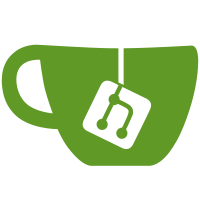
Made call to vesp_TurnChangeManagerOFF prior to converting a database and a call to vesp_TurnChangeManagerON after converting a database to improve adding 16-bit data to an existing 32-bit database Made call to vesp_TurnChangeManagerOFF prior to fixing transitions and a call to vesp_TurnChangeManagerON after fixing transitions to improve adding 16-bit data to an existing 32-bit database Modified PartialMatch method to handle cases when XTSETID.DBF entry for abbyproc does not have the .prc extension Modified ExactMatch method to handle cases when XTSETID.DBF entry for abbyproc does not have the .prc extension Added stored procedure stubs for vesp_TurnChangeManagerOFF and vesp_TurnChangeManagerON
1103 lines
39 KiB
C#
1103 lines
39 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.Configuration;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using Volian.MSWord;
|
|
using vlnObjectLibrary;
|
|
using vlnServerLibrary;
|
|
using VEPROMS.CSLA.Library;
|
|
using Config;
|
|
using Volian.Controls.Library;
|
|
using Volian.Base.Library;
|
|
using System.Data.SqlClient;
|
|
|
|
|
|
[assembly: log4net.Config.XmlConfigurator(Watch = true)]
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class frmLoader : Form
|
|
{
|
|
#region Log4Net
|
|
public static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region settings
|
|
private bool _FormatsOnly = false;
|
|
public bool FormatsOnly
|
|
{
|
|
get { return _FormatsOnly; }
|
|
set { _FormatsOnly = value; }
|
|
}
|
|
#endregion
|
|
private bool _Loading = true;
|
|
|
|
private FolderTreeNode _topnode;
|
|
private bool UseVeTree = false;
|
|
private Loader ldr;
|
|
public TreeView TV { get { return tv; } }
|
|
public int pbProcMaximum { get { return pbProc.Maximum; } set { pbProc.Maximum = value; } }
|
|
public int pbSectMaximum { get { return pbSect.Maximum; } set { pbSect.Maximum = value; } }
|
|
public int pbSectValue { get { return pbSect.Value; } set { pbSect.Value = value; } }
|
|
public int pbStepMaximum { get { return pbStep.Maximum; } set { pbStep.Maximum = value; } }
|
|
public int pbStepValue { get { return pbStep.Value; } set { pbStep.Value = value; } }
|
|
public int pbProcValue { get { return pbProc.Value; } set { pbProc.Value = value; } }
|
|
public int SkipProcedures
|
|
{
|
|
get { return MySettings.Skip; }
|
|
}
|
|
public string Status
|
|
{
|
|
get { return toolStripStatusLabel1.Text; }
|
|
set
|
|
{
|
|
toolStripStatusLabel1.Text = value;
|
|
Application.DoEvents();
|
|
}
|
|
}
|
|
private frmErrors _MyFrmErrors = null;
|
|
public frmErrors MyFrmErrors
|
|
{
|
|
get
|
|
{
|
|
if (_MyFrmErrors == null)
|
|
{
|
|
_MyFrmErrors = new frmErrors(this);
|
|
_MyFrmErrors.FormClosing += new FormClosingEventHandler(_MyFrmErrors_FormClosing);
|
|
}
|
|
return _MyFrmErrors;
|
|
}
|
|
}
|
|
void _MyFrmErrors_FormClosing(object sender, FormClosingEventArgs e)
|
|
{
|
|
_MyFrmErrors = null;
|
|
}
|
|
public string MyError
|
|
{
|
|
get { return tsslError.Text; }
|
|
set
|
|
{
|
|
MyFrmErrors.Add(value, MessageType.Error);
|
|
_MyLog.ErrorFormat(value);
|
|
tsslError.Text = string.Format("{0} Errors", MyFrmErrors.ErrorCount);
|
|
}
|
|
}
|
|
public string MyWarning
|
|
{
|
|
get { return tsslError.Text; }
|
|
set
|
|
{
|
|
MyFrmErrors.Add(value, MessageType.Warning);
|
|
_MyLog.WarnFormat(value);
|
|
//tsslError.Text = string.Format("{0} Errors", MyFrmErrors.ErrorCount);
|
|
}
|
|
}
|
|
public string MyInfo
|
|
{
|
|
get { return tsslError.Text; }
|
|
set
|
|
{
|
|
MyFrmErrors.Add(value, MessageType.Information);
|
|
_MyLog.Info(value);
|
|
//tsslError.Text = string.Format("{0} Errors", MyFrmErrors.ErrorCount);
|
|
}
|
|
}
|
|
public void AddError(string format, params object[] objs)
|
|
{
|
|
MyError = string.Format(format, objs);
|
|
}
|
|
public void AddWarn(string format, params object[] objs)
|
|
{
|
|
MyWarning = string.Format(format, objs);
|
|
}
|
|
public void AddInfo(string format, params object[] objs)
|
|
{
|
|
MyInfo = string.Format(format, objs);
|
|
}
|
|
public void AddError(Exception ex, string format, params object[] objs)
|
|
{
|
|
StringBuilder sb = new StringBuilder(string.Format(format, objs));
|
|
int indent = 0;
|
|
while (ex != null)
|
|
{
|
|
sb.Append("\r\n" + "".PadRight((++indent) * 2, ' ') + string.Format("{0} - {1}", ex.GetType().Name, ex.Message));
|
|
sb.Append(ex.StackTrace);
|
|
ex = ex.InnerException;
|
|
}
|
|
MyError = sb.ToString();
|
|
}
|
|
|
|
public frmLoader()
|
|
{
|
|
ldr = new Loader(_MyLog, this);
|
|
InitializeComponent();
|
|
MSWordToPDF.FormForPlotGraphics = this;
|
|
lblTime.Tag = DateTime.Now;
|
|
}
|
|
private void btnConvertSelected_Click(object sender, EventArgs e)
|
|
{
|
|
if (UseVeTree)
|
|
{
|
|
VETreeNode tn = (VETreeNode)tv.SelectedNode;
|
|
if (tn == null)
|
|
{
|
|
MessageBox.Show("Must select a version node (working draft, approved, etc)", "No Node Selected");
|
|
return;
|
|
}
|
|
object o = tn.VEObject;
|
|
if (o.GetType() != typeof(DocVersionInfo))
|
|
{
|
|
MessageBox.Show("Must select a version node (working draft, approved, etc)", "No Node Selected");
|
|
return;
|
|
}
|
|
DocVersion v = ((DocVersionInfo)o).Get();
|
|
ldr.MigrateDocVersion(v);
|
|
if (v.MyItem != null)
|
|
{
|
|
tn.Checked = true;
|
|
}
|
|
|
|
}
|
|
else
|
|
{
|
|
TreeNode tn = tv.SelectedNode;
|
|
if (tn == null)
|
|
{
|
|
MessageBox.Show("Must select a version node (working draft, approved, etc)", "No Node Selected");
|
|
return;
|
|
}
|
|
object o = tn.Tag;
|
|
if (o.GetType() != typeof(DocVersion))
|
|
{
|
|
MessageBox.Show("Must select a version node (working draft, approved, etc)", "No Node Selected");
|
|
return;
|
|
}
|
|
DocVersion v = (DocVersion)o;
|
|
ldr.MigrateDocVersion(v);
|
|
if (v.MyItem != null)
|
|
{
|
|
tn.Checked = true;
|
|
}
|
|
}
|
|
}
|
|
private void tv_BeforeExpand(object sender, TreeViewCancelEventArgs e)
|
|
{
|
|
if (UseVeTree)
|
|
{
|
|
((VETreeNode)e.Node).LoadChildren();
|
|
return;
|
|
}
|
|
|
|
TreeNode tn = e.Node;
|
|
object o = tn.Tag;
|
|
switch (o.GetType().ToString())
|
|
{
|
|
case "Volian.CSLA.Library.FolderInfo":
|
|
FolderInfo fld = (FolderInfo)o;
|
|
if (fld.ChildFolderCount > 0)
|
|
tn.Checked = ldr.LoadChildren(fld, tn); // load docversions.
