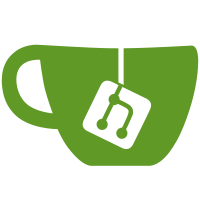
Use Command Line Parameters ass override for settings. Add logic to support partial processing of procedure sets (appending to a previously processed set.
517 lines
16 KiB
C#
517 lines
16 KiB
C#
//using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.IO;
|
|
using System.Data.OleDb;
|
|
using System.Data;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
public bool BuildApprovedRevision()
|
|
{
|
|
if(!frmMain.RunScript("PROMSApproveApprove.sql", frmMain.DBName)) return false;
|
|
bool rv = false;
|
|
using (Stage myStage = GetApprovedStage())
|
|
{
|
|
if (myStage == null)
|
|
return rv;
|
|
DocVersionInfoList dvil = DocVersionInfoList.Get();
|
|
foreach (DocVersionInfo dvi in dvil)
|
|
{
|
|
if (dvi.Procedures.Count > 0)
|
|
{
|
|
if (!frmMain.MySettings.OnlyThisSet || frmMain.ProcedureSetPath.ToUpper() == dvi.MyFolder.Title.ToUpper())
|
|
{
|
|
string approvedFolder = dvi.MyFolder.Title + @"\APPROVED";
|
|
//B2012-094 fix
|
|
if (!Directory.Exists(approvedFolder)) frmMain.MyWarning = "**** " + dvi.MyFolder.Title + " Approved folder does not exist";
|
|
else if (!File.Exists(approvedFolder + @"\SET.DBF")) frmMain.MyWarning = "**** " + dvi.MyFolder.Title + " Approved Set.dbf does not exist";
|
|
else if (!File.Exists(approvedFolder + @"\USAGERO.DBF")) frmMain.MyWarning = "**** " + dvi.MyFolder.Title + " Approved UsageRO.dbf does not exist";
|
|
else if (!Directory.Exists(approvedFolder + @"\PDFS")) frmMain.MyWarning = "**** " + dvi.MyFolder.Title + " Approved Pdfs folder does not exist";
|
|
else if (Directory.GetFiles(approvedFolder + @"\PDFS","*.PDF").Length == 0) frmMain.MyWarning = "**** " + dvi.MyFolder.Title + " Approved Pdfs folder does not contain any pdf files";
|
|
else rv |= BuildApprovedRevision(myStage, approvedFolder, dvi);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return rv;
|
|
}
|
|
|
|
private static Stage GetApprovedStage()
|
|
{
|
|
StageInfoList sil = StageInfoList.Get();
|
|
foreach (StageInfo si in sil)
|
|
{
|
|
if (si.Name.ToUpper() == "APPROVED")
|
|
return si.Get();
|
|
}
|
|
return null;
|
|
}
|
|
private bool BuildApprovedRevision(Stage myStage, string approvedFolder, DocVersionInfo dvi)
|
|
{
|
|
bool rv = false;
|
|
if (dvi.MultiUnitCount <= 1)
|
|
{
|
|
ApprovedFolder af = new ApprovedFolder(approvedFolder);
|
|
foreach (ProcedureInfo pi in dvi.Procedures)
|
|
{
|
|
rv |= BuildApprovedRevision(myStage, af, pi, 0);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
ApprovedFolder af = new ApprovedFolder(approvedFolder, dvi);
|
|
foreach (ProcedureInfo pi in dvi.Procedures)
|
|
{
|
|
for (int i = 1; i <= dvi.MultiUnitCount; i++)
|
|
rv |= BuildApprovedRevision(myStage, af, pi, i);
|
|
}
|
|
}
|
|
return rv;
|
|
}
|
|
private bool BuildApprovedRevision(Stage myStage, ApprovedFolder af, ProcedureInfo pi, int selectedSlave)
|
|
{
|
|
bool rv = false;
|
|
if (selectedSlave > 0)
|
|
pi.MyDocVersion.DocVersionConfig.SelectedSlave = selectedSlave;
|
|
frmMain.MyInfo = string.Format("Loading approved data for Procedure: {0}", pi.DisplayNumber);
|
|
// if working draft procedure does not exist in approved folder, don't bother processing
|
|
if (!af.EntryFromProc.ContainsKey(pi.DisplayNumber)) return rv;
|
|
using (Revision revision = CreateRevision(af, pi))
