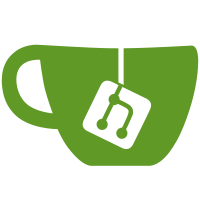
Changed logic so that VE-PROMS source code can be located in either 16Bit or 16-Bit folders This strips prerequisite step references from procedure text when printing Recursive transitions cannot be added to procedure text. If you try to add a transition to a step that contains the text of the step, you will be told that this is not permitted. Default Change IDs were not being properly updated. Added footer line to the debug rulers when creating a PDF. Removed Prerequisite Step references in the PDF bookmarks
690 lines
25 KiB
C#
690 lines
25 KiB
C#
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.IO;
|
|
|
|
namespace fmtxml
|
|
{
|
|
/// <summary>
|
|
/// Summary description for Form1.
|
|
/// </summary>
|
|
public class Form1 : System.Windows.Forms.Form
|
|
{
|
|
private System.Windows.Forms.Button btnCvtPagDoc;
|
|
private System.Windows.Forms.Label label1;
|
|
private System.Windows.Forms.Button btnExit;
|
|
private System.Windows.Forms.ListBox listBox1;
|
|
private System.Windows.Forms.Button btnCvtGenmac;
|
|
private System.Windows.Forms.Button btnCvtXmlToSVG;
|
|
//private C1.C1Pdf.C1PdfDocument c1PdfDocument1;
|
|
private Button btnCvtFormat;
|
|
private Button btnMergeFmtPagDoc;
|
|
private Label lblPathFmt;
|
|
private Button btnBrowseFmt;
|
|
private TextBox tbFmtPath;
|
|
private TextBox tbGenmacPath;
|
|
private Button btnBrowseGenmac;
|
|
private Label lblPathGenmac;
|
|
private Label lblResult;
|
|
private TextBox tbResultPath;
|
|
private Button btnBrowseResult;
|
|
private StatusStrip statusStrip1;
|
|
private ToolStripStatusLabel tsslStatus;
|
|
private Button btnRtfToSvg;
|
|
private Button btnWestinghouse;
|
|
/// <summary>
|
|
/// Required designer variable.
|
|
/// </summary>
|
|
private System.ComponentModel.Container components = null;
|
|
|
|
public Form1()
|
|
{
|
|
//
|
|
// Required for Windows Form Designer support
|
|
//
|
|
InitializeComponent();
|
|
if (Directory.Exists(@"c:\16bit\ve-proms\format"))
|
|
tbFmtPath.Text = @"c:\16bit\ve-proms\format";
|
|
else if (Directory.Exists(@"c:\16-bit\ve-proms\format"))
|
|
tbFmtPath.Text = @"c:\16-bit\ve-proms\format";
|
|
else
|
|
MessageBox.Show("Specify Path for format files.", "Cannot find 16 Bit Formats", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
tbGenmacPath.Text = @"C:\16bit\promsnt\genmac";
|
|
tbResultPath.Text = @"c:\development\";
|
|
//
|
|
// TODO: Add any constructor code after InitializeComponent call
|
|
//
|
|
}
|
|
public string MyStatus
|
|
{
|
|
get { return tsslStatus.Text; }
|
|
set { tsslStatus.Text = value; Application.DoEvents(); }
|
|
}
|
|
|
|
/// <summary>
|
|
/// Clean up any resources being used.
|
|
/// </summary>
|
|
protected override void Dispose( bool disposing )
|
|
{
|
|
if( disposing )
|
|
{
|
|
if (components != null)
|
|
{
|
|
components.Dispose();
|
|
}
|
|
}
|
|
base.Dispose( disposing );
|
|
}
|
|
|
|
#region Windows Form Designer generated code
|
|
/// <summary>
|
|
/// Required method for Designer support - do not modify
|
|
/// the contents of this method with the code editor.
