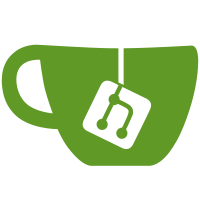
Added PurgeDataCommand class based on CSLA CommandBase class Added CanTransitionBeCreatedCommand class based on CSLA CommandBase class Added WillTransitionsBeValidCommand class based on CSLA CommandBase class Added InvalidTransition class Added ProposedTransition class Changed NodeText_Changed method to support multi unit Added BigNum class to support applicability for multi unit
745 lines
23 KiB
C#
745 lines
23 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using Csla;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Reflection;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public delegate void VETreeNodeEvent(object sender, VETreeNodeEventArgs args);
|
|
public class VETreeNodeEventArgs : EventArgs
|
|
{
|
|
public VETreeNodeEventArgs() { ; }
|
|
public VETreeNodeEventArgs(string info)
|
|
{
|
|
_Info = info;
|
|
}
|
|
public VETreeNodeEventArgs(int value)
|
|
{
|
|
_Value = value;
|
|
}
|
|
public VETreeNodeEventArgs(string info, int value)
|
|
{
|
|
_Info = info;
|
|
_Value = value;
|
|
}
|
|
private int _Value;
|
|
|
|
public int Value
|
|
{
|
|
get { return _Value; }
|
|
set { _Value = value; }
|
|
}
|
|
private string _Info;
|
|
|
|
public string Info
|
|
{
|
|
get { return _Info; }
|
|
set { _Info = value; }
|
|
}
|
|
|
|
}
|
|
public class VETreeNode : TreeNode
|
|
{
|
|
#region Events
|
|
public event VETreeNodeEvent LoadingChildrenSQL;
|
|
public event VETreeNodeEvent LoadingChildrenMax;
|
|
public event VETreeNodeEvent LoadingChildrenValue;
|
|
public event VETreeNodeEvent LoadingChildrenDone;
|
|
private void OnLoadingChildrenSQL(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
if (LoadingChildrenSQL != null) LoadingChildrenSQL(sender, args);
|
|
}
|
|
private void OnLoadingChildrenMax(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
if (LoadingChildrenMax != null) LoadingChildrenMax(sender, args);
|
|
}
|
|
private void OnLoadingChildrenValue(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
if (LoadingChildrenValue != null) LoadingChildrenValue(sender, args);
|
|
}
|
|
private void OnLoadingChildrenDone(object sender, VETreeNodeEventArgs args)
|
|
{
|
|
if (LoadingChildrenDone != null) LoadingChildrenDone(sender, args);
|
|
}
|
|
#endregion
|
|
#region Business Methods
|
|
protected IVEDrillDownReadOnly _VEObject;
|
|
public IVEDrillDownReadOnly VEObject
|
|
{
|
|
get { return _VEObject; }
|
|
set
|
|
{
|
|
_VEObject = value;
|
|
base.Text = _VEObject.ToString();
|
|
ResetNode("Dummy Set_VEObject");
|
|
}
|
|
}
|
|
//public void Refresh()
|
|
//{
|
|
// if (_VEObject != null)
|
|
// Text = _VEObject.ToString();
|
|
//}
|
|
// Only load the Children Once
|
|
protected bool _ChildrenLoaded = false;
|
|
public bool ChildrenLoaded
|
|
{
|
|
get { return _ChildrenLoaded; }
|
|
set { _ChildrenLoaded = value; }
|
|
}
|
|
// Reset Node
|
|
//public void CloseNode()
|
|
//{
|
|
// ResetNode();
|
|
//}
|
|
private void SetProperty(string name)
|
|
{
|
|
PropertyInfo propertyInfoObj = _VEObject.GetType().GetProperty(name);
|
|
if (propertyInfoObj == null) return;
|
|
PropertyInfo propertyInfoThis = this.GetType().GetProperty(name);
|
|
if (propertyInfoThis == null) return;
|
|
try
|
|
{
|
|
propertyInfoThis.SetValue(this, propertyInfoObj.GetValue(_VEObject, null), null);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("Error: {0}", ex.Message);
|
|
}
|
|
}
|
|
public void ResetNode(string dummy)
|
|
{
|
|
if (_VEObject!=null && _VEObject.HasChildren && _ChildrenLoaded == false && CheckForParts())
|
|
{
|
|
_ChildrenLoaded = false;// Reset the children loaded flag
|
|
this.Nodes.Add(dummy);// Add a Dummy Node so that the item will appear to be expanable.
