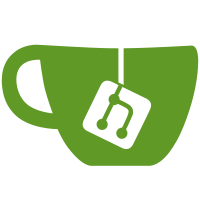
If WalkProcedure or Update History is called with a null, reset the contents and return Fix the section title
1377 lines
39 KiB
C#
1377 lines
39 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using iTextSharp.text.pdf;
|
|
using iTextSharp.text;
|
|
using System.IO;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace Volian.Print.Library
|
|
{
|
|
public class PDFChronologyReport
|
|
{
|
|
private string _FileName;
|
|
public string FileName
|
|
{
|
|
get { return _FileName; }
|
|
set { _FileName = value; }
|
|
}
|
|
private ProcedureInfo _MyProc;
|
|
public ProcedureInfo MyProc
|
|
{
|
|
get { return _MyProc; }
|
|
set { _MyProc = value; }
|
|
}
|
|
private ContentAuditInfoList _AuditList;
|
|
public ContentAuditInfoList AuditList
|
|
{
|
|
get { return _AuditList; }
|
|
set { _AuditList = value; }
|
|
}
|
|
private AnnotationAuditInfoList _AnnotationList;
|
|
public AnnotationAuditInfoList AnnotationList
|
|
{
|
|
get { return _AnnotationList; }
|
|
set { _AnnotationList = value; }
|
|
}
|
|
public PDFChronologyReport(string fileName, ProcedureInfo myProc, ContentAuditInfoList auditList, AnnotationAuditInfoList annotationList)
|
|
{
|
|
_FileName = fileName;
|
|
_MyProc = myProc;
|
|
_AuditList = auditList;
|
|
_AnnotationList = annotationList;
|
|
}
|
|
public void BuildChronology()
|
|
{
|
|
iTextSharp.text.Document document = new iTextSharp.text.Document(PageSize.LETTER, 36, 36, 36, 36);
|
|
if (!CreateResultsPDF(document)) return;
|
|
try
|
|
{
|
|
BuildChronologyReport(document);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
StringBuilder msg = new StringBuilder();
|
|
document.Add(new Paragraph("Error:"));
|
|
while (ex != null)
|
|
{
|
|
document.Add(new Paragraph(ex.GetType().Name));
|
|
document.Add(new Paragraph(ex.Message));
|
|
ex = ex.InnerException;
|
|
}
|
|
}
|
|
finally
|
|
{
|
|
if (document.IsOpen())
|
|
{
|
|
document.Close();
|
|
System.Diagnostics.Process.Start(_FileName);
|
|
}
|
|
}
|
|
}
|
|
public void BuildSummary()
|
|
{
|
|
bool hasData = false;
|
|
iTextSharp.text.Document document = new iTextSharp.text.Document(PageSize.LETTER, 36, 36, 36, 36);
|
|
if (!CreateResultsPDF(document)) return;
|
|
try
|
|
{
|
|
BuildSummaryReport(document);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
StringBuilder msg = new StringBuilder();
|
|
document.Add(new Paragraph("Error:"));
|
|
while (ex != null)
|
|
{
|
|
document.Add(new Paragraph(ex.GetType().Name));
|
|
document.Add(new Paragraph(ex.Message));
|
|
ex = ex.InnerException;
|
|
}
|
|
}
|
|
finally
|
|
{
|
|
if (document.IsOpen())
|
|
{
|
|
document.Close();
|
|
System.Diagnostics.Process.Start(_FileName);
|
|
}
|
|
}
|
|
}
|
|
private bool CreateResultsPDF(iTextSharp.text.Document document)
|
|
{
|
|
bool result = false;
|
|
string suffix = "";
|
|
int i = 0;
|
|
// Try to open a file for creating the PDF.
