent.ItemPartCount == 0)
|
|
return null;
|
|
else
|
|
{
|
|
return parent.ItemParts[0].MyContent;
|
|
}
|
|
}
|
|
}
|
|
public ItemInfo MyParent
|
|
{
|
|
get
|
|
{
|
|
//if (ItemDocVersionCount > 0) return ItemDocVersions[0]; Need to create one interface to support Folders, DocVersions and Items
|
|
ContentInfo parentContent = ParentContent;
|
|
if (parentContent == null || parentContent.ContentItemCount == 0) return null;
|
|
return parentContent.ContentItems[0];
|
|
}
|
|
}
|
|
private ItemInfoList Lookup(int fromType, ref ItemInfoList itemInfoList)
|
|
{
|
|
if (itemInfoList != null) return itemInfoList;
|
|
if (MyContent.ContentPartCount != 0)
|
|
foreach (PartInfo partInfo in MyContent.ContentParts)
|
|
if (partInfo.FromType == fromType)
|
|
{
|
|
itemInfoList = partInfo._MyItems = ItemInfoList.GetList(partInfo.ItemID,partInfo.FromType);
|
|
return itemInfoList;
|
|
}
|
|
return null;
|
|
}
|
|
private ItemInfoList _Procedures;
|
|
public ItemInfoList Procedures
|
|
{ get { return Lookup(1,ref _Procedures); } }
|
|
private ItemInfoList _Sections;
|
|
public ItemInfoList Sections
|
|
{ get { return Lookup(2,ref _Sections); } }
|
|
private ItemInfoList _Cautions;
|
|
public ItemInfoList Cautions
|
|
{ get { return Lookup(3,ref _Cautions); } }
|
|
private ItemInfoList _Notes;
|
|
public ItemInfoList Notes
|
|
{ get { return Lookup(4,ref _Notes); } }
|
|
private ItemInfoList _RNOs;
|
|
public ItemInfoList RNOs
|
|
{ get { return Lookup(5,ref _RNOs); } }
|
|
private ItemInfoList _Steps;
|
|
public ItemInfoList Steps
|
|
{ get { return Lookup(6,ref _Steps); } }
|
|
private ItemInfoList _Tables;
|
|
public ItemInfoList Tables
|
|
{ get { return Lookup(7,ref _Tables); } }
|
|
//public XmlDocument ToXml()
|
|
//{
|
|
// XmlDocument retval = new XmlDocument();
|
|
// retval.LoadXml("<root/>");
|
|
// return ToXml(retval.DocumentElement);
|
|
//}
|
|
//public void AddList(XmlNode xn,string name, ItemInfoList itemInfoList)
|
|
//{
|
|
// if (itemInfoList != null)
|
|
// {
|
|
// XmlNode nd = xn.OwnerDocument.CreateElement(name);
|
|
// xn.AppendChild(nd);
|
|
// itemInfoList.ToXml(xn);
|
|
// }
|
|
//}
|
|
//public XmlDocument ToXml(XmlNode xn)
|
|
//{
|
|
// XmlNode nd = MyContent.ToXml(xn);
|
|
// // Now add the children
|
|
// AddList(nd, "Procedures", Procedures);
|
|
// AddList(nd, "Sections", Sections);
|
|
// AddList(nd, "Cautions", Cautions);
|
|
// AddList(nd, "Notes", Notes);
|
|
// AddList(nd, "RNOs", RNOs);
|
|
// AddList(nd, "Steps", SubItems);
|
|
// AddList(nd, "Tables", Tables);
|
|
// return xn.OwnerDocument;
|
|
//}
|
|
public string TabToolTip
|
|
{
|
|
get
|
|
{
|
|
if (MyContent.MyEntry == null)
|
|
return MyContent.Number + " - " + MyContent.Text;
|
|
string toolTip = MyProcedure.TabToolTip + "\r\n";
|
|
if (MyContent.Number != "")
|
|
toolTip += MyContent.Number + " - " + MyContent.Text;
|
|
else
|
|
toolTip += MyContent.Text;
|
|
DocumentInfo myDocument = MyContent.MyEntry.MyDocument;
|
|
if (myDocument.LibTitle != "")
|
|
{
|
|
toolTip += string.Format("\r\n(Library Document - {0})", myDocument.LibTitle);
|
|
toolTip += myDocument.LibraryDocumentUsage;
