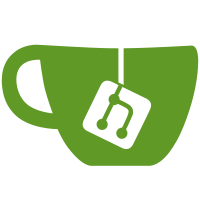
Shutoff SpellCheck for StepRTB Use using for temporary StepRTB Use using for vlnFlexGrid Added debug output to track StepRTBs and vlnFlexGrids in memory Limit bottom margin to 0 or above Dispose of roImage after it is done being used Dispose of Figure after it is done being used. Use GetJustRODB so that images are not loaded. Added ErrorHandler if annotation is deleted after a search Track create, dispose and finalize Add static variable to control if SpellCheck is used Use using for temporary StepRTB Lazy Load SelectionStack Clean-up on Dispose Track create, dispose and finalize Make MyCopyInfo Lazy Loaded Use using for temporary StepRTB Add Dispose method for TableCellEditor Cleanup on Dispose Only kill MSWord instances that are invisible
463 lines
15 KiB
C#
463 lines
15 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public delegate void RoUsageInfoEvent(object sender);
|
|
/// <summary>
|
|
/// RoUsageInfo Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(RoUsageInfoConverter))]
|
|
public partial class RoUsageInfo : ReadOnlyBase<RoUsageInfo>, IDisposable
|
|
{
|
|
public event RoUsageInfoEvent Changed;
|
|
private void OnChange()
|
|
{
|
|
if (Changed != null) Changed(this);
|
|
}
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Collection
|
|
private static List<RoUsageInfo> _CacheList = new List<RoUsageInfo>();
|
|
protected static void AddToCache(RoUsageInfo roUsageInfo)
|
|
{
|
|
if (!_CacheList.Contains(roUsageInfo)) _CacheList.Add(roUsageInfo); // In AddToCache
|
|
}
|
|
protected static void RemoveFromCache(RoUsageInfo roUsageInfo)
|
|
{
|
|
while (_CacheList.Contains(roUsageInfo)) _CacheList.Remove(roUsageInfo); // In RemoveFromCache
|
|
}
|
|
private static Dictionary<string, List<RoUsageInfo>> _CacheByPrimaryKey = new Dictionary<string, List<RoUsageInfo>>();
|
|
private static void ConvertListToDictionary()
|
|
{
|
|
while (_CacheList.Count > 0) // Move RoUsageInfo(s) from temporary _CacheList to _CacheByPrimaryKey
|
|
{
|
|
RoUsageInfo tmp = _CacheList[0]; // Get the first RoUsageInfo
|
|
string pKey = tmp.ROUsageID.ToString();
|
|
if (!_CacheByPrimaryKey.ContainsKey(pKey))
|
|
{
|
|
_CacheByPrimaryKey[pKey] = new List<RoUsageInfo>(); // Add new list for PrimaryKey
|
|
}
|
|
_CacheByPrimaryKey[pKey].Add(tmp); // Add to Primary Key list
|
|
_CacheList.RemoveAt(0); // Remove the first RoUsageInfo
|
|
}
|
|
}
|
|
internal static void AddList(RoUsageInfoList lst)
|
|
{
|
|
foreach (RoUsageInfo item in lst) AddToCache(item);
|
|
}
|
|
protected static RoUsageInfo GetCachedByPrimaryKey(int rOUsageID)
|
|
{
|
|
ConvertListToDictionary();
|
|
string key = rOUsageID.ToString();
|
|
if (_CacheByPrimaryKey.ContainsKey(key)) return _CacheByPrimaryKey[key][0];
|
|
return null;
|
|
}
|
|
#endregion
|
|
#region Business Methods
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
protected RoUsage _Editable;
|
|
private IVEHasBrokenRules HasBrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = null;
|
|
if (_Editable != null)
|
|
hasBrokenRules = _Editable.HasBrokenRules;
|
|
return hasBrokenRules;
|
|
}
|
|
}
|
|
private int _ROUsageID;
|
|
[System.ComponentModel.DataObjectField(true, true)]
|
|
public int ROUsageID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("ROUsageID", true);
|
|
return _ROUsageID;
|
|
}
|
|
}
|
|
private int _ContentID;
|
|
public int ContentID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("ContentID", true);
|
|
if (_MyContent != null) _ContentID = _MyContent.ContentID;
|
|
return _ContentID;
|
|
}
|
|
}
|
|
private ContentInfo _MyContent;
|
|
public ContentInfo MyContent
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyContent", true);
|
|
if (_MyContent == null && _ContentID != 0) _MyContent = ContentInfo.Get(_ContentID);
|
|
return _MyContent;
|
|
}
|
|
}
|
|
private string _ROID = string.Empty;
|
|
public string ROID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("ROID", true);
|
|
return _ROID;
|
|
}
|
|
}
|
|
private string _Config = string.Empty;
|
|
public string Config
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("Config", true);
|
|
return _Config;
|
|
}
|
|
}
|
|
private DateTime _DTS = new DateTime();
|
|
public DateTime DTS
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("DTS", true);
|
|
return _DTS;
|
|
}
|
|
}
|
