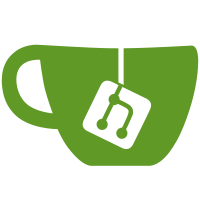
Changed Through Transition Format and User Interface Added Automatic Print Options to shutoff DebugText (/NT), DebugPagination (/NP) and Compare (/NC) Added logic to allow the use of the default path for PDFs. Update the Print_PDFLocation property of the Working Draft when the user changes the value on the Working Draft property page. Fixed Bug B2013-117 Set the date/time stamp for the Document based upon the current time. Make sure that the output is set to Final without revisions and comments before ROs are replaced. Added function to restore valid previous version of MSWord document if the current version is not a valid document. Check for Null to eliminate errors. Removed Unused field DocPDF Support MSWord document restore function. Add check for Transition Node count before updating values. Restore latest valid MSWord document if the current version is not valid. Eliminate File Commands so that the user can only close the MSWord section, and then verify if the changes to the document will be saved.
821 lines
30 KiB
C#
821 lines
30 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.IO;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Print.Library;
|
|
//using Volian.Controls.Library;
|
|
using Volian.Base.Library;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
public partial class DlgPrintProcedure : DevComponents.DotNetBar.Office2007Form
|
|
{
|
|
private bool _Automatic;
|
|
public bool Automatic
|
|
{
|
|
get { return _Automatic; }
|
|
set { _Automatic = value; }
|
|
}
|
|
private void RunAutomatic()
|
|
{
|
|
cbxDebugPagination.Checked = true;
|
|
cbxDebugText.Checked = true;
|
|
Application.DoEvents();
|
|
string[] parameters = System.Environment.CommandLine.Split(" ".ToCharArray());
|
|
bool ranAuto = false;
|
|
foreach (string parameter in parameters)
|
|
{
|
|
if (parameter.ToUpper() == "/NT")
|
|
cbxDebugText.Checked = false;
|
|
else if (parameter.ToUpper() == "/NP")
|
|
cbxDebugPagination.Checked = false;
|
|
else if (parameter.ToUpper() == "/NC")
|
|
cbxDebug.Checked = false;
|
|
}
|
|
CreatePDFs();
|
|
this.Close();
|
|
}
|
|
private DocVersionInfo _DocVersionInfo = null;
|
|
private bool _AllProcedures;
|
|
private DocVersionConfig _DocVersionConfig;
|
|
public string RevNum
|
|
{
|
|
get { return txbRevNum.Text; }
|
|
set { txbRevNum.Text = value; }
|
|
}
|
|
public string RevNumAndDate
|
|
{
|
|
get
|
|
{
|
|
// the RevDate and Rev number are stored in the procedure config separtly as strings
|
|
// we put these two strings to gether to pass into the Print module.
|
|
// the Rev Number followed by a forward slash or backslash logic is from the 16-bit code
|
|
// and is treated as just string.
|
|
// Therefore we need to build a revison number string with a revdate appended to it but only
|
|
// if either the DoRevDate or the RevDateWithForwardSlash is true. otherwise we should use
|
|
// only the RevNumber.
|
|
if (RevDate == "" ||
|
|
(RevNum.Contains("\\") || RevNum.Contains("/")) ||
|
|
(!MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.RevDateWithForwardSlash &&
|
|
!MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.DoRevDate))
|
|
return RevNum;
|
|
if (MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.RevDateWithForwardSlash)
|
|
return RevNum + "\\" + RevDate;
|
|
else
|
|
return RevNum + "/" + RevDate;
|
|
}
|
|
}
|
|
public string ProcNum
|
|
{
|
|
get { return MyProcedure.DisplayNumber; }
|
|
}
|
|
public string PDFPath
|
|
{
|
|
get { return txbPDFLocation.Text; }
|
|
set { txbPDFLocation.Text = value; }
|
|
}
|
|
public string RevDate
|
|
{
|
|
get { return txbRevDate.Text; }
|
|
set { txbRevDate.Text = value; }
|
|
}
|
|
public string ReviewDate
|
|
{
|
|
get { return txbReviewDate.Text; }
|
|
set { txbReviewDate.Text = value; }
|
|
}
|
|
public string PDFPathSetting
|
|
{
|
|
get { return txbPdfLocationS.Text; }
|
|
set { txbPdfLocationS.Text = value; }
|
|
}
|
|
public DlgPrintProcedure(DocVersionInfo dvi, bool automatic)
|
|
{
|
|
_Automatic = automatic;
|
|