|
|
}
|
|
else
|
|
{
|
|
_ChildrenLoaded = true;// Reset the children loaded flag
|
|
}
|
|
if (base.Text != _VEObject.ToString()) base.Text = _VEObject.ToString();
|
|
SetProperty("ForeColor");
|
|
SetProperty("BackColor");
|
|
SetProperty("ImageIndex");
|
|
SetProperty("IsVisible");
|
|
SetProperty("SelectedImageIndex");
|
|
// ToDo: Need to reset object as well
|
|
}
|
|
|
|
private bool CheckForParts()
|
|
{
|
|
if (_allParts) return true;
|
|
// only has 'children' if parts are steps or sections
|
|
if (_VEObject.GetType() == typeof(StepInfo) || _VEObject.GetType() == typeof(SectionInfo) || _VEObject.GetType() == typeof(ItemInfo))
|
|
{
|
|
IList ol = ((ItemInfo)_VEObject).GetChildren(_allParts);
|
|
if (ol == null || ol.Count == 0) return false;
|
|
}
|
|
return true;
|
|
}
|
|
public void RefreshNode()
|
|
{
|
|
ResetNode("Dummy RefreshNode");// Drop Children
|
|
LoadChildren();// Load Children
|
|
}
|
|
public static VETreeNode GetFolder(int folderID)
|
|
{
|
|
VETreeNode tn = new VETreeNode(FolderInfo.Get(folderID));
|
|
//tn.Nodes.Add("Dummy");
|
|
tn.ResetNode("Dummy GetFolder");
|
|
return tn;
|
|
}
|
|
// public abstract void LoadChildren();
|
|
//private long _Start;
|
|
//private Dictionary<string, long> _Timings=new Dictionary<string,long>();
|
|
//private void tReset()
|
|
//{
|
|
// _Timings = new Dictionary<string, long>();
|
|
// _Start = DateTime.Now.Ticks;
|
|
//}
|
|
//private void tNext(string msg)
|
|
//{
|
|
// long tEnd = DateTime.Now.Ticks;
|
|
// long tDiff = tEnd - _Start;
|
|
// if (_Timings.ContainsKey(msg)) tDiff += _Timings[msg];
|
|
// _Timings[msg] = tDiff;
|
|
// _Start = DateTime.Now.Ticks;
|
|
//}
|
|
//private void tShowResults()
|
|
//{
|
|
// Console.WriteLine("Timings");
|
|
// long total=0;
|
|
// foreach (string msg in _Timings.Keys)
|
|
// {
|
|
// total += _Timings[msg];
|
|
// Console.WriteLine("{0}\t\"{1}\"", TimeSpan.FromTicks(_Timings[msg]).TotalMilliseconds, msg);
|
|
// }
|
|
// Console.WriteLine("{0}\t\"Total\"", TimeSpan.FromTicks(total).TotalMilliseconds);
|
|
//}
|
|
public virtual void LoadChildren()
|
|
{
|
|
LoadChildren(true);
|
|
}
|
|
private bool _allParts = true;
|
|
public virtual void LoadChildren(bool allParts)
|
|
{
|
|
_allParts = allParts;
|
|
if (!_ChildrenLoaded)
|
|
{
|
|
this.Nodes.Clear();
|
|
//tReset();
|
|
DateTime tStart = DateTime.Now;
|
|
//TVAddChildren(_VEObject, this);
|
|
OnLoadingChildrenSQL(this, new VETreeNodeEventArgs());
|
|
IList ol;
|
|
ItemInfo item = _VEObject as ItemInfo;
|
|
if (item != null) item.RefreshItemParts();
|
|
if (_VEObject.GetType() == typeof(StepInfo) || _VEObject.GetType() == typeof(SectionInfo) || _VEObject.GetType() == typeof(ItemInfo))
|
|
ol = ((ItemInfo)_VEObject).GetChildren(allParts);
|
|
else
|
|
ol = _VEObject.GetChildren();
|
|
//tNext("GetChildren");
|
|
if (ol != null)
|
|
{
|
|
OnLoadingChildrenMax(this, new VETreeNodeEventArgs(ol.Count));