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
}
|
|
private void tv_AfterSelect(object sender, TreeViewEventArgs e)
|
|
{
|
|
if (UseVeTree) return;
|
|
|
|
TreeNode tn = e.Node;
|
|
object o = tn.Tag;
|
|
tn.Expand();
|
|
if (o.GetType() == typeof(DocVersion)) MySettings.ProcedureSetPath = ((DocVersion)o).Title;
|
|
}
|
|
private void btnLoadTreeDB_Click(object sender, EventArgs e)
|
|
{
|
|
// When loading folders, i.e. the tree from dBase (old 16-bit)
|
|
// always clear the data
|
|
ldr.ClearData();
|
|
bool suc = ldr.LoadFolders(MySettings.VEPromsPath);
|
|
}
|
|
private string GetScript(string scriptName)
|
|
{
|
|
StreamReader sr = File.OpenText(Application.StartupPath + "\\" + scriptName);
|
|
string myScript = sr.ReadToEnd();
|
|
sr.Close();
|
|
return myScript;
|
|
}
|
|
private DateTime _ProcessTime;
|
|
public DateTime ProcessTime
|
|
{
|
|
get { return _ProcessTime; }
|
|
set
|
|
{
|
|
_ProcessTime = value;
|
|
// Set the Log File Name when the ProcessTime is set
|
|
ChangeLogFileName("LogFileAppender", MySettings.DBName + " " + ProcessTime.ToString("yyyyMMdd HHmmss") + " DMErrorLog.txt");
|
|
}
|
|
}
|
|
private void btnConvert_Click(object sender, System.EventArgs e)
|
|
{
|
|
// Set Connection String
|
|
Database.VEPROMS_Connection = MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName);
|
|
// Setup based upon RedPDF Setting
|
|
// if in debug mode, pdf output is red
|
|
VlnSettings.DebugMode = (MySettings.ExecutionMode == ExecutionMode.Debug) && MySettings.RedPDFs;
|
|
if (VlnSettings.DebugMode) // Debug Mode
|
|
{
|
|
MSWordToPDF.DebugStatus = 1;
|
|
Loader.OverrideColor = Color.Red;
|
|
}
|
|
else
|
|
{
|
|
MSWordToPDF.DebugStatus = 0;
|
|
Loader.OverrideColor = Color.Empty;
|
|
}
|
|
//if (!CheckLogPath()) return;
|
|
//#if (!DEBUG)
|
|
if (!VlnSettings.DebugMode)
|
|
{
|
|
DialogResult dlgrst = MessageBox.Show("The VE-PROMS data currently in SQL Server (Express) will be deleted.\r\n\nProceed with Data Conversion?", "WARNING", MessageBoxButtons.YesNo, MessageBoxIcon.Warning);
|
|
if (dlgrst == DialogResult.No) return;
|
|
}
|
|
//#endif
|
|
try
|
|
{
|
|
//TextConvert.ResetSpecialCharacters();
|
|
//MyFrmErrors.Clear();
|
|
System.Diagnostics.Process[] wordProcesses = WordDoc.WordProcesses;
|
|
if (!FormatsOnly && wordProcesses.Length > 0)
|
|
{
|
|
AddError("{0} copies of MS Word are running", wordProcesses.Length);
|
|
if (MessageBox.Show("MS Word is Running and must be stopped before proceeding.\n\nStop MS Word?", "MS Word is Running", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
WordDoc.TerminateProcesses(wordProcesses);
|
|
MyFrmErrors.Clear();
|
|
}
|
|
else
|
|
return;
|
|
}
|
|
Database.LoggingInfo = false;
|
|
bool success = true;
|
|
if (FormatsOnly)
|
|
{
|
|
// ASSUMES No Formats/genmacs exist in database.
|
|
//MessageBox.Show(@"Format files are taken from c:\development\fmtall");
|
|
//ldr._FmtAllPath = @"c:\development\fmtall";
|
|
//ldr._GenmacAllPath = @"c:\development\genmacall";
|
|
Format.UpdateFormats(MySettings.FormatFolder, MySettings.GenMacFolder);
|
|
//Format.UpdateFormats(@"c:\development\fmtall",@"c:\development\genmacall"); //ldr.LoadAllFormats();
|
|
MessageBox.Show("Formats Loaded");
|
|
return;
|
|
}
|
|
// Create Database
|
|
|
|
// if purge data, purge it all & reload folders & security.