InitializeComponent();
|
|
_AllProcedures = true;
|
|
_DocVersionConfig = dvi.DocVersionConfig;
|
|
_DocVersionInfo = dvi;
|
|
_MyProcedure = dvi.Procedures[0].MyProcedure;
|
|
btnCreatePDF.Text = "Create PDFs";
|
|
HandleDocVersionSettings();
|
|
PrepForAllOrOne(false);
|
|
// don't open all PDFs if doing All Procedures
|
|
//cbxOpenAfterCreate2.Checked = dvi.DocVersionConfig.Print_AlwaysViewPDFAfterCreate;
|
|
}
|
|
public DlgPrintProcedure(DocVersionInfo dvi)
|
|
{
|
|
_Automatic = false;
|
|
InitializeComponent();
|
|
_AllProcedures = true;
|
|
_DocVersionConfig = dvi.DocVersionConfig;
|
|
_DocVersionInfo = dvi;
|
|
_MyProcedure = dvi.Procedures[0].MyProcedure;
|
|
btnCreatePDF.Text = "Create PDFs";
|
|
HandleDocVersionSettings();
|
|
PrepForAllOrOne(false);
|
|
// don't open all PDFs if doing All Procedures
|
|
//cbxOpenAfterCreate2.Checked = dvi.DocVersionConfig.Print_AlwaysViewPDFAfterCreate;
|
|
}
|
|
private void PrepForAllOrOne(bool oneProcedure)
|
|
{
|
|
txbPDFName.Visible = lblPDFFileName.Visible = oneProcedure;
|
|
txbRevNum.Visible = lblRevNum.Visible = oneProcedure;
|
|
txbReviewDate.Visible = lblReviewDate.Visible = oneProcedure;
|
|
txbRevDate.Visible = lblRevDate.Visible = oneProcedure;
|
|
cbxOpenAfterCreate2.Visible = oneProcedure;
|
|
cbxOpenAfterCreate2.Checked = false;
|
|
cbxOverwritePDF2.Visible = oneProcedure;
|
|
cbxOverwritePDF2.Checked = true;
|
|
gpnlDebug.Visible = Volian.Base.Library.VlnSettings.DebugMode;
|
|
}
|
|
private string _UnitNumber;
|
|
public string UnitNumber
|
|
{
|
|
get { return _UnitNumber; }
|
|
set { _UnitNumber = value; }
|
|
}
|
|
private int _UnitIndex;
|
|
public int UnitIndex
|
|
{
|
|
get { return _UnitIndex; }
|
|
set { _UnitIndex = value; }
|
|
}
|
|
public DlgPrintProcedure(ProcedureInfo pi)
|
|
{
|
|
InitializeComponent();
|
|
_AllProcedures = false;
|
|
_DocVersionConfig = pi.MyDocVersion.DocVersionConfig;
|
|
_MyProcedure = pi;
|
|
btnCreatePDF.Text = "Create PDF";
|
|
HandleDocVersionSettings();
|
|
PrepForAllOrOne(true);
|
|
cbxOpenAfterCreate2.Checked = pi.MyDocVersion.DocVersionConfig.Print_AlwaysViewPDFAfterCreate;
|
|
}
|
|
/// <summary>
|
|
/// RHM 20120925 Added so that the dialog would center over the PROMS window
|
|
/// </summary>
|
|
/// <param name="e"></param>
|
|
protected override void OnActivated(EventArgs e)
|
|
{
|
|
base.OnActivated(e);
|
|
if (Owner != null)
|
|
Location = new Point(Owner.Left + Owner.Width / 2 - Width / 2, Owner.Top + Owner.Height/2 - Height/2);
|
|
}
|
|
private void HandleDocVersionSettings()
|
|
{
|
|
|
|
GetDocVersionSettings();
|
|
|
|
// set to a default PDF location if none was specified in the DocVersions property
|
|
if (txbPDFLocation.Text == null || txbPDFLocation.Text.Length == 0 || !Directory.Exists(txbPDFLocation.Text))
|
|
txbPDFLocation.Text = VlnSettings.TemporaryFolder;
|
|
SetCompareVisibility();
|
|
// if the default setting is 'SelectBeforePrinting', put up a message box to determine whether the user
|
|
// wants change bars. If not, 'Change Bar' on the Setting tab is 'OFF' and no Change bar tab.
|
|
// If yes, the Change bar tab is the selected tab.
|
|
if (_DocVersionConfig.Print_ChangeBar == PrintChangeBar.SelectBeforePrinting)
|
|
{
|
|
DialogResult dr = DialogResult.Yes;
|
|
if(!Automatic)
|
|
dr = MessageBox.Show("Do you want change bars?", "Change Bars", MessageBoxButtons.YesNo);
|
|
if (dr == DialogResult.Yes)
|
|
{
|
|
btnChgBarOn.PerformClick();
|
|
if(!Automatic) tabControl1.SelectedTab = tbChangeBars;
|
|
rbFormatDefault.PerformClick();
|
|
rbFormatDefault.Checked = true; // default to 'Format Default'
|
|
}
|
|
else
|
|
{
|
|
btnChgBarOff.PerformClick();
|
|
}
|
|
}
|
|
}
|
|
|
|
private void GetDocVersionSettings()
|
|
{
|
|
_DocVersionConfig.SaveChangesToDocVersionConfig = false;
|
|
_DocVersionConfig.ParentLookup = false;
|
|
// PDF Location
|
|
PDFPath = _DocVersionConfig.Print_PDFLocation;
|
|
// Overwrite PDF
|
|
cbxOverwritePDF2.Checked = _DocVersionConfig.Print_AlwaysOverwritePDF;
|
|
// Open PDF After Create
|
|
