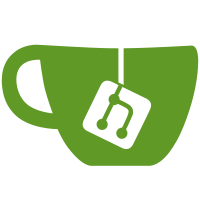
Added code to fix transitions that involve Section, SubSections and Steps as part of the path to the transition and how this is rendered in the step's text.
306 lines
11 KiB
C#
306 lines
11 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using Volian.Controls.Library;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Text.RegularExpressions;
|
|
using Volian.Base.Library;
|
|
using System.Xml;
|
|
using System.IO;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public delegate void TransitionFixerEvent(object sender, TransitionFixerEventArgs args);
|
|
public class TransitionFixerEventArgs
|
|
{
|
|
private string _MyStatus;
|
|
|
|
public string MyStatus
|
|
{
|
|
get { return _MyStatus; }
|
|
set { _MyStatus = value; }
|
|
}
|
|
public TransitionFixerEventArgs(string myStatus)
|
|
{
|
|
_MyStatus = myStatus;
|
|
}
|
|
}
|
|
class TransitionFixer
|
|
{
|
|
public static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
public event TransitionFixerEvent StatusChanged;
|
|
private void OnStatusChanged(object sender, TransitionFixerEventArgs args)
|
|
{
|
|
if (StatusChanged != null)
|
|
StatusChanged(sender, args);
|
|
}
|
|
private string _Status;
|
|
|
|
public string Status
|
|
{
|
|
get { return _Status; }
|
|
set
|
|
{
|
|
_Status = value;
|
|
OnStatusChanged(this, new TransitionFixerEventArgs(_Status));
|
|
}
|
|
}
|
|
private int _ErrorCount = 0;
|
|
|
|
public int ErrorCount
|
|
{
|
|
get { return _ErrorCount; }
|
|
set { _ErrorCount = value; }
|
|
}
|
|
|
|
private StepRTB _MyStepRTB;
|
|
|
|
public StepRTB MyStepRTB
|
|
{
|
|
get { return _MyStepRTB; }
|
|
}
|
|
private string _LogPath;
|
|
private frmLoader _MyLoader;
|
|
public TransitionFixer(StepRTB myStepRTB,string logpath, frmLoader myLoader)
|
|
{
|
|
_MyStepRTB = myStepRTB;
|
|
_LogPath = logpath;
|
|
_MyLoader = myLoader;
|
|
}
|
|
public TimeSpan Process(bool checkRTF)
|
|
{
|
|
DateTime tstart = DateTime.Now;
|
|
ContentInfo.StaticContentInfoChange += new StaticContentInfoEvent(ContentInfo_StaticContentInfoChange);
|
|
ProcessTransitions(checkRTF);
|
|
ContentInfo.StaticContentInfoChange -= new StaticContentInfoEvent(ContentInfo_StaticContentInfoChange);
|
|
return DateTime.Now - tstart;
|
|
}
|
|
|
|
void ContentInfo_StaticContentInfoChange(object sender, StaticContentInfoEventArgs args)
|
|
{
|
|
_MyLoader.AddInfo("Fixed Transition for {0}, changed from {1} to {2}", (sender as ContentInfo).ContentID, args.OldValue, args.NewValue);
|
|
}
|
|
private void ProcessTransitions(bool checkRTF)
|
|
{
|
|
Status = "Getting List...";
|
|
// Loop through all Items and check before and after text
|
|
ItemInfoList myListFrom = ItemInfoList.GetListTranFrom(); //ItemInfoList.GetByContentID(99732);//
|
|
ItemInfoList myListTo = ItemInfoList.GetListTranTo(); //ItemInfoList.GetByContentID(99732); //
|
|
ConversionRTBProblems myProblems = new ConversionRTBProblems();
|
|
int i = 0;
|
|
foreach (ItemInfo item in myListFrom)
|
|
{
|
|
Status = string.Format("Processing {0} of {1} steps", ++i, myListFrom.Count);
|
|
//MyStepRTB.ViewRTB = false;
|
|
string originalText = item.MyContent.Text;
|
|
string updatedText = item.MyContent.Text;
|
|
if (item.MyContent.MyGrid != null)
|
|
{
|
|
originalText = item.MyContent.MyGrid.Data;
|
|
updatedText = (item.MyContent.MyGrid.Data.Replace("<START]", "<START]")).Replace("[END>", "[END>");
|
|
}
|
|
// Exclude items that are not connected (Dummy steps for invalid transition destinations)
|
|
if (item.ItemDocVersionCount != 0 || item.MyPrevious != null || item.MyParent != null)
|
|
{
|
|
if (item.MyContent.ContentTransitionCount > 0)
|
|
{
|
|