|
|
if (MySettings.PurgeExistingData)
|
|
{
|
|
if(!RunScript("BuildVEPROMS.Sql", "Master")) return;
|
|
if(!RunScript("PROMS2010.SQL", MySettings.DBName)) return;
|
|
Status = "Purging Data";
|
|
ldr.ClearData();
|
|
Status = "Loading Folders";
|
|
success = ldr.LoadFolders(MySettings.VEPromsPath);
|
|
if (success)
|
|
{
|
|
Status = "Loading Security";
|
|
success = ldr.LoadSecurity(MySettings.VESamFile, MySettings.VEPromsPath);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// check if images have been zipped & if not, zip them:
|
|
ROImageInfo.ZipImages();
|
|
success = ldr.LoadFoldersIntoExisting(MySettings.VEPromsPath);
|
|
}
|
|
if (success)
|
|
{
|
|
TimeSpan ts = new TimeSpan();
|
|
DocVersionInfoList vl = DocVersionInfoList.Get();
|
|
//DocVersion v = null;
|
|
MyInfo = "Computer Name: " + SystemInformation.ComputerName.ToUpper();
|
|
foreach (DocVersionInfo vi in vl)
|
|
{
|
|
if (!MySettings.OnlyThisSet || (vi.Title.ToUpper() == MySettings.ProcedureSetPath.ToUpper())) // is this the procedure set we want to convert?
|
|
{
|
|
using (DocVersion v = DocVersion.Get(vi.VersionID))
|
|
{
|
|
//v = DocVersion.Get(vi.VersionID);
|
|
Status = "Load " + v.Title + " - " + v.Name;
|
|
lblCurSetFolder.Text = v.Title;
|
|
lblCurSetFolder.Visible = true;
|
|
lblProcessing.Visible = true;
|
|
MyInfo = "Data Set: " + v.Title;
|
|
ts += ldr.MigrateDocVersion(v, true);
|
|
}
|
|
}
|
|
}
|
|
string ConversionTime = string.Format("Conversion completion time: {0:D2}:{1:D2}:{2:D2}.{3}", ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds);
|
|
MyInfo = ConversionTime;
|
|
SaveLogFiles();
|
|
if (!ProcessComplete) MessageBox.Show(string.Format("{0}\r\n\n({1} Total Seconds)", ConversionTime, ts.TotalSeconds));
|
|
//MessageBox.Show(string.Format("Conversion completion time: {0:D2}:{1:D2}:{2:D2}.{3}\r\n\n({4} Total Seconds)", ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds, ts.TotalSeconds));
|
|
//MessageBox.Show(string.Format("{0} seconds", ts.TotalSeconds));
|
|
//TextConvert.ListSpecialCharacters();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
AddError(ex, "===================== Fatal Error ==========================\r\n{0} - {1}", ex.GetType().Name, ex.Message);
|
|
SaveLogFiles();
|
|
MessageBox.Show(ex.Message, "Fatal Error During Loading", MessageBoxButtons.OK, MessageBoxIcon.Error);
|
|
_MyLog.Fatal(ex.Message);
|
|
}
|
|
}
|
|
public bool RunScript(string scriptName, string dbName)
|
|
{
|
|
bool ok = false;
|
|
Status = String.Format("Running Script '{0}'", scriptName);
|
|
string script = GetScript(scriptName);
|
|
script = script.Replace("{DBName}", MySettings.DBName);
|
|
script = script.Replace("{DBPath}", MySettings.DBPath);
|
|
SQLScriptRunner ssr = new SQLScriptRunner(script, MySettings.ConnectionString.Replace("{DBName}", dbName));
|
|
ssr.InfoMessage += new SQLScriptRunnerEvent(ssr_InfoMessage);
|
|
try
|
|
{
|
|
ssr.Run();
|
|
Status = String.Format("Script '{0}' Complete", scriptName);
|
|
ok = true;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
AddInfo("====****====");
|
|
AddError(ex, "While processing database {0}", dbName);
|
|
AddInfo("====****====");
|
|
Status = String.Format("Script '{0}' Failed", scriptName);
|
|
}
|
|
return ok;
|
|
}
|
|
public bool RunScript(string script)
|
|
{
|
|
bool ok = false;
|
|
Status = String.Format("Running {0}", script);
|
|
script = script.Replace("{DBName}", MySettings.DBName);
|
|
script = script.Replace("{DBPath}", MySettings.DBPath);
|
|
SQLScriptRunner ssr = new SQLScriptRunner(script, MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName));
|
|
ssr.InfoMessage += new SQLScriptRunnerEvent(ssr_InfoMessage);
|
|
try
|
|
{
|
|
ssr.Run();
|
|
Status = "Script Complete";
|
|
ok = true;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
AddInfo("====****====");
|
|
AddError(ex, "While processing database {0}", MySettings.DBName);
|
|
AddInfo("====****====");
|
|
Status = String.Format("Script {0} Failed", script);
|
|
}
|
|
return ok;
|
|
}
|
|
private void Backup(string suffix)
|
|
{
|
|
SQLScriptRunner ssrbu = new SQLScriptRunner(MySettings.DBName, MySettings.BackupFolder,
|
|
MySettings.ConnectionString.Replace("{DBName}", "Master"), ProcessTime, suffix);
|
|
ssrbu.InfoMessage += new SQLScriptRunnerEvent(ssr_InfoMessage);
|
|
ssrbu.Run();
|
|
}
|
|
void ssr_InfoMessage(object sender, System.Data.SqlClient.SqlInfoMessageEventArgs args)
|
|
{
|
|
MyInfo = args.Message;
|
|
}
|
|
private bool _ShowSections = false;
|
|
public bool ShowSections
|
|
{
|
|
get { return _ShowSections; }
|
|
set { _ShowSections = value; }
|
|
}
|
|
public void UpdateLabels(int incPrc, int incSec, int incStp)
|
|
{
|
|
if (incPrc == 0 && incSec == 0 && incStp == 0)//Reset
|
|
{
|
|
pbProc.Value = 0;
|
|
pbSect.Value = 0;
|
|
pbStep.Value = 0;
|
|
}
|
|
else
|
|
{
|
|
try
|
|
{
|
|
pbProc.Value += incPrc;
|
|
pbSect.Value += incSec;
|
|
pbStep.Value += incStp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
_MyLog.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
AddError(ex, "UpdateLabels");
|
|
}
|
|
}
|
|
//Database.LoggingInfo = (pbProc.Value > 153 && pbSect.Value > 11 && pbStep.Value > 61);
|
|
lblProc.Text = string.Format("{0}/{1} Procedures", pbProc.Value, pbProc.Maximum);
|
|
if (ShowSections)
|
|
{
|
|
lblSection.Text = string.Format("{0}/{1} Sections", pbSect.Value, pbSect.Maximum);
|
|
lblStep.Text = string.Format("{0}/{1} Steps", pbStep.Value, pbStep.Maximum);
|
|
}
|
|
else
|
|
{
|
|
lblSection.Text = "";
|
|
lblStep.Text = "";
|
|
}
|
|
//pbProc.Value = iPrc;
|
|
//pbSect.Value = iSec;
|
|
//pbStep.Value = iStp;
|
|
TimeSpan ts = new TimeSpan(DateTime.Now.Ticks - ProcessTime.Ticks);
|
|
lblTime.Text = string.Format("{0:D2}:{1:D2}:{2:D2} Elapsed", ts.Hours, ts.Minutes, ts.Seconds);
|
|
Application.DoEvents();
|
|
}
|
|
private void btnLoadTreeCSLA_Click(object sender, EventArgs e)
|
|
{
|
|
_topnode = FolderTreeNode.BuildTreeList();
|
|
tv.Nodes.Add(_topnode);
|
|
tv.Nodes[0].Expand();
|
|
UseVeTree = false;
|
|
}
|
|
private void btnVesam_Click(object sender, EventArgs e)
|
|
{
|
|
// if purge data, purge it all & reload folders.