|
|
}
|
|
return toolTip;
|
|
}
|
|
}
|
|
public string TabTitle
|
|
{
|
|
get
|
|
{
|
|
if (MyContent.MyEntry == null)
|
|
return MyContent.Number;
|
|
if (MyContent.Number != "")
|
|
return MyContent.Number;
|
|
if (MyContent.Text.Length <= 10)
|
|
return MyContent.Text;
|
|
return MyContent.Text.Split(" ,.;:-_".ToCharArray())[0] + "...";
|
|
}
|
|
}
|
|
#region IVEReadOnlyItem
|
|
PartInfoList _PartInfoList;
|
|
public System.Collections.IList GetChildren()
|
|
{
|
|
_PartInfoList = this.MyContent.ContentParts;
|
|
if (_PartInfoList.Count == 1 && ( _PartInfoList[0].ToString() == "Sections" || _PartInfoList[0].ToString() == "Steps"))
|
|
return _PartInfoList[0].GetChildren();
|
|
return _PartInfoList;
|
|
}
|
|
public System.Collections.IList GetChildren(bool allParts)
|
|
{
|
|
_PartInfoList = this.MyContent.ContentParts;
|
|
if (allParts)
|
|
{
|
|
if (_PartInfoList.Count == 1 && (_PartInfoList[0].ToString() == "Sections" || _PartInfoList[0].ToString() == "Steps"))
|
|
return _PartInfoList[0].GetChildren();
|
|
return _PartInfoList;
|
|
}
|
|
else // Steps and Sections only
|
|
{
|
|
for(int i = 0;i<_PartInfoList.Count;i++)
|
|
if(_PartInfoList[i].ToString() == "Sections" || _PartInfoList[i].ToString() == "Steps")
|
|
return _PartInfoList[i].GetChildren();
|
|
return null;
|
|
}
|
|
}
|
|
//public bool ChildrenAreLoaded
|
|
//{
|
|
// get { return _PartInfoList == null; }
|
|
//}
|
|
public bool HasChildren
|
|
{
|
|
get { return this.MyContent.ContentPartCount > 0; }
|
|
}
|
|
public ItemInfo MyProcedure
|
|
{
|
|
get
|
|
{
|
|
// Walk up active parents until the parent is not an item
|
|
ItemInfo tmp = this;
|
|
while (tmp.ActiveParent.GetType() != typeof(DocVersionInfo)) tmp = (ItemInfo)tmp.ActiveParent;
|
|
return tmp;
|
|
}
|
|
}
|
|
private IVEDrillDownReadOnly _ActiveParent = null;
|
|
public IVEDrillDownReadOnly MyActiveParent { get { return _ActiveParent; } }
|
|
public IVEDrillDownReadOnly ActiveParent
|
|
{
|
|
get
|
|
{
|
|
if (_ActiveParent == null)
|
|
{
|
|
if (MyPrevious != null)
|
|
_ActiveParent = _MyPrevious.ActiveParent;
|
|
else
|
|
{
|
|
if (ItemDocVersionCount > 0)
|
|
_ActiveParent = this.ItemDocVersions[0];
|
|
else
|
|
{
|
|
ContentInfo parentContent = ParentContent;
|
|
if (parentContent == null || parentContent.ContentItemCount == 0)
|
|
_ActiveParent = this;
|
|
else
|
|
_ActiveParent = parentContent.ContentItems[0];
|
|
}
|
|
}
|
|
}
|
|
return _ActiveParent==this ? null : _ActiveParent;
|
|
}
|
|
internal set
|
|
{
|
|
_ActiveParent = value;
|
|
}
|
|
}
|
|
private ItemInfo _ActiveSection = null;
|
|
public ItemInfo MyActiveSection { get { return _ActiveSection; } }
|
|
public ItemInfo ActiveSection
|
|
{
|
|
get
|
|
{
|
|
if (_ActiveSection == null)
|
|
{
|
|
if (IsSection)
|
|
_ActiveSection = this;
|
|
else
|
|
{
|
|
ItemInfo parent = ActiveParent as ItemInfo;
|
|
if (parent != null)
|
|
_ActiveSection = parent.ActiveSection;
|
|
else
|
|
_ActiveSection = this;
|
|
}
|
|
}
|
|
return _ActiveSection.IsSection ? _ActiveSection : null;
|
|
}
|
|
set
|
|
{
|
|
_ActiveSection = value;
|
|
}
|
|
}
|
|
