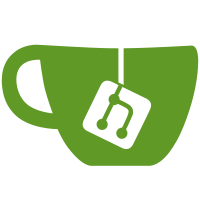
Added code to delete ro usages and transitions that are converted to text. Added code to handle "dashes" and "unicode dashes" Added code to support converting to text transitions to external destinations with internal style transition Added wait cursor for log running processes so application shows it is busy and added code to to fix searches that should return results but are not returning results when doiing transitions searches. Added code to make Refresh Referenced Objects and Refresh Transitions button under Review tab of StepTabRibbon not visible
928 lines
39 KiB
C#
928 lines
39 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Xml;
|
|
using System.Data.SqlClient;
|
|
using System.Data;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class Content
|
|
{
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0} {1}", Number, Text);
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran)
|
|
{
|
|
FixTransitionText(tran, false);
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran, bool forceConvertToText)
|
|
{
|
|
//string transText = tran.ResolvePathTo();
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
////string lookFor = string.Format(@"<START\]\\v0 ([^#]*?)\\v #Link:Transition[^:]*?:{0} {1} [0-9]*\[END>", tran.TranType, tran.TransitionID);
|
|
//Match m = Regex.Match(Text, lookFor);
|
|
//if (m != null && m.Groups.Count > 1)
|
|
//{
|
|
// System.Text.RegularExpressions.Group g = m.Groups[3];
|
|
// if (g.ToString() != transText)
|
|
// Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
//}
|
|
string newvalue = tran.ResolvePathTo();
|
|
if (forceConvertToText)
|
|
newvalue = "?";
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
foreach (Match mm in ms)
|
|
{
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = Text.Substring(myIndex, myLength);
|
|
newvalue = newvalue.Replace("{", @"\{").Replace("}", @"\}");
|
|
if (gg != newvalue && newvalue == "?")
|
|
{
|
|
string rv = ConvertTransitionToText(tran, newvalue);
|
|
//Text = Text.Substring(0, myIndex - 14) + gg + Text.Substring(myIndex + myLength);
|
|
Annotation.MakeAnnotation(this.ContentItems[0].MyItem, ItemInfo.VolianCommentType, "", string.Format("Transition ({0}) converted to text", ItemInfo.ConvertToDisplayText(gg)), null);
|
|
Transition.Delete(tran.TransitionID);
|
|
break;
|
|
}
|
|
else if ((gg.Contains("\\u8209?") ? gg.Replace("\\u8209?", "-") : gg) != (newvalue.Contains("\\u8209?") ? newvalue.Replace("\\u8209?", "-") : newvalue))
|
|
{
|
|
Text = Text.Substring(0, myIndex) + newvalue + Text.Substring(myIndex + myLength);
|
|
break; // Text has been processed
|
|
}
|
|
}
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (tran.MyContent.MyGrid != null)
|
|
{
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
//Match mg = Regex.Match(MyGrid.Data, lookForXml);
|
|
//if (mg != null && mg.Groups.Count > 1)
|
|
//{
|
|
// System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
// if (g.ToString() != transText)
|
|
// MyGrid.Data = MyGrid.Data.Substring(0, g.Index) + transText + MyGrid.Data.Substring(g.Index + g.Length);
|
|
//}
|
|
string findLinkXml = @"<START\].*?\[END>";
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookForXml = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}} \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
MatchCollection msg = Regex.Matches(MyGrid.Data, findLinkXml);
|
|
foreach (Match mmg in msg)
|
|
{
|
|
Match mg = Regex.Match(mmg.Value, lookForXml);// Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