|
private string _UserID = string.Empty;
|
|
public string UserID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UserID", true);
|
|
return _UserID;
|
|
}
|
|
}
|
|
private int _RODbID;
|
|
public int RODbID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("RODbID", true);
|
|
if (_MyRODb != null) _RODbID = _MyRODb.RODbID;
|
|
return _RODbID;
|
|
}
|
|
}
|
|
private RODbInfo _MyRODb;
|
|
public RODbInfo MyRODb
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyRODb", true);
|
|
if (_MyRODb == null && _RODbID != 0) _MyRODb = RODbInfo.GetJustRODB(_RODbID);
|
|
return _MyRODb;
|
|
}
|
|
}
|
|
// CSLATODO: Replace base RoUsageInfo.ToString function as necessary
|
|
/// <summary>
|
|
/// Overrides Base ToString
|
|
/// </summary>
|
|
/// <returns>A string representation of current RoUsageInfo</returns>
|
|
//public override string ToString()
|
|
//{
|
|
// return base.ToString();
|
|
//}
|
|
// CSLATODO: Check RoUsageInfo.GetIdValue to assure that the ID returned is unique
|
|
/// <summary>
|
|
/// Overrides Base GetIdValue - Used internally by CSLA to determine equality
|
|
/// </summary>
|
|
/// <returns>A Unique ID for the current RoUsageInfo</returns>
|
|
protected override object GetIdValue()
|
|
{
|
|
return MyRoUsageInfoUnique; // Absolutely Unique ID
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
private static int _RoUsageInfoUnique = 0;
|
|
private static int RoUsageInfoUnique
|
|
{ get { return ++_RoUsageInfoUnique; } }
|
|
private int _MyRoUsageInfoUnique = RoUsageInfoUnique;
|
|
public int MyRoUsageInfoUnique // Absolutely Unique ID - Info
|
|
{ get { return _MyRoUsageInfoUnique; } }
|
|
protected RoUsageInfo()
|
|
{/* require use of factory methods */
|
|
AddToCache(this);
|
|
}
|
|
private bool _Disposed = false;
|
|
private static int _CountCreated = 0;
|
|
private static int _CountDisposed = 0;
|
|
private static int _CountFinalized = 0;
|
|
private static int IncrementCountCreated
|
|
{ get { return ++_CountCreated; } }
|
|
private int _CountWhenCreated = IncrementCountCreated;
|
|
public static int CountCreated
|
|
{ get { return _CountCreated; } }
|
|
public static int CountNotDisposed
|
|
{ get { return _CountCreated - _CountDisposed; } }
|
|
public static int CountNotFinalized
|
|
{ get { return _CountCreated - _CountFinalized; } }
|
|
~RoUsageInfo()
|
|
{
|
|
_CountFinalized++;
|
|
}
|
|
public void Dispose()
|
|
{
|
|
if (_Disposed) return;
|
|
_CountDisposed++;
|
|
_Disposed = true;
|
|
RemoveFromCache(this);
|
|
if (!_CacheByPrimaryKey.ContainsKey(ROUsageID.ToString())) return;
|
|
List<RoUsageInfo> listRoUsageInfo = _CacheByPrimaryKey[ROUsageID.ToString()]; // Get the list of items
|
|
while (listRoUsageInfo.Contains(this)) listRoUsageInfo.Remove(this); // Remove the item from the list
|
|
if (listRoUsageInfo.Count == 0) // If there are no items left in the list
|
|
_CacheByPrimaryKey.Remove(ROUsageID.ToString()); // remove the list
|
|
}
|
|
public virtual RoUsage Get()
|
|
{
|
|
return _Editable = RoUsage.Get(_ROUsageID);
|
|
}
|
|
public static void Refresh(RoUsage tmp)
|
|
{
|
|
string key = tmp.ROUsageID.ToString();
|
|
ConvertListToDictionary();
|
|
if (_CacheByPrimaryKey.ContainsKey(key))
|
|
foreach (RoUsageInfo tmpInfo in _CacheByPrimaryKey[key])
|
|
tmpInfo.RefreshFields(tmp);
|
|
}
|
|
protected virtual void RefreshFields(RoUsage tmp)
|
|
{
|
|
if (_ContentID != tmp.ContentID)
|
|
{
|
|
if (MyContent != null) MyContent.RefreshContentRoUsages(); // Update List for old value
|
|
_ContentID = tmp.ContentID; // Update the value
|
|
}
|
|
_MyContent = null; // Reset list so that the next line gets a new list
|
|
if (MyContent != null) MyContent.RefreshContentRoUsages(); // Update List for new value
|
|
_ROID = tmp.ROID;
|
|
_Config = tmp.Config;
|
|
_DTS = tmp.DTS;
|
|
_UserID = tmp.UserID;
|
|
if (_RODbID != tmp.RODbID)
|
|
{
|
|
if (MyRODb != null) MyRODb.RefreshRODbRoUsages(); // Update List for old value
|
|
_RODbID = tmp.RODbID; // Update the value
|
|
}
|
|
_MyRODb = null; // Reset list so that the next line gets a new list
|
|
if (MyRODb != null) MyRODb.RefreshRODbRoUsages(); // Update List for new value
|
|
_RoUsageInfoExtension.Refresh(this);
|
|
OnChange();// raise an event
|
|
}
|
|
public static void Refresh(ContentRoUsage tmp)
|
|
{
|
|
string key = tmp.ROUsageID.ToString();
|
|
