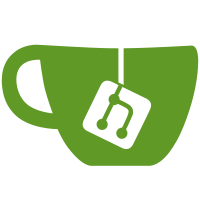
- Aligned Tables properly - Skip Section Title - Calculated Table Width - Fixed ChangeBar logic - Added Text and Debug layers - Adedd debug output to PDF - Changed Paginate logic - Adedd debug output to PDF - Move GetParagraphHeight - Added GetTableWidth - Added ChangeBar Properties - Added Debug and Text layers Removed fixed override color for SVG Removed simple PageBreak
551 lines
14 KiB
C#
551 lines
14 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using iTextSharp.text.pdf;
|
|
using iTextSharp.text;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Print.Library
|
|
{
|
|
public abstract partial class vlnPrintObject
|
|
{
|
|
protected static float _WidthAdjust = 1; // 1 Point - adjusted to match 16-bit line wrapping. For editting it's 3.
|
|
protected static float _WidthAdjustBox = 6;
|
|
protected static float _CharsToTwips = 6; // assumes 12 char per inch
|
|
internal static float _SixLinesPerInch = 12; // twips
|
|
protected static float _SevenLinesPerInch = 72F / 7F;
|
|
|
|
protected float _XOffset;
|
|
public float XOffset
|
|
{
|
|
get { return _XOffset; }
|
|
set { _XOffset = value; }
|
|
}
|
|
protected float _YOffset;
|
|
public float YOffset
|
|
{
|
|
get { return _YOffset; }
|
|
set { _YOffset = value; }
|
|
}
|
|
protected vlnParagraph _MyParent;
|
|
public vlnParagraph MyParent
|
|
{
|
|
get { return _MyParent; }
|
|
set
|
|
{
|
|
_MyParent = value;
|
|
if (_MyParent != null)
|
|
{
|
|
|
|
}
|
|
}
|
|
}
|
|
private string _Rtf; // may become iTextSharp paragraph
|
|
public string Rtf
|
|
{
|
|
get { return _Rtf; }
|
|
set { _Rtf = value; }
|
|
}
|
|
iTextSharp.text.Paragraph _IParagraph;
|
|
public iTextSharp.text.Paragraph IParagraph
|
|
{
|
|
get
|
|
{
|
|
if (_IParagraph == null)
|
|
{
|
|
_IParagraph = RtfToParagraph(Rtf);
|
|
}
|
|
return _IParagraph;
|
|
}
|
|
set { _IParagraph = value; }
|
|
}
|
|
private float _Width;
|
|
public float Width
|
|
{
|
|
get { return _Width; }
|
|
set { _Width = value; }
|
|
}
|
|
private float _Height;
|
|
public float Height
|
|
{
|
|
get
|
|
{
|
|
if (_Height == 0)
|
|
_Height = GetParagraphHeight(MyContentByte, IParagraph, Width);
|
|
return _Height;
|
|
}
|
|
}
|
|
public static float GetParagraphHeight(PdfContentByte cb, Paragraph iParagraph, float width)
|
|
{
|
|
return GetParagraphHeight(cb, iParagraph, width, true);
|
|
}
|
|
public static float GetParagraphHeight(PdfContentByte cb, Paragraph iParagraph, float width, bool throwException)
|
|
{
|
|
ColumnText myColumnText = new ColumnText(cb);
|
|
myColumnText.SetSimpleColumn(0, 792F, width, 0); // Bottom margin
|
|
myColumnText.AddElement(iParagraph);
|
|
//myColumnText.UseAscender = true;// Adjusts to the top of the text box.
|
|
int status = myColumnText.Go(true); // Check to see if it will fit on the page.
