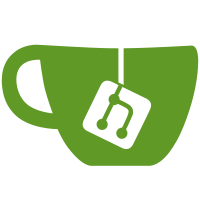
Use Temporary Folder for SearchResults Remove override of Equals - return to default Comment-out Debug Remove PDFs that are older than one hour Added a ShowLocalStack that takes a format and a list of parameters
719 lines
25 KiB
C#
719 lines
25 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class TransitionInfo
|
|
{
|
|
public string PathTo
|
|
{
|
|
get
|
|
{
|
|
return MyItemToID.Path;
|
|
}
|
|
}
|
|
public string PathFrom
|
|
{
|
|
get
|
|
{
|
|
return MyContent.ContentItems[0].Path;
|
|
}
|
|
}
|
|
public string ResolvePathTo(FormatInfo fi, ItemInfo itminfo, int tranType, ItemInfo toitem, ItemInfo rangeitem)
|
|
{
|
|
return TransitionText.GetResolvedText(fi, itminfo, tranType, toitem, rangeitem);
|
|
}
|
|
public string ResolvePathTo()
|
|
{
|
|
ItemInfo item = MyContent.ContentItems[0];
|
|
//Console.WriteLine("Format = {0}", item.ActiveFormat);
|
|
//Console.WriteLine("item = {0}", item.ItemID);
|
|
//Console.WriteLine("TranType = {0}", TranType);
|
|
//Console.WriteLine("MyItemToID = {0}", MyItemToID);
|
|
//Console.WriteLine("MyItemRangeID = {0}", MyItemRangeID);
|
|
return ResolvePathTo(item.ActiveFormat, item, TranType, MyItemToID, MyItemRangeID);
|
|
}
|
|
}
|
|
#region AffectedTransitons
|
|
public partial class TransitionInfoList
|
|
{
|
|
[Serializable()]
|
|
private class AffectedTransitonsCriteria
|
|
{
|
|
public AffectedTransitonsCriteria(int itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
public static TransitionInfoList GetAffected(int itemID)
|
|
{
|
|
try
|
|
{
|
|
TransitionInfoList tmp = DataPortal.Fetch<TransitionInfoList>(new AffectedTransitonsCriteria(itemID));
|
|
TransitionInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on TransitionInfoList.GetAffected", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(AffectedTransitonsCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] TransitionInfoList.DataPortal_FetchAffected", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getAffectedTransitions";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new TransitionInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("TransitionInfoList.DataPortal_FetchAffected", ex);
|
|
throw new DbCslaException("TransitionInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
public string Summarize()
|
|
{
|
|
StringBuilder sb = new StringBuilder("");
|
|
foreach (TransitionInfo trans in this)
|
|
sb.Append("\r\n" + trans.PathFrom);
|
|
return sb.ToString();
|
|
}
|
|
#endregion
|
|
#region ExternalTransitionsToChildren
|
|
private class ExternalTransitionsToChildrenCriteria
|
|
{
|
|
public ExternalTransitionsToChildrenCriteria(int itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
public static TransitionInfoList GetExternalTransitionsToChildren(int itemID)
|
|
{
|
|
try
|
|
{
|
|
TransitionInfoList tmp = DataPortal.Fetch<TransitionInfoList>(new ExternalTransitionsToChildrenCriteria(itemID));
|
|
TransitionInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on TransitionInfoList.GetExternalTransitionsToChildren", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ExternalTransitionsToChildrenCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] TransitionInfoList.DataPortal_FetchExternalTransitionsToChildren", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getExternalTransitionsToChildren";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new TransitionInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("TransitionInfoList.DataPortal_FetchExternalTransitionsToChildren", ex);
|
|
throw new DbCslaException("TransitionInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
#endregion
|
|
#region ExternalTransitions
|
|
private class ExternalTransitionsCriteria
|
|
{
|
|
public ExternalTransitionsCriteria(int itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
public static TransitionInfoList GetExternalTransitions(int itemID)
|
|
{
|
|
try
|
|
{
|
|
TransitionInfoList tmp = DataPortal.Fetch<TransitionInfoList>(new ExternalTransitionsCriteria(itemID));
|
|
TransitionInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on TransitionInfoList.GetExternalTransitions", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ExternalTransitionsCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] TransitionInfoList.DataPortal_FetchExternalTransitions", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getExternalTransitions";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new TransitionInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("TransitionInfoList.DataPortal_FetchExternalTransitions", ex);
|
|
throw new DbCslaException("TransitionInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
#endregion
|
|
#region PastedAffectedTransitions
|
|
private class PastedAffectedTransitonsCriteria
|
|
{
|
|
public PastedAffectedTransitonsCriteria(int itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
internal static TransitionInfoList GetPastedAffected(int itemID)
|
|
{
|
|
try
|
|
{
|
|
TransitionInfoList tmp = DataPortal.Fetch<TransitionInfoList>(new PastedAffectedTransitonsCriteria(itemID));
|
|
TransitionInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on TransitionInfoList.GetPastedAffected", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PastedAffectedTransitonsCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] TransitionInfoList.DataPortal_FetchPastedAffected", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getPastedAffectedTransitions";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read()) this.Add(new TransitionInfo(dr));
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("TransitionInfoList.DataPortal_FetchPastedAffected", ex);
|
|
throw new DbCslaException("TransitionInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
#endregion
|
|
}
|
|
public static class TransitionText
|
|
{
|
|
private delegate bool TransitionAppendFunction(bool textAdded, TransitionBuilder tb, string token, string nonToken);
|
|
private struct TransitionBuilder
|
|
{
|
|
public StringBuilder _Results;
|
|
public FormatData _FormatData;
|
|
public string _TransFormat;
|
|
public E_TransUI _TransUI;
|
|
public ItemInfo _FromItem;
|
|
public int _TranType;
|
|
public ItemInfo _ToItem;
|
|
public ItemInfo _RangeItem;
|
|
public bool _UsedRangeAncestor;
|
|
}
|
|
private static Dictionary<string, TransitionAppendFunction> _AppendMethods;
|
|
private static void SetupMethods()
|
|
{
|
|
_AppendMethods = new Dictionary<string, TransitionAppendFunction>();
|
|
_AppendMethods.Add("{Proc Num}", AddTransitionProcNum);
|
|
_AppendMethods.Add("{?.Proc Num}", AddOptionalTransitionProcNum);
|
|
_AppendMethods.Add("{Proc Title}", AddTransitionProcTitle);
|
|
_AppendMethods.Add("{?.Proc Title}", AddOptionalTransitionProcTitle);
|
|
_AppendMethods.Add("{First Step}", AddStepNumber);
|
|
_AppendMethods.Add("{Last Step}", AddRangeStepNumber);
|
|
_AppendMethods.Add("{.}", AddIncludedStepNumber);
|
|
_AppendMethods.Add("{Sect Hdr}", AddTranGetSectionHdr);
|
|
_AppendMethods.Add("{?.Sect Hdr}", AddOptionalTranGetSectionHdr);
|
|
_AppendMethods.Add("{Sect Title}", AddTranGetSectionHdr);
|
|
_AppendMethods.Add("{?.Sect Title}", AddOptionalTranGetSectionHdr);
|
|
_AppendMethods.Add("{Sect Num}", AddTranGetSectionNumber);
|
|
}
|
|
public static string GetResolvedText(ItemInfo fromInfo, int tranType, ItemInfo toItem, ItemInfo rangeItem)
|
|
{
|
|
return GetResolvedText(fromInfo.ActiveFormat, fromInfo, tranType, toItem, rangeItem);
|
|
}
|
|
public static string GetResolvedText(FormatInfo formatInfo, ItemInfo fromInfo, int tranType, ItemInfo toItem, ItemInfo rangeItem)
|
|
{
|
|
TransitionBuilder tb = SetupTransitionBuilder(formatInfo, fromInfo, tranType, toItem,
|
|
toItem.ItemID==rangeItem.ItemID && !toItem.IsHigh?toItem.LastSibling:rangeItem);
|
|
if(_AppendMethods==null)
|
|
SetupMethods();
|
|
return BuildString(tb);
|
|
}
|
|
private static TransitionBuilder SetupTransitionBuilder(FormatInfo formatInfo, ItemInfo fromInfo, int tranType, ItemInfo toItem, ItemInfo rangeItem)
|
|
{
|
|
TransitionBuilder tb = new TransitionBuilder();
|
|
tb._Results = new StringBuilder();
|
|
tb._FormatData = formatInfo.PlantFormat.FormatData;
|
|
// get the format of the transition string based on this transition's index into the TransData part of
|
|
// format....