|
|
this.TreeView.BeginUpdate();
|
|
ExpandChildren(ol);
|
|
//}
|
|
//tNext("Set Nodes");
|
|
this.TreeView.EndUpdate();
|
|
//tNext("End Update");
|
|
//tShowResults();
|
|
}
|
|
_ChildrenLoaded = true;
|
|
OnLoadingChildrenDone(this, new VETreeNodeEventArgs(TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds.ToString()));
|
|
}
|
|
}
|
|
|
|
private void ExpandChildren(IList ol)
|
|
{
|
|
int icnt = 0;
|
|
bool lastWasSection = false;
|
|
foreach (IVEDrillDownReadOnly o in ol)
|
|
{
|
|
OnLoadingChildrenValue(this, new VETreeNodeEventArgs(++icnt));
|
|
//tNext("Cycle");
|
|
try
|
|
{
|
|
bool skipIt = false;
|
|
bool isStepPart = (o is PartInfo && (o as PartInfo).PartType == E_FromType.Step);
|
|
if (isStepPart)
|
|
{
|
|
// get parent section and see if it has the Editable flag set. Only skip
|
|
// if this flag is set to "N".
|
|
ItemInfo sectInfo = (ItemInfo)(o as PartInfo).ActiveParent;
|
|
if (sectInfo != null)
|
|
{
|
|
SectionConfig sc = sectInfo.MyConfig as SectionConfig;
|
|
if (sc != null && sc.SubSection_Edit == "N") skipIt = true;
|
|
}
|
|
}
|
|
if (!skipIt)
|
|
{
|
|
VETreeNode tmp = new VETreeNode(o, _allParts);
|
|
if (o.HasChildren)
|
|
{
|
|
if (o is PartInfo)
|
|
{
|
|
tmp.Nodes.Clear();
|
|
tmp.ExpandChildren(o.GetChildren());
|
|
tmp._ChildrenLoaded = true;
|
|
}
|
|
// OLD: RHM 20100115 : I don't think that the following lines are necessary since the "new VETreeNode(o)"
|
|
// above includes a similar function.
|
|
//else
|
|
// tmp.Nodes.Add(string.Format("dummy: {0}", o.GetType().Name));// Add a Dummy Node so that the item will appear to be expanable.
|
|
}
|
|
else
|
|
tmp._ChildrenLoaded = true;// Reset the children loaded flag
|
|
if (lastWasSection)
|
|
this.Nodes.Insert(0, tmp);
|
|
else
|
|
this.Nodes.Add(tmp);
|
|
// if last thing was section & this is step, do insert - i.e. so that steps go before sections.
|
|
lastWasSection = (o is PartInfo && (o as PartInfo).PartType == E_FromType.Section);
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
}
|
|
}
|
|
}
|
|
private void TVAddChildren(IVEDrillDownReadOnly veobj, VETreeNode tn)
|
|
{
|
|
OnLoadingChildrenSQL(tn, new VETreeNodeEventArgs());
|
|
IList ol;
|
|
ItemInfo item = veobj as ItemInfo;
|
|
if (veobj.GetType() == typeof(StepInfo) || veobj.GetType() == typeof(SectionInfo) || veobj.GetType() == typeof(ItemInfo))
|
|
ol = ((ItemInfo)veobj).GetChildren(true);
|
|
else
|
|
ol = veobj.GetChildren();
|
|
if (ol != null)
|
|
{
|
|
OnLoadingChildrenMax(this, new VETreeNodeEventArgs(ol.Count));
|
|
this.TreeView.BeginUpdate();
|
|
int icnt = 0;
|
|
foreach (IVEDrillDownReadOnly o in ol)
|
|
{
|
|
OnLoadingChildrenValue(this, new VETreeNodeEventArgs(++icnt));
|
|
try
|
|
{
|
|
VETreeNode tmp = new VETreeNode(o);
|
|
PartInfo pi = o as PartInfo;
|
|
if (pi == null && o.HasChildren)
|
|
tmp.Nodes.Add("Dummy");// Add a Dummy Node so that the item will appear to be expanable.
