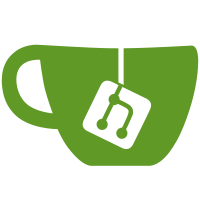
Added code to Just Get RODb and not all of it's children. Added code to create an RoImageFile (Temporary) to be used to view and print images Additional DEBUG info
142 lines
4.1 KiB
C#
142 lines
4.1 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Collections.Specialized;
|
|
using System.Text;
|
|
using System.IO;
|
|
using System.Xml.Serialization;
|
|
using System.Xml;
|
|
using System.Xml.XPath;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Data.SqlClient;
|
|
using System.Data;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class RODbInfo
|
|
{
|
|
#region RODb Config
|
|
[NonSerialized]
|
|
private RODbConfig _RODbConfig;
|
|
public RODbConfig RODbConfig
|
|
{ get { return (_RODbConfig != null ? _RODbConfig : _RODbConfig = new RODbConfig(this)); } }
|
|
private void RODbConfigRefresh()
|
|
{
|
|
_RODbConfig = null;
|
|
}
|
|
#endregion
|
|
}
|
|
public partial class RODb
|
|
{
|
|
public static RODb GetJustRoDb(int rODbID)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a RODb");
|
|
try
|
|
{
|
|
RODb tmp = DataPortal.Fetch<RODb>(new PKCriteriaJustRoDb(rODbID));
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up RODb
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on RODb.Get", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PKCriteriaJustRoDb criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] RODb.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getJustRODb";
|
|
cm.Parameters.AddWithValue("@RODbID", criteria.RODbID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
// Don't load child objects
|
|
//dr.NextResult();
|
|
//_RODbROFsts = RODbROFsts.Get(dr);
|
|
// load child objects
|
|
//dr.NextResult();
|
|
//_RODbROImages = RODbROImages.Get(dr);
|
|
// load child objects
|
|
//dr.NextResult();
|
|
//_RODbRoUsages = RODbRoUsages.Get(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("RODb.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("RODb.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
protected class PKCriteriaJustRoDb
|
|
{
|
|
private int _RODbID;
|
|
public int RODbID
|
|
{ get { return _RODbID; } }
|
|
public PKCriteriaJustRoDb(int rODbID)
|
|
{
|
|
_RODbID = rODbID;
|
|
}
|
|
}
|
|
|
|
#region Log4Net
|
|
private static readonly log4net.ILog log = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
|
|
#region RODb Config
|
|
[NonSerialized]
|
|
private RODbConfig _RODbConfig;
|
|
public RODbConfig RODbConfig
|
|
{
|
|
get
|
|
{
|
|
if (_RODbConfig == null)
|
|
{
|
|
_RODbConfig = new RODbConfig(this);
|
|
_RODbConfig.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(_RODbConfig_PropertyChanged);
|
|
}
|
|
return _RODbConfig;
|
|
}
|
|
}
|
|
private void _RODbConfig_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
|
|
{
|
|
Config = _RODbConfig.ToString();
|
|
}
|
|
#endregion
|
|
}
|
|
}
|