|
|
if (tranType > tb._FormatData.TransData.TransTypeList.Count)
|
|
tranType = 0;
|
|
// Replace 3 tokens ", {.}, {.}, {.}" with a single token "{.}"
|
|
tb._TransFormat = tb._FormatData.TransData.TransTypeList[tranType].TransFormat.Replace(", {.}, {.}, {.}", "{.}");
|
|
tb._TransUI = (E_TransUI)tb._FormatData.TransData.TransTypeList[tranType].TransUI;
|
|
tb._FromItem = fromInfo;
|
|
tb._TranType = tranType;
|
|
tb._ToItem = toItem;
|
|
tb._RangeItem = rangeItem;
|
|
return tb;
|
|
}
|
|
private static string BuildString(TransitionBuilder tb)
|
|
{
|
|
int startIndex = 0;
|
|
int index = -1;
|
|
string nonToken = null;
|
|
bool textAdded = false;
|
|
while ((index = tb._TransFormat.IndexOf("{", startIndex)) > -1)
|
|
{
|
|
if (index > startIndex) nonToken = tb._TransFormat.Substring(startIndex, index - startIndex);
|
|
if (startIndex == 0 && nonToken != null && nonToken.Length > 0)
|
|
{
|
|
tb._Results.Append(nonToken);
|
|
//textAdded = true;
|
|
nonToken = "";
|
|
}
|
|
int endtokn = tb._TransFormat.IndexOf("}", index);
|
|
string token = tb._TransFormat.Substring(index, endtokn - index + 1);
|
|
if (token == "{.}" && tb._ToItem.ItemID == tb._RangeItem.ItemID)
|
|
{
|
|
startIndex = tb._TransFormat.Length; // skip the rest of the trans format
|
|
break;
|
|
}
|
|
if (_AppendMethods.ContainsKey(token))
|
|
textAdded = _AppendMethods[token](textAdded, tb, token, nonToken);
|
|
else
|
|
tb._Results.Append("\\"+token.Substring(0,token.Length -1)+"\\}");
|
|
startIndex = endtokn + 1;
|
|
if (startIndex >= tb._TransFormat.Length) break;
|
|
}
|
|
if (startIndex < tb._TransFormat.Length) Append(tb, tb._TransFormat.Substring(startIndex, tb._TransFormat.Length - startIndex - 1), false);
|
|
return (tb._Results.ToString());
|
|
}
|
|
private static void Append(TransitionBuilder tb, string str, bool doflags)
|
|
{
|
|
if (str == null || str == "") return;
|
|
if (doflags && tb._FormatData.TransData.XchngTranSpForHard)
|
|
{
|
|
int indx = str.IndexOf(' ');
|
|
if (indx > -1)
|
|
{
|
|
str.Remove(indx, 1);
|
|
str.Insert(indx, @"\u160?");
|
|
}
|
|
}
|
|
tb._Results.Append(str);
|
|
}
|
|
// TODO: TStepNoFlag, i.e. include step number in range items.
|
|
// TODO: TStepNoFlag, i.e. include step number in range items.