private FormatInfo _ActiveFormat = null;
|
|
public FormatInfo ActiveFormat
|
|
{
|
|
get
|
|
{
|
|
if(_ActiveFormat == null)
|
|
_ActiveFormat = (LocalFormat != null ? LocalFormat : ActiveParent.ActiveFormat);
|
|
return _ActiveFormat;
|
|
}
|
|
//get { return LocalFormat != null ? LocalFormat : ActiveParent.ActiveFormat; }
|
|
}
|
|
public FormatInfo LocalFormat
|
|
{
|
|
get { return MyContent.MyFormat; }
|
|
}
|
|
private DynamicTypeDescriptor _MyConfig=null;
|
|
public DynamicTypeDescriptor MyConfig
|
|
{
|
|
get
|
|
{
|
|
if (_MyConfig == null)
|
|
{
|
|
switch (MyContent.Type / 10000)
|
|
{
|
|
case 0:
|
|
_MyConfig = new ProcedureConfig(MyContent.Config);
|
|
break;
|
|
case 1:
|
|
_MyConfig = new SectionConfig(MyContent.Config);
|
|
break;
|
|
}
|
|
}
|
|
return _MyConfig;
|
|
}
|
|
}
|
|
//public bool HasStandardSteps()
|
|
//{ return MyContent.ContentItemCount > 1; }
|
|
public Color ForeColor
|
|
{ get { return (HasBrokenRules != null ? Color.Red : (MyContent.ContentItemCount > 1 ? Color.Blue : Color.Black)); } }
|
|
public Color BackColor
|
|
{ get { return (ItemAnnotationCount > 0 ? Color.Yellow : Color.White); } }
|
|
#endregion
|
|
internal ItemInfo(SafeDataReader dr, bool forItem)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ItemInfo.Constructor", GetHashCode());
|
|
try
|
|
{
|
|
ReadData(dr);
|
|
AddContent(dr);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ItemInfo.Constructor", ex);
|
|
throw new DbCslaException("ItemInfo.Constructor", ex);
|
|
}
|
|
}
|
|
private void AddContent(SafeDataReader dr)
|
|
{
|
|
_MyContent = new ContentInfo(dr, true);
|
|
}
|
|
}
|
|
#endregion ItemInfo
|
|
#region ItemInfoList
|
|
public partial class ItemInfoList
|
|
{
|
|
//public void ToXml(XmlNode xn)
|
|
//{
|
|
// foreach (ItemInfo itemInfo in this)
|
|
// {
|
|
// itemInfo.ToXml(xn);
|
|
// }
|
|
//}
|
|
internal ItemInfoList(ItemInfo itemInfo)
|
|
{
|
|
AddItem(itemInfo);
|
|
}
|
|
public static ItemInfoList GetList(int? itemID,int type)
|
|
{
|
|
try
|
|
{
|
|
ItemInfoList tmp = DataPortal.Fetch<ItemInfoList>(new ItemListCriteria(itemID,type));
|
|
ItemInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
#if (!ItemWithContent) // If ItemWithContent is set, the content is returned with the ItemInfoList
|
|
ContentInfoList.GetList(itemID); // Performance - Load All Content
|
|
#endif
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ItemInfoList.GetChildren", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ItemListCriteria
|
|
{
|
|
public ItemListCriteria(int? itemID,int type)
|
|
{
|
|
_ItemID = itemID;
|
|
_Type = type;
|
|
}
|
|
private int? _ItemID;
|
|
public int? ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
private int _Type;
|
|
public int Type
|
|
{
|
|
get { return _Type; }
|
|
set { _Type = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ItemListCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
#if ItemWithContent
|
|
cm.CommandText = "vesp_ListItemsAndContent";
|
|
#else
|
|
cm.CommandText = "vesp_ListItems";
|
|
#endif
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
while (dr.Read())
|
|
{
|
|