|
|
/// </summary>
|
|
private void InitializeComponent()
|
|
{
|
|
this.btnCvtPagDoc = new System.Windows.Forms.Button();
|
|
this.label1 = new System.Windows.Forms.Label();
|
|
this.btnExit = new System.Windows.Forms.Button();
|
|
this.listBox1 = new System.Windows.Forms.ListBox();
|
|
this.btnCvtGenmac = new System.Windows.Forms.Button();
|
|
this.btnCvtXmlToSVG = new System.Windows.Forms.Button();
|
|
this.btnCvtFormat = new System.Windows.Forms.Button();
|
|
this.btnMergeFmtPagDoc = new System.Windows.Forms.Button();
|
|
this.lblPathFmt = new System.Windows.Forms.Label();
|
|
this.btnBrowseFmt = new System.Windows.Forms.Button();
|
|
this.tbFmtPath = new System.Windows.Forms.TextBox();
|
|
this.tbGenmacPath = new System.Windows.Forms.TextBox();
|
|
this.btnBrowseGenmac = new System.Windows.Forms.Button();
|
|
this.lblPathGenmac = new System.Windows.Forms.Label();
|
|
this.lblResult = new System.Windows.Forms.Label();
|
|
this.tbResultPath = new System.Windows.Forms.TextBox();
|
|
this.btnBrowseResult = new System.Windows.Forms.Button();
|
|
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
|
|
this.tsslStatus = new System.Windows.Forms.ToolStripStatusLabel();
|
|
this.btnRtfToSvg = new System.Windows.Forms.Button();
|
|
this.btnWestinghouse = new System.Windows.Forms.Button();
|
|
this.statusStrip1.SuspendLayout();
|
|
this.SuspendLayout();
|
|
//
|
|
// btnCvtPagDoc
|
|
//
|
|
this.btnCvtPagDoc.Location = new System.Drawing.Point(217, 63);
|
|
this.btnCvtPagDoc.Name = "btnCvtPagDoc";
|
|
this.btnCvtPagDoc.Size = new System.Drawing.Size(201, 51);
|
|
this.btnCvtPagDoc.TabIndex = 0;
|
|
this.btnCvtPagDoc.Text = "Convert Page and Doc Styles in format directory (Step 2)";
|
|
this.btnCvtPagDoc.Click += new System.EventHandler(this.btnCvtPagDoc_Click);
|
|
//
|
|
// label1
|
|
//
|
|
this.label1.Location = new System.Drawing.Point(12, 237);
|
|
this.label1.Name = "label1";
|
|
this.label1.Size = new System.Drawing.Size(200, 18);
|
|
this.label1.TabIndex = 1;
|
|
this.label1.Text = "Converted:";
|
|
//
|
|
// btnExit
|
|
//
|
|
this.btnExit.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnExit.Location = new System.Drawing.Point(560, 224);
|
|
this.btnExit.Name = "btnExit";
|
|
this.btnExit.Size = new System.Drawing.Size(63, 28);
|
|
this.btnExit.TabIndex = 2;
|
|
this.btnExit.Text = "Exit";
|
|
this.btnExit.Click += new System.EventHandler(this.btnExit_Click);
|
|
//
|
|
// listBox1
|
|
//
|
|
this.listBox1.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
|
|
| System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.listBox1.ItemHeight = 16;
|
|
this.listBox1.Location = new System.Drawing.Point(10, 258);
|
|
this.listBox1.Name = "listBox1";
|
|
this.listBox1.Size = new System.Drawing.Size(613, 212);
|
|
this.listBox1.TabIndex = 3;
|
|
//
|
|
// btnCvtGenmac
|
|
//
|
|
this.btnCvtGenmac.Enabled = false;
|
|
this.btnCvtGenmac.Location = new System.Drawing.Point(10, 172);
|
|
this.btnCvtGenmac.Name = "btnCvtGenmac";
|
|
this.btnCvtGenmac.Size = new System.Drawing.Size(183, 46);
|
|
this.btnCvtGenmac.TabIndex = 4;
|
|
this.btnCvtGenmac.Text = "Convert genmac files to XML (Genmac Step 1)";
|
|
this.btnCvtGenmac.Click += new System.EventHandler(this.btnCvtGenmac_Click);
|
|
//
|
|
