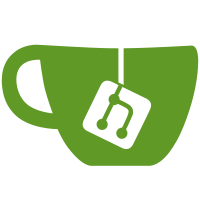
Add RoFstLookup Dicitonary Entries for Multiple Return Values AddByROFstIDImageIDs - Add a list of figures based upon a List of ImageIDs Fix regular expression to find RO Text to replace GetDROUsagesByROIDs - get a list of ROUSages for a list of ROIDs GetJustRODB - Get Just RODB object without children GetJustROFst - Get Just ROFst or ROFstInfo object without children Reduce duplicate gets of RODB and ROFst Improve ROFst Update Performance GetByRODbIDNoData - Get RoImageInfo objects without graphic data for ROFst Update comparison GetROUSagesByROIDs - Get a list of ROUSages by ROIDs. This reduces the number of ROIDs to be checked when updating ROFst. Use GetJustRoFst and GetJustRoDB to improve the performance to see if the "Update ROFst" button should be active.
230 lines
7.4 KiB
C#
230 lines
7.4 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Xml;
|
|
using System.Data.SqlClient;
|
|
using System.Data;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class Content
|
|
{
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0} {1}", Number, Text);
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran)
|
|
{
|
|
string transText = tran.ResolvePathTo();
|
|
string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
//string lookFor = string.Format(@"<START\]\\v0 ([^#]*?)\\v #Link:Transition[^:]*?:{0} {1} [0-9]*\[END>", tran.TranType, tran.TransitionID);
|
|
Match m = Regex.Match(Text, lookFor);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = m.Groups[3];
|
|
if (g.ToString() != transText)
|
|
Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (tran.MyContent.MyGrid != null)
|
|
{
|
|
string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
Match mg = Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
if (g.ToString() != transText)
|
|
MyGrid.Data = MyGrid.Data.Substring(0, g.Index) + transText + MyGrid.Data.Substring(g.Index + g.Length);
|
|
}
|
|
}
|
|
}
|
|
public void FixContentText(RoUsageInfo rousg, ROFSTLookup.rochild roch, ROFstInfo origROFstInfo) // string newvalue)
|
|
{
|
|
string newvalue = roch.value;
|
|
string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^\[]*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
Match m = Regex.Match(Text, lookFor,RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = m.Groups[3];
|
|
if (g.ToString() != newvalue)
|
|
Text = Text.Substring(0, g.Index) + newvalue + Text.Substring(g.Index + g.Length);
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (rousg.MyContent.MyGrid != null)
|
|
{
|
|
if (roch.type == (int)E_ROValueType.Table) // if change in rotable data...
|
|
{
|
|
List<string> retlist = origROFstInfo.OnROTableUpdate(this, new ROFstInfoROTableUpdateEventArgs(newvalue, MyGrid.Data));
|
|
if (Text != retlist[0])Text = retlist[0];
|
|
if (MyGrid.Data != retlist[1]) MyGrid.Data = retlist[1];
|
|
}
|
|
else
|
|
{
|
|
// if it's an ro within a table, need to process into an flex grid to save the grid data:
|
|
|
|
string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
Match mg = Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
if (g.ToString() != newvalue)
|
|
MyGrid.Data = MyGrid.Data.Substring(0, g.Index) + newvalue + MyGrid.Data.Substring(g.Index + g.Length);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
public partial class ContentInfo
|
|
{
|
|
public PartInfoList LocalContentParts
|
|
{
|
|
get { return _ContentParts; }
|
|
}
|
|
public void AddPart(SafeDataReader dr, ItemInfo itemInfo)
|
|
{
|
|
if (_ContentParts == null)
|
|
_ContentParts = new PartInfoList(dr, itemInfo);
|
|
else
|
|
_ContentParts.AddPartInfo(dr, itemInfo);
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0} {1}", Number, Text);
|
|
}
|
|
public void ShowChange()
|
|
{
|
|
OnChange();
|
|
}
|
|
//public XmlNode ToXml(XmlNode xn)
|
|
//{
|
|
// XmlNode nd = xn.OwnerDocument.CreateElement("Content");
|
|
// xn.AppendChild(nd);
|
|
// AddAttribute(nd, "Number", _Number);
|
|
// AddAttribute(nd, "Text", _Text);
|
|
// AddAttribute(nd, "FormatID", _FormatID);
|
|
// AddAttribute(nd, "Config", _Config);
|
|
// return nd;
|
|
//}
|
|
//public void AddAttribute(XmlNode xn, string name, object o)
|
|
//{
|
|
// if (o != null && o.ToString() != "")
|
|
// {
|
|
// XmlAttribute xa = xn.OwnerDocument.CreateAttribute(name);
|
|
// xa.Value = o.ToString();
|
|
// xn.Attributes.Append(xa);
|
|
// }
|
|
//}
|
|
internal ContentInfo(SafeDataReader dr,bool ForItem)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ContentInfo.Constructor", GetHashCode());
|
|
try
|
|
{
|
|
ReadDataItemList(dr);
|
|
_CacheList.Add(this);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ContentInfo.Constructor", ex);
|
|
throw new DbCslaException("ContentInfo.Constructor", ex);
|
|
}
|
|
}
|
|
private void ReadDataItemList(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ContentInfo.ReadDataItemList", GetHashCode());
|
|
try
|
|
{
|
|
_ContentID = dr.GetInt32("ContentID");
|
|
_Number = dr.GetString("Number");
|
|
_Text = dr.GetString("Text");
|
|
_Type = (int?)dr.GetValue("Type");
|
|
_FormatID = (int?)dr.GetValue("FormatID");
|
|
_Config = dr.GetString("Config");
|
|
_DTS = dr.GetDateTime("cDTS");
|
|
_UserID = dr.GetString("cUserID");
|
|
_ContentDetailCount = dr.GetInt32("DetailCount");
|
|
_ContentEntryCount = dr.GetInt32("EntryCount");
|
|
_ContentGridCount = dr.GetInt32("GridCount");
|
|
_ContentImageCount = dr.GetInt32("ImageCount");
|
|
_ContentItemCount = dr.GetInt32("ItemCount");
|
|
_ContentPartCount = dr.GetInt32("cPartCount");
|
|
_ContentRoUsageCount = dr.GetInt32("RoUsageCount");
|
|
_ContentTransitionCount = dr.GetInt32("TransitionCount");
|
|
_ContentZContentCount = dr.GetInt32("ZContentCount");
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ContentInfo.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("ContentInfo.ReadData", ex);
|
|
}
|
|
}
|
|
|
|
}
|
|
public partial class ContentInfoList
|
|
{
|
|
public static ContentInfoList GetList(int? itemID)
|
|
{
|
|
try
|
|
{
|
|
ContentInfoList tmp = DataPortal.Fetch<ContentInfoList>(new ContentListCriteria(itemID));
|
|
ContentInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ItemInfoList.GetChildren", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ContentListCriteria
|
|
{
|
|
public ContentListCriteria(int? itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int? _ItemID;
|
|
public int? ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ContentListCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "vesp_ListContentsByItemID";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read())
|
|
{
|
|
ContentInfo contentInfo = new ContentInfo(dr);
|
|
this.Add(contentInfo);
|
|
}
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Database.LogException("ContentInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("ContentInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
}
|
|
}
|