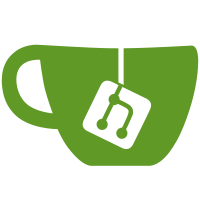
Fixed logic that determines if the debug output should be created in the PDF. Added ability to track DB actions. Change cursor to a wait curcor when the print button is pressed. Added Profile debug Added a time-out for determining if a RO.FST file is newer. Added a property (PrintAllAtOnce) to the ItemInfo object so that the code can differentiate between objects that are being used for edit or print. Added logic to improve print performance. Specifically initialize a number of item properties when the procedure is loaded for printing, rather than waiting for the properties to be lazy-loaded. Added Profile debug Changed NextItem property to use GetNextItem method when printing.
176 lines
4.9 KiB
C#
176 lines
4.9 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using Volian.Print.Library;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Base.Library;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
public partial class frmPDFStatusForm : Form
|
|
{
|
|
private bool _CancelPrinting = false;
|
|
public bool CancelPrinting
|
|
{
|
|
get { return _CancelPrinting; }
|
|
set { _CancelPrinting = value; }
|
|
}
|
|
private bool _CloseWhenDone = false;
|
|
public bool CloseWhenDone
|
|
{
|
|
get { return _CloseWhenDone; }
|
|
set { _CloseWhenDone = value; }
|
|
}
|
|
private bool _CancelStop = false;
|
|
public bool CancelStop
|
|
{
|
|
get { return _CancelStop; }
|
|
set { _CancelStop = value; }
|
|
}
|
|
private bool _Stop = false;
|
|
public bool Stop
|
|
{
|
|
get { return _Stop; }
|
|
set { _Stop = value; }
|
|
}
|
|
private string _PDFPath;
|
|
|
|
public string PDFPath
|
|
{
|
|
get { return _PDFPath; }
|
|
set { _PDFPath = value; }
|
|
}
|
|
private PromsPrinter _MyPromsPrinter;
|
|
|
|
public PromsPrinter MyPromsPrinter
|
|
{
|
|
get { return _MyPromsPrinter; }
|
|
set { _MyPromsPrinter = value; }
|
|
}
|
|
|
|
private bool _OpenPDF;
|
|
|
|
public bool OpenPDF
|
|
{
|
|
get { return _OpenPDF; }
|
|
set { _OpenPDF = value; }
|
|
}
|
|
private Point _NewLocation;
|
|
public frmPDFStatusForm(ItemInfo myItem, string rev, string watermark, bool debugOutput, bool origPgBrk, bool openPDF, bool overWrite, string pdfPath, ChangeBarDefinition cbd,string pdfFile, Point newLocation,bool insertBlankPages)
|
|
{
|
|
OpenPDF = openPDF;
|
|
InitializeComponent();
|
|
// if the version number of PROMS is 1.0, then we are running a Demo version.
|
|
// When running a Demo version, force a "Sample" watermark when printing.
