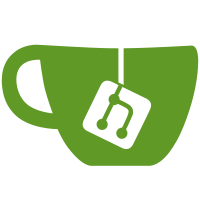
Added PurgeDataCommand class based on CSLA CommandBase class Added CanTransitionBeCreatedCommand class based on CSLA CommandBase class Added WillTransitionsBeValidCommand class based on CSLA CommandBase class Added InvalidTransition class Added ProposedTransition class Changed NodeText_Changed method to support multi unit Added BigNum class to support applicability for multi unit
567 lines
18 KiB
C#
567 lines
18 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Diagnostics;
|
|
using System.Collections.Generic;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// Database Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
public static partial class Database
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
private static int _DefaultTimeout = 600; // 600 seconds, i.e. 10 minutes
|
|
|
|
public static int DefaultTimeout
|
|
{
|
|
get { return _DefaultTimeout; }
|
|
set { _DefaultTimeout = value; }
|
|
}
|
|
|
|
public static void LogException(string s, Exception ex)
|
|
{
|
|
int i = 0;
|
|
Console.WriteLine("Error - {0}", s);
|
|
for (; ex != null; ex = ex.InnerException)
|
|
{
|
|
Console.WriteLine("{0}{1} - {2}", "".PadLeft(++i * 2), ex.GetType().ToString(), ex.Message);
|
|
}
|
|
}
|
|
private static bool _LoggingInfo = false; // By default don't log info
|
|
public static bool LoggingInfo
|
|
{
|
|
get { return _LoggingInfo; }
|
|
set { _LoggingInfo = value; }
|
|
}
|
|
static System.Diagnostics.Process _CurrentProcess = System.Diagnostics.Process.GetCurrentProcess();
|
|
public static void LogInfo(string s, int hashCode)
|
|
{
|
|
if (_LoggingInfo)
|
|
Console.WriteLine("{0} MB {1}", _CurrentProcess.WorkingSet64 / 1000000, string.Format(s, hashCode));
|
|
}
|
|
public static void LogDebug(string s, int hashCode)
|
|
{
|
|
if (_LoggingInfo)
|
|
Console.WriteLine("{0} MB {1}", _CurrentProcess.WorkingSet64 / 1000000, string.Format(s, hashCode));
|
|
}
|
|
private static string _ConnectionName = "VEPROMS";
|
|
public static string ConnectionName
|
|
{
|
|
get { return Database._ConnectionName; }
|
|
set { Database._ConnectionName = value; _VEPROMS_Connection = null; /* Reset Connection */ }
|
|
}
|
|
private static string _VEPROMS_Connection;
|
|
public static string VEPROMS_Connection
|
|
{
|
|
get
|
|
{
|
|
if (_VEPROMS_Connection != null) // Use Lazy Load
|
|
return _VEPROMS_Connection;
|
|
DateTime.Today.ToLongDateString();
|
|
// If DBConnection.XML exists, use the connection string from DBConnection.XML
|
|
string cnOverride = System.Windows.Forms.Application.StartupPath + @"\DBConnection.XML";
|
|
if (System.IO.File.Exists(cnOverride))
|
|
{
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.Load(cnOverride);
|
|
System.Xml.XmlNode xn = xd.SelectSingleNode("DBConnection/@string");
|
|
if (xn != null)
|
|
{
|
|
return _VEPROMS_Connection = xn.InnerText;
|
|
}
|
|
}
|
|
// Otherwise get the value from the ConfigurationManager
|
|
ConnectionStringSettings cs = ConfigurationManager.ConnectionStrings[ConnectionName];
|
|
if (cs == null)
|
|
{
|
|
throw new ApplicationException("Database.cs Could not find connection " + ConnectionName);
|
|
}
|
|
string constr = cs.ConnectionString;
|
|
if (constr.Contains("{MENU}"))
|
|
{
|
|
constr = ChooseDatabase(constr);
|
|
}
|
|
return _VEPROMS_Connection = constr;
|
|
}
|
|
set { _VEPROMS_Connection = value; }
|
|
}
|
|
private static string _SelectedDatabase;
|
|
public static string SelectedDatabase
|
|
{
|
|
get { return Database._SelectedDatabase; }
|
|
set { Database._SelectedDatabase = value; }
|
|
}
|
|
private static string _LastDatabase="NoDefault";
|
|
public static string LastDatabase
|
|
{
|
|
get { return Database._LastDatabase; }
|
|
set { Database._LastDatabase = value; }
|
|
}
|
|
private static string ChooseDatabase(string constr)
|
|
{
|
|
if (_SelectedDatabase == null)
|
|
{
|
|
if (LastDatabase != "NoDefault" && LastDatabase != "")
|
|
{
|
|
if (System.Windows.Forms.MessageBox.Show("Open " + LastDatabase, "Reopen Last Database", System.Windows.Forms.MessageBoxButtons.YesNo, System.Windows.Forms.MessageBoxIcon.Question) == System.Windows.Forms.DialogResult.Yes)
|
|
{
|
|