// btnCvtXmlToSVG
|
|
//
|
|
this.btnCvtXmlToSVG.Enabled = false;
|
|
this.btnCvtXmlToSVG.Location = new System.Drawing.Point(199, 170);
|
|
this.btnCvtXmlToSVG.Name = "btnCvtXmlToSVG";
|
|
this.btnCvtXmlToSVG.Size = new System.Drawing.Size(183, 50);
|
|
this.btnCvtXmlToSVG.TabIndex = 5;
|
|
this.btnCvtXmlToSVG.Text = "Convert Genmac_XML to SVG (Genmac Step 2)";
|
|
this.btnCvtXmlToSVG.Click += new System.EventHandler(this.btnCvtXmlToSVG_Click);
|
|
//
|
|
// btnCvtFormat
|
|
//
|
|
this.btnCvtFormat.Location = new System.Drawing.Point(10, 63);
|
|
this.btnCvtFormat.Name = "btnCvtFormat";
|
|
this.btnCvtFormat.Size = new System.Drawing.Size(201, 51);
|
|
this.btnCvtFormat.TabIndex = 8;
|
|
this.btnCvtFormat.Text = "Convert Format in format directory (Step 1)";
|
|
this.btnCvtFormat.UseVisualStyleBackColor = true;
|
|
this.btnCvtFormat.Click += new System.EventHandler(this.btnCvtFormat_Click);
|
|
//
|
|
// btnMergeFmtPagDoc
|
|
//
|
|
this.btnMergeFmtPagDoc.Location = new System.Drawing.Point(424, 63);
|
|
this.btnMergeFmtPagDoc.Name = "btnMergeFmtPagDoc";
|
|
this.btnMergeFmtPagDoc.Size = new System.Drawing.Size(201, 51);
|
|
this.btnMergeFmtPagDoc.TabIndex = 9;
|
|
this.btnMergeFmtPagDoc.Text = "Integrate Fmt, Doc & Pag to single xml (Step 3)";
|
|
this.btnMergeFmtPagDoc.UseVisualStyleBackColor = true;
|
|
this.btnMergeFmtPagDoc.Click += new System.EventHandler(this.btnMergeFmtPagDoc_Click);
|
|
//
|
|
// lblPathFmt
|
|
//
|
|
this.lblPathFmt.AutoSize = true;
|
|
this.lblPathFmt.Location = new System.Drawing.Point(7, 42);
|
|
this.lblPathFmt.Name = "lblPathFmt";
|
|
this.lblPathFmt.Size = new System.Drawing.Size(139, 17);
|
|
this.lblPathFmt.TabIndex = 10;
|
|
this.lblPathFmt.Text = "Path To Format Files";
|
|
//
|
|
// btnBrowseFmt
|
|
//
|
|
this.btnBrowseFmt.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseFmt.Location = new System.Drawing.Point(476, 36);
|
|
this.btnBrowseFmt.Name = "btnBrowseFmt";
|
|
this.btnBrowseFmt.Size = new System.Drawing.Size(153, 23);
|
|
this.btnBrowseFmt.TabIndex = 11;
|
|
this.btnBrowseFmt.Text = "Browse (Format)";
|
|
this.btnBrowseFmt.UseVisualStyleBackColor = true;
|
|
this.btnBrowseFmt.Click += new System.EventHandler(this.btnBrowseFmt_Click);
|
|
//
|
|
// tbFmtPath
|
|
//
|
|
this.tbFmtPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbFmtPath.Location = new System.Drawing.Point(155, 37);
|
|
this.tbFmtPath.Name = "tbFmtPath";
|
|
this.tbFmtPath.Size = new System.Drawing.Size(303, 22);
|
|
this.tbFmtPath.TabIndex = 12;
|
|
//
|
|
// tbGenmacPath
|
|
//
|
|
this.tbGenmacPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbGenmacPath.Enabled = false;
|
|
this.tbGenmacPath.Location = new System.Drawing.Point(155, 144);
|
|
this.tbGenmacPath.Name = "tbGenmacPath";
|
|
this.tbGenmacPath.Size = new System.Drawing.Size(303, 22);
|
|
this.tbGenmacPath.TabIndex = 15;
|
|
//
|
|
// btnBrowseGenmac
|
|
//
|
|
this.btnBrowseGenmac.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseGenmac.Enabled = false;
|
|
this.btnBrowseGenmac.Location = new System.Drawing.Point(476, 143);
|
|
this.btnBrowseGenmac.Name = "btnBrowseGenmac";
|
|
this.btnBrowseGenmac.Size = new System.Drawing.Size(153, 23);
|
|
this.btnBrowseGenmac.TabIndex = 14;
|
|
this.btnBrowseGenmac.Text = "Browse (Genmac)";
