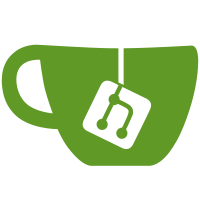
Load all at once code to support print. Open Command from the Start (PROMS2010) Menu. Enter key on treeview executes Open. The Treeview was retaining focus after opening procedures or accessory documents. This was fixed. Tab Bar initialized to the full width. Items were not always being displayed after the tree was clicked. The RO and Transition Info tabs are made invisible for accessory documents. The Tags, RO and Transition tabs are made invisible when no doucment is selected. Enter the DSO Framer window when it is created. Enter the Accessory page editor or step editor when a tab is selected. Remember the last selection in a step editor page when you return. Activate one of the remaining tabs when a tab is closed. Embed the pushpin images.
1584 lines
50 KiB
C#
1584 lines
50 KiB
C#
using System;
|
|
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using System.Collections.Specialized;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using Csla;
|
|
using DevComponents;
|
|
using DevComponents.DotNetBar;
|
|
using DevComponents.DotNetBar.Rendering;
|
|
using Csla.Validation;
|
|
using VEPROMS.Properties;
|
|
using Volian.Controls.Library;
|
|
using DescriptiveEnum;
|
|
|
|
[assembly: log4net.Config.XmlConfigurator(Watch = true)]
|
|
|
|
namespace VEPROMS
|
|
{
|
|
enum PropPgStyle { Button = 1, Tab = 2, Grid = 3 };
|
|
|
|
public partial class frmVEPROMS : DevComponents.DotNetBar.Office2007RibbonForm
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog log = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
Folder _LastFolder = null;
|
|
FolderInfo _LastFolderInfo = null;
|
|
Procedure _LastProcedure = null;
|
|
ProcedureInfo _LastProcedureInfo = null;
|
|
DocVersion _LastDocVersion = null;
|
|
DocVersionInfo _LastDocVersionInfo = null;
|
|
SectionInfo _LastSectionInfo = null;
|
|
Section _LastSection = null;
|
|
VETreeNode _LastTreeNode = null;
|
|
Step _LastStep = null;
|
|
StepInfo _LastStepInfo = null;
|
|
Color _CommentTitleBckColor;
|
|
StepRTB _MyRTB;
|
|
VETreeNode _PrevBookMark = null;
|
|
string _PathToROs = @"G:\PROMSDAT\VEHLP\RO\RO.FST";
|
|
|
|
public frmVEPROMS()
|
|
{
|
|
InitializeComponent();
|
|
|
|
// set the color of the ribbon
|
|
RibbonPredefinedColorSchemes.ChangeOffice2007ColorTable((eOffice2007ColorScheme)Settings.Default.SystemColor);
|
|
|
|
// LATER: get the user setting to control what is displayed for each tree node
|
|
// The logic is in the ToString() functions in FolderExt.cs and DocVersionExt.cs
|
|
//the GetFolder(1) function will read in the frist node (using ToString() to assign node text)
|
|
|
|
// Get the saved Tree Node Diplay settings
|
|
//if (Settings.Default["UseNameOnTreeNode"])
|
|
//{
|
|
//}
|
|
//if (Settings.Default["UseTitleOnTreeNode"])
|
|
//{
|
|
//}
|
|
|
|
VETreeNode tn = VETreeNode.GetFolder(1);
|
|
tv.Nodes.Add(tn);
|
|
tv.NodeNew += new Volian.Controls.Library.vlnTreeViewTreeNodeEvent(tv_NodeNew);
|
|
tv.NodeDragDrop += new Volian.Controls.Library.vlnTreeViewEvent(tv_NodeDragDrop);
|
|
tv.NodeDelete += new Volian.Controls.Library.vlnTreeViewBoolEvent(tv_NodeDelete);
|
|
tv.NodeProperties += new Volian.Controls.Library.vlnTreeViewEvent(tv_NodeProperties);
|
|
_CommentTitleBckColor = epAnnotations.TitleStyle.BackColor1.Color;
|
|
if (!btnAnnoDetailsPushPin.Checked)
|
|
epAnnotations.Expanded = false;
|
|
infoPanel.Expanded = false;
|
|
}
|
|
|
|
private void frmVEPROMS_FormClosing(object sender, FormClosingEventArgs e)
|
|
{
|
|
// Save the location and size of the VE-PROMS appication for this user
|
|
if (this.WindowState == FormWindowState.Normal)
|
|
{
|
|
Settings.Default.Location = this.Location;
|
|
Settings.Default.Size = this.Size;
|
|
}
|
|
Settings.Default.WindowState = this.WindowState;
|
|
SaveMRU();
|
|
//Settings.Default.Save();
|
|
}
|
|
private void ShutDownRibbons()
|
|
{
|
|
rtabEdit.Visible = false;
|
|
rtabHelp.Visible = false;
|
|
rtabHome.Visible = false;
|
|
rtabTools.Visible = false;
|
|
ribbonControl1.Height = 50;
|
|
}
|
|
private void frmVEPROMS_Load(object sender, EventArgs e)
|
|
{
|
|
// get the saved location and size of the VE-PROMS appication for this user
|
|
if (Settings.Default["Location"] != null) this.Location = Settings.Default.Location;
|
|
if (Settings.Default["Size"] != null) this.Size = Settings.Default.Size;
|
|
if (Settings.Default["WindowState"] != null) this.WindowState = Settings.Default.WindowState;
|
|
ShutDownRibbons();
|
|
_MyMRIList = MostRecentItemList.GetMRILst((System.Collections.Specialized.StringCollection)(Properties.Settings.Default["MRIList"]));
|
|
_MyBookMarks = MostRecentItemList.GetMRILst((System.Collections.Specialized.StringCollection)(Properties.Settings.Default["BookMarks"]));
|
|
SetupMRU();
|
|
SetupBookMarks();
|
|
SetupAnnotations();
|
|
}
|
|
|
|
private void SetupAnnotations()
|
|
{
|
|
cbAnnoType.DisplayMember = "Name";
|
|
cbAnnoType.DataSource = AnnotationTypeInfoList.Get();
|
|
lbResults.MouseMove += new MouseEventHandler(lbResults_MouseMove);
|
|
|
|
cbGridAnnoType.DisplayMember = "Name";
|
|
cbGridAnnoType.ValueMember = "TypeId";
|
|
cbGridAnnoType.DataSource = AnnotationTypeInfoList.Get().Clone();
|
|
}
|
|
|
|
#region MRU
|
|
private MostRecentItemList _MyMRIList;
|
|
private MostRecentItemList _MyBookMarks;
|
|
private void SetupBookMarks()
|
|
{
|
|
// TODO: load previously set bookmarks
|
|
lbxBookMarks.SelectedValueChanged += new EventHandler(lbxBookMarks_SelectedValueChanged);
|
|
RefreshBookMarkData();
|
|
btnPrevPos.Enabled = false;
|
|
//lbxBookMarks.Enabled = false;
|
|
_PrevBookMark = null;
|
|
}
|
|
|
|
void lbxBookMarks_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
btnRmvCurBookMrk.Enabled = (lbxBookMarks.SelectedIndex >= 0);