ItemInfo itemInfo = null;
|
|
switch ((E_FromType)criteria.Type)
|
|
{
|
|
case E_FromType.Procedure:
|
|
itemInfo = new ProcedureInfo(dr);
|
|
break;
|
|
case E_FromType.Section:
|
|
itemInfo = new SectionInfo(dr);
|
|
break;
|
|
default:
|
|
itemInfo = new StepInfo(dr);
|
|
break;
|
|
}
|
|
IsReadOnly = false;
|
|
this.Add(itemInfo);
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Database.LogException("ItemInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("ItemInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
internal void AddItem(ItemInfo itemInfo)
|
|
{
|
|
IsReadOnly = false;
|
|
this.Add(itemInfo);
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
#endregion
|
|
#region ProcedureInfo
|
|
[Serializable()]
|
|
public partial class ProcedureInfo : ItemInfo, IVEDrillDownReadOnly
|
|
{
|
|
#if ItemWithContent
|
|
public ProcedureInfo(SafeDataReader dr) : base(dr, true) { }
|
|
#else
|
|
public ProcedureInfo(SafeDataReader dr) : base(dr) { }
|
|
#endif
|
|
public new Procedure Get()
|
|
{
|
|
return (Procedure) (_Editable = Procedure.Get(ItemID));
|
|
}
|
|
#region ProcedureConfig
|
|
[NonSerialized]
|
|
private ProcedureConfig _ProcedureConfig;
|
|
public ProcedureConfig ProcedureConfig
|
|
{ get { return (_ProcedureConfig != null ? _ProcedureConfig : _ProcedureConfig = new ProcedureConfig(this)); } }
|
|
#endregion
|
|
public new DynamicTypeDescriptor MyConfig
|
|
{
|
|
get { return ProcedureConfig ; }
|
|
}
|
|
}
|
|
#endregion
|
|
#region Procedure
|
|
[Serializable()]
|
|
public partial class Procedure : Item
|
|
{
|
|
public new static Procedure Get(int itemID)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Item");
|
|
try
|
|
{
|
|
Procedure tmp = (Procedure)GetExistingByPrimaryKey(itemID);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Procedure>(new PKCriteria(itemID));
|
|
_AllList.Add(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found") tmp = null;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Item.Get", ex);
|
|
}
|
|
}
|
|
public new Procedure Save()
|
|
{
|
|
return (Procedure)base.Save();
|
|
}
|
|
#region ProcedureConfig
|
|
[NonSerialized]
|
|
private ProcedureConfig _ProcedureConfig;
|
|
public ProcedureConfig ProcedureConfig
|
|
{
|
|
get
|
|
{
|
|
if (_ProcedureConfig == null)
|
|
{
|
|
_ProcedureConfig = new ProcedureConfig(this);
|
|
_ProcedureConfig.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(_ProcedureConfig_PropertyChanged);
|
|
}
|
|
return _ProcedureConfig;
|
|
}
|
|
}
|
|
private void _ProcedureConfig_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
|
|
{
|
|
MyContent.Config = _ProcedureConfig.ToString();
|
|
}
|
|
#endregion
|
|
}
|
|
#endregion
|
|
#region SectionInfo
|
|
[Serializable()]
|
|
public partial class SectionInfo : ItemInfo, IVEDrillDownReadOnly
|
|
{
|
|
#if ItemWithContent
|
|
public SectionInfo(SafeDataReader dr) : base(dr, true) { }
|
|
#else
|
|
public SectionInfo(SafeDataReader dr) : base(dr) { }
|
|
#endif
|
|
public new Section Get()
|
|
{
|
|
return (Section)(_Editable = Section.Get(ItemID));
|
|
}
|
|
#region SectionConfig
|
|
[NonSerialized]
|
|
