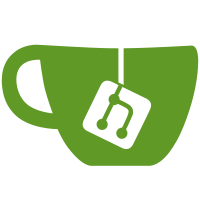
Eliminate SelChange funtions during Initialization Eliminate Moving GridItem to the Left and Right on selection Fixed a bug where SelectedEditItem was null Eliminate SelChange funtions during Initialization Use FlexGrid CellRange (Selection) to Get and Set Range Borders Added Unknown value to GridLinePattern enum Set Range Borders based upon CellRange (Selection)
1041 lines
34 KiB
C#
1041 lines
34 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Text.RegularExpressions;
|
|
//using System.IO;
|
|
using System.Windows.Forms;
|
|
using System.Xml;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Base.Library;
|
|
using C1.Win.C1FlexGrid;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class GridItem : EditItem
|
|
{
|
|
#region Fields
|
|
public VlnFlexGrid MyFlexGrid
|
|
{
|
|
get { return _MyFlexGrid; }
|
|
set { _MyFlexGrid = value; }
|
|
}
|
|
private int _GridMargin = 11;
|
|
/// <summary>
|
|
/// Margin between the EditItem and the VlnFlexGrid. Appears on the Right.
|
|
/// Will allow space to draw a Change Bar on the right side of the EditItem.
|
|
/// </summary>
|
|
public int GridMargin
|
|
{
|
|
get { return _GridMargin; }
|
|
set { _GridMargin = value; }
|
|
}
|
|
#endregion
|
|
|
|
#region Event Handlers
|
|
/// <summary>
|
|
/// Raises an ItemClick event when the user clicks on the Tab
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void lblTab_MouseDown(object sender, MouseEventArgs e)
|
|
{
|
|
MyStepPanel.OnItemClick(this, new StepPanelEventArgs(this, e));
|
|
}
|
|
void MyFlexGrid_Resize(object sender, EventArgs e)
|
|
{
|
|
this.Size = new Size(MyFlexGrid.Width + GridMargin, MyFlexGrid.Height + GridMargin);
|
|
AdjustTableWidthAndLocation();
|
|
AdjustLocation();
|
|
}
|
|
void MyFlexGrid_Enter(object sender, EventArgs e)
|
|
{
|
|
if (MyStepPanel.DisplayItemChanging) return;
|
|
MyStepRTB.MyItemInfo = MyItemInfo; // should be in vlnFlexGrid
|
|
MyStepPanel.SelectedEditItem = this;
|
|
if (MyFlexGrid.IsRoTable)
|
|
{
|
|
MyFlexGrid.Cols.Fixed = MyFlexGrid.Cols.Count;
|
|
MyFlexGrid.Rows.Fixed = MyFlexGrid.Rows.Count;
|
|
}
|
|
}
|
|
void MyFlexGrid_KeyDown(object sender, KeyEventArgs e)
|
|
{
|
|
switch (e.KeyCode)
|
|
{
|
|
case Keys.F5:
|
|
if (e.Shift)
|
|
{
|
|
e.Handled = true;
|
|
if (!CheckClipboard()) return; // check if 'clipboard' contains a step.
|
|
SetMenu("StepPaste");
|
|
}
|
|
else if (!e.Control && !e.Alt)
|
|
{
|
|
e.Handled = true;
|
|
CopyStep();
|
|
}
|
|
break;
|
|
case Keys.Delete:
|
|
MyFlexGrid.ClearSelectedCellText();
|
|
break;
|
|
}
|
|
}
|
|
void SetMenu(string contentMenu)
|
|
{
|
|
if (contentMenu == null)
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.ClearContextMenu();
|
|
else if (contentMenu == "OpenContextMenu")
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetGridCellContextMenu(); //.SetContextMenu();
|
|
else
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetShortCutContextMenu(contentMenu);
|
|
}
|
|
void MyFlexGrid_CursorMovement(object sender, VlnFlexGridCursorMovementEventArgs args)
|
|
{
|
|
MyStepPanel.CursorMovement(this,new Point(0,0), args.Key);
|
|
}
|
|
void MyStepRTB_LinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
MyStepPanel.OnLinkModifyTran(sender, args);
|
|
}
|
|
void MyStepRTB_LinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
MyStepPanel.OnLinkModifyRO(sender, args);
|
|
}
|
|
void MyStepRTB_LinkGoTo(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
MyStepPanel.OnLinkClicked(sender, args);
|
|
}
|
|
void MyStepRTB_CursorKeyPress(object sender, KeyEventArgs args)
|
|
{
|
|
MyStepPanel.StepCursorKeys(this, args);
|
|
}
|
|
void MyStepRTB_AdjustTableWidth(object sender, StepRTBTableWidthEventArgs args)
|
|
{
|
|
//if ((!_MyItemInfo.IsSection && !_MyItemInfo.IsProcedure) && (_MyItemInfo.IsTable || _MyItemInfo.IsFigure))
|
|
if (args.EditMode)
|
|
{
|
|
int colR = MyStepPanel.ToDisplay(MyStepSectionLayoutData.ColRTable, MyItemInfo.ColumnMode);
|
|
int widS = /* _WidthAdjust + borderWidth + */ MyStepPanel.ToDisplay(MyStepSectionLayoutData.WidSTableEdit, MyItemInfo.ColumnMode);
|
|
int wNew = 70 + widS + colR * MyItemInfo.ColumnMode;
|
|
if (wNew > ItemWidth)
|
|
{
|
|
ItemWidth = wNew;
|
|
ItemLocation = TableLocation(MyParentEditItem, MyStepSectionLayoutData, wNew);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
int newwidth = MyFlexGrid.Width;
|
|
if (ItemWidth != newwidth)
|
|
{
|
|
ItemWidth = newwidth;
|
|
ItemLocation = TableLocation(MyParentEditItem, MyStepSectionLayoutData, newwidth);