|
|
}
|
|
private void SetupMRU()
|
|
{
|
|
icRecentDocs.SubItems.Clear();
|
|
if (_MyMRIList.Count > 0)
|
|
{
|
|
LabelItem lblItem = new LabelItem();
|
|
lblItem.Text = "Recent Documents:";
|
|
icRecentDocs.SubItems.Add(lblItem);
|
|
//icRecentDocs.SubItems.Add();
|
|
for (int i = 0; i < _MyMRIList.Count; i++)
|
|
{
|
|
MostRecentItem mri = _MyMRIList[i];
|
|
ButtonItem btnItem = new ButtonItem();
|
|
if (i < 9)
|
|
btnItem.Text = string.Format("<b>&{0}.</b> {1}", i + 1, mri.MenuTitle);
|
|
else
|
|
btnItem.Text = string.Format(" {1}", i + 1, mri.MenuTitle);
|
|
btnItem.Tag = mri.ItemID;
|
|
btnItem.Tooltip = mri.ToolTip;
|
|
btnItem.Click += new EventHandler(btnItem_Click);
|
|
icRecentDocs.SubItems.Add(btnItem);
|
|
}
|
|
}
|
|
}
|
|
#if (DEBUG)
|
|
// to display a property page view
|
|
//private frmPropGrid f;
|
|
#endif
|
|
|
|
void btnItem_Click(object sender, EventArgs e)
|
|
{
|
|
ButtonItem btnItem = (ButtonItem)sender;
|
|
MostRecentItem mri = _MyMRIList.Add((int)(btnItem.Tag));
|
|
//SaveMRU();
|
|
SetupMRU();
|
|
if (mri != null) tc.OpenItem(mri.MyItemInfo);
|
|
#if (DEBUG)
|
|
// display a property page contianing the Tab Cotrol information
|
|
//f = new frmPropGrid(tc);
|
|
//f.Show();
|
|
#endif
|
|
}
|
|
private void SaveMRU()
|
|
{
|
|
Properties.Settings.Default.MRIList = _MyMRIList.ToSettings();
|
|
Properties.Settings.Default.BookMarks = _MyBookMarks.ToSettings();
|
|
Properties.Settings.Default.Save();
|
|
}
|
|
#endregion
|
|
#region Tree View
|
|
/// <summary>
|
|
/// Get the selected tree node's properties
|
|
/// </summary>
|
|
/// <param name="node">VETreeNode</param>
|
|
void SetupNodes(VETreeNode node)
|
|
{
|
|
//if (!node.Equals(_LastTreeNode))
|
|
//{
|
|
_LastTreeNode = node;
|
|
_LastFolderInfo = null;
|
|
_LastFolder = null;
|
|
_LastDocVersion = null;
|
|
_LastProcedure = null;
|
|
_LastSection = null;
|
|
_LastStep = null;
|
|
epAnnotations.Expanded = false;
|
|
rtxbComment.Text = "";
|
|
epAnnotations.TitleStyle.BackColor1.Color = _CommentTitleBckColor;
|
|
if (_MyMRIList.Add(node.VEObject) != null)
|
|
SetupMRU();
|
|
if (node.VEObject.GetType() == typeof(FolderInfo))
|
|
{
|
|
_LastFolderInfo = (FolderInfo)(_LastTreeNode.VEObject);
|
|
_LastFolder = _LastFolderInfo.Get();
|
|
}
|
|
else if (node.VEObject.GetType() == typeof(DocVersionInfo))
|
|
{
|
|
_LastDocVersionInfo = (DocVersionInfo)(node.VEObject);
|
|
_LastDocVersion = _LastDocVersionInfo.Get();
|
|
}
|
|
else if (node.VEObject.GetType() == typeof(ProcedureInfo))
|
|
{
|
|
_LastProcedureInfo = (ProcedureInfo)(_LastTreeNode.VEObject);
|
|
_LastProcedure = _LastProcedureInfo.Get();
|
|
|
|
tc.OpenItem((ItemInfo)_LastProcedureInfo);
|
|
// Display any annotations
|
|
//itemAnnotationsBindingSource.DataSource = _LastProcedureInfo.ItemAnnotations;
|
|
}
|
|
else if (node.VEObject.GetType() == typeof(SectionInfo))
|
|
{
|
|
_LastSectionInfo = (SectionInfo)(_LastTreeNode.VEObject);
|
|
_LastSection = _LastSectionInfo.Get();
|
|
tc.OpenItem((ItemInfo)_LastSectionInfo);
|
|
// Display any annotations
|
|
//itemAnnotationsBindingSource.DataSource = _LastSectionInfo.ItemAnnotations;
|
|
}
|
|
else if (node.VEObject.GetType() == typeof(StepInfo))
|
|
{
|
|
_LastStepInfo = (StepInfo)(_LastTreeNode.VEObject);
|
|
_LastStep = _LastStepInfo.Get();
|
|
tc.OpenItem((ItemInfo)_LastStepInfo);
|
|
// Display any annotations
|
|
//itemAnnotationsBindingSource.DataSource = _LastStepInfo.ItemAnnotations;
|
|
#region Sample Display Table Code
|
|
// display an exiting table in that rtf grid thing
|
|
//if ((_LastStepInfo.MyContent.Type == 20007) || (_LastStepInfo.MyContent.Type == 20009))
|
|
//{
|
|
// //MessageBox.Show("Source Grid");
|
|
// //frmTable newtable1 = new frmTable(_LastStepInfo.MyContent.Text);
|
|
// //newtable1.ShowDialog();
|
|
// //MessageBox.Show("IGrid");
|
|
// //frmIGrid newtable2 = new frmIGrid(_LastStepInfo.MyContent.Text);
|
|
// //newtable2.ShowDialog();
|
|
// //MessageBox.Show("GridView"); //standard Visual Studio Control
|
|
// //frmGridView newtable3 = new frmGridView(_LastStepInfo.MyContent.Text);
|
|
// //newtable3.ShowDialog();
|
|
// MessageBox.Show("FlexCell");
|
|
// frmFlexCell newtable4 = new frmFlexCell(_LastStepInfo.MyContent.Text);
|
|
// newtable4.ShowDialog();
|
|
//}
|
|
#endregion
|
|
}
|
|
//break;
|
|
//default:
|
|
//break;
|
|
//}
|
|
SetCaption((VETreeNode)node);
|
|
//}
|
|
}
|
|
|
|
private void SetCaption(VETreeNode tn)
|
|
{
|
|
StringBuilder caption = new StringBuilder();
|
|
string sep = string.Empty;
|
|
while (tn != null)
|
|
{
|
|
if (tn.VEObject.GetType() == typeof(FolderInfo) || tn.VEObject.GetType() == typeof(DocVersionInfo))
|
|
{
|
|
caption.Append(sep + tn.Text);
|
|
sep = " - ";
|
|
}
|
|
tn = (VETreeNode)tn.Parent;
|
|
}
|
|
this.Text = caption.ToString();
|
|
}
|
|
|
|
bool tv_NodeDelete(object sender, Volian.Controls.Library.vlnTreeEventArgs args)
|
|
{
|
|
SetupNodes((VETreeNode)args.Node);
|
|
if (_LastTreeNode.Nodes.Count > 0)
|
|
{
|
|
MessageBox.Show("Folder not empty", "Folder must be empty");
|
|
return false;
|
|
}
|
|
else
|
|
{
|
|
if (_LastFolderInfo != null)
|
|
{
|
|
DialogResult result = MessageBox.Show("Are you sure you want to delete " + _LastFolderInfo.Name, "Verify Folder Delete",
|
|
MessageBoxButtons.YesNo, MessageBoxIcon.Question);
|
|
if (result == DialogResult.Yes)
|
|
{
|
|
Folder.Delete(_LastFolderInfo.FolderID);
|
|
return true;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
MessageBox.Show("Not a folder", "Only Folder can be deleted");
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
bool IsFolder(VETreeNode veTreeNode)
|
|
{
|
|
return (veTreeNode.VEObject.GetType() == typeof(FolderInfo));
|
|
}
|
|
|
|