cbxOpenAfterCreate2.Checked = _DocVersionConfig.Print_AlwaysViewPDFAfterCreate;
|
|
// Changebars on/off
|
|
if (_DocVersionConfig.Print_ChangeBar == PrintChangeBar.Without)
|
|
btnChgBarOff.PerformClick();
|
|
else
|
|
btnChgBarOn.PerformClick();
|
|
// Watermark on/off
|
|
if (_DocVersionConfig.Print_Watermark == PrintWatermark.None)
|
|
btnWaterMarkOff.PerformClick();
|
|
else
|
|
btnWaterMarkOn.PerformClick();
|
|
// Auto Duplexing on/off
|
|
|
|
if ((_MyProcedure.ActiveParent as DocVersionInfo).ActiveFormat.PlantFormat.FormatData.PrintData.AllowDuplex)
|
|
{
|
|
lblAutoDuplexing.Visible = true;
|
|
btnDuplxOff.Visible = true;
|
|
btnDuplxOn.Visible = true;
|
|
if (_DocVersionConfig.Print_DisableDuplex)
|
|
btnDuplxOff.PerformClick();
|
|
else
|
|
btnDuplxOn.PerformClick();
|
|
}
|
|
else
|
|
{
|
|
lblAutoDuplexing.Visible = false;
|
|
btnDuplxOff.Visible = false;
|
|
btnDuplxOn.Visible = false;
|
|
}
|
|
SetCompareVisibility();
|
|
|
|
// default to using OriginalPageBreaks (16bit page breaks) if App.config is set
|
|
// to true:
|
|
cbxOrPgBrk.Visible = VlnSettings.OriginalPageBreak;
|
|
cbxOrPgBrk.Checked = false;
|
|
}
|
|
|
|
private void SetCompareVisibility()
|
|
{
|
|
// default to print Debug info if App.config is set to debug mode
|
|
// This checkbox is now labeled as "Compare PDF"
|
|
// If the PDF location has a Compare folder or we are in Debug Mode
|
|
// then make the checkbox visible
|
|
string cmpfldr = txbPDFLocation.Text;
|
|
if (!cmpfldr.EndsWith("\\")) cmpfldr += "\\";
|
|
cmpfldr += "Compare";
|
|
cbxDebug.Visible = Directory.Exists(cmpfldr) || VlnSettings.DebugMode;
|
|
cbxDebug.Checked = VlnSettings.DebugMode;
|
|
}
|
|
|
|
private void DlgPrintProcedure_Load(object sender, EventArgs e)
|
|
{
|
|
SetupForProcedure();
|
|
_MyTimer = new Timer();
|
|
_MyTimer.Tick += new EventHandler(_MyTimer_Tick);
|
|
_MyTimer.Interval = 100;
|
|
_MyTimer.Enabled = true;
|
|
btnCreatePDF.Select();
|
|
}
|
|
|
|
void _MyTimer_Tick(object sender, EventArgs e)
|
|
{
|
|
_MyTimer.Enabled = false;
|
|
BringToFront();
|
|
if (Automatic) RunAutomatic();
|
|
}
|
|
|
|
private Timer _MyTimer;
|
|
private void SetupForProcedure()
|
|
{
|
|
if(_DocVersionInfo == null)this.Text = "Create PDF for " + ProcNum;
|
|
// get list of previous pdf files
|
|
// if no previous pdf file, then get path from frmVersionProperties
|
|
// dlgSelectFile.InitialDirectory = pdf path from frmVersionProperties
|
|
//cbxPDF.Text = string.Format(@"{0}\{1}.pdf", _PDFPath, _ProcNum);
|
|
// General 2 settings
|
|
//txbPDFLocation.Text = _PDFPath;
|
|
if (ProcNum == string.Empty)
|
|
txbPDFName.Text = this.UnitNumber + ".pdf";
|
|
else
|
|
txbPDFName.Text = string.Format("{0}.pdf", _MyProcedure.PDFNumber);
|
|
if (txbPDFName.Text.StartsWith("*"))
|
|
txbPDFName.Text = txbPDFName.Text.Replace("*", this.UnitNumber);
|
|
ProcedureConfig pc = _MyProcedure.MyConfig as ProcedureConfig;
|
|
if(SelectedSlave > 0) pc.SelectedSlave = SelectedSlave;
|
|
if (pc != null)
|
|
{
|
|
RevNum = pc.Print_Rev;
|
|
RevDate = pc.Print_RevDate; //== null || pc.Print_RevDate=="" ? DateTime.Today : Convert.ToDateTime(pc.Print_RevDate);
|
|
ReviewDate = pc.Print_ReviewDate; // == null ? DateTime.Today : Convert.ToDateTime(pc.Print_ReviewDate);
|
|
//Now check the format flags to determine if/how the Rev string should be parsed.
|
|
// This will covert the old way (16-bit) of setting a RevDate (appending it to the RevNumber)
|
|
// to the new way saving the RevNumber and RevDate in there own config fields
|
|
if ((_MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.DoRevDate && RevNum.Contains("/"))
|
|
|| (_MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.RevDateWithForwardSlash && RevNum.Contains("\\")))
|
|
{
|
|
int indx = RevNum.IndexOf(_MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.RevDateWithForwardSlash ? '\\' : '/');
|
|
pc.Print_RevDate = RevDate = RevNum.Substring(indx + 1);
|
|
pc.Print_Rev = RevNum = RevNum.Substring(0, indx);
|
|
// save the RevNumber and RevDate to the procedure's config.