|
|
while (result == false)
|
|
{
|
|
string fileName = _FileName.ToLower().Replace(".pdf", suffix + ".pdf");
|
|
try
|
|
{
|
|
PdfWriter writer = PdfWriter.GetInstance(document, new FileStream(fileName, FileMode.Create));
|
|
document.SetMargins(36, 36, 36, 36);
|
|
document.Open();
|
|
_FileName = fileName;
|
|
result = true;
|
|
}
|
|
catch (System.IO.IOException exIO)
|
|
{
|
|
|
|
if (exIO.Message.Contains("because it is being used by another process"))
|
|
suffix = string.Format("_{0}", ++i);// If this file is in use, increment the suffix and try again
|
|
else // If some other error, display a message and don't print the results
|
|
{
|
|
ShowException(exIO);
|
|
return false;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
ShowException(ex);
|
|
return false; // Could not open the output file
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
private static void ShowException(Exception ex)
|
|
{
|
|
Console.WriteLine("{0} - {1}", ex.GetType().Name, ex.Message);
|
|
StringBuilder msg = new StringBuilder();
|
|
string sep = "";
|
|
string indent = "";
|
|
while (ex != null)
|
|
{
|
|
msg.Append(string.Format("{0}{1}{2}:\r\n{1}{3}", sep, indent, ex.GetType().Name, ex.Message));
|
|
ex = ex.InnerException;
|
|
sep = "\r\n";
|
|
indent += " ";
|
|
}
|
|
System.Windows.Forms.MessageBox.Show(msg.ToString(), "Error during PDF creation for search:", System.Windows.Forms.MessageBoxButtons.OK, System.Windows.Forms.MessageBoxIcon.Exclamation);
|
|
}
|
|
private PdfPCell BlankCell(int colSpan)
|
|
{
|
|
PdfPCell c = new PdfPCell();
|
|
c.Colspan = colSpan;
|
|
c.Border = 0;
|
|
return c;
|
|
}
|
|
private void BuildChronologyReport(iTextSharp.text.Document doc)
|
|
{
|
|
int cols = 5;
|
|
int borders = 0;
|
|
int paddingBottom = 6;
|
|
PdfPTable t = new PdfPTable(cols);
|
|
t.HeaderRows = 4;
|
|
t.DefaultCell.Padding = 4;
|
|
t.WidthPercentage = 100;
|
|
float[] widths = new float[] { 1f, 1f, 1f, 1f, 4f };
|
|
t.SetWidths(widths);
|
|
//t.HorizontalAlignment = 0;
|
|
iTextSharp.text.Font f1 = pdf.GetFont("Arial Unicode MS", 14, 1, Color.BLACK);
|
|
iTextSharp.text.Font f2 = pdf.GetFont("Arial Unicode MS", 10, 0, Color.BLACK);
|
|
iTextSharp.text.Font f3 = pdf.GetFont("Arial Unicode MS", 9, 0, Color.BLACK);
|
|
iTextSharp.text.Font f4 = pdf.GetFont("Arial Unicode MS", 9, 1, Color.BLACK);
|
|
//report title
|
|
Phrase h = new Phrase();
|
|
h.Font = f1;
|
|
h.Add("Chronology of Changes Report");
|
|
PdfPCell c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
//procedure title
|
|
h = new Phrase();
|
|
h.Font = f2;
|
|
h.Add(string.Format("{0} - {1}", MyProc.DisplayNumber, MyProc.DisplayText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
string section = string.Empty;
|
|
string stepnum = string.Empty;
|
|
DateTime maxDTS = DateTime.MinValue;
|
|
int lastID = 0;
|
|
ContentAuditInfo oldCAI = null;
|
|
// Dictionary<long, AnnotationAuditInfo> processedAAI = new Dictionary<long, AnnotationAuditInfo>();
|
|
Dictionary<AnnotationAuditInfo, AnnotationAuditInfo> processedAAI = new Dictionary<AnnotationAuditInfo, AnnotationAuditInfo>();
|
|
foreach (ContentAuditInfo cai in AuditList)
|
|
{
|
|
//raw path
|
|
string[] NewPath = SplitPath(cai.Path);
|
|
if (NewPath[0] != section)
|
|
{
|
|
section = NewPath[0];
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add(section);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