|
|
else
|
|
tmp._ChildrenLoaded = true;// Reset the children loaded flag
|
|
tn.Nodes.Add(tmp);
|
|
if (pi != null && o.HasChildren)
|
|
TVAddChildren(o, tmp);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
}
|
|
}
|
|
|
|
//}
|
|
this.TreeView.EndUpdate();
|
|
}
|
|
}
|
|
void myItemInfo_Deleted(object sender)
|
|
{
|
|
VETreeNode parnode = Parent as VETreeNode;
|
|
if (parnode == null) return;
|
|
PartInfo pi = parnode.VEObject as PartInfo;
|
|
Remove();
|
|
// get rid of 'Steps', 'RNOs', i.e. grouping nodes, if there are no children.
|
|
if (pi != null && parnode.Nodes.Count == 0)
|
|
{
|
|
VETreeNode grndparnode = parnode.Parent as VETreeNode;
|
|
parnode.Remove();
|
|
// if only 'Steps' node is left, move steps 'up' a level.
|
|
if (grndparnode != null && grndparnode.Nodes.Count == 1)
|
|
{
|
|
VETreeNode sibnode = grndparnode.Nodes[0] as VETreeNode;
|
|
PartInfo pisib = sibnode.VEObject as PartInfo;
|
|
if (pisib != null && (pisib.ToString() == "Steps"))
|
|
{
|
|
if (!sibnode.ChildrenLoaded) sibnode.LoadChildren();
|
|
while (sibnode.Nodes.Count > 0)
|
|
{
|
|
VETreeNode tmp = sibnode.Nodes[0] as VETreeNode;
|
|
tmp.Remove();
|
|
grndparnode.Nodes.Add(tmp);
|
|
}
|
|
sibnode.Remove();
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
//public IVEReadOnlyItem GetCsla()
|
|
//{
|
|
// return _VEObject;
|
|
//}
|
|
#endregion
|
|
#region Factory Methods
|
|
// Constructors
|
|
public VETreeNode() : base("NoText") { ; }
|
|
public VETreeNode(IVEDrillDownReadOnly o, bool allParts)
|
|
: base(o.ToString())
|
|
{
|
|
_allParts = allParts;
|
|
_VEObject = o;// Save the BusinessObject
|
|
ResetNode("Dummy VETreeNode(IVEDrillDownReadOnly o)");
|
|
ItemInfo myItemInfo = o as ItemInfo;
|
|
if (myItemInfo != null)
|
|
{
|
|
myItemInfo.Deleted += new ItemInfoEvent(myItemInfo_Deleted);
|
|
myItemInfo.ChildrenDeleted += new ItemInfoEvent(myItemInfo_ChildrenDeleted);
|
|
myItemInfo.MyContent.Changed += new ContentInfoEvent(NodeText_Changed);
|
|
myItemInfo.OrdinalChanged += new ItemInfoEvent(NodeText_Changed);
|
|
myItemInfo.NewSiblingAfter += new ItemInfoInsertEvent(myItemInfo_NewSiblingAfter);
|
|
myItemInfo.NewSiblingBefore += new ItemInfoInsertEvent(myItemInfo_NewSiblingBefore);
|
|
myItemInfo.NewChild += new ItemInfoInsertEvent(myItemInfo_NewChild);
|
|
}
|
|
}
|
|
void NodeText_Changed(object sender)
|
|
{
|
|
Text = _VEObject.ToString();
|
|
ItemInfo myItemInfo = _VEObject as ItemInfo;
|
|
if (myItemInfo != null && myItemInfo.MyDocVersion.DocVersionConfig.SelectedSlave > 0)
|
|
{
|
|
int k = myItemInfo.MyDocVersion.DocVersionConfig.SelectedSlave;
|
|
myItemInfo.MyDocVersion.DocVersionConfig.SelectedSlave = 0;
|
|
Text = myItemInfo.ToString();
|
|
myItemInfo.MyDocVersion.DocVersionConfig.SelectedSlave = k;
|
|
}
|
|
if (myItemInfo != null && myItemInfo.IsSection)
|
|
{
|
|
myItemInfo.RefreshConfig();
|
|
// check if metasection & has subsections. This could be a change in config
|
|
// item, i.e. Editable Data, that would add or remove any step nodes, i.e. requires a
|
|
// tree refresh.
