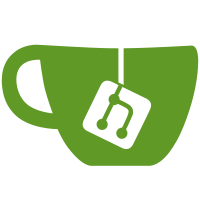
Added administrative tools of Identifying Disconnected Items and NonEditable Items Removed administrative tools CSLA methods since they were moved to AdminToolsExt. Added new module to handle administrative tools CSLA methods Added methods for administrative tools of Identifying Disconnected Items and NonEditable Items
96 lines
2.4 KiB
C#
96 lines
2.4 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using Csla.Validation;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class Pdf
|
|
{
|
|
public static void DeleteAll(int docID)
|
|
{
|
|
if (!CanDeleteObject())
|
|
throw new System.Security.SecurityException("User not authorized to remove a Pdf");
|
|
try
|
|
{
|
|
DataPortal.Delete(new DocIDCriteria(docID));
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Pdf.Delete", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
protected class DocIDCriteria
|
|
{
|
|
private int _DocID;
|
|
public int DocID
|
|
{ get { return _DocID; } }
|
|
public DocIDCriteria(int docID)
|
|
{
|
|
_DocID = docID;
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
private void DataPortal_Delete(DocIDCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Pdf.DataPortal_Delete", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "deleteAllPdfs";
|
|
cm.Parameters.AddWithValue("@DocID", criteria.DocID);
|
|
cm.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Pdf.DataPortal_Delete", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Pdf.DataPortal_Delete", ex);
|
|
}
|
|
}
|
|
|
|
}
|
|
public partial class PdfInfo
|
|
{
|
|
public static PdfInfo Get(ItemInfo sect)
|
|
{
|
|
int count = 0;
|
|
while (count < 2)
|
|
{
|
|
DocStyle myDocStyle = sect.ActiveSection.MyDocStyle;
|
|
SectionConfig sc = sect.ActiveSection.MyConfig as SectionConfig;
|
|
PdfInfo myPdf = null;
|
|
int ss = sect.MyDocVersion.DocVersionConfig.SelectedSlave;
|
|
if (sc != null && sc.Section_WordMargin == "Y")
|
|
{
|
|
myPdf = Get(sect.MyContent.MyEntry.DocID, ss*10 + MSWordToPDF.DebugStatus, 0, 0, 0, 0);
|
|
}
|
|
else
|
|
{
|
|
myPdf = Get(sect.MyContent.MyEntry.DocID, ss*10 + MSWordToPDF.DebugStatus, (int)myDocStyle.Layout.TopMargin, (int)myDocStyle.Layout.PageLength,
|
|
(int)myDocStyle.Layout.LeftMargin, (int)myDocStyle.Layout.PageWidth);
|
|
}
|
|
if (myPdf != null) return myPdf;
|
|
if (count > 0) return null; // Could not find or create a pdf
|
|
MSWordToPDF.SetDocPdf(sect.MyContent.MyEntry.MyDocument, sect);
|
|
count++;
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
public partial class PdfInfoList
|
|
{
|
|
}
|
|
}
|