}
|
|
//step
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
//see if any annotations for old stepnum here and add them
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
if (oldCAI != null && aai.IContentID == oldCAI.ContentID)
|
|
{
|
|
//add annotation to minilist
|
|
// processedAAI.Add(aai.AnnotationID, aai);
|
|
processedAAI.Add(aai, aai);
|
|
//write row to table
|
|
t.AddCell(BlankCell(1));
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add("Annotation " + aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
oldCAI = cai;
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
else
|
|
t.AddCell(BlankCell(1));
|
|
//what
|
|
if (cai.ContentID != lastID) maxDTS = DateTime.MinValue;
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
string actionWhat = (cai.ActionWhat == "Added" && cai.DTS <= MyProc.DTS) ? "Original" : cai.ActionWhat != "Changed" ? cai.ActionWhat : cai.DTS <= maxDTS ? "Restored" : cai.DTS > MyProc.DTS ? cai.ActionWhat : "Original";
|
|
if (actionWhat == "Deleted" || actionWhat == "Restored")
|
|
h.Add(actionWhat + "\r\n" + cai.ActionWhen.ToString());
|
|
else
|
|
h.Add(actionWhat);
|
|
if (cai.DTS > maxDTS)
|
|
maxDTS = cai.DTS;
|
|
lastID = cai.ContentID;
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.DTS.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
//h.Add(cai.Text);
|
|
if (cai.Type == 20008)
|
|
{
|
|
GridAuditInfoList gail = GridAuditInfoList.Get(cai.ContentID);
|
|
if (gail.Count > 0)
|
|
{
|
|
GridAuditInfo gai = gail[0];
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.LoadXml(gai.Data);
|
|
//int grows = int.Parse(xd.SelectSingleNode("C1FlexGrid/RowInfo/Count").InnerText);
|
|
int gcols = int.Parse(xd.SelectSingleNode("C1FlexGrid/ColumnInfo/Count").InnerText);
|
|
string data = ItemInfo.ConvertToDisplayText(cai.Text);
|
|
PdfPTable gtbl = new PdfPTable(gcols);
|
|
while (data.Length > 0)
|
|
{
|
|
string val = data.Substring(0, data.IndexOf(";"));
|
|
data = data.Substring(data.IndexOf(";") + 1);
|
|
Phrase hh = new Phrase();
|
|
hh.Font = f4;
|
|
hh.Add(val);
|
|
PdfPCell cc = new PdfPCell(hh);
|
|
gtbl.AddCell(cc);
|
|
}
|
|
c = new PdfPCell(gtbl);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
//c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add(ItemInfo.ConvertToDisplayText(cai.Text));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
////old text
|
|
//t.AddCell(BlankCell(3));
|
|
//h = new Phrase();
|
|
//h.Font = f4;
|
|
//h.Add("Old text: " + cai.OldText);
|
|
//c = new PdfPCell(h);
|
|
//c.Colspan = 7;
|
|
//c.Border = 0;
|
|
//t.AddCell(c);
|
|
}
|
|
//see if any annotations for old stepnum here and add them
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
if (oldCAI != null && aai.IContentID == oldCAI.ContentID)
|
|
{
|
|
//add annotation to minilist
|
|
// processedAAI.Add(aai.AnnotationID, aai);
|
|
processedAAI.Add(aai, aai);
|
|
//write row to table
|
|
t.AddCell(BlankCell(1));
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add("Annotation " + aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
bool annotationHeader = false;
|
|
stepnum = string.Empty;
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
// if (!processedAAI.ContainsKey(aai.AnnotationID))
|
|
if (!processedAAI.ContainsKey(aai))
|
|
{
|
|
if (!annotationHeader)
|
|
{
|
|
t.AddCell(BlankCell(cols));