int myIndex = mg.Groups[4].Index + mmg.Index;
|
|
int myLength = mg.Groups[4].Length;
|
|
if (mg.Groups[3].Value != " ")
|
|
{
|
|
myIndex = mg.Groups[3].Index + mmg.Index;
|
|
myLength += mg.Groups[3].Length;
|
|
}
|
|
string gg = MyGrid.Data.Substring(myIndex, myLength);
|
|
if (newvalue.Contains(@"\u8209?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
if (newvalue.Contains(@"\u8593?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8593?", "^");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8593?", m.Groups[1].Value + @"\u8593?" + m.Groups[3].Value);
|
|
}
|
|
if (gg != newvalue)
|
|
{
|
|
MyGrid.Data = MyGrid.Data.Substring(0, myIndex) + newvalue + MyGrid.Data.Substring(myIndex + myLength);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
public string ConvertTransitionToText(TransitionInfo tran, string value)
|
|
{
|
|
string retval = null;
|
|
string newvalue = value;
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
//string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
int lastIndex = 0;
|
|
string newText = Text;
|
|
foreach (Match mm in ms)
|
|
{
|
|
int offset = mm.Index;
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
string prefix = GetMyPrefix(mm.Index, lastIndex);
|
|
string suffix = GetMySuffix(mm.Index + mm.Length);
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = newText.Substring(myIndex, myLength);
|
|
retval = gg;
|
|
gg = gg.Replace("{", @"\{").Replace("}", @"\}");
|
|
string part1 = newText.Substring(0, mm.Index);
|
|
string part2 = gg;
|
|
string part3 = newText.Substring(mm.Index + mm.Length);
|
|
//modify part1 based on prefix
|
|
if (prefix == @"\v ")
|
|
part1 = part1.Substring(0, part1.Length - 3);
|
|
else
|
|
part1 = part1.Substring(0, part1.Length - 3) + " ";
|
|
//modify part3 based on suffix
|
|
if (suffix == @"\v0 ")
|
|
part3 = part3.Substring(4);
|
|
else
|
|
part3 = suffix.Replace(@"\v0", "") + part3.Substring(suffix.Length);
|
|
if (gg.Contains(@"\u8209?"))
|
|
{
|
|
Match mmm = Regex.Match(gg, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (mmm == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
Text = part1 + part2 + part3;
|
|
break; // Text has been processed
|
|
}
|
|
lastIndex = mm.Index + mm.Length;
|
|
}
|
|
//Console.WriteLine("Text: {0} NewText: {1}", Text, newText);
|
|
return retval;
|
|
}
|
|
public string ConvertTransitionToText(int tranID, int tranType, string value)
|
|
{
|
|
string retval = null;
|
|
string newvalue = value;
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tranType, tranID);
|
|
int lastIndex = 0;
|
|
string newText = Text;
|
|
foreach (Match mm in ms)
|
|
{
|
|
int offset = mm.Index;
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
string prefix = GetMyPrefix(mm.Index, lastIndex);
|
|
string suffix = GetMySuffix(mm.Index + mm.Length);
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = newText.Substring(myIndex, myLength);
|
|
retval = gg;
|
|
gg = gg.Replace("{", @"\{").Replace("}", @"\}");
|
|
string part1 = newText.Substring(0, mm.Index);
|
|
string part2 = gg;
|
|
string part3 = newText.Substring(mm.Index + mm.Length);
|
|
//modify part1 based on prefix
|
|
if (prefix == @"\v ")
|
|
part1 = part1.Substring(0, part1.Length - 3);
|
|
else
|
|
part1 = part1.Substring(0, part1.Length - 3) + " ";
|
|
//modify part3 based on suffix
|
|
if (suffix == @"\v0 ")
|
|
part3 = part3.Substring(4);
|
|
else
|
|
part3 = suffix.Replace(@"\v0", "") + part3.Substring(suffix.Length);
|
|
if (gg.Contains(@"\u8209?"))