|
|
}
|
|
}
|
|
}
|
|
bool MyStepRTB_IsNotCurrentSelection(object sender, EventArgs args)
|
|
{
|
|
return MyStepPanel.SelectedEditItem != this;
|
|
}
|
|
|
|
void MyStepRTB_OpenAnnotations(object sender, EventArgs args)
|
|
{
|
|
OpenAnnotations();
|
|
}
|
|
void MyFlexGrid_OpenAnnotations(object sender, EventArgs args)
|
|
{
|
|
OpenAnnotations();
|
|
}
|
|
void MyStepRTB_DoMouseWheel(object sender, MouseEventArgs args)
|
|
{
|
|
DoMouseWheel(args);
|
|
}
|
|
void MyStepRTB_DoSaveContents(object sender, EventArgs args)
|
|
{
|
|
SaveCurrentAndContents();
|
|
}
|
|
|
|
|
|
void MyStepRTB_VisibleChanged(object sender, EventArgs e)
|
|
{
|
|
MyStepRTB.EditMode = MyStepRTB.Visible;
|
|
//Console.WriteLine("GridItem: EditMode = {0}", MyStepRTB.EditMode);
|
|
}
|
|
void MyStepRTB_ReturnToEditor(object sender, EventArgs args)
|
|
{
|
|
MyFlexGrid.Focus();
|
|
MyFlexGrid.StartEditing();
|
|
}
|
|
void MyFlexGrid_Click(object sender, EventArgs e)
|
|
{
|
|
MyFlexGrid.Focus();
|
|
//MyStepPanel.MyStepTabPanel.MyStepTabRibbon.ToggleTableDesignButtons(false);
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetRibbonForGrid();
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetTableButtonsForMergeRangeSelection();
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetGridContextMenu();
|
|
}
|
|
void MyStepRTB_EditModeChanged(object sender, EventArgs args)
|
|
{
|
|
AdjustColorsForEditMode();
|
|
MyStepPanel.MyStepTabPanel.MyStepTabRibbon.SetRibbonForGrid();
|
|
}
|
|
|
|
private void AdjustColorsForEditMode()
|
|
{
|
|
MyFlexGrid.StyleBackColor = Color.White;
|
|
if (MyStepRTB.EditMode)
|
|
{
|
|
MyFlexGrid.Styles["Focus"].ForeColor = MyFlexGrid.Styles["Focus"].BackColor =
|
|
MyFlexGrid.Styles["Highlight"].ForeColor = MyFlexGrid.Styles["Highlight"].BackColor = Color.SkyBlue;
|
|
}
|
|
else
|
|
{
|
|
MyFlexGrid.Styles["Focus"].ForeColor = MyFlexGrid.Styles["Highlight"].ForeColor = Color.Black;
|
|
MyFlexGrid.Styles["Focus"].BackColor = MyFlexGrid.Styles["Highlight"].BackColor = Color.LightCyan;
|
|
}
|
|
}
|
|
private string _OrigRtf; // used to store original rtf to allow for 'escape' key restore
|
|
void MyFlexGrid_SelChange(object sender, EventArgs e)
|
|
{
|
|
if (Initializing) return;
|
|
//Volian.Base.Library.vlnStackTrace.ShowStackLocal("MyFlexGrid_SelChange {0}",MyFlexGrid.Selection);
|
|
RTBLastFocus = false;
|
|
MyStepRTB.Visible = false; // Hide the editor if the Selection Changes
|
|
if (MyFlexGrid.Selection.IsSingleCell && MyFlexGrid.Row >= 0 && MyFlexGrid.Col >= 0)
|
|
{
|
|
object obj = MyFlexGrid[MyFlexGrid.Row, MyFlexGrid.Col];
|
|
_OrigRtf = obj == null ? string.Empty : obj.ToString();
|
|
}
|
|
}
|
|
void MyStepRTB_KeyDown(object sender, KeyEventArgs e)
|
|
{
|
|
if (!e.Control && !e.Shift && !e.Alt && e.KeyCode == Keys.Escape && MyStepRTB.EditMode)
|
|
{
|
|
MyStepRTB.Rtf = _OrigRtf;
|
|
MyFlexGrid.Select(MyFlexGrid.Selection);
|
|
}
|
|
}
|
|
private string MyFlexGrid_AdjustPastedText(object sender, VlnFlexGridPasteEventArgs args)
|
|
{
|
|
string tmpForLink = args.Text;
|
|
tmpForLink = Regex.Replace(tmpForLink, @"#Link:ReferencedObject:[0-9]+ ", @"#Link:ReferencedObject:<NewID> ");
|
|
tmpForLink = Regex.Replace(tmpForLink, @"#Link:Transition:([0-9]+) [0-9]+ ", @"#Link:Transition:$1 <NewID> ");
|
|
tmpForLink = Regex.Replace(tmpForLink, @"#Link:TransitionRange:([0-9]+) [0-9]+ ", @"#Link:TransitionRange:$1 <NewID> ");
|
|
return tmpForLink;
|
|
}
|
|
#endregion // Event Handlers
|
|
#region Constructors
|
|
public GridItem()
|
|
{
|
|
InitializeComponent();
|
|
}
|
|
public GridItem(ItemInfo itemInfo, StepPanel myStepPanel, EditItem myParentEditItem, ChildRelation myChildRelation, bool expand)
|
|
{
|
|
InitializeComponent();
|
|
SetupEventHandlers();
|
|
SetupEditItem(itemInfo, myStepPanel, myParentEditItem, myChildRelation, expand, null);
|
|
}
|
|
public GridItem(ItemInfo itemInfo, StepPanel myStepPanel, EditItem myParentEditItem, ChildRelation myChildRelation, bool expand, EditItem nextEditItem)
|
|
{
|
|
InitializeComponent();
|
|
SetupEventHandlers();
|
|
SetupEditItem(itemInfo, myStepPanel, myParentEditItem, myChildRelation, expand, nextEditItem);
|
|
}
|
|
private void SetupEventHandlers()
|
|
{
|
|
this.MyFlexGrid.Resize += new EventHandler(MyFlexGrid_Resize);
|
|
this.MyFlexGrid.Enter += new EventHandler(MyFlexGrid_Enter);
|
|
this.MyFlexGrid.CursorMovement += new VlnFlexGridCursorMovementEvent(MyFlexGrid_CursorMovement);
|
|
this.MyFlexGrid.OpenAnnotations += new VlnFlexGridEvent(MyFlexGrid_OpenAnnotations);
