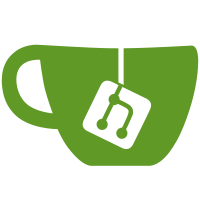
Add Memory Usage summary to the log file. GetJustFormat to reduce memory use Fix code so that children are not disposed while they are being used Added new method GetJustFormat to reduce memory use Fix logic so that content is not used after it is disposed Verify that Content object is not disposed before using Text Dispose of parts when Content object is disposed Verify that ContentInfo object is not disposed before using Text Dispose of parts when ContentInfo object is disposed Place brackets around DB names to support names containing periods. Dispose of parts when Item object is disposed Removed inapproriate Dispose in MakeItem Dispose of parts when ItemInfo object is disposed Remove event handler when ItemInfoList object is disposed Dispose of parts when Transtion object is disposed Dispose of parts when ZTransition object is disposed
474 lines
14 KiB
C#
474 lines
14 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.Xml;
|
|
using System.Drawing;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class Format
|
|
{
|
|
public static Format GetJustFormat(int formatID)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Format");
|
|
try
|
|
{
|
|
Format tmp = GetCachedByPrimaryKey(formatID);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Format>(new PKCriteriaJustFormat(formatID));
|
|
AddToCache(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up Format
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Format.GetJustFormat", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
protected class PKCriteriaJustFormat
|
|
{
|
|
private int _FormatID;
|
|
public int FormatID
|
|
{ get { return _FormatID; } }
|
|
public PKCriteriaJustFormat(int formatID)
|
|
{
|
|
_FormatID = formatID;
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PKCriteriaJustFormat criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Format.DataPortal_Fetch JustFormat", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getJustFormat";
|
|
cm.Parameters.AddWithValue("@FormatID", criteria.FormatID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Format.DataPortal_Fetch JustFormat", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Format.DataPortal_Fetch JustFormat", ex);
|
|
}
|
|
}
|
|
|
|
}
|
|
public partial interface IFormatOrFormatInfo
|
|
{
|
|
PlantFormat PlantFormat { get;}
|
|
IFormatOrFormatInfo MyIParent { get;}
|
|
string Data { get; }
|
|
string ToString();
|
|
string FullName { get; }
|
|
}
|
|
public class FormatEventArgs
|
|
{
|
|
private string _Status;
|
|
public string Status
|
|
{
|
|
get { return _Status; }
|
|
set { _Status = value; }
|
|
}
|
|
public FormatEventArgs(string status)
|
|
{
|
|
_Status = status;
|
|
}
|
|
}
|
|
public delegate void FormatEvent(object sender,FormatEventArgs args);
|
|
public partial class Format:IFormatOrFormatInfo
|
|
{
|
|
#region PlantFormat
|
|
[NonSerialized]
|
|
private PlantFormat _PlantFormat;
|
|
public PlantFormat PlantFormat
|
|
{ get { return (_PlantFormat != null ? _PlantFormat : _PlantFormat = new PlantFormat(this)); } }
|
|
#endregion
|
|
public static event FormatEvent FormatLoaded;
|
|
private static void OnFormatLoaded(object sender, FormatEventArgs args)
|
|
{
|
|
if (FormatLoaded != null)
|
|
FormatLoaded(sender, args);
|
|
}
|
|
public IFormatOrFormatInfo MyIParent { get { return MyParent; } }
|
|
public override string ToString()
|
|
{
|
|
//return Name;
|
|
//return PlantFormat.FormatData.Name;
|
|
return FullName;
|
|
}
|
|
public string FullName
|
|
{
|
|
get
|
|
{
|
|
if (ParentID == 1) return Description + " (" + Name + ")";
|
|
return MyParent.Description + " - " + Description + " (" + Name + ")";
|
|
}
|
|
}
|
|
|
|
public static void UpdateFormats(string fmtPath, string genmacPath)
|
|
{
|
|
Format basefmt = null;
|
|
Format parent = null;
|
|
|
|
|
|
// Load base format.
|
|
basefmt = AddFormatToDB(null, "base", false, DateTime.Now, "Migration", fmtPath, genmacPath);
|
|
if (basefmt == null) return;
|
|
// now loop through all files... If there is an _ in the name, it's a subformat
|
|
// (for example, AEP_00.xml) skip it in main loop, it's handled for each format.
|
|
DirectoryInfo di = new DirectoryInfo(fmtPath); //(@"c:\development\fmtall");
|
|
FileInfo[] fis = di.GetFiles("*.xml");
|
|
|
|
foreach (FileInfo fi in fis)
|
|
{
|
|
//if (fi.Name.ToUpper() == "WCN2ALL.XML"|| fi.Name.ToUpper() == "OHLPALL.XML")
|
|
//{
|
|
bool issub = (fi.Name.IndexOf("_") > 0) ? true : false;
|
|
if (!issub && fi.Name.ToLower() != "baseall.xml")
|
|
{
|
|
string fmtname = fi.Name.Substring(0, fi.Name.Length - 7);
|
|
// remove the all.xml part of the filename.