|
|
{
|
|
Match mmm = Regex.Match(gg, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (mmm == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
Text = part1 + part2 + part3;
|
|
break; // Text has been processed
|
|
}
|
|
lastIndex = mm.Index + mm.Length;
|
|
}
|
|
//Console.WriteLine("Text: {0} NewText: {1}", Text, newText);
|
|
return retval;
|
|
}
|
|
private string GetMySuffix(int start)
|
|
{
|
|
string txt = Text.Substring(start);
|
|
int firstSlashVeeZero = txt.IndexOf(@"\v0");
|
|
if (firstSlashVeeZero == 0 && txt.Length > 3 && txt[3] == ' ') //"\v0 "
|
|
return Text.Substring(start, 4);
|
|
return Text.Substring(start, firstSlashVeeZero + 3); //everything upto \v0"
|
|
}
|
|
private string GetMyPrefix(int start, int lastIndex)
|
|
{
|
|
string defPrefix = Text.Substring(start - 3, 3);
|
|
if (defPrefix != @"\v ") throw new Exception(string.Format("rtf string {0} does not match expected format", defPrefix));
|
|
string txt = Text.Substring(lastIndex, start - lastIndex - 3);
|
|
int lastSlash = txt.LastIndexOf(@"\");
|
|
int lastSpace = txt.LastIndexOf(" ");
|
|
int lastCR = txt.LastIndexOf("\r");
|
|
if (lastSpace < lastCR)
|
|
lastSpace = lastCR;
|
|
if (lastSlash <= lastSpace) //this will return "\v "
|
|
return defPrefix;
|
|
txt = txt.Substring(lastSlash);
|
|
if(txt.StartsWith(@"\'")) //this is a hex
|
|
return defPrefix;
|
|
if (Regex.IsMatch(txt, @"\\u[0-9].*")) //this is unicode
|
|
return defPrefix;
|
|
return @"\v";
|
|
}
|
|
public string ConvertROToText(RoUsageInfo rousg, string value, int rotype, ROFstInfo origROFstInfo)
|
|
{
|
|
string retval = null;
|
|
string newvalue = value;
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
int lastIndex = 0;
|
|
string newText = Text;
|
|
foreach (Match mm in ms)
|
|
{
|
|
int offset = mm.Index;
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
string prefix = GetMyPrefix(mm.Index, lastIndex);
|
|
string suffix = GetMySuffix(mm.Index + mm.Length);
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = newText.Substring(myIndex, myLength);
|
|
retval = gg;
|
|
gg = gg.Replace("{", @"\{").Replace("}", @"\}");
|
|
string part1 = newText.Substring(0, mm.Index);
|
|
string part2 = gg;
|
|
string part3 = newText.Substring(mm.Index + mm.Length);
|
|
//modify part1 based on prefix
|
|
if (prefix == @"\v ")
|
|
part1 = part1.Substring(0, part1.Length - 3);
|
|
else
|
|
part1 = part1.Substring(0, part1.Length - 3) + " ";
|
|
//modify part3 based on suffix
|
|
if (suffix == @"\v0 ")
|
|
part3 = part3.Substring(4);
|
|
else
|
|
part3 = suffix.Replace(@"\v0","") + part3.Substring(suffix.Length);
|
|
if (gg.Contains(@"\u8209?"))
|
|
{
|
|
Match mmm = Regex.Match(gg, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (mmm == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
//System.Text.RegularExpressions.Group g2 = m.Groups[2];
|
|
//if (g2.Value.StartsWith(@"\u8209?"))
|
|
//{
|
|
// string gg = g2.Value + " " + g.Value;
|
|
// retval = gg;
|
|
// part2 = retval;
|
|
//}
|
|
//else
|
|
////else if (g.ToString() != newvalue)
|
|
//{
|
|
// retval = g.Value;
|
|
// part2 = retval;
|
|
//}
|
|
Text = part1 + part2 + part3;
|
|
break; // Text has been processed
|
|
}
|
|
lastIndex = mm.Index + mm.Length;
|
|
}
|
|
//Console.WriteLine("Text: {0} NewText: {1}", Text, newText);
|
|
// see if there is a grid to update too.
|
|
if (rousg.MyContent.MyGrid != null)
|
|
{
|
|
if (rotype == (int)E_ROValueType.Table) // if change in rotable data...
|
|
{
|
|
if (origROFstInfo != null)
|
|
{
|
|
List<string> retlist = origROFstInfo.OnROTableUpdate(this, new ROFstInfoROTableUpdateEventArgs(newvalue, MyGrid.Data));
|
|
if (MyGrid.Data != retlist[1])
|
|
{
|
|
MyGrid.Data = retlist[1];
|
|
retval = Text;
|
|
if (Text != retlist[0])
|
|
Text = retlist[0];
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// if it's an ro within a table, need to process into an flex grid to save the grid data:
|
|
string findLinkXml = @"<START\].*?\[END>";
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookForXml = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}} \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
MatchCollection msg = Regex.Matches(MyGrid.Data, findLinkXml);
|
|
int nmsg = msg.Count;
|
|
for (int i = nmsg - 1; i >= 0; i--)
|
|
{
|
|
Match mmg = msg[i];
|
|
int offset = 0; // crashed in substring line below if using mmg.Index; Set to 0 and it worked - KBR.