|
|
if (ColumnText.HasMoreText(status) && throwException)
|
|
throw (new Exception("Paragraph longer than a page"));
|
|
return 792F - myColumnText.YLine; // This gives the height of the Paragraph
|
|
}
|
|
public static float GetTableWidth(PdfContentByte cb, Paragraph iParagraph)
|
|
{
|
|
int iWidth = 128 * (int) _CharsToTwips; // maximum width in Characters
|
|
float h = GetParagraphHeight(cb, iParagraph, iWidth);
|
|
int iWidthMax = iWidth;
|
|
int iDelta = iWidth / 2;
|
|
iWidth = iWidth / 2;
|
|
while (iDelta > 0)
|
|
{
|
|
float h2 = GetParagraphHeight(cb, iParagraph, iWidth,false);
|
|
iDelta = iDelta / 2;
|
|
if (h2 == h) iWidthMax = iWidth;
|
|
iWidth += (h2>h ? 1: -1) * iDelta;
|
|
}
|
|
return (float) iWidthMax;
|
|
}
|
|
private PdfContentByte _MyContentByte;
|
|
public PdfContentByte MyContentByte
|
|
{
|
|
get { return _MyContentByte; }
|
|
set { _MyContentByte = value; }
|
|
}
|
|
private VlnSvgPageHelper _MyPageHelper;
|
|
public VlnSvgPageHelper MyPageHelper
|
|
{
|
|
get
|
|
{
|
|
if(_MyPageHelper == null)
|
|
_MyPageHelper = MyContentByte.PdfWriter.PageEvent as VlnSvgPageHelper;
|
|
return _MyPageHelper;
|
|
}
|
|
set { _MyPageHelper = value; }
|
|
}
|
|
public vlnPrintObject()
|
|
{
|
|
}
|
|
/// <summary>
|
|
/// No conversion necessary: twips -> twips
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public static int ToPrint(int value)
|
|
{
|
|
return value;
|
|
}
|
|
/// <summary>
|
|
/// No conversion necessary: int? twips -> int twips
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public static int ToPrint(int? value)
|
|
{
|
|
return ToPrint((int)value);
|
|
}
|
|
/// <summary>
|
|
/// No conversion necessary: string twips -> int twips
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public static int ToPrint(string value)
|
|
{
|
|
return ToPrint(Convert.ToInt32(value));
|
|
}
|
|
/// <summary>
|
|
/// No conversion necessary: value from a list in a string, twips -> int twips
|
|
/// </summary>
|
|
/// <param name="value"></param>
|
|
/// <returns></returns>
|
|
public static int ToPrint(string value, int i)
|
|
{
|
|
string s = value.Split(",".ToCharArray())[i];
|
|
return ToPrint(s);
|
|
}
|
|
public float AdjustToCharPosition(float position)
|
|
{
|
|
int iPosition = (int)position;
|
|
iPosition = (iPosition / (int)_CharsToTwips) * (int)_CharsToTwips;
|
|
return (float)iPosition;
|
|
}
|
|
}
|
|
public partial class vlnPrintObjects : List<vlnPrintObject>
|
|
{
|
|
};
|
|
public enum PartLocation : int
|
|
{
|
|
None = 0, // Non-printable
|
|
Below = 1, // RNO Separator
|
|
Above = 2, // Tab Headers, Separator?
|
|
Right = 3, // Change Bars
|
|
Left = 4, // Tabs, Checkoffs? (maybe part of tab)
|
|
Container = 5 // Box
|
|
};
|
|
public partial class vlnParagraphs : List<vlnParagraph>
|
|
{
|
|
private vlnParagraph _Parent;
|
|
public vlnParagraph Parent
|
|
{
|
|
get { return _Parent; }
|
|
set { _Parent = value; }
|
|
}
|
|
public vlnParagraphs(vlnParagraph parent)
|
|
{
|
|
_Parent = parent;
|
|
}
|
|
};
|
|
public partial class vlnParagraph : vlnPrintObject