|
|
// TODO: For hlp: LowerCaseTranNumber - lower case substep numbers in transitions
|
|
private static bool AddTransitionProcNum(bool textAdded, TransitionBuilder tb, string token, string nonToken) // Coded for HLP
|
|
{
|
|
string retstr = tb._ToItem.MyProcedure.MyContent.Number;
|
|
// LATER: start with UnitSpecific procedure number.
|
|
// LATER: Format Flag TruncateProcNmAfter1stSpace (dropped plants)
|
|
// LATER: Format Flag HardSpTranProcNumb (active plants)
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
Append(tb, retstr, true);
|
|
if (retstr != null && retstr != "") return true;
|
|
return false;
|
|
}
|
|
private static bool AddOptionalTransitionProcNum(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// token is passed in for future use, i.e. if text is placed between "?" and "." to
|
|
// request other processing.
|
|
|
|
// for now the only test is to check if the toitem is in the current procedure.
|
|
if (tb._FromItem.MyProcedure.ItemID == tb._ToItem.MyProcedure.ItemID) return textAdded ? true : false;
|
|
return AddTransitionProcNum(textAdded, tb, token, nonToken);
|
|
}
|
|
|
|
private static bool AddTransitionProcTitle(bool textAdded, TransitionBuilder tb, string token, string nonToken)// Coded for HLP
|
|
{
|
|
if (tb._ToItem.PreviousID == null && tb._ToItem.ItemPartCount == 0 && tb._ToItem.ItemDocVersionCount == 0)
|
|
{
|
|
Append(tb, "?", true);
|
|
return true;
|
|
}
|
|
string parenstr = tb._ToItem.MyProcedure.MyContent.Text;
|
|
StringBuilder lretstr = new StringBuilder();
|
|
// LATER: For an else - Do I need to strip underlining here? See promsnt\lib\edit\gettran.c
|
|
if (parenstr != "<NO TITLE>" || tb._FormatData.ProcData.PrintNoTitle)
|
|
{
|
|
lretstr.Append(tb._FormatData.TransData.DelimiterForTransitionTitle);
|
|
lretstr.Append(tb._FormatData.TransData.CapsTransitions ? parenstr.ToUpper().Replace(@"\U", @"\u") :
|
|
tb._FormatData.TransData.Cap1stCharTrans ? CapFirstLetterOnly(parenstr, 0) :
|
|
parenstr);
|
|
lretstr.Append(tb._FormatData.TransData.DelimiterForTransitionTitle);
|
|
}
|
|
// LATER: if (DoSectionTransitions && GetSTepNO(TSeq1)) TransitionCat(AddCommaStep", Step"));
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
Append(tb, lretstr.ToString(), true);
|
|
if (lretstr.Length != 0) return true;
|
|
return false;
|
|
}
|
|
private static bool AddOptionalTransitionProcTitle(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// token is passed in for future use, i.e. if text is placed between "?" and "." to
|
|
// request other processing.
|
|
|
|
// for now the only test is to check if the toitem is in the current procedure.
|
|
if (tb._FromItem.MyProcedure.ItemID == tb._ToItem.MyProcedure.ItemID) return textAdded ? true : false;
|
|
return AddTransitionProcTitle(textAdded, tb, token, nonToken);
|
|
}
|
|
private static string Tab(ItemInfo item)
|
|
{
|
|
if (item == null) return "";
|
|
string sret = "";
|
|
switch (item.MyContent.Type / 10000)
|
|
{
|
|
case 0: //procedure
|
|
sret = ProcedureInfo.Get(item.ItemID).MyTab.CleanText;
|
|
break;
|
|
case 1: // section
|
|
sret = SectionInfo.Get(item.ItemID).MyTab.CleanText;
|
|
break;
|
|
case 2: // step
|
|
|
|
ItemInfo pitem = item;
|
|
while (!pitem.IsHigh)
|
|
{
|
|
string thisTab = StepInfo.Get(pitem.ItemID).MyTab.CleanText;
|
|
if (pitem.IsRNOPart)
|
|
{
|
|
//string mytb = sret.Trim(" .)".ToCharArray());
|
|
if (thisTab == null || thisTab == "")
|
|
sret = "RNO." + sret;
|
|
else
|
|
{
|
|
thisTab = thisTab.Trim(" ".ToCharArray());
|
|
sret = "RNO." + thisTab + sret;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
//sret = Tab(item.ActiveParent as ItemInfo) + "." + sret.Trim(" .)".ToCharArray()); //original
|
|
if (thisTab != null && thisTab != "")
|
|
{
|
|
thisTab = thisTab.Trim(" ".ToCharArray());
|
|
if (!thisTab.EndsWith(".") && !thisTab.EndsWith(")")) thisTab = thisTab + ".";
|
|
}
|
|
sret = thisTab + sret;
|
|
}
|
|
pitem = pitem.ActiveParent as ItemInfo;
|
|
if (pitem == null) break;
|
|
}
|
|
// add hls tab.