|
|
this.MyFlexGrid.KeyDown +=new KeyEventHandler(MyFlexGrid_KeyDown);
|
|
this.MyStepRTB.LinkGoTo += new StepRTBLinkEvent(MyStepRTB_LinkGoTo);
|
|
this.MyStepRTB.LinkModifyRO += new StepRTBLinkEvent(MyStepRTB_LinkModifyRO);
|
|
this.MyStepRTB.LinkModifyTran += new StepRTBLinkEvent(MyStepRTB_LinkModifyTran);
|
|
this.MyStepRTB.CursorKeyPress += new StepRTBCursorKeysEvent(MyStepRTB_CursorKeyPress);
|
|
this.MyStepRTB.AdjustTableWidth += new StepRTBTableWidthEvent(MyStepRTB_AdjustTableWidth);
|
|
this.MyStepRTB.IsNotCurrentSelection += new StepRTBBooleanEvent(MyStepRTB_IsNotCurrentSelection);
|
|
this.MyStepRTB.OpenAnnotations += new StepRTBEvent(MyStepRTB_OpenAnnotations);
|
|
this.MyStepRTB.DoMouseWheel += new StepRTBMouseEvent(MyStepRTB_DoMouseWheel);
|
|
this.MyStepRTB.DoSaveContents += new StepRTBEvent(MyStepRTB_DoSaveContents);
|
|
this.MyStepRTB.VisibleChanged += new EventHandler(MyStepRTB_VisibleChanged);
|
|
this.MyStepRTB.ReturnToEditor += new StepRTBEvent(MyStepRTB_ReturnToEditor);
|
|
this.MyFlexGrid.Click += new EventHandler(MyFlexGrid_Click);
|
|
this.MyStepRTB.EditModeChanged += new StepRTBEvent(MyStepRTB_EditModeChanged);
|
|
this.MyStepRTB.KeyDown += new KeyEventHandler(MyStepRTB_KeyDown);
|
|
this.MyFlexGrid.SelChange += new EventHandler(MyFlexGrid_SelChange);
|
|
this.MyStepRTB.GotFocus += new EventHandler(MyStepRTB_GotFocus);
|
|
this.MyStepRTB.RoInsert += new StepRTBRoEvent(MyStepRTB_RoInsert);
|
|
this.MyFlexGrid.AdjustPastedText += new VlnFlexGridPasteEvent(MyFlexGrid_AdjustPastedText);
|
|
}
|
|
void MyStepRTB_RoInsert(object sender, StepRTBRoEventArgs args)
|
|
{
|
|
if (MyFlexGrid.IsRoTable)
|
|
{
|
|
MyFlexGrid.Clear();
|
|
ConvertTableToGrid(args.RawValText);
|
|
MyFlexGrid.RODbId = args.RODbID;
|
|
MyFlexGrid.ROID = args.ROID;
|
|
SaveContents();
|
|
}
|
|
else
|
|
MyStepRTB.UpdateStepRtb(args.LinkText, args.ValText);
|
|
}
|
|
void MyStepRTB_GotFocus(object sender, EventArgs e)
|
|
{
|
|
RTBLastFocus = true;
|
|
}
|
|
#endregion
|
|
#region Override Method and Properties
|
|
public override int BorderWidth { get { return (MyFlexGrid.Width - MyFlexGrid.ClientRectangle.Width); } }
|
|
|
|
public override Point ContentLocation
|
|
{
|
|
get { return new Point(Location.X + MyFlexGrid.Left, Location.Y); }
|
|
set { Location = new Point(value.X - MyFlexGrid.Left, value.Y); }
|
|
}
|
|
public override string TabFormat { get { return _TabFormat; } set { _TabFormat = value; } }
|
|
public override void AdjustTableWidthAndLocation()
|
|
{
|
|
//_MyStepRTB.Font = MyStepData.Font.WindowsFont;
|
|
//ItemWidth = (int)GetTableWidth(MyStepRTB.Font, MyItemInfo.MyContent.Text, true);
|
|
//ItemWidth = MyFlexGrid.Width;
|
|
//ItemLocation = new Point(50, _MyParentEditItem.Bottom);
|
|
Point newLocation = TableLocation(_MyParentEditItem, MyStepSectionLayoutData, ItemWidth);
|
|
if (!newLocation.Equals(ItemLocation)) ItemLocation = newLocation;
|
|
}
|
|
public override void SetToolTip(string tip)
|
|
{
|
|
DevComponents.DotNetBar.SuperTooltipInfo tpi = new DevComponents.DotNetBar.SuperTooltipInfo("", "", tip, null, null, DevComponents.DotNetBar.eTooltipColor.Lemon);
|
|
_MyToolTip.MinimumTooltipSize = new Size(0, 24);
|
|
_MyToolTip.TooltipDuration = 3;
|
|
_MyToolTip.SetSuperTooltip(MyStepRTB, tpi);
|
|
}
|
|
public override void RefreshContent()
|
|
{
|
|
using (Grid myGrid = MyItemInfo.MyContent.MyGrid.Get())
|
|
{
|
|
MyItemInfo.MyContent.MyGrid.ResetContent(myGrid);
|
|
}
|
|
RefreshDisplay(_ActiveMode);
|
|
IdentifyMe(false);
|
|
}
|
|
public override int TableWidth { get { return 0; } } // DONE
|
|
public override int ItemLeft
|
|
{
|
|
get { return Left + lblTab.Left; }
|
|
set { Left = value - lblTab.Left; }
|
|
}
|
|
public override int ContentLeft { get { return Left + MyFlexGrid.Left; } }
|
|
public override int ContentWidth
|
|
{
|
|
get { return MyFlexGrid.Width; }
|
|
set
|
|
{
|
|
Width = value + lblTab.Left + lblTab.Width;
|
|
}
|
|
}
|
|
public override Point ItemLocation
|
|
{
|
|
get { return new Point(Location.X + lblTab.Left, Location.Y); }
|
|
set { Location = new Point(value.X - lblTab.Left, value.Y); }
|
|
}
|
|
public override int ItemWidth
|
|
{
|
|
get { return Width - lblTab.Left; }
|
|
set
|
|
{
|
|
Width = GridMargin + value + lblTab.Left;
|
|
}
|
|
}
|
|
public override void RefreshOrdinal()
|
|
{
|
|
TabFormat = null; // Reset Tab
|
|
}
|
|
public override bool Expanded
|
|
{
|
|
get {return !Colapsing;}
|
|
set {;} // Tables are always expanded.
