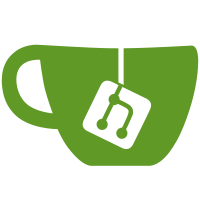
Changed Through Transition Format and User Interface Added Automatic Print Options to shutoff DebugText (/NT), DebugPagination (/NP) and Compare (/NC) Added logic to allow the use of the default path for PDFs. Update the Print_PDFLocation property of the Working Draft when the user changes the value on the Working Draft property page. Fixed Bug B2013-117 Set the date/time stamp for the Document based upon the current time. Make sure that the output is set to Final without revisions and comments before ROs are replaced. Added function to restore valid previous version of MSWord document if the current version is not a valid document. Check for Null to eliminate errors. Removed Unused field DocPDF Support MSWord document restore function. Add check for Transition Node count before updating values. Restore latest valid MSWord document if the current version is not valid. Eliminate File Commands so that the user can only close the MSWord section, and then verify if the changes to the document will be saved.
264 lines
8.5 KiB
C#
264 lines
8.5 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayTabItem : DevComponents.DotNetBar.DockContainerItem
|
|
{
|
|
#region Private Fields
|
|
private DisplayTabControl _MyDisplayTabControl;
|
|
private ItemInfo _MyItemInfo;
|
|
private StepTabPanel _MyStepTabPanel;
|
|
private string _MyKey;
|
|
private DSOTabPanel _MyDSOTabPanel;
|
|
private DocumentInfo _MyDocumentInfo;
|
|
|
|
|
|
#endregion
|
|
#region Properties
|
|
/// <summary>
|
|
/// ItemInfo associated with this DisplayTabItem
|
|
/// </summary>
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
//set { _MyItemInfo = value; }
|
|
}
|
|
/// <summary>
|
|
/// get Key Either:
|
|
/// "Item - " + Procedure ItemID for step pages
|
|
/// "Doc - " + DocumentID for Word Documents
|
|
/// </summary>
|
|
public string MyKey
|
|
{
|
|
get { return _MyKey; }
|
|
}
|
|
/// <summary>
|
|
/// Related StepTabPanel for a Step page
|
|
/// </summary>
|
|
public StepTabPanel MyStepTabPanel
|
|
{
|
|
get { return _MyStepTabPanel; }
|
|
set { _MyStepTabPanel = value; }
|
|
}
|
|
/// <summary>
|
|
/// Related DSOTabPanle for a Word page
|
|
/// </summary>
|
|
public DSOTabPanel MyDSOTabPanel
|
|
{
|
|
get { return _MyDSOTabPanel; }
|
|
set { _MyDSOTabPanel = value; }
|
|
}
|
|
/// <summary>
|
|
/// Current SelectedItemInfo for this page
|
|
/// </summary>
|
|
public ItemInfo SelectedItemInfo
|
|
{
|
|
get
|
|
{
|
|
if (_MyStepTabPanel == null) return null;
|
|
return _MyStepTabPanel.SelectedItemInfo;
|
|
}
|
|
set { _MyStepTabPanel.SelectedItemInfo = value; }
|
|
}
|
|
/// <summary>
|
|
/// Current DocumentInfo for this page - only set if library document
|
|
/// </summary>
|
|
public DocumentInfo MyDocumentInfo
|
|
{
|
|
get { return _MyDocumentInfo; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public DisplayTabItem(IContainer container, DisplayTabControl myDisplayTabControl, ItemInfo myItemInfo, string myKey)
|
|
{
|
|
_MyKey = myKey;
|
|
_MyDisplayTabControl = myDisplayTabControl;
|
|
_MyItemInfo = myItemInfo;
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.Click += new EventHandler(DisplayTabItem_Click);