|
|
Match mg = Regex.Match(mmg.Value, lookForXml);// Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
int myIndex = mg.Groups[4].Index + mmg.Index;
|
|
int myLength = mg.Groups[4].Length;
|
|
if (mg.Groups[3].Value != " ")
|
|
{
|
|
myIndex = mg.Groups[3].Index + mmg.Index;
|
|
myLength += mg.Groups[3].Length;
|
|
}
|
|
string gg = MyGrid.Data.Substring(myIndex, myLength);
|
|
if (newvalue.Contains(@"\u8209?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
if (gg != newvalue)
|
|
{
|
|
MyGrid.Data = MyGrid.Data.Substring(0, myIndex) + newvalue + MyGrid.Data.Substring(myIndex + myLength);
|
|
}
|
|
//System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
//if (g.ToString() != newvalue)
|
|
//{
|
|
// retval = g.Value;
|
|
// MyGrid.Data = MyGrid.Data.Substring(0, offset + mmg.Index + g.Index) + newvalue + MyGrid.Data.Substring(offset + mmg.Index + g.Index + g.Length);
|
|
//}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return retval == null ? "" : retval;
|
|
}
|
|
public string FixContentText(RoUsageInfo rousg, string value, int rotype, ROFstInfo origROFstInfo) // string newvalue)
|
|
{
|
|
return FixContentText(rousg, value, rotype, origROFstInfo, null);
|
|
}
|
|
public string FixContentText(RoUsageInfo rousg, string value, int rotype, ROFstInfo origROFstInfo,string fileNameOnly) // string newvalue)
|
|
{
|
|
string retval = null;
|
|
if (value == "?")
|
|
{
|
|
retval = this.ConvertROToText(rousg, value, rotype, origROFstInfo);
|
|
if (retval == null) Console.WriteLine("null");
|
|
Annotation.MakeAnnotation(this.ContentItems[0].MyItem, ItemInfo.VolianCommentType, "", string.Format("RO value ({0}) converted to text", ItemInfo.ConvertToDisplayText(retval)), null);
|
|
RoUsage.Delete(rousg.ROUsageID);
|
|
return retval;
|
|
}
|
|
string newvalue = value;
|
|
newvalue = newvalue.Replace("{", @"\{").Replace("}", @"\}");
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
foreach (Match mm in ms)
|
|
{
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if(m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = Text.Substring(myIndex,myLength);
|
|
if ((gg.Replace(@"\'b0", @"\'B0") != newvalue.Replace(@"\'b0", @"\'B0")) && ((fileNameOnly == null) || (gg != fileNameOnly)))
|
|
{
|
|
retval = gg;
|
|
Text = Text.Substring(0, myIndex) + newvalue + Text.Substring(myIndex + myLength);
|
|
break; // Text has been processed
|
|
}
|
|
}
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (rousg.MyContent.MyGrid != null)
|
|
{
|
|
if (rotype == (int)E_ROValueType.Table) // if change in rotable data...
|
|
{
|
|
if (origROFstInfo != null)
|
|
{
|
|
List<string> retlist = origROFstInfo.OnROTableUpdate(this, new ROFstInfoROTableUpdateEventArgs(newvalue, MyGrid.Data));
|
|
if (MyGrid.Data != retlist[1])
|
|
{
|
|
MyGrid.Data = retlist[1];
|
|
retval = Text;
|
|
if (Text != retlist[0])
|
|
Text = retlist[0];
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// if it's an ro within a table, need to process into an flex grid to save the grid data:
|
|
string findLinkXml = @"<START\].*?\[END>";
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookForXml = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}} \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
MatchCollection msg = Regex.Matches(MyGrid.Data, findLinkXml);
|
|
foreach (Match mmg in msg)
|
|
{
|
|
Match mg = Regex.Match(mmg.Value, lookForXml);// Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
int myIndex = mg.Groups[4].Index + mmg.Index;
|
|
int myLength = mg.Groups[4].Length;
|
|
if (mg.Groups[3].Value != " ")
|
|
{
|
|
myIndex = mg.Groups[3].Index + mmg.Index;
|
|
myLength += mg.Groups[3].Length;
|
|
}
|
|
string gg = MyGrid.Data.Substring(myIndex, myLength);
|
|
if (newvalue.Contains(@"\u8209?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
if (newvalue.Contains(@"\u8593?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8593?", "^");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8593?", m.Groups[1].Value + @"\u8593?" + m.Groups[3].Value);