|
|
{
|
|
private static string _Prefix = "";
|
|
public static string Prefix
|
|
{
|
|
get { return vlnParagraph._Prefix; }
|
|
set { vlnParagraph._Prefix = value; }
|
|
}
|
|
protected float _YBottomMost; // Bottom of the paragraph including the children
|
|
public float YBottomMost
|
|
{
|
|
get { return _YBottomMost; }
|
|
set { _YBottomMost = value; }
|
|
}
|
|
protected float _YTopMost; // Top of the paragraph including the children
|
|
public float YTopMost
|
|
{
|
|
get { return _YTopMost; }
|
|
set { _YTopMost = value; }
|
|
}
|
|
protected float _YTop; // Top of the paragraph including parts
|
|
public float YTop
|
|
{
|
|
get { return _YTop; }
|
|
set { _YTop = value; }
|
|
}
|
|
public float YSize // How big this paragraph is with all of its children
|
|
{
|
|
get { return _YBottomMost - _YTopMost; }
|
|
}
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set { _MyItemInfo = value; }
|
|
}
|
|
private vlnParagraph _MyHighLevelParagraph;
|
|
|
|
public vlnParagraph MyHighLevelParagraph
|
|
{
|
|
get
|
|
{
|
|
if (_MyHighLevelParagraph == null)
|
|
{
|
|
_MyHighLevelParagraph = GetHighLevelParagraph();
|
|
}
|
|
return _MyHighLevelParagraph;
|
|
}
|
|
}
|
|
private vlnParagraph GetHighLevelParagraph()
|
|
{
|
|
if (MyItemInfo.IsHigh) return this;
|
|
return MyParent.GetHighLevelParagraph();
|
|
}
|
|
// Tab, Separator, ChangeBar, Box, Circle, Checkoff
|
|
private vlnPrintObjects _PartsAbove;
|
|
public vlnPrintObjects PartsAbove
|
|
{
|
|
get
|
|
{
|
|
if (_PartsAbove == null) _PartsAbove = new vlnPrintObjects();
|
|
return _PartsAbove;
|
|
}
|
|
}
|
|
private vlnPrintObjects _PartsBelow;
|
|
public vlnPrintObjects PartsBelow
|
|
{
|
|
get
|
|
{
|
|
if (_PartsBelow == null) _PartsBelow = new vlnPrintObjects();
|
|
return _PartsBelow;
|
|
}
|
|
}
|
|
private vlnPrintObjects _PartsRight;
|
|
public vlnPrintObjects PartsRight
|
|
{
|
|
get
|
|
{
|
|
if (_PartsRight == null) _PartsRight = new vlnPrintObjects();
|
|
return _PartsRight;
|
|
}
|
|
}
|
|
private vlnPrintObjects _PartsLeft;
|
|
public vlnPrintObjects PartsLeft
|
|
{
|
|
get
|
|
{
|
|
if (_PartsLeft == null) _PartsLeft = new vlnPrintObjects();
|
|
return _PartsLeft;
|
|
}
|
|
}
|
|
private vlnPrintObjects _PartsContainer;
|
|
public vlnPrintObjects PartsContainer
|
|
{
|
|
get
|
|
{
|
|
if (_PartsContainer == null) _PartsContainer = new vlnPrintObjects();
|
|
return _PartsContainer;
|
|
}
|
|
}
|
|
private vlnParagraphs _ChildrenAbove;
|
|
public vlnParagraphs ChildrenAbove
|
|
{
|
|
get
|
|
{
|
|
if (_ChildrenAbove == null) _ChildrenAbove = new vlnParagraphs(this);
|
|
return _ChildrenAbove;
|
|
}
|
|
}
|
|
private vlnParagraphs _ChildrenBelow;
|
|
public vlnParagraphs ChildrenBelow
|
|
{
|
|
get
|
|
{
|
|
if (_ChildrenBelow == null) _ChildrenBelow = new vlnParagraphs(this);
|
|
return _ChildrenBelow;
|
|
}
|
|
}
|
|
private vlnParagraphs _ChildrenRight;
|
|
public vlnParagraphs ChildrenRight
|
|
{
|
|
get
|
|
{
|
|
if (_ChildrenRight == null) _ChildrenRight = new vlnParagraphs(this);
|
|
return _ChildrenRight;
|
|
}
|
|