|
|
}
|
|
public override void RefreshTab() { TabFormat = null;}
|
|
public override void SetFocus() { MyFlexGrid.Focus();}
|
|
public override void SaveContents()
|
|
{
|
|
List<int> RtfRoUsageList = new List<int>();
|
|
List<int> RtfTransList = new List<int>();
|
|
|
|
int r = 0;
|
|
int c = 0;
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
|
|
string srchtxt = MyFlexGrid.GetSearchableText();
|
|
// find the rousages within the grid cell. If this is an ro table, there will only be one usage
|
|
// if it is a modify and there will be no usages if it is new (the usage gets created on the save)
|
|
if (!MyFlexGrid.IsRoTable)
|
|
{
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
if (MyFlexGrid[r, c] != null)
|
|
{
|
|
// see if there are any links and save these so that any deleted ROs or transitions in the
|
|
// steprtb can have associated usages/transitions records removed from the database.
|
|
string lookFor = string.Format(@"<START\](\\[^v \\]+)*\\v0(\\[^v \\]+)* (.*?)(\\[^v \\]+)*\\v(\\[^v \\]+)* #Link:(ReferencedObject|Transition[^:]*?):.*?\[END>");
|
|
MatchCollection matches = Regex.Matches((string)MyFlexGrid[r, c], lookFor);
|
|
for (int i = matches.Count - 1; i >= 0; i--)
|
|
{
|
|
Match m = matches[i];
|
|
if (m != null && m.Groups.Count > 6 && m.Groups[6].ToString() == "ReferencedObject")
|
|
{
|
|
Regex regRefObj = new Regex(@"\#Link\:ReferencedObject\:([0-9]*) ([0-9a-zA-Z]*) ([0-9]*)", RegexOptions.Singleline);
|
|
Match myMatch = regRefObj.Match(m.Value);
|
|
if (myMatch.Success)
|
|
{
|
|
int usgid = int.Parse(myMatch.Groups[1].Value);
|
|
RtfRoUsageList.Add(usgid);
|
|
}
|
|
}
|
|
if (m != null && m.Groups.Count > 6 && m.Groups[6].ToString().StartsWith("Transition"))
|
|
{
|
|
Regex regRefObj = new Regex(@"\#Link\:Transition[a-zA-Z]*\:([0-9]*) ([0-9]*) ([0-9]*)", RegexOptions.Singleline);
|
|
Match myMatch = regRefObj.Match(m.Value);
|
|
if (myMatch.Success)
|
|
{
|
|
int tid = int.Parse(myMatch.Groups[2].Value);
|
|
RtfTransList.Add(tid);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// new roid is in flexgrid.roid. Compare to previous, if there was a previous. If there
|
|
// is a modify RO, then need to delete the existing rousage record because a new usage
|
|
// record will get created that contains the selected ROID.
|
|
Regex regRefObj = new Regex(@"\#Link\:ReferencedObject\:([0-9]*) ([0-9a-zA-Z]*) ([0-9]*)", RegexOptions.Singleline);
|
|
Match myMatch = regRefObj.Match(MyItemInfo.MyContent.Text);
|
|
if (myMatch.Success)
|
|
{
|
|
int usgid = int.Parse(myMatch.Groups[1].Value);
|
|
RoUsageInfo rui = RoUsageInfo.Get(usgid);
|
|
if (rui.ROID == MyFlexGrid.ROID)RtfRoUsageList.Add(usgid);
|
|
}
|
|
}
|
|
// compare ro usages & transitions from database and list from grid contents
|
|
// if in both lists, don't do anything
|
|
// if only in database list, delete usage.
|
|
// never will have condition where it is not in database list, but is in steprtb text list (this
|
|
// case is handled when ro is inserted.