|
|
}
|
|
if (gg != newvalue)
|
|
{
|
|
retval = gg;
|
|
MyGrid.Data = MyGrid.Data.Substring(0, myIndex) + newvalue + MyGrid.Data.Substring(myIndex + myLength);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return retval;
|
|
}
|
|
}
|
|
public delegate void StaticContentInfoEvent(object sender, StaticContentInfoEventArgs args);
|
|
public class StaticContentInfoEventArgs
|
|
{
|
|
string _OldValue;
|
|
public string OldValue
|
|
{
|
|
get { return _OldValue; }
|
|
set { _OldValue = value; }
|
|
}
|
|
string _NewValue;
|
|
public string NewValue
|
|
{
|
|
get { return _NewValue; }
|
|
set { _NewValue = value; }
|
|
}
|
|
string _Type;
|
|
public string Type
|
|
{
|
|
get { return _Type; }
|
|
set { _Type = value; }
|
|
}
|
|
public StaticContentInfoEventArgs(string oldValue, string newValue, string type)
|
|
{
|
|
_OldValue = oldValue;
|
|
_NewValue = newValue;
|
|
_Type = type;
|
|
}
|
|
}
|
|
public partial class ContentInfo
|
|
{
|
|
public static event StaticContentInfoEvent StaticContentInfoChange;
|
|
private static void OnStaticContentInfoChange(object sender, StaticContentInfoEventArgs args)
|
|
{
|
|
if (StaticContentInfoChange != null)
|
|
StaticContentInfoChange(sender, args);
|
|
}
|
|
public string MyContentMessage = string.Empty;
|
|
public string MyGridMessage = string.Empty;
|
|
public bool InList(params int[] IDs)
|
|
{
|
|
foreach (int id in IDs)
|
|
if (id == ContentID) return true;
|
|
return false;
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran, TransitionLookup tranLookup, ItemInfo ii)
|
|
{
|
|
FixTransitionText(tran, tranLookup, ii, false);
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran, TransitionLookup tranLookup, ItemInfo ii, bool forceConvertToText)
|
|
{
|
|
//string transText = tran.ResolvePathTo();
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
////string lookFor = string.Format(@"<START\]\\v0 ([^#]*?)\\v #Link:Transition[^:]*?:{0} {1} [0-9]*\[END>", tran.TranType, tran.TransitionID);
|
|
//Match m = Regex.Match(Text, lookFor);
|
|
//if (m != null && m.Groups.Count > 1)
|
|
//{
|
|
// System.Text.RegularExpressions.Group g = m.Groups[3];
|
|
// if (g.ToString() != transText)
|
|
// _Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
//}
|
|
string newvalue;
|
|
MyContentMessage = string.Empty;
|
|
if (tranLookup == null)
|
|
newvalue = tran.ResolvePathTo();
|
|
else
|
|
newvalue = tran.ResolvePathTo(tranLookup);
|
|
if (forceConvertToText)
|
|
newvalue = "?";
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
foreach (Match mm in ms)
|
|
{
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = Text.Substring(myIndex, myLength);
|
|
MyContentMessage = string.Format("ContentExt.ContentInfo:Content:{0}, {1}, {2}", this.ContentID, tran.TransitionID, gg);
|
|
newvalue = newvalue.Replace("{", @"\{").Replace("}", @"\}");
|
|
if ((gg.Contains("\\u8209?") ? gg.Replace("\\u8209?", "-") : gg) != (newvalue.Contains("\\u8209?") ? newvalue.Replace("\\u8209?", "-") : newvalue))
|
|
{
|
|
_Text = Text.Substring(0, myIndex) + newvalue + Text.Substring(myIndex + myLength);
|
|
OnStaticContentInfoChange(ii, new StaticContentInfoEventArgs(gg, newvalue,"TX"));
|
|
break; // Text has been processed
|
|
}
|
|
}
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (tran.MyContent.MyGrid != null)
|
|
{
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
//Match mg = Regex.Match(MyGrid.Data, lookForXml);
|
|
//if (mg != null && mg.Groups.Count > 1)
|
|
//{
|
|
// System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
// //if (g.ToString() != transText)
|
|
// // MyGrid.Data = MyGrid.Data.Substring(0, g.Index) + transText + MyGrid.Data.Substring(g.Index + g.Length);
|
|
//}
|
|
MyGridMessage = string.Empty;
|
|
string findLinkXml = @"<START\].*?\[END>";
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookForXml = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}} \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