|
|
if (pitem.IsHigh)
|
|
{
|
|
string hlsTab = StepInfo.Get(pitem.ItemID).MyTab.CleanText;
|
|
hlsTab = hlsTab.Trim(" ".ToCharArray());
|
|
if (!hlsTab.EndsWith(".") && !hlsTab.EndsWith(")")) hlsTab = hlsTab + ".";
|
|
sret = hlsTab + sret;
|
|
}
|
|
break;
|
|
}
|
|
sret = sret.Trim(" .)".ToCharArray());
|
|
return sret;
|
|
}
|
|
private static string RhmTab(ItemInfo item)
|
|
{
|
|
if (item == null) return "";
|
|
//if (item.ItemID == 2065) Console.WriteLine("here");
|
|
string sret = "";
|
|
switch (item.MyContent.Type / 10000)
|
|
{
|
|
case 0: //procedure
|
|
sret = ProcedureInfo.Get(item.ItemID).MyTab.CleanText;
|
|
break;
|
|
case 1: // section
|
|
sret = SectionInfo.Get(item.ItemID).MyTab.CleanText;
|
|
break;
|
|
case 2: // step
|
|
sret = StepInfo.Get(item.ItemID).MyTab.CleanText;
|
|
if (!item.IsHigh)
|
|
{
|
|
if (item.IsRNOPart)
|
|
{
|
|
string mytb = sret.Trim(" .)".ToCharArray());
|
|
if (mytb == null || mytb == "")
|
|
sret = Tab(item.ActiveParent as ItemInfo) + ".RNO";
|
|
else
|
|
sret = Tab(item.ActiveParent as ItemInfo) + ".RNO." + sret.Trim(" .)".ToCharArray());
|
|
}
|
|
else
|
|
{
|
|
//sret = Tab(item.ActiveParent as ItemInfo) + "." + sret.Trim(" .)".ToCharArray()); //original
|
|
string tmp1 = sret.Contains(@")") ? ")" : ".";
|
|
string tmp2 = sret.Trim(" .)".ToCharArray());
|
|
string srettmp = Tab(item.ActiveParent as ItemInfo);
|
|
sret = srettmp + sret.Trim(" ".ToCharArray());
|
|
if (!sret.EndsWith(".") && !sret.EndsWith(")")) sret = sret + ".";
|
|
}
|
|
}
|
|
else
|
|
{
|
|
sret = sret.Trim(" ".ToCharArray());
|
|
if (!sret.EndsWith(".") && !sret.EndsWith(")")) sret = sret + ".";
|
|
}
|
|
break;
|
|
}
|
|
//sret = sret.Trim(" .)".ToCharArray()); // original
|
|
return sret;
|
|
}
|
|
private static bool AddStepNumber(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// If we're on a step put out the step number.