SelectedDatabase = LastDatabase;
|
|
return constr.Replace("{MENU}", _SelectedDatabase);
|
|
}
|
|
}
|
|
System.Windows.Forms.ContextMenuStrip cms = BuildDatabaseMenu(constr);
|
|
while (_SelectedDatabase == null)
|
|
{
|
|
cms.Show(new System.Drawing.Point((System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Width - cms.Width) / 2, (System.Windows.Forms.Screen.PrimaryScreen.WorkingArea.Height - cms.Height) / 2));
|
|
System.Windows.Forms.Application.DoEvents();
|
|
}
|
|
}
|
|
return constr.Replace("{MENU}", _SelectedDatabase);
|
|
}
|
|
private static System.Windows.Forms.ContextMenuStrip BuildDatabaseMenu(string constr)
|
|
{
|
|
string tmp = constr.Replace("{MENU}", "master");
|
|
SqlConnection cn = new SqlConnection(tmp);
|
|
cn.Open();
|
|
// SqlDataAdapter da = new SqlDataAdapter("select name from sysdatabases where name like 'VEP%' order by name", cn);
|
|
//SqlDataAdapter da = new SqlDataAdapter("select name, case when object_id('[' + name + ']..Items') is null then 'Not PROMS' when object_id('[' + name + ']..Revisions') is not null then 'Approval' when object_id('[' + name + ']..ContentAudits') is not null then 'Change Manager' else 'Original' end functionality from sysdatabases where name not in ('master','model','msdb','tempdb') order by name", cn);
|
|
SqlDataAdapter da = new SqlDataAdapter("select name, 'Approval' functionality from sysdatabases where name not in ('master','model','msdb','tempdb') order by name", cn);
|
|
da.SelectCommand.CommandTimeout = 300; // 300 sec timeout
|
|
DataSet ds = new DataSet();
|
|
try
|
|
{
|
|
da.Fill(ds);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
System.Windows.Forms.MessageBox.Show(ex.GetType().Name, ex.Message);
|
|
throw(new Exception("Cannot Load Data List",ex));
|
|
}
|
|
cn.Close();
|
|
System.Windows.Forms.ContextMenuStrip cms = new System.Windows.Forms.ContextMenuStrip();
|
|
cms.Items.Add("Choose Database");
|
|
System.Windows.Forms.ToolStripMenuItem tsmi = cms.Items[0] as System.Windows.Forms.ToolStripMenuItem;
|
|
tsmi.BackColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaption);// System.Drawing.Color.Pink;
|
|
tsmi.ForeColor = System.Drawing.Color.FromKnownColor(System.Drawing.KnownColor.ActiveCaptionText);
|
|
tsmi.Font = new System.Drawing.Font(tsmi.Font, System.Drawing.FontStyle.Bold);
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
{
|
|
if (dr["functionality"].ToString() == "Approval")
|
|
cms.Items.Add(dr["name"].ToString(), null, new EventHandler(Database_Click));
|
|
}
|
|
return cms;
|
|
}
|
|
|
|
static void Database_Click(object sender, EventArgs e)
|
|
{
|
|
System.Windows.Forms.ToolStripMenuItem tsmi = sender as System.Windows.Forms.ToolStripMenuItem;
|
|
if (tsmi != null)
|
|
{
|
|
_SelectedDatabase = tsmi.Text;
|
|
}
|
|
}
|
|
|
|
public static SqlConnection VEPROMS_SqlConnection
|
|
{
|
|
get
|
|
{
|
|
string strConn = VEPROMS_Connection; // If failure - Fail (Don't try to catch)
|
|
// Attempt to make a connection
|
|
try
|
|
{
|
|
SqlConnection cn = new SqlConnection(strConn);
|
|
cn.Open();
|
|
return cn;
|
|
}
|
|
catch (SqlException exsql)
|
|
{
|
|
const string strAttachError = "An attempt to attach an auto-named database for file ";
|
|
if (exsql.Message.StartsWith(strAttachError))
|
|
{// Check to see if the file is missing
|
|
string sFile = exsql.Message.Substring(strAttachError.Length);
|
|
sFile = sFile.Substring(0, sFile.IndexOf(" failed"));
|
|
// "An attempt to attach an auto-named database for file <mdf file> failed"
|
|
if (strConn.ToLower().IndexOf("user instance=true") < 0)
|
|
{
|
|
throw new ApplicationException("Connection String missing attribute: User Instance=True");
|
|
}
|
|
if (System.IO.File.Exists(sFile))
|
|
{
|
|
throw new ApplicationException("Database file " + sFile + " Cannot be opened\r\n", exsql);
|
|
}
|
|
else
|
|
{
|
|
throw new FileNotFoundException("Database file " + sFile + " Not Found", exsql);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
throw new ApplicationException("Failure on Connect", exsql);
|
|
}
|
|
}
|
|
catch (Exception ex)// Throw Application Exception on Failure
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Connection Error", ex);