|
|
using (Item itm = Item.Get(MyProcedure.ItemID))
|
|
{
|
|
itm.MyContent.Config = MyProcedure.MyConfig.ToString();
|
|
itm.Save();
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
RevNum = "0";
|
|
RevDate = DateTime.Today.ToShortDateString();
|
|
ReviewDate = null;
|
|
}
|
|
cbxWaterMark.DataSource = EnumDetail<PrintWatermark>.Details();
|
|
cbxWaterMark.DisplayMember = "Description";
|
|
cbxWaterMark.ValueMember = "EValue";
|
|
PrintWatermark pw = _DocVersionConfig.Print_Watermark; // MyProcedure.ProcedureConfig.Print_Watermark;
|
|
cbxWaterMark.Text = pw.ToString();
|
|
|
|
//ppCmbxChgBarPos.DataSource = EnumDetail<PrintChangeBarLoc>.Details();
|
|
//ppCmbxChgBarPos.DisplayMember = "Description";
|
|
//ppCmbxChgBarPos.ValueMember = "EValue";
|
|
//ppCmbxChgBarPos.SelectedIndex = (int)MyProcedure.MyDocVersion.DocVersionConfig.Print_ChangeBarLoc;
|
|
|
|
ppCmbxChgBarTxtType.DataSource = EnumDetail<PrintChangeBarText>.Details();
|
|
ppCmbxChgBarTxtType.DisplayMember = "Description";
|
|
ppCmbxChgBarTxtType.ValueMember = "EValue";
|
|
ppCmbxChgBarTxtType.SelectedIndex = (int)_DocVersionConfig.Print_ChangeBarText;
|
|
|
|
ppTxbxChangeBarUserMsgOne.Text = _DocVersionConfig.Print_UserCBMess1;
|
|
ppTxbxChangeBarUserMsgTwo.Text = _DocVersionConfig.Print_UserCBMess2;
|
|
ppGpbxUserSpecTxt.Enabled = ppCmbxChgBarTxtType.SelectedIndex == (int)PrintChangeBarText.UserDef;
|
|
bool hasReviewDate = _MyProcedure.ActiveFormat.PlantFormat.HasPageListToken("{REVIEWDATE}");
|
|
bool usesRevDate = _MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.DoRevDate || _MyProcedure.ActiveFormat.PlantFormat.FormatData.PrintData.RevDateWithForwardSlash;
|
|
if (_MyProcedure.Sections != null)
|
|
{
|
|
foreach (SectionInfo mysection in _MyProcedure.Sections)
|
|
hasReviewDate |= mysection.ActiveFormat.PlantFormat.HasPageListToken("{REVIEWDATE}");
|
|
}
|
|
// Only the New HLP format and the MYA format use this
|
|
// South Texas does not use their new HLP format and MYA (Main Yankee) has dropped us (they closed)
|
|
// thus there is no supporting code to print a Review Date
|
|
// thus setting "hasReviewDate" to false so the Review Date entry box does not appear on the print dialog
|
|
// - jsj 4/3/2013
|
|
hasReviewDate = false; // jsj 4/3/2013
|
|
lblReviewDate.Visible = txbReviewDate.Visible = !_AllProcedures && hasReviewDate;
|
|
lblRevDate.Visible = txbRevDate.Visible = !_AllProcedures && usesRevDate;
|
|
}
|
|
|
|
private void btnCancel_Click(object sender, EventArgs e)
|
|
{
|
|
this.Close();
|
|
}
|
|
|
|
private void btnAvChgBarOn_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnChgBarOn.Checked)
|
|
{
|
|
btnChgBarOn.Checked = true;
|
|
btnChgBarOff.Checked = false;
|
|
tbChangeBars.Visible = true;
|
|
// to set which radio button (Format default or Custom) is checked, look at config data
|
|
switch (_DocVersionConfig.Print_ChangeBar)
|
|
{
|
|
case PrintChangeBar.WithUserSpecified:
|
|
rbCustom.Checked = true;
|
|
break;
|
|
case PrintChangeBar.SelectBeforePrinting:
|
|
case PrintChangeBar.WithDefault:
|
|
default:
|
|
rbFormatDefault.Checked = true;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
private void btnAvChgBarOff_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnChgBarOff.Checked)
|
|
{
|
|
btnChgBarOff.Checked = true;
|
|
btnChgBarOn.Checked = false;
|
|
tbChangeBars.Visible = false;
|
|
}
|
|
}
|
|
|
|
private void btnAdvDuplxOn_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnDuplxOn.Checked)
|
|
{
|
|
btnDuplxOn.Checked = true;
|
|
btnDuplxOff.Checked = false;
|
|
}
|
|
}
|
|
|
|
private void btnAdvDuplxOff_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnDuplxOff.Checked)
|
|
{
|
|
btnDuplxOff.Checked = true; ;
|
|
btnDuplxOn.Checked = false;
|
|
}
|
|
}
|
|
|
|
private void btnAdvWaterMarkOn_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnWaterMarkOn.Checked)
|
|
{
|
|
btnWaterMarkOn.Checked = true;
|
|
btnWaterMarkOff.Checked = false;
|
|
cbxWaterMark.Visible = true;
|
|
}
|
|
}
|
|
|
|
private void btnAdvWaterMarkOff_Click(object sender, EventArgs e)
|
|
{
|
|
if (!btnWaterMarkOff.Checked)
|
|
{
|
|
btnWaterMarkOff.Checked = true;
|
|
btnWaterMarkOn.Checked = false;
|
|
cbxWaterMark.Visible = false;