|
|
if (myItemInfo.Sections != null && myItemInfo.Sections.Count > 0 && myItemInfo.ActiveFormat.PlantFormat.FormatData.SectData.UseMetaSections)
|
|
{
|
|
// see if change in children tree nodes.
|
|
this.Nodes.Clear();
|
|
_ChildrenLoaded = false;
|
|
LoadChildren(true);
|
|
}
|
|
}
|
|
}
|
|
public VETreeNode(IVEDrillDownReadOnly o)
|
|
: base(o.ToString())
|
|
{
|
|
_VEObject = o;// Save the BusinessObject
|
|
ResetNode("Dummy VETreeNode(IVEDrillDownReadOnly o)");
|
|
ItemInfo myItemInfo = o as ItemInfo;
|
|
if (myItemInfo != null)
|
|
{
|
|
myItemInfo.Deleted += new ItemInfoEvent(myItemInfo_Deleted);
|
|
myItemInfo.ChildrenDeleted += new ItemInfoEvent(myItemInfo_ChildrenDeleted);
|
|
myItemInfo.MyContent.Changed += new ContentInfoEvent(NodeText_Changed);
|
|
myItemInfo.OrdinalChanged += new ItemInfoEvent(NodeText_Changed);
|
|
myItemInfo.NewSiblingAfter += new ItemInfoInsertEvent(myItemInfo_NewSiblingAfter);
|
|
myItemInfo.NewSiblingBefore += new ItemInfoInsertEvent(myItemInfo_NewSiblingBefore);
|
|
myItemInfo.NewChild += new ItemInfoInsertEvent(myItemInfo_NewChild);
|
|
}
|
|
}
|
|
|
|
void myItemInfo_ChildrenDeleted(object sender)
|
|
{
|
|
//Console.WriteLine("Fix Children");
|
|
ItemInfo myItemInfo = VEObject as ItemInfo;
|
|
//if (Nodes.Count > 1)
|
|
// Console.WriteLine("{0}, {1}",Nodes[0], Nodes[1]);
|
|
|
|
if (!myItemInfo.HasChildren && Nodes.Count>0 && !(Nodes[0] is VETreeNode))
|
|
Nodes.Clear();
|
|
}
|
|
void myItemInfo_NewChild(object sender, ItemInfoInsertEventArgs args)
|
|
{
|
|
if (args.ItemInserted.NextItem != null) // insert before
|
|
{
|
|
int nextItemID = args.ItemInserted.NextItem.ItemID;
|
|
VETreeNode nextNode = FindChildOrGrandChild(nextItemID);
|
|
if(nextNode != null)
|
|
nextNode.myItemInfo_NewSiblingBefore(sender, args);
|
|
return;
|
|
}
|
|
if (args.ItemInserted.MyPrevious != null) // insert after
|
|
{
|
|
int prevItemID = args.ItemInserted.MyPrevious.ItemID;
|
|
VETreeNode prevNode = FindChildOrGrandChild(prevItemID);
|
|
prevNode.myItemInfo_NewSiblingAfter(sender, args);
|
|
return;
|
|
}
|
|
bool isExpanded = IsExpanded;
|
|
// Restore the tree as it currently is expanded.
