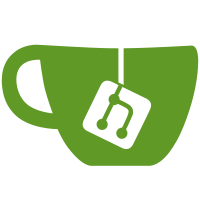
- If the range does not have any characters return an empty string Refresh Most Recent Items when the office button is pressed Added OrdinalChanged Event Used OrdinalChanged Event rather than MyContent.Changed Event when Ordinal is updated. Use OrdinalChanged Event to update Tree Node Refresh MRU list as items are added Use OrdinalChanged Event to update Step Tab - RTBFillIn changed to only update the RTB when the contents change - AdjustSizeForContents changed to raise the HeightChanged event when the Height changes Update the treeview tabs even if the step is not open.
2387 lines
79 KiB
C#
2387 lines
79 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Text.RegularExpressions;
|
|
using Volian.Base.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
#region Enums
|
|
public enum ChildRelation : int
|
|
{
|
|
None = 0,
|
|
After = 1,
|
|
Before = 2,
|
|
RNO = 3
|
|
}
|
|
public enum ExpandingStatus : int
|
|
{
|
|
No = 0,
|
|
Expanding = 1,
|
|
Colapsing = 2,
|
|
Hiding = 4,
|
|
Showing = 8,
|
|
Done = 16
|
|
}
|
|
#endregion
|
|
public partial class StepItem : UserControl
|
|
{
|
|
//private static int _StepItemUnique = 0;
|
|
//private static int StepItemUnique
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_StepItemUnique == 3)
|
|
// Console.WriteLine("here");
|
|
// return ++_StepItemUnique;
|
|
// }
|
|
//}
|
|
//private int _MyStepItemUnique = StepItemUnique;
|
|
|
|
//public int MyStepItemUnique
|
|
//{
|
|
// get {return _MyStepItemUnique; }
|
|
//}
|
|
|
|
#region Private Fields
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
private StepPanel _MyStepPanel;
|
|
|
|
public StepPanel MyStepPanel
|
|
{
|
|
get { return _MyStepPanel; }
|
|
set { _MyStepPanel = value; }
|
|
}
|
|
private ChildRelation _MyChildRelation;
|
|
private StepItem _MyParentStepItem = null;
|
|
private StepItem _MySectionStepItem;
|
|
private StepItem _MyPreviousStepItem = null;
|
|
private StepItem _MyNextStepItem = null;
|
|
private bool _ChildrenLoaded = false;
|
|
public bool HasChildren
|
|
{
|
|
get {return _MyBeforeStepItems != null || _MyRNOStepItems != null || _MyAfterStepItems != null;}
|
|
}
|
|
private List<StepItem> _MyBeforeStepItems;
|
|
|
|
public List<StepItem> MyBeforeStepItems
|
|
{
|
|
get { return _MyBeforeStepItems; }
|
|
set { _MyBeforeStepItems = value; }
|
|
}
|
|
private List<StepItem> _MyAfterStepItems;
|
|
|
|
public List<StepItem> MyAfterStepItems
|
|
{
|
|
get { return _MyAfterStepItems; }
|
|
set { _MyAfterStepItems = value; }
|
|
}
|
|
private List<StepItem> _MyRNOStepItems;
|
|
|
|
public List<StepItem> MyRNOStepItems
|
|
{
|
|
get { return _MyRNOStepItems; }
|
|
set { _MyRNOStepItems = value; }
|
|
}
|
|
public Label MyLabel
|
|
{ get { return lblTab; } }
|
|
private StepSectionLayoutData _MyStepSectionLayoutData;
|
|
public StepSectionLayoutData MyStepSectionLayoutData
|
|
{
|
|
get { return _MyStepSectionLayoutData; }
|
|
set { _MyStepSectionLayoutData = value; }
|
|
}
|
|
private bool _Loading = true;
|
|
private StepData _MyStepData;
|
|
public StepData MyStepData
|
|
{
|
|
get { return _MyStepData; }
|
|
set { _MyStepData = value; }
|
|
}
|
|
private ItemInfo _MyItemInfo;
|
|
private static int _WidthAdjust = 3;
|
|
|
|
private int _ExpandPrefix = 0;
|
|
private int _ExpandSuffix = 0;
|
|
private ExpandingStatus _MyExpandingStatus = ExpandingStatus.No;
|
|
private bool _Colapsing = false;
|
|
private bool _Moving = false;
|
|
private int _RNOLevel = 0;
|
|
private int _SeqLevel = 0;
|
|
private int _Type;
|
|
private bool _Circle = false;
|
|
private bool _CheckOff = false;
|
|
private bool _ChangeBar = false;
|
|
#endregion
|
|
#region Properties
|
|
/// <summary>
|
|
/// This returns the section or procedure for the current item.
|
|
/// If the item is a step or section, it returns the section
|
|
/// If it is a procedure, it returns the procedure
|
|
/// </summary>
|
|
public StepItem MySectionStepItem
|
|
{
|
|
get
|
|
{
|
|
if (_MySectionStepItem == null)
|
|
{
|
|
if (MyItemInfo.IsSection || MyItemInfo.IsProcedure) _MySectionStepItem = this;
|
|
else _MySectionStepItem = _MyParentStepItem.MySectionStepItem;
|
|
}
|
|
return _MySectionStepItem;
|
|
}
|
|
set { _MySectionStepItem = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or Sets ItemInfo
|
|
/// </summary>
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set
|
|
{
|
|
_MyItemInfo = value;
|
|
if (VlnSettings.StepTypeToolType)SetToolTip(_MyItemInfo.ToolTip);
|
|
ChangeBar = _MyItemInfo.HasChangeBar();
|
|
value.MyContent.Changed += new ContentInfoEvent(MyContent_Changed);
|
|
value.OrdinalChanged += new ItemInfoEvent(value_OrdinalChanged);
|
|
}
|
|
}
|
|
void value_OrdinalChanged(object sender)
|
|
{
|
|
TabFormat = null; // Reset Tab
|
|
}
|
|
private void SetToolTip(string tip)
|
|
{
|
|
DevComponents.DotNetBar.SuperTooltipInfo tpi = new DevComponents.DotNetBar.SuperTooltipInfo("", "", tip, null, null, DevComponents.DotNetBar.eTooltipColor.Lemon);
|
|
_MyToolTip.MinimumTooltipSize = new Size(0, 24);
|
|
_MyToolTip.TooltipDuration = 3;
|
|
_MyToolTip.SetSuperTooltip(MyStepRTB, tpi);
|
|
}
|
|
|
|
public StepItem ActiveParent
|
|
{
|
|
get { return _MyParentStepItem!=null ? _MyParentStepItem : _MyPreviousStepItem.ActiveParent; }
|
|
}
|
|
void MyContent_Changed(object sender)
|
|
{
|
|
// Update the text to reflect the content change
|
|
MyStepRTB.MyItemInfo.RefreshItemAnnotations();
|
|
MyStepRTB.MyItemInfo=MyStepRTB.MyItemInfo; // Reset Text
|
|
SetExpandAndExpander(MyItemInfo);
|
|
// TODO: Need code to update tabs ? not sure what this is - maybe for
|
|
// transitions?
|
|
}
|
|
/// <summary>
|
|
/// Used to connect the RichTextBox with the menus and toolbars
|
|
/// </summary>
|
|
public StepRTB MyStepRTB
|
|
{
|
|
get { return _MyStepRTB; }
|
|
}
|
|
/// <summary>
|
|
/// Return the Parent StepItem
|
|
/// </summary>
|
|
private StepItem UpOneStepItem
|
|
{
|
|
get
|
|
{
|
|
StepItem tmp = this;
|
|
while (tmp != null && tmp.MyParentStepItem == null) tmp = tmp.MyPreviousStepItem;
|
|
if (tmp != null) return tmp.MyParentStepItem;
|
|
return null;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Sets the parent and postions the item with respect to the parent
|
|
/// </summary>
|
|
public StepItem MyParentStepItem
|
|
{
|
|
get { return _MyParentStepItem; }
|
|
set
|
|
{
|
|
LastMethodsPush("set_MyParentStepItem");
|
|
_MyParentStepItem = value;
|
|
if (_MyParentStepItem != null)
|
|
{
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.None: // Same as after
|
|
case ChildRelation.After: // Procedures, sections, substeps, and tables/figures
|
|
// The size depends upon the parent type
|
|
int iType = (int)_MyParentStepItem._Type;
|
|
|
|
switch (iType / 10000)
|
|
{
|
|
case 0: // Procedure
|
|
ItemLocation = new Point(_MyParentStepItem.ItemLocation.X + 20, _MyParentStepItem.Bottom);
|
|
ItemWidth = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColT) + _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidT);
|
|
break;
|
|
case 1: // Section
|
|
if(this == TopMostStepItem)
|
|
ItemLocation = new Point(_MyParentStepItem.ItemLocation.X + 20, _MyParentStepItem.Bottom);
|
|
else
|
|
TopMostStepItem.ItemLocation = new Point(TopMostStepItem.ItemLocation.X, _MyParentStepItem.Bottom);
|
|
int borderWidth = _MyStepRTB.Width - _MyStepRTB.ClientRectangle.Width;
|
|
//TextWidth = _WidthAdjust + borderWidth + _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidSTableEdit, Convert.ToInt32(_MyStepSectionLayoutData.PMode) - 1);
|
|
TextWidth = _WidthAdjust + borderWidth + _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidSTableEdit, MyItemInfo.ColumnMode);
|
|
break;
|
|
case 2: // Step
|
|
// if Table then determine width and location based upon it's parent's location
|
|
if (_MyStepData.Type == "Table" || _MyStepData.ParentType == "Table")
|
|
{
|
|
_MyStepRTB.Font = _MyStepData.Font.WindowsFont;
|
|
ItemWidth = (int)TableWidth(_MyStepRTB.Font, _MyItemInfo.MyContent.Text, true);
|
|
ItemLocation = new Point(50, _MyParentStepItem.Bottom);
|
|
ItemLocation = TableLocation(_MyParentStepItem, _MyStepSectionLayoutData, ItemWidth);
|
|
}
|
|
else
|
|
{
|
|
ItemLocation = new Point(_MyParentStepItem.TextLeft, _MyParentStepItem.Bottom);
|
|
ItemWidth = _MyParentStepItem.TextWidth;
|
|
}
|
|
break;
|
|
}
|
|
break;
|
|
case ChildRelation.RNO:
|
|
// this is set so that TextWidth command below will be correct. TextWidth uses
|
|
// the tab start & tab width to calculate the overall width.
|
|
TabFormat = ""; // this is set so that TextWidth command below will be correct
|
|
_IgnoreResize = true;
|
|
TextWidth = _MyParentStepItem.TextWidth;
|
|
_IgnoreResize = false;
|
|
if (RNOLevel <= _MyItemInfo.ColumnMode)
|
|
{
|
|
//int colR = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColRTable, Convert.ToInt32(_MyStepSectionLayoutData.PMode) - 1);
|
|
int colR = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColRTable, MyItemInfo.ColumnMode);
|
|
if (colR - _MyParentStepItem.Width < 0) colR = _MyParentStepItem.Width + 0;
|
|
_MyStepPanel.ItemMoving++;
|
|
//Left = _MyParentStepItem.ItemLeft + RNOLevel * colR;
|
|
//ItemLocation = new Point(_MyParentStepItem.ItemLeft + RNOLevel * colR, _MyParentStepItem.Top);
|
|
LastMethodsPush(string.Format("set_MyParentStepItem RNO Right {0}", MyID));
|
|
ItemLocation = new Point(_MyParentStepItem.ItemLeft + RNOLevel * colR, _MyParentStepItem.Top);
|
|
int top = _MyParentStepItem.FindTop(_MyParentStepItem.Top);
|
|
if (top != _MyParentStepItem.Top)
|
|
{
|
|
_MyParentStepItem.LastMethodsPush(string.Format("set_MyParentStepItem RNO Right {0}", MyID));
|
|
_MyParentStepItem.Top = top;
|
|
_MyParentStepItem.LastMethodsPop();
|
|
Top = top;
|
|
}
|
|
LastMethodsPop();
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
else
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
LastMethodsPush(string.Format("set_MyParentStepItem RNO Below {0} {1} {2}", MyID, _MyParentStepItem.BottomMostStepItem.MyID, _MyParentStepItem.BottomMostStepItem.Bottom));
|
|
TextLocation = new Point(_MyParentStepItem.TextLeft, _MyParentStepItem.BottomMostStepItem.Bottom);
|
|
LastMethodsPop();
|
|
//TextLocation = new Point(_MyParentStepItem.TextLeft, FindTop(_MyParentStepItem.Top));
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
// Same size as the Parent
|
|
break;
|
|
case ChildRelation.Before: // Cautions and Notes
|
|
//if(_WatchThis > 0 && MyID > 2111)
|
|
// Console.WriteLine("Setting MyParent: \r\n\tParent {0},{1} \r\n\tTopMostItem {2},{3} \r\n\tNext {4}, {5}", _MyParentStepItem.MyID, _MyParentStepItem
|
|
// ,_MyParentStepItem.TopMostStepItem.MyID,_MyParentStepItem.TopMostStepItem
|
|
// , _MyNextStepItem == null ? 0 : _MyNextStepItem.MyID, _MyNextStepItem == null ? "None" : _MyNextStepItem.ToString());
|
|
|
|
//Location = new Point(_MyParentStepItem.Left + 20, max(_MyParentStepItem.Top, (_MyNextStepItem == null ? 0 : _MyNextStepItem.Top )) ?? 0);
|
|
_IgnoreResize = true;
|
|
Width = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidT);
|
|
_IgnoreResize = false;
|
|
_MyStepPanel.ItemMoving++;
|
|
//Location = new Point(_MyParentStepItem.Left + 20, FindTop(_MyParentStepItem.Top));
|
|
int myTop = MyNextStepItem == null ? _MyParentStepItem.Top : MyNextStepItem.Top;
|
|
Location = new Point(_MyParentStepItem.Left + 20, FindTop(myTop));
|
|
_MyStepPanel.ItemMoving--;
|
|
//_MyParentStepItem.Top = Bottom;
|
|
// Could be a Caution or Note - Need to get WidT
|
|
break;
|
|
}
|
|
}
|
|
LastMethodsPop();
|
|
}
|
|
}
|
|
private Stack<string> _LastMethods= new Stack<string>();
|
|
//private string _LastMethod = "";
|
|
private void LastMethodsPush(string str)
|
|
{
|
|
_MyStepPanel._LastAdjust = str;
|
|
_LastMethods.Push(str);
|
|
}
|
|
private string LastMethodsPop()
|
|
{
|
|
//_MyStepPanel._LastAdjust = "";
|
|
return _LastMethods.Pop();
|
|
}
|
|
private bool LastMethodsEmpty
|
|
{
|
|
get { return _LastMethods.Count == 0; }
|
|
}
|
|
/// <summary>
|
|
/// This should find the item that precedes the current item vertically
|
|
/// and then return the Bottom of that item.