|
|
throw new ApplicationException("Failure on Connect", ex);
|
|
}
|
|
}
|
|
}
|
|
public static void PurgeData()
|
|
{
|
|
try
|
|
{
|
|
//SqlConnection cn = VEPROMS_SqlConnection;
|
|
//SqlCommand cmd = new SqlCommand("purgedata", cn);
|
|
using (SqlConnection cn = VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand("purgedata", cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
cmd.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Purge Error", ex);
|
|
throw new ApplicationException("Failure on Purge", ex);
|
|
}
|
|
}
|
|
}
|
|
public class DbCslaException : Exception
|
|
{
|
|
internal DbCslaException(string message, Exception innerException) : base(message, innerException) { ;}
|
|
internal DbCslaException(string message) : base(message) { ;}
|
|
internal DbCslaException() : base() { ;}
|
|
} // Class
|
|
#region commandbase object jcb
|
|
[Serializable()]
|
|
public class PurgeDataCommand : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private int _RowsAffected;
|
|
public int RowsAffected
|
|
{
|
|
get { return _RowsAffected; }
|
|
set { _RowsAffected = value; }
|
|
}
|
|
#region Factory Methods
|
|
public static int Execute()
|
|
{
|
|
PurgeDataCommand cmd = new PurgeDataCommand();
|
|
cmd = DataPortal.Execute<PurgeDataCommand>(cmd);
|
|
return cmd.RowsAffected;
|
|
}
|
|
private PurgeDataCommand()
|
|
{ /* require use of factory methods */ }
|
|
#endregion
|
|
#region Server-Side code
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
//SqlConnection cn = VEPROMS_SqlConnection;
|
|
//SqlCommand cmd = new SqlCommand("purgedata", cn);
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand("purgedata", cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.CommandTimeout = 0;
|
|
RowsAffected = cmd.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Purge Error", ex);
|
|
throw new ApplicationException("Failure on Purge", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
[Serializable()]
|
|
public class CanTransitionBeCreatedCommand : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
private ProposedTransition _ProposedTransition;
|
|
public ProposedTransition ProposedTransition
|
|
{
|
|
get { return _ProposedTransition; }
|
|
set { _ProposedTransition = value; }
|
|
}
|
|
private int _FromID;
|
|
public int FromID
|
|
{
|
|
get { return _FromID; }
|
|
set { _FromID = value; }
|
|
}
|
|
private int _ToID;
|
|
public int ToID
|
|
{
|
|
get { return _ToID; }
|
|
set { _ToID = value; }
|
|
}
|
|
#region Factory Methods
|
|
public static ProposedTransition Execute(int fromID, int toID)
|
|
{
|
|
CanTransitionBeCreatedCommand cmd = new CanTransitionBeCreatedCommand();
|
|
cmd.FromID = fromID;
|
|
cmd.ToID = toID;
|
|
cmd = DataPortal.Execute<CanTransitionBeCreatedCommand>(cmd);
|
|
return cmd.ProposedTransition;
|
|
}
|
|
private CanTransitionBeCreatedCommand()
|
|
{ /* require use of factory methods */ }
|
|
#endregion
|
|
#region Server-Side code
|
|
private void ReadData(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] CanTransitionBeCreatedCommand.ReadData", GetHashCode());
|
|
try
|
|
{
|
|
_ProposedTransition = new ProposedTransition(dr.GetInt32("status"), dr.GetString("fromappl"), dr.GetString("toappl"), dr.GetString("fromstep"), dr.GetString("tostep"));
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Detail.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("CanTransitionBeCreatedCommand.ReadData", ex);
|
|
}
|
|
}
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
//SqlConnection cn = VEPROMS_SqlConnection;
|
|
//SqlCommand cmd = new SqlCommand("purgedata", cn);
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand("vesp_CanTransitionBeCreated", cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.Parameters.AddWithValue("fromItemID", _FromID);
|
|
cmd.Parameters.AddWithValue("toItemID", _ToID);
|
|
using (SafeDataReader dr = new SafeDataReader(cmd.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("CanTransitionBeCreatedCommand Error", ex);
|
|
throw new ApplicationException("Failure on CanTransitionBeCreatedCommand", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
[Serializable()]
|
|
public class WillTransitionsBeValidCommand : CommandBase