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add("ANNOTATIONS");
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
annotationHeader = true;
|
|
}
|
|
string[] NewPath = GetNewPath(AuditList, aai.IContentID);
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
else
|
|
{
|
|
t.AddCell(BlankCell(1));
|
|
}
|
|
//what
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
if (t.Rows.Count == t.HeaderRows)
|
|
{
|
|
c = new PdfPCell(new Phrase("No changes to report"));
|
|
c.Colspan = cols;
|
|
t.AddCell(c);
|
|
}
|
|
doc.Add(t);
|
|
}
|
|
private static string[] SplitPath(string path)
|
|
{
|
|
// Remove Procedure Number and Title
|
|
string sectionAndStep = Regex.Replace(path + "\x7\x7\x7", "^.+?\\u0007", "");
|
|
sectionAndStep = sectionAndStep.Replace("\x11", sectionAndStep[0] == '\x11' ? "" : " - ");
|
|
// Split Section frrom Steps
|
|
return sectionAndStep.Split("\x7".ToCharArray());
|
|
}
|
|
|
|
private string[] GetNewPath(ContentAuditInfoList AuditList, int contentID)
|
|
{
|
|
foreach (ContentAuditInfo cai in AuditList)
|
|
{
|
|
if(cai.ContentID == contentID)
|
|
return SplitPath(cai.Path);
|
|
}
|
|
ItemInfoList iil = ItemInfoList.GetByContentID(contentID);
|
|
if (iil.Count > 0)
|
|
{
|
|
return SplitPath(iil[0].SearchPath);
|
|
}
|
|
return "Section\x7Step".Split("\x7".ToCharArray());
|
|
}
|
|
private void BuildSummaryReport2(iTextSharp.text.Document doc)
|
|
{
|
|
string section = string.Empty;
|
|
string stepnum = string.Empty;
|
|
ContentAuditInfo firstCAI = null;
|
|
ContentAuditInfo nextCAI = null;
|
|
ContentAuditInfo lastCAI = null;
|
|
List<ContentAuditInfo> auditList = new List<ContentAuditInfo>();
|
|
foreach (ContentAuditInfo cai in AuditList)
|
|
{
|
|
string[] NewPath = SplitPath(cai.Path);
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
stepnum = NewPath[1];
|
|
if (firstCAI != null)
|
|
{
|
|
if (lastCAI == null)
|
|
{
|
|
auditList.Add(firstCAI);
|
|
}
|
|
else if (lastCAI.ActionWhat == "Deleted" && firstCAI.DTS < MyProc.DTS)
|
|
{
|
|
auditList.Add(lastCAI);
|
|
}
|
|
else if (lastCAI.ActionWhat == "Changed" && lastCAI.Text != firstCAI.Text)
|
|
{
|
|
auditList.Add(firstCAI);
|
|
auditList.Add(lastCAI);
|
|
}
|
|
else if (lastCAI.ActionWhat == "Changed" && firstCAI.ActionWhat == "Added" && lastCAI.Text == firstCAI.Text)
|
|
{
|
|
auditList.Add(firstCAI);
|
|
}
|
|
else if (lastCAI == null && firstCAI.ActionWhat == "Added")
|
|
{
|
|
auditList.Add(firstCAI);
|
|
}
|
|
lastCAI = null;
|
|
}
|
|
firstCAI = cai;
|
|
}
|
|
else
|
|
{
|
|
lastCAI = cai;
|
|
}
|
|
}
|
|
if (firstCAI != null)
|
|
auditList.Add(firstCAI);
|
|
if (lastCAI != null)
|
|
auditList.Add(lastCAI);
|
|
#region annotations
|
|
AnnotationAuditInfo firstAAI = null;
|
|
AnnotationAuditInfo nextAAI = null;
|
|
AnnotationAuditInfo lastAAI = null;
|
|
int icontentID = 0;
|
|
List<AnnotationAuditInfo> annotationList = new List<AnnotationAuditInfo>();
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
if (aai.IContentID != icontentID)
|
|
{
|
|
icontentID = aai.IContentID;
|
|
if (firstAAI != null)
|
|
{
|
|
if (lastAAI == null)
|
|
{
|
|
annotationList.Add(firstAAI);
|
|
}
|
|
else if (lastAAI.ActionWhat == "Deleted" && firstAAI.DTS < MyProc.DTS)
|
|
{
|
|
annotationList.Add(lastAAI);
|
|
}
|
|
else if (lastAAI.ActionWhat == "Changed" && lastAAI.SearchText != firstAAI.SearchText)
|
|
{
|
|
annotationList.Add(firstAAI);
|
|
annotationList.Add(lastAAI);