|
|
try
|
|
{
|
|
parent = AddFormatToDB(basefmt, fmtname, issub, DateTime.Now, "Migration", fmtPath, genmacPath);
|
|
if (parent != null)
|
|
{
|
|
// now see if there are any subformats associated with this, if so
|
|
// add them here...
|
|
DirectoryInfo sdi = new DirectoryInfo(fmtPath); //("c:\\development\\fmtall");
|
|
FileInfo[] sfis = di.GetFiles(fmtname + "_*.xml");
|
|
foreach (FileInfo sfi in sfis)
|
|
{
|
|
string nm = sfi.Name.Substring(0, sfi.Name.Length - 7);
|
|
OnFormatLoaded(null, new FormatEventArgs("Loading Format " + sfi.Name));
|
|
//Console.WriteLine("Processing {0}", sfi.Name);
|
|
//frmMain.Status = string.Format("Processing Format {0}", sfi.Name);
|
|
try
|
|
{
|
|
AddFormatToDB(parent, nm, true, DateTime.Now, "Migration",fmtPath,genmacPath);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//frmMain.AddError(ex, "LoadAllFormats() '{0}'", sfi.Name);
|
|
Console.WriteLine("{0} - {1}", ex.GetType().Name, ex.Message);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//frmMain.AddError(ex, "LoadAllFormats() '{0}'", fi.Name);
|
|
Console.WriteLine("{0} - {1}", ex.GetType().Name, ex.Message);
|
|
}
|
|
}
|
|
//}
|
|
}
|
|
OnFormatLoaded(null, new FormatEventArgs("Formats Updated"));
|
|
}
|
|
|
|
private static Dictionary<string, int> _LookupFormats;
|
|
public static Dictionary<string, int> LookupFormats
|
|
{
|
|
get
|
|
{
|
|
if (_LookupFormats == null)
|
|
{
|
|
_LookupFormats = new Dictionary<string, int>();
|
|
FormatInfoList allFormats = FormatInfoList.Get();
|
|
foreach (FormatInfo myFormat in allFormats)
|
|
{
|
|
_LookupFormats.Add(myFormat.Name, myFormat.FormatID);
|
|
}
|
|
}
|
|
return _LookupFormats;
|
|
}
|
|
}
|
|
|
|
private static Format AddFormatToDB(Format parent, string format, bool issub, DateTime Dts, string Userid, string fmtPath, string genmacPath)
|
|
{
|
|
string fmtdata = null;
|
|
string genmacdata = null;
|
|
XmlDocument xd = null;
|
|
|
|
OnFormatLoaded(null, new FormatEventArgs("Loading Format "+format));
|
|
//string path = "c:\\development\\fmtall\\" + format + "all.xml";
|
|
string path = fmtPath + "\\" + format + "all.xml";
|
|
if (File.Exists(path))
|
|
{
|
|
try
|
|
{
|
|
StreamReader srf = new StreamReader(path);
|
|
xd = new XmlDocument();
|
|
xd.Load(srf);
|
|
//xd.Load(path);
|
|
fmtdata = xd.OuterXml;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//frmMain.AddError(ex, "AddFormatToDB('{0}','{1}')", parent.Name, path);
|
|
return null;
|
|
}
|
|
}
|
|
|
|
// Do we need genmac - only if non-subformat
|
|
if (!issub)
|
|
{
|
|
//path = "c:\\development\\genmacall\\" + format + ".svg";
|
|
path = genmacPath + "\\" + format + ".svg";
|
|
if (File.Exists(path))
|
|
{
|
|
try
|
|
{
|
|
StreamReader sr = new StreamReader(path);
|
|
XmlDocument xdg = new XmlDocument();
|
|
xdg.Load(sr);
|
|
//xdg.Load(path);
|
|
genmacdata = xdg.OuterXml;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//frmMain.AddError(ex, "AddFormatToDB('{0}','{1}')", parent.Name, path);
|
|
return null;
|
|
}
|
|
}
|
|
}
|
|
// Get the name & then create the record.
|
|
if (Userid == null || Userid == "") Userid = "Migration";
|
|
|
|
string nmattr = "Default";
|
|
|
|
// use xpath to get name.
|
|
if (parent != null)
|
|
{
|
|
XmlNode nmnode = xd.SelectSingleNode("//FormatData");
|
|
if (nmnode is XmlElement)
|
|
{
|
|
XmlElement xm = (XmlElement)nmnode;
|
|
nmattr = xm.GetAttribute("Name");
|
|
}
|
|
}
|
|
// use the format name if base or non sub. If it's a sub, remove the "_".