|
|
if (MySettings.PurgeExistingData)
|
|
{
|
|
ldr.ClearData();
|
|
ldr.LoadFolders(MySettings.VEPromsPath);
|
|
}
|
|
bool sec = ldr.LoadSecurity(MySettings.VESamFile, MySettings.VEPromsPath);
|
|
}
|
|
|
|
private void btnVETree_CSLA_Click(object sender, EventArgs e)
|
|
{
|
|
tv.Nodes.Add(VETreeNode.GetFolder(1));
|
|
UseVeTree = true;
|
|
}
|
|
|
|
private void btnGroup_Click(object sender, EventArgs e)
|
|
{
|
|
GroupProp f = new GroupProp();
|
|
f.ShowDialog();
|
|
}
|
|
|
|
private void btnCtTok_Click(object sender, EventArgs e)
|
|
{
|
|
frmCntTkn frm = new frmCntTkn();
|
|
frm.ShowDialog();
|
|
}
|
|
public void UpdateLabelsSetProc(int prc)
|
|
{
|
|
pbProc.Maximum = prc;
|
|
}
|
|
public void UpdateLabelsSetSect(int sec)
|
|
{
|
|
pbSect.Maximum = sec;
|
|
}
|
|
public void UpdateLabelsLibDocs(int incLib, int incUsages)
|
|
{
|
|
if (incLib == 0 && incUsages == 0)//Reset
|
|
{
|
|
pbProc.Value = 0;
|
|
pbSect.Value = 0;
|
|
}
|
|
else
|
|
{
|
|
pbProc.Value += incLib;
|
|
pbSect.Value += incUsages;
|
|
}
|
|
lblProc.Text = string.Format("{0}/{1} Lib Docs", pbProc.Value, pbProc.Maximum);
|
|
lblSection.Text = string.Format("{0}/{1} Usages", pbSect.Value, pbSect.Maximum);
|
|
lblStep.Text = "";
|
|
TimeSpan ts = new TimeSpan(DateTime.Now.Ticks - ProcessTime.Ticks);
|
|
lblTime.Text = string.Format("{0:D2}:{1:D2}:{2:D2} Elapsed", ts.Hours, ts.Minutes, ts.Seconds);
|
|
Application.DoEvents();
|
|
}
|
|
public void UpdateLabelsImages(int incImages)
|
|
{
|
|
if (incImages == 0)//Reset
|
|
pbProc.Value = 0;
|
|
else
|
|
pbProc.Value += incImages;
|
|
lblProc.Text = string.Format("{0}/{1} Images", pbProc.Value, pbProc.Maximum);
|
|
lblSection.Text = "";
|
|
lblStep.Text = "";
|
|
TimeSpan ts = new TimeSpan(DateTime.Now.Ticks - ProcessTime.Ticks);
|
|
lblTime.Text = string.Format("{0:D2}:{1:D2}:{2:D2} Elapsed", ts.Hours, ts.Minutes, ts.Seconds);
|
|
Application.DoEvents();
|
|
}
|
|
public void UpdateLabelsFormats(int incFormats)
|
|
{
|
|
if (incFormats == 0)//Reset
|
|
pbProc.Value = 0;
|
|
else
|
|
pbProc.Value += incFormats;
|
|
lblProc.Text = string.Format("{0}/{1} Formats", pbProc.Value, pbProc.Maximum);
|
|
lblSection.Text = "";
|
|
lblStep.Text = "";
|
|
TimeSpan ts = new TimeSpan(DateTime.Now.Ticks - ProcessTime.Ticks);
|
|
lblTime.Text = string.Format("{0:D2}:{1:D2}:{2:D2} Elapsed", ts.Hours, ts.Minutes, ts.Seconds);
|
|
Application.DoEvents();
|
|
}
|
|
private DataLoaderSettings _MySettings;
|
|
internal DataLoaderSettings MySettings
|
|
{
|
|
get
|
|
{
|
|
if (_MySettings == null)
|
|
_MySettings = new DataLoaderSettings();
|
|
return _MySettings;
|
|
}
|
|
set { _MySettings = value; }
|
|
}
|
|
private void LoadSettings()
|
|
{
|
|
Console.WriteLine("Start");
|
|
if (Properties.Settings.Default["VePromsFilename"].ToString() != "")
|
|
MySettings.VEPromsPath = Properties.Settings.Default.VePromsFilename;
|
|
if (Properties.Settings.Default["VeSamFilename"].ToString() != "")
|
|
MySettings.VESamFile = Properties.Settings.Default.VeSamFilename;
|
|
if (Properties.Settings.Default["DbfPathname"].ToString() != "")
|
|
MySettings.ProcedureSetPath = Properties.Settings.Default.DbfPathname;
|
|
if (Properties.Settings.Default["ProcessOnlyInLocation"].ToString() != "")
|
|
MySettings.ProcessOnlyInLocation = Properties.Settings.Default.ProcessOnlyInLocation;
|
|
if (Properties.Settings.Default["BackupFileName"].ToString() != "")
|
|
MySettings.BackupFileName = Properties.Settings.Default.BackupFileName;
|
|
if (Properties.Settings.Default["BackupFolder"].ToString() != "")
|
|
MySettings.BackupFolder = Properties.Settings.Default.BackupFolder;
|
|
if (Properties.Settings.Default["LogFileLoc"].ToString() != "")
|
|
MySettings.LogFilePath = Properties.Settings.Default.LogFileLoc;
|
|
if (Properties.Settings.Default["ConnectionString"].ToString() != "")
|
|
MySettings.ConnectionString = Properties.Settings.Default.ConnectionString;
|
|
if (Properties.Settings.Default["DBName"].ToString() != "")
|
|
MySettings.DBName = Properties.Settings.Default.DBName;
|
|
if (Properties.Settings.Default["DBPath"].ToString() != "")
|
|
MySettings.DBPath = Properties.Settings.Default.DBPath;
|
|
MySettings.PurgeExistingData = (Properties.Settings.Default.PurgeData == CheckState.Checked);
|
|
if (Properties.Settings.Default["PDFFolder"].ToString() != "")
|
|
MySettings.PDFFolder = Properties.Settings.Default.PDFFolder;
|
|
MySettings.OnlyThisSet = (Properties.Settings.Default.OnlyThisSet == CheckState.Checked);
|
|
MySettings.CheckRTF = (Properties.Settings.Default.CheckRTF == CheckState.Checked);
|
|
MySettings.Skip = Properties.Settings.Default.Skip;
|
|
MySettings.ConvertTo = (AccPageConversion)Properties.Settings.Default.ConvertTo;
|
|
MySettings.ExecutionMode = (ExecutionMode)Properties.Settings.Default.ExecutionMode;
|
|
MySettings.Phase1Suffix = Properties.Settings.Default.Phase1;
|
|