|
|
if (MyItemInfo.MyContent.ContentRoUsages != null)
|
|
{
|
|
List<int> delRoUsgs = new List<int>();
|
|
foreach (RoUsageInfo ru in MyItemInfo.MyContent.ContentRoUsages)
|
|
if (!RtfRoUsageList.Contains(ru.ROUsageID)) delRoUsgs.Add(ru.ROUsageID);
|
|
foreach (int dRU in delRoUsgs) RoUsage.Delete(dRU);
|
|
}
|
|
if (MyItemInfo.MyContent.ContentTransitions != null)
|
|
{
|
|
List<int> delTrans = new List<int>();
|
|
foreach (TransitionInfo ti in MyItemInfo.MyContent.ContentTransitions)
|
|
if (!RtfTransList.Contains(ti.TransitionID)) delTrans.Add(ti.TransitionID);
|
|
foreach (int dt in delTrans) Transition.Delete(dt);
|
|
}
|
|
|
|
bool success = FinishSave(srchtxt);
|
|
if (success && !MyFlexGrid.IsRoTable)
|
|
{
|
|
MyStepRTB.FindAllLinks();
|
|
MyStepRTB.OrigRTF = MyStepRTB.Rtf;
|
|
MyStepRTB.ClearUndo();
|
|
}
|
|
}
|
|
private string DoLinkForRoTable()
|
|
{
|
|
// if no ro has been defined yet, just return null
|
|
if (MyFlexGrid.ROID == null) return null;
|
|
ContentRoUsage rousg = null;
|
|
using (Item itm = MyItemInfo.Get())
|
|
{
|
|
using (RODb rodb = RODb.GetJustRoDb(MyFlexGrid.RODbId))
|
|
{
|
|
string padroid = (MyFlexGrid.ROID.Length <= 12) ? MyFlexGrid.ROID + "0000" : MyFlexGrid.ROID;
|
|
rousg = itm.MyContent.ContentRoUsages.Add(MyFlexGrid.ROID, rodb);
|
|
}
|
|
itm.Save();
|
|
}
|
|
MyItemInfo.MyContent.RefreshContentRoUsages();
|
|
return string.Format(@"#Link:ReferencedObject:{0} {1} {2}", rousg.ROUsageID, MyFlexGrid.ROID, MyFlexGrid.RODbId);
|
|
}
|
|
private void ConvertTableToGrid(string valtext)
|
|
{
|
|
VE_Font vefont = MyItemInfo.GetItemFont();
|
|
MyFlexGrid.Font = vefont.WindowsFont;
|
|
Initializing = true;
|
|
MyFlexGrid.ParseTableFromText(valtext);
|
|
MyFlexGrid.AutoSizeCols();
|
|
MyFlexGrid.AutoSizeRows();
|
|
MyFlexGrid.MakeRTFcells();
|
|
Initializing = false;
|
|
}
|
|
private bool FinishSave(string searchableText)
|
|
{
|
|
// Just in case if the grid was in a mode to change sizes, clear out that setting
|
|
// in the grid:
|
|
MyFlexGrid.Cols.Fixed = 0;
|
|
MyFlexGrid.Rows.Fixed = 0;
|
|
MyFlexGrid.StyleBackColor = Color.White;
|
|
|
|
string xml = MyFlexGrid.GetXMLData();
|
|
using (Item itm = MyItemInfo.Get())
|
|
{
|
|
itm.MyContent.MyGrid.Data = xml;
|
|
// if this is the initial save of an ro table, then the 'DoLinkForRoTable' will
|
|
// create the usage for it. this code gets run on modify of the ro table and also
|
|
// on exit of the griditem. We don't want to save the ro usage again, if it's already
|
|
// been saved.
|
|
if (MyFlexGrid.IsRoTable && MyFlexGrid.ROID != null && itm.MyContent.ContentRoUsageCount < 1)
|
|
{
|
|
searchableText = string.Format(@"\v<START]\v0 {0} \v {1}[END>\v0 ", searchableText, DoLinkForRoTable());
|
|
itm.MyContent.Text = searchableText;
|
|
}
|
|
if (!MyFlexGrid.IsRoTable)itm.MyContent.Text = searchableText;
|
|
itm.Save();
|
|
MyItemInfo.MyContent.MyGrid.ResetContent(itm.MyContent.MyGrid);
|
|
}
|
|
return true;
|
|
}
|
|
public void BasicSave()
|
|
{
|
|
using (Item itm = MyItemInfo.Get())
|
|
{
|
|
itm.MyContent.MyGrid.Data = MyFlexGrid.GetXMLData();
|
|
itm.Save();
|
|
//MyItemInfo.MyContent.MyGrid.ResetContent(itm.MyContent.MyGrid);
|
|
}
|
|
}
|
|
public override bool CanExpand { get { return false; } set { ;} }
|
|
public override void HandleResize() { ;} // DONE
|
|
public override void MatchExpanded() { ;} // DONE
|
|
public override void ItemSelect()
|
|
{
|
|
// Was getting an Error that _MyStepRTB was Disposed RHM 20101217
|
|
if (!MyFlexGrid.Disposing)
|
|
{
|
|
Focus();
|
|
MyFlexGrid.Focus();
|
|
MyFlexGrid.Select(0, 0);
|
|
}
|
|
else
|
|
{
|
|
_MyLog.WarnFormat("Attempt to give Focus to Disposed Object {0}", MyID);
|
|
}
|
|
ScrollToCenter();
|
|
}
|
|
public override void ItemShow()
|
|
{
|
|
MyFlexGrid.Focus();
|
|
ScrollToCenter();
|
|
}
|
|
public StepRTB DisplayRoStepRTB;
|
|
public override StepRTB MyStepRTB
|
|
{
|
|
get
|
|
{
|
|
return MyFlexGrid.TableCellEditor;
|
|
}
|
|
}
|
|
public override DialogResult ReplaceText(string rpltxt, string fndstr, bool caseSensitive, bool matchWholeWord, bool reverse, bool prompt, IWin32Window fndrpldlg)
|
|
{
|
|
int r = MyFlexGrid.Row;
|
|
int c = MyFlexGrid.Col;
|
|
|
|
if (!reverse)
|
|
{
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
int ss = MyStepRTB.SelectionStart;
|
|
int sl = MyStepRTB.SelectionLength;
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(ss, sl); // need to reset selection because startediting positions to end.
