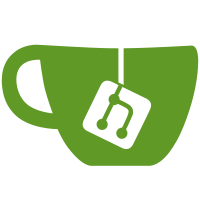
Changed Through Transition Format and User Interface Added Automatic Print Options to shutoff DebugText (/NT), DebugPagination (/NP) and Compare (/NC) Added logic to allow the use of the default path for PDFs. Update the Print_PDFLocation property of the Working Draft when the user changes the value on the Working Draft property page. Fixed Bug B2013-117 Set the date/time stamp for the Document based upon the current time. Make sure that the output is set to Final without revisions and comments before ROs are replaced. Added function to restore valid previous version of MSWord document if the current version is not a valid document. Check for Null to eliminate errors. Removed Unused field DocPDF Support MSWord document restore function. Add check for Transition Node count before updating values. Restore latest valid MSWord document if the current version is not valid. Eliminate File Commands so that the user can only close the MSWord section, and then verify if the changes to the document will be saved.
1212 lines
43 KiB
C#
1212 lines
43 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.IO;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Drawing.Imaging;
|
|
using VEPROMS.Properties;
|
|
using Volian.Controls.Library;
|
|
using DescriptiveEnum;
|
|
using DevComponents.DotNetBar;
|
|
using DevComponents.DotNetBar.Controls;
|
|
using System.Xml.Serialization;
|
|
using Volian.Base.Library;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
public partial class frmVersionsProperties : DevComponents.DotNetBar.Office2007Form
|
|
{
|
|
private List<MiniConfig> _Apples;
|
|
private List<MiniConfig> _DeletedApples;
|
|
private bool _Initializing = false;
|
|
private DocVersionConfig _DocVersionConfig;
|
|
|
|
// Default values
|
|
private string _DefaultFormatName = null;
|
|
private string _DefaultROPrefix = null;
|
|
private string _DefaultImagePrefix = null;
|
|
//private string _DefaultPagination = null;
|
|
private string _DefaultWatermark = null;
|
|
private string _DefaultChgBarType = null;
|
|
private string _DefaultChgBarLoc = null;
|
|
private string _DefaultChgBarText = null;
|
|
private string _DefaultChgBarUsrMsg1 = null;
|
|
private string _DefaultChgBarUsrMsg2 = null;
|
|
private bool _DefaultDisableDuplex = false;
|
|
|
|
// For the initial release, we are assuming there will be only one rofst for a docversion. Changes
|
|
// will be needed here if more than 1.
|
|
private ROFstInfo _SelectedROFst;
|
|
public ROFstInfo SelectedROFst
|
|
{
|
|
get
|
|
{
|
|
if (_SelectedROFst == null)
|
|
{
|
|
if (_DocVersionConfig.MyDocVersion.DocVersionAssociationCount < 1) return null;
|
|
_SelectedROFst = ROFstInfo.GetJustROFst(_DocVersionConfig.MyDocVersion.DocVersionAssociations[0].ROFstID);
|
|
}
|
|
return _SelectedROFst;
|
|
}
|
|
set { _SelectedROFst = value; }
|
|
}
|
|
//
|
|
|
|
public frmVersionsProperties(DocVersionConfig docVersionConfig)
|
|
{
|
|
_DocVersionConfig = docVersionConfig;
|
|
_Initializing = true;
|
|
InitializeComponent();
|
|
btnGeneral.PerformClick(); // always start with General tab or button
|
|
_Initializing = false;
|
|
//build the caption
|
|
this.Text = string.Format("{0} Properties", _DocVersionConfig.Name);
|
|
}
|
|
private string AddSlaveNode(MiniConfig mc)
|
|
{
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.LoadXml(_DocVersionConfig.ToString());
|
|
System.Xml.XmlNodeList nl = xd.SelectNodes("//Slave");
|
|
int max = 0;
|
|
foreach (System.Xml.XmlNode n in nl)
|
|
{
|
|
max = int.Parse(n.Attributes.GetNamedItem("index").InnerText);
|
|
}
|
|
max++;
|
|
System.Xml.XmlNode nn = xd.CreateElement("Slave");
|
|
AddSlaveAttribute(nn,"index",max.ToString());
|
|
AddSlaveAttribute(nn, "ID", mc.ID);
|
|
AddSlaveAttribute(nn, "Name", mc.Name);
|
|
AddSlaveAttribute(nn, "Number", mc.Number);
|
|
AddSlaveAttribute(nn, "Text", mc.Text);
|
|
AddSlaveAttribute(nn, "ProcedureNumber", mc.ProcedureNumber);
|
|
AddSlaveAttribute(nn, "SetID", mc.SetID);
|
|
AddSlaveAttribute(nn, "SetName", mc.SetName);
|
|
AddSlaveAttribute(nn, "OtherID", mc.OtherID);
|
|
AddSlaveAttribute(nn, "OtherName", mc.OtherName);
|
|
AddSlaveAttribute(nn, "OtherNumber", mc.OtherNumber);
|
|
AddSlaveAttribute(nn, "OtherText", mc.OtherText);
|
|
System.Xml.XmlNode sn = xd.SelectSingleNode("//Slaves");
|
|
sn.AppendChild(nn);
|
|
return xd.OuterXml;
|
|
}
|
|
private void AddSlaveAttribute(System.Xml.XmlNode nn,string name,string value)
|
|
{
|
|
System.Xml.XmlAttribute xa = nn.OwnerDocument.CreateAttribute(name);
|
|
xa.InnerText = value;
|
|
nn.Attributes.SetNamedItem(xa);
|
|
}
|
|
private void btnVersionsPropOK_Click(object sender, EventArgs e)
|
|
{
|
|
if (_DocVersionConfig.Unit_Count > 1)
|
|
{
|
|
if (_DeletedApples != null && _DeletedApples.Count > 0)
|
|
{
|
|
foreach(MiniConfig mc in _DeletedApples)
|
|
_DocVersionConfig.RemoveSlave(mc.Index);
|
|
}
|
|
foreach (MiniConfig mc in _Apples)
|
|
{
|
|
if (mc.IsDirty)
|
|
{
|
|
if (mc.Index < 0)
|
|
{
|
|
mc.Index = _DocVersionConfig.MaxSlaveIndex + 1;
|
|
_DocVersionConfig.AddSlave(mc.MyXml);
|
|
}
|
|
else
|
|
{
|
|
int k = _DocVersionConfig.SelectedSlave;
|
|
_DocVersionConfig.SelectedSlave = mc.Index;
|
|
_DocVersionConfig.Unit_ID = mc.ID;
|
|
_DocVersionConfig.Unit_Name = mc.Name;
|
|
_DocVersionConfig.Unit_Number = mc.Number;
|
|
_DocVersionConfig.Unit_Text = mc.Text;
|
|
_DocVersionConfig.Unit_ProcedureNumber = mc.ProcedureNumber;
|
|
_DocVersionConfig.Unit_ProcedureSetID = mc.SetID;
|
|
_DocVersionConfig.Unit_ProcedureSetName = mc.SetName;
|
|
_DocVersionConfig.Other_Unit_ID = mc.OtherID;
|
|
_DocVersionConfig.Other_Unit_Name = mc.OtherName;
|
|
_DocVersionConfig.Other_Unit_Number = mc.OtherNumber;
|
|
_DocVersionConfig.Other_Unit_Text = mc.OtherText;
|
|
_DocVersionConfig.SelectedSlave = k;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
docVersionConfigBindingSource.EndEdit();
|
|
// Save Default settings for User
|
|
//
|
|
// Save whether we should display the default values on this property page
|
|
Settings.Default.ShowDefaultVersionProp = ppCbShwDefSettings.Checked;
|
|
Settings.Default.Save();
|
|
DialogResult = DialogResult.OK;
|
|
_DocVersionConfig.MyDocVersion.Config = _DocVersionConfig.ToString();
|
|
_DocVersionConfig.MyDocVersion.Save().Dispose();
|
|
this.Close();
|
|
}
|
|
|
|
private void btnFldrPropCancel_Click(object sender, EventArgs e)
|
|
{
|
|
docVersionConfigBindingSource.CancelEdit();
|
|
this.Close();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Use the ParentLookup to grab the default values
|
|
/// - set the watermark property (where applicable) of the control with that value
|
|
/// - set the default setting labels with that value
|
|
/// ** the default setting labels appear when the Show Default Values checkbox is checked by the user.