MySettings.Phase2Suffix = Properties.Settings.Default.Phase2;
|
|
MySettings.Phase3Suffix = Properties.Settings.Default.Phase3;
|
|
MySettings.FormatFolder = Properties.Settings.Default.FormatFolder;
|
|
MySettings.GenMacFolder = Properties.Settings.Default.GenMacFolder;
|
|
MySettings.LoadApproved = Properties.Settings.Default.LoadApproved;
|
|
MySettings.Phase4Suffix = Properties.Settings.Default.Phase4;
|
|
MySettings.RedPDFs = (Properties.Settings.Default.RedPDFs == CheckState.Checked);
|
|
string validity = MySettings.ValidityCheck;
|
|
if (validity != "")
|
|
{
|
|
MessageBox.Show(validity, "Settings Incorrect", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
OpenSettings();
|
|
}
|
|
}
|
|
private void OpenSettings()
|
|
{
|
|
DataLoaderSettings tmpDLS = (DataLoaderSettings)MySettings.Clone();
|
|
frmPG myPG = new frmPG("Data Loader Settings", tmpDLS);
|
|
if (myPG.ShowDialog() == DialogResult.OK)
|
|
{
|
|
MySettings = tmpDLS;
|
|
SaveSettings();
|
|
}
|
|
string validity = MySettings.ValidityCheck;
|
|
if (validity != "")
|
|
{
|
|
processToolStripMenuItem.Enabled = false;
|
|
oldToolStripMenuItem.Enabled = false;
|
|
}
|
|
else
|
|
{
|
|
processToolStripMenuItem.Enabled = true;
|
|
oldToolStripMenuItem.Enabled = true;
|
|
}
|
|
}
|
|
private void SaveSettings()
|
|
{
|
|
Properties.Settings.Default.DbfPathname = MySettings.ProcedureSetPath;
|
|
Properties.Settings.Default.VePromsFilename = MySettings.VEPromsPath;
|
|
Properties.Settings.Default.VeSamFilename = MySettings.VESamFile;
|
|
Properties.Settings.Default.DbfPathname = MySettings.ProcedureSetPath;
|
|
Properties.Settings.Default.ProcessOnlyInLocation = MySettings.ProcessOnlyInLocation;
|
|
Properties.Settings.Default.BackupFileName = MySettings.BackupFileName;
|
|
Properties.Settings.Default.BackupFolder = MySettings.BackupFolder;
|
|
Properties.Settings.Default.LogFileLoc = MySettings.LogFilePath;
|
|
Properties.Settings.Default.ConnectionString = MySettings.ConnectionString;
|
|
Properties.Settings.Default.DBName = MySettings.DBName;
|
|
Properties.Settings.Default.DBPath = MySettings.DBPath;
|
|
Properties.Settings.Default.PurgeData = MySettings.PurgeExistingData ? CheckState.Checked : CheckState.Unchecked;
|
|
Properties.Settings.Default.PDFFolder = MySettings.PDFFolder;
|
|
Properties.Settings.Default.OnlyThisSet = MySettings.OnlyThisSet ? CheckState.Checked : CheckState.Unchecked;
|
|
Properties.Settings.Default.CheckRTF = MySettings.CheckRTF ? CheckState.Checked : CheckState.Unchecked;
|
|
Properties.Settings.Default.Skip = MySettings.Skip;
|
|
Properties.Settings.Default.ConvertTo = (int)MySettings.ConvertTo;
|
|
Properties.Settings.Default.ExecutionMode = (int)MySettings.ExecutionMode;
|
|
Properties.Settings.Default.Phase1 = MySettings.Phase1Suffix;
|
|
Properties.Settings.Default.Phase2 = MySettings.Phase2Suffix;
|
|
Properties.Settings.Default.Phase3 = MySettings.Phase3Suffix;
|
|
Properties.Settings.Default.FormatFolder = MySettings.FormatFolder;
|
|
Properties.Settings.Default.GenMacFolder = MySettings.GenMacFolder;
|
|
Properties.Settings.Default.LoadApproved = MySettings.LoadApproved;
|
|
Properties.Settings.Default.Phase4 = MySettings.Phase4Suffix;
|
|
Properties.Settings.Default.RedPDFs = MySettings.RedPDFs ? CheckState.Checked : CheckState.Unchecked;
|
|
Properties.Settings.Default.Save();
|
|
}
|
|
private void frmLoader_Load(object sender, EventArgs e)
|
|
{
|
|
LoadSettings();
|
|
_Loading = false;
|
|
MSWordToPDF.CloseWordWhenDone = false;
|
|
Format.FormatLoaded += new FormatEvent(Format_FormatLoaded);
|
|
StepRTB.DoSpellCheck = false;
|
|
}
|
|
void Format_FormatLoaded(object sender, FormatEventArgs args)
|
|
{
|
|
if (args.Status.StartsWith("Loading Format"))
|
|
UpdateLabelsFormats(1);
|
|
else if (!args.Status.StartsWith("Format") && !args.Status.StartsWith("Loading "))
|
|
{
|
|
pbProcMaximum = int.Parse(args.Status.Split(" ".ToCharArray())[0]);
|
|
UpdateLabelsFormats(0);
|
|
}
|
|
MyInfo = args.Status;
|
|
}
|
|
private void btnFixTransitions_Click(object sender, EventArgs e)
|
|
{
|
|
List<int> cacheContentInfo = ContentInfo.CacheList;
|
|
List<int> cacheItemInfo = ItemInfo.CacheList;
|
|
//List<int> cacheEntryInfo = EntryInfo.CacheList;
|
|
//List<int> cachePdfInfo = PdfInfo.CacheList;
|
|
//List<int> cacheDocVersionInfo = DocVersionInfo.CacheList;
|
|
//if (!CheckLogPath()) return;
|
|
using (StepRTB rtb = new StepRTB())
|
|
{
|
|
TransitionFixer myFixer = new TransitionFixer(rtb, MySettings.LogFilePath);
|
|
myFixer.StatusChanged += new TransitionFixerEvent(myFixer_StatusChanged);
|
|
TimeSpan howlong = myFixer.Process(MySettings.CheckRTF);
|
|
string TransFixTime = string.Format("Fix Transitions completion time: {0:D2}:{1:D2}:{2:D2}.{3}", howlong.Hours, howlong.Minutes, howlong.Seconds, howlong.Milliseconds);
|
|
MyInfo = TransFixTime;
|
|
//if (!ProcessComplete) MessageBox.Show(string.Format("{0}\r\n\n({1} Total Seconds)", TransFixTime, howlong.TotalSeconds));
