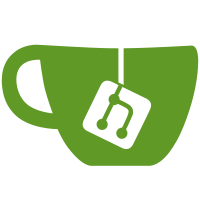
Use Temporary Folder for SearchResults Remove override of Equals - return to default Comment-out Debug Remove PDFs that are older than one hour Added a ShowLocalStack that takes a format and a list of parameters
64 lines
1.4 KiB
C#
64 lines
1.4 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.IO;
|
|
|
|
namespace Volian.Base.Library
|
|
{
|
|
public static class TmpFile
|
|
{
|
|
public static void RemoveAllTmps()
|
|
{
|
|
RemoveTmpPDFs();
|
|
RemoveTmpDocs();
|
|
RemoveTmps("*.png");
|
|
}
|
|
public static void RemoveTmpPDFs()
|
|
{
|
|
string tmpFolder = VlnSettings.TemporaryFolder;
|
|
DirectoryInfo di = new DirectoryInfo(tmpFolder);
|
|
if (!di.Exists) return;
|
|
foreach (FileInfo fi in di.GetFiles("*.pdf"))
|
|
{
|
|
try
|
|
{
|
|
// only delete pdfs if they are older than an hour - just to be
|
|
// safe. During creation of pdf's, separate pdfs are created for
|
|
// word sections and imported into the main resulting pdf.
|
|
if (fi.LastAccessTime.Ticks < (DateTime.Now.Ticks - TimeSpan.TicksPerHour))
|
|
fi.Delete();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
continue; // if an error, go onto next file.
|
|
}
|
|
}
|
|
|
|
}
|
|
public static void RemoveTmpDocs()
|
|
{
|
|
RemoveTmps("*.doc");
|
|
}
|
|
|
|
private static void RemoveTmps(string extension)
|
|
{
|
|
string tmpFolder = VlnSettings.TemporaryFolder;
|
|
DirectoryInfo di = new DirectoryInfo(tmpFolder);
|
|
if (!di.Exists) return;
|
|
foreach (FileInfo fi in di.GetFiles(extension))
|
|
{
|
|
try
|
|
{
|
|
// delete any docs. If it fails, just go on to the next one becase
|
|
// it may be open from another process.
|
|
fi.Delete();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
continue; // if an error, go onto next file.
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|