|
|
/// </summary>
|
|
private void FindDefaultValues()
|
|
{
|
|
_DocVersionConfig.ParentLookup = true;
|
|
|
|
// get default format
|
|
_DefaultFormatName = _DocVersionConfig.DefaultFormatSelection;
|
|
SetupDefault(_DefaultFormatName, ppLblFormatDefault, ppCmbxFormat);
|
|
|
|
// Get the default Change Bar Type
|
|
_DefaultChgBarType = _DocVersionConfig.Print_ChangeBar.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_DocVersionConfig.Print_ChangeBar), ppLblChangeBarTypeDefault, ppCmbxChangeBarType);
|
|
|
|
// Get the default Change Bar Location
|
|
_DefaultChgBarLoc = _DocVersionConfig.Print_ChangeBarLoc.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_DocVersionConfig.Print_ChangeBarLoc), ppLblChgBarPosDefault, ppCmbxChgBarPos);
|
|
|
|
// Get the default Change Bar text
|
|
_DefaultChgBarText = _DocVersionConfig.Print_ChangeBarText.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_DocVersionConfig.Print_ChangeBarText), ppLblChgBarTxtTypeDefault, ppCmbxChgBarTxtType);
|
|
|
|
// Get the default User Change Bar Message 1
|
|
_DefaultChgBarUsrMsg1 = _DocVersionConfig.Print_UserCBMess1;
|
|
if (!(_DefaultChgBarUsrMsg1.Equals("")))
|
|
{
|
|
ppLblChgBarUserMsgOneDefault.Text = string.Format("({0})", _DefaultChgBarUsrMsg1);
|
|
}
|
|
|
|
// Get the default User Change Bar Message 2
|
|
_DefaultChgBarUsrMsg2 = _DocVersionConfig.Print_UserCBMess2;
|
|
if (!(_DefaultChgBarUsrMsg2.Equals("")))
|
|
{
|
|
ppLblChgBarUserMsgTwoDefault.Text = string.Format("{0}", _DefaultChgBarUsrMsg2);
|
|
}
|
|
|
|
// Get the ro path - there is no 'default'
|
|
if (_DocVersionConfig.MyDocVersion.DocVersionAssociationCount > 0)
|
|
{
|
|
RODbInfo rdi = RODbInfo.GetJustRODB(SelectedROFst.RODbID);
|
|
tbRoDb.Text = string.Format("{0} ({1})", rdi.ROName, rdi.FolderPath);
|
|
}
|
|
else
|
|
{
|
|
int selindx = -1;
|
|
cmbRoDb.Items.Clear();
|
|
int myrodbid = -1;
|
|
if (_DocVersionConfig.MyDocVersion.DocVersionAssociationCount >= 1) myrodbid = SelectedROFst.RODbID;
|
|
foreach (RODbInfo rdi in RODbInfoList.Get())
|
|
{
|
|
int i = cmbRoDb.Items.Add(string.Format("{0} ({1})", rdi.ROName, rdi.FolderPath));
|
|
if (rdi.RODbID == myrodbid) selindx = i;
|
|
}
|
|
if (cmbRoDb.Items.Count > 0) cmbRoDb.SelectedIndex = selindx;
|
|
}
|
|
|
|
// Get the default Print Pagination
|
|
//_DefaultPagination = _DocVersionConfig.Print_Pagination.ToString();
|
|
//SetupDefault(EnumDescConverter.GetEnumDescription(_DocVersionConfig.Print_Pagination), ppLblPaginationDefault, ppCmbxPagination);
|
|
|
|
// Get the default Watermark
|
|
_DefaultWatermark = _DocVersionConfig.Print_Watermark.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_DocVersionConfig.Print_Watermark), ppLblWatermarkDefault, ppCmbxWatermark);
|
|
|
|
// Get the default Disable Duplex
|
|
_DefaultDisableDuplex = _DocVersionConfig.Print_DisableDuplex;
|
|
ppLblAutoDuplexDefault.Text = string.Format("(Duplex {0})", (_DefaultDisableDuplex) ? "OFF" : "ON");
|
|
|
|
_DocVersionConfig.ParentLookup = false;
|
|
}
|
|
|
|
|
|
private void frmVersionsProperties_Load(object sender, EventArgs e)
|
|
{
|
|
_Initializing = true;
|
|
docVersionConfigBindingSource.DataSource = _DocVersionConfig;
|
|
|
|
//formatInfoListBindingSource.DataSource = FormatInfoList.Get();
|
|
imageCodecInfoBindingSource.DataSource = ImageCodecInfo.GetImageDecoders();
|
|
|
|
ppCmbxFormat.DataSource = null;
|
|
ppCmbxFormat.DisplayMember = "FullName";
|
|
ppCmbxFormat.ValueMember = "FullName";
|
|
ppCmbxFormat.DataSource = FormatInfoList.SortedFormatInfoList;
|
|
|
|
// Get the saved settings for this user
|
|
//
|
|
// Get setting telling us whether to display the default values on this property page
|
|
ppCbShwDefSettings.Checked = (Settings.Default["ShowDefaultVersionProp"] != null) ? Settings.Default.ShowDefaultVersionProp : false;
|
|
|
|
// Get the User's property page style "PropPageStyle" (this is a system wide user setting)
|
|
// 1 - Button Dialog (default)
|
|
// 2 - Tab Dialog
|
|
if ((int)Settings.Default["PropPageStyle"] == (int)PropPgStyle.Tab)
|
|
{
|
|
tcVersions.TabsVisible = true;
|
|
panVerBtns.Visible = false;
|
|
this.Width -= panVerBtns.Width;
|
|
}
|
|
|
|
// Get the default values for the property page information
|
|
FindDefaultValues();
|
|
|
|
ppCmbxChangeBarType.DataSource = EnumDetail<PrintChangeBar>.Details();
|
|
ppCmbxChangeBarType.DisplayMember = "Description";
|
|
ppCmbxChangeBarType.ValueMember = "EValue";
|
|
ppCmbxChangeBarType.SelectedIndex = -1;
|
|
|
|
ppCmbxChgBarPos.DataSource = EnumDetail<PrintChangeBarLoc>.Details();
|
|
ppCmbxChgBarPos.DisplayMember = "Description";
|
|
ppCmbxChgBarPos.ValueMember = "EValue";
|
|
ppCmbxChgBarPos.SelectedIndex = -1;
|
|
|
|
ppCmbxChgBarTxtType.DataSource = EnumDetail<PrintChangeBarText>.Details();
|
|