|
|
}
|
|
}
|
|
|
|
private void txbPDFLocation_Leave(object sender, EventArgs e)
|
|
{
|
|
if (!Directory.Exists(PDFPath))
|
|
{
|
|
string msg = string.Format("'{0}' does not exist. \n\nCreate it?", PDFPath);
|
|
DialogResult dr= MessageBox.Show(msg, "Folder Not Found", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
|
|
if (dr == DialogResult.Yes)
|
|
{
|
|
try
|
|
{
|
|
Directory.CreateDirectory(PDFPath);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
MessageBox.Show(ex.Message, "Error trying to create folder", MessageBoxButtons.OK, MessageBoxIcon.Error);
|
|
PDFPath = _DocVersionConfig.Print_PDFLocation;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
PDFPath = _DocVersionConfig.Print_PDFLocation;
|
|
}
|
|
}
|
|
}
|
|
|
|
private void DlgPrintProcedure_FormClosed(object sender, FormClosedEventArgs e)
|
|
{
|
|
_DocVersionConfig.SaveChangesToDocVersionConfig = true;
|
|
//_DocVersionConfig.ParentLookup = true;
|
|
|
|
ProcedureConfig pc = _MyProcedure.MyConfig as ProcedureConfig;
|
|
if (SelectedSlave > 0) pc.SelectedSlave = 0;
|
|
}
|
|
|
|
private ProcedureInfo _MyProcedure;
|
|
|
|
public ProcedureInfo MyProcedure
|
|
{
|
|
get { return _MyProcedure; }
|
|
set { _MyProcedure = value; }
|
|
}
|
|
private int _SelectedSlave = 0;
|
|
public int SelectedSlave
|
|
{
|
|
get { return _SelectedSlave; }
|
|
set
|
|
{
|
|
_SelectedSlave = value;
|
|
lblMultiunitPdfLocation.Visible = cbxMultiunitPdfLocation.Visible = (value > -1);
|
|
cbxMultiunitPdfLocation.SelectedIndex = 1;
|
|
}
|
|
}
|
|
private string _MultiunitPdfLocation = string.Empty;
|
|
private void CreatePDFs()
|
|
{
|
|
CreateDebugFiles();
|
|
// If file exists, determine if overwrite checkbox allows overwrite, if not prompt.
|
|
|
|
Volian.Print.Library.Rtf2Pdf.PdfDebug = true;
|
|
PrintWatermark pw = (btnWaterMarkOn.Checked) ? (PrintWatermark)cbxWaterMark.SelectedValue : PrintWatermark.None;
|
|
// Determine change bar settings. First get from config & then see if override from dialog.
|
|
// Also check that format allows override.
|
|
ChangeBarDefinition cbd = DetermineChangeBarSettings();
|
|
int n = _DocVersionInfo.Procedures.Count;
|
|
int i = 0;
|
|
pbPDFsStatus.Maximum = n;
|
|
pbPDFsStatus.Visible = true;
|
|
this.Text = string.Format("Processing {0}", _DocVersionInfo.MyFolder.Name);
|
|
foreach (ProcedureInfo myProc in _DocVersionInfo.Procedures)
|
|
{
|
|
MyProcedure = myProc;
|
|
if (myProc.Sections != null)
|
|
{
|
|
if (SelectedSlave > 0)
|
|
{
|
|
MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave = SelectedSlave;
|
|
SetupForProcedure();
|
|
pbPDFsStatus.TextVisible = true;
|
|
pbPDFsStatus.Text = string.Format("Creating PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
pbPDFsStatus.Value = i;
|
|
// this.Text = string.Format("Create PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
MyProcedure = ProcedureInfo.GetItemAndChildrenByUnit(MyProcedure.ItemID, 0, MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave);
|
|
GetMultiunitPDFPath();
|
|
// RHM 20120925 Overlay the bottom of the dialog so that cancel button is covered.
|
|
frmPDFStatusForm frmStatus = new frmPDFStatusForm(MyProcedure, RevNumAndDate,pw.ToString(), cbxDebug.Checked, cbxOrPgBrk.Checked, cbxOpenAfterCreate2.Checked, cbxOverwritePDF2.Checked, PDFPath, cbd, txbPDFName.Text, new Point(Left,Bottom-50));
|
|
frmStatus.CloseWhenDone = true;
|
|
Application.DoEvents();
|
|
frmStatus.CancelStop = true;
|
|
frmStatus.ShowDialog();
|
|
if (frmStatus.CancelPrinting) break;
|
|
}
|
|
else if (SelectedSlave == 0)
|
|
{
|
|
for (int k = 1; k <= MyProcedure.MyDocVersion.MultiUnitCount; k++)
|
|
{
|
|
MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave = k;
|
|
SetupForProcedure();
|
|
pbPDFsStatus.TextVisible = true;
|
|
pbPDFsStatus.Text = string.Format("Creating PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
pbPDFsStatus.Value = i;
|
|
// this.Text = string.Format("Create PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
MyProcedure = ProcedureInfo.GetItemAndChildrenByUnit(MyProcedure.ItemID, 0, MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave);
|
|
// RHM 20120925 Overlay the bottom of the dialog so that cancel button is covered.
