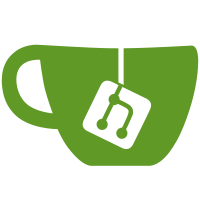
Use new settings (ConvertTo and ExecutableMode) New Settings Use new setting (PDF Folder) Use and Control new settings
109 lines
4.7 KiB
C#
109 lines
4.7 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
public TimeSpan MigrateDocVersion(DocVersion docver)
|
|
{
|
|
return MigrateDocVersion(docver, true);
|
|
}
|
|
|
|
private OutsideTransition _OutTran;
|
|
|
|
public TimeSpan MigrateDocVersion(DocVersion docver, bool convertProcedures)
|
|
{
|
|
long lTime = DateTime.Now.Ticks;
|
|
string pth = docver.Title;
|
|
|
|
if (!File.Exists(pth + @"\set.dbf") || !File.Exists(pth + @"\curset.dat")) return new TimeSpan(); // Open connection
|
|
OleDbConnection cn = new OleDbConnection(@"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + pth + ";Extended Properties=dBase III;Persist Security Info=False");
|
|
if (convertProcedures)
|
|
{
|
|
// JSJ 02/10/2010 - Remove all the .INF files before proceeding to convert.
|
|
// The INF files contain a path to a corresponding NDX file - had data with bat NDX path
|
|
DeleteINFFiles(pth);
|
|
_OutTran = new OutsideTransition(cn);
|
|
MigrateROFST(pth, docver);
|
|
// Migrate library documents
|
|
MigrateLibDocs(cn, pth);
|
|
// Initialize Dictionaries
|
|
dicTrans_ItemDone = new Dictionary<string, Item>();
|
|
dicTrans_ItemIds = new Dictionary<string, Item>();
|
|
dicTrans_MigrationErrors = new Dictionary<string, List<Item>>();
|
|
|
|
// Create a 'dummy' content record. This will be used for any transitions 'to'
|
|
// that don't exist when dbf is processed. At end, use this to see if there
|
|
// are missing transitions.
|
|
TransDummyCont = Content.MakeContent(null, "DUMMY CONTENT FOR TRANSITION MIGRATION", null, null, null);
|
|
}
|
|
// Process Procedures
|
|
Item itm = null;
|
|
if (convertProcedures || docver.VersionType == (int)VEPROMS.CSLA.Library.VersionTypeEnum.WorkingDraft || docver.VersionType == (int)VEPROMS.CSLA.Library.VersionTypeEnum.Approved)
|
|
itm = MigrateProcedures(cn, pth, docver, convertProcedures, docver.MyDocVersionInfo.ActiveFormat);
|
|
// Show any Missing Transtitons (i.e. Transitions which have not been processed)
|
|
lTime = DateTime.Now.Ticks - lTime;
|
|
if (convertProcedures)
|
|
{
|
|
ShowMissingTransitions(docver);
|
|
//frmMain.Status = string.Format("{0}\r\nConversion completed in {1} seconds.", pth, TimeSpan.FromTicks(lTime).TotalSeconds);
|
|
TimeSpan ts = TimeSpan.FromTicks(lTime);
|
|
frmMain.Status = string.Format("{0}\r\nConversion completion time: {1:D2}:{2:D2}:{3:D2}.{4} ({5} Total Seconds)", pth, ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds, ts.TotalSeconds);
|
|
log.InfoFormat("Completed Migration of {0}", pth);
|
|
|
|
//MessageBox.Show("Completed Migration of " + pth); //jsj commented out to let it run through
|
|
if (rofstinfo != null) rofstinfo.ROFSTLookup.Close();
|
|
dicTrans_ItemDone.Clear();
|
|
dicTrans_ItemDone = null;
|
|
}
|
|
else
|
|
frmMain.Status = string.Format("{0}\r\nDone.", pth);
|
|
cn.Close();
|
|
if (itm != null)
|
|
{
|
|
docver.MyItem = itm;
|
|
if (convertProcedures) docver.Title = ""; // clearing this tell us this docver (path) was converted?
|
|
if (!docver.IsSavable) ErrorRpt.ErrorReport(docver);
|
|
if(frmMain.MySettings.ExecutionMode == ExecutionMode.Debug)
|
|
docver.DocVersionConfig.Print_PDFLocation = frmMain.MySettings.PDFFolder;
|
|
docver.Save();
|
|
}
|
|
return TimeSpan.FromTicks(lTime);
|
|
}
|
|
private void DeleteINFFiles(string pth)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo(pth);
|
|
FileInfo [] myFiles = di.GetFiles("*.INF");
|
|
foreach (FileInfo myFile in myFiles)
|
|
myFile.Delete();
|
|
}
|
|
private VEPROMS.CSLA.Library.VersionTypeEnum DocVersionType(string s)
|
|
{
|
|
if (s.EndsWith("approved")) return VEPROMS.CSLA.Library.VersionTypeEnum.Approved;
|
|
if (s.EndsWith("chgsht")) return VEPROMS.CSLA.Library.VersionTypeEnum.Revision;
|
|
if (s.EndsWith("tmpchg")) return VEPROMS.CSLA.Library.VersionTypeEnum.Temporary;
|
|
return VEPROMS.CSLA.Library.VersionTypeEnum.WorkingDraft;
|
|
}
|
|
}
|
|
} |