|
|
MyPromsPrinter = new PromsPrinter(myItem, rev, (VlnSettings.ReleaseMode.Equals("DEMO")) ? "Sample" : watermark, debugOutput, origPgBrk, pdfPath + @"\\Compare", false, overWrite, cbd, pdfFile, insertBlankPages);//openPDF);
|
|
PDFPath = pdfPath;
|
|
this.Text = "Creating PDF of " + myItem.DisplayNumber;
|
|
_NewLocation = newLocation;
|
|
DialogResult = DialogResult.OK;
|
|
}
|
|
public bool AllowAllWatermarks
|
|
{
|
|
get { return MyPromsPrinter.AllowAllWatermarks; }
|
|
set { MyPromsPrinter.AllowAllWatermarks = value; }
|
|
}
|
|
void pp_StatusChanged(object sender, PromsPrintStatusArgs args)
|
|
{
|
|
if (args.Type == PromsPrinterStatusType.ProgressSetup)
|
|
pb.Maximum = args.Progress;
|
|
else if (args.Type == PromsPrinterStatusType.Progress)
|
|
{
|
|
pb.Value = args.Progress;
|
|
if (args.Progress == pb.Maximum)
|
|
pb.Text = args.MyStatus;
|
|
else
|
|
pb.Text = string.Format("Processing {0} ({1} of {2})", args.MyStatus, args.Progress + 1, pb.Maximum);
|
|
}
|
|
MyStatus = args.MyStatus;
|
|
Application.DoEvents();
|
|
}
|
|
public string MyStatus
|
|
{
|
|
get { return lblStatus.Text; }
|
|
set { lblStatus.Text = value; Application.DoEvents(); }
|
|
}
|
|
|
|
private void frmPDFStatusForm_Load(object sender, EventArgs e)
|
|
{
|
|
Location = _NewLocation;
|
|
tmrRun.Enabled = true;
|
|
}
|
|
|
|
private string _PdfFile;
|
|
|
|
private bool _MakePlaceKeeper = false;
|
|
|
|
public bool MakePlaceKeeper
|
|
{
|
|
get { return _MakePlaceKeeper; }
|
|
set { _MakePlaceKeeper = value; }
|
|
}
|
|
private void tmrRun_Tick(object sender, EventArgs e)
|
|
{
|
|
tmrRun.Enabled = false;
|
|
if (CancelStop) btnCancel.Visible = true;
|
|
Application.DoEvents();
|
|
MyPromsPrinter.StatusChanged += new PromsPrinterStatusEvent(pp_StatusChanged);
|
|
DateTime tStart = DateTime.Now;
|
|
if (!CancelStop) PromsPrinter.ClearTransPageNumProblems();
|
|
do
|
|
{
|
|
int profileDepth = ProfileTimer.Push(">>>> MyPromsPrinter.Print");
|
|
_PdfFile = MyPromsPrinter.Print(PDFPath, MakePlaceKeeper);
|
|
ProfileTimer.Pop(profileDepth);
|
|
}
|
|
while (_PdfFile == null && MessageBox.Show("Try Again?", "PDF Creation Failed", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes);
|
|
if (_PdfFile == null)
|
|
{
|
|
this.Close();
|
|
return;
|
|
}
|
|
if (!CancelStop)
|
|
{
|
|
if (PromsPrinter.ReportTransPageNumProblems() == DialogResult.Yes && MyPromsPrinter.BeforePageNumberPdf != null)
|
|
{
|
|
System.Diagnostics.Process.Start(MyPromsPrinter.BeforePageNumberPdf);
|
|
}
|
|
}
|
|
DateTime tEnd = DateTime.Now;
|
|
MyStatus = _PdfFile + " created.";
|
|
MyStatus = string.Format("{0} created in {1:0.} milliseconds", _PdfFile, (TimeSpan.FromTicks(tEnd.Ticks - tStart.Ticks).TotalMilliseconds));
|
|
if (OpenPDF)
|
|
{
|
|
System.Diagnostics.Process.Start(_PdfFile);
|
|
this.Close();
|
|
return;
|
|
}
|
|
btnOpenFolder.Visible = btnOpenPDF.Visible = true;
|
|
if (CloseWhenDone)
|
|
{
|
|
this.Close();
|
|
return;
|
|
}
|
|
}
|
|
private void btnOpenPDF_Click(object sender, EventArgs e)
|
|
{
|
|
System.Diagnostics.Process.Start(_PdfFile);
|
|
this.Close();
|
|
}
|
|
private void btnOpenFolder_Click(object sender, EventArgs e)
|
|
{
|
|
System.Diagnostics.Process.Start("Explorer", "/select," + _PdfFile);
|
|
this.Close();
|
|
}
|
|
private void btnCancel_Click(object sender, EventArgs e)
|
|
{
|
|
CancelPrinting = true;
|
|
btnCancel.Text = "Cancelled";
|
|
btnCancel.Enabled = false;
|
|
}
|
|
}
|
|
} |