//updatedText = Volian.Controls.Library.DisplayText.StaticRemoveRtfStyles(updatedText, item);
|
|
foreach (TransitionInfo tran in item.MyContent.ContentTransitions)
|
|
{
|
|
try
|
|
{
|
|
string oldtext = item.MyContent.Text;
|
|
item.MyContent.FixTransitionText(tran);
|
|
string newtext = item.MyContent.Text;
|
|
if (newtext != oldtext)
|
|
{
|
|
using (Content c = item.MyContent.Get())
|
|
{
|
|
c.FixTransitionText(tran);
|
|
c.Save();
|
|
|
|
}
|
|
}
|
|
if (item.NewTransToUnNumberedItem)
|
|
{
|
|
using (Item itm = item.Get())
|
|
{
|
|
ItemAnnotation ia = itm.ItemAnnotations.Add(VerificationRequiredType);
|
|
ia.SearchText = "Transition to un-numbered step";
|
|
ia.UserID = "Migration";
|
|
itm.Save();
|
|
}
|
|
}
|
|
//_MyLoader.AddInfo("{0}", item.MyContent.MyContentMessage);
|
|
//if (item.MyContent.MyGridMessage != string.Empty)
|
|
// _MyLoader.AddInfo("{0}", item.MyContent.MyGridMessage);
|
|
// updatedText = FixTransitionText(originalText, tran, item.MyContent.ContentID);
|
|
//if (item.MyContent.MyGrid != null)
|
|
// updatedText = FixTableTransitionText(originalText, tran, item.MyContent.Get());
|
|
//else
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("{0} {1}", ex.GetType().Name, ex.Message);
|
|
_MyLog.WarnFormat("Transition Conversion Error: {0}", ex.Message);
|
|
}
|
|
// Added for transitions to un-numbered steps
|
|
//if (tran.NewTransToUnNumberedItem) item.NewTransToUnNumberedItem = true;
|
|
}
|
|
}
|
|
//if (updatedText.EndsWith(" ")) updatedText = updatedText.Substring(0, updatedText.Length - 1);
|
|
//if (item.MyContent.MyGrid != null)
|
|
//{
|
|
// using (Item itm = item.Get())
|
|
// {
|
|
// updatedText = (updatedText.Replace("<START]", "<START]")).Replace("[END>", "[END>");
|
|
// string sstring = AdjustSizeAndGetSearchString(updatedText, itm);
|
|
// itm.MyContent.Text = sstring;
|
|
// if (item.NewTransToUnNumberedItem)
|
|
// {
|
|
// ItemAnnotation ia = itm.ItemAnnotations.Add(VerificationRequiredType);
|
|
// ia.SearchText = "Transition to un-numbered step";
|
|
// ia.UserID = "Migration";
|
|
// }
|
|
// itm.Save();
|
|
// }
|
|
//}
|
|
//else
|
|
//{
|
|
// if (item.NewTransToUnNumberedItem)
|
|
// {
|
|
// using (Item itm = item.Get())
|
|
// {
|
|
// ItemAnnotation ia = itm.ItemAnnotations.Add(VerificationRequiredType);
|
|
// ia.SearchText = "Transition to un-numbered step";
|
|
// ia.UserID = "Migration";
|
|
// itm.Save();
|
|
// }
|
|
// }
|
|
// using (Content c = item.MyContent.Get())
|
|
// {
|
|
// c.Text = updatedText;
|
|
// c.Save();
|
|
// }
|
|
//}
|
|
//// Added for transitions to un-numbered steps
|
|
if (checkRTF)
|
|
{
|
|
MyStepRTB.MyItemInfo = item;
|
|
// Force Save - This will put change bars on everything
|
|
if (MyStepRTB.Text.Contains("(Resolved Transition Text)") != false) MyStepRTB.OrigDisplayText.Save(MyStepRTB);
|
|
string afterText = item.MyContent.Text;
|
|
// aftertext is 'newrtf'
|
|
if (afterText != updatedText)
|
|
myProblems.RTBProblems.Add(item.ItemID, item.MyContent.ContentID, originalText, updatedText, MyStepRTB.Rtf, afterText, item.Path);
|
|
}
|
|
}
|
|
}
|
|
if (checkRTF)
|
|
{
|
|
Status = "Saving problems";
|
|
string logFile = _LogPath + @"\RTBProblems.xml";
|
|
ErrorCount = myProblems.RTBProblems.Count;
|
|
myProblems.Save(logFile);
|
|
if (ErrorCount > 0)
|
|
{
|
|
System.Windows.Forms.DialogResult answer = System.Windows.Forms.MessageBox.Show(
|
|
string.Format("{0} Differences found in Transition Text\r\nResults in {1}\r\n\r\nOpen Log File?",
|
|
ErrorCount, logFile), "Transitions Different", System.Windows.Forms.MessageBoxButtons.YesNo, System.Windows.Forms.MessageBoxIcon.Question);
|
|