|
|
string oldRtf = MyStepRTB.Rtf;
|
|
DialogResult dr = MyStepRTB.ReplaceText(rpltxt, fndstr, caseSensitive, matchWholeWord, reverse, prompt, fndrpldlg);
|
|
if (oldRtf != MyStepRTB.Rtf) MyFlexGrid[r, c] = MyStepRTB.Rtf;
|
|
if (dr == DialogResult.Yes || dr == DialogResult.Cancel) return dr;
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
if (r < h)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyStepRTB.Select(0, 0);
|
|
}
|
|
}
|
|
}
|
|
else // reverse
|
|
{
|
|
while (r >= 0)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
int ss = MyStepRTB.SelectionStart;
|
|
int sl = MyStepRTB.SelectionLength;
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(ss, sl);
|
|
string oldRtf = MyStepRTB.Rtf;
|
|
DialogResult dr = MyStepRTB.ReplaceText(rpltxt, fndstr, caseSensitive, matchWholeWord, reverse, prompt, fndrpldlg);
|
|
if (oldRtf != MyStepRTB.Rtf) MyFlexGrid[r, c] = MyStepRTB.Rtf;
|
|
if (dr == DialogResult.Yes || dr == DialogResult.Cancel) return dr;
|
|
}
|
|
c = c - 1;
|
|
if (c < 0)
|
|
{
|
|
c = MyFlexGrid.Cols.Count - 1;
|
|
r = r - 1;
|
|
}
|
|
cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (r >= 0 && cr.r1 == r && cr.c1 == c)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(MyStepRTB.Text.Length, 0);
|
|
}
|
|
}
|
|
}
|
|
return DialogResult.No;
|
|
}
|
|
public override bool FindText(string str, bool caseSensitive, bool matchWholeWord, bool reverse)
|
|
{
|
|
int r = MyFlexGrid.Row;
|
|
int c = MyFlexGrid.Col;
|
|
if (!reverse)
|
|
{
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
int ss = MyStepRTB.SelectionStart + MyStepRTB.SelectionLength;
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(ss, 0); // need to reset selection because startediting positions to end.
|
|
bool scn = MyStepRTB.FindText(str, caseSensitive, matchWholeWord, reverse);
|
|
if (scn) return true;
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
if (r < h)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyStepRTB.Select(0, 0);
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
while (r >= 0)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
int ss = MyStepRTB.SelectionStart;
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(ss, 0);
|
|
bool scn = MyStepRTB.FindText(str, caseSensitive, matchWholeWord, reverse);
|
|
if (scn) return true;
|
|
}
|
|
c = c - 1;
|
|
if (c < 0)
|
|
{
|
|
c = MyFlexGrid.Cols.Count - 1;
|
|
r = r - 1;
|
|
}
|
|
cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (r >= 0 && cr.r1 == r && cr.c1 == c)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(MyStepRTB.Text.Length, 0);
|
|
}
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
public override void PositionToEnd()
|
|
{
|
|
int r = MyFlexGrid.Rows.Count - 1;
|
|
int c = MyFlexGrid.Cols.Count - 1;
|
|
MyFlexGrid.Select(r, c);
|
|
while (r >= 0)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
MyFlexGrid.StartEditing();
|
|
return;
|
|
}
|
|
c = c - 1;
|
|
if (c < 0)
|
|
{
|
|
c = MyFlexGrid.Cols.Count - 1;
|
|
r = r - 1;
|
|
}
|
|
if (r >= 0)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyStepRTB.Select(MyStepRTB.Text.Length, 0);
|
|
}
|
|
}
|
|
}
|
|
public override void PositionToStart()
|
|
{
|
|
MyFlexGrid.Select(0,0);
|
|
MyFlexGrid.StartEditing();
|
|
MyStepRTB.Select(0, 0);
|
|
}
|
|
public override string SelectedTextForFind
|
|
{
|
|
get
|
|
{
|
|
StepRTB srtb = MyStepRTB;
|
|
if (srtb.SelectionLength > 0)
|
|
{
|
|
if (srtb.IsSelectionLinked(srtb.SelectionStart, srtb.SelectionLength))
|
|
return srtb.SelectedText.Substring(0, srtb.SelectedText.IndexOf("#Link"));
|
|
else
|
|
return srtb.SelectedText;
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
public override bool SpellCheckNext()
|
|
{
|
|
int r = MyFlexGrid.Row;
|
|
int c = MyFlexGrid.Col;
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
MyFlexGrid.Select(r, c);
|
|
MyFlexGrid.StartEditing();
|
|
string oldRtf = MyStepRTB.Rtf;
|
|
bool scn = MyStepRTB.SpellCheckNext();
|
|
if (oldRtf != MyStepRTB.Rtf) MyFlexGrid[r, c] = MyStepRTB.Rtf;
|
|
if (!scn) return false;
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
public override void IdentifyMe(bool highlight)
|
|
{
|
|
if (highlight)
|
|
{
|
|
MyFlexGrid.StyleBackColor = Color.Gray;
|
|
}
|
|
else
|
|
{
|
|
if (MyFlexGrid.Focused) // If active Set BackColor to the active color
|
|
SetActive();
|
|
else // Otherwise Set the BackColor to either the InactiveColor or the AnnotationColor
|
|
{
|
|
MyFlexGrid.StyleBackColor =
|
|
MyItemInfo.ItemAnnotationCount == 0 ? MyStepPanel.InactiveColor : MyStepPanel.AnnotationColor;
|
|
// Turn-off Size adjustment
|
|
MyFlexGrid.Cols.Fixed = 0;
|
|
MyFlexGrid.Rows.Fixed = 0;
|
|
}
|
|
}
|
|
}
|
|
public override void SetActive()
|
|
{
|
|
AdjustColorsForEditMode();
|
|
}
|
|
private bool _Empty = false;
|
|
public override bool Empty
|
|
{
|
|
get { return _Empty; }
|
|
set { _Empty = value; }
|
|
}
|
|
private bool _Initializing = false;
|
|
public bool Initializing
|
|
{
|
|
get { return _Initializing; }
|
|
set { _Initializing = value; }
|
|
}
|
|
private bool _ActiveMode = false;
|
|
public override void RefreshDisplay(bool activeMode)
|
|
{
|
|
_ActiveMode = activeMode;
|
|
//XmlDocument xd = new XmlDocument();
|
|
//xd.LoadXml(MyItemInfo.MyContent.MyGrid.Data);
|
|
//using (StringReader sr = new StringReader())
|
|
MyFlexGrid.Clear();
|
|
MyFlexGrid.MergedRanges.Clear();
|
|
Initializing = true;
|
|
MyFlexGrid.LoadGrid(MyItemInfo);
|
|
Initializing = false;
|
|
MyFlexGrid.KeyActionTab = KeyActionEnum.MoveAcross;
|
|
MyFlexGrid.AdjustGridControlSize();
|
|
// When a grid is read from XML, all of the styles are updated to the values in the XML.