|
|
this.btnBrowseGenmac.UseVisualStyleBackColor = true;
|
|
this.btnBrowseGenmac.Click += new System.EventHandler(this.btnBrowseGenmac_Click);
|
|
//
|
|
// lblPathGenmac
|
|
//
|
|
this.lblPathGenmac.AutoSize = true;
|
|
this.lblPathGenmac.Enabled = false;
|
|
this.lblPathGenmac.Location = new System.Drawing.Point(7, 149);
|
|
this.lblPathGenmac.Name = "lblPathGenmac";
|
|
this.lblPathGenmac.Size = new System.Drawing.Size(148, 17);
|
|
this.lblPathGenmac.TabIndex = 13;
|
|
this.lblPathGenmac.Text = "Path To Genmac Files";
|
|
//
|
|
// lblResult
|
|
//
|
|
this.lblResult.AutoSize = true;
|
|
this.lblResult.Location = new System.Drawing.Point(16, 10);
|
|
this.lblResult.Name = "lblResult";
|
|
this.lblResult.Size = new System.Drawing.Size(163, 17);
|
|
this.lblResult.TabIndex = 16;
|
|
this.lblResult.Text = "Path To Result Directory";
|
|
//
|
|
// tbResultPath
|
|
//
|
|
this.tbResultPath.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
|
|
| System.Windows.Forms.AnchorStyles.Right)));
|
|
this.tbResultPath.Location = new System.Drawing.Point(185, 8);
|
|
this.tbResultPath.Name = "tbResultPath";
|
|
this.tbResultPath.Size = new System.Drawing.Size(273, 22);
|
|
this.tbResultPath.TabIndex = 17;
|
|
//
|
|
// btnBrowseResult
|
|
//
|
|
this.btnBrowseResult.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
|
|
this.btnBrowseResult.Location = new System.Drawing.Point(476, 3);
|
|
this.btnBrowseResult.Name = "btnBrowseResult";
|
|
this.btnBrowseResult.Size = new System.Drawing.Size(153, 24);
|
|
this.btnBrowseResult.TabIndex = 18;
|
|
this.btnBrowseResult.Text = "Browse (Result)";
|
|
this.btnBrowseResult.UseVisualStyleBackColor = true;
|
|
this.btnBrowseResult.Click += new System.EventHandler(this.btnBrowseResult_Click);
|
|
//
|
|
// statusStrip1
|
|
//
|
|
this.statusStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
|
|
this.tsslStatus});
|
|
this.statusStrip1.Location = new System.Drawing.Point(0, 545);
|
|
this.statusStrip1.Name = "statusStrip1";
|
|
this.statusStrip1.Size = new System.Drawing.Size(631, 25);
|
|
this.statusStrip1.TabIndex = 19;
|
|
this.statusStrip1.Text = "statusStrip1";
|
|
//
|
|
// tsslStatus
|
|
//
|
|
this.tsslStatus.Name = "tsslStatus";
|
|
this.tsslStatus.Size = new System.Drawing.Size(50, 20);
|
|
this.tsslStatus.Text = "Ready";
|
|
//
|
|
// btnRtfToSvg
|
|
//
|
|
this.btnRtfToSvg.Location = new System.Drawing.Point(424, 167);
|
|
this.btnRtfToSvg.Name = "btnRtfToSvg";
|
|
this.btnRtfToSvg.Size = new System.Drawing.Size(182, 51);
|
|
this.btnRtfToSvg.TabIndex = 20;
|
|
this.btnRtfToSvg.Text = "Convert Genmac RTF to SVG (GenMac One Step)";
|
|
this.btnRtfToSvg.Click += new System.EventHandler(this.btnRtfToSvg_Click);
|
|
//
|
|
// btnWestinghouse
|
|
//
|
|
this.btnWestinghouse.Location = new System.Drawing.Point(387, 224);
|
|
this.btnWestinghouse.Name = "btnWestinghouse";
|
|
this.btnWestinghouse.Size = new System.Drawing.Size(164, 26);
|
|
this.btnWestinghouse.TabIndex = 21;
|
|
this.btnWestinghouse.Text = "Copy New Formats";
|
|
this.btnWestinghouse.UseVisualStyleBackColor = true;
|
|
this.btnWestinghouse.Click += new System.EventHandler(this.btnWestinghouse_Click);
|
|
//
|
|
// Form1
|
|
//
|
|
this.AutoScaleBaseSize = new System.Drawing.Size(6, 15);