ppCmbxChgBarTxtType.DisplayMember = "Description";
|
|
ppCmbxChgBarTxtType.ValueMember = "EValue";
|
|
ppCmbxChgBarTxtType.SelectedItem = -1;
|
|
|
|
ppCmbxWatermark.DataSource = EnumDetail<PrintWatermark>.Details();
|
|
ppCmbxWatermark.DisplayMember = "Description";
|
|
ppCmbxWatermark.ValueMember = "EValue";
|
|
ppCmbxWatermark.SelectedIndex = -1;
|
|
|
|
//ppCmbxPagination.DataSource = EnumDetail<PrintPagination>.Details();
|
|
//ppCmbxPagination.DisplayMember = "Description";
|
|
//ppCmbxPagination.ValueMember = "EValue";
|
|
//ppCmbxPagination.SelectedIndex = -1;
|
|
|
|
ppCmbxProcSetType.DataSource = EnumDetail<VersionTypeEnum>.Details();
|
|
ppCmbxProcSetType.DisplayMember = "Description";
|
|
ppCmbxProcSetType.ValueMember = "EValue";
|
|
ppCmbxProcSetType.Enabled = false; // maybe enable this if used for version creation
|
|
|
|
documentInfoListBindingSource.DataSource = DocumentInfoList.GetLibraries(false);
|
|
|
|
ppBtnRoDbBrowse.Visible = cmbRoDb.Visible = _DocVersionConfig.MyDocVersion.DocVersionAssociationCount == 0;
|
|
btnRoDbProperties.Visible = tbRoDb.Visible = !(_DocVersionConfig.MyDocVersion.DocVersionAssociationCount == 0);
|
|
ppBtnUpRoVals.Enabled = _DocVersionConfig.MyDocVersion.NewerRoFst; // only allow update if association
|
|
|
|
// Set the auto duplex controls based on whether the format allows this:
|
|
// Note that the controls' visibility would not set correctly using the following two lines of code. That
|
|
// is why the more explicit logic was done.
|
|
//ppChbxDisAutoDuplex.Visible = _DocVersionConfig.MyDocVersion.MyFormat.PlantFormat.FormatData.PrintData.AllowDuplex;
|
|
//ppLblAutoDuplexDefault.Visible = ppBtnDeftDisAutoDuplx.Visible = ppLblAutoDuplexDefault.Visible;
|
|
if (_DocVersionConfig.MyDocVersion.MyFormat != null && _DocVersionConfig.MyDocVersion.MyFormat.PlantFormat.FormatData.PrintData.AllowDuplex)
|
|
{
|
|
ppChbxDisAutoDuplex.Visible = true;
|
|
ppLblAutoDuplexDefault.Visible = true;
|
|
ppBtnDeftDisAutoDuplx.Visible = true;
|
|
}
|
|
else
|
|
{
|
|
ppChbxDisAutoDuplex.Visible = false;
|
|
ppLblAutoDuplexDefault.Visible = false;
|
|
ppBtnDeftDisAutoDuplx.Visible = false;
|
|
}
|
|
//add new applicability stuff
|
|
if (_DocVersionConfig.Unit_Count > 1)
|
|
{
|
|
int k = _DocVersionConfig.SelectedSlave;
|
|
_Apples = new List<MiniConfig>();
|
|
for (int i = 1; i <= _DocVersionConfig.MaxSlaveIndex; i++)
|
|
{
|
|
_DocVersionConfig.SelectedSlave = i;
|
|
try
|
|
{
|
|
MiniConfig cfg = new MiniConfig(i, _DocVersionConfig.Unit_ID, _DocVersionConfig.Unit_Name, _DocVersionConfig.Unit_Number, _DocVersionConfig.Unit_Text, _DocVersionConfig.Unit_ProcedureNumber, _DocVersionConfig.Unit_ProcedureSetID, _DocVersionConfig.Unit_ProcedureSetName, _DocVersionConfig.Other_Unit_ID, _DocVersionConfig.Other_Unit_Name, _DocVersionConfig.Other_Unit_Number, _DocVersionConfig.Other_Unit_Text);
|
|
_Apples.Add(cfg);
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
}
|
|
_DocVersionConfig.SelectedSlave = k;
|
|
bsApples.DataSource = _Apples;
|
|
}
|
|
else
|
|
{
|
|
btnApplicability.Visible = false;
|
|
tiApplicability.Visible = false;
|
|
}
|
|
ppTxtBxPDFLoc.TextChanged += new EventHandler(ppTxtBxPDFLoc_TextChanged);
|
|
//end add new applicability stuff
|
|
_Initializing = false;
|
|
}
|
|
// The following code was added to fix Bug B2013-117
|
|
void ppTxtBxPDFLoc_TextChanged(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing == false)
|
|
_DocVersionConfig.Print_PDFLocation = ppTxtBxPDFLoc.Text;
|
|
}
|
|
#region General tab
|
|
|
|
/// <summary>
|
|
/// This is the General button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnGeneral_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiGeneral, btnGeneral);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Referenced Objects
|
|
|
|
/// <summary>
|
|
/// This is the Referenced Objects button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnRefObjs_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiRefObjs, btnRefObjs);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Output Settings
|
|
|
|
/// <summary>
|
|
/// This is the Output Settings button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnOutputSettings_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiOutputSettings, btnOutputSettings);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Pagination combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
//private void ppCmbxPagination_SelectedValueChanged(object sender, EventArgs e)
|
|
//{
|
|
// if (!_Initializing)
|
|
// {
|
|
// PrintPagination pgtn = (PrintPagination)Enum.Parse(typeof(PrintPagination), _DefaultPagination);
|
|
// ProcessCmbxSelectionEnumChanged(ppCmbxPagination, pgtn, ppBtnDefPagination, ppLblPaginationDefault);
|
|
// }
|
|
//}
|
|
|
|
/// <summary>
|
|
/// Reset to the parent setting.