|
|
}
|
|
else if (lastAAI.ActionWhat == "Changed" && firstAAI.ActionWhat == "Added" && lastAAI.SearchText == firstAAI.SearchText)
|
|
{
|
|
annotationList.Add(firstAAI);
|
|
}
|
|
else if (lastAAI == null && firstAAI.ActionWhat == "Added")
|
|
{
|
|
annotationList.Add(firstAAI);
|
|
}
|
|
lastAAI = null;
|
|
}
|
|
firstAAI = aai;
|
|
}
|
|
else
|
|
lastAAI = aai;
|
|
}
|
|
if (firstAAI != null)
|
|
annotationList.Add(firstAAI);
|
|
if (lastAAI != null)
|
|
annotationList.Add(lastAAI);
|
|
#endregion
|
|
int cols = 5;
|
|
int borders = 0;
|
|
int paddingBottom = 6;
|
|
PdfPTable t = new PdfPTable(cols);
|
|
t.HeaderRows = 4;
|
|
t.DefaultCell.Padding = 4;
|
|
t.WidthPercentage = 100;
|
|
float[] widths = new float[] { 1f, 1f, 1f, 1f, 4f };
|
|
t.SetWidths(widths);
|
|
//t.HorizontalAlignment = 0;
|
|
iTextSharp.text.Font f1 = pdf.GetFont("Arial Unicode MS", 14, 1, Color.BLACK);
|
|
iTextSharp.text.Font f2 = pdf.GetFont("Arial Unicode MS", 10, 0, Color.BLACK);
|
|
iTextSharp.text.Font f3 = pdf.GetFont("Arial Unicode MS", 9, 0, Color.BLACK);
|
|
iTextSharp.text.Font f4 = pdf.GetFont("Arial Unicode MS", 9, 1, Color.BLACK);
|
|
//report title
|
|
Phrase h = new Phrase();
|
|
h.Font = f1;
|
|
h.Add("Summary of Changes Report");
|
|
PdfPCell c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
//procedure title
|
|
h = new Phrase();
|
|
h.Font = f2;
|
|
h.Add(string.Format("{0} - {1}", MyProc.DisplayNumber, MyProc.DisplayText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
foreach (ContentAuditInfo cai in auditList)
|
|
{
|
|
//raw path
|
|
string[] NewPath = SplitPath(cai.Path);
|
|
if (NewPath[0] != section)
|
|
{
|
|
section = NewPath[0];
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add(section);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
}
|
|
//step
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
firstCAI = cai;
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
else
|
|
{
|
|
t.AddCell(BlankCell(1));
|
|
nextCAI = cai;
|
|
}
|
|
//what
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.ActionWhen > MyProc.DTS ? cai.ActionWhat : "Original");
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(cai.Text));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
////old text
|
|
//t.AddCell(BlankCell(3));
|
|
//h = new Phrase();
|
|
//h.Font = f4;
|
|
//h.Add("Old text: " + cai.OldText);
|
|
//c = new PdfPCell(h);
|
|
//c.Colspan = 7;
|
|
//c.Border = 0;
|
|
//t.AddCell(c);
|
|
}
|
|
t.AddCell(BlankCell(cols));
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add("ANNOTATIONS");
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
stepnum = string.Empty;
|
|
foreach (AnnotationAuditInfo aai in annotationList)
|
|
{
|
|
foreach (ContentAuditInfo cai in auditList)
|
|
{
|
|
if (cai.ContentID == aai.IContentID)
|
|
{
|
|
string[] NewPath = SplitPath(cai.Path);
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
break;
|
|
}
|
|
else
|
|
{
|
|
t.AddCell(BlankCell(1));
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
//what
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
doc.Add(t);
|
|
}
|
|
private void BuildSummaryReport(iTextSharp.text.Document doc)
|
|
{
|
|
string section = string.Empty;
|
|
string stepnum = string.Empty;
|
|
int contentID = 0;
|
|
ContentAuditInfo firstCAI = null;
|
|
ContentAuditInfo nextCAI = null;
|
|
ContentAuditInfo lastCAI = null;
|
|