|
|
if (myItemInfo.MyContent.MyEntry == null)
|
|
SetupStepTabPanel();
|
|
else
|
|
SetupDSOTabPanel();
|
|
Name = string.Format("DisplayTabItem {0}", myItemInfo.ItemID);
|
|
}
|
|
public DisplayTabItem(IContainer container, DisplayTabControl myDisplayTabControl, DocumentInfo myDocumentInfo, string myKey)
|
|
{
|
|
_MyKey = myKey;
|
|
_MyDisplayTabControl = myDisplayTabControl;
|
|
_MyDocumentInfo = myDocumentInfo;
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
this.Click += new EventHandler(DisplayTabItem_Click);
|
|
SetupLibraryDocumentDSOTabPanel();
|
|
Name = string.Format("DisplayTabLibraryDocument {0}", myDocumentInfo.DocID);
|
|
}
|
|
|
|
protected override void OnDisplayedChanged()
|
|
{
|
|
//Console.WriteLine("=>=>=>=> OnDisplayedChanged");
|
|
if(_MyStepTabPanel != null)
|
|
_MyStepTabPanel.MyStepPanel.DisplayItemChanging = true;
|
|
base.OnDisplayedChanged();
|
|
if(_MyStepTabPanel != null)
|
|
_MyStepTabPanel.MyStepPanel.DisplayItemChanging = false;
|
|
//Console.WriteLine("<=<=<=<= OnDisplayedChanged");
|
|
}
|
|
|
|
//void DisplayTabItem_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_MouseUp");
|
|
//}
|
|
|
|
//void DisplayTabItem_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_MouseDown");
|
|
//}
|
|
|
|
//void DisplayTabItem_LostFocus(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_LostFocus");
|
|
//}
|
|
|
|
//void DisplayTabItem_GotFocus(object sender, EventArgs e)
|
|
//{
|
|
// Console.WriteLine("DisplayTabItem_GotFocus");
|
|
//}
|
|
#endregion
|
|
#region Event Handlers
|
|
/// <summary>
|
|
/// Updates SelectedRTBItem when the user selects a DisplayTabItem
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void DisplayTabItem_Click(object sender, EventArgs e)
|
|
{
|
|
// Tell the TabControl that the ItemSelected has changed
|
|
DisplayTabItem myTabItem = sender as DisplayTabItem;
|
|
if(myTabItem == null)return;
|
|
StepTabPanel myTabPanel = myTabItem.MyStepTabPanel as StepTabPanel;
|
|
if(myTabPanel == null) return;
|
|
if (MyStepTabPanel.SelectedEditItem == null) return;
|
|
_MyDisplayTabControl.OnItemSelectedChanged(this,new ItemSelectedChangedEventArgs(MyStepTabPanel.SelectedEditItem));
|
|
}
|
|
#endregion
|
|
#region private Methods
|
|
/// <summary>
|
|
/// Creates and sets-up a StepTabPanel
|
|
/// </summary>
|
|
private void SetupStepTabPanel()
|
|
{
|
|
((System.ComponentModel.ISupportInitialize)(_MyDisplayTabControl.MyBar)).BeginInit();
|
|
_MyDisplayTabControl.MyBar.SuspendLayout();
|
|
_MyStepTabPanel = new StepTabPanel(_MyDisplayTabControl);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyStepTabPanel;
|
|
Name = "tabItem Item " + _MyItemInfo.ItemID;
|
|
Text = _MyItemInfo.TabTitle;
|
|
Tooltip = _Tooltip = _MyItemInfo.TabToolTip.Replace("\u2011", "-");
|
|
MouseMove += new System.Windows.Forms.MouseEventHandler(DisplayTabItem_MouseMove);
|
|
LostFocus += new EventHandler(DisplayTabItem_LostFocus);
|
|
//
|
|
_MyDisplayTabControl.Controls.Add(_MyStepTabPanel);
|
|
_MyDisplayTabControl.MyBar.Items.Add(this);
|
|
_MyDisplayTabControl.MyBar.Width = 300; // This triggers the bar to resize itself
