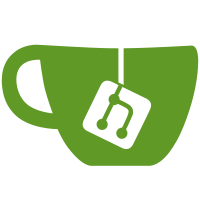
Add Memory Usage summary to the log file. GetJustFormat to reduce memory use Fix code so that children are not disposed while they are being used Added new method GetJustFormat to reduce memory use Fix logic so that content is not used after it is disposed Verify that Content object is not disposed before using Text Dispose of parts when Content object is disposed Verify that ContentInfo object is not disposed before using Text Dispose of parts when ContentInfo object is disposed Place brackets around DB names to support names containing periods. Dispose of parts when Item object is disposed Removed inapproriate Dispose in MakeItem Dispose of parts when ItemInfo object is disposed Remove event handler when ItemInfoList object is disposed Dispose of parts when Transtion object is disposed Dispose of parts when ZTransition object is disposed
87 lines
1.7 KiB
C#
87 lines
1.7 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.IO;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class frmErrors : Form
|
|
{
|
|
private Form _MyParent;
|
|
public frmErrors(Form myParent)
|
|
{
|
|
InitializeComponent();
|
|
_MyParent = myParent;
|
|
}
|
|
private int _ErrorCount = 0;
|
|
|
|
public int ErrorCount
|
|
{
|
|
get { return _ErrorCount; }
|
|
set { _ErrorCount = value; }
|
|
}
|
|
private int _WarningCount = 0;
|
|
public int WarningCount
|
|
{
|
|
get { return _WarningCount; }
|
|
set { _WarningCount = value; }
|
|
}
|
|
private int _InfoCount = 0;
|
|
public int InfoCount
|
|
{
|
|
get { return _InfoCount; }
|
|
set { _InfoCount = value; }
|
|
}
|
|
string sep = "";
|
|
public void Add(string err, MessageType messageType)
|
|
{
|
|
switch (messageType)
|
|
{
|
|
case MessageType.Information:
|
|
_InfoCount++;
|
|
break;
|
|
case MessageType.Warning:
|
|
_WarningCount++;
|
|
break;
|
|
case MessageType.Error:
|
|
_ErrorCount++;
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
tbErrors.SelectionStart = tbErrors.TextLength;
|
|
tbErrors.SelectedText = sep + err;
|
|
sep = "\r\n";
|
|
Show();
|
|
Application.DoEvents();
|
|
}
|
|
public void Clear()
|
|
{
|
|
tbErrors.Text = "";
|
|
sep = "";
|
|
Hide();
|
|
}
|
|
private void frmErrors_Load(object sender, EventArgs e)
|
|
{
|
|
Rectangle rec = Screen.GetWorkingArea(this);
|
|
Top = _MyParent.Top;
|
|
Left = _MyParent.Right + Width > rec.Right ? rec.Right - Width : _MyParent.Right;
|
|
}
|
|
public void Save(string path)
|
|
{
|
|
StreamWriter fs = new StreamWriter(path, false);
|
|
fs.Write(tbErrors.Text);
|
|
fs.Close();
|
|
}
|
|
}
|
|
public enum MessageType
|
|
{
|
|
Information,
|
|
Warning,
|
|
Error
|
|
}
|
|
} |