|
|
Collapse();
|
|
Nodes.Clear();
|
|
_ChildrenLoaded = false;
|
|
ResetNode("Dummy myItemInfo_NewChild");
|
|
ItemInfo item = VEObject as ItemInfo;
|
|
if (isExpanded && item != null && item.MyContent.ContentPartCount > 1) // || args.ItemInserted.NextItem != null || args.ItemInserted.MyPrevious != null))
|
|
Expand();
|
|
else
|
|
Collapse();
|
|
}
|
|
private VETreeNode FindChildOrGrandChild(int itemID)
|
|
{
|
|
foreach (TreeNode childNode in Nodes)
|
|
{
|
|
VETreeNode child = childNode as VETreeNode;
|
|
if (child != null)
|
|
{
|
|
ItemInfo item = child.VEObject as ItemInfo;
|
|
if (item != null && item.ItemID == itemID)
|
|
return child;
|
|
}
|
|
}
|
|
foreach (TreeNode childNode in Nodes)
|
|
{
|
|
VETreeNode child = childNode as VETreeNode;
|
|
if (child != null && child.VEObject is PartInfo)
|
|
foreach (VETreeNode grandchild in child.Nodes)
|
|
{
|
|
ItemInfo item = grandchild.VEObject as ItemInfo;
|
|
if (item != null && item.ItemID == itemID)
|
|
return grandchild;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
void myItemInfo_NewSiblingBefore(object sender, ItemInfoInsertEventArgs args)
|
|
{
|
|
if(this.Parent != null) // Only do this if the node has a parent - RHM 20100106
|
|
this.Parent.Nodes.Insert(Index,(new VETreeNode(args.ItemInserted)));
|
|
}
|
|
void myItemInfo_NewSiblingAfter(object sender, ItemInfoInsertEventArgs args)
|
|
{
|
|
if (this.Parent != null) // Only do this if the node has a parent - RHM 20100106
|
|
this.Parent.Nodes.Insert(Index + 1, (new VETreeNode(args.ItemInserted)));
|
|
}
|
|
public VETreeNode(string s)
|
|
: base(s)
|
|
{
|
|
_VEObject = null;// Save the BusinessObject
|
|
//ResetNode();
|
|
}
|
|
#endregion
|
|
}
|
|
//public class VETreeNodeBase<T> : VETreeNode
|
|
//where T : VETreeNode, new()
|
|
//{
|
|
// #region Factory Methods
|
|
// private static int _ICount = 0;
|
|
// public VETreeNodeBase(string s) : base(s) { ;}
|
|
// public VETreeNodeBase(IVEReadOnlyItem o) : base(o) { ;}
|
|
// protected VETreeNodeBase() : base() {
|
|
// }
|
|
// private DateTime tNext(DateTime tStart, string msg)
|
|
// {
|
|
// DateTime tEnd = DateTime.Now;
|
|
// TimeSpan ts = new TimeSpan(tEnd.Ticks-tStart.Ticks);
|
|
// Console.WriteLine("{0} - {1}", ts.TotalMilliseconds, msg);
|
|
// return tEnd;
|
|
// }
|
|
// public override void LoadChildren()
|
|
// {
|
|
// if (!_ChildrenLoaded)
|
|
// {
|
|
// this.Nodes.Clear();
|
|
// "".TrimEnd("\r\n".ToCharArray());
|
|
// IList ol = _VEObject.GetChildren();
|
|
// if (ol != null)
|
|
// {
|
|
// this.TreeView.BeginUpdate();
|
|
// DateTime tStart = DateTime.Now;
|
|
// T[] tmpa = new T[ol.Count];
|
|
// tStart = tNext(tStart, "Allocate Array");
|
|
// for (int i = 0; i < ol.Count; i++) tmpa[i] = new T();
|
|
// tStart = tNext(tStart, "Allocate Nodes");
|
|
// int ii = 0;
|
|
// foreach (IVEReadOnlyItem o in ol)
|
|
// {
|
|
// try
|
|
// {
|
|