List<ContentAuditInfo> auditList = new List<ContentAuditInfo>();
|
|
foreach (ContentAuditInfo cai in AuditList)
|
|
{
|
|
if (cai.ContentID != contentID) //new content
|
|
{
|
|
if (firstCAI != null) //not first row
|
|
{
|
|
if (lastCAI == null)
|
|
{
|
|
auditList.Add(firstCAI);
|
|
firstCAI = null;
|
|
}
|
|
else
|
|
{
|
|
if (lastCAI.DTS > firstCAI.DTS)
|
|
{
|
|
if (firstCAI.ActionWhat == "Added" && lastCAI.ActionWhat != "Deleted")
|
|
{
|
|
if (firstCAI.DTS > MyProc.DTS)
|
|
lastCAI.ActionWhat = firstCAI.ActionWhat;
|
|
auditList.Add(lastCAI);
|
|
firstCAI = null;
|
|
lastCAI = null;
|
|
}
|
|
}
|
|
else if (firstCAI.ActionWhat == "Added" && lastCAI.ActionWhat == "Deleted" && firstCAI.DTS == lastCAI.DTS)
|
|
{
|
|
auditList.Add(lastCAI);
|
|
firstCAI = null;
|
|
lastCAI = null;
|
|
}
|
|
}
|
|
}
|
|
firstCAI = cai;
|
|
contentID = cai.ContentID;
|
|
}
|
|
else
|
|
lastCAI = cai;
|
|
}
|
|
if (lastCAI == null)
|
|
{
|
|
if (firstCAI != null)
|
|
auditList.Add(firstCAI);
|
|
firstCAI = null;
|
|
}
|
|
else
|
|
{
|
|
if (lastCAI.DTS > firstCAI.DTS)
|
|
{
|
|
if (firstCAI.ActionWhat == "Added" && lastCAI.ActionWhat != "Deleted")
|
|
{
|
|
if (firstCAI.DTS > MyProc.DTS)
|
|
lastCAI.ActionWhat = firstCAI.ActionWhat;
|
|
auditList.Add(lastCAI);
|
|
firstCAI = null;
|
|
lastCAI = null;
|
|
}
|
|
}
|
|
else if (firstCAI.ActionWhat == "Added" && lastCAI.ActionWhat == "Deleted" && firstCAI.DTS == lastCAI.DTS)
|
|
{
|
|
auditList.Add(lastCAI);
|
|
firstCAI = null;
|
|
lastCAI = null;
|
|
}
|
|
}
|
|
#region annotations commented out
|
|
//AnnotationAuditInfo firstAAI = null;
|
|
//AnnotationAuditInfo nextAAI = null;
|
|
//AnnotationAuditInfo lastAAI = null;
|
|
//int icontentID = 0;
|
|
//List<AnnotationAuditInfo> annotationList = new List<AnnotationAuditInfo>();
|
|
//foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
//{
|
|
// if (aai.IContentID != icontentID)
|
|
// {
|
|
// icontentID = aai.IContentID;
|
|
// if (firstAAI != null)
|
|
// {
|
|
// if (lastAAI == null)
|
|
// {
|
|
// annotationList.Add(firstAAI);
|
|
// }
|
|
// else if (lastAAI.ActionWhat == "Deleted" && firstAAI.DTS < MyProc.DTS)
|
|
// {
|
|
// annotationList.Add(lastAAI);
|
|
// }
|
|
// else if (lastAAI.ActionWhat == "Changed" && lastAAI.SearchText != firstAAI.SearchText)
|
|
// {
|
|
// annotationList.Add(firstAAI);
|
|
// annotationList.Add(lastAAI);
|
|
// }
|
|
// else if (lastAAI.ActionWhat == "Changed" && firstAAI.ActionWhat == "Added" && lastAAI.SearchText == firstAAI.SearchText)
|
|
// {
|
|
// annotationList.Add(firstAAI);
|
|
// }
|
|
// else if (lastAAI == null && firstAAI.ActionWhat == "Added")
|
|
// {
|
|
// annotationList.Add(firstAAI);
|
|
// }
|
|
// lastAAI = null;
|
|
// }
|
|
// firstAAI = aai;
|
|
// }
|
|
// else
|
|
// lastAAI = aai;
|
|
//}
|
|
//if (firstAAI != null)
|
|
// annotationList.Add(firstAAI);
|
|
//if (lastAAI != null)
|
|
// annotationList.Add(lastAAI);
|
|
#endregion
|
|
int cols = 5;
|
|
int borders = 0;
|
|
int paddingBottom = 6;
|
|
PdfPTable t = new PdfPTable(cols);
|
|
t.HeaderRows = 4;
|
|
t.DefaultCell.Padding = 4;
|
|
t.WidthPercentage = 100;
|
|
float[] widths = new float[] { 1f, 1f, 1f, 1f, 4f };
|
|
t.SetWidths(widths);
|
|
//t.HorizontalAlignment = 0;
|
|
iTextSharp.text.Font f1 = pdf.GetFont("Arial Unicode MS", 14, 1, Color.BLACK);
|
|
iTextSharp.text.Font f2 = pdf.GetFont("Arial Unicode MS", 10, 0, Color.BLACK);