|
|
/// Find the parent setting and assign it to _DocVersionConfig.Print_Pagination.
|
|
/// This will force the database to be updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
//private void ppBtnDefPagination_Click(object sender, EventArgs e)
|
|
//{
|
|
// // Get the parent setting
|
|
// PrintPagination pgtn = (PrintPagination)Enum.Parse(typeof(PrintPagination), _DefaultPagination);
|
|
// // Compare parent setting with current setting
|
|
// if (pgtn != _DocVersionConfig.Print_Pagination)
|
|
// _DocVersionConfig.Print_Pagination = pgtn; // this will force a database update (write)
|
|
// ppCmbxPagination.SelectedIndex = -1;
|
|
// //tcpOutputSettings.Focus();
|
|
//}
|
|
|
|
/// <summary>
|
|
/// Selection in Watermark combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppCmbxWatermark_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_Initializing)
|
|
{
|
|
PrintWatermark wtr = (PrintWatermark)Enum.Parse(typeof(PrintWatermark), _DefaultWatermark);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxWatermark, wtr, ppBtnDefWatermark, ppLblWatermarkDefault);
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Reset to the parent setting.
|
|
/// Find the parent setting and assign it to _DocVersionConfig.Print_Watermark.
|
|
/// This will force the database to be updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void ppBtnDefWatermark_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
PrintWatermark wtr = (PrintWatermark)Enum.Parse(typeof(PrintWatermark), _DefaultWatermark);
|
|
// Compare parent setting with current setting
|
|
if (wtr != _DocVersionConfig.Print_Watermark)
|
|
_DocVersionConfig.Print_Watermark = wtr; // this will force a database update (write)
|
|
ppCmbxWatermark.SelectedIndex = -1;
|
|
//tcpOutputSettings.Focus();
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Format Settings
|
|
|
|
/// <summary>
|
|
/// This is the Format Settings button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnFmtSettings_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiFmtSettings, btnFmtSettings);
|
|
}
|
|
|
|
private void ppBtnDefaultFmt_Click(object sender, EventArgs e)
|
|
{
|
|
ppCmbxFormat.SelectedIndex = -1;
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
|
|
/// <summary>
|
|
/// If checked, will disable automatic duplexing (ex Foldout Pages)
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void ppChbxDisAutoDuplex_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_Initializing)
|
|
{
|
|
_DocVersionConfig.Print_DisableDuplex = ppChbxDisAutoDuplex.Checked;
|
|
ppBtnDeftDisAutoDuplx.Visible = (_DefaultDisableDuplex != ppChbxDisAutoDuplex.Checked);
|
|
ppLblAutoDuplexDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDeftDisAutoDuplx.Visible;
|
|
}
|
|
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Format combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppCmbxFormat_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
ProcessCmbxSelectedValueChange(ppCmbxFormat, _DefaultFormatName, ppBtnDefaultFmt, ppLblFormatDefault);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Enable or disable the user specified change bar options base on the type
|
|
/// of change bar selected.
|
|
/// </summary>
|
|
private void setEnabledUserSpecifiedChgBarCombos(PrintChangeBar pcb)
|
|
{
|
|
//ppGpbxUserSpecCB.Enabled =
|
|
//ppCmbxChgBarPos.Enabled =
|
|
//ppCmbxChgBarTxtType.Enabled =
|
|
//ppBtnDefaultCbPos.Enabled =
|
|
//ppBtnDefCbTxtTyp.Enabled = (ppCmbxChangeBarType.SelectedValue != null &&
|
|
//ppCmbxChangeBarType.SelectedValue.Equals(DocVersionConfig.PrintChangeBar.WithUserSpecified)) ||
|
|
//(ppCmbxChangeBarType.SelectedValue == null && pcb.Equals(DocVersionConfig.PrintChangeBar.WithUserSpecified));
|
|
|
|
ppGpbxUserSpecCB.Enabled = (ppCmbxChangeBarType.SelectedValue != null &&
|
|
ppCmbxChangeBarType.SelectedValue.Equals(PrintChangeBar.WithUserSpecified)) ||
|
|
(ppCmbxChangeBarType.SelectedValue == null && pcb.Equals(PrintChangeBar.WithUserSpecified));
|
|
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Change Bar combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppCmbxChangeBarType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_Initializing)
|
|
{
|
|
PrintChangeBar pcb = (PrintChangeBar)Enum.Parse(typeof(PrintChangeBar), _DefaultChgBarType);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxChangeBarType, pcb, ppBtnDefaultChgBar, ppLblChangeBarTypeDefault);
|
|
setEnabledUserSpecifiedChgBarCombos(pcb);
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Reset to the parent setting.
|
|
/// Find the parent setting and assign it to _DocVersionConfig.PrintChangeBar.
|
|
/// This will force the database to be updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void ppBtnDefaultChgBar_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
PrintChangeBar pcb = (PrintChangeBar)Enum.Parse(typeof(PrintChangeBar), _DefaultChgBarType);
|
|
// Compare parent setting with current setting
|
|
if (pcb != _DocVersionConfig.Print_ChangeBar)
|
|
_DocVersionConfig.Print_ChangeBar = pcb; // this will force a database update (write)
|
|
ppCmbxChangeBarType.SelectedIndex = -1; //reset combo box to the default Change Bar setting
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Change Bar Position combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppCmbxChgBarPos_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_Initializing)
|
|
{
|
|
PrintChangeBarLoc cbl = (PrintChangeBarLoc)Enum.Parse(typeof(PrintChangeBarLoc), _DefaultChgBarLoc);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxChgBarPos, cbl, ppBtnDefaultCbPos, ppLblChgBarPosDefault);
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Reset to the parent setting.
|
|
/// Find the parent setting and assign it to _DocVersionConfig.Print_ChangeBarLoc.