}
|
|
private vlnParagraphs _ChildrenLeft;
|
|
public vlnParagraphs ChildrenLeft
|
|
{
|
|
get
|
|
{
|
|
if (_ChildrenLeft == null) _ChildrenLeft = new vlnParagraphs(this);
|
|
return _ChildrenLeft;
|
|
}
|
|
}
|
|
//public vlnParagraph(vlnParagraph myParent, ItemInfo myItemInfo, float xoff, float yoff, float width, string rtf, ChildLocation childRelation)
|
|
//{
|
|
// MyParent = myParent;
|
|
// MyItemInfo = myItemInfo;
|
|
// XOffset = xoff;
|
|
// YOffset = yoff;
|
|
// Width = width;
|
|
// Rtf = rtf;
|
|
// switch (childRelation)
|
|
// {
|
|
// case ChildLocation.Below:
|
|
// myParent.ChildrenBelow.Add(this);
|
|
// break;
|
|
// case ChildLocation.Above:
|
|
// myParent.ChildrenAbove.Add(this);
|
|
// break;
|
|
// case ChildLocation.Right:
|
|
// myParent.ChildrenRight.Add(this);
|
|
// break;
|
|
// }
|
|
//}
|
|
|
|
public void AdjustXOffsetForTab(ItemInfo itemInfo, int maxRNO, FormatInfo formatInfo, vlnTab myTab)
|
|
{
|
|
float tabWidth = (myTab == null) ? 0 : myTab.TabWidth;
|
|
int typ = ((int)itemInfo.MyContent.Type) % 10000;
|
|
int? bxIndx = formatInfo.PlantFormat.FormatData.StepDataList[typ].StepLayoutData.STBoxindex;
|
|
if (bxIndx != null)
|
|
{
|
|
Box bx = formatInfo.PlantFormat.FormatData.BoxList[(int)bxIndx];
|
|
//XOffset += (float)bx.TxtStart + tabWidth + XOffsetBox;
|
|
XOffset = (float)itemInfo.MyDocStyle.Layout.LeftMargin + (float)bx.TxtStart + tabWidth + XOffsetBox;
|
|
if (myTab!=null)myTab.XOffset = XOffset - tabWidth;
|
|
}
|
|
else if (itemInfo.IsHigh)
|
|
{
|
|
XOffset += tabWidth - myTab.TabAlign;
|
|
if (myTab != null) myTab.XOffset += tabWidth - myTab.TabAlign;
|
|
}
|
|
else if (itemInfo.IsRNOPart && !((ItemInfo)itemInfo.ActiveParent).IsHigh)
|
|
{
|
|
// don't adjust for rno
|
|
}
|
|
else if (MyParent != null)
|
|
{
|
|
XOffset += tabWidth - (myTab == null ? 0 : myTab.TabAlign);
|
|
if (myTab != null) myTab.XOffset += tabWidth - myTab.TabAlign;
|
|
}
|
|
}
|
|
public void AdjustWidth(ItemInfo itemInfo, int maxRNO, FormatInfo formatInfo, vlnTab myTab)
|
|
{
|
|
float tabWidth = (myTab == null) ? 0 : myTab.TabWidth;
|
|
int typ = ((int)itemInfo.MyContent.Type) % 10000;
|
|
int? bxIndx = formatInfo.PlantFormat.FormatData.StepDataList[typ].StepLayoutData.STBoxindex;
|
|
if (bxIndx != null)
|
|
{
|
|
Box bx = formatInfo.PlantFormat.FormatData.BoxList[(int)bxIndx];
|
|
Width = _WidthAdjustBox + (float)bx.TxtWidth - tabWidth;
|
|
}
|
|
else if (itemInfo.IsHigh)
|
|
{
|
|
Width = _WidthAdjust + ToPrint(formatInfo.PlantFormat.FormatData.SectData.StepSectionData.StepSectionLayoutData.WidSTablePrint, maxRNO);
|
|
}
|
|
else if (MyParent == null)
|
|
{
|
|
// 72 points / inch - 7 inches (about width of page)
|
|
Width = 72 * 7;
|
|
}
|
|
else if (itemInfo.IsRNOPart && !((ItemInfo)itemInfo.ActiveParent).IsHigh)
|
|
{
|
|
Width = MyParent.Width;
|
|
}
|
|
else if (itemInfo.IsTablePart)
|
|
{
|
|
Width = 72*7; // TODO: Need to determine the Width of the Table based upon the contents
|
|
}
|
|
else
|
|
{
|
|
Width = MyParent.Width - tabWidth + (myTab == null ? 0 : myTab.TabAlign);