// tmpa[ii++].VEObject = o;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
// }
|
|
// }
|
|
// tStart = tNext(tStart, "Set Nodes");
|
|
// for (int i = 0; i < ol.Count; i++) tmpa[i].ResetNode();
|
|
// tStart = tNext(tStart, "Reset Nodes");
|
|
// this.Nodes.AddRange(tmpa);
|
|
// tStart = tNext(tStart, "Add Range");
|
|
// //foreach (IVEReadOnlyItem o in ol)
|
|
// //{
|
|
// // try
|
|
// // {
|
|
// // T tmp = new T();
|
|
// // tmp.VEObject = o;
|
|
// // this.Nodes.Add(tmp);
|
|
// // }
|
|
// // catch (Exception ex)
|
|
// // {
|
|
// // Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
// // }
|
|
// //}
|
|
// this.TreeView.EndUpdate();
|
|
// }
|
|
// _ChildrenLoaded = true;
|
|
// }
|
|
// }
|
|
//#endregion
|
|
//}
|
|
/*
|
|
public class VEFolder : VETreeNodeBase<VEVersion>
|
|
{
|
|
public static VEFolder GetFolder(int folderID)
|
|
{
|
|
return new VEFolder(FolderInfo.Get(folderID));
|
|
}
|
|
//public static VEFolder LoadTree()
|
|
//{
|
|
// VEFolder root = null;
|
|
// FolderInfoList fil = FolderInfoList.Get();
|
|
// Dictionary<int, VEFolder> dicMissing = new Dictionary<int, VEFolder>();
|
|
// Dictionary<int, VEFolder> dicExists = new Dictionary<int, VEFolder>();
|
|
// foreach (FolderInfo fi in fil)
|
|
// {
|
|
// VEFolder ftp = null;
|
|
// if (dicExists.ContainsKey(fi.ParentID))
|
|
// {
|
|
// ftp = dicExists[fi.ParentID];
|
|
// }
|
|
// else
|
|
// {
|
|
// if (fi.ParentID != fi.FolderID)
|
|
// {
|
|
// ftp = new VEFolder(fi.ParentID.ToString());
|
|
// dicMissing.Add(fi.ParentID, ftp);
|
|
// dicExists.Add(fi.ParentID, ftp);
|
|
// }
|
|
// }
|
|
// VEFolder ft = null;
|
|
// if (dicMissing.ContainsKey(fi.FolderID))
|
|
// {
|
|
// ft = dicMissing[fi.FolderID];
|
|
// ft.VEObject = fi;
|
|
// dicMissing.Remove(fi.FolderID);
|
|
// }
|
|
// else
|
|
// {
|
|
// ft = new VEFolder(fi);
|
|
// dicExists.Add(fi.FolderID, ft);
|
|
// }
|
|
// if (fi.ParentID == fi.FolderID)
|
|
// root = ft;
|
|
// else
|
|
// ftp.Nodes.Add(ft);
|
|
// }
|
|
// //root.FindTree = dicExists;
|
|
// return root;
|
|
//}
|
|
private VEFolder(string s) : base(s) { ;}
|
|
public VEFolder(IVEReadOnlyItem o) : base(o) { ;}
|
|
}
|
|
public class VEVersion : VETreeNodeBase<VEProcedure> { }
|
|
public class VEProcedure : VETreeNodeBase<VESection>
|
|
{
|
|
//public override void LoadChildren()
|
|
//{
|
|
// if (!_ChildrenLoaded)
|
|
// {
|
|
// this.Nodes.Clear();
|
|
// Dictionary<string, VESection> dicSect = new Dictionary<string, VESection>();
|
|
// SectionInfoList ol = (SectionInfoList)_VEObject.GetChildren();
|
|
// if (ol != null)
|
|
// {
|
|
// foreach (SectionInfo o in ol)
|
|
// {
|
|
// try
|
|
// {
|
|
// VESection tmp = new VESection();
|
|
// tmp.VEObject = o;
|
|
// if (dicSect.ContainsKey(o.PPath))
|
|
// dicSect[o.PPath].Nodes.Add(tmp);
|
|
// else
|
|
// this.Nodes.Add(tmp);
|
|
// dicSect[o.Path] = tmp;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
// }
|
|
// }
|
|
// }