|
|
//MessageBox.Show(string.Format("Fix Transitions completion time: {0:D2}:{1:D2}:{2:D2}.{3}\r\n\n({4} Total Seconds)", howlong.Hours, howlong.Minutes, howlong.Seconds, howlong.Milliseconds, howlong.TotalSeconds));
|
|
CreateBackupRestoreBatchFiles();
|
|
ItemInfo.RestoreCacheList(cacheItemInfo);
|
|
ContentInfo.RestoreCacheList(cacheContentInfo);
|
|
//EntryInfo.RestoreCacheList(cacheEntryInfo);
|
|
//PdfInfo.RestoreCacheList(cachePdfInfo);
|
|
//DocVersionInfo.RestoreCacheList(cacheDocVersionInfo);
|
|
MyInfo = CSLACache.UsageAll;
|
|
}
|
|
}
|
|
|
|
void myFixer_StatusChanged(object sender, TransitionFixerEventArgs args)
|
|
{
|
|
Status = args.MyStatus;
|
|
}
|
|
private bool _DidLogPathCheck = false;
|
|
//private string _LogFilePath = "";
|
|
|
|
//private bool CheckLogPath()
|
|
//{
|
|
// bool rtn = false;
|
|
// if (_DidLogPathCheck) return true;
|
|
// try
|
|
// {
|
|
// _LogFilePath = txbLogFileLoc.Text;
|
|
// if (!Directory.Exists(_LogFilePath))
|
|
// Directory.CreateDirectory(_LogFilePath);
|
|
// if (_LogFilePath.EndsWith(@"\")) _LogFilePath = _LogFilePath.Substring(0, _LogFilePath.Length - 1);
|
|
// rtn = true;
|
|
// _DidLogPathCheck = true;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// MessageBox.Show(ex.Message, "Invalid Path for Log Files", MessageBoxButtons.OK, MessageBoxIcon.Error);
|
|
// }
|
|
// return rtn;
|
|
//}
|
|
|
|
private void SaveLogFiles()
|
|
{
|
|
//if (_LogFilePath == "") return;
|
|
// Save the Glitches log
|
|
if (TextConvert.MyGlitches.Glitches.Count > 0)
|
|
TextConvert.MyGlitches.Save(MySettings.LogFilePath + @"\ConversionGlitches.xml");
|
|
// Save the Error Log
|
|
if (_MyFrmErrors.ErrorCount > 0)
|
|
_MyFrmErrors.Save(MySettings.LogFilePath + @"\ConversionErrors.txt");
|
|
}
|
|
|
|
private bool _EnteredFileLoc = false;
|
|
private void txbLogFileLoc_TextChanged(object sender, EventArgs e)
|
|
{
|
|
_DidLogPathCheck = false;
|
|
}
|
|
|
|
private void CreateBackupRestoreBatchFiles()
|
|
{
|
|
string pause = "pause";
|
|
string bckupFileName = MySettings.BackupFileName;
|
|
if (!bckupFileName.EndsWith(".bak")) bckupFileName += ".bak";
|
|
string backupPath = MySettings.LogFilePath + @"\" + bckupFileName + "'\"";
|
|
string bckupcmd = string.Format("sqlcmd -E -S.\\sqlexpress -Q \"backup database [{0}] to disk = '{1}", MySettings.DBName, backupPath);
|
|
string rstorecmd = string.Format("sqlcmd -E -S.\\sqlexpress -Q \"restore database [{0}] from disk = '{1}", MySettings.DBName, backupPath);
|
|
StreamWriter fsbackup = new StreamWriter(MySettings.LogFilePath + @"\Backup" + bckupFileName.Substring(0, bckupFileName.Length - 4) + ".bat");
|
|
fsbackup.WriteLine(bckupcmd);
|
|
fsbackup.WriteLine(pause);
|
|
fsbackup.Close();
|
|
StreamWriter fsrestore = new StreamWriter(MySettings.LogFilePath + @"\Restore" + bckupFileName.Substring(0, bckupFileName.Length - 4) + ".bat");
|
|
fsrestore.WriteLine(rstorecmd);
|
|
fsrestore.WriteLine(pause);
|
|
fsrestore.Close();
|
|
}
|
|
// Menu Items
|
|
#region File Menu Items
|
|
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
this.Close();
|
|
}
|
|
#endregion
|
|
#region Process Menu Items
|
|
private bool _ProcessFailed = false;
|
|
public bool ProcessFailed
|
|
{
|
|
get { return _ProcessFailed; }
|
|
set { _ProcessFailed = value; }
|
|
}
|
|
private bool _ProcessComplete = false;
|
|
public bool ProcessComplete
|
|
{
|
|
get { return _ProcessComplete; }
|
|
set { _ProcessComplete = value; }
|
|
}
|
|
static bool ChangeLogFileName(string AppenderName, string NewFilename)
|
|
{
|
|
log4net.Repository.ILoggerRepository RootRep;
|
|
RootRep = log4net.LogManager.GetRepository();
|
|
foreach (log4net.Appender.IAppender iApp in RootRep.GetAppenders())
|
|
{
|
|
if (iApp.Name.CompareTo(AppenderName) == 0
|
|
&& iApp is log4net.Appender.FileAppender)
|
|
{
|
|
log4net.Appender.FileAppender fApp = (log4net.Appender.FileAppender)iApp;
|
|
string folderPath = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
|
|
fApp.File = folderPath + @"\VEPROMS\" + NewFilename;
|
|
fApp.ActivateOptions();
|
|
return true; // Appender found and name changed to NewFilename
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
private void completeToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessFailed = false;
|
|
ProcessComplete = true;
|
|
ProcessTime = DateTime.Now;
|
|
MessageBuilder mb = new MessageBuilder("Performing Complete Process\r\n");
|
|
// Phase 1 - Convert dBase to SQL
|
|
btnConvert_Click(this, new System.EventArgs());
|
|
if (ProcessFailed) return;
|
|
mb.Append("dBase Conversion Complete");
|
|
Status = "Backing up Phase 1 Data";
|
|
Backup("_" + MySettings.Phase1Suffix);
|
|
mb.Append("Phase 1 Backup Complete");
|
|
// Phase 2 - Fix Transitions
|
|
btnFixTransitions_Click(this, new System.EventArgs());
|
|
mb.Append("Fix Transtions Complete");