|
|
this.ClientSize = new System.Drawing.Size(631, 570);
|
|
this.Controls.Add(this.btnWestinghouse);
|
|
this.Controls.Add(this.btnRtfToSvg);
|
|
this.Controls.Add(this.statusStrip1);
|
|
this.Controls.Add(this.btnBrowseResult);
|
|
this.Controls.Add(this.tbResultPath);
|
|
this.Controls.Add(this.lblResult);
|
|
this.Controls.Add(this.tbGenmacPath);
|
|
this.Controls.Add(this.btnBrowseGenmac);
|
|
this.Controls.Add(this.lblPathGenmac);
|
|
this.Controls.Add(this.tbFmtPath);
|
|
this.Controls.Add(this.btnBrowseFmt);
|
|
this.Controls.Add(this.lblPathFmt);
|
|
this.Controls.Add(this.btnMergeFmtPagDoc);
|
|
this.Controls.Add(this.btnCvtFormat);
|
|
this.Controls.Add(this.btnCvtXmlToSVG);
|
|
this.Controls.Add(this.btnCvtGenmac);
|
|
this.Controls.Add(this.listBox1);
|
|
this.Controls.Add(this.btnExit);
|
|
this.Controls.Add(this.label1);
|
|
this.Controls.Add(this.btnCvtPagDoc);
|
|
this.Name = "Form1";
|
|
this.Text = "Form1";
|
|
this.statusStrip1.ResumeLayout(false);
|
|
this.statusStrip1.PerformLayout();
|
|
this.ResumeLayout(false);
|
|
this.PerformLayout();
|
|
|
|
}
|
|
#endregion
|
|
|
|
/// <summary>
|
|
/// The main entry point for the application.
|
|
/// </summary>
|
|
[STAThread]
|
|
static void Main()
|
|
{
|
|
Application.Run(new Form1());
|
|
}
|
|
private static string[] _ExcludeList = "ANO,ATA,ATLAS,LPL,MYA,NAE,NAI,NAS,PAC,RBN,ROB,SCE,SRS,VP,WPF,YAE".Split(",".ToCharArray());
|
|
public static bool Excluded(FileInfo fi)
|
|
{
|
|
foreach (string prefix in _ExcludeList)
|
|
if (fi.Name.ToUpper().StartsWith(prefix)) return true;
|
|
return false;
|
|
}
|
|
|
|
private void btnCvtPagDoc_Click(object sender, System.EventArgs e)
|
|
{
|
|
CleanupDestinationFolder("*p.xml");
|
|
CleanupDestinationFolder("*d.xml");
|
|
// get all of the files in the e:\ve-proms\format directory.
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text); // "C:\\16bit\\ve-proms\\format");
|
|
FileInfo[] fis = di.GetFiles("*.pag");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
string fn = fi.Name.Substring(0, fi.Name.Length - 4);
|
|
AddFileToListBox(fi);
|
|
FmtToXml fx = new FmtToXml(fn, tbFmtPath.Text);
|
|
}
|
|
}
|
|
fis = di.GetFiles("*.z*");
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
AddFileToListBox(fi);
|
|
FmtToXml fx = new FmtToXml(fi.Name, tbFmtPath.Text);
|
|
}
|
|
}
|
|
MessageBox.Show("DONE Converting Page and Document Styles to XML");
|
|
}
|
|
|
|
private void AddFileToListBox(FileInfo fi)
|
|
{
|
|
this.listBox1.Items.Add(fi.Name);
|
|
this.listBox1.SelectedIndex = this.listBox1.Items.Count - 1;
|
|
MyStatus = "Processing " + fi.Name;
|
|
Application.DoEvents();
|
|
}
|
|
|
|
private void ClearListBox()
|
|
{
|
|
listBox1.Items.Clear();
|
|
}
|
|
|
|
private void btnExit_Click(object sender, System.EventArgs e)
|
|
{
|
|
Application.Exit();
|
|
}
|
|
|
|
private void btnCvtGenmac_Click(object sender, System.EventArgs e)
|
|
{
|
|
// before doing the genmac change, use cpp -DRTF file after genmac.h
|
|
// has been emptied out on files in \promsnt\genmac. This creates
|
|
// precompiled files only, i.e. gets rid of some of the junk in the files.
|
|
// This conversion is done 1 time. After 32-bit print is done, the xml
|
|
// files will be used rather than the genmac.
