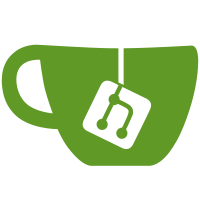
Added code to export individual procedure and to import individual procedure as a copy or overwrite in same procedure set or as a new procedure in a different procedure set Added code to handle events for exporting and importing procedures to same or different procedure sets within the same database Added code to allow user with both RO Editor and Writer permisssions to user RO Editor and edit procedure. Added menu items to export a procedure or import procedure and initiate their corresponding events.
80 lines
2.7 KiB
C#
80 lines
2.7 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
public partial class dlgCheckedOutProcedure : Form
|
|
{
|
|
public dlgCheckedOutProcedure(ProcedureInfo pi, SectionInfo si, UserInfo ui)
|
|
{
|
|
InitializeComponent();
|
|
_MyProcedureInfo = pi;
|
|
_MySectionInfo = si;
|
|
if (_MyProcedureInfo != null)
|
|
_MyOwnerInfo = OwnerInfo.GetByItemID(pi.ItemID, CheckOutType.Procedure);
|
|
else
|
|
_MyOwnerInfo = OwnerInfo.GetByItemID(si.MyContent.MyEntry.DocID, CheckOutType.Document);
|
|
_MySessionInfo = SessionInfo.Get(_MyOwnerInfo.SessionID);
|
|
_MyUserInfo = ui;
|
|
}
|
|
private ProcedureInfo _MyProcedureInfo;
|
|
public ProcedureInfo MyProcedureInfo
|
|
{
|
|
get { return _MyProcedureInfo; }
|
|
}
|
|
private SectionInfo _MySectionInfo;
|
|
public SectionInfo MySectionInfo
|
|
{
|
|
get { return _MySectionInfo; }
|
|
}
|
|
private OwnerInfo _MyOwnerInfo;
|
|
public OwnerInfo MyOwnerInfo
|
|
{
|
|
get { return _MyOwnerInfo; }
|
|
}
|
|
private SessionInfo _MySessionInfo;
|
|
public SessionInfo MySessionInfo
|
|
{
|
|
get { return _MySessionInfo; }
|
|
}
|
|
private UserInfo _MyUserInfo;
|
|
public UserInfo MyUserInfo
|
|
{
|
|
get { return _MyUserInfo; }
|
|
}
|
|
StringBuilder sb = new StringBuilder();
|
|
|
|
private void dlgCheckedOutProcedure_Load(object sender, EventArgs e)
|
|
{
|
|
if (MyProcedureInfo != null)
|
|
sb.AppendLine(string.Format("The procedure {0} - {1}", MyProcedureInfo.DisplayNumber, MyProcedureInfo.DisplayText));
|
|
else
|
|
sb.AppendLine(string.Format("The document {0}", MySectionInfo.DisplayText));
|
|
sb.AppendLine();
|
|
sb.AppendLine(string.Format("is currently checked out by {0} starting on {1}", MySessionInfo.UserID, MyOwnerInfo.DTSStart.ToString("MM/dd/yyyy @ HH:mm:ss")));
|
|
sb.AppendLine();
|
|
sb.AppendLine(string.Format("in a VEPROMS session on computer {0} that was started on {1}", MySessionInfo.MachineName, MySessionInfo.DTSDtart.ToString("MM/dd/yyyy @ HH:mm:ss")));
|
|
lblInfo.Text = sb.ToString();
|
|
if(MyProcedureInfo != null)
|
|
btnForce.Visible = MyUserInfo.IsAdministrator() || MyUserInfo.IsSetAdministrator(MyProcedureInfo.MyDocVersion) || (MyUserInfo.IsWriter(MyProcedureInfo.MyDocVersion) && MyOwnerInfo.OwnerItemID == MyProcedureInfo.ItemID);
|
|
else
|
|
btnForce.Visible = MyUserInfo.IsAdministrator() || MyUserInfo.IsSetAdministrator(MySectionInfo.MyProcedure.MyDocVersion);
|
|
}
|
|
|
|
private void btnForce_Click(object sender, EventArgs e)
|
|
{
|
|
MySessionInfo.CheckInItem(MyOwnerInfo.OwnerID);
|
|
sb.AppendLine();
|
|
sb.AppendLine("Forced Check In has been completed");
|
|
lblInfo.Text = sb.ToString();
|
|
btnForce.Enabled = false;
|
|
}
|
|
|
|
}
|
|
} |