|
|
// _ChildrenLoaded = true;
|
|
// }
|
|
//}
|
|
}
|
|
public class VESection : VETreeNodeBase<VEStep> { }
|
|
public class VEStep : VETreeNodeBase<VEStep> { }
|
|
*/
|
|
//public class VETree : VETreeNodeBase<VETree>
|
|
//{
|
|
// public VETree() { ;}
|
|
// public static VETree GetFolder(int folderID)
|
|
// {
|
|
// return new VETree(FolderInfo.Get(folderID));
|
|
// }
|
|
// //private VEFolder(string s) : base(s) { ;}
|
|
// public VETree(IVEReadOnlyItem o) : base(o) { ;}
|
|
//}
|
|
//public class VETree : VETreeNode
|
|
//{
|
|
// public VETree() { ;}
|
|
// public static VETree GetFolder(int folderID)
|
|
// {
|
|
// VETree tn = new VETree(FolderInfo.Get(folderID));
|
|
// tn.ResetNode();
|
|
// return tn;
|
|
// }
|
|
// //private VEFolder(string s) : base(s) { ;}
|
|
// public VETree(IVEReadOnlyItem o) : base(o) { ;}
|
|
// private DateTime tNext(DateTime tStart, string msg)
|
|
// {
|
|
// DateTime tEnd = DateTime.Now;
|
|
// TimeSpan ts = new TimeSpan(tEnd.Ticks - tStart.Ticks);
|
|
// Console.WriteLine("{0} - {1}", ts.TotalMilliseconds, msg);
|
|
// return tEnd;
|
|
// }
|
|
// public override void LoadChildren()
|
|
// {
|
|
// if (!_ChildrenLoaded)
|
|
// {
|
|
// this.Nodes.Clear();
|
|
// "".TrimEnd("\r\n".ToCharArray());
|
|
// IList ol = _VEObject.GetChildren();
|
|
// if (ol != null)
|
|
// {
|
|
// this.TreeView.BeginUpdate();
|
|
// DateTime tStart = DateTime.Now;
|
|
// //VETree[] tmpa = new VETree[ol.Count];
|
|
// //tStart = tNext(tStart, "Allocate Array");
|
|
// ////for (int i = 0; i < ol.Count; i++) tmpa[i] = new VETree();
|
|
// ////tStart = tNext(tStart, "Allocate Nodes");
|
|
// //int ii = 0;
|
|
// //foreach (IVEReadOnlyItem o in ol)
|
|
// //{
|
|
// // try
|
|
// // {
|
|
// // tmpa[ii++] = new VETree(o);
|
|
// // //tmpa[ii++].VEObject = o;
|
|
// // }
|
|
// // catch (Exception ex)
|
|
// // {
|
|
// // Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
// // }
|
|
// //}
|
|
// //tStart = tNext(tStart, "Set Nodes");
|
|
// //for (int i = 0; i < ol.Count; i++) tmpa[i].ResetNode();
|
|
// //tStart = tNext(tStart, "Reset Nodes");
|
|
// //this.Nodes.AddRange(tmpa);
|
|
// //tStart = tNext(tStart, "Add Range");
|
|
// this.TreeView.DrawMode = TreeViewDrawMode.OwnerDrawAll;
|
|
// foreach (IVEReadOnlyItem o in ol)
|
|
// {
|
|
// try
|
|
// {
|
|
// VETree tmp = new VETree(o);
|
|
// //tmp.VEObject = o;
|
|
// this.Nodes.Add(tmp);
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// Console.WriteLine("{0}\r\n{1}", ex.Message, ex.InnerException);
|
|
// }
|
|
// }
|
|
// tStart = tNext(tStart, "Set Nodes");
|
|
// this.TreeView.DrawMode = TreeViewDrawMode.Normal;
|
|
// tStart = tNext(tStart, "DrawMode");
|
|
// this.TreeView.EndUpdate();
|
|
// tStart = tNext(tStart, "End Update");
|
|
// this.TreeView.Refresh();
|
|
// tStart = tNext(tStart, "Refresh");
|
|
// }
|
|
// _ChildrenLoaded = true;
|
|
// }
|
|
// }
|
|
//}
|
|
}
|