|
|
|
|
DirectoryInfo di = new DirectoryInfo(tbGenmacPath.Text + @"\preproc"); // "C:\\16bit\\promsnt\\genmac\\preproc");
|
|
FileInfo[] fis = di.GetFiles("*.i");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// results go to e:\\proms.net\\genmac.xml\\convert
|
|
if (!Excluded(fi))
|
|
{
|
|
AddFileToListBox(fi);
|
|
GenToXml gn = new GenToXml(fi.Name, tbGenmacPath.Text, tbResultPath.Text);
|
|
}
|
|
}
|
|
MessageBox.Show("DONE Converting genmacs to XML - Results are in " + tbResultPath.Text + @"\genmacall\convert");
|
|
}
|
|
|
|
private void btnCvtXmlToSVG_Click(object sender, System.EventArgs e)
|
|
{
|
|
// delete current svg's
|
|
DirectoryInfo disvg = new DirectoryInfo(tbResultPath.Text + @"\genmacall"); //(@"C:\development\proms.net\genmac.xml");
|
|
FileInfo[] fissvg = disvg.GetFiles("*.svg");
|
|
foreach (FileInfo fi in fissvg)fi.Delete();
|
|
DirectoryInfo di = new DirectoryInfo(tbResultPath.Text + @"\genmacall\convert");
|
|
FileInfo[] fis = di.GetFiles("*.xml");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// results go to e:\proms.net\genmac.xml
|
|
AddFileToListBox(fi);
|
|
GenXmlToSvg gn = new GenXmlToSvg(fi.Name, tbResultPath.Text);
|
|
}
|
|
MessageBox.Show("DONE Converting genmacs.xmls to drawing svg - Results are in " + tbResultPath.Text + @"\genmacall");
|
|
}
|
|
|
|
|
|
private void btnCvtFormat_Click(object sender, EventArgs e)
|
|
{
|
|
CleanupDestinationFolder("*f.xml");
|
|
DateTime tStart = DateTime.Now;
|
|
// first convert base format - for now use genfmt.
|
|
MyStatus = "Processing Base";
|
|
FmtFileToXml gx = new FmtFileToXml(null, "BASE", "GEN", tbFmtPath.Text); // Create BASE from GEN format
|
|
|
|
// get all of the format files in the e:\ve-proms\format directory.
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text); // "C:\\16bit\\ve-proms\\format");
|
|
//DirectoryInfo di = new DirectoryInfo("E:\\proms.net\\exe\\fmtxml");
|
|
// This excludes Background format files *.BFM which use a different structure
|
|
FileInfo[] fis = di.GetFiles("*.fmt");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
string fn = fi.Name.Substring(0, fi.Name.Length - 4);
|
|
if (fn.ToLower() != "base")
|
|
{
|
|
AddFileToListBox(fi);
|
|
FmtFileToXml fx = new FmtFileToXml(gx, fn, fn, tbFmtPath.Text);
|
|
}
|
|
}
|
|
}
|
|
MyStatus = string.Format("DONE Converting Formats to XML {0:F3} seconds", TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds);
|
|
MessageBox.Show("DONE Converting Formats to XML");
|
|
}
|
|
|
|
private void CleanupDestinationFolder(string pattern)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo("fmt_xml");
|
|
if (di.Exists == false)
|
|
di.Create();
|
|
FileInfo[] fis = di.GetFiles(pattern);
|
|
foreach (FileInfo fi in fis) fi.Delete();
|
|
}
|
|
|
|
private void btnMergeFmtPagDoc_Click(object sender, EventArgs e)
|
|
{
|
|
ClearResults(tbResultPath.Text);
|
|
DateTime tStart = DateTime.Now;
|
|
MyStatus = "Merging...";
|
|
// get all of the generated xml format files in the fmt_xml directory and merge
|
|
// the fmt/doc/pag & subformats into one file.