|
|
/// This will force the database to be updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void ppBtnDefaultCbPos_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
PrintChangeBarLoc cbl = (PrintChangeBarLoc)Enum.Parse(typeof(PrintChangeBarLoc), _DefaultChgBarLoc);
|
|
// Compare parent setting with current setting
|
|
if (cbl != _DocVersionConfig.Print_ChangeBarLoc)
|
|
_DocVersionConfig.Print_ChangeBarLoc = cbl; // this will force a database update (write)
|
|
ppCmbxChgBarPos.SelectedIndex = -1;
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Enable or disable the user specified change bar text based on the selected
|
|
/// change bar text type (selected in the user specific change bar grouping)
|
|
/// </summary>
|
|
private void setEnabledUserSpecifiedChgBarText()
|
|
{
|
|
//ppGpbxUserSpecTxt.Enabled =
|
|
//ppTxbxChangeBarUserMsgOne.Enabled =
|
|
//ppTxbxChangeBarUserMsgTwo.Enabled =
|
|
//ppBtnDefCbTxt1.Enabled =
|
|
//ppBtnDefCbTxt2.Enabled = (ppCmbxChgBarTxtType.SelectedValue != null &&
|
|
//ppCmbxChgBarTxtType.SelectedValue.Equals(DocVersionConfig.PrintChangeBarText.UserDef));
|
|
|
|
//string decUserDef = EnumDescConverter.GetEnumDescription(DocVersionConfig.PrintChangeBarText.UserDef);
|
|
|
|
// This string is used to check against our default setting to see if User Defined Changebar Text is active
|
|
string decUserDef = PrintChangeBarText.UserDef.ToString();
|
|
|
|
ppGpbxUserSpecTxt.Enabled = (ppCmbxChgBarTxtType.SelectedValue != null &&
|
|
ppCmbxChgBarTxtType.SelectedValue.Equals(PrintChangeBarText.UserDef)) ||
|
|
(ppCmbxChgBarTxtType.SelectedIndex == -1 && _DefaultChgBarText.Equals(decUserDef));
|
|
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Change Bar Text Type combo box changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppCmbxChgBarTxtType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_Initializing)
|
|
{
|
|
PrintChangeBarText cbt = (PrintChangeBarText)Enum.Parse(typeof(PrintChangeBarText), _DefaultChgBarText);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxChgBarTxtType, cbt, ppBtnDefCbTxtTyp, ppLblChgBarTxtTypeDefault);
|
|
setEnabledUserSpecifiedChgBarText();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Reset to the parent setting.
|
|
/// Find the parent setting and assign it to _DocVersionConfig.Print_ChangeBarText.
|
|
/// This will force the database to be updated.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
private void ppBtnDefCbTxtTyp_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
PrintChangeBarText cbt = (PrintChangeBarText)Enum.Parse(typeof(PrintChangeBarText), _DefaultChgBarText);
|
|
// Compare parent setting with current setting
|
|
if (cbt != _DocVersionConfig.Print_ChangeBarText)
|
|
_DocVersionConfig.Print_ChangeBarText = cbt; // this will force a database update (write)
|
|
ppCmbxChgBarTxtType.SelectedIndex = -1;
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Change Bar User Message One text changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppTxbxChangeBarUserMsgOne_TextChanged(object sender, EventArgs e)
|
|
{
|
|
ppBtnDefCbTxt1.Visible = ((ppTxbxChangeBarUserMsgOne.Text != null) && !ppTxbxChangeBarUserMsgOne.Text.Equals(_DefaultChgBarUsrMsg1));
|
|
ppLblChgBarUserMsgOneDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefCbTxt1.Visible;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Selection in Change Bar User Message Two text changed.
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void ppTxbxChangeBarUserMsgTwo_TextChanged(object sender, EventArgs e)
|
|
{
|
|
ppBtnDefCbTxt2.Visible = ((ppTxbxChangeBarUserMsgTwo.Text != null) && !ppTxbxChangeBarUserMsgTwo.Text.Equals(_DefaultChgBarUsrMsg2));
|
|
ppLblChgBarUserMsgTwoDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefCbTxt2.Visible;
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Library Documents
|
|
|
|
/// <summary>
|
|
/// This is the Library Documents button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnLibDocs_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiLibDocs, btnLibDocs);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Generic functions used on this property page
|
|
|
|
/// <summary>
|
|
/// This is a generic Enter Event function for use with all of the property page tabs.
|
|
/// Found that the visiblity value of buttons is not recorded until the property page in which it resides is diplayed.
|
|
/// Thus we need to call defaultSettingVisiblity() to check and set visiblity states.
|
|
/// </summary>
|
|
/// <param name="sender"> type object</param>
|
|
/// <param name="e">type EventArgs</param>
|
|
private void tabpage_Enter(object sender, EventArgs e)
|
|
{
|
|
// Show or hide the labels containing the default values
|
|
//if (!_Initializing)
|
|
defaultSettingsVisiblity();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Determines what labels (showing default values) are visable on the property pages
|
|
/// </summary>
|
|
private void defaultSettingsVisiblity()
|
|
{
|
|
ppLblDefSettingsInfo.Visible = ppCbShwDefSettings.Checked;
|
|
|
|
ppLblChgBarUserMsgOneDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefCbTxt1.Visible;
|
|
ppLblChgBarUserMsgTwoDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefCbTxt2.Visible;
|
|
|
|
ppLblFormatDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefaultFmt.Visible;
|
|
ppLblChangeBarTypeDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefaultChgBar.Visible;
|
|
ppLblChgBarPosDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefaultCbPos.Visible;
|
|
ppLblChgBarTxtTypeDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefCbTxtTyp.Visible;
|
|
//ppLblPaginationDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefPagination.Visible;
|
|
ppLblWatermarkDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefWatermark.Visible;
|
|
ppLblAutoDuplexDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDeftDisAutoDuplx.Visible;
|
|
}
|
|
|
|
/// <summary>
|
|
/// For the Button Interface property page style, when a button is selected (pressed),
|
|
/// it will remain in the checked state even when a different button is selected. Thus
|
|
/// we must clear the checked state of the buttons when a button is selected, then set
|
|
/// the newly selected button's state to checked.