|
|
string fname = issub ? format.Replace("_", "") : format;
|
|
Format rec = null;
|
|
try
|
|
{
|
|
if (!LookupFormats.ContainsKey(fname))
|
|
{
|
|
rec = Format.MakeFormat(parent, fname, nmattr, fmtdata, genmacdata, Dts, Userid);
|
|
}
|
|
else
|
|
{
|
|
rec = Format.Get(LookupFormats[fname]);
|
|
rec.Data = fmtdata;
|
|
rec.GenMac = genmacdata;
|
|
rec = rec.Save();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
//frmMain.AddError(ex, "AddFormatToDB-make format('{0}','{1}')", parent.Name, path);
|
|
}
|
|
return rec;
|
|
}
|
|
}
|
|
public partial class FormatInfo : IFormatOrFormatInfo
|
|
{
|
|
public virtual Format GetJustFormat()
|
|
{
|
|
return _Editable = Format.GetJustFormat(_FormatID);
|
|
}
|
|
public static bool HasLatestChanges()
|
|
{
|
|
if (!HasSeqTabFmtTabToken()) return false;
|
|
return true;
|
|
}
|
|
private static bool HasSeqTabFmtTabToken()
|
|
{
|
|
using (FormatInfo fi = FormatInfo.Get("WCN2"))
|
|
{
|
|
XmlDocument xd = new XmlDocument();
|
|
xd.LoadXml(fi.Data);
|
|
XmlNodeList xl = xd.SelectNodes("//FormatData/SectData/StepSectionData/SequentialTabFormat/SeqTabFmt/@TabToken");//"//DocStyle/Layout/@TopMargin");
|
|
if (xl.Count == 0)
|
|
{
|
|
System.Windows.Forms.MessageBox.Show("FormatData SeqTab/TabToken is missing", "Inconsistent Format Files", System.Windows.Forms.MessageBoxButtons.OK, System.Windows.Forms.MessageBoxIcon.Error);
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
}
|
|
public static FormatInfo Get(string name)
|
|
{
|
|
try
|
|
{
|
|
FormatInfo tmp = DataPortal.Fetch<FormatInfo>(new NameCriteria(name));
|
|
//AddToCache(tmp);
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up FormatInfo
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex1)
|
|
{
|
|
Exception ex = ex1;
|
|
while (ex.InnerException != null)
|
|
ex = ex.InnerException;
|
|
if (ex.Message.StartsWith("Could not find stored procedure"))
|
|
{
|
|
int formatID = 0;
|
|
using (FormatInfoList fil = FormatInfoList.Get())
|
|
{
|
|
foreach (FormatInfo fi in fil)
|
|
{
|
|
if (fi.Name == name)
|
|
{
|
|
formatID = fi.FormatID;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
if (formatID != 0) return FormatInfo.Get(formatID);
|
|
throw new DbCslaException("Format not found " + name, ex);
|
|
}
|
|
else
|
|
throw new DbCslaException("Error on FormatInfo.Get By Name", ex);
|
|
}
|
|
}
|
|
protected class NameCriteria
|
|
{
|
|
private string _Name;
|
|
public string Name
|
|
{ get { return _Name; } }
|
|
public NameCriteria(string name)
|
|
{
|
|
_Name = name;
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(NameCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] FormatInfo.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getFormatByName";
|
|
cm.Parameters.AddWithValue("@Name", criteria.Name);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("FormatInfo.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("FormatInfo.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
#region PlantFormat
|
|
[NonSerialized]
|
|
private PlantFormat _PlantFormat;
|
|
public PlantFormat PlantFormat
|
|
{ get { return (_PlantFormat != null ? _PlantFormat : _PlantFormat = new PlantFormat(this)); } }
|
|
#endregion
|
|
public IFormatOrFormatInfo MyIParent { get { return MyParent; } }
|
|
public override string ToString()
|
|
{
|
|
//return Name;
|
|
//return PlantFormat.FormatData.Name;
|
|
return FullName;
|
|
}
|
|
public string FullName
|
|
{
|
|
get
|
|
{
|
|
if (ParentID == 1) return Description + " (" + Name + ")";
|
|
return MyParent.Description + " - " + Description + " (" + Name + ")";
|
|
}
|
|
}
|
|
public StepSectionLayoutData MyStepSectionLayoutData
|
|
{
|
|
get { return PlantFormat.FormatData.SectData.StepSectionData.StepSectionLayoutData; }
|
|
}
|
|
public StepSectionPrintData MyStepSectionPrintData
|
|
{
|
|
get { return PlantFormat.FormatData.SectData.StepSectionData.StepSectionPrintData; }
|
|
}
|
|
}
|
|
public partial class FormatInfoList
|
|
{
|
|
private static Csla.SortedBindingList<FormatInfo> _SortedFormatInfoList;
|
|
public static Csla.SortedBindingList<FormatInfo> SortedFormatInfoList
|
|
{
|
|
get
|
|
{
|
|
if (_SortedFormatInfoList == null)
|
|
{
|
|
_SortedFormatInfoList = new SortedBindingList<FormatInfo>(FormatInfoList.Get());
|
|
_SortedFormatInfoList.ApplySort("FullName", ListSortDirection.Ascending);
|
|
}
|
|
return _SortedFormatInfoList;
|
|
}
|
|
}
|
|
}
|
|
}
|