|
|
// Thus any specific settings need to be set after the grid is read.
|
|
MyFlexGrid.Styles["Highlight"].BackColor = Color.LightCyan;
|
|
MyFlexGrid.Styles["Focus"].BackColor = Color.LightCyan;
|
|
MyFlexGrid.HighLight = activeMode ? C1.Win.C1FlexGrid.HighLightEnum.Always : C1.Win.C1FlexGrid.HighLightEnum.WithFocus;
|
|
MyFlexGrid.VwMode = MyStepPanel.VwMode;
|
|
}
|
|
public override void ToggleEditView(E_ViewMode vwMode)
|
|
{
|
|
/*
|
|
* TODO:
|
|
* 1) SaveContents
|
|
*/
|
|
RefreshDisplay(false);
|
|
MyFlexGrid.VwMode = vwMode;
|
|
}
|
|
public override int TabLeft { get { return lblTab.Left; } set { lblTab.Left = value; } }
|
|
public override Font TabFont { get { return lblTab.Font; } set { lblTab.Font = value; } }
|
|
public override string TabText { get { return lblTab.Text; } }
|
|
public override Point TabLocation { get { return lblTab.Location; } }
|
|
public override Font ContentFont { get { return MyFlexGrid.Font; } set { MyFlexGrid.Font = value; } } // TODO how do we know what font tables are in?
|
|
public override float ContentTop { get { return MyFlexGrid.Top; } }
|
|
public override void SetupHeader(ItemInfo itemInfo) { ;} // tables do not have headers
|
|
public override void ShowExpanded() {;}
|
|
public override void SetText()
|
|
{
|
|
// if this is an RO Table, regenerate xml. This is done in the case that
|
|
// the rotable was updated by the ro editor.
|
|
//if (MyFlexGrid.IsRoTable) RefreshGridData();
|
|
RefreshDisplay(false);
|
|
IdentifyMe(false);
|
|
}
|
|
|
|
private void RefreshGridData()
|
|
{
|
|
string ROID = MyFlexGrid.ROID;
|
|
int rodbid = MyFlexGrid.RODbId;
|
|
MyFlexGrid.Clear();
|
|
ROFSTLookup MyROFSTLookup = MyItemInfo.MyDocVersion.DocVersionAssociations[0].MyROFst.ROFSTLookup;
|
|
ConvertTableToGrid(MyROFSTLookup.GetRoValue(ROID));
|
|
MyFlexGrid.RODbId = rodbid;
|
|
MyFlexGrid.ROID = ROID;
|
|
MyFlexGrid.IsRoTable = true;
|
|
SaveContents();
|
|
}
|
|
public override void SetExpandAndExpander(ItemInfo itemInfo) { CanExpand = false; } // can't expand a table
|
|
public void SavePastedCellRoTran()
|
|
{
|
|
if (!MyFlexGrid.GetXMLData().Contains("<NewID>")) return;
|
|
BasicSave();
|
|
// need to loop thru all pasted cells: for now do all cells.
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
int r = 0;
|
|
int c = 0;
|
|
String Rtf = null;
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
Rtf = (string)MyFlexGrid[r, c];
|
|
if (Rtf != null)
|
|
{
|
|
int startIndx = 0;
|
|
while (Rtf.IndexOf("<NewID>") > -1)
|
|
{
|
|
// first find the new link and determine whether it's RO or transition.
|
|
int indx = Rtf.IndexOf(@"#Link:ReferencedObject:<NewID>", startIndx);
|
|
if (indx > 0)
|
|
{
|
|
// only look in the substring limited by the first occurrance of [END>. If there is more
|
|
// than one, don't get multiple links in the string.