|
|
iTextSharp.text.Font f3 = pdf.GetFont("Arial Unicode MS", 9, 0, Color.BLACK);
|
|
iTextSharp.text.Font f4 = pdf.GetFont("Arial Unicode MS", 9, 1, Color.BLACK);
|
|
//report title
|
|
Phrase h = new Phrase();
|
|
h.Font = f1;
|
|
h.Add("Summary of Changes Report");
|
|
PdfPCell c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
//procedure title
|
|
h = new Phrase();
|
|
h.Font = f2;
|
|
h.Add(string.Format("{0} - {1}", MyProc.DisplayNumber, MyProc.DisplayText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
ContentAuditInfo oldCAI = null;
|
|
// Dictionary<long, AnnotationAuditInfo> processedAAI = new Dictionary<long, AnnotationAuditInfo>();
|
|
Dictionary<AnnotationAuditInfo, AnnotationAuditInfo> processedAAI = new Dictionary<AnnotationAuditInfo, AnnotationAuditInfo>();
|
|
foreach (ContentAuditInfo cai in auditList)
|
|
{
|
|
//raw path
|
|
string[] NewPath = SplitPath(cai.Path);
|
|
if (NewPath[0] != section)
|
|
{
|
|
section = NewPath[0];
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add(section);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
}
|
|
//step
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
//see if any annotations for old stepnum here and add them
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
if (oldCAI != null && aai.IContentID == oldCAI.ContentID)
|
|
{
|
|
//add annotation to minilist
|
|
// processedAAI.Add(aai.AnnotationID, aai);
|
|
processedAAI.Add(aai, aai);
|
|
//write row to table
|
|
t.AddCell(BlankCell(1));
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add("Annotation " + aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
oldCAI = cai;
|
|
firstCAI = cai;
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
else
|
|
{
|
|
t.AddCell(BlankCell(1));
|
|
nextCAI = cai;
|
|
}
|
|
//what
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
// h.Add(cai.ActionWhen > MyProc.DTS ? cai.ActionWhat : "Original");
|
|
string actionWhat = cai.ActionWhat; //(cai.ActionWhat == "Added" && cai.DTS <= MyProc.DTS) ? "Original" : cai.ActionWhat != "Changed" ? cai.ActionWhat : cai.DTS <= maxDTS ? "Restored" : cai.DTS > MyProc.DTS ? cai.ActionWhat : "Original";
|
|
if (actionWhat == "Deleted" || actionWhat == "Restored")
|
|
h.Add(actionWhat + "\r\n" + cai.ActionWhen.ToString());
|
|
else
|
|
h.Add(actionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(cai.DTS.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
//h.Add(ItemInfo.ConvertToDisplayText(cai.Text));
|
|
if (cai.Type == 20008)
|
|
{
|
|
GridAuditInfoList gail = GridAuditInfoList.Get(cai.ContentID);
|
|
if (gail.Count > 0)
|
|
{
|
|
GridAuditInfo gai = gail[0];
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.LoadXml(gai.Data);
|
|
//int grows = int.Parse(xd.SelectSingleNode("C1FlexGrid/RowInfo/Count").InnerText);
|
|
int gcols = int.Parse(xd.SelectSingleNode("C1FlexGrid/ColumnInfo/Count").InnerText);
|
|
string data = ItemInfo.ConvertToDisplayText(cai.Text);
|
|
PdfPTable gtbl = new PdfPTable(gcols);
|
|
while (data.Length > 0)
|
|
{
|
|
string val = data.Substring(0, data.IndexOf(";"));
|
|
data = data.Substring(data.IndexOf(";") + 1);
|
|
Phrase hh = new Phrase();
|
|
hh.Font = f4;
|
|
hh.Add(val);
|
|
PdfPCell cc = new PdfPCell(hh);
|
|
gtbl.AddCell(cc);
|
|
}
|
|
c = new PdfPCell(gtbl);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