|
|
ItemInfo secitm = TranGetSectionItem(tb._ToItem);
|
|
if ((!((tb._TransUI & E_TransUI.StepAllowNone) == E_TransUI.StepAllowNone)) || tb._ToItem.MyContent.Type > 20000)
|
|
{
|
|
if (textAdded)
|
|
{
|
|
// check for transition that goes to a procedure step section with title of 'Procedure steps' followed
|
|
// by ', Step'. This should output as 'Procedure step xyz' rather than 'Procedure steps, step xyz'
|
|
if (!tb._TransFormat.Contains("{Last Step}") && nonToken.ToUpper().Contains(", STEP") && tb._Results.ToString().ToUpper().EndsWith("PROCEDURE STEPS"))
|
|
{
|
|
tb._Results = tb._Results.Remove(tb._Results.Length-15,15); // remove "procedure steps"
|
|
tb._Results = tb._Results.Append("procedure Step");
|
|
nonToken = " ";
|
|
}
|
|
if (nonToken!=null)Append(tb, nonToken, false);
|
|
}
|
|
//Console.WriteLine("NEW - ItemID={0},Ordinal={1}", tb._ToItem.ItemID, tb._ToItem.Ordinal);
|
|
if (tb._ToItem.PreviousID == null && tb._ToItem.ItemPartCount == 0 && tb._ToItem.ItemDocVersionCount == 0)
|
|
Append(tb, "?", true);
|
|
else
|
|
Append(tb, Tab(tb._ToItem), true);
|
|
//Append(tb, tb._ToItem.Ordinal.ToString(), true);
|
|
//Append(tb, tb._ToItem.MyTab.CleanText, true);
|
|
textAdded = true;
|
|
}
|
|
return textAdded;
|
|
}
|
|
private static bool AddRangeStepNumber(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
if (tb._RangeItem.PreviousID == null && tb._RangeItem.ItemPartCount == 0 && tb._RangeItem.ItemDocVersionCount == 0)
|
|
Append(tb, "?", true);
|
|
else
|
|
Append(tb, Tab(tb._RangeItem), true);
|
|
//Append(tb, tb._RangeItem.Ordinal.ToString(), true);
|
|
//Append(tb, tb._RangeItem.MyTab.CleanText, true);
|
|
textAdded = true;
|
|
return textAdded;
|
|
}
|
|
private static bool AddIncludedStepNumber(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
Dictionary<int, ItemInfo> rangeAncestors = GetAncestors(tb._RangeItem);
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
bool usedRangeAncestor = false;
|
|
ItemInfo next = GetNextItem(tb._ToItem, rangeAncestors, ref usedRangeAncestor);
|
|
while (next.ItemID != tb._RangeItem.ItemID)
|
|
{
|
|
Append(tb, ", " + Tab(next), true); // TODO: Intermediate Range.
|
|
next = GetNextItem(next, rangeAncestors, ref usedRangeAncestor);
|
|
}
|
|
textAdded = true;
|
|
tb._UsedRangeAncestor = usedRangeAncestor;
|
|
return textAdded;
|
|
}
|
|
private static Dictionary<int, ItemInfo> GetAncestors(ItemInfo itemInfo)
|
|
{
|
|
Dictionary<int, ItemInfo> retval = new Dictionary<int,ItemInfo>();
|
|
while (!itemInfo.IsHigh)
|
|
{
|
|
ItemInfo parent = itemInfo.MyActiveParent as ItemInfo;
|
|
retval.Add(parent.ItemID, itemInfo.FirstSibling);
|
|
itemInfo = parent;
|
|
}
|
|
return retval;
|
|
}
|
|
private static ItemInfo GetNextItem(ItemInfo next, Dictionary<int, ItemInfo> rangeAncestors, ref bool usedRangeAncestor)
|
|
{
|
|
if (rangeAncestors.ContainsKey(next.ItemID))
|
|
return rangeAncestors[next.ItemID];
|
|
while (next.NextItem == null)
|
|
next = next.ActiveParent as ItemInfo;
|
|
return next.NextItem;
|
|
}
|
|
private static bool AddOptionalTranGetSectionHdr(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// output from 16-bit code looks like section header
|
|
// token is passed in for future use, i.e. if text is placed between "?" and "." to
|
|
// request other processing.
|
|
|
|
// If in same procedure, the section header is put out if not in the same section.
|
|
// If in a different procedure, the section header is put out if the 'to' item is not the
|
|
// default (or start) section.
