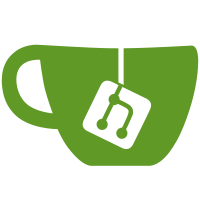
Added code to display message box when no procedures are found to be processed and terminates the processing. Utilized ChildrenProcessed property when processing procedures Added format changes to correct remaining Braidwood issues Added code to resolve unit information in section transition Modified page break logic to resolve issue with Braidwood deviation documents Added code to resolve page number when section is a word document section Added check to verify location was not already in list prior to adding it to list Reduced number of instances of Volian flexgrid during printing
148 lines
6.3 KiB
C#
148 lines
6.3 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Xml;
|
|
|
|
namespace fmtxml
|
|
{
|
|
public partial class FmtFileToXml
|
|
{
|
|
private void AddCWEfmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "475.2,252.2,144";
|
|
fmtdata.SectData.ReplaceStrData[3].Flag = "RNO, Caution, Note, Table, Substep, Attach";
|
|
fmtdata.SectData.ReplaceStrData[4].Flag = "RNO, Caution, Note, Table, Substep, Attach";
|
|
fmtdata.StepData[9].TabData.Ident = " {!C2}{numeric} ";
|
|
fmtdata.StepData[9].TabData.RNOIdent = " {!C2}{numeric} ";
|
|
fmtdata.BoxData[2].TabPos = 265F;
|
|
fmtdata.BoxData[2].Start = 128F;
|
|
fmtdata.BoxData[2].End = 420F;
|
|
fmtdata.BoxData[2].TxtStart = 151F;
|
|
fmtdata.BoxData[2].TxtWidth = 254F;
|
|
fmtdata.BoxData[1].TabPos = 265F;
|
|
fmtdata.BoxData[1].Start = 128F;
|
|
fmtdata.BoxData[1].End = 420F;
|
|
fmtdata.BoxData[1].TxtStart = 151F;
|
|
fmtdata.BoxData[1].TxtWidth = 254F;
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.RNOWidthAlt = "212,212";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.RNOWidthAdj = "-40";
|
|
fmtdata.StepData[6].StepLayoutData.EveryNLines = "-99";
|
|
fmtdata.StepData[7].StepLayoutData.EveryNLines = "-99";
|
|
}
|
|
private void AddCWE00fmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "489,229.2,144";
|
|
fmtdata.StepData[2].TabData.Ident = "{ALPHA}. ";
|
|
fmtdata.StepData[1].TabData.Ident = "{alpha}) ";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.BoxLeftAdj = "-43.2";
|
|
fmtdata.StepData[6].StepLayoutData.EveryNLines = "-99";
|
|
fmtdata.StepData[7].StepLayoutData.EveryNLines = "-99";
|
|
}
|
|
private void AddEXCLNfmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "475.2,229.2,144";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.ColRTable = "0,236.4,135.6";
|
|
fmtdata.SectData.ReplaceStrData[3].Flag = "RNO, Caution, Note, Table, Substep, Attach";
|
|
fmtdata.SectData.ReplaceStrData[4].Flag = "RNO, Caution, Note, Table, Substep, Attach";
|
|
fmtdata.StepData[9].TabData.Ident = " {!C2}{numeric} ";
|
|
fmtdata.StepData[9].TabData.RNOIdent = " {!C2}{numeric} ";
|
|
fmtdata.StepData[1].TabData.Ident = "{alpha}) ";
|
|
fmtdata.BoxData[2].TabPos = 265F;
|
|
fmtdata.BoxData[2].Start = 128F;
|
|
fmtdata.BoxData[2].End = 420F;
|
|
fmtdata.BoxData[2].TxtStart = 151F;
|
|
fmtdata.BoxData[2].TxtWidth = 254F;
|
|
fmtdata.BoxData[1].TabPos = 265F;
|
|
fmtdata.BoxData[1].Start = 128F;
|
|
fmtdata.BoxData[1].End = 420F;
|
|
fmtdata.BoxData[1].TxtStart = 151F;
|
|
fmtdata.BoxData[1].TxtWidth = 254F;
|
|
fmtdata.StepData[6].StepLayoutData.EveryNLines = "-99";
|
|
fmtdata.StepData[7].StepLayoutData.EveryNLines = "-99";
|
|
}
|
|
private void AddEXCLN00fmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "489,229.2,144";
|
|
fmtdata.StepData[2].TabData.Ident = "{ALPHA}. ";
|
|