//c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add(ItemInfo.ConvertToDisplayText(cai.Text));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
|
|
//c = new PdfPCell(h);
|
|
//c.Colspan = cols - 4;
|
|
//c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
//c.PaddingBottom = paddingBottom;
|
|
////c.Border = borders;
|
|
//t.AddCell(c);
|
|
////old text
|
|
//t.AddCell(BlankCell(3));
|
|
//h = new Phrase();
|
|
//h.Font = f4;
|
|
//h.Add("Old text: " + cai.OldText);
|
|
//c = new PdfPCell(h);
|
|
//c.Colspan = 7;
|
|
//c.Border = 0;
|
|
//t.AddCell(c);
|
|
}
|
|
//see if any annotations for old stepnum here and add them
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
if (oldCAI != null && aai.IContentID == oldCAI.ContentID)
|
|
{
|
|
//add annotation to minilist
|
|
// processedAAI.Add(aai.AnnotationID, aai);
|
|
processedAAI.Add(aai, aai);
|
|
//write row to table
|
|
t.AddCell(BlankCell(1));
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add("Annotation " + aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
bool annotationHeader = false;
|
|
//t.AddCell(BlankCell(cols));
|
|
//h = new Phrase();
|
|
//h.Font = f4;
|
|
//h.Add("ANNOTATIONS");
|
|
//c = new PdfPCell(h);
|
|
//c.Colspan = cols;
|
|
//c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
//c.PaddingBottom = paddingBottom;
|
|
////c.Border = borders;
|
|
//t.AddCell(c);
|
|
//t.AddCell(BlankCell(cols));
|
|
stepnum = string.Empty;
|
|
foreach (AnnotationAuditInfo aai in AnnotationList)
|
|
{
|
|
// if (!processedAAI.ContainsKey(aai.AnnotationID))
|
|
if (!processedAAI.ContainsKey(aai))
|
|
{
|
|
if (!annotationHeader)
|
|
{
|
|
t.AddCell(BlankCell(cols));
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
h.Add("ANNOTATIONS");
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
t.AddCell(BlankCell(cols));
|
|
annotationHeader = true;
|
|
}
|
|
string[] NewPath = GetNewPath(AuditList, aai.IContentID);
|
|
if (NewPath[1] != stepnum)
|
|
{
|
|
stepnum = NewPath[1];
|
|
string stepnum2 = Regex.Replace(stepnum, "([0-9])[.]([A-Za-z])", "$1 $2");
|
|
stepnum2 = stepnum2.Replace("RNO.", "RNO");
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(string.Format("Step {0}", stepnum2));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_RIGHT;
|
|
c.PaddingBottom = paddingBottom;
|
|
c.PaddingRight = 10;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
else
|
|
{
|
|
t.AddCell(BlankCell(1));
|
|
}
|
|
//what
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhat);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//who
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.UserID);
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//when
|
|
h = new Phrase();
|
|
h.Font = f3;
|
|
h.Add(aai.ActionWhen.ToString());
|
|
c = new PdfPCell(h);
|
|
c.Colspan = 1;
|
|
c.HorizontalAlignment = Element.ALIGN_CENTER;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
//new text
|
|
h = new Phrase();
|
|
h.Font = f4;
|
|
//h.Add(cai.Text);
|
|
h.Add(ItemInfo.ConvertToDisplayText(aai.SearchText));
|
|
c = new PdfPCell(h);
|
|
c.Colspan = cols - 4;
|
|
c.HorizontalAlignment = Element.ALIGN_LEFT;
|
|
c.PaddingBottom = paddingBottom;
|
|
//c.Border = borders;
|
|
t.AddCell(c);
|
|
}
|
|
}
|
|
if (t.Rows.Count == t.HeaderRows)
|
|
{
|
|
c = new PdfPCell(new Phrase("No changes to report"));
|
|
c.Colspan = cols;
|
|
t.AddCell(c);
|
|
}
|
|
doc.Add(t);
|
|
}
|
|
}
|
|
}
|