|
|
{
|
|
System.DateTime dts = pi.DTS;
|
|
string userID = af.EntryFromProc[pi.DisplayNumber].UserID;
|
|
using (Version version = Version.MakeVersion(revision, myStage, GetPDF(af,pi), GetSummaryPDF(), dts, userID))
|
|
{
|
|
using (Check check = Check.MakeCheck(revision, myStage, af.BuildConsistencyChecks(pi)))
|
|
{
|
|
rv |= true;
|
|
}
|
|
}
|
|
}
|
|
if(!rv)
|
|
frmMain.MyInfo = string.Format("Loading Procedure: {0} FAILED MISERABLY", pi.DisplayNumber);
|
|
return rv;
|
|
}
|
|
private byte[] GetSummaryPDF()
|
|
{
|
|
return null;
|
|
}
|
|
private byte[] GetPDF(ApprovedFolder af, ProcedureInfo pi)
|
|
{
|
|
string pdf = string.Format(@"{0}\PDFS\{1}.pdf", af.Path, pi.DisplayNumber);
|
|
FileInfo pdfFile = new FileInfo(pdf);
|
|
if (pdfFile.Exists)
|
|
{
|
|
FileStream fs = pdfFile.Open(FileMode.Open, FileAccess.Read, FileShare.ReadWrite);
|
|
byte[] pdfBuf = new byte[pdfFile.Length];
|
|
fs.Read(pdfBuf, 0, pdfBuf.Length);
|
|
fs.Close();
|
|
return pdfBuf;
|
|
}
|
|
return null;
|
|
}
|
|
private Revision CreateRevision(ApprovedFolder af, ProcedureInfo pi)
|
|
{
|
|
int typeID = 1; //revision not tempmod
|
|
ProcInfo pinf = af.EntryFromProc[pi.DisplayNumber];
|
|
string revisionNumber = pinf.Rev;
|
|
System.DateTime revisionDate = System.DateTime.Parse(pinf.RevDate ?? "1/1/1980");
|
|
string notes = "Migration Of Current Approved Revision";
|
|
RevisionConfig cfg = new RevisionConfig();
|
|
cfg.History_StartDate = pinf.StartDateTime;
|
|
if (pi.MyDocVersion.DocVersionConfig.SelectedSlave > 0)
|
|
cfg.Applicability_Index = pi.MyDocVersion.DocVersionConfig.SelectedSlave;
|
|
string config = cfg.ToString();
|
|
System.DateTime dts = pi.DTS;
|
|
string userID = pinf.UserID;
|
|
return Revision.MakeRevision(pi.ItemID, typeID, revisionNumber, revisionDate, notes, config, dts, userID);
|
|
}
|
|
}
|
|
public partial class ApprovedFolder
|
|
{
|
|
private OleDbConnection _Connection;
|
|
public OleDbConnection Connection
|
|
{
|
|
get
|
|
{
|
|
if(_Connection == null)
|
|
_Connection = new OleDbConnection(@"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + _Path + ";Extended Properties=dBase III;Persist Security Info=False");
|
|
return _Connection;
|
|
}
|
|
}
|
|
private string _Path;
|
|
public string Path
|
|
{
|
|
get { return _Path; }
|
|
set { _Path = value; }
|
|
}
|
|
private System.DateTime _ROFstDate = System.DateTime.MinValue;
|
|
public System.DateTime ROFstDate
|
|
{
|
|
get
|
|
{
|
|
if (_ROFstDate == System.DateTime.MinValue)
|
|
{
|
|
FileInfo fi = new FileInfo(string.Format(@"{0}\ro.fst",Path));
|
|
if(fi.Exists)
|
|
_ROFstDate = fi.LastWriteTimeUtc;
|
|
}
|
|
return _ROFstDate;
|
|
}
|
|
}
|
|
|
|
private Dictionary<string, string> _ROValues = new Dictionary<string, string>();
|
|
private Dictionary<string, System.DateTime> _LibDocDates = new Dictionary<string,System.DateTime>();
|
|
private Dictionary<string, List<string>> _ProcROs = new Dictionary<string,List<string>>();
|
|
private Dictionary<string, List<string>> _LibROs = new Dictionary<string,List<string>>();
|
|
private Dictionary<string, List<string>> _LibDocs = new Dictionary<string,List<string>>();
|
|
|
|
public string BuildConsistencyChecks(ProcedureInfo proc)
|
|
{
|
|
/*
|
|
<ConsistencyChecks ItemID="1">
|
|
<ROChecks ROFstDate="2011-04-11T14:13:44">