MatchCollection msg = Regex.Matches(MyGrid.Data, findLinkXml);
|
|
foreach (Match mmg in msg)
|
|
{
|
|
Match mg = Regex.Match(mmg.Value, lookForXml);// Regex.Match(MyGrid.Data, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
int myIndex = mg.Groups[4].Index + mmg.Index;
|
|
int myLength = mg.Groups[4].Length;
|
|
if (mg.Groups[3].Value != " ")
|
|
{
|
|
myIndex = mg.Groups[3].Index + mmg.Index;
|
|
myLength += mg.Groups[3].Length;
|
|
}
|
|
string gg = MyGrid.Data.Substring(myIndex, myLength);
|
|
if (newvalue.Contains(@"\u8209?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
if (newvalue.Contains(@"\u8593?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m == null)
|
|
newvalue = newvalue.Replace(@"\u8593?", "^");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8593?", m.Groups[1].Value + @"\u8593?" + m.Groups[3].Value);
|
|
}
|
|
MyGridMessage = string.Format("ContentExt.ContentInfo:Grid:{0}, {1}, {2}", this.ContentID, tran.TransitionID, gg);
|
|
if (gg != newvalue)
|
|
{
|
|
MyGrid.SetData(MyGrid.Data.Substring(0, myIndex) + newvalue + MyGrid.Data.Substring(myIndex + myLength));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran, ItemInfo ii)
|
|
{
|
|
FixTransitionText(tran, ii, false);
|
|
}
|
|
public void FixTransitionText(TransitionInfo tran, ItemInfo ii, bool forceConvertToText)
|
|
{
|
|
FixTransitionText(tran, null, ii, forceConvertToText);
|
|
//string transText = tran.ResolvePathTo(tranLookup);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
////string lookFor = string.Format(@"<START\]\\v0 ([^#]*?)\\v #Link:Transition[^:]*?:{0} {1} [0-9]*\[END>", tran.TranType, tran.TransitionID);
|
|
//Match m = Regex.Match(Text, lookFor);
|
|
//if (m != null && m.Groups.Count > 1)
|
|
//{
|
|
// System.Text.RegularExpressions.Group g = m.Groups[3];
|
|
// if (g.ToString() != transText)
|
|
// _Text = Text.Substring(0, g.Index) + transText + Text.Substring(g.Index + g.Length);
|
|
//}
|
|
//// see if there is a grid to update too.
|
|
//if (MyGrid != null)
|
|
//{
|
|
// string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* ([^#]*?)(\\[^v'? \\]+)*\\v(\\[^v \\]+)* #Link:Transition[^:]*?:{0} {1}( [0-9]*){{1,2}}\[END>", tran.TranType, tran.TransitionID);
|
|
// Match mg = Regex.Match(MyGrid.Data, lookForXml);
|
|
// if (mg != null && mg.Groups.Count > 1)
|
|
// {
|
|
// System.Text.RegularExpressions.Group g = mg.Groups[3];
|
|
// if (g.ToString() != transText)
|
|
// MyGrid.SetData(MyGrid.Data.Substring(0, g.Index) + transText + MyGrid.Data.Substring(g.Index + g.Length));
|
|
// }
|
|
//}
|
|
}
|
|
public void FixContentText(RoUsageInfo rousg, string value, int rotype, ROFstInfo origROFstInfo, ItemInfo ii) // string newvalue)
|
|
{
|
|
string newvalue = value;
|
|
newvalue = newvalue.Replace("{", @"\{").Replace("}", @"\}");
|
|
string findLink = @"<START\].*?\[END>";
|
|
MatchCollection ms = Regex.Matches(Text, findLink);
|
|
//string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookFor = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}~\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}}~ \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
foreach (Match mm in ms)
|
|
{
|
|
int offset = mm.Index;
|
|
Match m = Regex.Match(mm.Value, lookFor, RegexOptions.Singleline);
|
|
if (m != null && m.Groups.Count > 1)
|
|
{
|
|
int myIndex = m.Groups[4].Index + mm.Index;
|
|
int myLength = m.Groups[4].Length;
|
|
if (m.Groups[3].Value != " ")
|
|
{
|
|
myIndex = m.Groups[3].Index + mm.Index;
|
|
myLength += m.Groups[3].Length;
|
|
}
|
|
string gg = Text.Substring(myIndex, myLength);
|
|
if ((gg.Replace(@"\'b0", @"\'B0") != newvalue.Replace(@"\'b0", @"\'B0")))
|
|
{
|
|
_Text = Text.Substring(0, myIndex) + newvalue + _Text.Substring(myIndex + myLength);
|
|
OnStaticContentInfoChange(ii, new StaticContentInfoEventArgs(gg, newvalue, "RO"));
|
|
break; // Text has been processed
|
|
}
|
|
}
|
|
}
|
|
// see if there is a grid to update too.