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
private int FindTop(int bottom)
|
|
{
|
|
int lastBottomPrev = bottom; // This is necessary if the value of bottom can be negative.
|
|
if (_MyPreviousStepItem != null)
|
|
lastBottomPrev = _MyPreviousStepItem.BottomMostStepItem.Bottom;
|
|
int? bottomRNO = BottomOfParentRNO();
|
|
if (lastBottomPrev > bottom) bottom = (int)(lastBottomPrev); // RHM 20090615 ES02 Step8
|
|
// Moving from Step 8 to the Note preceeding step 8 caused the step 9 to be positioned in the wrong place.
|
|
//if (lastBottomParent > bottom) bottom = lastBottomParent;
|
|
//if (bottomRNO == null) return bottom;
|
|
return (int) max(bottomRNO, bottom);
|
|
}
|
|
/// <summary>
|
|
/// The left edge of the Tab
|
|
/// </summary>
|
|
public int ItemLeft
|
|
{
|
|
get { return Left + lblTab.Left; }
|
|
set { Left = value - lblTab.Left; }
|
|
}
|
|
/// <summary>
|
|
/// The Top of the StepItem
|
|
/// </summary>
|
|
public int ItemTop
|
|
{
|
|
get { return Top; }
|
|
set
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
Top = value;
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// The Location of the Tab
|
|
/// </summary>
|
|
public Point ItemLocation
|
|
{
|
|
get { return new Point(Location.X + lblTab.Left, Location.Y); }
|
|
set { Location = new Point(value.X - lblTab.Left, value.Y); }
|
|
}
|
|
/// <summary>
|
|
/// Width of the Tab and RTB
|
|
/// </summary>
|
|
public int ItemWidth
|
|
{
|
|
get { return Width - lblTab.Left; }
|
|
set
|
|
{
|
|
Width = value + lblTab.Left;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Width of the RichTextBox
|
|
/// </summary>
|
|
public int TextWidth
|
|
{
|
|
get { return _MyStepRTB.Width; }
|
|
set
|
|
{
|
|
Width = value + lblTab.Left + lblTab.Width;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Location of the RichTextBox
|
|
/// </summary>
|
|
public Point TextLocation
|
|
{
|
|
get { return new Point(Location.X + _MyStepRTB.Left, Location.Y); }
|
|
set { Location = new Point(value.X - _MyStepRTB.Left, value.Y); }
|
|
}
|
|
/// <summary>
|
|
/// Left edge of the RichTextBox
|
|
/// </summary>
|
|
public int TextLeft
|
|
{
|
|
get { return Left + _MyStepRTB.Left; }
|
|
}
|
|
/// <summary>
|
|
/// Tab Format used for outputing the Tab
|
|
/// </summary>
|
|
private string _TabFormat;
|
|
public string TabFormat
|
|
{
|
|
get
|
|
{
|
|
if (_TabFormat == null) TabFormat = null; // execute 'set' code.
|
|
return _TabFormat;
|
|
}
|
|
set
|
|
{
|
|
if (_MyItemInfo != null)
|
|
{
|
|
ItemInfo.ResetTabString(MyID);
|
|
string tabString = _MyItemInfo.MyTab.CleanText;
|
|
lblTab.Text = tabString;
|
|
lblTab.Width = tabString.Length * 8 * MyStepPanel.DPI / 96;// Adjust width for DPI
|
|
Invalidate();
|
|
_MyStepRTB.Left = lblTab.Left + lblTab.Width;// +2;
|
|
_MyStepRTB.Width = Width - _MyStepRTB.Left;
|
|
_TabFormat = value; // tabString;
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// This is the "number" of the item (1, 2, 3 etc.) for Substeps
|
|
/// </summary>
|
|
public int Ordinal
|
|
{
|
|
get
|
|
{
|
|
int count = 1;
|
|
for (StepItem tmp = this; tmp.MyPreviousStepItem != null; tmp = tmp.MyPreviousStepItem) count++;
|
|
return count;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Sets the previous item and adjusts locations
|
|
/// </summary>
|
|
public StepItem MyPreviousStepItem
|
|
{
|
|
get { return _MyPreviousStepItem; }
|
|
set
|
|
{
|
|
LastMethodsPush("set_MyPreviousStepItem");
|
|
_MyPreviousStepItem = value;
|
|
if (_MyPreviousStepItem != null)
|
|
{
|
|
_IgnoreResize=true;
|
|
if (_MyStepData != null && (_MyStepData.Type.ToLower().Contains("table") || _MyStepData.ParentType.ToLower().Contains("table")))
|
|
{
|
|
ItemWidth = (int)TableWidth(_MyStepRTB.Font, _MyItemInfo.MyContent.Text, true);
|
|
Location = new Point(_MyPreviousStepItem.Left, FindTop(_MyPreviousStepItem.BottomMostStepItem.Bottom));
|
|
}
|
|
else if (value.MyItemInfo.IsTablePart)
|
|
{
|
|
ItemLocation = new Point(value.MyParentStepItem.TextLeft, value.MyParentStepItem.Bottom);
|
|
ItemWidth = value.MyParentStepItem.TextWidth;
|
|
}
|
|
else
|
|
{
|
|
Width = MyPreviousStepItem.Width;
|
|
if (TopMostStepItem == this)
|
|
Location = new Point(_MyPreviousStepItem.Left, FindTop(_MyPreviousStepItem.BottomMostStepItem.Bottom));
|
|
else
|
|
TopMostStepItem.Location = new Point(TopMostStepItem.Left, FindTop(_MyPreviousStepItem.BottomMostStepItem.Bottom));
|
|
}
|
|
_IgnoreResize=false;
|
|
//ShowMe("");
|
|
//if (MyID > _StartingID)
|
|
// Console.WriteLine("{0}-->Setting MyPreviousStepItem {1},{2},{3},{4},{5},{6},{7}", WatchThisIndent, MyID, this
|
|
// , _MyPreviousStepItem
|
|
// ,Top
|
|
// , _MyPreviousStepItem.BottomMostStepItem.Bottom
|
|
// , FindTop(_MyPreviousStepItem.BottomMostStepItem.Bottom)
|
|
// , _MyPreviousStepItem.Bottom);
|
|
//Location = new Point(_MyPreviousStepItem.Left, _MyPreviousStepItem.BottomMostStepItem.Bottom);
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.None:
|
|
break;
|
|
case ChildRelation.After:
|
|
break;
|
|
case ChildRelation.RNO:
|
|
break;
|
|
case ChildRelation.Before:
|
|
//_MyStepPanel.ItemMoving++;
|
|
//UpOneStepItem.Top = BottomMostStepItem.Bottom;
|
|
//_MyStepPanel.ItemMoving--;
|
|
break;
|
|
}
|
|
if (_MyPreviousStepItem.MyNextStepItem != this) _MyPreviousStepItem.MyNextStepItem = this;
|
|
}
|
|
LastMethodsPop();
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Sets the next item and adjusts the location,
|
|
/// This also sets the previous for the "next" item
|
|
/// which adjusts other locations.
|
|
/// </summary>
|
|
public StepItem MyNextStepItem
|
|
{
|
|
get { return _MyNextStepItem; }
|
|
set
|
|
{
|
|
_MyNextStepItem = value;
|
|
if (_MyNextStepItem != null)
|
|
{
|
|
if (_MyNextStepItem.MyPreviousStepItem != this)
|
|
{
|
|
_MyNextStepItem.MyPreviousStepItem = this;
|
|
MyNextStepItem.Location = new Point(Left, Bottom);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// returns the TopMostChild
|
|
/// </summary>
|
|
public StepItem TopMostStepItem
|
|
{
|
|
get
|
|
{
|
|
if (Expanded && _MyBeforeStepItems != null) return _MyBeforeStepItems[0].TopMostStepItem;
|
|
return this;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Returns the bottom location (Top + Height)
|
|
/// </summary>
|
|
public new int Bottom
|
|
{
|
|
get
|
|
{
|
|
return Top + (Hidden ? 0 : Height);
|
|
}
|
|
}
|
|
private bool _Hidden = false;
|
|
public bool Hidden
|
|
{
|
|
get { return _Hidden; }
|
|
set { _Hidden = value; Visible = !value; }
|
|
}
|
|
/// <summary>
|
|
/// Bottom most child
|
|
/// </summary>
|
|
public StepItem BottomMostStepItem
|
|
{
|
|
get
|
|
{
|
|
StepItem tmpr = null; // BottomMost RNO
|
|
//int rnoOffset = 0;
|
|
if ((MyExpandingStatus != ExpandingStatus.No || Expanded) && _MyRNOStepItems != null)
|
|
{
|
|
//rnoOffset = this.Top - _MyRNOStepItems[0].Top;
|
|
tmpr = _MyRNOStepItems[_MyRNOStepItems.Count - 1].BottomMostStepItem;
|
|
}
|
|
StepItem tmpa = this; // BottomMost After
|
|
if ((MyExpandingStatus != ExpandingStatus.No || Expanded) & _MyAfterStepItems != null)
|
|
tmpa = _MyAfterStepItems[_MyAfterStepItems.Count - 1].BottomMostStepItem;
|
|
// return the bottom most of the two results
|
|
if (tmpr == null)
|
|
return tmpa;
|
|
//if (rnoOffset > 0)
|
|
//Console.WriteLine("RNO Bottom Offset {0}", rnoOffset);
|
|
//if (tmpa.Bottom >= (tmpr.Bottom + rnoOffset))
|
|
if (tmpa.Bottom >= (tmpr.Bottom))
|
|
return tmpa;
|
|
return tmpr;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Bottom most child excluding RNOs
|
|
/// </summary>
|
|
public StepItem BottomMostStepItemNoRNOs
|
|
{
|
|
get
|
|
{
|
|
StepItem tmpa = this; // BottomMost After
|
|
if ((MyExpandingStatus != ExpandingStatus.No || Expanded) & _MyAfterStepItems != null)
|
|
tmpa = _MyAfterStepItems[_MyAfterStepItems.Count - 1].BottomMostStepItem;
|
|
// return the bottom most
|
|
return tmpa;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// First sibling
|
|
/// </summary>
|
|
private StepItem FirstSiblingStepItem
|
|
{
|
|
get
|
|
{
|
|
StepItem tmp = this;
|
|
while (tmp.MyPreviousStepItem != null)
|
|
tmp = tmp.MyPreviousStepItem;
|
|
return tmp;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Last sibling
|
|
/// </summary>
|
|
private StepItem LastSiblingStepItem
|
|
{
|
|
get
|
|
{
|
|
StepItem tmp = this;
|
|
while (tmp.MyNextStepItem != null)
|
|
tmp = tmp.MyNextStepItem;
|
|
return tmp;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Returns the status of the vlnExpander unless it is in the process of expanding or collapsing
|
|
/// If it is colapsing it returns false
|
|
/// If it is expanding it returns true
|
|
/// </summary>
|
|
public bool Expanded
|
|
{
|
|
get
|
|
{
|
|
return !_Colapsing && (MyExpandingStatus != ExpandingStatus.No || _MyvlnExpander.Expanded);
|
|
}
|
|
set
|
|
{
|
|
_MyvlnExpander.Expanded = value;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Sets or Gets expanding status
|
|
/// </summary>
|
|
public ExpandingStatus MyExpandingStatus
|
|
{
|
|
get { return _MyExpandingStatus; }
|
|
set { _MyExpandingStatus = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or sets colapsing
|
|
/// </summary>
|
|
public bool Colapsing
|
|
{
|
|
get { return _Colapsing; }
|
|
set { _Colapsing = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets or Sets the ability to expand
|
|
/// </summary>
|
|
public bool CanExpand
|
|
{
|
|
get { return _MyvlnExpander.Visible; }
|
|
set { _MyvlnExpander.Visible = value; }
|
|
}
|
|
/// <summary>
|
|
/// Gets the text from the content
|
|
/// </summary>
|
|
public string MyText
|
|
{
|
|
get { return _MyItemInfo == null ? null : _MyItemInfo.MyContent.Text; }
|
|
}
|
|
/// <summary>
|
|
/// Gets the ItemID from the ItemInfo
|
|
/// </summary>
|
|
public int MyID
|
|
{
|
|
get { return _MyItemInfo == null ? 0 : _MyItemInfo.ItemID; }
|
|
}
|
|
/// <summary>
|
|
/// Tracks when a StepItem is moving
|
|
/// </summary>
|
|
public bool Moving
|
|
{
|
|
get { return _Moving; }
|
|
set { _Moving = value; }
|
|
}
|
|
/// <summary>
|
|
/// The RNO (Contingency) Level
