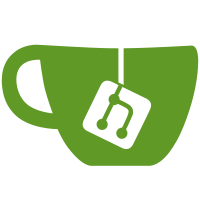
1. Open Properly if all tabs are closed 2. Open to a full window view 3. Set Current Item in the Tab Control Fixed Display Panel so that when you click on an item in an inactive panel, the item clicked-on become the selected item.
111 lines
3.0 KiB
C#
111 lines
3.0 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayTabItem : DevComponents.DotNetBar.DockContainerItem
|
|
{
|
|
private DisplayTabControl _MyTabControl;
|
|
private ItemInfo _MyItem;
|
|
|
|
public ItemInfo MyItem
|
|
{
|
|
get { return _MyItem; }
|
|
set { _MyItem = value; }
|
|
}
|
|
private DisplayTabPanel _MyTabPanel;
|
|
private string _MyKey;
|
|
public string MyKey
|
|
{
|
|
get { return _MyKey; }
|
|
}
|
|
public DisplayTabPanel MyTabPanel
|
|
{
|
|
get { return _MyTabPanel; }
|
|
set { _MyTabPanel = value; }
|
|
}
|
|
private DSOTabPanel _MyDSOTabPanel;
|
|
public DSOTabPanel MyDSOTabPanel
|
|
{
|
|
get { return _MyDSOTabPanel; }
|
|
set { _MyDSOTabPanel = value; }
|
|
}
|
|
public ItemInfo ItemSelected
|
|
{
|
|
get { return _MyTabPanel.ItemSelected; }
|
|
set { _MyTabPanel.ItemSelected = value; }
|
|
}
|
|
public DisplayTabItem(IContainer container, DisplayTabControl myTabControl, ItemInfo myItem, string myKey)
|
|
{
|
|
_MyKey = myKey;
|
|
_MyTabControl = myTabControl;
|
|
_MyItem = myItem;
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.Click += new EventHandler(DisplayTabItem_Click);
|
|
if (myItem.MyContent.MyEntry == null)
|
|
SetupDisplayPanel();
|
|
else
|
|
SetupDSOPanel();
|
|
Name = string.Format("DisplayTabItem {0}", myItem.ItemID);
|
|
}
|
|
void DisplayTabItem_Click(object sender, EventArgs e)
|
|
{
|
|
// See if I can tell the TabControl that the ItemSelected has changed
|
|
DisplayTabItem myTabItem = sender as DisplayTabItem;
|
|
if(myTabItem == null)return;
|
|
DisplayTabPanel myTabPanel = myTabItem.MyTabPanel as DisplayTabPanel;
|
|
if(myTabPanel == null) return;
|
|
_MyTabControl.OnItemSelectedChanged(this,new DisplayPanelEventArgs(MyTabPanel.SelectedItem,null));
|
|
}
|
|
private void SetupDisplayPanel()
|
|
{
|
|
((System.ComponentModel.ISupportInitialize)(_MyTabControl.MyBar)).BeginInit();
|
|
_MyTabControl.MyBar.SuspendLayout();
|
|
_MyTabPanel = new DisplayTabPanel(_MyTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyTabPanel;
|
|
Name = "tabItem Item " + _MyItem.ItemID;
|
|
Text = _MyItem.TabTitle;
|
|
Tooltip = _MyItem.TabToolTip;
|
|
//
|
|
_MyTabControl.Controls.Add(_MyTabPanel);
|
|
_MyTabControl.MyBar.Items.Add(this);
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyTabPanel.TabItem = this;
|
|
((System.ComponentModel.ISupportInitialize)(_MyTabControl.MyBar)).EndInit();
|
|
_MyTabControl.MyBar.ResumeLayout(false);
|
|
}
|
|
private void SetupDSOPanel()
|
|
{
|
|
EntryInfo myEntry = _MyItem.MyContent.MyEntry;
|
|
_MyDSOTabPanel = new DSOTabPanel(myEntry.MyDocument, _MyTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyDSOTabPanel;
|
|
Name = "tabItem Item " + _MyItem.ItemID;
|
|
Text = _MyItem.TabTitle;
|
|
Tooltip = _MyItem.TabToolTip;
|
|
//
|
|
_MyTabControl.Controls.Add(_MyDSOTabPanel);
|
|
_MyTabControl.MyBar.Items.AddRange(new DevComponents.DotNetBar.BaseItem[] {
|
|
this});
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyTabControl.SelectedTab = this;
|
|
_MyDSOTabPanel.TabItem = this;
|
|
}
|
|
}
|
|
}
|