private SectionConfig _SectionConfig;
|
|
public SectionConfig SectionConfig
|
|
{ get { return (_SectionConfig != null ? _SectionConfig : _SectionConfig = new SectionConfig(this)); } }
|
|
#endregion
|
|
public new DynamicTypeDescriptor MyConfig
|
|
{
|
|
get { return SectionConfig; }
|
|
}
|
|
}
|
|
#endregion
|
|
#region Section
|
|
[Serializable()]
|
|
public partial class Section : Item
|
|
{
|
|
public new static Section Get(int itemID)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Item");
|
|
try
|
|
{
|
|
Section tmp = (Section)GetExistingByPrimaryKey(itemID);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Section>(new PKCriteria(itemID));
|
|
_AllList.Add(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found") tmp = null;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Item.Get", ex);
|
|
}
|
|
}
|
|
|
|
public new Section Save()
|
|
{
|
|
return (Section)base.Save();
|
|
}
|
|
|
|
#region SectionConfig
|
|
[NonSerialized]
|
|
private SectionConfig _SectionConfig;
|
|
public SectionConfig SectionConfig
|
|
{
|
|
get
|
|
{
|
|
if (_SectionConfig == null)
|
|
{
|
|
_SectionConfig = new SectionConfig(this);
|
|
_SectionConfig.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(_SectionConfig_PropertyChanged);
|
|
}
|
|
return _SectionConfig;
|
|
}
|
|
}
|
|
private void _SectionConfig_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
|
|
{
|
|
MyContent.Config = _SectionConfig.ToString();
|
|
}
|
|
#endregion
|
|
}
|
|
#endregion
|
|
#region StepInfo
|
|
[Serializable()]
|
|
public partial class StepInfo : ItemInfo
|
|
{
|
|
//public override string ToString()
|
|
//{
|
|
// return "Step " + base.ToString();
|
|
//}
|
|
#if ItemWithContent
|
|
public StepInfo(SafeDataReader dr) : base(dr, true) { }
|
|
#else
|
|
public StepInfo(SafeDataReader dr) : base(dr) { }
|
|
#endif
|
|
public new Step Get()
|
|
{
|
|
return (Step)(_Editable = Step.Get(ItemID));
|
|
}
|
|
//public E_FromType FromType
|
|
//{ get { return E_FromType.Step; } }
|
|
}
|
|
|
|
#endregion
|
|
#region Step
|
|
[Serializable()]
|
|
public partial class Step : Item
|
|
{
|
|
public new static Step Get(int itemID)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Item");
|
|
try
|
|
{
|
|
Step tmp = (Step)GetExistingByPrimaryKey(itemID);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Step>(new PKCriteria(itemID));
|
|
_AllList.Add(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found") tmp = null;
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Item.Get", ex);
|
|
}
|
|
}
|
|
//#region StepConfig
|
|
//[NonSerialized]
|
|
//private StepConfig _StepConfig;
|
|
//public StepConfig StepConfig
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_StepConfig == null)
|
|
// {
|
|
// _StepConfig = new StepConfig(this);
|
|
// _StepConfig.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(_StepConfig_PropertyChanged);
|
|
// }
|
|
// return _SectionConfig;
|
|
// }
|
|
//}
|
|
//private void _StepConfig_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
|
|
//{
|
|
// MyContent.Config = _StepConfig.ToString();
|
|
//}
|
|
//#endregion
|
|
}
|
|
#endregion
|
|
}
|