|
|
/// </summary>
|
|
public int RNOLevel
|
|
{
|
|
get { return _RNOLevel; }
|
|
set { _RNOLevel = value; }
|
|
}
|
|
/// <summary>
|
|
/// Sequential Level - Only counts levels of Sequential substeps
|
|
/// </summary>
|
|
public int SeqLevel
|
|
{
|
|
get { return _SeqLevel; }
|
|
set { _SeqLevel = value; }
|
|
}
|
|
// TODO: This should be changed to get the Circle format from the data
|
|
/// <summary>
|
|
/// Show a circle or not
|
|
/// </summary>
|
|
public bool Circle
|
|
{
|
|
get { return _Circle; }
|
|
set { _Circle = value; }
|
|
}
|
|
// TODO: This should be changed to get the Checkoff status from the data
|
|
/// <summary>
|
|
/// Has a check-off or not
|
|
/// </summary>
|
|
public bool CheckOff
|
|
{
|
|
get { return _CheckOff; }
|
|
set { _CheckOff = value; }
|
|
}
|
|
// TODO: This should be changed to get the ChangeBar status from the data
|
|
/// <summary>
|
|
/// Has a changebar or not
|
|
/// </summary>
|
|
public bool ChangeBar
|
|
{
|
|
get { return _ChangeBar; }
|
|
set
|
|
{
|
|
_ChangeBar = value;
|
|
this.Invalidate();
|
|
}
|
|
}
|
|
#endregion // Properties
|
|
#region Constructors
|
|
public StepItem(ItemInfo itemInfo, StepPanel myStepPanel, StepItem myParentStepItem, ChildRelation myChildRelation, bool expand)
|
|
{
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB Top");
|
|
InitializeComponent();// TODO: Performance 25%
|
|
SetupStepItem(itemInfo, myStepPanel, myParentStepItem, myChildRelation, expand, null);
|
|
}
|
|
public StepItem(ItemInfo itemInfo, StepPanel myStepPanel, StepItem myParentStepItem, ChildRelation myChildRelation, bool expand, StepItem nextStepItem)
|
|
{
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB Top");
|
|
InitializeComponent();// TODO: Performance 25%
|
|
SetupStepItem(itemInfo, myStepPanel, myParentStepItem, myChildRelation, expand, nextStepItem);
|
|
}
|
|
private void SetupStepItem(ItemInfo itemInfo, StepPanel myStepPanel, StepItem myParentStepItem, ChildRelation myChildRelation, bool expand, StepItem nextStepItem)
|
|
{
|
|
//if (itemInfo.ItemID == 225) _MyStepRTB.Resize += new EventHandler(_MyStepRTB_Resize);
|
|
_MyStepRTB.MyStepItem = this;
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB InitComp");
|
|
BackColor = myStepPanel.PanelColor;
|
|
//_MyStepRTB.BackColor = myStepPanel.InactiveColor;
|
|
// TODO: Adjust top based upon format
|
|
// TODO: Remove Label and just output ident on the paint event
|
|
lblTab.Left = 20;
|
|
SetupHeader(itemInfo);
|
|
this.Paint += new PaintEventHandler(StepItem_Paint);
|
|
this.BackColorChanged += new EventHandler(StepItem_BackColorChanged);
|
|
if (itemInfo != null)
|
|
{
|
|
_Type = (int)itemInfo.MyContent.Type;
|
|
switch (_Type / 10000)
|
|
{
|
|
case 0: // Procedure
|
|
_MyStepRTB.Font = myStepPanel.ProcFont;// lblTab.Font = myStepPanel.ProcFont;
|
|
lblTab.Font = itemInfo.MyTab.MyFont.WindowsFont;
|
|
break;
|
|
case 1: // Section
|
|
_MyStepRTB.Font = myStepPanel.SectFont;// lblTab.Font = myStepPanel.SectFont;
|
|
lblTab.Font = itemInfo.MyTab.MyFont.WindowsFont;
|
|
break;
|
|
case 2: // Steps
|
|
_MyStepRTB.Font = myStepPanel.StepFont;//lblTab.Font = myStepPanel.StepFont;
|
|
lblTab.Font = itemInfo.MyTab.MyFont.WindowsFont;
|
|
_MyStepData = itemInfo.ActiveFormat.PlantFormat.FormatData.StepDataList[_Type % 10000];
|
|
break;
|
|
}
|
|
//this.Move += new EventHandler(DisplayItem_Move);
|
|
}
|
|
else
|
|
{
|
|
if (myStepPanel.MyFont != null) _MyStepRTB.Font = lblTab.Font = myStepPanel.MyFont;
|
|
}
|
|
if (expand) _MyvlnExpander.ShowExpanded();
|
|
_MyStepPanel = myStepPanel;
|
|
if (itemInfo != null) myStepPanel._LookupStepItems.Add(itemInfo.ItemID, this);
|
|
_MyChildRelation = myChildRelation;
|
|
if (myParentStepItem != null) RNOLevel = myParentStepItem.RNOLevel;
|
|
if (itemInfo != null)
|
|
{
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB before _Layout");
|
|
_MyStepSectionLayoutData = itemInfo.ActiveFormat.MyStepSectionLayoutData;
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB _Layout");
|
|
if (myParentStepItem != null)
|
|
SeqLevel = myParentStepItem.SeqLevel + ((myChildRelation == ChildRelation.After || myChildRelation == ChildRelation.Before) && itemInfo.IsSequential ? 1 : 0);
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB seqLevel");
|
|
MyItemInfo = itemInfo;
|
|
}
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB MyItem");
|
|
myStepPanel.Controls.Add(this);
|
|
|
|
switch (myChildRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
AddItem(myParentStepItem, ref myParentStepItem._MyAfterStepItems,nextStepItem);
|
|
break;
|
|
case ChildRelation.Before:
|
|
AddItem(myParentStepItem, ref myParentStepItem._MyBeforeStepItems, nextStepItem);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
RNOLevel = myParentStepItem.RNOLevel + 1;
|
|
AddItem(myParentStepItem, ref myParentStepItem._MyRNOStepItems, nextStepItem);
|
|
break;
|
|
case ChildRelation.None:
|
|
break;
|
|
}
|
|
if (itemInfo != null)
|
|
{
|
|
if (myChildRelation == ChildRelation.None)
|
|
{
|
|
if (_Type == 0 && _MyStepSectionLayoutData != null)
|
|
{
|
|
LastMethodsPush(string.Format("SetupStepItem {0}", MyID));
|
|
Width = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidT);
|
|
}
|
|
}
|
|
}
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB Parent");
|
|
SetText();
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB SetText");
|
|
if (itemInfo != null)
|
|
{
|
|
Name = string.Format("Item-{0}", itemInfo.ItemID);
|
|
SetExpandAndExpander(itemInfo);
|
|
if (expand && (itemInfo.MyContent.ContentPartCount != 0)) // If it should expand and it can expand
|
|
Expand(true);
|
|
else
|
|
if (myParentStepItem == null)// If it is the top node
|
|
if (_Type >= 20000) // and it is a step - fully expand
|
|
Expand(true);
|
|
else // otherwise only expand one level
|
|
Expand(false);
|
|
}
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB before Controls Add");
|
|
//myStepPanel.Controls.Add(this);
|
|
int top = FindTop(0);
|
|
if (Top < top)
|
|
{
|
|
LastMethodsPush("SetupStepItem");
|
|
_MyStepPanel.ItemMoving++;
|
|
Top = top;
|
|
_MyStepPanel.ItemMoving--;
|
|
LastMethodsPop();
|
|
}
|
|
_Loading = false;
|
|
//// TIMING: DisplayItem.TimeIt("CSLARTB Controls Add");
|
|
}
|
|
private void SetExpandAndExpander(ItemInfo itemInfo)
|
|
{
|
|
// Don't allow substeps to expand
|
|
switch (_Type / 10000)
|
|
{
|
|
case 1: // Section can expand
|
|
CanExpand = true;
|
|
// If a word document set the expander to attachment
|
|
_MyvlnExpander.Attachment = !(itemInfo.IsStepSection);
|
|
//OLD: _MyvlnExpander.Attachment = (itemInfo.MyContent.ContentPartCount == 0);
|
|
break;
|
|
case 2: // High level steps with children can expand
|
|
CanExpand = itemInfo.IsHigh && itemInfo.HasChildren; // TemporaryFormat.IsHigh(item); ;
|
|
break;
|
|
default://Procedures cannot expand, because they automatically expand
|
|
CanExpand = false;
|
|
break;
|
|
}
|
|
}
|
|
private void SetupHeader(ItemInfo itemInfo)
|
|
{
|
|
lblTab.Top = 3 + ((itemInfo.HasHeader) ? 23 : 0);
|
|
_MyStepRTB.Top = lblTab.Top; // 3 + ((itemInfo.HasHeader) ? 23 : 0);
|
|
//lblTab.Move += new EventHandler(lblTab_Move);
|
|
if (itemInfo.HasHeader)
|
|
SetupHeaderFooter(ref lblHeader, "Header", itemInfo.MyHeader);
|
|
else
|
|
{
|
|
// remove header from screen if it exists:
|
|
this.Controls.Remove(lblHeader);
|
|
lblHeader = null;
|
|
}
|
|
}
|
|
private void SetupHeader()
|
|
{
|
|
SetupHeader(MyItemInfo);
|
|
LastMethodsPush("SetupHeader");
|
|
Height = _MyStepRTB.Height + _MyStepRTB.Top + 7;
|
|
LastMethodsPop();
|
|
}
|
|
void _MyStepRTB_Resize(object sender, EventArgs e)
|
|
{
|
|
// Console.WriteLine("Left {0} Width {1}", Left, Width);
|
|
}
|
|
|
|
private Label lblHeader = null;
|
|
private Label lblFooter = null;
|
|
private void SetupHeaderFooter(ref Label lbl, string name, MetaTag mTag)
|
|
{
|
|
if (lbl==null)lbl = new Label();
|
|
lbl.BackColor = System.Drawing.Color.Transparent;
|
|
lbl.Location = new System.Drawing.Point(0, 0);
|
|
lbl.Name = name;
|
|
lbl.Size = new System.Drawing.Size(this.Width, 23);
|
|
lbl.Visible = true;
|
|
lbl.Font = mTag.MyFont.WindowsFont;
|
|
lbl.Text = mTag.CleanText;
|
|
lbl.TextAlign = mTag.Justify;
|
|
this.Controls.Add(lbl);
|
|
}
|
|
#endregion
|
|
#region AddItem
|
|
/// <summary>
|
|
/// Adds an item to a list
|
|
/// </summary>
|
|
/// <param name="parentStepItem">Parent Container</param>
|
|
/// <param name="siblingStepItems">StepItem List</param>
|
|
public void AddItem(StepItem parentStepItem, ref List<StepItem> siblingStepItems, StepItem nextStepItem)
|
|
{
|
|
if (siblingStepItems == null) // Create a list of siblings
|
|
{
|
|
siblingStepItems = new List<StepItem>();
|
|
siblingStepItems.Add(this);
|
|
MyParentStepItem = parentStepItem;
|
|
}
|
|
else // Add to the existing list
|
|
{
|
|
if (nextStepItem == null) // Add to the end of the list
|
|
{
|
|
StepItem lastChild = LastChild(siblingStepItems);
|
|
siblingStepItems.Add(this);
|
|
MyPreviousStepItem = lastChild;
|
|
}
|
|
else // Add to the middle of the list before a particular item
|
|
{
|
|
StepItem prevChild = nextStepItem.MyPreviousStepItem;
|
|
StepItem parent = nextStepItem.MyParentStepItem;
|
|
siblingStepItems.Insert(siblingStepItems.IndexOf(nextStepItem), this);
|
|
MyStepPanel.ItemMoving++;
|
|
_MyNextStepItem = nextStepItem;
|
|
nextStepItem._MyPreviousStepItem = this;
|
|
MyPreviousStepItem = prevChild;// If a previous exists - this will adjust the location and width of the StepItem
|
|
nextStepItem.MyParentStepItem = null;
|
|
MyParentStepItem = parent; // If a parent exists - this will adjust the location and width of the StepItem
|
|
//nextStepItem.MyPreviousStepItem = this;
|
|
MyStepPanel.ItemMoving--;
|
|
}
|
|
}
|
|
if (MyItemInfo.IsCaution || MyItemInfo.IsNote)
|
|
{
|
|
StepItem prev = this;
|
|
while (prev.MyPreviousStepItem != null) prev = prev.MyPreviousStepItem;
|
|
prev.SetAllTabs();
|
|
}
|
|
else
|
|
SetAllTabs();
|
|
}
|
|
// clear tabs, clears then all so that next 'get' will calculate new.