|
|
int endindx = Rtf.IndexOf(@"END>", indx) + 4;
|
|
Match mro = Regex.Match(Rtf.Substring(indx, endindx - indx), @"([A-Za-z]*):(.*)\[END>");
|
|
string linkstr = mro.Groups[2].Value;
|
|
string[] roparts = linkstr.Split(" ".ToCharArray());
|
|
ContentRoUsage rousg = null;
|
|
int oldid = -1;
|
|
using (Item itm = MyItemInfo.Get())
|
|
{
|
|
using (RODb rodb = RODb.GetJustRoDb(Convert.ToInt32(roparts[2])))
|
|
{
|
|
rousg = itm.MyContent.ContentRoUsages.Add(roparts[1], rodb);
|
|
}
|
|
oldid = rousg.ROUsageID;
|
|
int newid = Rtf.IndexOf("<NewID>", indx);
|
|
Rtf = Rtf.Remove(newid, 7);
|
|
Rtf = Rtf.Insert(newid, string.Format("<CROUSGID={0}>", rousg.ROUsageID));
|
|
itm.Save();
|
|
Rtf = Rtf.Replace(string.Format("<CROUSGID={0}>", oldid), rousg.ROUsageID.ToString());
|
|
itm.Save();
|
|
MyItemInfo.MyContent.RefreshContentRoUsages();
|
|
}
|
|
startIndx = endindx;
|
|
}
|
|
else
|
|
{
|
|
Match mt = Regex.Match(Rtf, @"#Link:Transition:([0-9]+) <NewID> ");
|
|
if (mt.Length <= 0) mt = Regex.Match(Rtf, @"#Link:TransitionRange:([0-9]+) <NewID> ");
|
|
if (mt.Length > 0)
|
|
{
|
|
int endtindx = Rtf.IndexOf("END>", mt.Index) + 4;
|
|
indx = mt.Index + 6; // get past '#Link:"
|
|
Match m = Regex.Match(Rtf.Substring(indx, endtindx - indx), @"([A-Za-z]*):(.*)\[END>");
|
|
bool isSingleTran = true;
|
|
if (Rtf.Substring(indx, 16).IndexOf("TransitionRange") >= 0) isSingleTran = false;
|
|
string linkstr = m.Groups[2].Value;
|
|
string[] tparts = linkstr.Split(" ".ToCharArray());
|
|
int type = System.Convert.ToInt32(tparts[0]);
|
|
int tr1 = System.Convert.ToInt32(tparts[2]); // tparts[2] is token for tranid
|
|
int tr2 = tr1;
|
|
if (tparts.Length > 3)
|
|
tr2 = System.Convert.ToInt32(tparts[3]); // tparts[3] is token for rangeid
|
|
bool dispose1 = false;
|
|
Item itm1 = null;
|
|
bool dispose2 = false;
|
|
Item itm2 = null;
|
|
using (Item itm = MyItemInfo.Get())
|
|
{
|
|
if (itm.ItemID == tr1)
|
|
itm1 = itm; // a transition that points to itself should not dispose
|
|
else
|
|
{
|
|
dispose1 = true;
|
|
itm1 = Item.Get(tr1);
|
|
}
|
|
if (itm.ItemID == tr2)
|
|
itm2 = itm; // a transition that points to itself should not dispose
|
|
else if (tr1 == tr2)
|
|
itm2 = itm1; // if both destinations are the same only dispose the first one
|
|
else
|
|
{
|
|
dispose2 = true;
|
|
itm2 = Item.Get(tr2);
|
|
}
|
|
ContentTransition ct = itm.MyContent.ContentTransitions.Add(itm1, itm2);
|
|
//Console.WriteLine("CT {0},{1},{2},{3}", ct.TransitionID, itm.ItemID, itm.MyContent.MyContentUnique, itm.MyContent.ContentTransitions.Count);
|
|
ct.TranType = type;
|
|
|
|
if (isSingleTran)
|
|
ct.IsRange = 0;
|
|
else if (tr1 != tr2)
|
|
ct.IsRange = 1;
|
|
else
|
|
ct.IsRange = 2;
|
|
|
|
int oldidt = ct.TransitionID;
|
|
int newidt = Rtf.IndexOf("<NewID>", indx);
|
|
Rtf = Rtf.Remove(newidt, 7);
|
|
Rtf = Rtf.Insert(newidt, string.Format("<CTID={0}>", ct.TransitionID));
|
|
//Rtf = Rtf.Replace("<NewID>", string.Format("<CTID={0}>", ct.TransitionID));
|
|
itm.Save();
|
|
Rtf = Rtf.Replace(string.Format("<CTID={0}>", oldidt), ct.TransitionID.ToString());
|
|
itm.Save();
|
|
MyItemInfo.MyContent.RefreshContentTransitions();
|
|
}
|
|
if (dispose2) itm2.Dispose();
|
|
if (dispose1) itm1.Dispose();
|
|
}
|
|
}
|
|
}
|
|
MyFlexGrid[r, c] = Rtf;
|
|
}
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
}
|
|
}
|
|
public override void SaveCurrentAndContents()
|
|
{
|
|
if (MyFlexGrid.Row >= 0 && MyFlexGrid.Col >= 0 && MyStepRTB.EditMode) // Only if a Cell is Selected
|
|
{
|
|
int row = MyFlexGrid.Row;
|
|
int col = MyFlexGrid.Col;
|
|
SaveContents();
|
|
MyStepRTB.Rtf = MyStepRTB.DoNewLinkInGridCell();
|
|
MyFlexGrid.Row = row;
|
|
MyFlexGrid.Col = col;
|
|
MyFlexGrid[MyFlexGrid.Row, MyFlexGrid.Col] = MyStepRTB.Rtf;
|
|
}
|
|
SaveContents();
|
|
}
|
|
#endregion
|
|
#region Miscellaneous Support
|
|
internal void SetSearchCell(string SearchString)
|
|
{
|
|
// Walk thru grid to find first occurance of SearchString and set that cell for
|
|
// edit.
|
|
int r = 0;
|
|
int c = 0;
|
|
int w = MyFlexGrid.Cols.Count;
|
|
int h = MyFlexGrid.Rows.Count;
|
|
while (r < h)
|
|
{
|
|
CellRange cr = MyFlexGrid.GetMergedRange(r, c);
|
|
if (cr.r1 == r && cr.c1 == c)
|
|
{
|
|
if (MyFlexGrid[r, c] != null)
|
|
{
|
|
MyFlexGrid.StartEditing(r, c);
|
|
TableCellEditor tce = MyFlexGrid.TableCellEditor;
|
|
// note that this method is only used from Search Results.
|
|
// The search query will handle the case sensitivity, so
|
|
// we can just see if contains the string without considering
|
|
// case sensitivity.
|
|
if (tce.Text.ToUpper().Contains(SearchString.ToUpper()))
|
|
return;
|
|
}
|
|
}
|
|
c = c + 1;
|
|
if (c == w)
|
|
{
|
|
c = 0;
|
|
r = r + 1;
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
}
|