|
|
<ROCheck ROID="0001000003960000" ROValue="1430 PSIG" />
|
|
</ROChecks>
|
|
<LibDocChecks>
|
|
<LibDocCheck DocID="1" DocDate="2011-12-06T11:12:40.633" />
|
|
</LibDocChecks>
|
|
</ConsistencyChecks>
|
|
*/
|
|
ConsistencyChecks cc = new ConsistencyChecks();
|
|
cc.ItemID = proc.ItemID;
|
|
BuildROChecks(cc, proc);
|
|
BuildLibDocChecks(cc, proc);
|
|
return cc.ToString().Replace("OldDocDate=\"0001-01-01T00:00:00\"", "");
|
|
}
|
|
|
|
private void BuildLibDocChecks(ConsistencyChecks cc, ProcedureInfo proc)
|
|
{
|
|
if (_LibDocs.ContainsKey(proc.DisplayNumber))
|
|
{
|
|
foreach (string libdoc in _LibDocs[proc.DisplayNumber])
|
|
{
|
|
FileInfo fi = new FileInfo(string.Format(@"{0}\RTFFILES\{1}.LIB",Path,libdoc));
|
|
if (fi.Exists)
|
|
{
|
|
//if (proc.MyDocVersion.VersionID > 1)
|
|
// System.Threading.Thread.Sleep(5000);
|
|
int docid = GetDocID(libdoc, proc.MyDocVersion.VersionID);
|
|
if (docid > 0)
|
|
cc.LibDocConsistency.AddLibDocCheck(docid, fi.LastWriteTimeUtc);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
private int GetDocID(string libdoc, int versionid)
|
|
{
|
|
DocumentInfo di = DocumentInfo.GetByLibDocName(libdoc, versionid);
|
|
return di.DocID;
|
|
}
|
|
|
|
private void BuildROChecks(ConsistencyChecks cc, ProcedureInfo proc)
|
|
{
|
|
cc.ROConsistency.ROFstDate = ROFstDate;
|
|
if (_ProcROs.ContainsKey(proc.DisplayNumber))
|
|
{
|
|
foreach (string roid in _ProcROs[proc.DisplayNumber])
|
|
cc.ROConsistency.AddROCheck(FormatROID(roid), GetROValue(FormatROID(roid)));
|
|
}
|
|
//int cnt = cc.ROConsistency.MyROChecks.Length;
|
|
if (_LibDocs.ContainsKey(proc.DisplayNumber))
|
|
{
|
|
foreach (string libdoc in _LibDocs[proc.DisplayNumber])
|
|
{
|
|
if (_LibROs.ContainsKey(libdoc))
|
|
{
|
|
foreach (string roid in _LibROs[libdoc])
|
|
cc.ROConsistency.AddROCheck(FormatROID(roid), GetROValue(FormatROID(roid)));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
private string GetROValue(string roid)
|
|
{
|
|
if (!_ROValues.ContainsKey(roid))
|
|
{
|
|
//if (roid.Contains("000128"))
|
|
// System.Console.WriteLine();
|
|
//string ss = _Lookup.GetRoValue(roid);
|
|
//ss = ItemInfo.ConvertToDisplayText(ss);
|
|
//ss = ss.Replace('\n', ';');
|
|
|
|
_ROValues.Add(roid, PrepareForXML(ItemInfo.ConvertToDisplayText(_Lookup.GetRoValue(roid))));
|
|
}
|
|
return _ROValues[roid];
|
|
}
|
|
|
|
private string PrepareForXML(string rovalue)
|
|
{
|
|
StringBuilder sb = new StringBuilder();
|
|
foreach (char c in rovalue)
|
|
{
|
|
if (c < ' ')
|
|
sb.Append(string.Format(@"\x{0:X4}", (int)c));
|
|
else
|
|
sb.Append(c);
|
|
}
|
|
return sb.ToString();
|
|
}
|
|
private string FormatROID(string roid)
|
|
{
|
|
if (roid.Length == 16 && roid.EndsWith("0000"))
|
|
return roid.Substring(0, 12).ToUpper();
|
|
return roid.ToUpper();
|
|
}
|
|
|
|
private Dictionary<string, ProcInfo> _EntryFromProc;
|
|
public Dictionary<string, ProcInfo> EntryFromProc
|
|
{
|
|
get
|
|
{
|
|
if (_EntryFromProc == null)
|
|
_EntryFromProc = FillEntryFromProc();
|
|
return _EntryFromProc;
|
|
}
|
|
}
|
|
private Dictionary<string, ProcInfo> FillEntryFromProc()
|
|
{
|
|
Dictionary<string, ProcInfo> rv = new Dictionary<string, ProcInfo>();
|
|
using (OleDbDataAdapter da = new OleDbDataAdapter("Select * from [set] where entry is not null", Connection))