|
|
public void SetAllTabs()
|
|
{
|
|
TabFormat = null; // reset TabFormat
|
|
SetupHeader();
|
|
if (_MyAfterStepItems != null) foreach (StepItem chld in _MyAfterStepItems) chld.SetAllTabs();
|
|
if (_MyNextStepItem != null) _MyNextStepItem.SetAllTabs();
|
|
// Update the RNO tab if it exists - RHM 20100106
|
|
if (_MyRNOStepItems != null) foreach (StepItem chld in _MyRNOStepItems) chld.SetAllTabs();
|
|
}
|
|
/// <summary>
|
|
/// Add the next item to a list
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <param name="expand"></param>
|
|
/// <returns></returns>
|
|
//public StepItem AddNext(ItemInfo myItemInfo, bool expand)
|
|
//{
|
|
// StepItem tmp = new StepItem(myItemInfo, _MyStepPanel, MyParentStepItem, ChildRelation.None, expand);
|
|
// MyNextStepItem = tmp;
|
|
// return tmp;
|
|
//}
|
|
#endregion
|
|
#region RemoveItem
|
|
protected void ShowTops(string title)
|
|
{
|
|
int TopMostY = TopMostStepItem.Top;
|
|
int? TopMostParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.TopMostStepItem.Top));
|
|
int? ParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.Top));
|
|
//Console.Write("{0}: TopMostY={1}, TopMostParentY={2}, ParentY = {3}",title, TopMostY, TopMostParentY, ParentY);
|
|
Console.Write("{0}{1},{2},{3}", title, TopMostY, TopMostParentY.ToString() ?? "null", ParentY.ToString() ?? "null");
|
|
}
|
|
private bool _BeingRemoved = false;
|
|
public bool BeingRemoved
|
|
{
|
|
get { return _BeingRemoved; }
|
|
set { _BeingRemoved = value; }
|
|
}
|
|
public void RemoveItem()
|
|
{
|
|
BeingRemoved = true;
|
|
MyStepPanel.SelectedStepRTB = null; // Unselect the item to be deleted
|
|
//ShowTops("\r\n");
|
|
int TopMostYBefore = TopMostStepItem.Top;
|
|
StepItem newFocus = DeleteItem();
|
|
if (newFocus == null) return;
|
|
newFocus.MyStepRTB.Focus();
|
|
Dispose();
|
|
newFocus.SetAllTabs();
|
|
int TopMostYAfter = newFocus.TopMostStepItem.Top;
|
|
if (TopMostYAfter > TopMostYBefore)
|
|
newFocus.TopMostStepItem.Top = TopMostYBefore;
|
|
newFocus.AdjustLocation();
|
|
//newFocus.ShowTops("");
|
|
}
|
|
public StepItem DeleteItem()
|
|
{
|
|
StepItem newFocus = null;
|
|
int? TopMostParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.TopMostStepItem.Top));
|
|
int? ParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.Top));
|
|
try
|
|
{
|
|
Item.DeleteItemAndChildren(MyItemInfo);
|
|
}
|
|
catch (System.Data.SqlClient.SqlException ex)
|
|
{
|
|
HandleSqlExceptionOnDelete(ex);
|
|
return null;
|
|
}
|
|
// Remove StepItems
|
|
RemoveFromParentsChildList();
|
|
if (MyNextStepItem != null)
|
|
{
|
|
if (MyPreviousStepItem != null)
|
|
{
|
|
MyNextStepItem.MyPreviousStepItem = MyPreviousStepItem;
|
|
MyPreviousStepItem = null;
|
|
newFocus = MyNextStepItem;
|
|
}
|
|
else
|
|
{
|
|
MyNextStepItem.MyParentStepItem = MyParentStepItem;
|
|
MyParentStepItem = null;
|
|
MyNextStepItem.MyPreviousStepItem = null;
|
|
newFocus = MyNextStepItem;
|
|
}
|
|
// Adjust the vertical locations of all of the items below the item deleted
|
|
MyNextStepItem.TopMostStepItem.AdjustLocation();
|
|
MyNextStepItem = null;
|
|
}
|
|
else if (MyPreviousStepItem != null)
|
|
{
|
|
MyPreviousStepItem.MyNextStepItem = null;
|
|
newFocus = MyPreviousStepItem;
|
|
MyPreviousStepItem = null;
|
|
//Console.Write(",\"Previous\",");
|
|
}
|
|
else
|
|
{
|
|
newFocus = MyParentStepItem;
|
|
MyParentStepItem = null;
|
|
//Console.Write(",\"Parent\",");
|
|
}
|
|
return newFocus;
|
|
}
|
|
|
|
private void HandleSqlExceptionOnDelete(System.Data.SqlClient.SqlException ex)
|
|
{
|
|
if (ex.Message.Contains("has External Transitions and has no next step"))
|
|
{
|
|
using (TransitionInfoList exTrans = TransitionInfoList.GetExternalTransitions(MyID))
|
|
{
|
|
DialogResult ans = MessageBox.Show("Transitions exist to this step and cannot be adjusted automatically." +
|
|
"\r\n\r\nDo you want to be placed on the " + (exTrans.Count > 1 ? "first " : "") + "substep with the problem Transition?" +
|
|
"\r\n\r\nSubsteps with Problem Transitions" +
|
|
exTrans.Summarize(),
|
|
"Cannot Delete This Step", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
|
|
if (ans == DialogResult.Yes)
|
|
{
|
|
MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OpenItem(exTrans[0].MyContent.ContentItems[0]);
|
|
|
|
}
|
|
else
|
|
this.MyStepRTB.Focus();
|
|
}
|
|
}
|
|
else if (ex.Message.Contains("has External Transitions to it's children"))
|
|
{
|
|
using (TransitionInfoList exTrans = TransitionInfoList.GetExternalTransitionsToChildren(MyID))
|
|
{
|
|
DialogResult ans = MessageBox.Show("Transitions exist to substeps of this step and cannot be adjusted automatically." +
|
|
"\r\n\r\nDo you want to be placed on the " + (exTrans.Count > 1 ? "first " : "") + "substep with the problem Transition?" +
|
|
"\r\n\r\nSubsteps with Problem Transitions:" +
|
|
exTrans.Summarize(),
|
|
"Cannot Delete This Step", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
|
|
if (ans == DialogResult.Yes)
|
|
{
|
|
MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OpenItem(exTrans[0].MyContent.ContentItems[0]);
|
|
}
|
|
}
|
|
}
|
|
else
|
|
MessageBox.Show(ex.Message, "SQL Exception", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
}
|
|
private void RemoveFromParentsChildList()
|
|
{
|
|
StepItem top = this;
|
|
while (top.MyPreviousStepItem != null) top = top.MyPreviousStepItem;
|
|
StepItem parentStepItem = top.MyParentStepItem;
|
|
if (parentStepItem == null) return; // No parent, nothing to remove.
|
|
if (parentStepItem.MyAfterStepItems != null && parentStepItem.MyAfterStepItems.Contains(this))
|
|
{
|
|
parentStepItem.MyAfterStepItems.Remove(this);
|
|
if (parentStepItem.MyAfterStepItems.Count == 0)
|
|
parentStepItem.MyAfterStepItems = null;
|
|
}
|
|
else if (parentStepItem.MyBeforeStepItems != null && parentStepItem.MyBeforeStepItems.Contains(this))
|
|
{
|
|
parentStepItem.MyBeforeStepItems.Remove(this);
|
|
if(parentStepItem.MyBeforeStepItems.Count == 0)
|
|
parentStepItem.MyBeforeStepItems = null;
|
|
}
|
|
else if (parentStepItem.MyRNOStepItems != null && parentStepItem.MyRNOStepItems.Contains(this))
|
|
{
|
|
parentStepItem.MyRNOStepItems.Remove(this);
|
|
if(parentStepItem.MyRNOStepItems.Count == 0)
|
|
parentStepItem.MyRNOStepItems = null;
|
|
}
|
|
if (parentStepItem.MyAfterStepItems == null && parentStepItem.MyBeforeStepItems == null && parentStepItem.MyRNOStepItems == null)
|
|
parentStepItem.CanExpand = false;
|
|
}
|
|
//private void ShowSiblings(string title)
|
|
//{
|
|
// Console.WriteLine("---{0} {1}---",title,MyID);
|
|
// StepItem top = this;
|
|
// while (top.MyPreviousStepItem != null) top = top.MyPreviousStepItem;
|
|
// do
|
|
// {
|
|
// Console.WriteLine("{0} StepItem - {1} {2}", top.MyID == MyID ? "*" : " ", top.MyID, top.MyItemInfo.MyContent.Text);
|
|
// top = top.MyNextStepItem;
|
|
// } while (top != null);
|
|
//}
|
|
#endregion
|
|
#region Add Children
|
|
/// <summary>
|
|
/// Add a child before (Notes, Cautions, etc.)
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <param name="expand"></param>
|
|
public void AddChildBefore(ItemInfo myItemInfo, bool expand)
|
|
{
|
|
StepItem child = new StepItem(myItemInfo, _MyStepPanel, this, ChildRelation.Before, expand);
|
|
}
|
|
/// <summary>
|
|
/// Add a list of children before
|
|
/// </summary>
|
|
/// <param name="myItemInfoList"></param>
|
|
/// <param name="expand"></param>
|
|
public void AddChildBefore(ItemInfoList myItemInfoList, bool expand)
|
|
{
|
|
if (myItemInfoList != null)
|
|
foreach (ItemInfo item in myItemInfoList)
|
|
AddChildBefore(item, expand);
|
|
}
|
|
/// <summary>
|
|
/// Add an RNO (Contingency) child
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <param name="expand"></param>
|
|
public void AddChildRNO(ItemInfo myItemInfo, bool expand)
|
|
{
|
|
StepItem child = new StepItem(myItemInfo, _MyStepPanel, this, ChildRelation.RNO, expand);
|
|
}
|
|
/// <summary>
|
|
/// Add a list of RNO (Contingency) children
|
|
/// </summary>
|
|
/// <param name="myItemInfoList"></param>
|
|
/// <param name="expand"></param>
|
|
public void AddChildRNO(ItemInfoList myItemInfoList, bool expand)
|
|
{
|
|
if (myItemInfoList != null)
|
|
foreach (ItemInfo item in myItemInfoList)
|
|
AddChildRNO(item, expand);
|
|
}
|
|
/// <summary>
|
|
/// Add a child after
|
|
/// </summary>
|
|
/// <param name="MyItemInfo"></param>
|
|
/// <param name="expand"></param>
|
|
public StepItem AddChildAfter(ItemInfo MyItemInfo, bool expand)
|
|
{
|
|
StepItem child = new StepItem(MyItemInfo, _MyStepPanel, this, ChildRelation.After, expand);
|
|
return child;
|
|
}
|
|
public StepItem AddChildAfter(ItemInfo MyItemInfo, StepItem nextStepItem)
|
|
{
|
|
StepItem child = new StepItem(MyItemInfo, _MyStepPanel, this, ChildRelation.After, true, nextStepItem);
|
|
return child;
|
|
}
|
|
public StepItem AddChildBefore(ItemInfo MyItemInfo, StepItem nextStepItem)
|
|
{
|
|
StepItem child = new StepItem(MyItemInfo, _MyStepPanel, this, ChildRelation.Before, true, nextStepItem);
|
|
return child;
|
|
}
|
|
public StepItem AddChildRNO(ItemInfo MyItemInfo, StepItem nextStepItem)
|
|
{
|
|
StepItem child = new StepItem(MyItemInfo, _MyStepPanel, this, ChildRelation.RNO, true, nextStepItem);
|
|
return child;
|
|
}
|
|
/// <summary>
|
|
/// Adds a sibling after the current StepItem
|
|
/// </summary>
|
|
public void AddSiblingAfter()
|
|
{
|
|
AddSiblingAfter("");
|
|
}
|
|
public void AddSiblingAfter(string text)
|
|
{
|
|
MyStepRTB.SaveText();
|
|
ItemInfo newItemInfo = MyItemInfo.InsertSiblingAfter(text);
|
|
DoAddSiblingAfter(newItemInfo);
|
|
}
|
|
public void AddSiblingAfter(int? type)
|
|
{
|
|
MyStepRTB.SaveText();
|
|
ItemInfo newItemInfo = MyItemInfo.InsertSiblingAfter("","",type);
|
|
DoAddSiblingAfter(newItemInfo);
|
|
}
|
|
private void DoAddSiblingAfter(ItemInfo newItemInfo)
|
|
{
|
|
StepItem newStepItem = null;
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
newStepItem = ActiveParent.AddChildAfter(newItemInfo, MyNextStepItem);
|
|
break;
|
|
case ChildRelation.Before:
|
|
newStepItem = ActiveParent.AddChildBefore(newItemInfo, MyNextStepItem);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
newStepItem = ActiveParent.AddChildRNO(newItemInfo, MyNextStepItem);
|
|
break;
|
|
default: // Need debug
|
|
break;
|
|
}
|
|
//StepItem newStepItem = ActiveParent.AddChildAfter(newItemInfo, );
|
|
_MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
}
|
|
private static int _WatchThis = 1;
|
|
public void AddSiblingBefore()
|
|
{
|
|
AddSiblingBefore("");
|
|
}
|
|
public void AddSiblingBefore(string text)
|
|
{
|
|
// Save RTB text before creating a new item because the process of creating
|
|
// a new item will save a change to iteminfo excluding text changes. This
|
|
// shouldn't be necessary for adding sibling after because the current step
|
|
// doesn't get saved during the insert after because of the MyPrevious field
|
|
// is only set on an insert before, which is what saves the item without
|
|
// any updates from the richtextbox text.