ConvertListToDictionary();
|
|
if (_CacheByPrimaryKey.ContainsKey(key))
|
|
foreach (RoUsageInfo tmpInfo in _CacheByPrimaryKey[key])
|
|
tmpInfo.RefreshFields(tmp);
|
|
}
|
|
protected virtual void RefreshFields(ContentRoUsage tmp)
|
|
{
|
|
_ROID = tmp.ROID;
|
|
_Config = tmp.Config;
|
|
_DTS = tmp.DTS;
|
|
_UserID = tmp.UserID;
|
|
if (_RODbID != tmp.RODbID)
|
|
{
|
|
if (MyRODb != null) MyRODb.RefreshRODbRoUsages(); // Update List for old value
|
|
_RODbID = tmp.RODbID; // Update the value
|
|
}
|
|
_MyRODb = null; // Reset list so that the next line gets a new list
|
|
if (MyRODb != null) MyRODb.RefreshRODbRoUsages(); // Update List for new value
|
|
_RoUsageInfoExtension.Refresh(this);
|
|
OnChange();// raise an event
|
|
}
|
|
public static void Refresh(RODbRoUsage tmp)
|
|
{
|
|
string key = tmp.ROUsageID.ToString();
|
|
ConvertListToDictionary();
|
|
if (_CacheByPrimaryKey.ContainsKey(key))
|
|
foreach (RoUsageInfo tmpInfo in _CacheByPrimaryKey[key])
|
|
tmpInfo.RefreshFields(tmp);
|
|
}
|
|
protected virtual void RefreshFields(RODbRoUsage tmp)
|
|
{
|
|
if (_ContentID != tmp.ContentID)
|
|
{
|
|
if (MyContent != null) MyContent.RefreshContentRoUsages(); // Update List for old value
|
|
_ContentID = tmp.ContentID; // Update the value
|
|
}
|
|
_MyContent = null; // Reset list so that the next line gets a new list
|
|
if (MyContent != null) MyContent.RefreshContentRoUsages(); // Update List for new value
|
|
_ROID = tmp.ROID;
|
|
_Config = tmp.Config;
|
|
_DTS = tmp.DTS;
|
|
_UserID = tmp.UserID;
|
|
_RoUsageInfoExtension.Refresh(this);
|
|
OnChange();// raise an event
|
|
}
|
|
public static RoUsageInfo Get(int rOUsageID)
|
|
{
|
|
//if (!CanGetObject())
|
|
// throw new System.Security.SecurityException("User not authorized to view a RoUsage");
|
|
try
|
|
{
|
|
RoUsageInfo tmp = GetCachedByPrimaryKey(rOUsageID);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<RoUsageInfo>(new PKCriteria(rOUsageID));
|
|
AddToCache(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up RoUsageInfo
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on RoUsageInfo.Get", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Data Access Portal
|
|
internal RoUsageInfo(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] RoUsageInfo.Constructor", GetHashCode());
|
|
try
|
|
{
|
|
ReadData(dr);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("RoUsageInfo.Constructor", ex);
|
|
throw new DbCslaException("RoUsageInfo.Constructor", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
protected class PKCriteria
|
|
{
|
|
private int _ROUsageID;
|
|
public int ROUsageID
|
|
{ get { return _ROUsageID; } }
|
|
public PKCriteria(int rOUsageID)
|
|
{
|
|
_ROUsageID = rOUsageID;
|
|
}
|
|
}
|
|
private void ReadData(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] RoUsageInfo.ReadData", GetHashCode());
|
|
try
|
|
{
|
|
_ROUsageID = dr.GetInt32("ROUsageID");
|
|
_ContentID = dr.GetInt32("ContentID");
|
|
_ROID = dr.GetString("ROID");
|
|
_Config = dr.GetString("Config");
|
|
_DTS = dr.GetDateTime("DTS");
|
|
_UserID = dr.GetString("UserID");
|
|
_RODbID = dr.GetInt32("RODbID");
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("RoUsageInfo.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("RoUsageInfo.ReadData", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PKCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] RoUsageInfo.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getRoUsage";
|
|
cm.Parameters.AddWithValue("@ROUsageID", criteria.ROUsageID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("RoUsageInfo.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("RoUsageInfo.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
// Standard Refresh
|
|
#region extension
|
|
RoUsageInfoExtension _RoUsageInfoExtension = new RoUsageInfoExtension();
|
|
[Serializable()]
|
|
partial class RoUsageInfoExtension : extensionBase { }
|
|
[Serializable()]
|
|
class extensionBase
|
|
{
|
|
// Default Refresh
|
|
public virtual void Refresh(RoUsageInfo tmp) { }
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Converter
|
|
internal class RoUsageInfoConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is RoUsageInfo)
|
|
{
|
|
// Return the ToString value
|
|
return ((RoUsageInfo)value).ToString();
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|