|
|
if (tb._FromItem.MyProcedure.ItemID == tb._ToItem.MyProcedure.ItemID)
|
|
{
|
|
if (TranGetSectionItem(tb._FromItem).ItemID == TranGetSectionItem(tb._ToItem).ItemID) return textAdded ? true : false;
|
|
}
|
|
else if (TranGetSectionItem(tb._ToItem).IsDefaultSection) return textAdded ? true : false;
|
|
return AddTranGetSectionHdr(textAdded, tb, token, nonToken);
|
|
}
|
|
private static bool AddTranGetSectionHdr(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// output from 16-bit code looks like section header
|
|
// if this is a step section & the default section, just return
|
|
//if (TranGetSectionItem(itminfo).IsDefaultSection) return;
|
|
StringBuilder retstr = new StringBuilder();
|
|
retstr.Append(TranGetSectionNumber(tb._ToItem));
|
|
string txt = TranGetSectionTitle(tb,tb._ToItem);
|
|
if (retstr.Length > 0 && txt.Length > 0) retstr.Append(", ");
|
|
retstr.Append(txt);
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
Append(tb, retstr.ToString(), true);
|
|
if (retstr.Length != 0) return true;
|
|
return false;
|
|
}
|
|
private static bool AddOptionalTranGetSectionTitle(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// token is passed in for future use, i.e. if text is placed between "?" and "." to
|
|
// request other processing.
|
|
|
|
// for now the only test is to check if the toitem is in the current section.
|
|
if (TranGetSectionItem(tb._FromItem).ItemID == TranGetSectionItem(tb._ToItem).ItemID) return textAdded ? true : false;
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
string retstr = TranGetSectionTitle(tb,tb._ToItem);
|
|
Append(tb, retstr, true);
|
|
if (retstr != null && retstr != "") return true;
|
|
return false;
|
|
}
|
|
private static bool AddTranGetSectionTitle(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
// LATER: Cap1stSectionTitle, CapFirstLetterOnly
|
|
if (tb._FormatData.TransData.UseSecTitles)
|
|
{
|
|
string retstr = TranGetSectionTitle(tb,tb._FromItem);
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
Append(tb, retstr, true);
|
|
if (retstr != null && retstr != "") return true;
|
|
}
|
|
return false;
|
|
}
|
|
private static bool AddTranGetSectionNumber(bool textAdded, TransitionBuilder tb, string token, string nonToken)
|
|
{
|
|
string retstr = TranGetSectionNumber(tb._ToItem);
|
|
if (textAdded) Append(tb, nonToken, false);
|
|
Append(tb, retstr, true);
|
|
if (retstr != null && retstr != "") return true;
|
|
return false;
|
|
}
|
|
private static string CapFirstLetterOnly(string retstr, int p)
|
|
{
|
|
string lretstr = retstr;
|
|
// LATER: write code to capitalize first letter (active plants)
|
|
return lretstr;
|
|
}
|
|
// TODO: Section methods are not complete....
|
|
private static ItemInfo TranGetSectionItem(ItemInfo itminfo)
|
|
{
|
|
ItemInfo tmpitm = itminfo;
|
|
while (tmpitm.MyContent.Type >= 20000) tmpitm = tmpitm.MyParent;
|
|
return tmpitm;
|
|
}
|
|
private static string TranGetSectionNumber(ItemInfo itminfo)
|
|
{
|
|
ItemInfo tmpitm = TranGetSectionItem(itminfo);
|
|
return (tmpitm.MyContent.Number);
|
|
}
|
|
private static string TranGetSectionTitle(TransitionBuilder tb, ItemInfo itminfo)
|
|
{
|
|
// LATER: Cap1stSectionTitle, CapFirstLetterOnly
|
|
if (tb._FormatData.TransData.UseSecTitles)
|
|
{
|
|
ItemInfo tmpitm = TranGetSectionItem(itminfo);
|
|
return (tmpitm.MyContent.Text);
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
}
|