|
|
_MyStepRTB.SaveText();
|
|
ItemInfo newItemInfo = MyItemInfo.InsertSiblingBefore(text);
|
|
StepItem newStepItem=null;
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
newStepItem = ActiveParent.AddChildAfter(newItemInfo, this);
|
|
break;
|
|
case ChildRelation.Before:
|
|
newStepItem = ActiveParent.AddChildBefore(newItemInfo, this);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
newStepItem = ActiveParent.AddChildRNO(newItemInfo, this);
|
|
break;
|
|
default: // Need debug
|
|
break;
|
|
}
|
|
_MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
}
|
|
public void AddChild(E_FromType fromType, int type)
|
|
{
|
|
AddChild("",fromType, type);
|
|
}
|
|
public void AddChild(string text, E_FromType fromType, int type)
|
|
{
|
|
if (_MyItemInfo.IsHigh || _MyItemInfo.IsSection)
|
|
this.CanExpand = true;
|
|
this.Expanded = true;
|
|
_WatchThis = 1;
|
|
ItemInfo newItemInfo = MyItemInfo.InsertChild(fromType, type, text);
|
|
// TODO: We need to determine where this will go in the stack of children
|
|
StepItem nextItem = null;
|
|
if (newItemInfo.NextItem != null)
|
|
nextItem = MyStepPanel.FindItem(newItemInfo.NextItem);
|
|
else if (fromType == E_FromType.Table && MyAfterStepItems != null)
|
|
nextItem = MyAfterStepItems[0];
|
|
// Cautions come before notes, so if this is a Caution and there are Notes, put this first
|
|
else if (fromType == E_FromType.Caution && ((ItemInfo)newItemInfo.ActiveParent).Notes != null
|
|
&& ((ItemInfo)newItemInfo.ActiveParent).Notes.Count > 0)
|
|
nextItem = MyStepPanel.FindItem(((ItemInfo)newItemInfo.ActiveParent).Notes[0]);
|
|
// TODO: May need similar logic if a Table is being added to a step that has substeps
|
|
// else if (fromType == E_FromType.Table && ((ItemInfo)newItemInfo.ActiveParent).Steps != null
|
|
//&& ((ItemInfo)newItemInfo.ActiveParent).Steps.Count > 0)
|
|
// nextItem = MyStepPanel.FindItem(((ItemInfo)newItemInfo.ActiveParent).Steps[0]);
|
|
StepItem newStepItem;
|
|
switch (fromType)
|
|
{
|
|
case E_FromType.Caution:
|
|
newStepItem = this.AddChildBefore(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.Note:
|
|
newStepItem = this.AddChildBefore(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.Procedure:
|
|
newStepItem = this.AddChildAfter(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.RNO:
|
|
newStepItem = this.AddChildRNO(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.Section:
|
|
newStepItem = this.AddChildAfter(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.Step:
|
|
newStepItem = this.AddChildAfter(newItemInfo, nextItem);
|
|
break;
|
|
case E_FromType.Table:
|
|
newStepItem = this.AddChildAfter(newItemInfo, nextItem);
|
|
break;
|
|
default:
|
|
newStepItem = this.AddChildAfter(newItemInfo, nextItem);
|
|
break;
|
|
}
|
|
_MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
}
|
|
/// <summary>
|
|
/// Add a list of children after
|
|
/// </summary>
|
|
/// <param name="myItemInfoList"></param>
|
|
/// <param name="expand"></param>
|
|
public StepItem AddChildAfter(ItemInfoList myItemInfoList, bool expand)
|
|
{
|
|
StepItem child = null;
|
|
if (myItemInfoList != null)
|
|
foreach (ItemInfo item in myItemInfoList)
|
|
child = AddChildAfter(item, expand);
|
|
return child;
|
|
}
|
|
#endregion
|
|
#region CopyPaste
|
|
public void PasteSiblingBefore(int copyStartID)
|
|
{
|
|
ItemInfo newItemInfo = MyItemInfo.PasteSiblingBefore(copyStartID);
|
|
StepItem newStepItem = null;
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
newStepItem = ActiveParent.AddChildAfter(newItemInfo, this);
|
|
break;
|
|
case ChildRelation.Before:
|
|
newStepItem = ActiveParent.AddChildBefore(newItemInfo, this);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
newStepItem = ActiveParent.AddChildRNO(newItemInfo, this);
|
|
break;
|
|
default: // Need debug
|
|
break;
|
|
}
|
|
if (newStepItem != null) _MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
_MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OnItemPaste(this, new vlnTreeItemInfoPasteEventArgs(newItemInfo, copyStartID, ItemInfo.EAddpingPart.Before, newItemInfo.MyContent.Type));
|
|
|
|
}
|
|
public void PasteSiblingAfter(int copyStartID)
|
|
{
|
|
ItemInfo newItemInfo = MyItemInfo.PasteSiblingAfter(copyStartID);
|
|
StepItem newStepItem = null;
|
|
switch (_MyChildRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
newStepItem = ActiveParent.AddChildAfter(newItemInfo, MyNextStepItem);
|
|
break;
|
|
case ChildRelation.Before:
|
|
newStepItem = ActiveParent.AddChildBefore(newItemInfo, MyNextStepItem);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
newStepItem = ActiveParent.AddChildRNO(newItemInfo, MyNextStepItem);
|
|
break;
|
|
default: // Need debug
|
|
break;
|
|
}
|
|
_MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
_MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OnItemPaste(this, new vlnTreeItemInfoPasteEventArgs(newItemInfo, copyStartID, ItemInfo.EAddpingPart.After, newItemInfo.MyContent.Type));
|
|
|
|
}
|
|
public StepItem PasteReplace(int copyStartID)
|
|
{
|
|
// To allow a Paste Step into an empty (new) step/substep, we need to add a character to the the Text field
|
|
// to simulate replacing an existing step - otherwise we will get null references.
|
|
if (MyStepPanel.SelectedStepRTB.Text == "")
|
|
MyStepPanel.SelectedStepRTB.Text = " ";
|
|
MyStepPanel.SelectedStepRTB = null; // Unselect the item to be deleted
|
|
ChildRelation childRelation = _MyChildRelation;
|
|
StepItem newFocus = null;
|
|
StepItem nextStepItem = MyNextStepItem;
|
|
StepItem prevStepItem = MyPreviousStepItem;
|
|
StepItem parentStepItem = ActiveParent;
|
|
|
|
int TopMostYBefore = TopMostStepItem.Top;
|
|
int? TopMostParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.TopMostStepItem.Top));
|
|
int? ParentY = (MyParentStepItem == null ? null : (int?)(MyParentStepItem.Top));
|
|
ItemInfo newItemInfo = null;
|
|
try
|
|
{
|
|
newItemInfo = Item.PasteReplace(MyItemInfo, copyStartID);
|
|
}
|
|
catch (System.Data.SqlClient.SqlException ex)
|
|
{
|
|
HandleSqlExceptionOnDelete(ex);
|
|
return this;
|
|
}
|
|
// Remove the StepItem that was the replaced item.
|
|
RemoveFromParentsChildList();
|
|
|
|
if (MyNextStepItem != null)
|
|
{
|
|
if (MyPreviousStepItem != null)
|
|
{
|
|
MyNextStepItem.MyPreviousStepItem = MyPreviousStepItem;
|
|
MyPreviousStepItem = null;
|
|
}
|
|
else
|
|
{
|
|
MyNextStepItem.MyParentStepItem = MyParentStepItem;
|
|
MyParentStepItem = null;
|
|
MyNextStepItem.MyPreviousStepItem = null;
|
|
}
|
|
MyNextStepItem = null;
|
|
}
|
|
else if (MyPreviousStepItem != null)
|
|
{
|
|
MyPreviousStepItem.MyNextStepItem = null;
|
|
newFocus = MyPreviousStepItem;
|
|
MyPreviousStepItem = null;
|
|
}
|
|
else
|
|
{
|
|
newFocus = MyParentStepItem;
|
|
MyParentStepItem = null;
|
|
}
|
|
|
|
// add copied item to ui where the replaced item was.
|
|
StepItem newStepItem = null;
|
|
switch (childRelation)
|
|
{
|
|
case ChildRelation.After:
|
|
newStepItem = parentStepItem.AddChildAfter(newItemInfo, nextStepItem);
|
|
break;
|
|
case ChildRelation.Before:
|
|
newStepItem = parentStepItem.AddChildBefore(newItemInfo, nextStepItem);
|
|
break;
|
|
case ChildRelation.RNO:
|
|
newStepItem = parentStepItem.AddChildRNO(newItemInfo, nextStepItem);
|
|
break;
|
|
default: // Need debug
|
|
break;
|
|
}
|
|
_MyStepPanel.SelectedStepRTB = newStepItem.MyStepRTB;//Update Screen
|
|
_MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OnItemPaste(this, new vlnTreeItemInfoPasteEventArgs(newItemInfo, copyStartID, ItemInfo.EAddpingPart.Replace, newItemInfo.MyContent.Type));
|
|
return newStepItem;
|
|
}
|
|
public void HighlightBackColor()
|
|
{
|
|
// Highlight all of the rtb's within this stepitem:
|
|
if (MyAfterStepItems != null)
|
|
{
|
|
foreach (StepItem sia in MyAfterStepItems)
|
|
{
|
|
sia.MyStepRTB.HighlightBackColor();
|
|
sia.HighlightBackColor();
|
|
}
|
|
}
|
|
if (MyBeforeStepItems != null)
|
|
{
|
|
foreach (StepItem sib in MyBeforeStepItems)
|
|
{
|
|
sib.MyStepRTB.HighlightBackColor();
|
|
sib.HighlightBackColor();
|
|
}
|
|
}
|
|
if (MyRNOStepItems != null)
|
|
{
|
|
foreach (StepItem sir in MyRNOStepItems)
|
|
{
|
|
sir.MyStepRTB.HighlightBackColor();
|
|
sir.HighlightBackColor();
|
|
}
|
|
}
|
|
}
|
|
public void SetBackColor()
|
|
{
|
|
// Set (removing highlighting) all of the rtb's within this stepitem:
|
|
if (MyAfterStepItems != null)
|
|
{
|
|
foreach (StepItem sia in MyAfterStepItems)
|
|
{
|
|
sia.MyStepRTB.SetBackColor();
|
|
sia.SetBackColor();
|
|
}
|
|
}
|
|
if (MyBeforeStepItems != null)
|
|
{
|
|
foreach (StepItem sib in MyBeforeStepItems)
|
|
{
|
|
sib.MyStepRTB.SetBackColor();
|
|
sib.SetBackColor();
|
|
}
|
|
}
|
|
if (MyRNOStepItems != null)
|
|
{
|
|
foreach (StepItem sir in MyRNOStepItems)
|
|
{
|
|
sir.MyStepRTB.SetBackColor();
|
|
sir.SetBackColor();
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region Event Handlers
|
|
/// <summary>
|
|
/// If the background changes, change the background of the RichTextBox
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void StepItem_BackColorChanged(object sender, EventArgs e)
|
|
{
|
|
//_MyStepRTB.BackColor = BackColor;
|
|
_MyStepRTB.SetBackColor();
|
|
}
|
|
/// <summary>
|
|
/// When the RichTextBox height changes, change the height of the control to match
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void _StepRTB_HeightChanged(object sender, EventArgs args)
|
|
{
|
|
if (this.Height != _MyStepRTB.Height+_MyStepRTB.Top+7) // add in 7 to make it look good // + 10)
|
|
{
|
|
//if (MyID == 2131 || MyID == 2132)
|
|
// Console.WriteLine("oops!");
|
|
LastMethodsPush(string.Format("_StepRTB_HeightChanged {0}", _MyStepRTB.Height));
|
|
this.Height = _MyStepRTB.Height + _MyStepRTB.Top + 7;
|
|
LastMethodsPop();
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Handle the colape event
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void vlnExp_BeforeColapse(object sender, vlnExpanderEventArgs args)
|
|
{
|
|
Cursor tmp = Cursor.Current;
|
|
Cursor.Current = Cursors.WaitCursor;
|
|
int top = TopMostStepItem.Top;// This does'nt work - this is since the last time it was expanded.