void tv_NodeDragDrop(object sender, Volian.Controls.Library.vlnTreeEventArgs args)
|
|
{
|
|
VETreeNode drag = (VETreeNode)args.Node;
|
|
VETreeNode drop = (VETreeNode)args.Destination;
|
|
if (IsFolder(drag) && IsFolder(drop))
|
|
{
|
|
FolderInfo fdragi = (FolderInfo)(drag.VEObject);
|
|
Folder fdrag = fdragi.Get();
|
|
FolderInfo fdropi = (FolderInfo)(drop.VEObject);
|
|
fdrag.MyParent = fdropi.Get();
|
|
fdrag.Save();
|
|
}
|
|
}
|
|
|
|
TreeNode tv_NodeNew(object sender, Volian.Controls.Library.vlnTreeEventArgs args)
|
|
{
|
|
SaveIfChanged();
|
|
FolderInfo fldi = (FolderInfo)((VETreeNode)(args.Node)).VEObject;
|
|
_LastFolder = Folder.MakeFolder(fldi.Get(), fldi.Get().MyConnection, "New Folder", string.Empty, "Short Name", null, string.Empty, DateTime.Now, "Test");
|
|
BrokenRulesCollection brs = _LastFolder.BrokenRulesCollection;// .BrokenRules();
|
|
if (brs != null)
|
|
{
|
|
foreach (BrokenRule br in brs)
|
|
{
|
|
Console.WriteLine("broken rule {0}", br.Description);
|
|
}
|
|
}
|
|
_LastFolderInfo = FolderInfo.Get(_LastFolder.FolderID);
|
|
VETreeNode tn = new VETreeNode((IVEDrillDownReadOnly)_LastFolderInfo);
|
|
SetupNodes(tn);
|
|
return tn;
|
|
}
|
|
|
|
private void SaveIfChanged()
|
|
{
|
|
SaveIfChanged<Folder>(_LastFolder);
|
|
SaveIfChanged<DocVersion>(_LastDocVersion);
|
|
SaveIfChanged2(_LastProcedure);
|
|
SaveIfChanged2(_LastSection);
|
|
SaveIfChanged2(_LastStep);
|
|
if (_LastTreeNode != null) _LastTreeNode.ResetNode();
|
|
}
|
|
|
|
private void SaveIfChanged<T>(BusinessBase<T> tmp)
|
|
where T : BusinessBase<T>
|
|
{
|
|
if (tmp == null) return;
|
|
if (tmp.IsDirty == false) return;
|
|
if (tmp.IsSavable)
|
|
tmp = tmp.Save();
|
|
else
|
|
{
|
|
foreach (Csla.Validation.BrokenRule br in tmp.BrokenRulesCollection)
|
|
{
|
|
Console.WriteLine("{0}", br.RuleName);
|
|
}
|
|
}
|
|
}
|
|
private void SaveIfChanged2(Item tmp)
|
|
{
|
|
SaveIfChanged<Item>(tmp);
|
|
}
|
|
/// <summary>
|
|
/// When the treeview is clicked - a timer is set
|
|
/// This is done because the focus is returned to the treeview after the click event
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void tv_Click(object sender, EventArgs e)
|
|
{
|
|
tmrTreeView.Enabled = true;
|
|
}
|
|
/// <summary>
|
|
/// This event is fired from the timer after the treeview click event completes
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void tmrTreeView_Tick(object sender, EventArgs e)
|
|
{
|
|
tmrTreeView.Enabled = false; // Timer has now fired
|
|
SaveIfChanged();
|
|
VETreeNode tn = tv.SelectedNode as VETreeNode;
|
|
if (tn != null) SetupNodes(tn);
|
|
}
|
|
private void tv_KeyPress(object sender, KeyPressEventArgs e)
|
|
{
|
|
if (e.KeyChar == '\r')
|
|
{
|
|
tv_Click(sender, new EventArgs());
|
|
e.Handled = true;
|
|
}
|
|
}
|
|
private void tv_BeforeExpand(object sender, TreeViewCancelEventArgs e)
|
|
{
|
|
VETreeNode tn = ((VETreeNode)e.Node);
|
|
tn.LoadingChildernDone += new VETreeNodeEvent(tn_LoadingChildernDone);
|
|
tn.LoadingChildernMax += new VETreeNodeEvent(tn_LoadingChildernMax);
|
|
tn.LoadingChildernValue += new VETreeNodeEvent(tn_LoadingChildernValue);
|
|
tn.LoadingChildrenSQL += new VETreeNodeEvent(tn_LoadingChildrenSQL);
|
|
tn.LoadChildren(true);
|
|
|
|
}
|
|
void tv_NodeProperties(object sender, Volian.Controls.Library.vlnTreeEventArgs args)
|
|
{
|
|
DisplayProperties();
|
|
}
|
|
#endregion
|
|
#region Property Page and Grid
|
|
|
|
/// <summary>
|
|
// this is used by tv_NodeProperties() and by ribbonFilePropertes_Click()
|
|
// the type of the args parameter for these two functions is different and
|
|
// type casting didn't work, so I put the guts in this function.
|
|
/// </summary>
|
|
private void DisplayProperties()
|
|
{
|
|
// check the user's preference on the style of poperty dialogs "PropPageStyle"
|
|
// 1 - Button dialog interface
|
|
// 2 - Tab dialog interface
|
|
// 3 - Property Grid interface
|
|
this.Cursor = Cursors.WaitCursor;
|
|
if ((int)Settings.Default["PropPageStyle"] == (int)PropPgStyle.Grid)
|
|
DoPropertyGrid(); // Will display a Property Grid interface
|
|
else
|
|
DoPropertyPages(); // Will display either a Button or a Tab dialog
|
|
this.Cursor = Cursors.Default;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Button from the Ribbon Bar
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnProperties_Click(object sender, EventArgs e)
|
|
{
|
|
DisplayProperties();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Get a System.Drawing.Color from an Argb or color name
|
|
/// </summary>
|
|
/// <param name="strColor">Color Name or "[(alpha,)red,green,blue]"</param>
|
|
/// <returns></returns>
|
|
private static Color cGetColor(string strColor)
|
|
{
|
|
// This was copied from frmFolderProperties.CS
|
|
Color rtnColor;
|
|
if (strColor == null || strColor.Equals(""))
|
|
rtnColor = Color.White;
|
|
else
|
|
{
|
|
if (strColor[0] == '[')
|
|
{
|
|
string[] parts = strColor.Substring(1, strColor.Length - 2).Split(",".ToCharArray());
|
|
int parts_cnt = 0;
|
|
foreach (string s in parts)
|
|
{
|
|
parts[parts_cnt] = parts[parts_cnt].TrimStart(' '); // remove preceeding blanks
|
|
parts_cnt++;
|
|
}
|
|
if (parts_cnt == 3)
|
|
rtnColor = Color.FromArgb(Int32.Parse(parts[0]), Int32.Parse(parts[1]), Int32.Parse(parts[2]));
|
|
else
|
|
rtnColor = Color.FromArgb(Int32.Parse(parts[0].Substring(2)), Int32.Parse(parts[1].Substring(2)), Int32.Parse(parts[2].Substring(2)), Int32.Parse(parts[3].Substring(2)));
|
|
}
|
|
else rtnColor = Color.FromName(strColor);
|
|
}
|
|
return rtnColor;
|
|
}
|
|
|
|
//private void SetupEditorColors(DisplayPanel vlnCSLAPanel1, TabPage pg)
|
|
//{
|
|
// // setup color
|
|
// if (_LastFolderInfo == null)
|
|
// {
|
|
// // user didn't select a FolderInfo type of node.