|
|
frmPDFStatusForm frmStatus = new frmPDFStatusForm(MyProcedure, RevNumAndDate, pw.ToString(), cbxDebug.Checked, cbxOrPgBrk.Checked, cbxOpenAfterCreate2.Checked, cbxOverwritePDF2.Checked, PDFPath, cbd, txbPDFName.Text, new Point(Left, Bottom - 50));
|
|
frmStatus.CloseWhenDone = true;
|
|
Application.DoEvents();
|
|
frmStatus.CancelStop = true;
|
|
frmStatus.ShowDialog();
|
|
if (frmStatus.CancelPrinting) break;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
SetupForProcedure();
|
|
pbPDFsStatus.TextVisible = true;
|
|
pbPDFsStatus.Text = string.Format("Creating PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
pbPDFsStatus.Value = i;
|
|
// this.Text = string.Format("Create PDF for {0} ({1} of {2})", myProc.DisplayNumber, ++i, n);
|
|
// RHM 20120925 Overlay the bottom of the dialog so that cancel button is covered.
|
|
MyProcedure = ProcedureInfo.GetItemAndChildren(MyProcedure.ItemID);
|
|
frmPDFStatusForm frmStatus = new frmPDFStatusForm(MyProcedure, RevNumAndDate, pw.ToString(), cbxDebug.Checked, cbxOrPgBrk.Checked, cbxOpenAfterCreate2.Checked, cbxOverwritePDF2.Checked, PDFPath, cbd, txbPDFName.Text, new Point(Left, Bottom - 50));
|
|
frmStatus.CloseWhenDone = true;
|
|
Application.DoEvents();
|
|
frmStatus.CancelStop = true;
|
|
frmStatus.ShowDialog();
|
|
if (frmStatus.CancelPrinting) break;
|
|
}
|
|
}
|
|
}
|
|
pbPDFsStatus.Visible = false;
|
|
if(!Automatic)
|
|
ShowDebugFiles();
|
|
}
|
|
|
|
private string GetMultiunitPDFPath()
|
|
{
|
|
string newPDFPath = string.Empty;
|
|
if (_MultiunitPdfLocation == "None")
|
|
newPDFPath = PDFPath;
|
|
if (_MultiunitPdfLocation == "Unit Name")
|
|
newPDFPath = PDFPath + @"\" + MyProcedure.MyDocVersion.DocVersionConfig.Unit_Name;
|
|
if (_MultiunitPdfLocation == "Unit Number")
|
|
newPDFPath = PDFPath + @"\" + MyProcedure.MyDocVersion.DocVersionConfig.Unit_Number;
|
|
if (_MultiunitPdfLocation == "Unit Text")
|
|
newPDFPath = PDFPath + @"\" + MyProcedure.MyDocVersion.DocVersionConfig.Unit_Text;
|
|
if (_MultiunitPdfLocation == "Unit ID")
|
|
newPDFPath = PDFPath + @"\" + MyProcedure.MyDocVersion.DocVersionConfig.Unit_ID;
|
|
if (newPDFPath == "") newPDFPath = Volian.Base.Library.VlnSettings.TemporaryFolder;
|
|
if (!Directory.Exists(newPDFPath))
|
|
Directory.CreateDirectory(newPDFPath);
|
|
return newPDFPath;
|
|
}
|
|
private void CreateDebugFiles()
|
|
{
|
|
if (cbxDebugPagination.Checked)
|
|
Volian.Base.Library.DebugPagination.Open(PDFPath + "\\DebugPagination.txt"); // RHM 20120925 Corrected spelling
|
|
if (cbxDebugText.Checked)
|
|
Volian.Base.Library.DebugText.Open(PDFPath + "\\DebugText.txt");
|
|
}
|
|
private void ShowDebugFiles()
|
|
{
|
|
if (cbxDebugPagination.Checked)
|
|
Volian.Base.Library.DebugPagination.Show();
|
|
if (cbxDebugText.Checked)
|
|
Volian.Base.Library.DebugText.Show();
|
|
}
|
|
private void CreatePDF()
|
|
{
|
|
CreateDebugFiles();
|
|
// If file exists, determine if overwrite checkbox allows overwrite, if not prompt.
|
|
|
|
Volian.Print.Library.Rtf2Pdf.PdfDebug = true;
|
|
PrintWatermark pw = (btnWaterMarkOn.Checked) ? (PrintWatermark)cbxWaterMark.SelectedValue : PrintWatermark.None;
|
|
// Determine change bar settings. First get from config & then see if override from dialog.
|
|
// Also check that format allows override.
|
|
ChangeBarDefinition cbd = DetermineChangeBarSettings();
|
|
if (MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave > 0)
|
|
MyProcedure = ProcedureInfo.GetItemAndChildrenByUnit(MyProcedure.ItemID, 0, MyProcedure.MyDocVersion.DocVersionConfig.SelectedSlave);
|
|
else
|
|
MyProcedure = ProcedureInfo.GetItemAndChildren(MyProcedure.ItemID);
|
|
// RHM 20120925 Overlay the bottom of the dialog so that cancel button is covered.
