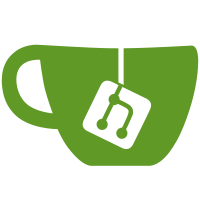
Made call to vesp_TurnChangeManagerOFF prior to converting a database and a call to vesp_TurnChangeManagerON after converting a database to improve adding 16-bit data to an existing 32-bit database Made call to vesp_TurnChangeManagerOFF prior to fixing transitions and a call to vesp_TurnChangeManagerON after fixing transitions to improve adding 16-bit data to an existing 32-bit database Modified PartialMatch method to handle cases when XTSETID.DBF entry for abbyproc does not have the .prc extension Modified ExactMatch method to handle cases when XTSETID.DBF entry for abbyproc does not have the .prc extension Added stored procedure stubs for vesp_TurnChangeManagerOFF and vesp_TurnChangeManagerON
284 lines
8.5 KiB
C#
284 lines
8.5 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
//using System.Collections.Specialized;
|
|
//using System.Collections.Generic;
|
|
//using System.Xml;
|
|
//using System.IO;
|
|
//using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
class OutsideTransition
|
|
{
|
|
private OleDbConnection _DbConnect;
|
|
|
|
public OleDbConnection DbConnect
|
|
{
|
|
get { return _DbConnect; }
|
|
set { _DbConnect = value; }
|
|
}
|
|
|
|
private Dictionary <string,ItemInfo> _TranLookup;
|
|
|
|
public OutsideTransition(OleDbConnection cn)
|
|
{
|
|
_DbConnect = cn;
|
|
_TranLookup = new Dictionary<string, ItemInfo>();
|
|
}
|
|
|
|
public ItemInfo GetItem(string key)
|
|
{
|
|
if (!_TranLookup.ContainsKey(key))
|
|
_TranLookup.Add(key, Find(key));
|
|
return _TranLookup[key];
|
|
}
|
|
|
|
private ItemInfo Find(string key)
|
|
{
|
|
string setid = key.Substring(0, 8);
|
|
string procid = key.Substring(8, 8);
|
|
string path = GetPath(setid);// Get set path from XTSETID
|
|
string proc = GetProc(setid, procid);// Get procedure number from XTPROCID
|
|
return Find(path,proc);// Return ItemInfo based upon path and procedure number
|
|
}
|
|
|
|
private ItemInfo Find(string path, string proc)
|
|
{
|
|
return OTLookup.Find(path, proc);
|
|
}
|
|
|
|
private string GetProc(string setid, string procid)
|
|
{
|
|
string retval = null;
|
|
string xtproccmd = "SELECT * FROM [xtprocid] WHERE [SETID]='" + setid + "' AND [PROCID]='" + procid + "'";
|
|
using (OleDbDataAdapter da = new OleDbDataAdapter(xtproccmd, _DbConnect))
|
|
{
|
|
try
|
|
{
|
|
DataSet ds = new DataSet();
|
|
da.Fill(ds);
|
|
DataTable dt = ds.Tables[0];
|
|
dt.CaseSensitive = true;
|
|
DataRow dr = dt.Rows[0]; // there should be only one entry!
|
|
retval = dr["Procnum"].ToString(); // this should be the procedure number!
|
|
ds.Dispose();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return "<Procedure Number>";//ex.GetType().Name + " " + ex.Message;
|
|
}
|
|
}
|
|
return retval;
|
|
}
|
|
|
|
private string GetPath(string setid)
|
|
{
|
|
try
|
|
{
|
|
string xtsetcmd = "SELECT * FROM [xtsetid] WHERE [SETID]='" + setid + "'";
|
|
OleDbDataAdapter da = new OleDbDataAdapter(xtsetcmd, _DbConnect);
|
|
DataSet ds = new DataSet();
|
|
da.Fill(ds);
|
|
DataTable dt = ds.Tables[0];
|
|
dt.CaseSensitive = true;
|
|
DataRow dr = dt.Rows[0]; // there should be only one entry!
|
|
string retval = dr["Path"].ToString();
|
|
da.Dispose();
|
|
ds.Dispose();
|
|
return retval; // dr["Path"].ToString(); // this should be the path to the proc set!