|
|
/// </summary>
|
|
private void ClearAllCheckedButtons()
|
|
{
|
|
btnGeneral.Checked = false;
|
|
btnRefObjs.Checked = false;
|
|
btnOutputSettings.Checked = false;
|
|
btnFmtSettings.Checked = false;
|
|
btnLibDocs.Checked = false;
|
|
btnApplicability.Checked = false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Select the corresponding tab and set the button's state to checked
|
|
/// </summary>
|
|
/// <param name="tab">Property Page Tab</param>
|
|
/// <param name="button">Corresponding Property Page Button</param>
|
|
private void ProcessButtonClick(TabItem tab, ButtonX button)
|
|
{
|
|
ClearAllCheckedButtons();
|
|
tcVersions.SelectedTab = tab;
|
|
button.Checked = true;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Process a change in the enum combo box selection
|
|
/// </summary>
|
|
/// <param name="cmbx">Combo Box Name</param>
|
|
/// <param name="defstr">the default enum value</param>
|
|
/// <param name="button">button to reset to default value</param>
|
|
/// <param name="deflabel">label containing the default</param>
|
|
private void ProcessCmbxSelectionEnumChanged(ComboBoxEx cmbx, object enumval, ButtonX button, Label deflabel)
|
|
{
|
|
if ((cmbx.SelectedIndex != -1) &&
|
|
cmbx.SelectedValue.Equals(enumval))
|
|
{
|
|
_Initializing = true;
|
|
button.Visible = true;
|
|
button.Focus();
|
|
button.PerformClick();
|
|
cmbx.SelectedIndex = -1; // This will hide the Default button
|
|
_Initializing = false;
|
|
}
|
|
button.Visible = (cmbx.SelectedValue != null);
|
|
deflabel.Visible = ppCbShwDefSettings.Checked && button.Visible;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Process a change in the combo box selection
|
|
/// </summary>
|
|
/// <param name="cmbx">Combo Box Name</param>
|
|
/// <param name="defstr">string containing default text</param>
|
|
/// <param name="button">button to reset to default value</param>
|
|
/// <param name="deflabel">label containing the default</param>
|
|
private void ProcessCmbxSelectedValueChange(ComboBoxEx cmbx, string defstr, ButtonX button, Label deflabel)
|
|
{
|
|
if ((cmbx.SelectedIndex != -1) && defstr != null && defstr.Equals(cmbx.SelectedValue))
|
|
{
|
|
button.Visible = true;
|
|
button.Focus();
|
|
button.PerformClick();
|
|
}
|
|
button.Visible = cmbx.SelectedValue != null;
|
|
deflabel.Visible = ppCbShwDefSettings.Checked && button.Visible;
|
|
}
|
|
/// <summary>
|
|
/// Set the watermark and default label
|
|
/// </summary>
|
|
/// <param name="defaultText">The default text</param>
|
|
/// <param name="lbl">Label that displays the current default when view defaults is set</param>
|
|
/// <param name="cmboEx">Combo box with a watermark property</param>
|
|
private static void SetupDefault(string defaultText, Label lbl, ComboBoxEx cmbo)
|
|
{
|
|
if (defaultText != null && !(defaultText.Equals("")))
|
|
{
|
|
string deftext = string.Format("{0}", defaultText);
|
|
lbl.Text = deftext;
|
|
cmbo.WatermarkText = deftext;
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
private void btnRoDbProperties_Click(object sender, EventArgs e)
|
|
{
|
|
frmRODbProperties dlgROProperties = new frmRODbProperties(_DocVersionConfig.MyDocVersion, SelectedROFst == null ? null : SelectedROFst.MyRODb);
|
|
dlgROProperties.ParentLocation = Location;
|
|
if (dlgROProperties.ShowDialog() == DialogResult.OK)
|
|
{
|
|
tbRoDb.Text = string.Format("{0} ({1})", SelectedROFst.MyRODb.ROName, SelectedROFst.MyRODb.FolderPath);
|
|
ppBtnUpRoVals.Enabled = _DocVersionConfig.MyDocVersion.NewerRoFst; // only allow update if association
|
|
}
|
|
}
|
|
|
|
private void ppBtnUpRoVals_Click(object sender, EventArgs e)
|
|
{
|
|
// use rodb directory path of the first rofst for the this document version. Bring up a file
|
|
// selection dialog starting with this path. The user can select another path.
|
|
// RHM question - we talked about just using the current path, but what is the current path when
|
|
// a docversion can have more than one rofst (for now, just use first?) - should I bring up a dialog?
|
|
if (_DocVersionConfig.MyDocVersion.DocVersionAssociations.Count < 1)
|
|
{
|
|
MessageBox.Show("Error Updating ro.fst. No associated ro.fst");
|
|
return;
|
|
}
|
|
foreach (DocVersionAssociation dva in _DocVersionConfig.MyDocVersion.DocVersionAssociations)
|
|
{
|
|
RODbInfo rdi = RODbInfo.Get(dva.ROFst_RODbID);
|
|
|
|
string rofstPath = rdi.FolderPath + @"\ro.fst";
|
|
if (!File.Exists(rofstPath))
|
|
{
|
|
MessageBox.Show("No existing ro.fst in path " + rdi.FolderPath + ". Check for invalid path");
|
|
break;
|
|
}
|
|
FileInfo fiRofst = new FileInfo(rofstPath);
|
|
if (SelectedROFst.DTS == fiRofst.LastWriteTimeUtc)
|
|
{
|
|
MessageBox.Show("ro.fst files are same for path " + rdi.FolderPath + ", import of that ro.fst will not be done");
|
|
break;
|
|
}
|
|
if (SelectedROFst.DTS > fiRofst.LastWriteTimeUtc)
|
|
{
|
|
MessageBox.Show("Cannot copy older ro.fst from " + rdi.FolderPath + ", import of that ro.fst will not be done");
|
|
break;
|
|
}
|
|
Cursor = Cursors.WaitCursor;
|
|
SelectedROFst.ROTableUpdate += new ROFstInfoROTableUpdateEvent(roFstInfo_ROTableUpdate);
|
|
ROFst newrofst = ROFstInfo.UpdateRoFst(rdi, dva, _DocVersionConfig.MyDocVersion, SelectedROFst);
|
|
SelectedROFst.ROTableUpdate -= new ROFstInfoROTableUpdateEvent(roFstInfo_ROTableUpdate);
|
|
ppBtnUpRoVals.Enabled = _DocVersionConfig.MyDocVersion.NewerRoFst;
|
|
Cursor = Cursors.Default;
|
|
}
|
|
}
|
|
public List<string> roFstInfo_ROTableUpdate(object sender, ROFstInfoROTableUpdateEventArgs args)
|
|
{
|
|
string xml = null;
|
|
string srchtxt = null;
|
|
using (VlnFlexGrid myGrid = new VlnFlexGrid())
|
|
{
|
|
using (StringReader sr = new StringReader(args.OldGridXml))
|
|
{
|
|
myGrid.ReadXml(sr);
|
|
myGrid.KeyActionTab = C1.Win.C1FlexGrid.KeyActionEnum.MoveAcross;
|
|
sr.Close();
|
|
}
|
|
string roid = myGrid.ROID;
|
|
int rodbid = myGrid.RODbId;
|
|
Font GridFont = myGrid.Font;
|
|
myGrid.Clear();
|
|
myGrid.ParseTableFromText(args.ROText, GridLinePattern.Single);
|
|
myGrid.AutoSizeCols();
|
|
myGrid.AutoSizeRows();
|
|
myGrid.MakeRTFcells();
|
|
myGrid.RODbId = rodbid;
|
|
myGrid.ROID = roid;
|
|
myGrid.IsRoTable = true;
|
|
using (StringWriter sw = new StringWriter())
|
|
{
|
|
myGrid.WriteXml(sw);
|
|
xml = sw.GetStringBuilder().ToString();
|
|
sw.Close();
|
|
}
|
|
srchtxt = myGrid.GetSearchableText();
|
|
}
|
|
List<string> retlist = new List<string>();
|
|
retlist.Add(srchtxt);
|
|
retlist.Add(xml);
|
|
return retlist;
|
|
}
|
|
private void ppBtnResetRoVals_Click(object sender, EventArgs e)
|
|
{
|
|
// This will not be supported in the first release. A discussion was held on 1/6/09 with MRC, JSJ, RHM &
|
|
// KBR & it was decided that we'd wait to see if users end up needing this. It can be done by going
|
|
// into the sql database and modify/delete records if needed.