|
|
{
|
|
using (DataSet ds = new DataSet())
|
|
{
|
|
da.Fill(ds);
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
if (!rv.ContainsKey(dr["NUMBER"].ToString()))
|
|
rv.Add(dr["NUMBER"].ToString(), new ProcInfo(dr, Path));
|
|
}
|
|
}
|
|
return rv;
|
|
}
|
|
private ROFSTLookup _Lookup;
|
|
public ApprovedFolder(string path)
|
|
{
|
|
_Path = path;
|
|
FillROs();
|
|
FillLibDocs();
|
|
_Lookup = new ROFSTLookup(string.Format(@"{0}\RO.FST", Path));
|
|
}
|
|
public ApprovedFolder(string path, DocVersionInfo dvi)
|
|
{
|
|
_Path = path;
|
|
FillROs();
|
|
FillLibDocs();
|
|
_Lookup = new ROFSTLookup(string.Format(@"{0}\RO.FST", Path));
|
|
_EntryFromProc = FillEntryFromProcMulti(dvi);
|
|
}
|
|
|
|
private Dictionary<string, ProcInfo> FillEntryFromProcMulti(DocVersionInfo dvi)
|
|
{
|
|
Dictionary<string, ProcInfo> rv = new Dictionary<string, ProcInfo>();
|
|
for (int i = 1; i <= dvi.MultiUnitCount; i++)
|
|
{
|
|
foreach (ProcedureInfo pi in dvi.Procedures)
|
|
{
|
|
pi.MyDocVersion.DocVersionConfig.SelectedSlave = i;
|
|
rv.Add(pi.DisplayNumber, new ProcInfo(pi, Path));
|
|
}
|
|
}
|
|
return rv;
|
|
}
|
|
|
|
private void FillLibDocs()
|
|
{
|
|
using (OleDbDataAdapter da = new OleDbDataAdapter("Select distinct FROMNUMBER, TONUMBER from [tran] where tonumber like 'DOC%'", Connection))
|
|
{
|
|
using (DataSet ds = new DataSet())
|
|
{
|
|
da.Fill(ds);
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
{
|
|
string number = dr["FROMNUMBER"].ToString();
|
|
string libdoc = dr["TONUMBER"].ToString();
|
|
if (!_LibDocs.ContainsKey(number))
|
|
_LibDocs.Add(number, new List<string>());
|
|
if (!_LibDocs[number].Contains(libdoc))
|
|
_LibDocs[number].Add(libdoc);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
private void FillROs()
|
|
{
|
|
using (OleDbDataAdapter da = new OleDbDataAdapter("Select distinct NUMBER, ROID from [usagero]", Connection))
|
|
{
|
|
using (DataSet ds = new DataSet())
|
|
{
|
|
da.Fill(ds);
|
|
foreach (DataRow dr in ds.Tables[0].Rows)
|
|
{
|
|
string number = dr["NUMBER"].ToString();
|
|
string roid = dr["ROID"].ToString();
|
|
if (number.StartsWith("DOC"))
|
|
{
|
|
if (!_LibROs.ContainsKey(number))
|
|
_LibROs.Add(number, new List<string>());
|
|
if (!_LibROs[number].Contains(roid))
|
|
_LibROs[number].Add(roid);
|
|
}
|
|
else
|
|
{
|
|
if (!_ProcROs.ContainsKey(number))
|
|
_ProcROs.Add(number, new List<string>());
|
|
if (!_ProcROs[number].Contains(roid))
|
|
_ProcROs[number].Add(roid);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
public partial class ProcInfo
|
|
{
|
|
private log4net.ILog log;
|
|
private string _Number;
|
|
public string Number
|
|
{
|
|
get { return _Number; }
|
|
set { _Number = value; }
|
|
}
|
|
private string _Entry;
|
|
public string Entry
|
|
{
|
|
get { return _Entry; }
|
|
set { _Entry = value; }
|
|
}
|
|
private string _UserID;
|
|
public string UserID
|
|
{
|
|
get { return _UserID; }
|
|
set { _UserID = value; }
|
|
}
|
|
private System.DateTime _StartDateTime;
|
|
public System.DateTime StartDateTime
|
|
{
|
|
get { return _StartDateTime; }
|
|
set { _StartDateTime = value; }
|
|
}
|
|
private string _Rev;
|
|
public string Rev
|
|
{
|
|
get { return _Rev; }
|
|
set { _Rev = value; }
|
|
}
|
|
private string _RevDate;
|
|