|
|
Status = "Backing up Phase 2 Data";
|
|
Backup("_" + MySettings.Phase2Suffix);
|
|
mb.Append("Phase 2 Backup Complete");
|
|
// Phase 3 - Convert to Change Manager Version
|
|
if(!ConvertToChangeManager()) return;
|
|
mb.Append("Conversion to Change Manager Complete");
|
|
Status = "Backing up Phase 3 Data";
|
|
Backup("_" + MySettings.Phase3Suffix);
|
|
mb.Append("Phase 3 Backup Complete");
|
|
//Phase 4 - Convert to Approval Version
|
|
if(!ConvertToApproval()) return;
|
|
if(!FixProceduresAndFunctions()) return;
|
|
mb.Append("Conversion to Approval Complete");
|
|
if (MySettings.LoadApproved)
|
|
{
|
|
Status = "Backing up Phase 4 Data";
|
|
Backup("_" + MySettings.Phase4Suffix);
|
|
mb.Append("Phase 4 Backup Complete");
|
|
//Phase 5 - Load Approved Data
|
|
LoadApprovedData();
|
|
mb.Append("Conversion of Approved Data Complete");
|
|
}
|
|
Status = "Backing up Data";
|
|
Backup("");
|
|
mb.Append("Backup Complete");
|
|
mb.LastTime = ProcessTime; // Reset time to get total time.
|
|
mb.Append("Processing Complete");
|
|
Status = "Processing Complete";
|
|
ProcessComplete = false;
|
|
//Clipboard.Clear();
|
|
//Clipboard.SetText(mb.ToString());
|
|
AddInfo(mb.ToString());
|
|
MessageBox.Show(mb.ToString(), "Processing Complete", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private bool ConvertToChangeManager()
|
|
{
|
|
return RunScript("PROMStoCM.sql", MySettings.DBName);
|
|
}
|
|
private bool ConvertToApproval()
|
|
{
|
|
return RunScript("PROMStoAPPR.sql", MySettings.DBName);
|
|
}
|
|
private void formatOnlyToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessTime = DateTime.Now;
|
|
FormatsOnly = true;
|
|
btnConvert_Click(this, new System.EventArgs());
|
|
if (ProcessFailed) return;
|
|
MyInfo = "Format Load Complete";
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Format Change Data";
|
|
Backup("_" + "FormatChange");
|
|
MyInfo = "Format Change Backup Complete";
|
|
}
|
|
//Status = "Format Change Complete";
|
|
//MessageBox.Show("Format Change Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private void convertDBToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessTime = DateTime.Now;
|
|
RunScript("vesp_TurnChangeManagerOFF");
|
|
btnConvert_Click(this, new System.EventArgs());
|
|
RunScript("vesp_TurnChangeManagerON");
|
|
if (ProcessFailed) return;
|
|
MyInfo = "dBase Conversion Complete";
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Phase 1 Data";
|
|
Backup("_" + MySettings.Phase1Suffix);
|
|
MyInfo = "Phase 1 Backup Complete";
|
|
}
|
|
//Status = "dBase Conversion Complete";
|
|
//MessageBox.Show("dBase Conversion Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private void fixTransitionsToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
Database.VEPROMS_Connection = MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName);
|
|
ProcessTime = DateTime.Now;
|
|
RunScript("vesp_TurnChangeManagerOFF");
|
|
btnFixTransitions_Click(this, new System.EventArgs());
|
|
RunScript("vesp_TurnChangeManagerON");
|
|
MyInfo = "Fix Transtions Complete";
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Phase 2 Data";
|
|
Backup("_" + MySettings.Phase2Suffix);
|
|
MyInfo = "Phase 2 Backup Complete";
|
|
}
|
|
Status = "Fix Transtions Complete";
|
|
MessageBox.Show("Fix Transtions Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private void convertToChangeManagerToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessTime = DateTime.Now;
|
|
if(!ConvertToChangeManager()) return;
|
|
MyInfo = "Conversion to Change Manager Complete";
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Phase 3 Data";
|
|
Backup("_" + MySettings.Phase3Suffix);
|
|
MyInfo = "Phase 3 Backup Complete";
|
|
}
|
|
//Status = "Conversion to Change Manager Complete";
|
|
//MessageBox.Show("Conversion to Change Manager Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private void convertToApprovalToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessTime = DateTime.Now;
|
|
if(!ConvertToApproval()) return;
|
|
MyInfo = "Conversion to Approval Complete";
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Phase 4 Data";
|
|
Backup("_" + MySettings.Phase4Suffix);
|
|
MyInfo = "Phase 4 Backup Complete";
|
|
}
|
|
//Status = "Conversion to Approval Complete";
|
|
//MessageBox.Show("Conversion to Approval Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private void load16BitApprovalToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
Database.VEPROMS_Connection = MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName);
|
|
ProcessTime = DateTime.Now;
|
|
if (LoadApprovedData())
|
|
{
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Data";
|
|
Backup("");
|
|
MyInfo = "Backup Complete";
|
|
}
|
|
Status = "Processing Complete";
|
|
}
|
|
else
|
|
Status = "Loading 16 Bit Approval Data Failed";
|
|