|
|
_Colapsing = true;
|
|
// Hide Children
|
|
HideChildren();
|
|
// Adjust Positions
|
|
_ExpandPrefix = Top - top;
|
|
_ExpandSuffix = BottomMostStepItem.Bottom - Bottom;
|
|
if (Top != top)
|
|
{
|
|
LastMethodsPush(string.Format("Colapse {0}", MyID));
|
|
_MyStepPanel.ItemMoving++;
|
|
Top = top;
|
|
_MyStepPanel.ItemMoving--;
|
|
LastMethodsPop();
|
|
}
|
|
else
|
|
AdjustLocation();
|
|
BottomMostStepItem.AdjustLocation();
|
|
_Colapsing = false;
|
|
Cursor.Current = tmp;
|
|
}
|
|
/// <summary>
|
|
/// Handle the expand event
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void vlnExp_BeforeExpand(object sender, vlnExpanderEventArgs args)
|
|
{
|
|
Cursor tmp = Cursor.Current;
|
|
Cursor.Current = Cursors.WaitCursor;
|
|
if (!_Loading && MyExpandingStatus == ExpandingStatus.No)
|
|
Expand(_Type >= 20000);
|
|
Cursor.Current = tmp;
|
|
}
|
|
bool _IgnoreResize = false;
|
|
/// <summary>
|
|
/// Adjust the locations when the StepItem is resized
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void StepItem_Resize(object sender, EventArgs e)
|
|
{
|
|
if (_MyItemInfo == null) return;
|
|
if (_IgnoreResize) return;
|
|
AdjustLocation();
|
|
if (lblHeader != null) lblHeader.Width = this.Width;
|
|
}
|
|
private string WatchThisIndent
|
|
{
|
|
get { return "".PadLeft(_WatchThis, '\t'); }
|
|
}
|
|
/// <summary>
|
|
/// Handles movement of the StepItems
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void StepItem_Move(object sender, EventArgs e)
|
|
{
|
|
int watchThis = _WatchThis;
|
|
//if (MyID == _lookForID || MyID == 2111)
|
|
//{
|
|
// //vlnStackTrace.ShowStack("{0} Move TO {1} - {2}, BottomMost {3}", MyID, Top, MyPath, BottomMostStepItem.MyPath);
|
|
// Console.WriteLine("{0} Move TO {1} - {2}, BottomMost {3}", MyID, Top, MyPath, BottomMostStepItem.MyPath);
|
|
//}
|
|
//if (MyID > _StartingID)
|
|
// Console.WriteLine("{0}--------------- {1} Top = {2} Bottom {3}", WatchThisIndent, MyID, Top, Bottom);
|
|
if (_MyStepPanel.ItemMoving == 0)
|
|
{
|
|
//vlnStackTrace.ScrollInStack();
|
|
return; // If 0 - Indicates scrolling which requires no action.
|
|
}
|
|
//ShowMe("Move");
|
|
if (_MyItemInfo == null)
|
|
return;
|
|
//if (_WatchThis > 0 && MyID > _StartingID)
|
|
//{
|
|
|
|
// Console.WriteLine("{0}Start Move {1},{2}", WatchThisIndent, MyID, this);
|
|
// if (MyID == _LookForID)
|
|
// Console.WriteLine("{0}---------------", WatchThisIndent,MyID, this);
|
|
// _WatchThis++;
|
|
//}
|
|
if (MyExpandingStatus == ExpandingStatus.Expanding)
|
|
{
|
|
_WatchThis=watchThis;
|
|
return;
|
|
}
|
|
Moving = true;
|
|
StepItem tmp = (StepItem)sender;
|
|
if (tmp._MyPreviousStepItem == null && tmp._MyParentStepItem == null)
|
|
{
|
|
_WatchThis=watchThis;
|
|
return;
|
|
}
|
|
if (RNOBelow) // Adjust substeps first
|
|
{
|
|
//Console.WriteLine("RNOBelow");
|
|
AdjustLocation();
|
|
MoveRNO();
|
|
}
|
|
else // Adjust RNO First
|
|
{
|
|
if (RNORight)
|
|
{
|
|
//Console.WriteLine("RNORight");
|
|
MoveRNO();
|
|
}
|
|
AdjustLocation();
|
|
}
|
|
Moving = false;
|
|
StepItem btm = BottomMostStepItem;
|
|
if(this != btm)
|
|
btm.AdjustLocation();
|
|
//if (_WatchThis > 0 && MyID > _StartingID)
|
|
//{
|
|
// Console.WriteLine("{0}Finish Move {1},{2}",WatchThisIndent, MyID, this);
|
|
//}
|
|
_WatchThis=watchThis;
|
|
}
|
|
private bool RNOBelow
|
|
{
|
|
get
|
|
{
|
|
if (_MyRNOStepItems != null)
|
|
{
|
|
return _MyRNOStepItems[0].RNOLevel > _MyItemInfo.ColumnMode;
|
|
//return _MyRNOStepItems[0].Left == Left;
|
|
}
|
|
return false;
|
|
}
|
|
}
|
|
private bool RNORight
|
|
{
|
|
get
|
|
{
|
|
if (_MyRNOStepItems != null)
|
|
{
|
|
return _MyRNOStepItems[0].RNOLevel <= _MyItemInfo.ColumnMode;
|
|
//return _MyRNOStepItems[0].Left != Left;
|
|
}
|
|
return false;
|
|
}
|
|
}
|
|
private void MoveRNO()
|
|
{
|
|
if (_MyRNOStepItems != null)
|
|
{
|
|
if (_MyRNOStepItems[0].TopMostStepItem.Top != Top)
|
|
{
|
|
//if(_MyLog.IsDebugEnabled)_MyLog.DebugFormat("\r\n'Adjust RNO',{0},'Move',{1}", MyID, _RNO[0].MyID);
|
|
StepItem rnoTop = _MyRNOStepItems[0].TopMostStepItem;
|
|
if (rnoTop.RNOLevel <= _MyItemInfo.ColumnMode)
|
|
{
|
|
//StepItem tmpBottom = this;
|
|
//if (_MyAfterStepItems != null) tmpBottom = _MyAfterStepItems[_MyAfterStepItems.Count - 1].BottomMostStepItem;
|
|
_MyStepPanel.ItemMoving++;
|
|
rnoTop.LastMethodsPush(string.Format("StepItem_Move RNO Right {0}", rnoTop.MyID));
|
|
//rnoTop.Top = tmpBottom.Bottom;
|
|
rnoTop.Top = Top;
|
|
rnoTop.LastMethodsPop();
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
else
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
rnoTop.LastMethodsPush(string.Format("StepItem_Move RNO Below {0} {1} {2}", rnoTop.MyID, BottomMostStepItemNoRNOs.MyID, BottomMostStepItemNoRNOs.Bottom));
|
|
rnoTop.Top = BottomMostStepItemNoRNOs.Bottom;
|
|
rnoTop.LastMethodsPop();
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Handle the LinkGoTO event
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void _StepRTB_LinkGoTo(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyLog.DebugFormat("_DisplayRTB_LinkGoTo " + args.LinkInfoText);
|
|
_MyStepPanel.OnLinkClicked(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Raises an ItemClick event when the user clicks on the Tab
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void lblTab_MouseDown(object sender, MouseEventArgs e)
|
|
{
|
|
_MyStepPanel.OnItemClick(this, new StepPanelEventArgs(this, e));
|
|
}
|
|
/// <summary>
|
|
/// When a RichTextBox is entered, the selected StepRTB is set
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void _StepRTB_Enter(object sender, EventArgs e)
|
|
{
|
|
if (_MyStepPanel.DisplayItemChanging) return;
|
|
//vlnStackTrace.ShowStack("_StepRTB_Enter {0}",this.MyID);
|
|
_MyStepPanel.SelectedStepRTB = _MyStepRTB;
|
|
}
|
|
/// <summary>
|
|
/// Raise a OnLinkModifyTran event, when the user chooses to Modify a transition
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void _StepRTB_LinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyStepPanel.OnLinkModifyTran(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Raise a OnLinkModifyRO event, when the user chooses to modify an RO
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void _StepRTB_LinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
_MyStepPanel.OnLinkModifyRO(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Pass the AttachmentClick event to the StepPanel control.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
private void vlnExp_AttachmentClick(object sender, vlnExpanderEventArgs args)
|
|
{
|
|
_MyStepPanel.OnAttachmentClicked(sender, new StepPanelAttachmentEventArgs(this));
|
|
}
|
|
private void _MyStepRTB_CursorKeyPress(object sender, KeyEventArgs args)
|
|
{
|
|
_MyStepPanel.StepCursorKeys(sender as StepRTB, args);
|
|
}
|
|
private void _MyStepRTB_CursorMovement(object sender, StepRTBCursorMovementEventArgs args)
|
|
{
|
|
_MyStepPanel.CursorMovement(sender as StepRTB, args.CursorLocation, args.Key);
|
|
}
|
|
private void _MyStepRTB_ModeChange(object sender, StepRTBModeChangeEventArgs args)
|
|
{
|
|
_MyStepPanel.OnModeChange(sender as StepRTB, args);
|
|
}
|
|
#endregion // Event Handlers
|
|
#region Private Methods
|
|
/// <summary>
|
|
/// Finds the last child in a list
|
|
/// </summary>
|
|
/// <param name="childStepItems"></param>
|
|
/// <returns></returns>
|
|
private static StepItem LastChild(List<StepItem> childStepItems)
|
|
{
|
|
return childStepItems[childStepItems.Count - 1];
|
|
}
|
|
/// <summary>
|
|
/// Calculate TableWidth based upon the the contents
|
|
/// </summary>
|
|
/// <param name="myFont"></param>
|
|
/// <param name="txt"></param>
|
|
/// <param name="addBorder"></param>
|
|
/// <returns></returns>
|
|
public float TableWidth(Font myFont, string txt, bool addBorder)
|
|
{
|
|
string[] lines = txt.Split("\n".ToCharArray());
|
|
float max = 0;
|
|
using (Graphics g = this.CreateGraphics())
|
|
{
|
|
PointF pnt = new PointF(0, 0);
|
|
foreach (string line in lines)
|
|
{
|
|
string lineAdj = Regex.Replace(line, @"\\u....\?", "X"); // Replace Special characters
|
|
//line2 = Regex.Replace(line2, @"\\.*? ", ""); // Remove RTF Commands - Really should not be any
|
|
lineAdj = StepRTB.RemoveLinkComments(lineAdj);
|
|
// MeasureString doesn't work properly if the line include graphics characters.
|
|
// So, Measure a string of the same length with 'M's.