|
|
DirectoryInfo di = new DirectoryInfo("fmt_xml");
|
|
//testDirectoryInfo di = new DirectoryInfo("E:\\proms.net\\exe\\fmtxml");
|
|
FileInfo[] fis = di.GetFiles("*f.xml");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
// see if all three, format, doc & pag exist - if not, print error,
|
|
// if so, process
|
|
string docname = "fmt_xml\\" + fi.Name.Substring(0, fi.Name.Length - 5) + "p.xml";
|
|
string pagname = "fmt_xml\\" + fi.Name.Substring(0, fi.Name.Length - 5) + "d.xml";
|
|
if (File.Exists(docname) && File.Exists(pagname))
|
|
{
|
|
AddFileToListBox(fi);
|
|
EntireFormat ef = new EntireFormat("fmt_xml\\" + fi.Name, tbResultPath.Text);
|
|
}
|
|
else
|
|
Console.WriteLine("For {0}, page or document file does not exist.", fi.Name);
|
|
}
|
|
MyStatus = string.Format("DONE Merging Formats {0:F3} seconds", TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds);
|
|
MessageBox.Show("DONE Merging Formats - Results are in " + tbResultPath.Text + @"fmtall'");
|
|
}
|
|
|
|
private void ClearResults(string path)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo(path);
|
|
if (di.Exists == false) return;
|
|
di = di.GetDirectories("fmtall")[0];
|
|
FileInfo[] fis = di.GetFiles("*.xml");
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (fi.IsReadOnly) fi.IsReadOnly = false;
|
|
fi.Delete();
|
|
}
|
|
}
|
|
private void btnBrowseFmt_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbFmtPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
if (Directory.Exists(fbd.SelectedPath)) tbFmtPath.Text = fbd.SelectedPath;
|
|
}
|
|
|
|
private void btnBrowseGenmac_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbFmtPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
{
|
|
if (Directory.Exists(fbd.SelectedPath)) tbGenmacPath.Text = fbd.SelectedPath;
|
|
}
|
|
}
|
|
|
|
private void btnBrowseResult_Click(object sender, EventArgs e)
|
|
{
|
|
FolderBrowserDialog fbd = new FolderBrowserDialog();
|
|
|
|
fbd.SelectedPath = tbResultPath.Text;
|
|
if (fbd.ShowDialog() == DialogResult.OK)
|
|
{
|
|
if (Directory.Exists(fbd.SelectedPath)) tbResultPath.Text = fbd.SelectedPath;
|
|
}
|
|
}
|
|
private void btnRtfToSvg_Click(object sender, EventArgs e)
|
|
{
|
|
// delete current svg's
|
|
DirectoryInfo disvg = new DirectoryInfo(tbResultPath.Text + @"\genmacall"); //(@"C:\development\proms.net\genmac.xml");
|
|
FileInfo[] fissvg = disvg.GetFiles("*.svg");
|
|
foreach (FileInfo fi in fissvg)
|
|
{
|
|
if (fi.IsReadOnly) fi.IsReadOnly = false;
|
|
fi.Delete();
|
|
}
|
|
|
|
DirectoryInfo di = new DirectoryInfo(tbFmtPath.Text);
|
|
FileInfo[] fis = di.GetFiles("*.rtf");
|
|
ClearListBox();
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
if (!Excluded(fi))
|
|
{
|
|
|
|
// results go to e:\proms.net\genmac.xml
|
|
AddFileToListBox(fi);
|
|
RtfToSvg myConvert = new RtfToSvg(fi);
|
|
if (myConvert.IsGenMac)
|
|
myConvert.Save(tbResultPath.Text + @"genmacall\" + fi.Name.ToLower().Replace(".rtf", ".svg"));
|
|
}
|
|
}
|
|
// The NSPIFG2 file did not exist. Copy over NSPIFG to it.