public string RevDate
|
|
{
|
|
get { return _RevDate; }
|
|
set { _RevDate = value; }
|
|
}
|
|
private string _ReviewDate;
|
|
public string ReviewDate
|
|
{
|
|
get { return _ReviewDate; }
|
|
set { _ReviewDate = value; }
|
|
}
|
|
public ProcInfo(ProcedureInfo pi, string afpath)
|
|
{
|
|
Number = pi.DisplayNumber;
|
|
Entry = pi.DisplayText;
|
|
UserID = pi.UserID;
|
|
StartDateTime = pi.DTS;
|
|
RevDate = pi.ProcedureConfig.Print_RevDate;
|
|
Rev = pi.ProcedureConfig.Print_Rev;
|
|
}
|
|
public ProcInfo(DataRow dr, string afpath)
|
|
{
|
|
Number = dr["NUMBER"].ToString();
|
|
Entry = dr["ENTRY"].ToString();
|
|
UserID = dr["INITIALS"].ToString();
|
|
if (UserID == string.Empty) UserID = "UNKNOWN";
|
|
//string date = GetDTS(dr["DATE"].ToString(), dr["TIME"].ToString()).ToString("MM/dd/yyyy HH:MM");//System.DateTime.Parse(dr["DATE"].ToString()).ToString("MM/dd/yyyy");
|
|
//string time = dr["TIME"].ToString();
|
|
//if (time.EndsWith(":"))
|
|
// date += " " + time.Substring(0, 2) + ":" + time.Substring(2, 2);
|
|
//else
|
|
// date += " " + time.Substring(0, 2) + ":" + time.Substring(3, 2);
|
|
//StartDateTime = System.DateTime.Parse(date);
|
|
StartDateTime = GetDTS(dr["DATE"].ToString(), dr["TIME"].ToString());
|
|
RevDate = StartDateTime.ToString("MM/dd/yyyy");
|
|
FixItems fis = new FixItems(new FileInfo(string.Format(@"{0}\{1}.fix", afpath, Entry)));
|
|
if (fis.Count > 0)
|
|
{
|
|
Rev = fis[0].Rev;
|
|
RevDate = fis[0].RevDate ?? GetDBFRevDate(afpath);
|
|
ReviewDate = fis[0].ReviewDate;
|
|
}
|
|
}
|
|
public System.DateTime GetDTS(string date, string time)
|
|
{
|
|
// Set the date/time stamp. If there is no 'date', set the date
|
|
// to 1/1/2000 (this can be changed!). If there is not 'time',
|
|
// set the time to 0:0:0 (midnight).
|
|
|
|
System.DateTime dts = System.DateTime.Now;
|
|
string month = "01";
|
|
string day = "01";
|
|
string year = "2000";
|
|
string hour = "";
|
|
string minute = "";
|
|
try
|
|
{
|
|
if (date != null && date != "")
|
|
{
|
|
int indx1 = date.IndexOf("/");
|
|
month = date.Substring(0, indx1);
|
|
int indx2 = date.IndexOf("/", indx1 + 1);
|
|
day = date.Substring(indx1 + 1, indx2 - indx1 - 1);
|
|
year = date.Substring(indx2 + 1, 4);
|
|
}
|
|
if (time == null || time == "")
|
|
{
|
|
hour = "0";
|
|
minute = "0";
|
|
}
|
|
else
|
|
{
|
|
|
|
hour = time.Substring(0, 2);
|
|
int indxc = time.IndexOfAny(":A-".ToCharArray());
|
|
if (indxc == time.Length - 1)
|
|
minute = time.Substring(2, 2);
|
|
else
|
|
minute = time.Substring(indxc + 1, time.Length - indxc - 1);
|
|
}
|
|
dts = new System.DateTime(System.Convert.ToInt32(year), System.Convert.ToInt32(month), System.Convert.ToInt32(day),
|
|
System.Convert.ToInt32(hour), System.Convert.ToInt32(minute), 0);
|
|
}
|
|
catch (System.Exception ex)
|
|
{
|
|
log.ErrorFormat("Bad Date/Time {0} {1}. Set to NOW.", date, time);
|
|
log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
//frmMain.AddError(ex, "Bad Date/Time {0} {1}", date, time);
|
|
log.ErrorFormat(ex.StackTrace);
|
|
return dts;
|
|
}
|
|
return dts;
|
|
}
|
|
|
|
private string GetDBFRevDate(string afpath)
|
|
{
|
|
FileInfo fi = new FileInfo(afpath + @"\" + Entry + ".dbf");
|
|
if (fi.Exists)
|
|
return fi.LastWriteTime.ToShortDateString();
|
|
return null;
|
|
}
|
|
}
|
|
|
|
}
|