|
|
SizeF siz = g.MeasureString("".PadLeft(lineAdj.Length + (addBorder ? 2 : 0), 'M'), myFont, pnt, StringFormat.GenericTypographic);
|
|
float wid = siz.Width;
|
|
if (wid > max)
|
|
{
|
|
max = wid;
|
|
}
|
|
}
|
|
}
|
|
float widLimit = (float) _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.WidSTableEdit, MyItemInfo.ColumnMode);
|
|
widLimit += (float)_MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColS);
|
|
widLimit += (float) _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColRTable, MyItemInfo.ColumnMode);
|
|
max += _MyStepPanel.MyStepPanelSettings.TableWidthAdjust;
|
|
return Math.Min(max,widLimit);
|
|
}
|
|
/// <summary>
|
|
/// Calculates the table location
|
|
/// </summary>
|
|
/// <param name="myParentStepItem"></param>
|
|
/// <param name="myStepSectionLayoutData"></param>
|
|
/// <param name="width"></param>
|
|
/// <returns></returns>
|
|
public Point TableLocation(StepItem myParentStepItem, StepSectionLayoutData myStepSectionLayoutData, int width)
|
|
{
|
|
// Should center on parent unless it is a centered table in the AER column
|
|
int center = myParentStepItem.TextLeft + myParentStepItem.TextWidth / 2;
|
|
int rightLimit = myParentStepItem.Right;
|
|
// Then should center on the wid Limit
|
|
if (MyItemInfo.FormatStepData.Type.Contains("AER") == false && MyItemInfo.RNOLevel == 0)
|
|
{
|
|
int colR = _MyStepPanel.ToDisplay(_MyStepSectionLayoutData.ColRTable, MyItemInfo.ColumnMode);
|
|
rightLimit += colR * MyItemInfo.ColumnMode;
|
|
center += (colR * MyItemInfo.ColumnMode) / 2;
|
|
center -= (myParentStepItem.TextLeft - (int)MyItemInfo.MyDocStyle.Layout.LeftMargin) / 2;
|
|
}
|
|
|
|
// Calulate the x location
|
|
//int x = myParentStepItem.TextLeft;
|
|
int x = center - width / 2;
|
|
if (x + width > rightLimit) x = rightLimit - width;
|
|
int colT = _MyStepPanel.ToDisplay(myStepSectionLayoutData.ColT);
|
|
if (x < colT) x = colT;
|
|
int y = FindTop(myParentStepItem.Bottom);
|
|
return new Point(x, y);
|
|
}
|
|
/// <summary>
|
|
/// Sets the Item and as a result the text for the RichTextBox
|
|
/// </summary>
|
|
private void SetText()
|
|
{
|
|
LastMethodsPush("SetText");
|
|
if (_MyItemInfo != null)
|
|
this._MyStepRTB.MyItemInfo = _MyItemInfo;
|
|
LastMethodsPop();
|
|
}
|
|
/// <summary>
|
|
/// If the selected StepItem is within the window leave it as it is.
|
|
/// If not, scroll so that the selected window is centered.
|
|
/// </summary>
|
|
private void ScrollToCenter()
|
|
{
|
|
//vlnStackTrace.ShowStack("CenterScroll {0} Current {1} Top {2} Bottom {3} Limit {4}", _MyItem.ItemID, _Panel.VerticalScroll.Value, Top, Bottom, _Panel.Height);// Show StackTrace
|
|
//Console.WriteLine("CenterScroll {0} Current {1} Top {2} Bottom {3} Limit {4}", _MyItem.ItemID, _Panel.VerticalScroll.Value, Top, Bottom, _Panel.Height);
|
|
if (Top >= 0 && Bottom <= _MyStepPanel.Height) return;// Don't move if within screen.
|
|
int scrollValue = _MyStepPanel.VerticalScroll.Value + (Top - (_MyStepPanel.Height / 2)); // calculate scroll center for the item
|
|
//Console.WriteLine("CenterScroll {0} Current {1} New {2} Min {3} Max {4}", _MyItem.ItemID, _Panel.VerticalScroll.Value, scrollValue, _Panel.VerticalScroll.Minimum, _Panel.VerticalScroll.Maximum);
|
|
if (scrollValue >= _MyStepPanel.VerticalScroll.Minimum && scrollValue <= _MyStepPanel.VerticalScroll.Maximum) // If it is within range
|
|
_MyStepPanel.VerticalScroll.Value = scrollValue; // Center the item
|
|
}
|
|
// TODO: the format of the circles, Checkoffs and Changebars should come from the format file
|
|
/// <summary>
|
|
/// This adds drawing items to the StepItem as needed including
|
|
/// Circle around the Tab Number
|
|
/// Check-Offs
|
|
/// Change Bars
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void StepItem_Paint(object sender, PaintEventArgs e)
|
|
{
|
|
Graphics g = e.Graphics;
|
|
g.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
|
|
//g.DrawString(lblTab.Text, MyItemInfo.MyTab.MyFont.WindowsFont, Brushes.Red, new RectangleF(new PointF(_MyStepPanel.MyStepPanelSettings.NumberLocationX, _MyStepPanel.MyStepPanelSettings.NumberLocationY), _MyStepPanel.MyStepPanelSettings.NumberSize), StringFormat.GenericDefault);
|
|
// adjust x location of label by 7, to position correctly.
|
|
//g.DrawString(lblTab.Text, MyItemInfo.MyTab.MyFont.WindowsFont, Brushes.Blue, new RectangleF(new PointF(Convert.ToSingle(lblTab.Location.X-7), Convert.ToSingle(lblTab.Location.Y)), _MyStepPanel.MyStepPanelSettings.NumberSize), StringFormat.GenericDefault);
|
|
//g.DrawLine(Pens.DarkGreen, lblTab.Location.X-7, 0, lblTab.Location.X-7, this.Height);
|
|
//g.DrawLine(Pens.DarkGreen, MyStepRTB.Location.X - 7, 0, MyStepRTB.Location.X - 7, this.Height);
|
|
g.DrawString(lblTab.Text, MyItemInfo.MyTab.MyFont.WindowsFont, Brushes.Black, new RectangleF(new PointF(Convert.ToSingle(lblTab.Location.X), Convert.ToSingle(lblTab.Location.Y)), _MyStepPanel.MyStepPanelSettings.NumberSize), StringFormat.GenericDefault);
|
|
//g.DrawLine(Pens.DarkGreen, lblTab.Location.X, 0, lblTab.Location.X, this.Height);
|
|
//g.DrawLine(Pens.DarkGreen, MyStepRTB.Location.X, 0, MyStepRTB.Location.X, this.Height);
|
|
if (Circle)
|
|
{
|
|
Pen penC = new Pen(_MyStepPanel.MyStepPanelSettings.CircleColor, _MyStepPanel.MyStepPanelSettings.CircleWeight);
|
|
g.DrawArc(penC, lblTab.Left + 1, 0, _MyStepPanel.MyStepPanelSettings.CircleDiameter, _MyStepPanel.MyStepPanelSettings.CircleDiameter, 0F, 360F);
|
|
}
|
|
if (CheckOff)
|
|
{
|
|
Pen penCO = new Pen(_MyStepPanel.MyStepPanelSettings.CheckOffColor, _MyStepPanel.MyStepPanelSettings.CheckOffWeight);
|
|
g.DrawRectangle(penCO, _MyStepPanel.MyStepPanelSettings.CheckOffX, _MyStepPanel.MyStepPanelSettings.CheckOffY, _MyStepPanel.MyStepPanelSettings.CheckOffSize, _MyStepPanel.MyStepPanelSettings.CheckOffSize);
|
|
}
|
|
if (ChangeBar)
|
|
{
|
|
Pen penCB = new Pen(_MyStepPanel.MyStepPanelSettings.ChangeBarColor, _MyStepPanel.MyStepPanelSettings.ChangeBarWeight);
|
|
g.DrawLine(penCB, 0, _MyStepRTB.Top, 0, Height);
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Hide a Items Children - Part of colapsing
|
|
/// </summary>
|
|
protected void HideChildren()
|
|
{
|
|
HideChildren(_MyBeforeStepItems);
|
|
HideChildren(_MyRNOStepItems);
|
|
HideChildren(_MyAfterStepItems);
|
|
}
|
|
/// <summary>
|
|
/// Hide a list of children - Part of colapsing
|
|
/// </summary>
|
|
/// <param name="childStepItems"></param>
|
|
private void HideChildren(List<StepItem> childStepItems)
|
|
{
|
|
if (childStepItems != null)
|
|
{
|
|
foreach (StepItem child in childStepItems)
|
|
{
|
|
if (child.Expanded) child.HideChildren();
|
|
child.Hidden = true;
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Unhide an items Children - Part of expanding
|
|
/// </summary>
|
|
/// <param name="expand"></param>
|
|
protected void UnhideChildren(bool expand)
|
|
{
|
|
UnhideChildren(_MyBeforeStepItems, expand);
|
|
UnhideChildren(_MyRNOStepItems, expand);
|
|
UnhideChildren(_MyAfterStepItems, expand);
|
|
if (!_MyvlnExpander.Expanded)
|
|
_MyvlnExpander.ShowExpanded();
|
|
}
|
|
/// <summary>
|
|
/// Unhide a list of children - part of expanding
|
|
/// </summary>
|
|
/// <param name="childStepItems"></param>
|
|
/// <param name="expand"></param>
|
|
private void UnhideChildren(List<StepItem> childStepItems, bool expand)
|
|
{
|
|
if (childStepItems != null)
|
|
{
|
|
foreach (StepItem child in childStepItems)
|
|
{
|
|
if (child.Expanded)
|
|
child.UnhideChildren(expand);
|
|
else if (expand)
|
|
child.Expand(expand);
|
|
child.Hidden = false;
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Move children as necessary
|
|
/// </summary>
|
|
protected void AdjustChildren()
|
|
{
|
|
AdjustChildren(_MyBeforeStepItems);
|
|
AdjustChildren(_MyRNOStepItems);
|
|
AdjustChildren(_MyAfterStepItems);
|
|
}
|
|
/// <summary>
|
|
/// Move a list of children
|
|
/// </summary>
|
|
/// <param name="childStepItems"></param>
|
|
private void AdjustChildren(List<StepItem> childStepItems)
|
|
{
|
|
if (childStepItems != null)
|
|
{
|
|
foreach (StepItem child in childStepItems)
|
|
{
|
|
child.AdjustLocation();
|
|
if (child.Expanded) child.AdjustChildren();
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Expand a list of children
|
|
/// </summary>
|
|
/// <param name="childStepItems"></param>
|
|
private void ExpandChildren(List<StepItem> childStepItems)
|
|
{
|
|
if (childStepItems != null)
|
|
{
|
|
foreach (StepItem child in childStepItems)
|
|
{
|
|
if (child.CanExpand)
|
|
{
|
|
child.Expand(true);
|
|
}
|
|
child.Hidden = false;
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Expand children
|
|
/// </summary>
|
|
private void ExpandChildren()
|
|
{
|
|
// Walk though Children performing Expand
|
|
ExpandChildren(_MyBeforeStepItems);
|
|
ExpandChildren(_MyRNOStepItems);
|
|
ExpandChildren(_MyAfterStepItems);
|
|
}
|
|
private string MyPath
|
|
{
|
|
get
|
|
{
|
|
if (MyItemInfo.MyContent.Type >= 20000)
|
|
return MyItemInfo.Path.Substring(MyItemInfo.ActiveSection.Path.Length);
|
|
return MyItemInfo.MyContent.ToString();
|
|
}
|
|
}
|
|
private StepItem AERStepItem
|
|
{
|
|
get
|
|
{
|
|
if(RNOLevel == 0)return null;
|
|
if (MyParentStepItem != null)
|
|
{
|
|
if (MyParentStepItem.RNOLevel < RNOLevel)
|
|
return MyParentStepItem;
|
|
else
|
|
return MyParentStepItem.AERStepItem;
|
|
}
|
|
else if (MyPreviousStepItem != null)
|
|
{
|
|
return MyPreviousStepItem.AERStepItem;
|
|
}
|
|
//Volian.Base.Library.vlnStackTrace.ShowStackLocal("'AERStepItem',{0},{1}", MyID, MyItemInfo.DBSequence);
|
|
return null;
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Adjust the Location of all items below the current item.
|
|
/// </summary>
|
|
internal void AdjustLocation()
|
|
{
|
|
//Console.WriteLine("'AdjustLocation',{0},{1},'{2}'", MyID, MyItemInfo.DBSequence, Volian.Base.Library.vlnStackTrace.CalledFrom4);
|
|
if (RNORight) MoveRNO(); // This is needed when an AER is Deleted that has an RNO.