|
|
FileInfo fix = new FileInfo(tbResultPath.Text + @"genmacall\nspifg.svg");
|
|
fix.CopyTo(tbResultPath.Text + @"genmacall\nspifg2.svg");
|
|
MessageBox.Show("DONE converting GenMac RTF files to drawing svg - Results are in " + tbResultPath.Text + @"genmacall");
|
|
}
|
|
|
|
private void btnWestinghouse_Click(object sender, EventArgs e)
|
|
{
|
|
DateTime tStart = DateTime.Now;
|
|
CopyFormat("wst1all.xml");
|
|
CopyFormat("wst2all.xml");
|
|
CopyFormat("wstcklall.xml");
|
|
CopyFormat("wstalrall.xml");
|
|
CopyFormat("wstbckall.xml");
|
|
CopyFormat("wstdcsall.xml");
|
|
CopySVG("wst1.svg");
|
|
CopySVG("wst2.svg");
|
|
CopySVG("wstckl.svg");
|
|
CopySVG("wstalr.svg");
|
|
CopySVG("wstbck.svg");
|
|
CopySVG("wstdcs.svg");
|
|
|
|
CopyFormat("fnpnmpall.xml");
|
|
CopySVG("fnpnmp.svg");
|
|
|
|
CopyFormat("hlpfsgall.xml");
|
|
CopySVG("hlpfsg.svg");
|
|
|
|
// Comanche Peak Flex Format
|
|
CopyFormat("ComPeakFlexall.xml");
|
|
CopySVG("ComPeakFlex.svg");
|
|
|
|
MyStatus = string.Format("Copied Developed Formats/Genmacs {0:F3} seconds", TimeSpan.FromTicks(DateTime.Now.Ticks - tStart.Ticks).TotalSeconds);
|
|
MessageBox.Show("DONE Copy Developed Formats/Genmacs - Results are in " + tbResultPath.Text + @"fmtall' and 'genmacall'");
|
|
}
|
|
private void CopyFormat(string fileName)
|
|
{
|
|
FileInfo fi1 = new FileInfo(Application.StartupPath + "\\" + fileName);
|
|
FileInfo fi2 = new FileInfo(@"..\..\" + fileName);
|
|
if (fi2.Exists && (!fi1.Exists ||(fi1.Exists && fi2.LastAccessTimeUtc > fi1.LastAccessTimeUtc)))
|
|
{
|
|
if (fi1.Exists && fi1.IsReadOnly) fi1.IsReadOnly = false;
|
|
fi2.CopyTo(fi1.FullName, true);
|
|
fi1 = new FileInfo(Application.StartupPath + "\\" + fileName);
|
|
}
|
|
if (fi1.Exists)
|
|
{
|
|
FileInfo fio = new FileInfo(tbResultPath.Text + @"fmtall\" + fileName);
|
|
if (fio.Exists && fio.IsReadOnly) fio.IsReadOnly = false;
|
|
fi1.CopyTo(fio.FullName, true);
|
|
}
|
|
}
|
|
private void CopySVG(string fileName)
|
|
{
|
|
FileInfo fi1 = new FileInfo(Application.StartupPath + "\\" + fileName);
|
|
FileInfo fi2 = new FileInfo(@"..\..\" + fileName);
|
|
if (fi2.Exists && (!fi1.Exists || (fi1.Exists && fi2.LastAccessTimeUtc > fi1.LastAccessTimeUtc)))
|
|
{
|
|
if (fi1.Exists && fi1.IsReadOnly) fi1.IsReadOnly = false;
|
|
fi2.CopyTo(fi1.FullName, true);
|
|
fi1 = new FileInfo(Application.StartupPath + "\\" + fileName);
|
|
}
|
|
if (fi1.Exists)
|
|
{
|
|
FileInfo fio = new FileInfo(tbResultPath.Text + @"genmacall\" + fileName);
|
|
if (fio.Exists && fio.IsReadOnly) fio.IsReadOnly = false;
|
|
fi1.CopyTo(fio.FullName, true);
|
|
}
|
|
}
|
|
// old code:
|
|
//private void button5_Click(object sender, System.EventArgs e)
|
|
//{
|
|
|
|
// DirectoryInfo di = new DirectoryInfo("C:\\16bit\\ve-proms.net\\genmac.xml");
|
|
// FileInfo[] fis = di.GetFiles("*.xml");
|
|
// listBox1.Items.Clear();
|
|
// foreach (FileInfo fi in fis)
|
|
// {
|
|
// this.listBox1.Items.Add(fi.Name);
|
|
// //XmlToPdf pdf = new XmlToPdf("C:\\16bit\\ve-proms.net\\genmac.xml",fi.Name);
|
|
// }
|
|
// MessageBox.Show("DONE generating PDF files from XML");
|
|
//}
|
|
|
|
//private void button6_Click(object sender, System.EventArgs e)
|
|
//{
|
|
// DirectoryInfo di = new DirectoryInfo("C:\\16bit\\proms.net\\genmac.xml");
|
|
// FileInfo[] fis = di.GetFiles("*.svg");
|
|
// listBox1.Items.Clear();
|
|
// foreach (FileInfo fi in fis)
|
|
// {
|
|
// this.listBox1.Items.Add(fi.Name);
|
|
// //SvgToPdf pdf = new SvgToPdf("C:\\16bit\\proms.net\\genmac.xml", fi.Name);
|
|
// }
|
|
// MessageBox.Show("DONE generating PDF files from SVG");
|
|
//}
|
|
|
|
}
|
|
}
|