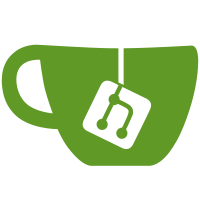
MyConfig for ItemInfo Fixed various problems with DisplayTabControl VEPROMS ribbon for DisplayTabPanel
117 lines
3.5 KiB
C#
117 lines
3.5 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayTabItem : DevComponents.DotNetBar.DockContainerItem
|
|
{
|
|
private DisplayTabControl _MyTabControl;
|
|
private ItemInfo _MyItem;
|
|
private DisplayTabPanel _MyTabPanel;
|
|
private string _MyKey;
|
|
public string MyKey
|
|
{
|
|
get { return _MyKey; }
|
|
}
|
|
public DisplayTabPanel MyTabPanel
|
|
{
|
|
get { return _MyTabPanel; }
|
|
set { _MyTabPanel = value; }
|
|
}
|
|
private DSOTabPanel _MyDSOTabPanel;
|
|
public ItemInfo ItemSelected
|
|
{
|
|
get { return _MyTabPanel.ItemSelected; }
|
|
set { _MyTabPanel.ItemSelected = value; }
|
|
}
|
|
public DisplayTabItem(IContainer container, DisplayTabControl myTabControl, ItemInfo myItem, string myKey)
|
|
{
|
|
_MyKey = myKey;
|
|
_MyTabControl = myTabControl;
|
|
_MyItem = myItem;
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.Click += new EventHandler(DisplayTabItem_Click);
|
|
if (myItem.MyContent.MyEntry == null)
|
|
SetupDisplayPanel();
|
|
else
|
|
SetupDSOPanel();
|
|
this.Disposed+=new EventHandler(DisplayTabItem_Disposed);
|
|
//_MyTabPanel.HandleDestroyed += new EventHandler(_MyTabPanel_HandleDestroyed);
|
|
//_MyTabPanel.Disposed += new EventHandler(_MyTabPanel_Disposed);
|
|
}
|
|
void DisplayTabItem_Click(object sender, EventArgs e)
|
|
{
|
|
// See if I can tell the TabControl that the ItemSelected has changed
|
|
DisplayTabItem myTabItem = sender as DisplayTabItem;
|
|
if(myTabItem == null)return;
|
|
DisplayTabPanel myTabPanel = myTabItem.MyTabPanel as DisplayTabPanel;
|
|
if(myTabPanel == null) return;
|
|
_MyTabControl.OnItemSelectedChanged(this,new DisplayPanelEventArgs(MyTabPanel.SelectedItem,null));
|
|
}
|
|
//void _MyTabPanel_Disposed(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("_MyTabPanel_Disposed");
|
|
//}
|
|
//void _MyTabPanel_HandleDestroyed(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("_MyTabPanel_HandleDestroyed");
|
|
//}
|
|
void DisplayTabItem_Disposed(object sender, EventArgs e)
|
|
{
|
|
Console.WriteLine("DisplayTabItem_Disposed");
|
|
}
|
|
private void SetupDisplayPanel()
|
|
{
|
|
((System.ComponentModel.ISupportInitialize)(_MyTabControl.MyBar)).BeginInit();
|
|
_MyTabControl.MyBar.SuspendLayout();
|
|
_MyTabPanel = new DisplayTabPanel(_MyTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyTabPanel;
|
|
Name = "tabItem Item " + _MyItem.ItemID;
|
|
Text = _MyItem.TabTitle;
|
|
Tooltip = _MyItem.TabToolTip;
|
|
//
|
|
_MyTabControl.Controls.Add(_MyTabPanel);
|
|
_MyTabControl.MyBar.Items.Add(this);
|
|
// TODO: Cleanup _MyTabControl.Tabs.Add(this);
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyTabPanel.TabItem = this;
|
|
((System.ComponentModel.ISupportInitialize)(_MyTabControl.MyBar)).EndInit();
|
|
_MyTabControl.MyBar.ResumeLayout(false);
|
|
//_MyTabPanel.MyItem = _MyItem;
|
|
}
|
|
private void SetupDSOPanel()
|
|
{
|
|
EntryInfo myEntry = _MyItem.MyContent.MyEntry;
|
|
_MyDSOTabPanel = new DSOTabPanel(myEntry.MyDocument, _MyTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyDSOTabPanel;
|
|
Name = "tabItem Item " + _MyItem.ItemID;
|
|
Text = _MyItem.TabTitle;
|
|
Tooltip = _MyItem.TabToolTip;
|
|
//
|
|
_MyTabControl.Controls.Add(_MyDSOTabPanel);
|
|
_MyTabControl.MyBar.Items.AddRange(new DevComponents.DotNetBar.BaseItem[] {
|
|
this});
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyTabControl.SelectedTab = this;
|
|
_MyDSOTabPanel.TabItem = this;
|
|
//_MyTabControl.SetToolTip(this, "Header", "Footer", "Body");
|
|
}
|
|
}
|
|
}
|