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return "<Procedure Set Location>";//ex.GetType().Name + " " + ex.Message;
|
|
}
|
|
}
|
|
|
|
private string GetTitle(string setid, string procid)
|
|
{
|
|
try
|
|
{
|
|
|
|
string xtproccmd = "SELECT * FROM [xtprocid] WHERE [SETID]='" + setid + "' AND [PROCID]='" + procid + "'";
|
|
OleDbDataAdapter da = new OleDbDataAdapter(xtproccmd, _DbConnect);
|
|
DataSet ds = new DataSet();
|
|
da.Fill(ds);
|
|
DataTable dt = ds.Tables[0];
|
|
dt.CaseSensitive = true;
|
|
DataRow dr = dt.Rows[0]; // there should be only one entry!
|
|
string retval = dr["Proctitle"].ToString();
|
|
da.Dispose();
|
|
ds.Dispose();
|
|
return retval; // dr["Proctitle"].ToString(); // this should be the procedure number!
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return "<Procedure Title>";//ex.GetType().Name + " " + ex.Message;
|
|
}
|
|
}
|
|
|
|
public string GetDescription(string key)
|
|
{
|
|
string setid = key.Substring(0, 8);
|
|
string procid = key.Substring(8, 8);
|
|
string path = GetPath(setid);// Get set path from XTSETID
|
|
string proc = GetProc(setid, procid);// Get procedure number from XTPROCID
|
|
string title = GetTitle(setid, procid);// Get procedure title from XTPROCID
|
|
return string.Format("{0}, {1} in folder {2}",proc,title,path);
|
|
}
|
|
public string GetTransitionText(string key)
|
|
{
|
|
string setid = key.Substring(0, 8);
|
|
string procid = key.Substring(8, 8);
|
|
string proc = GetProc(setid, procid);// Get procedure number from XTPROCID
|
|
string title = GetTitle(setid, procid);// Get procedure title from XTPROCID
|
|
proc = proc.Replace("-", @"\u8209?"); // in proc number, replace dash and spaces with
|
|
proc = proc.Replace(" ", @"\u160?"); // nonbreak unicode dash & hardspace.
|
|
title = title.Replace("-", @"\u8209?"); // in title, just do the hardspace.
|
|
return string.Format("{0}, {1}", proc, title);
|
|
}
|
|
//private string ProcessOutSideTrans(OleDbConnection cn, string thekey, string pth)
|
|
//{
|
|
// string xtransstring = null;
|
|
// DataTable dt = null;
|
|
// DataSet ds = null;
|
|
// OleDbDataAdapter da = null;
|
|
|
|
// DataTable dt2 = null;
|
|
// DataSet ds2 = null;
|
|
// OleDbDataAdapter da2 = null;
|
|
|
|
// string tosetid = thekey.Substring(0, 8);
|
|
// string toprocid = thekey.Substring(8, 8);
|
|
|
|
// /// Get the path to the procedure set from the SETID
|
|
// string xtsetcmd = "SELECT * FROM [xtsetid] WHERE [SETID]='" + tosetid + "'";
|
|
// string xtproccmd = "SELECT * FROM [xtprocid] WHERE [SETID]='" + tosetid + "' AND [PROCID]='" + toprocid + "'";
|
|
|
|
// da = new OleDbDataAdapter(xtsetcmd, cn);
|
|
// da2 = new OleDbDataAdapter(xtproccmd, cn);
|
|
|
|
// // get xtsetid records.
|
|
// ds = new DataSet();
|
|
// try
|
|
// {
|
|
// da.Fill(ds);
|
|
// dt = ds.Tables[0];
|
|
// dt.CaseSensitive = true;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// log.Error("Error getting XTSETID");
|
|
// log.ErrorFormat("to number = {0}", thekey);
|
|
// log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
// //return textm;
|
|
// }
|
|
// DataRow dr = dt.Rows[0]; // there should be only one entry!
|
|
// string setpth = dr["Path"].ToString(); // this should be the path to the proc set!