|
|
// // walk up the tree to find the first FolderInfo node type
|
|
// VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
|
// while (tn != null && tn.VEObject.GetType() != typeof(FolderInfo))
|
|
// tn = (VETreeNode)tn.Parent;
|
|
// _LastFolderInfo = (FolderInfo)(tn.VEObject);
|
|
// _LastFolder = _LastFolderInfo.Get();
|
|
// }
|
|
|
|
// if ((_LastFolderInfo.FolderConfig.Color_editbackground != null) && !(_LastFolderInfo.FolderConfig.Color_editbackground.Equals("")))
|
|
// {
|
|
// vlnCSLAPanel1.ActiveColor = cGetColor(_LastFolderInfo.FolderConfig.Color_editbackground);
|
|
// }
|
|
// if ((_LastFolderInfo.FolderConfig.Default_BkColor != null) && !(_LastFolderInfo.FolderConfig.Default_BkColor.Equals("")))
|
|
// {
|
|
// vlnCSLAPanel1.InactiveColor = _LastFolderInfo.FolderConfig.Default_BkColor;
|
|
// vlnCSLAPanel1.TabColor = vlnCSLAPanel1.InactiveColor;
|
|
// vlnCSLAPanel1.PanelColor = vlnCSLAPanel1.InactiveColor;
|
|
// pg.BackColor = vlnCSLAPanel1.InactiveColor;
|
|
// }
|
|
//}
|
|
|
|
/// <summary>
|
|
/// Display a Property Page for the current tree node
|
|
/// </summary>
|
|
private void DoPropertyPages()
|
|
{
|
|
// Display a property page. (the ppStyle selects either a tabbed or button interface)
|
|
VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
|
if (tn.VEObject.GetType() == typeof(FolderInfo))
|
|
{
|
|
frmFolderProperties frmfld = new frmFolderProperties(_LastFolder.FolderConfig);
|
|
if (frmfld.ShowDialog() == DialogResult.OK)
|
|
_LastFolder.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(DocVersionInfo))
|
|
{
|
|
frmVersionsProperties frmver = new frmVersionsProperties(_LastDocVersion.DocVersionConfig);
|
|
if (frmver.ShowDialog() == DialogResult.OK)
|
|
_LastDocVersion.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(ProcedureInfo))
|
|
{
|
|
frmProcedureProperties frmproc = new frmProcedureProperties(_LastProcedure.ProcedureConfig);
|
|
if (frmproc.ShowDialog() == DialogResult.OK)
|
|
_LastProcedure.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(SectionInfo))
|
|
{
|
|
frmSectionProperties frmsec = new frmSectionProperties(_LastSection.SectionConfig);
|
|
if (frmsec.ShowDialog() == DialogResult.OK)
|
|
_LastSection.Save();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Display a property grid for the current tree node
|
|
/// </summary>
|
|
private void DoPropertyGrid()
|
|
{
|
|
// Display a property grid
|
|
VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
|
frmPropGrid propGrid = null;
|
|
string title = "";
|
|
if (tn.VEObject.GetType() == typeof(FolderInfo))
|
|
{
|
|
title = string.Format("{0} Properties", _LastFolder.FolderConfig.Name);
|
|
propGrid = new frmPropGrid(_LastFolder.FolderConfig, title);
|
|
if (propGrid.ShowDialog() == DialogResult.OK)
|
|
_LastFolder.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(DocVersionInfo))
|
|
{
|
|
title = string.Format("{0} Properties", _LastDocVersion.DocVersionConfig.Name);
|
|
propGrid = new frmPropGrid(_LastDocVersion.DocVersionConfig, title);
|
|
if (propGrid.ShowDialog() == DialogResult.OK)
|
|
_LastDocVersion.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(ProcedureInfo))
|
|
{
|
|
title = string.Format("{0} {1} Properties", _LastProcedure.ProcedureConfig.Number, _LastProcedure.ProcedureConfig.Title);
|
|
propGrid = new frmPropGrid(_LastProcedure.ProcedureConfig, title);
|
|
if (propGrid.ShowDialog() == DialogResult.OK)
|
|
_LastProcedure.Save();
|
|
}
|
|
else if (tn.VEObject.GetType() == typeof(SectionInfo))
|
|
{
|
|
if (_LastSection.SectionConfig.Number.Length > 0)
|
|
title = string.Format("{0} {1} Properties", _LastSection.SectionConfig.Number, _LastSection.SectionConfig.Title);
|
|
else
|
|
title = string.Format("{0} Properties", _LastSection.SectionConfig.Title);
|
|
propGrid = new frmPropGrid(_LastSection.SectionConfig, title);
|
|
if (propGrid.ShowDialog() == DialogResult.OK)
|
|
_LastSection.Save();
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
#region Table Insert Sample Code
|
|
private void btnInsTable_Click(object sender, EventArgs e)
|
|
{
|
|
Point loc = btnInsTable.DisplayRectangle.Location;
|
|
loc.X += 300;
|
|
int top = this.Top + (btnInsTable.Size.Height * 2);
|
|
TablePickerDlg(sender, e, loc, top);
|
|
}
|
|
|
|
private void TablePickerDlg(object sender, EventArgs e, Point loc, int top)
|
|
{
|
|
Accentra.Controls.TablePicker tp = new Accentra.Controls.TablePicker();
|
|
tp.Location = loc;
|
|
tp.Top += top;
|
|
tp.Show();
|
|
while (tp.Visible)
|
|
{
|
|
Application.DoEvents();
|
|
System.Threading.Thread.Sleep(0);
|
|
}
|
|
// This was used to display a dialog containing a table grid
|
|
// using a product called Source Grid - was for demo purposes only
|
|
//
|
|
//if (!tp.Cancel)
|
|
//{
|
|
// frmTable newtable = new frmTable(tp.SelectedRows, tp.SelectedColumns);
|
|
// newtable.Show();
|
|
//}
|
|
}
|
|
#endregion
|
|
#region Edit/View Modes
|
|
/// <summary>
|
|
/// Edit Mode button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnEditMode_Click(object sender, EventArgs e)
|
|
{
|
|
btnEditView.Text = btnEditMode.Text;
|
|
}
|
|
|
|
/// <summary>
|
|
/// View Mode button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnViewMode_Click(object sender, EventArgs e)
|
|
{
|
|
btnEditView.Text = btnViewMode.Text;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Insert button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnInsertMode_Click(object sender, EventArgs e)
|
|
{
|
|
btnInsOvr.Text = btnInsertMode.Text;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Overwrite button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnOverstrikeMode_Click(object sender, EventArgs e)
|
|
{
|
|
btnInsOvr.Text = btnOverstrikeMode.Text;
|
|
}
|
|
|
|
#endregion
|
|
#region Multi User
|
|
|
|
/// <summary>
|
|
/// Set Lock button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnSetLock_Click(object sender, EventArgs e)
|
|
{
|
|
btnLckUlck.Image = btnSetLock.Image;
|
|
btnLckUlck.Text = "Locked";
|
|
}
|
|
|
|
/// <summary>
|
|
/// Release Lock button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnReleaseLck_Click(object sender, EventArgs e)
|
|
{
|
|
btnLckUlck.Image = btnReleaseLck.Image;
|
|
btnLckUlck.Text = "Unlocked";
|
|
}
|
|
|
|
#endregion
|
|
#region Progress Bar
|
|
|
|
/// <summary>
|
|
/// Used for the status bar in the lower left corner of the main screen
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void tn_LoadingChildrenSQL(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
ProgBarText = "Loading SQL";
|
|
}
|
|
|
|
/// <summary>
|
|
/// Used for the status bar in the lower left corner of the main screen
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void tn_LoadingChildernValue(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
ProgBarValue = args.Value;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Used for the status bar in the lower left corner of the main screen
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void tn_LoadingChildernMax(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
ProgBarMax = args.Value;
|
|
ProgBarText = "Loading...";
|
|
}
|
|
|
|
/// <summary>
|
|
/// Used for the status bar in the lower left corner of the main screen
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
void tn_LoadingChildernDone(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
ProgBarText = args.Info + " Seconds";
|
|
}
|
|
|
|
public int ProgBarMax
|
|
{
|
|
get { return bottomProgBar.Maximum; }
|
|
set { bottomProgBar.Maximum = value; }
|
|
}
|
|
|
|
public int ProgBarValue
|
|
{
|
|
get { return bottomProgBar.Value; }
|
|
set { bottomProgBar.Value = value; }
|
|
}
|
|
|
|
public string ProgBarText
|
|
{
|
|
get { return bottomProgBar.Text; }
|
|
set
|
|
{
|
|
bottomProgBar.TextVisible = true;
|
|
bottomProgBar.Text = value;
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
#region Views: Procedure Background Deviation
|
|
/// <summary>
|
|
/// Procedure Step View button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnViewPrcStp_Click(object sender, EventArgs e)
|
|
{
|
|
btnViewTypes.Text = btnViewPrcStp.Text;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Background Text View button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnViewBckgnd_Click(object sender, EventArgs e)
|
|
{
|
|
