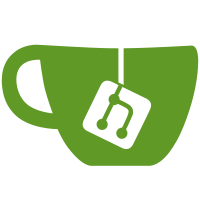
Added code to Just Get RODb and not all of it's children. Added code to create an RoImageFile (Temporary) to be used to view and print images Additional DEBUG info
247 lines
5.9 KiB
C#
247 lines
5.9 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.IO;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class ROImageInfo
|
|
{
|
|
public static ROImageInfo GetByROFstID_FileName(int rOFstID, string fileName)
|
|
{
|
|
//if (!CanGetObject())
|
|
// throw new System.Security.SecurityException("User not authorized to view a ROImage");
|
|
try
|
|
{
|
|
ROImageInfo tmp = DataPortal.Fetch<ROImageInfo>(new ROFstID_FileNameCriteria(rOFstID, fileName));
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up ROImage
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on ROImage.GetByROFstID_FileName", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(ROFstID_FileNameCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] ROImageInfo.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getROImageByROFstID_FileName";
|
|
cm.Parameters.AddWithValue("@ROFstID", criteria.ROFstID);
|
|
cm.Parameters.AddWithValue("@FileName", criteria.FileName);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("ROImageInfo.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("ROImageInfo.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ROFstID_FileNameCriteria
|
|
{
|
|
private int _ROFstID;
|
|
|
|
public int ROFstID
|
|
{ get { return _ROFstID; } }
|
|
private string _FileName;
|
|
public string FileName
|
|
{ get { return _FileName; } }
|
|
public ROFstID_FileNameCriteria(int rOFstID, string fileName)
|
|
{
|
|
_ROFstID = rOFstID;
|
|
_FileName = fileName;
|
|
}
|
|
}
|
|
}
|
|
public class ROImageFile : IDisposable
|
|
{
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#region Fields
|
|
private bool _IsDisposed;
|
|
private static string _TemporaryFolder = null;
|
|
#endregion
|
|
#region Properties
|
|
public static string TemporaryFolder
|
|
{
|
|
get
|
|
{
|
|
if (_TemporaryFolder == null)
|
|
{
|
|
// This will create a Temp\VE-PROMS folder in the LocalSettings Folder.
|
|
//XP - C:\Documents and Settings\{user}\Local Settings\Application Data\Temp\VE-PROMS
|
|
//Vista - C:\Users\{user}\AppData\Local\Temp\VE-PROMS
|
|
_TemporaryFolder = Environment.GetFolderPath(Environment.SpecialFolder.LocalApplicationData) + @"\Temp";
|
|
if (!Directory.Exists(TemporaryFolder)) Directory.CreateDirectory(TemporaryFolder);
|
|
_TemporaryFolder += @"\VE-PROMS";
|
|
if (!Directory.Exists(TemporaryFolder)) Directory.CreateDirectory(TemporaryFolder);
|
|
}
|
|
return _TemporaryFolder;
|
|
}
|
|
}
|
|
private ROImageInfo _MyROImage = null;
|
|
public ROImageInfo MyROImage
|
|
{
|
|
get { return _MyROImage; }
|
|
set
|
|
{
|
|
TryDelete();
|
|
_MyROImage = value;
|
|
CreateFile();
|
|
}
|
|
}
|
|
private FileInfo _MyFile = null;
|
|
public FileInfo MyFile
|
|
{
|
|
get { return _MyFile; }
|
|
}
|
|
private string _Extension = "TIF";
|
|
public string Extension
|
|
{
|
|
get { return _Extension; }
|
|
set { _Extension = value; }
|
|
}
|
|
#endregion
|
|
#region Private Methods
|
|
private void TryDelete()
|
|
{
|
|
if (_MyROImage == null) return;
|
|
if (_MyFile == null) return;
|
|
if (_MyFile.Exists)
|
|
{
|
|
try
|
|
{
|
|
_MyFile.Delete();
|
|
}
|
|
catch (IOException ex)
|
|
{
|
|
_MyLog.Error("TryDelete", ex);
|
|
}
|
|
finally
|
|
{
|
|
_MyFile = null;
|
|
_MyROImage = null;
|
|
}
|
|
}
|
|
}
|
|
private bool _Created = false;
|
|
private int _Unique = 0;
|
|
private string Unique
|
|
{
|
|
get
|
|
{
|
|
string retval = "";
|
|
if (_Unique != 0) retval = "_" + _Unique.ToString();
|
|
_Unique++;
|
|
return retval;
|
|
}
|
|
}
|
|
private void CreateFile()
|
|
{
|
|
while (!_Created)
|
|
CreateTemporaryFile();
|
|
}
|
|
|
|
private void CreateTemporaryFile()
|
|
{
|
|
try
|
|
{
|
|
if (_MyROImage != null)
|
|
{
|
|
_MyFile = new FileInfo(string.Format(@"{0}\tmp_{1}{2}", TemporaryFolder, Unique, MyROImage.FileName));
|
|
FileStream fs = _MyFile.Create();
|
|
fs.Write(MyROImage.Content, 0, MyROImage.Content.Length);
|
|
fs.Close();
|
|
_MyFile.CreationTime = MyROImage.DTS;
|
|
_MyFile.LastWriteTime = MyROImage.DTS;
|
|
_Created = true;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine(ex.Message);
|
|
}
|
|
}
|
|
public string FullName
|
|
{
|
|
get { return _MyFile.FullName; }
|
|
set
|
|
{
|
|
if (FullName != value)
|
|
_MyFile = new FileInfo(value);
|
|
}
|
|
}
|
|
public void SaveFile()
|
|
{
|
|
// TODO: Add Try & Catch logic
|
|
if (_MyROImage == null) return;
|
|
ROImage roImage = _MyROImage.Get();
|
|
FileStream fs = _MyFile.Open(FileMode.Open, FileAccess.Read, FileShare.ReadWrite);
|
|
Byte[] buf = new byte[_MyFile.Length];
|
|
fs.Read(buf, 0, buf.Length);
|
|
fs.Close();
|
|
//roImage.FileName;
|
|
roImage.Content = buf;
|
|
roImage.UserID = Environment.UserName;
|
|
roImage.DTS = _MyFile.LastWriteTime;
|
|
roImage.Save();
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public ROImageFile(ROImageInfo myROImage)
|
|
{
|
|
MyROImage = myROImage;
|
|
}
|
|
#endregion
|
|
#region Destructor
|
|
~ROImageFile()
|
|
{
|
|
Dispose(false);
|
|
}
|
|
public void Dispose()
|
|
{
|
|
Dispose(false);
|
|
GC.SuppressFinalize(this);
|
|
}
|
|
|
|
protected void Dispose(bool disposing)
|
|
{
|
|
if (!_IsDisposed)
|
|
{
|
|
_IsDisposed = true;
|
|
TryDelete();
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
}
|