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
private List<InvalidTransition> _InvalidTransitions;
|
|
public List<InvalidTransition> InvalidTransitions
|
|
{
|
|
get { return _InvalidTransitions; }
|
|
}
|
|
private int _ItemID;
|
|
public int ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
private string _NewAppl;
|
|
public string NewAppl
|
|
{
|
|
get { return _NewAppl; }
|
|
set { _NewAppl = value; }
|
|
}
|
|
#region Factory Methods
|
|
public static List<InvalidTransition> Execute(int itemID, string newAppl)
|
|
{
|
|
WillTransitionsBeValidCommand cmd = new WillTransitionsBeValidCommand();
|
|
cmd.ItemID = itemID;
|
|
cmd.NewAppl = newAppl;
|
|
cmd = DataPortal.Execute<WillTransitionsBeValidCommand>(cmd);
|
|
return cmd.InvalidTransitions;
|
|
}
|
|
private WillTransitionsBeValidCommand()
|
|
{ /* require use of factory methods */ }
|
|
#endregion
|
|
#region Server-Side code
|
|
private void ReadData(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Detail.ReadData", GetHashCode());
|
|
try
|
|
{
|
|
do
|
|
{
|
|
_InvalidTransitions.Add(new InvalidTransition(dr.GetInt32("myitemid"), dr.GetString("srcappl"), dr.GetString("tgtappl"), dr.GetString("srcstep"), dr.GetString("tgtstep")));
|
|
} while (dr.Read());
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("WillTransitionsBeValidCommand.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("WillTransitionsBeValidCommand.ReadData", ex);
|
|
}
|
|
}
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
try
|
|
{
|
|
//SqlConnection cn = VEPROMS_SqlConnection;
|
|
//SqlCommand cmd = new SqlCommand("purgedata", cn);
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cmd = new SqlCommand("vesp_WillTransitionsBeValid", cn))
|
|
{
|
|
cmd.CommandType = CommandType.StoredProcedure;
|
|
cmd.Parameters.AddWithValue("ItemID", _ItemID);
|
|
cmd.Parameters.AddWithValue("NewAppl", _NewAppl);
|
|
using (SafeDataReader dr = new SafeDataReader(cmd.ExecuteReader()))
|
|
{
|
|
_InvalidTransitions = new List<InvalidTransition>();
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("WillTransitionsBeValidCommand Error", ex);
|
|
throw new ApplicationException("Failure on WillTransitionsBeValidCommand", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
[Serializable()]
|
|
public class InvalidTransition
|
|
{
|
|
private int _MyItemID;
|
|
public int MyItemID
|
|
{
|
|
get { return _MyItemID; }
|
|
set { _MyItemID = value; }
|
|
}
|
|
private string _SrcAppl;
|
|
public string SrcAppl
|
|
{
|
|
get { return _SrcAppl; }
|
|
set { _SrcAppl = value; }
|
|
}
|
|
private string _TgtAppl;
|
|
public string TgtAppl
|
|
{
|
|
get { return _TgtAppl; }
|
|
set { _TgtAppl = value; }
|
|
}
|
|
private string _SrcStep;
|
|
public string SrcStep
|
|
{
|
|
get { return _SrcStep; }
|
|
set { _SrcStep = value; }
|
|
}
|
|
private string _TgtStep;
|
|
public string TgtStep
|
|
{
|
|
get { return _TgtStep; }
|
|
set { _TgtStep = value; }
|
|
}
|
|
public InvalidTransition(int myItemID, string srcAppl, string tgtAppl, string srcStep, string tgtStep)
|
|
{
|
|
_MyItemID = myItemID;
|
|
_SrcAppl = srcAppl;
|
|
_TgtAppl = tgtAppl;
|
|
_SrcStep = srcStep;
|
|
_TgtStep = tgtStep;
|
|
}
|
|
}
|
|
[Serializable()]
|
|
public class ProposedTransition
|
|
{
|
|
private int _Status;
|
|
public int Status
|
|
{
|
|
get { return _Status; }
|
|
set { _Status = value; }
|
|
}
|
|
private string _FromAppl;
|
|
public string FromAppl
|
|
{
|
|
get { return _FromAppl; }
|
|
set { _FromAppl = value; }
|
|
}
|
|
private string _ToAppl;
|
|
public string ToAppl
|
|
{
|
|
get { return _ToAppl; }
|
|
set { _ToAppl = value; }
|
|
}
|
|
private string _FromStep;
|
|
public string FromStep
|
|
{
|
|
get { return _FromStep; }
|
|
set { _FromStep = value; }
|
|
}
|
|
private string _ToStep;
|
|
public string ToStep
|
|
{
|
|
get { return _ToStep; }
|
|
set { _ToStep = value; }
|
|
}
|
|
public ProposedTransition(int status, string fromAppl, string toAppl, string fromStep, string toStep)
|
|
{
|
|
_Status = status;
|
|
_FromAppl = fromAppl;
|
|
_ToAppl = toAppl;
|
|
_FromStep = fromStep;
|
|
_ToStep = toStep;
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|