btnViewTypes.Text = btnViewBckgnd.Text;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Deviation Text View button on the bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnViewDev_Click(object sender, EventArgs e)
|
|
{
|
|
btnViewTypes.Text = btnViewDev.Text;
|
|
}
|
|
#endregion
|
|
#region Bookmarks
|
|
|
|
/// <summary>
|
|
/// Mark Position button on bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnMarkPrevPos_Click(object sender, EventArgs e)
|
|
{
|
|
btnSetBookMrk_Click(sender, e);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Previous button on bottom tool bar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnPrevPos_Click(object sender, EventArgs e)
|
|
{
|
|
//if (_PrevBookMark != null)
|
|
//{
|
|
// VETreeNode jumpToHere = _PrevBookMark; // save current previous
|
|
// btnSetBookMrk_Click(sender, e); // save current as the new previous
|
|
// tv.SelectedNode = jumpToHere; // jump to the saved previous
|
|
//}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Clear Bookmarks button on Information pannel
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnClrBookMrks_Click(object sender, EventArgs e)
|
|
{
|
|
_MyBookMarks.Clear();
|
|
RefreshBookMarkData();
|
|
//lbxBookMarks.Items.Clear();
|
|
//lbxBookMarks.Enabled = false;
|
|
//btnPrevPos.Enabled = false;
|
|
//btnClrBookMrks.Enabled = false;
|
|
//btnRmvCurBookMrk.Enabled = false;
|
|
//_PrevBookMark = null;
|
|
}
|
|
|
|
private void RefreshBookMarkData()
|
|
{
|
|
lbxBookMarks.DataSource = null;
|
|
lbxBookMarks.DisplayMember = "MenuTitle";
|
|
lbxBookMarks.DataSource = _MyBookMarks;
|
|
btnClrBookMrks.Enabled = (lbxBookMarks.Items.Count > 0);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Remove Bookmark button on Information pannel
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnRmvCurBookMrk_Click(object sender, EventArgs e)
|
|
{
|
|
_MyBookMarks.RemoveAt(lbxBookMarks.SelectedIndex);
|
|
RefreshBookMarkData();
|
|
// lbxBookMarks.Items.RemoveAt(lbxBookMarks.SelectedIndex);
|
|
// if (lbxBookMarks.Items.Count == 0)
|
|
// {
|
|
// //lbxBookMarks.Enabled = false;
|
|
// btnPrevPos.Enabled = false;
|
|
// btnClrBookMrks.Enabled = (lbxBookMarks.Items.Count > 0);
|
|
// //btnRmvCurBookMrk.Enabled = (lbxBookMarks.SelectedIndex >= 0);
|
|
// _PrevBookMark = null;
|
|
// }
|
|
}
|
|
|
|
/// <summary>
|
|
/// Adds the given tree node to the list of bookmarks
|
|
/// </summary>
|
|
/// <param name="bkmrk"></param>
|
|
//private void AddToBookMarkList(VETreeNode bkmrk)
|
|
//{
|
|
// if (!(lbxBookMarks.Items.Contains(bkmrk)))
|
|
// lbxBookMarks.Items.Add(bkmrk);
|
|
//}
|
|
|
|
/// <summary>
|
|
/// Set Bookmark button on the Information pannel
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnSetBookMrk_Click(object sender, EventArgs e)
|
|
{
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
_MyBookMarks.Add(dtp.SelectedStepItem.MyItemInfo);
|
|
RefreshBookMarkData();
|
|
//VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
|
//AddToBookMarkList(tn);
|
|
//_PrevBookMark = tn;
|
|
//lbxBookMarks.Enabled = true;
|
|
//btnPrevPos.Enabled = true;
|
|
//btnClrBookMrks.Enabled = true;
|
|
//btnRmvCurBookMrk.Enabled = true;
|
|
}
|
|
|
|
#endregion
|
|
#region DisplayPanel
|
|
|
|
/// <summary>
|
|
/// Jump to the location referenced by the transition
|
|
/// </summary>
|
|
/// <param name="item"></param>
|
|
private void OpenTransition(ItemInfo item)
|
|
{
|
|
tc.OpenItem(item);
|
|
}
|
|
|
|
//private void OpenItem(ItemInfo item)
|
|
//{
|
|
// DisplayPanel vlnCSLAPanel1 = AddProcedureTab(item.MyProcedure);
|
|
// vlnCSLAPanel1.ItemSelect(item);
|
|
//}
|
|
|
|
private void vlnCSLAPanel1_LinkClicked(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
switch (args.MyLinkText.MyParsedLinkType)
|
|
{
|
|
case ParsedLinkType.ReferencedObject:
|
|
MessageBox.Show(args.MyLinkText.Roid, args.MyLinkText.MyParsedLinkType.ToString(), MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
break;
|
|
case ParsedLinkType.Transition:
|
|
case ParsedLinkType.TransitionRange:
|
|
tc.OpenItem(args.MyLinkText.MyTransitionInfo.MyItemToID);
|
|
break;
|
|
}
|
|
}
|
|
|
|
//private DisplayPanel GetPanelFromPage(TabPage tp)
|
|
//{
|
|
// return (DisplayPanel)tp.Controls["vlnCSLAPanel1"];
|
|
//}
|
|
|
|
//private DisplayPanel AddProcedureTab(ItemInfo pi)
|
|
//{
|
|
// if (tc.TabPages.ContainsKey(pi.MyContent.Number))
|
|
// {
|
|
// tc.SelectTab(pi.MyContent.Number);
|
|
// return GetPanelFromPage(tc.SelectedTab);
|
|
// }
|
|
// else
|
|
// {
|
|
// TabPage pg = new TabPage(pi.MyContent.Number);
|
|
// pg.Name = pi.MyContent.Number;
|
|
// tc.TabPages.Add(pg);
|
|
// tc.SelectedTab = pg;
|
|
// DisplayPanel vlnCSLAPanel1 = new Volian.Controls.Library.DisplayPanel(this.components);
|
|
// pg.Controls.Add(vlnCSLAPanel1);
|
|
// //
|
|
// // vlnCSLAPanel1
|
|
// //
|
|
// vlnCSLAPanel1.ActiveColor = System.Drawing.Color.SkyBlue;
|
|
// vlnCSLAPanel1.AutoScroll = true;
|
|
// vlnCSLAPanel1.Dock = System.Windows.Forms.DockStyle.Fill;
|
|
// vlnCSLAPanel1.InactiveColor = System.Drawing.Color.Linen;
|
|
// vlnCSLAPanel1.Name = "vlnCSLAPanel1";
|
|
// vlnCSLAPanel1.PanelColor = System.Drawing.Color.LightGray;
|
|
// vlnCSLAPanel1.ProcFont = new System.Drawing.Font("Arial", 12F, System.Drawing.FontStyle.Bold);
|
|
// vlnCSLAPanel1.SectFont = new System.Drawing.Font("Arial", 10F, System.Drawing.FontStyle.Bold);
|
|
// vlnCSLAPanel1.StepFont = new System.Drawing.Font("Arial", 10F);
|
|
// vlnCSLAPanel1.TabColor = System.Drawing.Color.Beige;
|
|
// SetupEditorColors(vlnCSLAPanel1,pg); // check for overridden color values
|
|
// vlnCSLAPanel1.TabIndex = 9;
|
|
// vlnCSLAPanel1.LinkClicked += new Volian.Controls.Library.DisplayPanelLinkEvent(this.vlnCSLAPanel1_LinkClicked);
|
|
// vlnCSLAPanel1.MyItem = pi;
|
|
// return vlnCSLAPanel1;
|
|
// }
|
|
//}
|
|
#endregion
|
|
#region Font
|
|
|
|
/// <summary>
|
|
/// Bold button on the ribbon
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnBold_Click(object sender, EventArgs e)
|
|
{
|
|
btnBold.Checked = !btnBold.Checked;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Italics button on the ribbon
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnItalics_Click(object sender, EventArgs e)
|
|
{
|
|
btnItalics.Checked = !btnItalics.Checked;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Underline button on the ribbon
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnUline_Click(object sender, EventArgs e)
|
|
{
|
|
btnUline.Checked = !btnUline.Checked;
|
|
}
|
|
#endregion
|
|
#region Find/Replace and Search
|
|
|
|
/// <summary>
|
|
/// Find/Replace button on the ribbon
|
|
/// Display the Find/Replace dialog
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnFindRplDlg_Click_1(object sender, EventArgs e)
|
|
{
|
|
FindReplace frmFindRepl = new FindReplace();
|
|
frmFindRepl.Show();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Global Search button on the ribbon
|
|
/// Opens the Information Pannel and selects the Results tab
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnGlbSrch_Click(object sender, EventArgs e)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabResults;
|
|
btnSrchRslt.Checked = true;
|
|
}
|
|
|
|
#endregion
|
|
#region Similar Steps
|
|
|
|
/// <summary>
|
|
/// Similar Steps button on the ribbon
|
|
/// Opens the Information Pannel and selects the Results tab
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnSimStps_Click(object sender, EventArgs e)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabResults;
|
|
btnSimStpsRslt.Checked = true;
|
|
}
|
|
|
|
#endregion
|
|
#region Help/About
|
|
|
|
/// <summary>
|
|
/// About button on the ribbon
|
|
/// Display the About dialog
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnAbout_Click(object sender, EventArgs e)
|
|
{
|
|
AboutVEPROMS about = new AboutVEPROMS();
|
|
about.ShowDialog();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Volian Web button on the ribbon
|
|
/// display the Volian web site on a pop up form
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnVlnWeb_Click(object sender, EventArgs e)
|
|
{
|
|
VlnWeb veWWW = new VlnWeb();
|
|
veWWW.Show();
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Ribbon
|
|
/// <summary>
|
|
/// This Opens the treeView or opens the selected item in the TreeView
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnOpen_Click(object sender, EventArgs e)
|
|
{
|
|
if (!expandablePanel2.Expanded) // If panel not expanded - expand it.