//MessageBox.Show("Loading 16 Bit Approval Data Complete", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private bool LoadApprovedData()
|
|
{
|
|
Database.VEPROMS_Connection = MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName);
|
|
Status = "Converting 16-Bit Approved Data";
|
|
Database.VEPROMS_Connection = MySettings.ConnectionString.Replace("{DBName}", MySettings.DBName);
|
|
bool ok = ldr.BuildApprovedRevision();
|
|
Status = "Conversion " + (ok ? "Succeeded" : "Failed");
|
|
return ok;
|
|
}
|
|
private void fixesToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
if (!FixProceduresAndFunctions()) return;
|
|
if (MessageBox.Show("Backup Database?", "Backup", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
Status = "Backing up Data";
|
|
Backup("_Fixed");
|
|
MyInfo = "Backup Complete";
|
|
}
|
|
//Status = "Processing Complete";
|
|
//MessageBox.Show("Fixed Stored Procedures", "Status", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
private bool FixProceduresAndFunctions()
|
|
{
|
|
return RunScript("PROMSFixes.sql", MySettings.DBName);
|
|
}
|
|
#endregion
|
|
#region Settings Menu Items
|
|
private void settingsToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
OpenSettings();
|
|
}
|
|
#endregion
|
|
#region Old Menu Items
|
|
private void convertSecurityToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnVesam_Click(this, new EventArgs());
|
|
}
|
|
private void convertTopFoldersToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnLoadTreeDB_Click(this, new EventArgs());
|
|
}
|
|
private void loadTreeFromCSLAToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnLoadTreeCSLA_Click(this, new EventArgs());
|
|
}
|
|
private void loadVETreeFromCSLAToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnVETree_CSLA_Click(this, new EventArgs());
|
|
}
|
|
private void groupSecurityToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnGroup_Click(this, new EventArgs());
|
|
}
|
|
private void countTokensToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnCtTok_Click(this, new EventArgs());
|
|
}
|
|
private void convertDbfSelectedInTreeToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
btnConvertSelected_Click(this, new EventArgs());
|
|
}
|
|
#endregion
|
|
|
|
private void approvalDatabasesToolStripMenuItem_Click(object sender, EventArgs e)
|
|
{
|
|
this.Cursor = Cursors.WaitCursor;
|
|
//MySettings.ConnectionString
|
|
//"{DBName}", dbName
|
|
string tmp = MySettings.ConnectionString.Replace("{DBName}", "master");
|
|
SqlConnection cn = new SqlConnection(tmp);
|
|
cn.Open();
|
|
SqlDataAdapter da = new SqlDataAdapter("select name, case when object_id(name + '..Items') is null then 'Not PROMS' when object_id(name + '..Revisions') is not null then 'Approval' when object_id(name + '..ContentAudits') is not null then 'Change Manager' else 'Original' end functionality from sysdatabases where name not in ('master','model','msdb','tempdb') order by name", cn);
|
|
DataSet ds = new DataSet();
|
|
da.Fill(ds);
|
|
cn.Close();
|
|
List<string> cms = new List<string>();
|
|
StringBuilder sb = new StringBuilder();
|
|
sb.Append("The following databases will be fixed...\r\n");
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
{
|
|
if (dr["functionality"].ToString() == "Approval")
|
|
{
|
|
cms.Add(dr["name"].ToString());
|
|
sb.Append("\r\n " + dr["name"].ToString());
|
|
}
|
|
}
|
|
this.Cursor = Cursors.Default;
|
|
if (MessageBox.Show(sb.ToString(), "Data Loader Fixes", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning) == DialogResult.OK)
|
|
{
|
|
int good = 0;
|
|
int bad = 0;
|
|
foreach (string s in cms)
|
|
{
|
|
AddInfo("\r\nFixing database {0}\r\n", s);
|
|
if (RunScript("PROMSFixes.sql", s)) good++;
|
|
else bad++;
|
|
}
|
|
AddInfo("\r\nFixing databases complete. {0} Fixed, {1} Failures", good, bad);
|
|
Status = String.Format("Fixing databases complete. {0} Fixed, {1} Failures", good, bad);
|
|
}
|
|
}
|
|
}
|
|
public class MessageBuilder
|
|
{
|
|
private StringBuilder _MyStringBulider = new StringBuilder();
|
|
private DateTime _LastTime = DateTime.Now;
|
|
public DateTime LastTime
|
|
{
|
|
get { return _LastTime; }
|
|
set { _LastTime = value; }
|
|
}
|
|
public MessageBuilder(string heading)
|
|
{
|
|
_MyStringBulider.Append(heading);
|
|
}
|
|
public void Append(string format, params object[] args)
|
|
{
|
|
string msg = string.Format(format, args);
|
|
DateTime now = DateTime.Now;
|
|
TimeSpan ts = TimeSpan.FromTicks(now.Ticks - _LastTime.Ticks);
|
|
string timestamp = string.Format("{0:D2}:{1:D2}:{2:D2}.{3:D3}", ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds);
|
|
_LastTime = now;
|
|
GC.Collect();
|
|
long memUse = GC.GetTotalMemory(true);
|
|
_MyStringBulider.Append("\r\n" + timestamp + "\t" + memUse.ToString() + "\t" + msg);
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return _MyStringBulider.ToString();
|
|
}
|
|
}
|
|
}
|