|
|
frmPDFStatusForm frmStatus = new frmPDFStatusForm(MyProcedure, RevNumAndDate, pw.ToString(), cbxDebug.Checked, cbxOrPgBrk.Checked, cbxOpenAfterCreate2.Checked, cbxOverwritePDF2.Checked, PDFPath, cbd, txbPDFName.Text, new Point(Left, Bottom - 50));
|
|
frmStatus.ShowDialog();
|
|
this.Close();
|
|
ShowDebugFiles();
|
|
}
|
|
|
|
// Determine if any dialog selections for change bars have overridden the data
|
|
// supplied in the DocVconfig data or format file. Settings are location and text.
|
|
private ChangeBarDefinition DetermineChangeBarSettings()
|
|
{
|
|
ChangeBarDefinition cbd = new ChangeBarDefinition();
|
|
|
|
// if the dialog has 'without change bar' selected, then just set without and return
|
|
if (btnChgBarOff.Checked)
|
|
{
|
|
cbd.MyChangeBarType = PrintChangeBar.Without;
|
|
return cbd;
|
|
}
|
|
// Get settings from dialog because these are used to set the initial dialog values and
|
|
// any changes from user overrides the initial settings.
|
|
ChangeBarData changeBarData = MyProcedure.ActiveFormat.PlantFormat.FormatData.ProcData.ChangeBarData;
|
|
if (rbFormatDefault.Checked || _DocVersionConfig == null || _DocVersionConfig.Print_ChangeBar == PrintChangeBar.WithDefault)
|
|
{
|
|
cbd.MyChangeBarType = PrintChangeBar.WithDefault;
|
|
cbd.MyChangeBarText = changeBarData.ChangeBarMessage == "ChgID" ? PrintChangeBarText.ChgID :
|
|
changeBarData.ChangeBarMessage == "DateAndChgID" ? PrintChangeBarText.DateChgID :
|
|
changeBarData.ChangeBarMessage == "None" ? PrintChangeBarText.None :
|
|
changeBarData.ChangeBarMessage == "RevNum" ? PrintChangeBarText.RevNum : PrintChangeBarText.UserDef;
|
|
}
|
|
else if (rbCustom.Checked || _DocVersionConfig.Print_ChangeBar == PrintChangeBar.WithUserSpecified)
|
|
{
|
|
cbd.MyChangeBarLoc = _DocVersionConfig.Print_ChangeBarLoc; // (PrintChangeBarLoc)ppCmbxChgBarPos.SelectedIndex;
|
|
cbd.MyChangeBarText = (PrintChangeBarText)ppCmbxChgBarTxtType.SelectedIndex;
|
|
}
|
|
else
|
|
{
|
|
cbd.MyChangeBarLoc = _DocVersionConfig.Print_ChangeBarLoc;
|
|
cbd.MyChangeBarText = _DocVersionConfig.Print_ChangeBarText;
|
|
}
|
|
|
|
// now figure out the location.
|
|
if (cbd.MyChangeBarType != PrintChangeBar.Without)
|
|
{
|
|
// if the format has the absolutefixedchangecolumn format flag, then always use the fixedchangecolumn from the
|
|
// format, otherwise, use the default column based on the selected location, stored in the base format.
|
|
cbd.MyChangeBarColumn = (changeBarData.AbsoluteFixedChangeColumn) ?
|
|
(int)changeBarData.FixedChangeColumn :
|
|
System.Convert.ToInt32(changeBarData.DefaultCBLoc.Split(" ,".ToCharArray())[System.Convert.ToInt32(cbd.MyChangeBarLoc)]);
|
|
//cbd.MyChangeBarColumn = (int)changeBarData.FixedChangeColumn;
|
|
if (cbd.MyChangeBarText == PrintChangeBarText.UserDef)
|
|
cbd.MyChangeBarMessage = _DocVersionConfig.Print_UserCBMess1 + @"\n" + _DocVersionConfig.Print_UserCBMess2;
|
|
|
|
}
|
|
return cbd;
|
|
}
|
|
private void btnCreatePDF_Click(object sender, EventArgs e)
|
|
{
|
|
Rtf2iTextSharp.DoingComparison = cbxDebug.Checked;
|
|
if (_AllProcedures)
|
|
{
|
|
DateTime dtStart = DateTime.Now;
|
|
_MultiunitPdfLocation = cbxMultiunitPdfLocation.SelectedItem.ToString();
|
|
CreatePDFs();
|
|
if (VlnSettings.DebugMode)
|
|
{
|
|
MessageBox.Show(string.Format("{0} Minutes to Print All Procedures"
|
|
, TimeSpan.FromTicks(DateTime.Now.Ticks - dtStart.Ticks).TotalMinutes),
|
|
"Printing Duration", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
this.Close();
|
|
}
|
|
else
|
|
CreatePDF();
|
|
}
|
|
private bool _Initializing = false;
|
|
private void tbSettings_Click(object sender, EventArgs e)
|
|
{
|
|
// Fill in the settings from the config. This tab is used
|
|
// to update the settings for PdfLocation, RevNum, RevDate and ReviewDate
|
|
_Initializing = true;
|