|
|
{
|
|
expandablePanel2.Expanded = true;
|
|
if(tv.Nodes.Count > 0)tv.SelectedNode = tv.Nodes[0];
|
|
tv.Focus();
|
|
}
|
|
else
|
|
{
|
|
VETreeNode tn = (VETreeNode)(tv.SelectedNode);
|
|
if (tn != null)
|
|
{
|
|
if (tn.VEObject.GetType() == typeof(FolderInfo) || tn.VEObject.GetType() == typeof(DocVersionInfo))
|
|
{
|
|
if (tn.Nodes.Count > 0)
|
|
{
|
|
tn.Expand();
|
|
tv.SelectedNode = tn.Nodes[0];
|
|
tv.Focus();
|
|
}
|
|
}
|
|
else
|
|
SetupNodes(tn);
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Options button on the dialog that appears when the V icon is clicked (top left of application window)
|
|
/// note that the "V icon" is also called the Office 2007 Start Button
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnOptions_Click(object sender, EventArgs e)
|
|
{
|
|
frmSysOptions VeSysOpts = new frmSysOptions();
|
|
VeSysOpts.ShowDialog();
|
|
|
|
}
|
|
|
|
/// <summary>
|
|
/// Exit button on the dialog that appears when the V icon is clicked (top left of application window)
|
|
/// note that the "V icon" is also called the Office 2007 Start Button
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void btnExit_Click(object sender, EventArgs e)
|
|
{
|
|
this.Close();
|
|
}
|
|
#endregion
|
|
#region InfoTabRO
|
|
private void infotabRO_Click(object sender, EventArgs e)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabRO;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
ROFST rofst = new ROFST(_PathToROs); //"g:\\vehlp\\ro\\ro.fst");
|
|
displayRO.MyROFST = rofst;
|
|
displayRO.MyRTB = dtp.MyStepPanel.SelectedStepItem.MyStepRTB;
|
|
}
|
|
#endregion
|
|
#region InfoTabTransition
|
|
private void infotabTransition_Click(object sender, EventArgs e)
|
|
{
|
|
if (tc == null || tc.SelectedDisplayTabItem == null) return;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
displayTransition.MyRTB = dtp.MyStepPanel.SelectedStepItem.MyStepRTB;
|
|
displayTransition.CurTrans = null;
|
|
}
|
|
private void btnInsTrans_Click(object sender, EventArgs e)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabTransition;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
displayTransition.MyRTB = _MyRTB;
|
|
displayTransition.CurTrans = null;
|
|
}
|
|
#endregion
|
|
private AnnotationInfoList _Annotations;
|
|
private ItemInfo _CurrentItem = null;
|
|
|
|
private void tc_ItemSelectedChanged(object sender, ItemSelectedChangedEventArgs args)
|
|
{
|
|
//CurrentAnnotation = null;
|
|
if (args == null)
|
|
{
|
|
_CurrentItem = null;
|
|
CurrentAnnotation = null;
|
|
_Annotations = null;
|
|
itemAnnotationsBindingSource.DataSource = _Annotations;
|
|
AnnotationPanelView();
|
|
infotabRO.Visible = infotabTransition.Visible = infotabTags.Visible = false;
|
|
return;
|
|
}
|
|
if (_CurrentItem != args.MyItemInfo)
|
|
_CurrentItem = args.MyItemInfo; //tc.SelectedDisplayTabItem.MyItemInfo;
|
|
//vlnStackTrace.ShowStack("enter tc_ItemSelectedChanged {0}", _CurrentItem);
|
|
if (args.MyStepItem == null)
|
|
{
|
|
infotabRO.Visible = infotabTransition.Visible = false;
|
|
infotabTags.Visible = true;
|
|
//vlnStackTrace.ShowStack("enter tc_ItemSelectedChanged {0}", _CurrentItem);
|
|
}
|
|
else
|
|
{
|
|
//vlnStackTrace.ShowStack("enter tc_ItemSelectedChanged {0}", _CurrentItem);
|
|
infotabRO.Visible = infotabTransition.Visible = infotabTags.Visible = true;
|
|
displayTransition.MyRTB = args.MyStepItem.MyStepRTB;
|
|
displayRO.MyRTB = args.MyStepItem.MyStepRTB;
|
|
}
|
|
UpdateAnnotationGrid();
|
|
}
|
|
private void UpdateAnnotationGrid()
|
|
{
|
|
//ShowItemAnnotations("UpdateAnnotationGrid before RefreshItemAnnotations");
|
|
//_CurrentItem.RefreshItemAnnotations();
|
|
//ShowItemAnnotations("UpdateAnnotationGrid");
|
|
_LoadingGrid = true;
|
|
_Annotations = _CurrentItem.ItemAnnotations;
|
|
itemAnnotationsBindingSource.DataSource = _Annotations;
|
|
AnnotationPanelView();
|
|
if ((CurrentAnnotation == null || (_CurrentItem.ItemID != CurrentAnnotation.ItemID)))
|
|
{
|
|
if (_Annotations != null && _Annotations.Count > 0)
|
|
CurrentAnnotation = _Annotations[0];
|
|
else
|
|
CurrentAnnotation = null;
|
|
//InitializeAnnotation();
|
|
}
|
|
FindCurrentAnnotation();
|
|
_LoadingGrid = false;
|
|
}
|
|
private void FindCurrentAnnotation()
|
|
{
|
|
int row = 0;
|
|
if (CurrentAnnotation != null)
|
|
{
|
|
if (_Annotations != null)
|
|
{
|
|
foreach (AnnotationInfo ai in _Annotations)
|
|
{
|
|
if (ai.AnnotationID == CurrentAnnotation.AnnotationID)
|
|
{
|
|
row = _Annotations.IndexOf(ai) + 1;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
//Console.WriteLine("Row = {0}", row);
|
|
c1AnnotationGrid.Select(row, 0, true);
|
|
}
|
|
//private void ShowItemAnnotations(string title)
|
|
//{
|
|
// Console.WriteLine("{0} CurrentItem {1} {2} ItemAnnotationsCount {3}", title, _CurrentItem.ItemID, _CurrentItem.MyItemInfoUnique, _CurrentItem.ItemAnnotationCount);
|
|
//}
|
|
//private void itemAnnotationsBindingSource_DataSourceChanged(object sender, EventArgs e)
|
|
//{
|
|
//_LoadingGrid = true;
|
|
//Console.WriteLine("itemAnnotationsBindingSource_DataSourceChanged 1");
|
|