fmtdata.StepData[1].TabData.Ident = "{alpha}) ";
|
|
}
|
|
private void AddEXCLN01fmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "489,229.2,144";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.BoxLeftAdj = "-43.2";
|
|
fmtdata.StepData[6].StepLayoutData.EveryNLines = "-99";
|
|
fmtdata.StepData[7].StepLayoutData.EveryNLines = "-99";
|
|
}
|
|
private void AddEXEDEVfmt(ref FormatData fmtdata)
|
|
{
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidSTablePrint = "471,180,120";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.RNOWidthAlt = "200,200";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.ColS = 66F;
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.WidT = 526F;
|
|
fmtdata.StepData[2].TabData.RNOIdent = "ERG STEP : ";
|
|
fmtdata.StepData[3].TabData.RNOIdent = "ERG NOTE : ";
|
|
fmtdata.StepData[6].TabData.RNOIdent = "ERG CAUTION : ";
|
|
fmtdata.StepData[7].TabData.RNOIdent = "ERG NOTE : ";
|
|
fmtdata.StepData[9].TabData.RNOIdent = "ERG CAUTION : ";
|
|
fmtdata.StepData[43].ColOverride = "42";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.UseRNOParentIdent = "True";
|
|
fmtdata.SectData.StepSectionData.StpSectLayData.DevNoteOrCautionTabOffset = "42";
|
|
}
|
|
}
|
|
public partial class FmtToXml
|
|
{
|
|
private void AddCWEfmt(ref PageStyles pgstyles)
|
|
{
|
|
pgstyles.PgStyles[0].Items[5].Col = 270F;
|
|
}
|
|
private void AddEXCLN00fmt(ref PageStyles pgstyles)
|
|
{
|
|
pgstyles.PgStyles[0].Items[4].Col = 446F;
|
|
pgstyles.PgStyles[0].Items[4].Row = 30F;
|
|
pgstyles.PgStyles[0].Items[5].Col = 230F;
|
|
}
|
|
private void AddEXCLN00Doc(ref DocStyles dcstyles)
|
|
{
|
|
dcstyles.DcStyles[0].PageWidth = 576.2F;
|
|
dcstyles.DcStyles[0].PageLength = 600F;
|
|
dcstyles.DcStyles[0].FooterLen = 24F;
|
|
}
|
|
private void AddCWEDoc(ref DocStyles dcstyles)
|
|
{
|
|
dcstyles.DcStyles[0].PageWidth = 580.2F;
|
|
dcstyles.DcStyles[1].LeftMargin = 48F;
|
|
dcstyles.DcStyles[1].PageWidth = 579.2F;
|
|
dcstyles.DcStyles[1].PageLength = 578F;
|
|
}
|
|
private void AddEXEDEVDoc(ref DocStyles dcstyles)
|
|
{
|
|
dcstyles.DcStyles[0].LeftMargin = 36F;
|
|
}
|
|
}
|
|
public partial class RtfToSvg
|
|
{
|
|
private void AddCWE(XmlDocument myDoc)
|
|
{
|
|
XmlDocument xdNew = new XmlDocument();
|
|
xdNew.LoadXml("<svg xmlns=\"http://www.w3.org/2000/svg\"> <g id=\"C2\">" +
|
|
"<line x1=\"7\" y1=\"-16\" x2=\"-5\" y2=\"-4\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"-5\" y1=\"-4\" x2=\"7\" y2=\"8\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"7\" y1=\"8\" x2=\"19\" y2=\"-4\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"19\" y1=\"-4\" x2=\"7\" y2=\"-16\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"</g></svg>");
|
|
myDoc.DocumentElement.AppendChild(myDoc.ImportNode(xdNew.DocumentElement.ChildNodes[0], true));
|
|
}
|
|
private void AddEXCLN(XmlDocument myDoc)
|
|
{
|
|
XmlDocument xdNew = new XmlDocument();
|
|
xdNew.LoadXml("<svg xmlns=\"http://www.w3.org/2000/svg\"> <g id=\"C2\">" +
|
|
"<line x1=\"7\" y1=\"-16\" x2=\"-5\" y2=\"-4\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"-5\" y1=\"-4\" x2=\"7\" y2=\"8\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"7\" y1=\"8\" x2=\"19\" y2=\"-4\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"<line x1=\"19\" y1=\"-4\" x2=\"7\" y2=\"-16\" stroke=\"black\" stroke-width=\"0.85\" />" +
|
|
"</g></svg>");
|
|
myDoc.DocumentElement.AppendChild(myDoc.ImportNode(xdNew.DocumentElement.ChildNodes[0], true));
|
|
}
|
|
}
|
|
}
|