|
|
}
|
|
|
|
private void cmbRoDb_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
// The only way that a selected index can change on the rodb combo box is if there was no rofst
|
|
// to docversion association. If a selection is made, make that association and then change the
|
|
// combo box to a non-editable text box with button becoming a properties rather than browse.
|
|
if (_Initializing) return;
|
|
// add the rofst for the selected rodb (note that it may already exist, and will use the current
|
|
// one.
|
|
// get the rodb from the selection - and then do an 'update rofst'...
|
|
RODbInfoList rdil = RODbInfoList.Get();
|
|
RODbInfo rdi = rdil[cmbRoDb.SelectedIndex];
|
|
ROFst tmp = ROFstInfo.AddRoFst(rdi, _DocVersionConfig.MyDocVersion);
|
|
if (tmp == null)
|
|
{
|
|
MessageBox.Show("Invalid ro fst directory, use the Property dialog to fix directory path to ro.fst.");
|
|
frmRODbProperties dlgROProperties = new frmRODbProperties(_DocVersionConfig.MyDocVersion, rdi);
|
|
if (dlgROProperties.ShowDialog() == DialogResult.OK)
|
|
{
|
|
tbRoDb.Text = string.Format("{0} ({1})", SelectedROFst.MyRODb.ROName, SelectedROFst.MyRODb.FolderPath);
|
|
ppBtnUpRoVals.Enabled = _DocVersionConfig.MyDocVersion.NewerRoFst; // only allow update if association
|
|
}
|
|
else
|
|
return;
|
|
}
|
|
cmbRoDb.Visible = ppBtnRoDbBrowse.Visible = false;
|
|
tbRoDb.Visible = btnRoDbProperties.Visible = true;
|
|
tbRoDb.Text = string.Format("{0} ({1})", tmp.MyRODb.ROName, tmp.MyRODb.FolderPath);
|
|
}
|
|
|
|
private void ppBtnRoDbBrowse_Click(object sender, EventArgs e)
|
|
{
|
|
frmRODbProperties dlgROProperties = new frmRODbProperties(_DocVersionConfig.MyDocVersion, SelectedROFst == null ? null : SelectedROFst.MyRODb);
|
|
dlgROProperties.ParentLocation = Location;
|
|
// if a user has entered a valid rodb, then this docversion will be conntected to it - change
|
|
// to a non-editable text box and the button becomes a properties button rather than browse.
|
|
if (dlgROProperties.ShowDialog() == DialogResult.OK)
|
|
{
|
|
cmbRoDb.Items.Clear();
|
|
_DocVersionConfig.MyDocVersion.Reset_DocVersionAssociations();
|
|
if (_DocVersionConfig.MyDocVersion.DocVersionAssociations != null && _DocVersionConfig.MyDocVersion.DocVersionAssociationCount > 0)
|
|
{
|
|
cmbRoDb.Visible = ppBtnRoDbBrowse.Visible = false;
|
|
tbRoDb.Visible = btnRoDbProperties.Visible = true;
|
|
tbRoDb.Text = string.Format("{0} ({1})", SelectedROFst.MyRODb.ROName, SelectedROFst.MyRODb.FolderPath);
|
|
return;
|
|
}
|
|
RODbInfoList.Reset();
|
|
int selindx = -1;
|
|
int myrodbid = (SelectedROFst == null) ? -1 : SelectedROFst.RODbID;
|
|
foreach (RODbInfo rdi in RODbInfoList.Get())
|
|
{
|
|
int i = cmbRoDb.Items.Add(rdi.ROName + " (" + rdi.FolderPath + ")");
|
|
if (rdi.RODbID == myrodbid) selindx = i;
|
|
}
|
|
if (cmbRoDb.Items.Count > 0) cmbRoDb.SelectedIndex = selindx;
|
|
}
|
|
}
|
|
|
|
private void ppBtnPDFLoc_Click(object sender, EventArgs e)
|
|
{
|
|
PDFLocationBrowserDialog.SelectedPath = ppTxtBxPDFLoc.Text;
|
|
DialogResult dr = PDFLocationBrowserDialog.ShowDialog();
|
|
if (dr == DialogResult.OK)
|
|
ppTxtBxPDFLoc.Text = PDFLocationBrowserDialog.SelectedPath;
|
|
}
|
|
|
|
private void ppBtnDefCbTxt1_Click(object sender, EventArgs e)
|
|
{
|
|
// Compare default setting with current setting
|
|
// Reset with the default and hide the default button and label
|
|
if (_DefaultChgBarUsrMsg1 != _DocVersionConfig.Print_UserCBMess1)
|
|
{
|
|
_DocVersionConfig.Print_UserCBMess1 = _DefaultChgBarUsrMsg1;
|
|
ppLblChgBarUserMsgOneDefault.Visible = false;
|
|
ppBtnDefCbTxt1.Visible = false;
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
}
|
|
|
|
private void ppBtnDefCbTxt2_Click(object sender, EventArgs e)
|
|
{
|
|
// Compare default setting with current setting
|
|
// Reset with the default and hide the default button and label
|
|
if (_DefaultChgBarUsrMsg2 != _DocVersionConfig.Print_UserCBMess2)
|
|
{
|
|
_DocVersionConfig.Print_UserCBMess2 = _DefaultChgBarUsrMsg2;
|
|
ppLblChgBarUserMsgTwoDefault.Visible = false;
|
|
ppBtnDefCbTxt2.Visible = false;
|
|
//tcpFormatSettings.Focus();
|
|
}
|
|
}
|
|
|
|
private void ppBtnDeftDisAutoDuplx_Click(object sender, EventArgs e)
|
|
{
|
|
ppChbxDisAutoDuplex.Checked = _DefaultDisableDuplex;
|
|
_DocVersionConfig.Print_DisableDuplex = ppChbxDisAutoDuplex.Checked;
|
|
ppBtnDeftDisAutoDuplx.Visible = false;
|
|
ppLblAutoDuplexDefault.Visible = false;
|
|
//tcpOutputSettings.Focus();
|
|
}
|
|
|
|
private void frmVersionsProperties_Shown(object sender, EventArgs e)
|
|
{
|
|
ppRTxtName.Focus();
|
|
}
|
|
|
|
private void ppRTxtName_Leave(object sender, EventArgs e)
|
|
{
|
|
if (ppRTxtName.Text == null || ppRTxtName.Text == "")
|
|
{
|
|
MessageBox.Show("Cannot have a blank Name.");
|
|
return;
|
|
}
|
|
}
|
|
|
|
private void btnApplicability_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiApplicability, btnApplicability);
|
|
}
|
|
|
|