|
|
if (RNOLevel > 0 && AERStepItem != null)
|
|
AERStepItem.AdjustLocation();
|
|
StepItem nextStepItem = NextDownStepItem;
|
|
//if (MyID == 2138)
|
|
// Console.WriteLine("2138");
|
|
//if (_WatchThis > 0 && MyID > _StartingID)
|
|
//if((nextStepItem == null ? 0 : nextStepItem.MyID) == MyID)
|
|
//if(_LookForID.Contains(MyID))
|
|
// Console.WriteLine("{0}AdjustLocation {1},{2},{3} -> {4},{5} ({6}) {7}", WatchThisIndent, MyID, MyItemInfo.Ordinal, MyPath,
|
|
// nextStepItem == null ? 0 : nextStepItem.MyID, nextStepItem == null ? 0 : nextStepItem.MyItemInfo.Ordinal, nextStepItem == null ? "Null" : nextStepItem.MyPath,
|
|
// _NextDownStepItemPath);
|
|
//if (_WatchThis && MyID == 2119)
|
|
// Console.WriteLine("I'm here");
|
|
//if (MyID > 2120)
|
|
// Console.WriteLine("{0}\t{1}", MyID, nextStepItem == null ? 0 : nextStepItem.MyID);
|
|
if (nextStepItem != null)
|
|
{
|
|
//int bottom = BottomMostStepItem.Bottom;
|
|
if (nextStepItem != null)
|
|
{
|
|
//if (MyID == 2123)
|
|
// _LookForID = 2123;
|
|
int bottom = nextStepItem.FindTop(Bottom);
|
|
if (nextStepItem.Top != bottom)
|
|
// if (nextStepItem != null && !nextStepItem.Moving && nextStepItem.Top != bottom)
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
nextStepItem.LastMethodsPush(string.Format("AdjustLocation {0}",MyID));
|
|
nextStepItem._NextDownStepItemPath = _NextDownStepItemPath;
|
|
nextStepItem.Top = bottom;
|
|
nextStepItem.LastMethodsPop();
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
Console.WriteLine("{0}** No Adjustment next = {1}, moving = {2}", WatchThisIndent, nextStepItem, nextStepItem == null ? false : nextStepItem.Moving);
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Automatically expands Steps if not currently expanded
|
|
/// </summary>
|
|
internal void AutoExpand()
|
|
{
|
|
if (CanExpand && Expanded == false)// TODO: May need to do some additional checking for subsections
|
|
{
|
|
//vlnStackTrace.ShowStack(">AutoExpand ID {0} - Can {1} Expanded {2}", _MyItem.ItemID, CanExpand, Expanded);
|
|
Expand(_Type >= 20000);
|
|
//Console.WriteLine("<AutoExpand ID {0} - Can {1} Expanded {2}",_MyItem.ItemID, CanExpand, Expanded);
|
|
}
|
|
}
|
|
#endregion // Private Methods
|
|
#region Public Methods
|
|
/// <summary>
|
|
/// Sets the focus to this StepItem and positions the cursor to the begining of the string
|
|
/// </summary>
|
|
public void ItemSelect()
|
|
{
|
|
_MyStepRTB.Focus();
|
|
_MyStepRTB.Select(0, 0);
|
|
// if (CanExpand) AutoExpand(); // Expand the item if you can
|
|
ScrollToCenter();
|
|
}
|
|
/// <summary>
|
|
/// Sets the focus to this StepItem
|
|
/// </summary>
|
|
public void ItemShow()
|
|
{
|
|
_MyStepRTB.Focus();
|
|
ScrollToCenter();
|
|
}
|
|
/// <summary>
|
|
/// Expand an item and it's children
|
|
/// <para/>If the children have been loaded then just expand them
|
|
/// <para/>If not, load the children and expand their children etc.
|
|
/// </summary>
|
|
/// <param name="expand">normally equal to _Type > = 20000 (Step)</param>
|
|
public void Expand(bool expand)
|
|
{
|
|
//// TIMING: DisplayItem.TimeIt("Expand Start");
|
|
if (_ChildrenLoaded)
|
|
{
|
|
// Unhide Children
|
|
MyExpandingStatus = ExpandingStatus.Showing;
|
|
UnhideChildren(expand);
|
|
if (_ExpandPrefix != 0)
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
TopMostStepItem.Top = Top;
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
else
|
|
TopMostStepItem.AdjustLocation();
|
|
AdjustChildren();
|
|
//if(_Before != null )
|
|
// Top = _Before[_Before.Count - 1].BottomMost.Bottom;
|
|
}
|
|
else
|
|
{
|
|
MyExpandingStatus = ExpandingStatus.Expanding;
|
|
_ChildrenLoaded = true;
|
|
//_Panel.SuspendLayout();
|
|
AddChildBefore(MyItemInfo.Cautions, expand);
|
|
AddChildBefore(MyItemInfo.Notes, expand);
|
|
AddChildAfter(MyItemInfo.Procedures, expand);
|
|
AddChildAfter(MyItemInfo.Sections, expand);
|
|
if (MyItemInfo.RNOs != null && MyItemInfo.RNOLevel < MyItemInfo.ColumnMode)
|
|
AddChildRNO(MyItemInfo.RNOs, expand);
|
|
AddChildAfter(MyItemInfo.Tables, expand);
|
|
AddChildAfter(MyItemInfo.Steps, expand);
|
|
if (MyItemInfo.RNOs != null && MyItemInfo.RNOLevel >= MyItemInfo.ColumnMode)
|
|
AddChildRNO(MyItemInfo.RNOs, expand);
|
|
if (!_MyvlnExpander.Expanded)
|
|
_MyvlnExpander.ShowExpanded();
|
|
}
|
|
MyExpandingStatus = ExpandingStatus.Done;
|
|
BottomMostStepItem.AdjustLocation();
|
|
MyExpandingStatus = ExpandingStatus.No;
|
|
//// TIMING: DisplayItem.TimeIt("Expand End");
|
|
}
|
|
public StepItem BeforeItem
|
|
{
|
|
get
|
|
{
|
|
if (_MyAfterStepItems != null)
|
|
{
|
|
foreach (StepItem stepItem in _MyAfterStepItems)
|
|
{
|
|
if (stepItem._MyBeforeStepItems != null)
|
|
return stepItem.TopMostStepItem;
|
|
StepItem beforeItem = stepItem.BeforeItem;
|
|
if (beforeItem != null) return beforeItem;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
public StepItem ItemAbove
|
|
{
|
|
get
|
|
{
|
|
if (_MyChildRelation == ChildRelation.Before) return _MyParentStepItem.ItemAbove;
|
|
if (_MyPreviousStepItem != null) return _MyPreviousStepItem.BottomMostStepItem;
|
|
if (_MyChildRelation == ChildRelation.After) return _MyParentStepItem;
|
|
if (_MyChildRelation == ChildRelation.RNO) return _MyParentStepItem;
|
|
return null;
|
|
}
|
|
}
|
|
private static int? max(int? value1, int value2)
|
|
{
|
|
if (value1 == null || value2 > value1) return value2;
|
|
return value1;
|
|
}
|
|
private static int min(int value1, int value2)
|
|
{
|
|
if (value2 < value1) return value2;
|
|
return value1;
|
|
}
|
|
public int FindRight()
|
|
{
|
|
if (!RNORight) return Right;
|
|
return _MyRNOStepItems[0].FindRight();
|
|
}
|
|
public int? BottomOfParentRNO()
|
|
{
|
|
int? bottom = null;
|
|
StepItem stepItem = this;
|
|
if (!MyItemInfo.IsTablePart)
|
|
{
|
|
if (stepItem._MyChildRelation == ChildRelation.None)
|
|
return null;
|
|
if (stepItem._MyChildRelation == ChildRelation.After && !RNORight)
|
|
return null;
|
|
}
|
|
while (stepItem != null && stepItem._MyChildRelation != ChildRelation.None && stepItem._MyChildRelation != ChildRelation.After)
|
|
stepItem = stepItem.UpOneStepItem;
|
|
if (stepItem == null || stepItem._MyChildRelation == ChildRelation.None)
|
|
return null;
|
|
StepItem parent = stepItem.UpOneStepItem;
|
|
int right = FindRight();
|
|
bool centeredTable = (MyItemInfo.IsTablePart && MyItemInfo.FormatStepData.Type.Contains("AER") == false && MyItemInfo.RNOLevel == 0);
|
|
while (parent != null && parent.MyItemInfo.IsSection == false)
|
|
{
|
|
if (parent._MyRNOStepItems != null)
|
|
{
|
|
if (centeredTable || right > parent._MyRNOStepItems[0].Left)
|
|
{
|
|
if(parent._MyRNOStepItems[0].BottomMostStepItem.RNOLevel > RNOLevel && RNOLevel < _MyItemInfo.ColumnMode)
|
|
bottom = max(bottom, parent._MyRNOStepItems[0].BottomMostStepItem.Bottom);
|
|
}
|
|
}
|
|
parent = parent.UpOneStepItem;
|
|
}
|
|
return bottom;
|
|
}
|
|
private string _NextDownStepItemPath = "None";
|
|
/// <summary>
|
|
/// This finds the next StepItem down.
|
|
/// </summary>
|
|
public StepItem NextDownStepItem
|
|
{
|
|
get
|
|
{
|
|
StepItem stepItem = this;
|
|
_NextDownStepItemPath = "Path 1";
|
|
// If this item appears before it's parent, and it doesn't have anything below it, return the parent
|
|
if (MyNextStepItem == null && MyAfterStepItems == null && FirstSiblingStepItem._MyChildRelation == ChildRelation.Before)
|
|
return UpOneStepItem;
|
|
_NextDownStepItemPath = "Path 2";
|
|
if (Expanded && _MyAfterStepItems != null)// check to see if there is a _After
|
|
return MyAfterStepItems[0].TopMostStepItem;// if there is, go that way
|
|
_NextDownStepItemPath = "Path 3";
|
|
if (Expanded && MyRNOStepItems != null && MyItemInfo.RNOLevel >= MyItemInfo.ColumnMode)// check to see if there is a _After
|
|
return MyRNOStepItems[0].TopMostStepItem;// if there is, go that way
|
|
while (stepItem != null && stepItem.MyNextStepItem == null) // if no Next walk up the parent path
|
|
{
|
|
bool lastWasRNO = (stepItem._MyChildRelation == ChildRelation.RNO);
|
|
stepItem = stepItem.UpOneStepItem;
|
|
_NextDownStepItemPath = "Path 4";
|
|
if (stepItem == null) // No Parent
|
|
return null;
|
|
_NextDownStepItemPath = string.Format("Path 5 {0}, {1}",stepItem.MyExpandingStatus,stepItem.Moving);
|
|
if (stepItem.MyExpandingStatus == ExpandingStatus.Expanding || stepItem.Moving) // Parent Expanding or Moving - Wait
|
|
return null;
|
|
_NextDownStepItemPath = "Path 5 RNO";
|
|
if (stepItem.RNOBelow && !Ancestor(stepItem.MyRNOStepItems[0]))
|
|
return stepItem.MyRNOStepItems[0];
|
|
_NextDownStepItemPath = "Path 6";
|
|
if (stepItem.MyNextStepItem == null && stepItem.FirstSiblingStepItem._MyChildRelation == ChildRelation.Before)
|
|
return stepItem.UpOneStepItem;
|
|
StepItem btm = stepItem.BottomMostStepItem; // Find the Bottom StepItem of this ancestor
|
|
StepItem beforeItem = stepItem.BeforeItem;
|
|
if (lastWasRNO && beforeItem != null)
|
|
{
|
|
_NextDownStepItemPath = "Path 7";
|
|
if (beforeItem.ItemAbove.Bottom > this.Bottom) return null;
|
|
_NextDownStepItemPath = "Path 8";
|
|
return beforeItem;
|
|
}
|
|
if (this != btm) // If this is not the bottom, then just adjust things with respect to the bottom
|
|
{
|
|
StepItem btmNext = btm.NextDownStepItem;
|
|
//if (stepItem.MyNextStepItem != null && stepItem.MyNextStepItem.TopMostStepItem.Top != btm.Bottom)
|
|
if (btmNext != null)
|
|
{
|
|
int bottom = btmNext.FindTop(btm.Bottom);
|
|
if(btmNext.Top != bottom)
|
|
{
|
|
_MyStepPanel.ItemMoving++;
|
|
//stepItem.MyNextStepItem.TopMostStepItem.Top = btm.Bottom;
|
|
//Console.WriteLine("{0}***Move in NextDownStepItem {1},{2} From {3} To {4}",WatchThisIndent, btmNext.MyID, btmNext, btmNext.Top, btm.Bottom);
|
|
btmNext.LastMethodsPush(string.Format("NextDownStepItem {0} {1}", MyID, stepItem.MyID));
|
|
//ShowMe(string.Format("FindTop = {0}", btmNext.FindTop(btm.Bottom)));
|
|
btmNext.Top = bottom;
|
|
btmNext.LastMethodsPop();
|
|
_MyStepPanel.ItemMoving--;
|
|
}
|
|
}
|
|
_NextDownStepItemPath = string.Format("Path 9 {0}",btm);
|
|
return null; // Not the bottom - don't adjust anything else
|
|
}
|
|
//else
|
|
//{
|
|
//}
|
|
}
|
|
if (stepItem != null)
|
|
{
|
|
// Need to verify that the bottom of the parents RNO does not excede the bottom of this item.
|
|
StepItem next = stepItem.MyNextStepItem.TopMostStepItem;
|
|
_NextDownStepItemPath = "Path A";
|
|
//if (Bottom >= (BottomOfParentRNO(next) ?? Bottom))
|
|
return next;// if no _After - check to see if there is a Next
|
|
//_NextDownStepItemPath = "Path B";
|
|
//return null;
|
|
}
|
|
_NextDownStepItemPath = "Path C";
|
|
return null;
|
|
}
|
|
}
|
|
|
|
private bool Ancestor(StepItem stepItem)
|
|
{
|
|
if (MyID == stepItem.MyID) return true;
|
|
if (MyItemInfo.IsHigh) return false;
|
|
return UpOneStepItem.Ancestor(stepItem);
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return _MyItemInfo == null ? base.ToString() : string.Format("{0},'{1}'", MyID, MyPath); // + "-" + MyItemInfo.MyContent.Text;
|
|
}
|
|
#endregion
|
|
}
|
|
}
|