|
|
|
|
// // get xtprocid records
|
|
// ds2 = new DataSet();
|
|
// try
|
|
// {
|
|
// da2.Fill(ds2);
|
|
// dt2 = ds2.Tables[0];
|
|
// dt2.CaseSensitive = true;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// log.Error("Error getting XTPROCID");
|
|
// log.ErrorFormat("to number = {0}", thekey);
|
|
// log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
// //return textm;
|
|
// }
|
|
// DataRow dr2 = dt2.Rows[0]; // there should be only one entry!
|
|
// string procnum = dr2["Procnum"].ToString(); // this should be the procedure number!
|
|
|
|
// xtransstring = setpth + "\\" + procnum; // ex: VEHLP\PROCS\0POP05-EO00
|
|
|
|
// //if (pathsAreEqual(setpth, pth))
|
|
// //{
|
|
// // // Outside Transiton is to this procedure set (not really outsde)
|
|
// // // need to find the item id that references the proc number and title
|
|
// // // - find the setpth in the doc.version
|
|
// //}
|
|
// //else
|
|
// //return thekey; // is a true outside transition
|
|
|
|
// return xtransstring;
|
|
//}
|
|
}
|
|
|
|
internal static class OTLookup
|
|
{
|
|
private static Dictionary<string, ItemInfo> _Lookup = null;
|
|
public static ItemInfo Find(string path, string procnum)
|
|
{
|
|
string key = string.Format(@"{0}\{1}",path,procnum);
|
|
if (_Lookup == null)
|
|
_Lookup = new Dictionary<string, ItemInfo>();
|
|
if (!_Lookup.ContainsKey(key))
|
|
_Lookup.Add(key, FindItem(path, procnum));
|
|
return _Lookup[key];
|
|
}
|
|
|
|
private static DocVersionInfoList _DocVersions = null;
|
|
private static ItemInfo FindItem(string path, string procnum)
|
|
{
|
|
if (_DocVersions == null)
|
|
_DocVersions = DocVersionInfoList.Get();
|
|
DocVersionInfo dvi = ExactMatch(path);
|
|
if (dvi == null) dvi = PartialMatch(path);
|
|
if (dvi != null) return FindItem(dvi, procnum);
|
|
return null;
|
|
}
|
|
|
|
private static ItemInfo FindItem(DocVersionInfo dvi, string procnum)
|
|
{
|
|
foreach (ItemInfo itm in dvi.Procedures)
|
|
if (itm.DisplayNumber == procnum)
|
|
return itm;
|
|
return null;
|
|
}
|
|
|
|
private static DocVersionInfo PartialMatch(string path)
|
|
{
|
|
string partial = GetPartial(path);
|
|
if (partial != null)
|
|
{
|
|
foreach (DocVersionInfo dvi in _DocVersions)
|
|
{
|
|
if (dvi.MyFolder.Title.ToUpper().EndsWith(partial.ToUpper()))
|
|
return dvi;
|
|
}
|
|
if (path.ToUpper().EndsWith("ABBYPROC"))
|
|
return PartialMatch(path + ".prc");
|
|
}
|
|
return null;
|
|
}
|
|
private static string GetPartial(string path)
|
|
{
|
|
//Console.WriteLine(string.Format("GetPartial path = {0}",path));
|
|
if (path.Contains("\\"))
|
|
{
|
|
string[] parts = path.Split("\\".ToCharArray());
|
|
return "\\" + parts[parts.Length - 2] + "\\" + parts[parts.Length - 1];
|
|
}
|
|
else
|
|
return null;
|
|
}
|
|
private static DocVersionInfo ExactMatch(string path)
|
|
{
|
|
foreach (DocVersionInfo dvi in _DocVersions)
|
|
if (dvi.MyFolder.Title.ToUpper().EndsWith(path.ToUpper()))
|
|
return dvi;
|
|
if (path.ToUpper().EndsWith("ABBYPROC"))
|
|
return ExactMatch(path + ".prc");
|
|
return null;
|
|
}
|
|
}
|
|
}
|