|
txbPdfLocationS.Text = txbPDFLocation.Text;
|
|
_Initializing = false;
|
|
}
|
|
|
|
|
|
private void btnPdfLocation_Click(object sender, EventArgs e)
|
|
{
|
|
DlgBrowseFolder.SelectedPath = PDFPathSetting;
|
|
DialogResult drslt = DlgBrowseFolder.ShowDialog();
|
|
if (drslt == DialogResult.OK)
|
|
{
|
|
//cbxPDFloc.Text = DlgBrowseFolder.SelectedPath;
|
|
txbPdfLocationS.Text = DlgBrowseFolder.SelectedPath;
|
|
txbPDFLocation.Text = txbPdfLocationS.Text;
|
|
//lblCurPDFLoc.Text = cbxPDFloc.Text;
|
|
//UpdateDropDown2();
|
|
}
|
|
}
|
|
|
|
private void rbFormatDefault_Click(object sender, EventArgs e)
|
|
{
|
|
SetCustomControls(false);
|
|
// Make Custom controls invisible
|
|
ppGpbxUserSpecCB.Visible = false;
|
|
}
|
|
|
|
private void SetCustomControls(bool enabled)
|
|
{
|
|
ppCmbxChgBarPos.Enabled = enabled;
|
|
ppCmbxChgBarTxtType.Enabled = enabled;
|
|
ppTxbxChangeBarUserMsgOne.Enabled = enabled;
|
|
ppTxbxChangeBarUserMsgTwo.Enabled = enabled;
|
|
}
|
|
|
|
private void rbCustom_Click(object sender, EventArgs e)
|
|
{
|
|
SetCustomControls(true);
|
|
// Make Custom controls visible
|
|
ppGpbxUserSpecCB.Visible = true;
|
|
}
|
|
|
|
private void rbFormatDefault_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
SetCustomControls(false);
|
|
// Make Custom controls invisible
|
|
ppGpbxUserSpecCB.Visible = false;
|
|
}
|
|
|
|
private void ppCmbxChgBarTxtType_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
ppGpbxUserSpecTxt.Enabled = ppCmbxChgBarTxtType.SelectedIndex == (int)PrintChangeBarText.UserDef;
|
|
}
|
|
private void txbRevDate_Enter(object sender, EventArgs e)
|
|
{
|
|
txbDate = txbRevDate;
|
|
grpDateSelector.Text = "Select Revision Date";
|
|
grpDateSelector.Visible = calDateSelector.Visible = true;
|
|
|
|
}
|
|
|
|
private void txbRevDate_Leave(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
txbDate = null;
|
|
grpDateSelector.Visible = calDateSelector.Visible = false;
|
|
// save the RevDate to the procedure's config.
|
|
ProcedureConfig pc = MyProcedure.MyConfig as ProcedureConfig;
|
|
if (pc == null) return;
|
|
pc.Print_RevDate = txbRevDate.Text;
|
|
using (Item itm = Item.Get(MyProcedure.ItemID))
|
|
{
|
|
itm.MyContent.Config = MyProcedure.MyConfig.ToString();
|
|
itm.Save();
|
|
}
|
|
|
|
}
|
|
|
|
private void txbReviewDate_Leave(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
txbDate = null;
|
|
grpDateSelector.Visible = calDateSelector.Visible = false;
|
|
// save the ReviewDate to the procedure's config.
|
|
ProcedureConfig pc = MyProcedure.MyConfig as ProcedureConfig;
|
|
if (pc == null) return;
|
|
pc.Print_ReviewDate = txbReviewDate.Text;
|
|
using (Item itm = Item.Get(MyProcedure.ItemID))
|
|
{
|
|
itm.MyContent.Config = MyProcedure.MyConfig.ToString();
|
|
itm.Save();
|
|
// need to reset config to !dirty?
|
|
}
|
|
|
|
}
|
|
private void txbReviewDate_Enter(object sender, EventArgs e)
|
|
{
|
|
txbDate = txbReviewDate;
|
|
grpDateSelector.Text = "Select Review Date";
|
|
grpDateSelector.Visible = calDateSelector.Visible = true;
|
|
}
|
|
|
|
private TextBox txbDate = null;
|
|
private void calDateSelector_DateSelected(object sender, DateRangeEventArgs e)
|
|
{
|
|
if (txbDate != null)
|
|
txbDate.Text = e.Start.ToShortDateString();
|
|
}
|
|
|
|
private void txbRevNum_Leave(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
// save the RevNum to the procedure's config.
|
|
ProcedureConfig pc = MyProcedure.MyConfig as ProcedureConfig;
|
|
if (pc == null) return;
|
|
pc.Print_Rev = txbRevNum.Text;
|
|
using (Item itm = Item.Get(MyProcedure.ItemID))
|
|
{
|
|
itm.MyContent.Config = MyProcedure.MyConfig.ToString();
|
|
itm.Save();
|
|
}
|
|
}
|
|
//private void cbxDebug_CheckedChanged(object sender, EventArgs e)
|
|
//{
|
|
// cbxCmpPRMSpfd.Visible = cbxDebug.Checked;
|
|
//}
|
|
}
|
|
} |