private void lbApplicabilities_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
if (lbApplicabilities.SelectedIndex > -1)
|
|
{
|
|
bsMiniApple.DataSource = bsApples.Current as MiniConfig;
|
|
btnDelApple.Enabled = true;
|
|
}
|
|
|
|
}
|
|
private void btnNewApple_Click(object sender, EventArgs e)
|
|
{
|
|
MiniConfig cfg = new MiniConfig();
|
|
cfg.Name = "New Applicability";
|
|
_Apples.Add(cfg);
|
|
bsApples.DataSource = null;
|
|
bsApples.DataSource = _Apples;
|
|
lbApplicabilities.SelectedItem = cfg;
|
|
}
|
|
private void btnDelApple_Click(object sender, EventArgs e)
|
|
{
|
|
MiniConfig cfg = bsApples.Current as MiniConfig;
|
|
if (MessageBox.Show(string.Format("Are you sure you want to delete {0}", cfg.Name), "Confirm Delete", MessageBoxButtons.YesNoCancel, MessageBoxIcon.Question) == DialogResult.Yes)
|
|
{
|
|
if (_DeletedApples == null)
|
|
_DeletedApples = new List<MiniConfig>();
|
|
_DeletedApples.Add(cfg);
|
|
_Apples.Remove(cfg);
|
|
bsApples.DataSource = null;
|
|
bsApples.DataSource = _Apples;
|
|
}
|
|
}
|
|
}
|
|
|
|
[XmlRoot("Slave")]
|
|
public class MiniConfig
|
|
{
|
|
private static int lastindex = 0;
|
|
private bool _isDeleted;
|
|
[XmlIgnore]
|
|
public bool IsDeleted
|
|
{
|
|
get { return _isDeleted; }
|
|
set { _isDeleted = value; }
|
|
}
|
|
private bool _isDirty;
|
|
[XmlIgnore]
|
|
public bool IsDirty
|
|
{
|
|
get { return _isDirty; }
|
|
set { _isDirty = value; }
|
|
}
|
|
private int _index;
|
|
[XmlAttribute("index")]
|
|
public int Index
|
|
{
|
|
get { return _index; }
|
|
set { _index = value; IsDirty = true; }
|
|
}
|
|
private string _iD;
|
|
[XmlAttribute("ID")]
|
|
public string ID
|
|
{
|
|
get { return _iD; }
|
|
set { _iD = value; IsDirty = true; }
|
|
}
|
|
private string _name;
|
|
[XmlAttribute("Name")]
|
|
public string Name
|
|
{
|
|
get { return _name; }
|
|
set { _name = value; IsDirty = true; }
|
|
}
|
|
private string _number;
|
|
[XmlAttribute("Number")]
|
|
public string Number
|
|
{
|
|
get { return _number; }
|
|
set { _number = value; IsDirty = true; }
|
|
}
|
|
private string _text;
|
|
[XmlAttribute("Text")]
|
|
public string Text
|
|
{
|
|
get { return _text; }
|
|
set { _text = value; IsDirty = true; }
|
|
}
|
|
private string _procedureNumber;
|
|
[XmlAttribute("ProcedureNumber")]
|
|
public string ProcedureNumber
|
|
{
|
|
get { return _procedureNumber; }
|
|
set { _procedureNumber = value; IsDirty = true; }
|
|
}
|
|
private string _setID;
|
|
[XmlAttribute("SetID")]
|
|
public string SetID
|
|
{
|
|
get { return _setID; }
|
|
set { _setID = value; IsDirty = true; }
|
|
}
|
|
private string _setName;
|
|
[XmlAttribute("SetName")]
|
|
public string SetName
|
|
{
|
|
get { return _setName; }
|
|
set { _setName = value; IsDirty = true; }
|
|
}
|
|
private string _otherID;
|
|
[XmlAttribute("OtherID")]
|
|
public string OtherID
|
|
{
|
|
get { return _otherID; }
|
|
set { _otherID = value; IsDirty = true; }
|
|
}
|
|
private string _otherName;
|
|
[XmlAttribute("OtherName")]
|
|
public string OtherName
|
|
{
|
|
get { return _otherName; }
|
|
set { _otherName = value; IsDirty = true; }
|
|
}
|
|
private string _otherNumber;
|
|
[XmlAttribute("OtherNumber")]
|
|
public string OtherNumber
|
|
{
|
|
get { return _otherNumber; }
|
|
set { _otherNumber = value; IsDirty = true; }
|
|
}
|
|
private string _otherText;
|
|
[XmlAttribute("OyherText")]
|
|
public string OtherText
|
|
{
|
|
get { return _otherText; }
|
|
set { _otherText = value; IsDirty = true; }
|
|
}
|
|
public MiniConfig()
|
|
{
|
|
_index = --lastindex;
|
|
_iD = string.Empty;
|
|
_name = string.Empty;
|
|
_number = string.Empty;
|
|
_text = string.Empty;
|
|
_procedureNumber = string.Empty;
|
|
_setID = string.Empty;
|
|
_setName = string.Empty;
|
|
_otherID = string.Empty;
|
|
_otherName = string.Empty;
|
|
_otherNumber = string.Empty;
|
|
_otherText = string.Empty;
|
|
_isDirty = false;
|
|
}
|
|
public MiniConfig(int index,string id,string name,string number,string text,string procedurenumber,string setid,string setname,string otherid,string othername,string othernumber,string othertext)
|
|
{
|
|
_index = index;
|
|
_iD = id;
|
|
_name = name;
|
|
_number = number;
|
|
_text = text;
|
|
_procedureNumber = procedurenumber;
|
|
_setID = setid;
|
|
_setName = setname;
|
|
_otherID = otherid;
|
|
_otherName = othername;
|
|
_otherNumber = othernumber;
|
|
_otherText = othertext;
|
|
_isDirty = false;
|
|
}
|
|
public override string ToString()
|
|
{
|
|
return Name;
|
|
}
|
|
public string MyXml
|
|
{
|
|
get { return GenericSerializer<MiniConfig>.StringSerialize(this); }
|
|
}
|
|
//#region XML Serializer Namespaces
|
|
//private XmlSerializerNamespaces _Namespaces = null;
|
|
//[XmlNamespaceDeclarations]
|
|
//public XmlSerializerNamespaces Namespaces
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_Namespaces == null)
|
|
// {
|
|
// _Namespaces = new XmlSerializerNamespaces();
|
|
// }
|
|
// return _Namespaces;
|
|
// }
|
|
// set { _Namespaces = value; }
|
|
//}
|
|
//#endregion
|
|
}
|
|
} |