|
|
if (MyGrid != null)
|
|
{
|
|
if (rotype == (int)E_ROValueType.Table) // if change in rotable data...
|
|
{
|
|
List<string> retlist = origROFstInfo.OnROTableUpdate(this, new ROFstInfoROTableUpdateEventArgs(newvalue, MyGrid.Data));
|
|
if (Text != retlist[0]) _Text = retlist[0];
|
|
//if (MyGrid.Data != retlist[1]) MyGrid.Data = retlist[1];
|
|
}
|
|
else
|
|
{
|
|
// if it's an ro within a table, need to process into an flex grid to save the grid data:
|
|
string findLinkXml = @"<START\].*?\[END>";
|
|
//string lookForXml = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v '?\\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>", rousg.ROUsageID);
|
|
string lookForXml = string.Format(@"^<START\](\\[^v \\]+)*\\v0(\\[^v '?{{}}\\]+)*( |\\u[0-9]{{1,4}}?|\\'[0-9a-fA-F]{{2}}|\\[{{}}~])(.*?)(\\[^v'?{{}} \\]+)*\\v(\\[^v \\]+)* #Link:ReferencedObject:{0} .*?\[END>$", rousg.ROUsageID);
|
|
MatchCollection msg = Regex.Matches(MyGrid.Data, findLinkXml);
|
|
foreach (Match mmg in msg)
|
|
{
|
|
//int offset = 0; // crashed in substring line below if using mmg.Index; Set to 0 and it worked - KBR.
|
|
Match mg = Regex.Match(mmg.Value, lookForXml);
|
|
if (mg != null && mg.Groups.Count > 1)
|
|
{
|
|
int myIndex = mg.Groups[4].Index;
|
|
int myLength = mg.Groups[4].Length;
|
|
if (mg.Groups[3].Value != " ")
|
|
{
|
|
myIndex = mg.Groups[3].Index ;
|
|
myLength += mg.Groups[3].Length;
|
|
}
|
|
string gg = MyGrid.Data.Substring(myIndex+ mmg.Index, myLength);
|
|
if (newvalue.Contains(@"\u8209?"))
|
|
{
|
|
Match m = Regex.Match(gg, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m.Groups.Count < 3)
|
|
newvalue = newvalue.Replace(@"\u8209?", "-");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8209?", m.Groups[1].Value + @"\u8209?" + m.Groups[3].Value);
|
|
}
|
|
if (newvalue.Contains(@"\u8593?"))
|
|
{
|
|
Match m = Regex.Match(newvalue, @"(\\f[0-9]+)(\\u[0-9]{1,4}\?)(\\f[0-9]+ ?)");
|
|
if (m.Groups.Count < 3)
|
|
newvalue = newvalue.Replace(@"\u8593?", "^");
|
|
else
|
|
newvalue = newvalue.Replace(@"\u8593?", m.Groups[1].Value + @"\u8593?" + m.Groups[3].Value);
|
|
}
|
|
if (gg != newvalue)
|
|
{
|
|
string prefix1 = MyGrid.Data.Substring(0,mmg.Index);
|
|
string prefix2 = mmg.Value.Substring(0,myIndex);
|
|
string suffix1 = MyGrid.Data.Substring(mmg.Index + mmg.Length);
|
|
string suffix2 = mmg.Value.Substring(myIndex + myLength);
|
|
MyGrid.SetData(prefix1 + prefix2 + newvalue + suffix2 + suffix1);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
if (_Text == string.Empty)
|
|
_Text = "?";
|
|
}
|
|
public void LoadNonCachedGrid()
|
|
{
|
|
_MyGrid = GridInfo.GetNonCached(ContentID);
|
|
//Console.WriteLine("LoadNonCachedGrid {0},{1},{2}",ContentID,_MyGrid==null,_MyContentInfoUnique);
|
|
}
|
|
public PartInfoList LocalContentParts
|
|
{
|
|
get { return _ContentParts; }
|
|
}
|
|
public void AddPart(SafeDataReader dr, ItemInfo itemInfo)
|
|
{
|
|
if (_ContentParts == null)
|
|
_ContentParts = new PartInfoList(dr, itemInfo);
|
|
else
|
|
_ContentParts.AddPartInfo(dr, itemInfo);
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0} {1}", Number, Text);
|
|
}
|
|
public void ShowChange()
|
|
{
|
|
OnChange();
|
|
}
|
|
//public XmlNode ToXml(XmlNode xn)
|
|
//{
|
|
// XmlNode nd = xn.OwnerDocument.CreateElement("Content");
|
|
// xn.AppendChild(nd);
|
|