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyStepTabPanel.MyDisplayTabItem = this;
|
|
((System.ComponentModel.ISupportInitialize)(_MyDisplayTabControl.MyBar)).EndInit();
|
|
_MyDisplayTabControl.MyBar.ResumeLayout(false);
|
|
DocVersionInfo dvi = _MyItemInfo.MyProcedure.ActiveParent as DocVersionInfo; //MyRTBItem.MyItemInfo.MyProcedure.ActiveParent as DocVersionInfo;
|
|
if (dvi == null) return;
|
|
if (dvi.VersionType > 127)
|
|
MyStepTabPanel.MyStepPanel.VwMode = E_ViewMode.View;
|
|
}
|
|
void DisplayTabItem_LostFocus(object sender, EventArgs e)
|
|
{
|
|
if(Tooltip != _Tooltip)
|
|
Tooltip = _Tooltip;
|
|
}
|
|
private string _Tooltip;
|
|
public void SetPrivateTooltip(string tt)
|
|
{
|
|
_Tooltip = tt;
|
|
}
|
|
void DisplayTabItem_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
|
|
{
|
|
string newTooltip = e.Y > 30 ? null : _Tooltip;
|
|
if(Tooltip != newTooltip)
|
|
Tooltip = newTooltip;
|
|
}
|
|
/// <summary>
|
|
/// Creates and sets-up a DSOTabPanel
|
|
/// </summary>
|
|
private void SetupDSOTabPanel()
|
|
{
|
|
EntryInfo myEntry = _MyItemInfo.MyContent.MyEntry;
|
|
_MyDSOTabPanel = new DSOTabPanel(myEntry.MyDocument, _MyDisplayTabControl, _MyItemInfo);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyDSOTabPanel;
|
|
Name = "tabItem Item " + _MyItemInfo.ItemID;
|
|
Text = _MyItemInfo.TabTitle;
|
|
Tooltip = _Tooltip = _MyItemInfo.TabToolTip.Replace("\u2011", "-");
|
|
MouseMove += new System.Windows.Forms.MouseEventHandler(DisplayTabItem_MouseMove);
|
|
LostFocus += new EventHandler(DisplayTabItem_LostFocus);
|
|
_MyDisplayTabControl.Controls.Add(_MyDSOTabPanel);
|
|
DSOTabPanel.IgnoreEnter = true;
|
|
//Console.WriteLine("AddRange {0}", Name);
|
|
_MyDisplayTabControl.MyBar.Items.AddRange(new DevComponents.DotNetBar.BaseItem[] {
|
|
this});
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyDisplayTabControl.SelectDisplayTabItem(this);
|
|
_MyDSOTabPanel.MyDisplayTabItem = this;
|
|
DSOTabPanel.IgnoreEnter = false;
|
|
}
|
|
private void SetupLibraryDocumentDSOTabPanel()
|
|
{
|
|
_MyDSOTabPanel = new DSOTabPanel(_MyDocumentInfo, _MyDisplayTabControl, _MyItemInfo);
|
|
//
|
|
// tabItem
|
|
//
|
|
Control = _MyDSOTabPanel;
|
|
Name = "tabLibraryDocument " + _MyDocumentInfo.DocID;
|
|
Text = _MyDocumentInfo.LibTitle;
|
|
DocumentConfig dc = new DocumentConfig(_MyDocumentInfo);
|
|
Tooltip = _Tooltip = dc.LibDoc_Comment;
|
|
MouseMove += new System.Windows.Forms.MouseEventHandler(DisplayTabItem_MouseMove);
|
|
LostFocus += new EventHandler(DisplayTabItem_LostFocus);
|
|
_MyDisplayTabControl.Controls.Add(_MyDSOTabPanel);
|
|
DSOTabPanel.IgnoreEnter = true;
|
|
_MyDisplayTabControl.MyBar.Items.AddRange(new DevComponents.DotNetBar.BaseItem[] {
|
|
this});
|
|
//
|
|
// tabPanel
|
|
//
|
|
_MyDisplayTabControl.SelectDisplayTabItem(this);
|
|
_MyDSOTabPanel.MyDisplayTabItem = this;
|
|
DSOTabPanel.IgnoreEnter = false;
|
|
}
|
|
#endregion
|
|
public override string ToString()
|
|
{
|
|
if (MyDSOTabPanel != null)
|
|
return MyDSOTabPanel.ToString();
|
|
if (MyStepTabPanel != null)
|
|
return MyStepTabPanel.ToString();
|
|
return "NULL";
|
|
}
|
|
}
|
|
}
|