|
|
}
|
|
}
|
|
}
|
|
public enum ChildLocation : int
|
|
{
|
|
None = 0,
|
|
Below = 1,
|
|
Above = 2,
|
|
Right = 3, // RNO
|
|
Left = 4
|
|
}
|
|
public partial class vlnTab : vlnPrintObject
|
|
{
|
|
public float TabWidth
|
|
{
|
|
get { return _CharsToTwips * TabText.Length; }
|
|
}
|
|
private float? _TabAlign;
|
|
/// <summary>
|
|
/// Used to Align Tabs for numeric tabs that can go to 2 digits
|
|
/// </summary>
|
|
public float TabAlign // Offset to Last printable character
|
|
{
|
|
get
|
|
{
|
|
if (_TabAlign == null)
|
|
{
|
|
_TabAlign = 0;
|
|
if (_TabText != null)
|
|
{
|
|
while (_TabText[(int)_TabAlign] == ' ')
|
|
_TabAlign++;
|
|
if ("0123456789".Contains(_TabText[(int)_TabAlign].ToString()))
|
|
{
|
|
while ("0123456789".Contains(_TabText[(int)_TabAlign].ToString()))
|
|
_TabAlign++;
|
|
_TabAlign--;
|
|
}
|
|
}
|
|
}
|
|
return (float)_TabAlign * _CharsToTwips;
|
|
}
|
|
}
|
|
private float? _TabOffset;
|
|
public float TabOffset // Offset to first printable character
|
|
{
|
|
get
|
|
{
|
|
if (_TabOffset == null)
|
|
{
|
|
_TabOffset = 0;
|
|
if(_TabText != null)
|
|
while(_TabText[(int)_TabOffset]==' ')
|
|
_TabOffset ++;
|
|
}
|
|
return (float)_TabOffset * _CharsToTwips;
|
|
}
|
|
}
|
|
private string _TabText;
|
|
public string TabText
|
|
{
|
|
get { return _TabText; }
|
|
set { _TabText = value; }
|
|
}
|
|
private VE_Font _MyFont;
|
|
public VE_Font MyFont
|
|
{
|
|
get { return _MyFont; }
|
|
set { _MyFont = value; }
|
|
}
|
|
}
|
|
public partial class vlnHeader : vlnPrintObject
|
|
{
|
|
public float HeaderWidth
|
|
{
|
|
get { return _WidthAdjust + (_CharsToTwips * HeaderText.Length); }
|
|
}
|
|
private string _HeaderText;
|
|
public string HeaderText
|
|
{
|
|
get { return _HeaderText; }
|
|
set { _HeaderText = value; }
|
|
}
|
|
private VE_Font _MyFont;
|
|
public VE_Font MyFont
|
|
{
|
|
get { return _MyFont; }
|
|
set { _MyFont = value; }
|
|
}
|
|
private ContentAlignment _ContentAlignment;
|
|
public ContentAlignment ContentAlignment
|
|
{
|
|
get { return _ContentAlignment; }
|
|
set { _ContentAlignment = value; }
|
|
}
|
|
}
|
|
public partial class vlnBox : vlnPrintObject
|
|
{
|
|
private int _LineType; /* how to represent?? */
|
|
private System.Drawing.Color _Color;
|
|
public System.Drawing.Color Color
|
|
{
|
|
get { return _Color; }
|
|
set { _Color = value; }
|
|
}
|
|
public vlnBox(vlnParagraph paragraph/*, FormatBox box*/)
|
|
{
|
|
}
|
|
}
|
|
public partial class vlnChangeBar : vlnPrintObject
|
|
{
|
|
public vlnChangeBar(vlnParagraph parent, float xoff, float yoff)
|
|
{
|
|
_XOffset = xoff;
|
|
_YOffset = yoff;
|
|
_MyParent = parent;
|
|
}
|
|
}
|
|
public partial class vlnRNOSeparator : vlnPrintObject
|
|
{
|
|
private VE_Font _MyFont;
|
|
public VE_Font MyFont
|
|
{
|
|
get { return _MyFont; }
|
|
set { _MyFont = value; }
|
|
}
|
|
}
|
|
public partial class vlnMacro : vlnPrintObject
|
|
{
|
|
private string _MacroDef;
|
|
public string MacroDef
|
|
{
|
|
get { return _MacroDef; }
|
|
set { _MacroDef = value; }
|
|
}
|
|
}
|
|
}
|