//AnnotationInfoList myAnnotations = itemAnnotationsBindingSource.DataSource as AnnotationInfoList;
|
|
//Console.WriteLine("itemAnnotationsBindingSource_DataSourceChanged 2");
|
|
//AnnotationPannelView(_Annotations);
|
|
//Console.WriteLine("itemAnnotationsBindingSource_DataSourceChanged 3");
|
|
//_LoadingGrid = false;
|
|
//}
|
|
private void AnnotationPanelView()
|
|
{
|
|
if (_Annotations != null && _Annotations.Count != 0)
|
|
{
|
|
if (Settings.Default.AutoPopUpAnnotations) //cbAnnotationPopup.Checked
|
|
epAnnotations.Expanded = true;
|
|
else
|
|
epAnnotations.TitleStyle.BackColor1.Color = Color.Yellow;
|
|
rtxbComment.SelectionStart = rtxbComment.TextLength;
|
|
}
|
|
else
|
|
{
|
|
if (!btnAnnoDetailsPushPin.Checked)
|
|
epAnnotations.Expanded = false;
|
|
epAnnotations.TitleStyle.BackColor1.Color = _CommentTitleBckColor;
|
|
rtxbComment.Text = null;
|
|
}
|
|
}
|
|
|
|
private AnnotationInfo _CurrentAnnotation = null;
|
|
private AnnotationInfo CurrentAnnotation
|
|
{
|
|
get { return _CurrentAnnotation; }
|
|
set
|
|
{
|
|
if (_CurrentAnnotation == null && value == null) return; // No Change
|
|
if (_CurrentAnnotation != null && value != null)
|
|
if (_CurrentAnnotation.AnnotationID == value.AnnotationID) return; // No Change
|
|
//vlnStackTrace.ShowStack("CurrentAnnotation = '{0}' Old = '{1}'", value, _CurrentAnnotation);
|
|
if (_CurrentAnnotation != null || _AddingAnnotation)
|
|
{
|
|
//if (_CurrentAnnotation.TypeID != (int)cbGridAnnoType.SelectedValue) SaveAnnotation();
|
|
//else if (_CurrentAnnotation.RtfText != string.Empty && _CurrentAnnotation.RtfText != rtxbComment.Rtf)
|
|
// SaveAnnotation();
|
|
//else if (_CurrentAnnotation.RtfText == string.Empty && _CurrentAnnotation.SearchText != rtxbComment.Text)
|
|
if (AnnotationDirty)
|
|
SaveAnnotation();
|
|
}
|
|
_CurrentAnnotation = value;
|
|
InitializeAnnotation();
|
|
}
|
|
}
|
|
|
|
private void SaveAnnotation()
|
|
{
|
|
if (cbGridAnnoType.SelectedIndex == -1) return;
|
|
if (rtxbComment.Text == string.Empty) return;
|
|
using (AnnotationType annotationType = AnnotationType.Get((int)cbGridAnnoType.SelectedValue))
|
|
{
|
|
if (_AddingAnnotation)
|
|
{
|
|
_AddingAnnotation = false;
|
|
using (Item myItem = _CurrentItem.Get())
|
|
{
|
|
using (Annotation annotation = Annotation.MakeAnnotation(myItem, annotationType, rtxbComment.Rtf, rtxbComment.Text, ""))
|
|
{
|
|
CurrentAnnotation = AnnotationInfo.Get(annotation.AnnotationID);
|
|
annotation.DTS = DateTime.Now;
|
|
annotation.Save();
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
using (Annotation annotation = CurrentAnnotation.Get())
|
|
{
|
|
annotation.RtfText = rtxbComment.Rtf;
|
|
annotation.SearchText = rtxbComment.Text;
|
|
annotation.MyAnnotationType = annotationType;
|
|
annotation.DTS = DateTime.Now;
|
|
//Console.WriteLine("Before Annotation Type = {0} ({1})", CurrentAnnotation.MyAnnotationType, CurrentAnnotation.MyAnnotationType.AnnotationTypeAnnotations.Count);
|
|
//Console.WriteLine("Before Annotation Type = {0}", CurrentAnnotation.MyAnnotationType);
|
|
annotation.Save();
|
|
//Console.WriteLine("After Annotation Type = {0} ({1})", CurrentAnnotation.MyAnnotationType, CurrentAnnotation.MyAnnotationType.AnnotationTypeAnnotations.Count);
|
|
}
|
|
}
|
|
}
|
|
AnnotationDirty = false;
|
|
UpdateAnnotationGrid();
|
|
//UpdateAnnotationSearchResults();
|
|
}
|
|
|
|
|
|
private void btnSave_Click(object sender, EventArgs e)
|
|
{
|
|
|
|
}
|
|
private void tc_LinkActiveChanged(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
// determine if any infotabs are visisble, and if it is the Transition,
|
|
// change the curitem for the transition to the current item.
|
|
if (infoPanel.Expanded == true && infoTabs.SelectedTab == infotabTransition)
|
|
{
|
|
displayTransition.CurTrans = null;
|
|
}
|
|
}
|
|
private void tc_LinkInsertTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabTransition;
|
|
displayTransition.CurTrans = null;
|
|
}
|
|
private void tc_LinkInsertRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabRO;
|
|
ROFST rofst = new ROFST(_PathToROs); //"g:\\vehlp\\ro\\ro.fst");
|
|
displayRO.MyROFST = rofst;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
displayRO.MyRTB = dtp.MyStepPanel.SelectedStepItem.MyStepRTB;
|
|
displayRO.CurROLink = null;
|
|
}
|
|
private void tc_LinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabTransition;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
displayTransition.MyRTB = dtp.MyStepPanel.SelectedStepItem.MyStepRTB;
|
|
displayTransition.CurTrans = args.MyLinkText.MyTransitionInfo;
|
|
}
|
|
|
|
private void tc_LinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
infoPanel.Expanded = true;
|
|
infoTabs.SelectedTab = infotabRO;
|
|
ROFST rofst = new ROFST(_PathToROs); //"g:\\vehlp\\ro\\ro.fst");
|
|
displayRO.MyROFST = rofst;
|
|
StepTabPanel dtp = ((DisplayTabItem)tc.SelectedDisplayTabItem).MyStepTabPanel;
|
|
if (dtp == null) return;
|
|
displayRO.MyRTB = dtp.MyStepPanel.SelectedStepItem.MyStepRTB;
|
|
displayRO.CurROLink = args.MyLinkText.RoUsageid; // this is wrong - use for now.