if(answer == System.Windows.Forms.DialogResult.Yes)
|
|
System.Diagnostics.Process.Start(logFile);
|
|
}
|
|
}
|
|
Status = "Done comparing";
|
|
}
|
|
// Added for transitions to un-numbered steps
|
|
private AnnotationType _VerificationRequiredType; // Using this to flag table to grid conversions
|
|
public AnnotationType VerificationRequiredType
|
|
{
|
|
get
|
|
{
|
|
if (_VerificationRequiredType == null)
|
|
_VerificationRequiredType = AnnotationType.GetByName("Verification Required");
|
|
return _VerificationRequiredType;
|
|
}
|
|
}
|
|
public string FixTransitionText(string Text, TransitionInfo tran, int contentID)
|
|
{
|
|
string lookFor = string.Format(@"<START\]\\v0 ([^#]*?)\\v #Link:Transition[^:]*?:{0} {1}( [0-9]*){2}\[END>", tran.TranType, tran.TransitionID, "{1,2}");
|
|
string transText = tran.ResolvePathTo();
|
|
//Console.WriteLine(">>>>> FixTransitionText");
|
|
//Console.WriteLine("Text = {0}", Text);
|
|
//Console.WriteLine("lookFor = {0}", lookFor);
|
|
//Console.WriteLine("TransText = {0}", transText);
|
|
Match m = Regex.Match(Text, lookFor);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = m.Groups[1];
|
|
_MyLoader.AddInfo("DataLoader.TransitionFixer:Content:{0}, {1}, {2}", contentID, tran.TransitionID, g.ToString());
|
|
//Console.WriteLine("DataLoader.TransitionFixer:Content:{0}, {1}", tran.TransitionID, g.ToString());
|
|
if (g.ToString() != transText)
|
|
Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
}
|
|
else
|
|
Console.WriteLine("Transition not Found");
|
|
return Text;
|
|
}
|
|
public string FixTableTransitionText(string Text, TransitionInfo tran, Content content)
|
|
{
|
|
string lookFor = string.Format(@"<START\]\\cf1\\v0 ([^#]*?)\\cf0\\v #Link:Transition[^:]*?:{0} {1}( [0-9]*){2}\[END>", tran.TranType, tran.TransitionID, "{1,2}");
|
|
string transText = tran.ResolvePathTo();
|
|
//Console.WriteLine(">>>>> FixTransitionText");
|
|
//Console.WriteLine("Text = {0}", Text);
|
|
//Console.WriteLine("lookFor = {0}", lookFor);
|
|
//Console.WriteLine("TransText = {0}", transText);
|
|
Match m = Regex.Match(Text, lookFor);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = m.Groups[1];
|
|
_MyLoader.AddInfo("DataLoader.TransitionFixer:Grid:{0}, {1}, {2}", content.ContentID, tran.TransitionID, g.ToString());
|
|
// Console.WriteLine("DataLoader.TransitionFixer:Grid:{0}, {1}", tran.TransitionID, g.ToString());
|
|
if (g.ToString() != transText)
|
|
Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
}
|
|
else
|
|
Console.WriteLine("Transition not Found");
|
|
|
|
//VlnFlexGrid grd = new VlnFlexGrid(1, 1);
|
|
//XmlDocument xd = new XmlDocument();
|
|
//xd.LoadXml(Text);
|
|
//grd.ReadXml(xd);
|
|
//grd.FixTableCellsHeightWidth(); // resize the column width/height
|
|
//using (StringWriter sw = new StringWriter())
|
|
//{
|
|
// grd.WriteXml(sw);
|
|
// //Console.WriteLine(sw.GetStringBuilder().ToString());
|
|
// content.MyGrid.Data = sw.GetStringBuilder().ToString();
|
|
// sw.Close();
|
|
//}
|
|
return Text;
|
|
}
|
|
private string AdjustSizeAndGetSearchString(string strXML, Item itm)
|
|
{
|
|
string rstring = "";
|
|
VlnFlexGrid grd = new VlnFlexGrid(1, 1);
|
|
XmlDocument xd = new XmlDocument();
|
|
xd.LoadXml(strXML);
|
|
grd.ReadXml(xd);
|
|
//using (StringReader sr = new StringReader(strXML))
|
|
//{
|
|
// grd.ReadXml(sr);
|
|
// sr.Close();
|
|
//}
|
|
grd.FixTableCellsHeightWidth(); // resize the column width/height
|
|
rstring = grd.GetSearchableText();
|
|
using (StringWriter sw = new StringWriter())
|
|
{
|
|
grd.WriteXml(sw);
|
|
itm.MyContent.MyGrid.Data = sw.GetStringBuilder().ToString();
|
|
sw.Close();
|
|
}
|
|
return rstring;
|
|
}
|
|
}
|
|
}
|