// AddAttribute(nd, "Number", _Number);
|
|
// AddAttribute(nd, "Text", _Text);
|
|
// AddAttribute(nd, "FormatID", _FormatID);
|
|
// AddAttribute(nd, "Config", _Config);
|
|
// return nd;
|
|
//}
|
|
//public void AddAttribute(XmlNode xn, string name, object o)
|
|
//{
|
|
// if (o != null && o.ToString() != "")
|
|
// {
|
|
// XmlAttribute xa = xn.OwnerDocument.CreateAttribute(name);
|
|
// xa.Value = o.ToString();
|
|
// xn.Attributes.Append(xa);
|
|
// }
|
|
//}
|
|
internal ContentInfo(SafeDataReader dr,bool ForItem)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ContentInfo.Constructor", GetHashCode());
|
|
try
|
|
{
|
|
ReadDataItemList(dr);
|
|
_CacheList.Add(this);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ContentInfo.Constructor", ex);
|
|
throw new DbCslaException("ContentInfo.Constructor", ex);
|
|
}
|
|
}
|
|
private void ReadDataItemList(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ContentInfo.ReadDataItemList", GetHashCode());
|
|
try
|
|
{
|
|
_ContentID = dr.GetInt32("ContentID");
|
|
_Number = dr.GetString("Number");
|
|
_Text = dr.GetString("Text");
|
|
_Type = (int?)dr.GetValue("Type");
|
|
_FormatID = (int?)dr.GetValue("FormatID");
|
|
_Config = dr.GetString("Config");
|
|
_DTS = dr.GetDateTime("cDTS");
|
|
_UserID = dr.GetString("cUserID");
|
|
_ContentDetailCount = dr.GetInt32("DetailCount");
|
|
_ContentEntryCount = dr.GetInt32("EntryCount");
|
|
_ContentGridCount = dr.GetInt32("GridCount");
|
|
_ContentImageCount = dr.GetInt32("ImageCount");
|
|
_ContentItemCount = dr.GetInt32("ItemCount");
|
|
_ContentPartCount = dr.GetInt32("cPartCount");
|
|
_ContentRoUsageCount = dr.GetInt32("RoUsageCount");
|
|
_ContentTransitionCount = dr.GetInt32("TransitionCount");
|
|
_ContentZContentCount = dr.GetInt32("ZContentCount");
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ContentInfo.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("ContentInfo.ReadData", ex);
|
|
}
|
|
}
|
|
|
|
}
|
|
public partial class ContentInfoList
|
|
{
|
|
public static ContentInfoList GetList(int? itemID)
|
|
{
|
|
try
|
|
{
|
|
ContentInfoList tmp = DataPortal.Fetch<ContentInfoList>(new ContentListCriteria(itemID));
|
|
ContentInfo.AddList(tmp);
|
|
tmp.AddEvents();
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ItemInfoList.GetChildren", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ContentListCriteria
|
|
{
|
|
public ContentListCriteria(int? itemID)
|
|
{
|
|
_ItemID = itemID;
|
|
}
|
|
private int? _ItemID;
|
|
public int? ItemID
|
|
{
|
|
get { return _ItemID; }
|
|
set { _ItemID = value; }
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ContentListCriteria criteria)
|
|
{
|
|
this.RaiseListChangedEvents = false;
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "vesp_ListContentsByItemID";
|
|
cm.Parameters.AddWithValue("@ItemID", criteria.ItemID);
|
|
cm.CommandTimeout = Database.DefaultTimeout;
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
IsReadOnly = false;
|
|
while (dr.Read())
|
|
{
|
|
ContentInfo contentInfo = new ContentInfo(dr);
|
|
this.Add(contentInfo);
|
|
}
|
|
IsReadOnly = true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Database.LogException("ContentInfoList.DataPortal_Fetch", ex);
|
|
throw new DbCslaException("ContentInfoList.DataPortal_Fetch", ex);
|
|
}
|
|
this.RaiseListChangedEvents = true;
|
|
}
|
|
}
|
|
}
|