|
|
}
|
|
|
|
private bool _LoadingList = false;
|
|
private void cbAnnoType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
UpdateAnnotationSearchResults();
|
|
}
|
|
|
|
private void UpdateAnnotationSearchResults()
|
|
{
|
|
AnnotationTypeInfo ati = cbAnnoType.SelectedValue as AnnotationTypeInfo;
|
|
_LoadingList = true;
|
|
lbResults.DisplayMember = "SearchText";
|
|
lbResults.DataSource = ati.AnnotationTypeAnnotations;
|
|
lbResults.SelectedIndex = -1;
|
|
LastResultsMouseOverIndex = -1;
|
|
_LoadingList = false;
|
|
}
|
|
|
|
private int LastResultsMouseOverIndex = -1;
|
|
void lbResults_MouseMove(object sender, MouseEventArgs e)
|
|
{
|
|
int ResultsMouseOverIndex = lbResults.IndexFromPoint(e.Location);
|
|
if (ResultsMouseOverIndex != -1 && ResultsMouseOverIndex != LastResultsMouseOverIndex)
|
|
{
|
|
AnnotationInfo ai = lbResults.Items[ResultsMouseOverIndex] as AnnotationInfo;
|
|
|
|
toolTip1.SetToolTip(lbResults, ai.MyItem.Path);
|
|
LastResultsMouseOverIndex = ResultsMouseOverIndex;
|
|
}
|
|
}
|
|
private void lbResults_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingList)
|
|
{
|
|
//AnnotationInfo ai = lbResults.SelectedValue as AnnotationInfo;
|
|
//if(ai != null)
|
|
// tc.OpenItem(ai.MyItem);
|
|
CurrentAnnotation = lbResults.SelectedValue as AnnotationInfo;
|
|
if (CurrentAnnotation != null)
|
|
{
|
|
tc.OpenItem(CurrentAnnotation.MyItem);
|
|
}
|
|
FindCurrentAnnotation();
|
|
}
|
|
}
|
|
private void lbxBookMarks_Click(object sender, EventArgs e)
|
|
{
|
|
MostRecentItem itm = lbxBookMarks.SelectedValue as MostRecentItem;
|
|
tc.OpenItem(itm.MyItemInfo);
|
|
}
|
|
private void cbGridAnnoType_Validating(object sender, CancelEventArgs e)
|
|
{
|
|
//try
|
|
//{
|
|
// //bool test1 = c1AnnotationGrid.IsCellSelected(c1AnnotationGrid.RowSel, c1AnnotationGrid.ColSel);
|
|
// //bool test2 = c1AnnotationGrid.IsCellValid(c1AnnotationGrid.RowSel, c1AnnotationGrid.ColSel);
|
|
// //AnnotationTypeInfo celldat = (AnnotationTypeInfo)c1AnnotationGrid.GetData(c1AnnotationGrid.RowSel, c1AnnotationGrid.ColSel);
|
|
// AnnotationTypeInfo newcelldat = (AnnotationTypeInfo)cbGridAnnoType.SelectedItem;
|
|
|
|
// AnnotationInfo ani = ((AnnotationInfoList)itemAnnotationsBindingSource.DataSource)[c1AnnotationGrid.RowSel - 1] as AnnotationInfo;
|
|
// Annotation ano = ani.Get();
|
|
// if (ano.TypeID != newcelldat.TypeID)
|
|
// {
|
|
// ano.Update(newcelldat.TypeID);
|
|
// //btnCancelAnnoation.Enabled = true;
|
|
// c1AnnotationGrid.Refresh();
|
|
// }
|
|
//}
|
|
//catch (Exception ex)
|
|
//{
|
|
// log.ErrorFormat("Error saving Annotation Type Change: {0}", ex.Message);
|
|
//}
|
|
}
|
|
private void epAnnotations_Layout(object sender, LayoutEventArgs e)
|
|
{
|
|
//cbGridAnnoType.Visible = false;
|
|
}
|
|
//private void btnSaveAnnoation_Click(object sender, EventArgs e)
|
|
//{
|
|
// AnnotationInfoList ail = (AnnotationInfoList)itemAnnotationsBindingSource.DataSource;
|
|
// foreach (AnnotationInfo ai in ail)
|
|
// {
|
|
// Annotation ano = ai.Get();
|
|
// ano.CommitChanges();
|
|
// }
|
|
// btnCancelAnnoation.Enabled = false;
|
|
//}
|
|
private bool _AddingAnnotation = false;
|
|
private void btnAddAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
c1AnnotationGrid.Row = -1;
|
|
CurrentAnnotation = null;
|
|
_AddingAnnotation = true;
|
|
//InitializeAnnotation();
|
|
}
|
|
private bool _LoadingAnnotation = false;
|
|
private bool _LoadingGrid = false;
|
|
private void c1AnnotationGrid_EnterCell(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingGrid) // Only set the Current Annotation when not loading the grid
|
|
{
|
|
if ((_Annotations != null) && (c1AnnotationGrid.Row > 0))
|
|
CurrentAnnotation = _Annotations[c1AnnotationGrid.Row - 1];
|
|
else
|
|
CurrentAnnotation = null;
|
|
}
|
|
//InitializeAnnotation();
|
|
}
|
|
private void InitializeAnnotation()
|
|
{
|
|
//vlnCSLAStackTrace.ShowStack("InitializeAnnotation - CurrentAnnotation = {0}", CurrentAnnotation);
|
|
_LoadingAnnotation = true;
|
|
if (CurrentAnnotation == null)
|
|
{
|
|
cbGridAnnoType.SelectedIndex = -1;
|
|
rtxbComment.Text = "";
|
|
}
|
|
else
|
|
{
|
|
cbGridAnnoType.SelectedValue = CurrentAnnotation.TypeID;
|
|
if (CurrentAnnotation.RtfText != "")
|
|
rtxbComment.Rtf = CurrentAnnotation.RtfText;
|
|
else
|
|
rtxbComment.Text = CurrentAnnotation.SearchText;
|
|
}
|
|
_LoadingAnnotation = false;
|
|
AnnotationDirty = false;
|
|
if (!_LoadingGrid)
|
|
{
|
|
rtxbComment.SelectionStart = rtxbComment.TextLength; // Position the cursor at the end of the current text
|
|
rtxbComment.Focus(); // Set the focus to the comment text
|
|
}
|
|
}
|
|
private bool _AnnotationDirty = false;
|
|
private bool AnnotationDirty
|
|
{
|
|
get { return _AnnotationDirty; }
|
|
set
|
|
{
|
|
btnRemoveAnnotation.Enabled = btnAddAnnotation.Enabled = !value;
|
|
btnSaveAnnotation.Enabled = btnCancelAnnoation.Enabled = value;
|
|
_AddingAnnotation = value && (CurrentAnnotation == null);
|
|
_AnnotationDirty = value;
|
|
}
|
|
}
|
|
|
|
private void rtxbComment_TextChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingAnnotation)
|
|
{
|
|
AnnotationDirty = true;
|
|
}
|
|
}
|
|
|
|
private void btnCancelAnnoation_Click(object sender, EventArgs e)
|
|
{
|
|
InitializeAnnotation();
|
|
}
|
|
|
|
private void cbGridAnnoType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingAnnotation)
|
|
{
|
|
AnnotationDirty = true;
|
|
}
|
|
|
|
}
|
|
|
|
private void btnSaveAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbGridAnnoType.SelectedIndex == -1)
|
|
{
|
|
MessageBox.Show("You Must Select an Annotation Type", "Annotation Type Not Selected", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
cbGridAnnoType.Focus();
|
|
return;
|
|
}
|
|
if (rtxbComment.Text == string.Empty)
|
|
{
|
|
MessageBox.Show("You Must Enter Annotation Text", "Annotation Text Is Blank", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
rtxbComment.Focus();
|
|
return;
|
|
}
|
|
SaveAnnotation();
|
|
}
|
|
|
|
private void btnRemoveAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
using (Annotation annotation = CurrentAnnotation.Get())
|
|
{
|
|
//ShowItemAnnotations("btnRemoveAnnotation_Click Start");
|
|
//annotation.DTS = DateTime.Now;
|
|
//annotation.Save();
|
|
//ShowItemAnnotations("btnRemoveAnnotation_Click After Change Save");
|
|
annotation.Delete();
|
|
_LoadingList = true;
|
|
annotation.Save();
|
|
_LoadingList = false;
|
|
CurrentAnnotation = null;
|
|
//ShowItemAnnotations("btnRemoveAnnotation_Click After Delete Save");
|
|
//c1AnnotationGrid.Row = -1;
|
|
//ShowItemAnnotations("btnRemoveAnnotation_Click Before Update Grid");
|
|
UpdateAnnotationGrid();
|
|
//ShowItemAnnotations("After UpdateAnnotationGrid After Update Grid");
|
|
UpdateAnnotationSearchResults();
|
|
}
|
|
}
|
|
|
|
}
|
|
}
|